Created
August 26, 2018 15:47
-
-
Save mspclaims/e07bf1ff657fa8eb4756bc0514a164fe to your computer and use it in GitHub Desktop.
Floating label issue
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
/* eslint-disable react/prop-types */ | |
import React from 'react'; | |
import PropTypes from 'prop-types'; | |
import classNames from 'classnames'; | |
import Select from 'react-select'; | |
import { withStyles } from '@material-ui/core/styles'; | |
import Typography from '@material-ui/core/Typography'; | |
import NoSsr from '@material-ui/core/NoSsr'; | |
import TextField from '@material-ui/core/TextField'; | |
import Input from "@material-ui/core/Input"; | |
import Paper from '@material-ui/core/Paper'; | |
import Chip from '@material-ui/core/Chip'; | |
import MenuItem from '@material-ui/core/MenuItem'; | |
import { emphasize } from '@material-ui/core/styles/colorManipulator'; | |
const cities = "100 Mile House, 108 Mile Ranch, 150 Mile House, Abbotsford, Agassiz, Ahousat, Aldergrove, Alert Bay, Alexis Creek, Anahim Lake, Anmore, Armstrong, Ashcroft, Atlin, Baldonnel, Bamfield, Barriere, Beaverdell, Bella Coola, Big Lake, Black Creek, Blue River, Boston Bar, Bowen Island, Bowser, Brackendale, Brentwood Bay, Bridge Lake, Buick, Burnaby, Burns Lake, Burton, Cache Creek, Campbell River, Canal Flats, Canoe, Canyon, Castlegar, Cawston, Celista, Charlie Lake, Chase, Chemainus, Cherryville, Chetwynd, Chilanko Forks, Chilliwack, Christina Lake, Clearwater, Clinton, Cobble Hill, Coldstream, Comox, Coombs, Coquitlam, Courtenay, Cowichan Bay, Cranbrook, Crawford Bay, Crescent Valley, Creston, Crofton, Cultus Lake, Cumberland, Danskin, D'Arcy, Dawson Creek, Dease Lake, Delta, Denman Island, Deroche, Dewdney, Dog Creek, Douglas Lake, Duncan, Dunster, Edgewater, Edgewood, Elkford, Enderby, Erickson, Errington, Falkland, Farmington, Fernie, Field, Forest Grove, Fort Fraser, Fort Langley, Fort Nelson, Fort St James, Fort St John, Fort Ware, Francois Lake, Fraser Lake, Fruitvale, Gabriola, Gabriola Island, Galiano Island, Garibaldi Highlands, Gibsons, Gitwinksihlkw, Gold Bridge, Gold River, Golden, Grand Forks, Granisle, Grasmere, Greenwood, Grindrod, Groundbirch, Hagensborg, Halfmoon Bay, Hanceville, Harrison Hot Springs, Hartley Bay, Hazelton, Hedley, Heffley Creek, Hixon, Holberg, Hope, Hornby Island, Horsefly, Houston, Hudson's Hope, Invermere, Iskut, Jaffray, Kaleden, Kamloops, Kaslo, Kelowna, Keremeos, Kimberley, Kincolith, Kitimat, Kitkatla, Kitwanga, Kyuquot, Lac La Hache, Ladysmith, Lake Cowichan, Langley, Lantzville, Lasqueti Island, Lax galts'ap, Lax Kw Alaams, Lazo, Likely, Lillooet, Lions Bay, Lister, Logan Lake, Lone Butte, Lower Nicola, Lower Post, Lumby, Lytton, Mackenzie, Madeira Park, Malakwa, Mansons Landing, Maple Ridge, Marysville, Masset, Mayne Island, McBride, Merritt, Merville, Midway, Mill Bay, Milner, Mission, Moberly Lake, Montney, Mount Lehman, Nakusp, Nanaimo, Nanoose Bay, Naramata, Nelson, Nemaiah Valley, New Aiyansh, New Denver, New Hazelton, New Westminster, Nimpo Lake, North Vancouver, Okanagan Falls, Oliver, Osoyoos, Oyama, Parksville, Peachland, Pemberton, Pender Island, Penticton, Pinantan Lake, Pitt Meadows, Port Alberni, Port Alice, Port Clements, Port Coquitlam, Port Edward, Port Hardy, Port McNeill, Port Moody, Port Renfrew, Pouce Coupe, Powell River, Prespatou, Prince George, Prince Rupert, Princeton, Qualicum Beach, Quathiaski Cove, Quatsino, Queen Charlotte, Queen Charlotte City, Quesnel, Revelstoke, Richmond, Roberts Creek, Robson, Rock Creek, Rolla, Rosedale, Rossland, Royston, Saanichton, Salmo, Salmon Arm, Salt Spring Island, Sandspit, Saturna Island, Savona, Sayward, Sechelt, Shalalth, Shawnigan Lake, Sicamous, Sidney, Simoom Sound, Skidegate, Slocan, Smithers, Sointula, Sooke, Sorrento, South Hazelton, South Slocan, Sparwood, Squamish, Stewart, Summerland, Surge Narrows, Surrey, Tahsis, Tappen, Tatla Lake, Taylor, Telegraph Creek, Telkwa, Terrace, Tofino, Tomslake, Topley, Trail, Tsawwassen, Tumbler Ridge, Ucluelet, Valemount, Van Anda, Vancouver, Vanderhoof, Vavenby, Vernon, Victoria, Waglisla, Wells, West Vancouver, Westbank, Westwold, Whistler, White Rock, Williams Lake, Willow River, Windermere, Winfield, Winlaw, Wonowon, Woss, Wynndel, Yahk, Yale, Zeballos"; | |
