Last active
October 13, 2024 00:49
-
-
Save mmozeiko/729860eeb414f1a2ee345d9d3ab4dd4e to your computer and use it in GitHub Desktop.
drawing pixels with SDL
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
// on Windows compile with: | |
// cl.exe sdl2_pixels.c -Zi -Iinclude -link -incremental:no -subsystem:windows SDL2.lib SDL2main.lib shell32.lib | |
#include <SDL.h> | |
#include <math.h> | |
int main(int argc, char* argv[]) | |
{ | |
int width = 1280; | |
int height = 720; | |
SDL_Init(SDL_INIT_VIDEO); | |
SDL_Window* window = SDL_CreateWindow("SDL pixels", SDL_WINDOWPOS_UNDEFINED, SDL_WINDOWPOS_UNDEFINED, width, height, SDL_WINDOW_SHOWN); | |
SDL_Surface* screen = SDL_GetWindowSurface(window); | |
SDL_Surface* pixels = SDL_CreateRGBSurfaceWithFormat(0, width, height,32, SDL_PIXELFORMAT_RGBX8888); | |
unsigned int t1 = SDL_GetTicks(); | |
float pos = 0; | |
for (;;) | |
{ | |
SDL_Event ev; | |
while (SDL_PollEvent(&ev)) | |
{ | |
if (ev.type == SDL_QUIT) | |
{ | |
return 0; | |
} | |
} | |
unsigned int t2 = SDL_GetTicks(); | |
float delta = (t2 - t1) / 1000.0f; | |
t1 = t2; | |
// clear pixels to black background | |
SDL_FillRect(pixels, NULL, 0); | |
// write the pixels | |
SDL_LockSurface(pixels); | |
{ | |
int pitch = pixels->pitch; | |
// move 100 pixels/second | |
pos += delta * 100.0f; | |
pos = fmodf(pos, width); | |
// draw red diagonal line | |
for (int i=0; i<height; i++) | |
{ | |
int y = i; | |
int x = ((int)pos + i) % width; | |
unsigned int* row = (unsigned int*)((char*)pixels->pixels + pitch * y); | |
row[x] = 0xff0000ff; // 0xAABBGGRR | |
} | |
} | |
SDL_UnlockSurface(pixels); | |
// copy to window | |
SDL_BlitSurface(pixels, NULL, screen, NULL); | |
SDL_UpdateWindowSurface(window); | |
} | |
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
// on Windows compile with: | |
// cl.exe sdl2_pixels_r.c -Zi -Iinclude -link -incremental:no -subsystem:windows SDL2.lib SDL2main.lib shell32.lib | |
#include <SDL.h> | |
#include <math.h> | |
int main(int argc, char* argv[]) | |
{ | |
int width = 1280; | |
int height = 720; | |
SDL_Init(SDL_INIT_VIDEO); | |
SDL_Window* window = SDL_CreateWindow("SDL pixels", SDL_WINDOWPOS_UNDEFINED, SDL_WINDOWPOS_UNDEFINED, width, height, SDL_WINDOW_SHOWN); | |
SDL_Renderer* renderer = SDL_CreateRenderer(window, -1, SDL_RENDERER_ACCELERATED | SDL_RENDERER_PRESENTVSYNC); | |
SDL_Texture* pixels = SDL_CreateTexture(renderer, SDL_PIXELFORMAT_RGBX8888, SDL_TEXTUREACCESS_STREAMING, width, height); | |
unsigned int t1 = SDL_GetTicks(); | |
float pos = 0; | |
for (;;) | |
{ | |
SDL_Event ev; | |
while (SDL_PollEvent(&ev)) | |
{ | |
if (ev.type == SDL_QUIT) | |
{ | |
return 0; | |
} | |
} | |
unsigned int t2 = SDL_GetTicks(); | |
float delta = (t2 - t1) / 1000.0f; | |
t1 = t2; | |
void* data; | |
int pitch; | |
SDL_LockTexture(pixels, NULL, &data, &pitch); | |
{ | |
// clear to black background | |
SDL_memset(data, 0, pitch * height); | |
// move 100 pixels/second | |
pos += delta * 100.0f; | |
pos = fmodf(pos, width); | |
// draw red diagonal line | |
for (int i=0; i<height; i++) | |
{ | |
int y = i; | |
int x = ((int)pos + i) % width; | |
unsigned int* row = (unsigned int*)((char*)data + pitch * y); | |
row[x] = 0xff0000ff; // 0xAABBGGRR | |
} | |
} | |
SDL_UnlockTexture(pixels); | |
// copy to window | |
SDL_RenderCopy(renderer, pixels, NULL, NULL); | |
SDL_RenderPresent(renderer); | |
} | |
} |
Curly braces like that are perfectly valid to use in C code. There's no reason to remove them. They just letting reader of source code to know that these lines of code should "go together" - because texture locking is happening around it.
