Last active
May 26, 2021 15:29
-
-
Save mmazzarolo/3ed3883d5c838c7010c353c6f3ac2be8 to your computer and use it in GitHub Desktop.
Simple animated stateless React-Native radio button
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Preview:
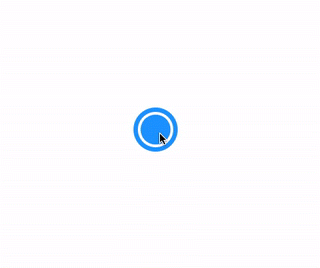