const suggestions = cities.split(',').map((value) => ({ label:value.trim(), value:value.trim() })); | |
const styles = theme => ({ | |
root: { | |
flexGrow: 1, | |
height: 250, | |
}, | |
input: { | |
display: 'flex', | |
padding: 0, | |
}, | |
valueContainer: { | |
display: 'flex', | |
flexWrap: 'wrap', | |
flex: 1, | |
alignItems: 'center', | |
}, | |
chip: { | |
margin: `${theme.spacing.unit / 2}px ${theme.spacing.unit / 4}px`, | |
}, | |
chipFocused: { | |
backgroundColor: emphasize( | |
theme.palette.type === 'light' ? theme.palette.grey[300] : theme.palette.grey[700], | |
0.08, | |
), | |
}, | |
noOptionsMessage: { | |
padding: `${theme.spacing.unit}px ${theme.spacing.unit * 2}px`, | |
}, | |
singleValue: { | |
fontSize: 16, | |
}, | |
placeholder: { | |
position: 'absolute', | |
left: 2, | |
fontSize: 16, | |
}, | |
paper: { | |
marginTop: theme.spacing.unit, | |
}, | |
divider: { | |
height: theme.spacing.unit * 2, | |
}, | |
}); | |
function NoOptionsMessage(props) { | |
return ( | |
<Typography | |
color="textSecondary" | |
className={props.selectProps.classes.noOptionsMessage} | |
{...props.innerProps} | |
> | |
{props.children} | |
</Typography> | |
); | |
} | |
function inputComponent({ inputRef, ...props }) { | |
return <div ref={inputRef} {...props} />; | |
} | |
function Control(props) { | |
return ( | |
<TextField | |
fullWidth | |
InputProps={{ | |
inputComponent, | |
inputProps: { | |
className: props.selectProps.classes.input, | |
inputRef: props.innerRef, | |
children: props.children, | |
...props.innerProps, | |
}, | |
}} | |
{...props.selectProps.textFieldProps} | |
/> | |
); | |
} | |
function Option(props) { | |
return ( | |
<MenuItem | |
buttonRef={props.innerRef} | |
selected={props.isFocused} | |
component="div" | |
style={{ | |
fontWeight: props.isSelected ? 500 : 400, | |
}} | |
{...props.innerProps} | |
> | |
{props.children} | |
</MenuItem> | |
); | |
} | |
function Placeholder(props) { | |
return ( | |
<Typography | |
color="textSecondary" | |
className={props.selectProps.classes.placeholder} | |
{...props.innerProps} | |
> | |
{props.children} | |
</Typography> | |
); | |
} | |
function SingleValue(props) { | |
return ( | |
<Typography className={props.selectProps.classes.singleValue} {...props.innerProps}> | |
{props.children} | |
</Typography> | |
); | |
} | |
function ValueContainer(props) { | |
return <div className={props.selectProps.classes.valueContainer}>{props.children}</div>; | |
} | |
function MultiValue(props) { | |
return ( | |
<Chip | |
tabIndex={-1} | |
label={props.children} | |
className={classNames(props.selectProps.classes.chip, { | |
[props.selectProps.classes.chipFocused]: props.isFocused, | |
})} | |
onDelete={event => { | |
props.removeProps.onClick(); | |
props.removeProps.onMouseDown(event); | |
}} | |
/> | |
); | |
} | |
function Menu(props) { | |
return ( | |
<Paper square className={props.selectProps.classes.paper} {...props.innerProps}> | |
{props.children} | |
</Paper> | |
); | |
} | |
const components = { | |
Option, | |
Control, | |
NoOptionsMessage, | |
Placeholder, | |
SingleValue, | |
MultiValue, | |
ValueContainer, | |
Menu, | |
}; | |
class IntegrationReactSelect extends React.Component { | |
state = { | |
single: {value:''}, | |
multi: null, | |
id:'' | |
}; | |
constructor (props) { | |
super(props); | |
this.state.id=props.id; | |
this.textInput = React.createRef(); | |
} | |
handleChange = name => value => { | |
this.setState({ | |
[name]: value, | |
}); | |
if (this.props.onSelect) { | |