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Hello I found problem.
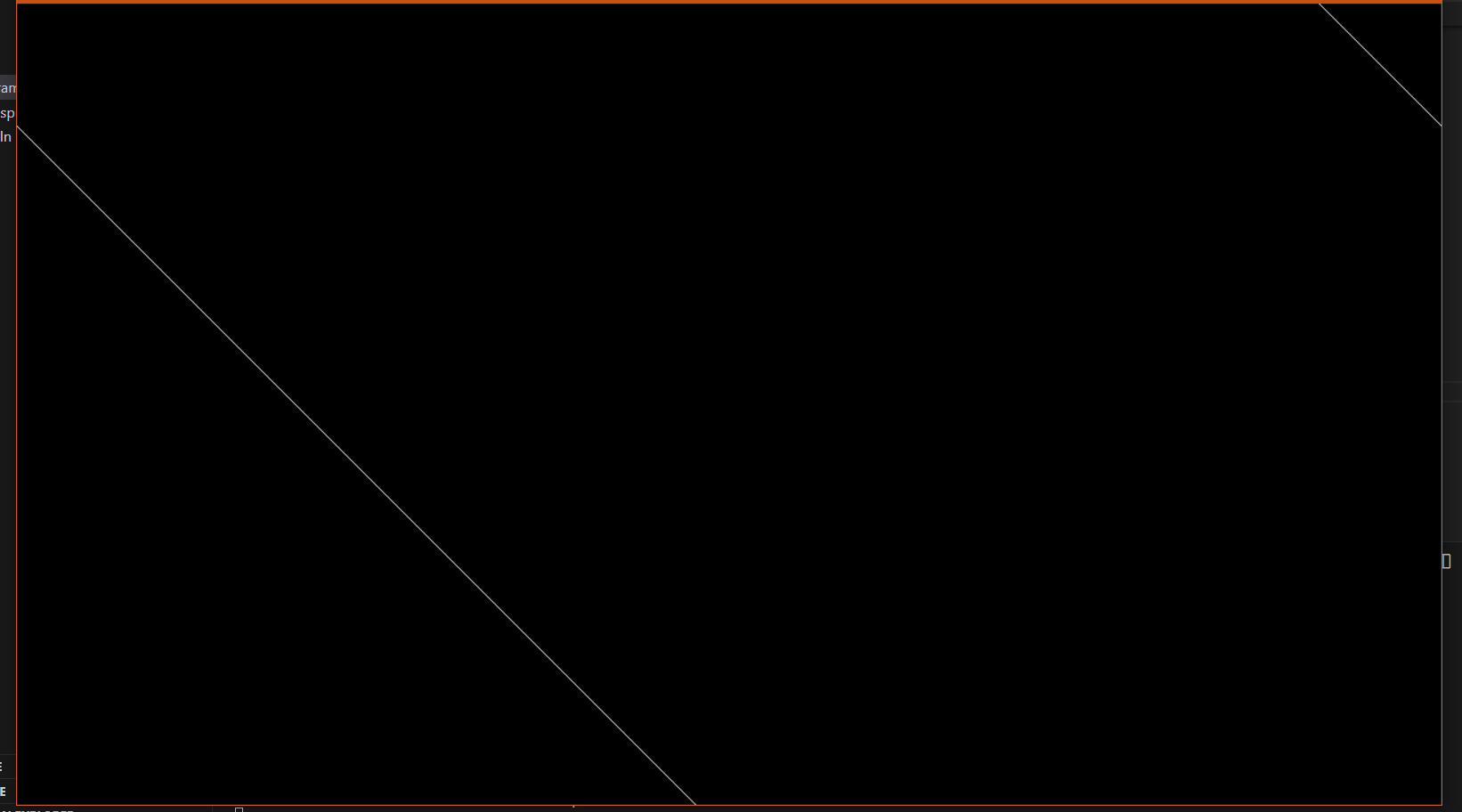
You should to remove
{}
after:SDL_LockTexture(pixels, NULL, &data, &pitch);
It works fine. :)
Result:
Nice idea - I am working on C# :)