Created
January 3, 2020 20:13
-
-
Save muhleder/b34aa55a19954b15bbc50617240c98b8 to your computer and use it in GitHub Desktop.
Chart memory leak example
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
// Flutter code sample for Scaffold | |
// This example shows a [Scaffold] with an [AppBar], a [BottomAppBar] and a | |
// [FloatingActionButton]. The [body] is a [Text] placed in a [Center] in order | |
// to center the text within the [Scaffold]. The [FloatingActionButton] is | |
// centered and docked within the [BottomAppBar] using | |
// [FloatingActionButtonLocation.centerDocked]. The [FloatingActionButton] is | |
// connected to a callback that increments a counter. | |
// | |
// 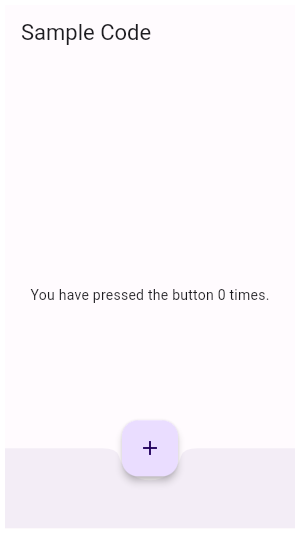 | |
import 'package:flutter/material.dart'; | |
import 'patched/syncfusion_flutter_charts/lib/charts.dart'; | |
void main() => runApp(MyApp()); | |
/// This Widget is the main application widget. | |
class MyApp extends StatelessWidget { | |
static const String _title = 'Flutter Code Sample'; | |
@override | |
Widget build(BuildContext context) { | |
return MaterialApp( | |
title: _title, | |
home: MyStatefulWidget(), | |
); | |
} | |
} | |
class MyStatefulWidget extends StatefulWidget { | |
MyStatefulWidget({Key key}) : super(key: key); | |
@override | |
_MyStatefulWidgetState createState() => _MyStatefulWidgetState(); | |
} | |
class DataPoint { | |
int end; | |
DateTime date; | |
} | |
class _MyStatefulWidgetState extends State<MyStatefulWidget> { | |
int _count = 0; | |
final myData = [ | |
DataPoint() | |
..date = DateTime(2020, 01, 01) | |
..end = 0, | |
DataPoint() | |
..date = DateTime(2020, 01, 02) | |
..end = 100, | |
]; | |
Widget build(BuildContext context) { | |
final chartKey = Key('chartKey'); | |
return Scaffold( | |
appBar: AppBar( | |
title: Text('Sample Code'), | |
), | |
body: Center( | |
child: Column( | |
children: <Widget>[ | |
Text('You have pressed the button $_count times.'), | |
SfCartesianChart( | |
// Uncomment to force dispose on rebuild and stop memory leak | |
// key: UniqueKey(), | |
primaryXAxis: DateTimeAxis(), | |
primaryYAxis: NumericAxis(), | |
key: chartKey, | |
series: <ChartSeries>[ | |
SplineSeries<DataPoint, DateTime>( | |
animationDuration: 0, | |
width: 1, | |
splineType: SplineType.monotonic, | |
dataSource: myData, | |
xValueMapper: (DataPoint s, _) => s.date, | |
yValueMapper: (DataPoint s, _) => s.end, | |
) | |
], | |
) | |
], | |
), | |
), | |
bottomNavigationBar: BottomAppBar( | |
shape: const CircularNotchedRectangle(), | |
child: Container( | |
height: 50.0, | |
), | |
), | |
floatingActionButton: FloatingActionButton( | |
onPressed: () => setState(() { | |
_count++; | |
}), | |
tooltip: 'Increment Counter', | |
child: Icon(Icons.add), | |
), | |
floatingActionButtonLocation: FloatingActionButtonLocation.centerDocked, | |
); | |
} | |
} |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment