Created
May 22, 2020 18:04
-
-
Save naywin-programmer/808518b445d0e92d111dc76b40646efe to your computer and use it in GitHub Desktop.
To Create Responsive Datatable With Flutter Built-in Datatable
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
// Flutter Responsive Datatable | |
// Flutter Datatable Class: https://api.flutter.dev/flutter/material/DataTable-class.html | |
// Flutter code sample for DataTable | |
// This sample shows how to display a [DataTable] with three columns: name, age, and | |
// role. The columns are defined by three [DataColumn] objects. The table | |
// contains three rows of data for three example users, the data for which | |
// is defined by three [DataRow] objects. | |
// | |
// 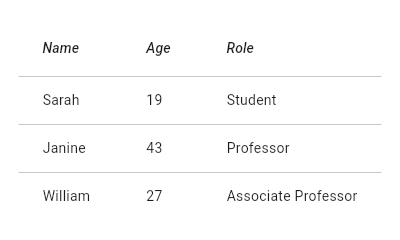 | |
import 'package:flutter/material.dart'; | |
void main() => runApp(MyApp()); | |
/// This Widget is the main application widget. | |
class MyApp extends StatelessWidget { | |
static const String _title = 'Flutter Code Sample'; | |
@override | |
Widget build(BuildContext context) { | |
return MaterialApp( | |
title: _title, | |
home: Scaffold( | |
appBar: AppBar(title: const Text(_title)), | |
body: MyStatelessWidget(), | |
), | |
); | |
} | |
} | |
/// This is the stateless widget that the main application instantiates. | |
class MyStatelessWidget extends StatelessWidget { | |
MyStatelessWidget({Key key}) : super(key: key); | |
@override | |
Widget build(BuildContext context) { | |
return DataTable( | |
columnSpacing: (MediaQuery.of(context).size.width / 10) * 0.5, | |
dataRowHeight: 80, | |
columns: const <DataColumn>[ | |
DataColumn( | |
label: Text( | |
'Name', | |
style: TextStyle(fontStyle: FontStyle.italic), | |
), | |
), | |
DataColumn( | |
label: Text( | |
'Age In \nCentury', | |
style: TextStyle(fontStyle: FontStyle.italic), | |
), | |
), | |
DataColumn( | |
label: Text( | |
'Description', | |
style: TextStyle(fontStyle: FontStyle.italic), | |
), | |
), | |
], | |
rows: [ | |
DataRow( | |
cells: <DataCell>[ | |
DataCell(Container(width: (MediaQuery.of(context).size.width / 10) * 3, child: Text('Sarahisbest playerintheworldplayerintheworldplayerintheworld'))), | |
DataCell(Container(width: (MediaQuery.of(context).size.width / 10) * 2, child: Text('19'))), | |
DataCell(Container(width: (MediaQuery.of(context).size.width / 10) * 3, child: Text('Student is so good.'))), | |
], | |
), | |
DataRow( | |
cells: <DataCell>[ | |
DataCell(Text('Janine is really talented player.')), | |
DataCell(Text('43')), | |
DataCell(Text('Professor')), | |
], | |
), | |
DataRow( | |
cells: <DataCell>[ | |
DataCell(Text('William')), | |
DataCell(Text('7')), | |
DataCell(Text('Associate Professor')), | |
], | |
), | |
], | |
); | |
} | |
} |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Small Screen
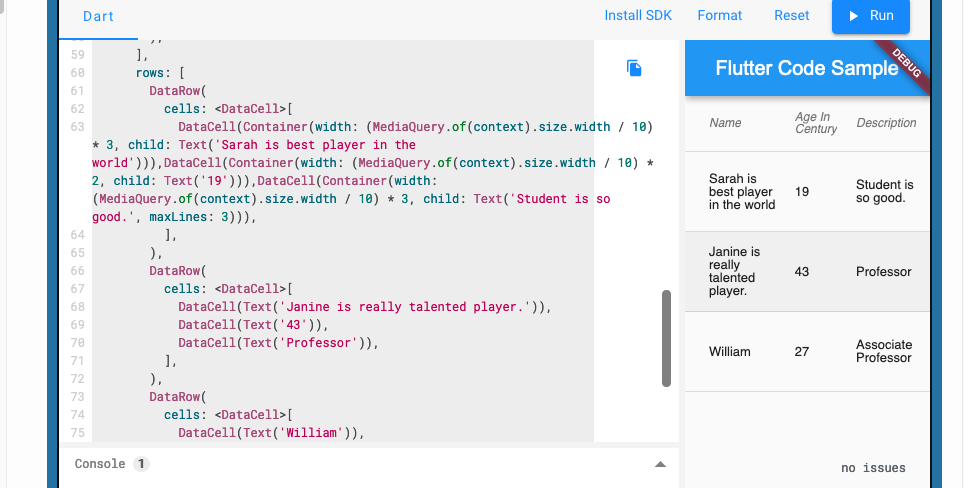
Wild Screen
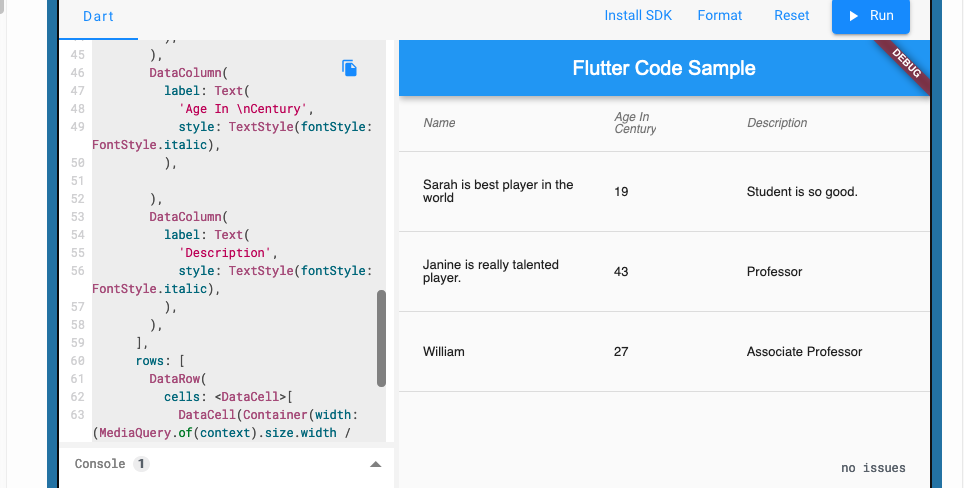