Using Unity 3D, it takes a text file where each line contains a float value. It reads the float values in pairs; the first value is considered the X coordinate and the second the Z coordinate, and so on. For each of these pairs, it instanciates a prefab (a sphere), with its position based on these coordinates, in order to plot all the points onto a scene.
The text file is generated using a Ruby script, which takes a binary file of raw COLI data.
The raw COLI data is extracted from a MAPINFO.BIN
by hand. Specifically, you find COLS in the bin,
and you copy the first COLI section, but only the bytes (that is, without the string COLI and without
the next 4 bytes after COLI; these 4 bytes is the size of the COLI section, and this section starts
after these 4 bytes).
By inspecting the results, it is obvious that you are looking to the boundaries (collision data) of the map.
This was generated from the "0000" COLI of COLS of MAPINFO.BIN of MFSY (Harbor area) on Disc 3:
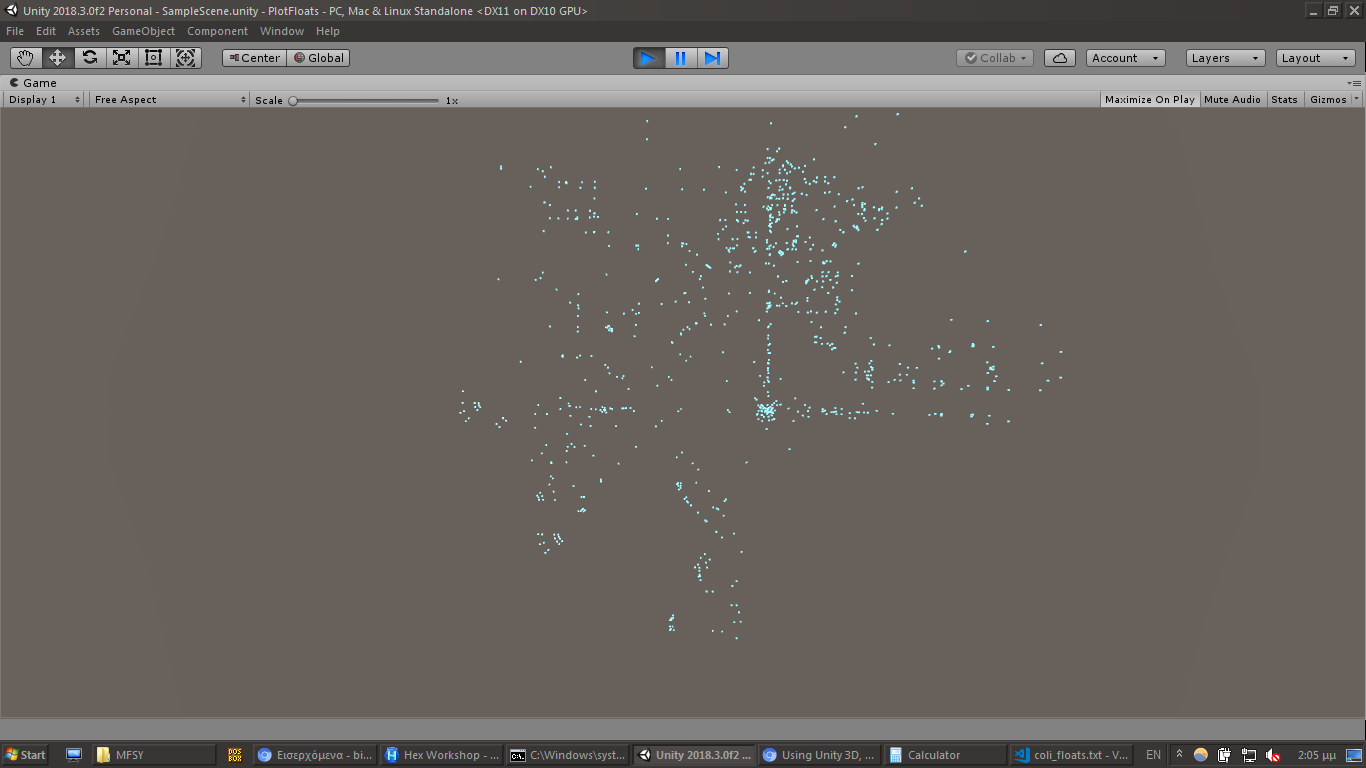