Last active
March 16, 2022 11:55
-
-
Save motorina0/e6bb1b32b3d5d2fc80e1bd63d5efea4f to your computer and use it in GitHub Desktop.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
// How to run: | |
// npm install -g ts-node typescript '@types/node' | |
// ts-node play9.ts | |
import * as ecc from 'tiny-secp256k1'; | |
import { ECPairFactory } from 'ecpair' | |
import { Psbt } from './ts_src/psbt' | |
import { p2tr } from './ts_src/payments/index' | |
import { testnet as network } from './ts_src/networks' | |
import * as bscript from './ts_src/script' | |
import { buildTapscriptFinalizer, toXOnly } from './test/psbt.utils'; | |
const ECPair = ECPairFactory(ecc); | |
const hex = (s: string) => Buffer.from(s, 'hex') | |
// one P2TR input, one P2TR output | |
const inP2trKey = ECPair.fromPrivateKey(hex('accaf12e04e11b08fc28f5fe75b47ea663843b698981e31f0000000000000000')) | |
const outP2trKey = ECPair.fromPrivateKey(hex('900afde76badc8914c9940379c74857d70b4d7da590097280000000000000000')) | |
// the leaf to be spent | |
const leafKey = ECPair.fromPrivateKey(hex('82fd530c9eb33570c7e05ca5e80b740bcf1118e8f4c73d440000000000000000')) | |
const leafScriptAsm = `${toXOnly(leafKey.publicKey).toString('hex')} OP_CHECKSIG` | |
const leafScript = bscript.fromASM(leafScriptAsm); | |
// Tap tree. We are going to spend one leaf | |
const scriptTree: any = [ | |
[ | |
{ | |
"output": bscript.fromASM("50929b74c1a04954b78b4b6035e97a5e078a5a0f28ec96d547bfee9ace803ac0 OP_CHECKSIG") | |
}, | |
[ | |
{ | |
"output": bscript.fromASM("50929b74c1a04954b78b4b6035e97a5e078a5a0f28ec96d547bfee9ace803ac1 OP_CHECKSIG") | |
}, | |
{ | |
"output": bscript.fromASM("2258b1c3160be0864a541854eec9164a572f094f7562628281a8073bb89173a7 OP_CHECKSIG") | |
} | |
] | |
], | |
[ | |
{ | |
"output": bscript.fromASM("50929b74c1a04954b78b4b6035e97a5e078a5a0f28ec96d547bfee9ace803ac2 OP_CHECKSIG") | |
