-
-
Save mmazzarolo/d4f2c9855ec723ec228f8958e43b13f6 to your computer and use it in GitHub Desktop.
Can be implemented easily in NavigationExperimental renderOverlay
:
_renderOverlay = (navigatorProps) => {
const currentRoute = navigatorProps.navigationState.children[navigatorProps.navigationState.index]
const { key, title } = currentRoute
const showDrawer = appRoutes[key].showInDrawer // Your logic here: should the route show the drawer or the back button? (I put all my routes and info in a json called appRoutes for example)
const onLeftPress = () => {
if (showDrawer) {
this.navigationActions.openDrawer() // Your action for handling the drawer button press
} else {
this.navigationActions.navigatePop() // Your action for handling the back button press
}
}
return (
<NavBar
title={title}
onLeftPress={onLeftPress}
showDrawer={showDrawer}
{...navigatorProps} // !!!important!!! navigator props used in the iOS Toolbar component
/>
)
}
@mmazzarolo mind throwing this into an example react-native app?
just a correction, looks like renderOverlay has been removed as a property, the new property to use should be renderHeader. Refer this
In the last days I've read all Reactive Native documentations.
Now I'm totally confused. Am I the only one?
Could someone please provide an integration demo from a root index.js?
By the way, I tried this with react-native-router-flux custom navbar option.
'Dimensions' does not work in my environment. What do I have to have present to get Dimensions?
Please help, I'm a looser, but some day I will do something like this...
https://www.youtube.com/watch?v=i__Hrjg3bEQ
I'm super mad because of Internet confusing UI Bars and thus creates a lot more misusage of this terminology.
https://developer.apple.com/ios/human-interface-guidelines/ui-bars/toolbars/
Preview:
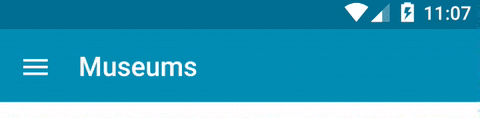
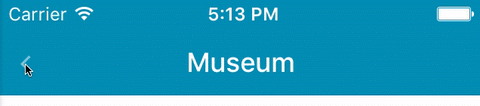