Last active
June 2, 2020 07:29
-
-
Save mkrishnan-codes/0b37049c8fb7b8709cbe0812a56031f2 to your computer and use it in GitHub Desktop.
Example for generic class in typescript
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
class Person { | |
name: string | |
age: number | |
constructor(name: string, age: number) { | |
this.name = name; | |
this.age = age; | |
} | |
} | |
class Fruit { | |
ripe: boolean; | |
expiry: string; | |
constructor(ripe: boolean, expiry: string) { | |
this.ripe = ripe; | |
this.expiry = expiry; | |
} | |
} | |
class Vehicle { | |
private wheels: number; | |
private engine: 'Petrol' | 'Diesel'; | |
private driver: Person; | |
getWheels() { | |
return this.wheels; | |
} | |
setWheels(wheels: number) { | |
this.wheels = wheels; | |
} | |
getEngine() { | |
return this.engine; | |
} | |
setEngine(engine: 'Petrol' | 'Diesel') { | |
this.engine = engine; | |
} | |
getDriver() { | |
return this.driver; | |
} | |
setDriver(driver: Person) { | |
this.driver = driver; | |
} | |
} | |
class Truck<T> extends Vehicle { | |
private load: T; | |
getLoad() { | |
return this.load | |
} | |
setLoad(load: T) { | |
this.load = load; | |
} | |
hasCarriage() { | |
return true; | |
} | |
} | |
class TruckCarrier extends Truck<TruckCarrier>{ | |
hasRefregiration() { | |
return false; | |
} | |
// ... other methods | |
} | |
class FruitsCarrier extends Truck<Fruit>{ | |
hasRefregiration() { | |
return true; | |
} | |
// ... other methods | |
} | |
let fruitsCarriage: FruitsCarrier = new FruitsCarrier(); | |
console.log('Fruit loaded') | |
fruitsCarriage.setLoad(new Fruit(true, 'July')) | |
// Async Transport ....... | |
console.log('Fruit Transported') | |
// Checking cargo by fruit vendor (reciever) | |
if (fruitsCarriage.getLoad().ripe) { | |
// since fruit carriage load type was fruits, the property of fruit ripe was accessible here | |
console.log('Fruits are ripen') | |
// do stuff if ripe | |
} | |
let truckCarriage: TruckCarrier = new TruckCarrier(); | |
console.log('Truck loaded with another truck') | |
truckCarriage.setLoad(new TruckCarrier()) | |
// Async Transport ....... | |
console.log('Truck Transported') | |
// checking cargo by Truck vendor | |
if (truckCarriage.getLoad().hasCarriage()) { | |
// since truck carriage load type was truck, | |
//the method of truck hasCarriage was accessible here | |
//this is not possible if the function get/setLoad | |
// was written with "any" as argument type | |
// do stuff if he found a carriable truck | |
console.log('Truck has another truck on it') | |
} | |
if (!truckCarriage.getLoad().hasRefregiration()) { | |
// do stuff if he found a carriable truck | |
console.log('No refregiration for the truck inside') | |
} |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Imagination
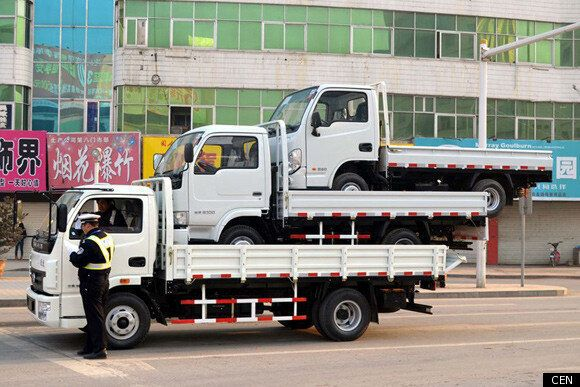
class TruckCarrier extends Truck<TruckCarrier>
FruitsCarrier extends Truck<Fruit>
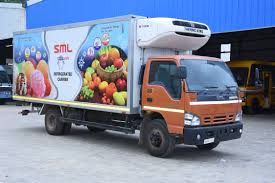