}, | |
[ | |
{ | |
"output": bscript.fromASM("50929b74c1a04954b78b4b6035e97a5e078a5a0f28ec96d547bfee9ace803ac3 OP_CHECKSIG") | |
}, | |
[ | |
{ | |
"output": bscript.fromASM("50929b74c1a04954b78b4b6035e97a5e078a5a0f28ec96d547bfee9ace803ac4 OP_CHECKSIG") | |
}, | |
{ | |
"output": leafScript | |
} | |
] | |
] | |
] | |
] | |
const redeem = { | |
output: leafScript, | |
redeemVersion: 192, | |
}; | |
// script-path spend from input | |
const inP2tr = p2tr({ internalPubkey: toXOnly(inP2trKey.publicKey), scriptTree, redeem, network }, { eccLib: ecc }) | |
// send to key-path only spent | |
const outP2tr = p2tr({ internalPubkey: toXOnly(outP2trKey.publicKey), network }, { eccLib: ecc }) | |
console.log('### inP2tr.address', inP2tr.address) | |
// Build the transaction | |
const psbt = new Psbt({ network, eccLib: ecc }) | |
// spend p2tr | |
psbt.addInput({ | |
hash: hex('d17efa28568bb71533c2a2a85252a617be2d98576fd6e87557b8d0a8503454c4').reverse(), | |
index: 1, | |
}).updateInput(0, { | |
witnessScript: redeem.output, | |
witnessUtxo: { script: inP2tr.output!, value: 10000 } | |
}) | |
psbt.addOutput({ | |
address: outP2tr.address!, | |
value: 9000 | |
}) | |
psbt.signInput(0, leafKey) | |
const finalScriptFunc = buildTapscriptFinalizer(inP2trKey.publicKey, scriptTree, network) | |
psbt.finalizeInput(0, finalScriptFunc) | |
const tx = psbt.extractTransaction() | |
const rawTx = tx.toBuffer() | |
console.log('### txId', tx.getId()) | |
console.log('### rawTx', rawTx.toString('hex')) | |
// broadcast with: https://mempool.space/testnet/tx/push |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Receive funds to taproot address. Testnet TX here
Script-path spend. Testnet TX here
Witness for script-path spend (app here):
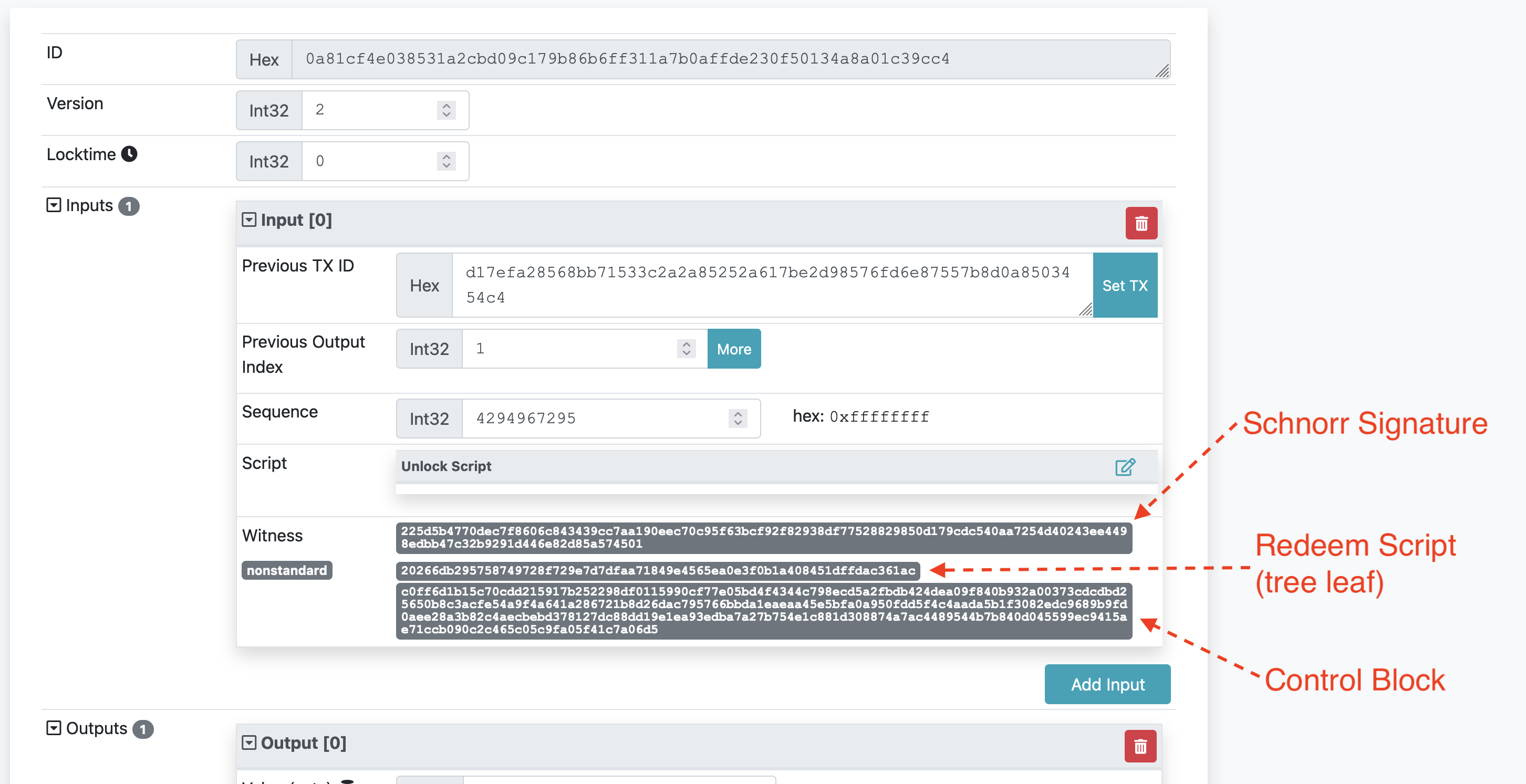