this.props.onSelect(); | |
} | |
if (this.props.labelRef) { | |
console.log('ready to move'); | |
//debugger; | |
//console.log(this.props.labelRef.current); | |
//this.inputRef.current.Ma | |
//this.textInput.current.setState({...this.textInput.current.state, hasValue: true}) | |
} | |
}; | |
render() { | |
const { classes, theme } = this.props; | |
const selectStyles = { | |
input: base => ({ | |
...base, | |
color: theme.palette.text.primary, | |
}), | |
}; | |
return ( | |
<div className={classes.root}> | |
<NoSsr> | |
<Select | |
classes={classes} | |
styles={selectStyles} | |
options={suggestions} | |
components={components} | |
value={this.state.single} | |
onChange={this.handleChange('single')} | |
placeholder="" | |
/> | |
</NoSsr> | |
{/* <TextField ref={this.textInput} id={this.state.id} type='hidden' value={this.state.single.value}/> */} | |
</div> | |
); | |
} | |
} | |
IntegrationReactSelect.propTypes = { | |
classes: PropTypes.object.isRequired, | |
theme: PropTypes.object.isRequired, | |
}; | |
export default withStyles(styles, { withTheme: true })(IntegrationReactSelect); |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
import React from "react"; | |
import classNames from "classnames"; | |
import PropTypes from "prop-types"; | |
// @material-ui/core components | |
import withStyles from "@material-ui/core/styles/withStyles"; | |
import FormControl from "@material-ui/core/FormControl"; | |
import InputLabel from "@material-ui/core/InputLabel"; | |
import Input from "@material-ui/core/Input"; | |
// @material-ui/icons | |
import Clear from "@material-ui/icons/Clear"; | |
import Check from "@material-ui/icons/Check"; | |
// core components | |
import customInputStyle from "assets/jss/material-dashboard-react/components/customInputStyle"; | |
import AutoComplete from "./AutoComplete"; | |
function CustomAutoComplete({ ...props }) { | |
const { | |
classes, | |
formControlProps, | |
labelText, | |
id, | |
labelProps, | |
inputProps, | |
error, | |
success | |
} = props; | |
const labelClasses = classNames({ | |
[" " + classes.labelRootError]: error, | |
[" " + classes.labelRootSuccess]: success && !error | |
}); | |
const underlineClasses = classNames({ | |
[classes.underlineError]: error, | |
[classes.underlineSuccess]: success && !error, | |
[classes.underline]: true | |
}); | |
const marginTop = classNames({ | |
[classes.marginTop]: labelText === undefined | |
}); | |
let labelRef = React.createRef(); | |
let margin3em = {marginTop:'3em'}; | |
let margin_1em = {marginTop:'-1em'}; | |
return ( | |
<FormControl | |
style = {margin3em} | |
{...formControlProps} | |
className={formControlProps.className + " " + classes.formControl} | |
> | |
{labelText !== undefined ? ( | |
<InputLabel | |
ref={labelRef} | |
style={margin_1em} | |
className={classes.labelRoot + labelClasses} | |
htmlFor={id} | |
{...labelProps} | |
> | |
{labelText} | |
</InputLabel> | |
) : null} | |
<AutoComplete | |
classes={{ | |
root: marginTop, | |
disabled: classes.disabled, | |
underline: underlineClasses | |
}} | |
labelRef = {labelRef} | |
id={id} | |
{...inputProps} | |
/> | |
{error ? ( | |
<Clear className={classes.feedback + " " + classes.labelRootError} /> | |
) : success ? ( | |
<Check className={classes.feedback + " " + classes.labelRootSuccess} /> | |
) : null} | |
</FormControl> | |
); | |
} | |
CustomAutoComplete.propTypes = { | |
classes: PropTypes.object.isRequired, | |
labelText: PropTypes.node, | |
labelProps: PropTypes.object, | |
id: PropTypes.string, | |
inputProps: PropTypes.object, | |
formControlProps: PropTypes.object, | |
error: PropTypes.bool, | |
success: PropTypes.bool | |
}; | |
export default withStyles(customInputStyle)(CustomAutoComplete); |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Component added to the page like follows:
When item selected, label stays :
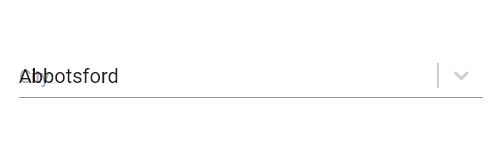