Last active
October 21, 2022 17:25
-
-
Save no-vac/f211c5fc20157d909fcac279e82639ac to your computer and use it in GitHub Desktop.
TamperMonkey script for HourGlass adding ability to save/load/delete presets
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
// ==UserScript== | |
// @name HourGlass Preset Mod (w/time&details save) | |
// @namespace cnovac.HourGlassPreset | |
// @downloadURL https://gist.githubusercontent.com/no-vac/f211c5fc20157d909fcac279e82639ac/raw/HourGlass%2520Preset%2520Mod | |
// @updateURL https://gist.githubusercontent.com/no-vac/f211c5fc20157d909fcac279e82639ac/raw/HourGlass%2520Preset%2520Mod | |
// @version 0.10 | |
// @description Adds adding/removing presets for product,project,activity,capitalize,international,time,details | |
// @author Cristian Novac | |
// @match https://sec.kmbs.us/version2/lab/hourglass/* | |
// @icon data:image/png;base64,iVBORw0KGgoAAAANSUhEUgAAABAAAAAQCAYAAAAf8/9hAAAAAXNSR0IArs4c6QAAAihJREFUOE+Nk81rGlEUxc+zSTok/bCRFluIUQiZ7kwXpcsaQksCDjqgC3FhpFTBRRsZ946FiinaQBcl2kWz0dKkMBZ10Z3+AQN2l9qNmCKUYGOQQkKTmfIMip+0Z/ce9/zmvnvPEIO0J4KKkHkQtQkFx+3zgM4OG8zRdvLh2dGvBQ3D/NAFg18mbupOSKfOKO0uKSD2Gu+8AA6r7PP5zCzLQhRFtFqtKoB7XQCtN0h7GypI9YB3ZAf86xaL5X08Hofb7Ua9Xsfi4iJkWfb2AahpPrsrEXXSW+X5Zg8kKwiCzeVyIZFIwGq1olAoIJPJRIYARumDUSGTYQJooYH28OXm3ZVben04HO7yKpUK/H4/fYZpCECr5qRPdg1RPI03b+3LM1fRMefzeaRSKfoEOugNADsjARTCLJm/P5ozLPSaRVGkRguAcqedcYAdjuM8/zK3tz9iXf9tHgUYMkei0dNra489x5/zH0eFg3agBbAFYJ3juL6BRWKxc93TJy+mV5ZNNbvDOw5QFgTBTHfcEZ02/bL+VfTylMnYJIrKK4BlVEoJy7JqMplELpcDhciyDH8g8Pt2YnNmymS8YKrIqqr6VQMUq7yz2NtJG0AvaDzT6TRKpRLeHVRPbrhdTF8hIV5VObcNppTOgO7UTLugCoVCYJ4FytMP7vdGGVDQJOqfoKKZeF6zOYPdHNxJbccar7dWT/e/mS/Nzv68srZavO7g98f8kUPXfwG+qeQRQ36Z2gAAAABJRU5ErkJggg== | |
// @grant none | |
// ==/UserScript== | |
function log(){ | |
console.log('[TM]:',...arguments) | |
} | |
(function () { | |
"use strict"; | |
const defaults = [ | |
{ name: "DSP meeting", product: 1, project: 336, activity: 9, capitalize: true, international: false }, | |
{ name: "DP meeting", product: 1, project: 234, activity: 9, capitalize: false, international: false }, | |
]; | |
// localStorage.setItem('presets', JSON.stringify(defaults)); | |
let presets = JSON.parse(localStorage.getItem("presets")); | |
log("presets", presets); | |
const productEl = document.querySelector("#product"); | |
const projectEl = document.querySelector("#project"); | |
const activityEl = document.querySelector("#activity"); | |
//NS- 10/4/2022 - add saving of time and details text | |
const timeEl = document.querySelector('#time'); | |
const timeUnitsEl = document.querySelector('#time-units'); | |
const detailsEl = document.querySelector('#details'); | |
const capitalYesEl = document.querySelector("#yes-select-button"); | |
const capitalNoEl = document.querySelector("#no-select-button"); | |
const internYesEl = document.querySelector("#yes-int-select-button"); | |
const internNoEl = document.querySelector("#no-int-select-button"); | |
//NS- 10/4/2022 - reformat preset selector line | |
// create DIV to hold presets line | |
const presetSelectorRowDiv = document.createElement("div"); | |
presetSelectorRowDiv.classList.add("form-group", "col-sm-12", "col-md-12") | |
// create PRESET label | |
const presetSelectorLabel = document.createElement("label"); | |
presetSelectorLabel.innerText = "PRESET"; | |
presetSelectorLabel.classList.add("control-label", "col-sm-3", "col-md-3") | |
presetSelectorLabel.style.cssText += 'background-color: #111111'; | |
// attach label to preset DIV | |
presetSelectorRowDiv.appendChild(presetSelectorLabel); | |
// create DIV to hold preset selector | |
const presetSelectorDiv = document.createElement("div"); | |
presetSelectorDiv.classList.add("col-sm-6", "col-md-6") | |
presetSelectorDiv.style.cssText += 'padding: 0 0 0 9px'; | |
// create preset SELECT | |
const presetSelectorEl = document.createElement("select"); | |
presetSelectorEl.classList.add("form-control","input-sm") | |
presetSelectorEl.id = "presetSelector"; | |
const defaultOptionEl = document.createElement("option"); | |
defaultOptionEl.value = ""; | |
defaultOptionEl.selected = true; | |
defaultOptionEl.disabled = true; | |
defaultOptionEl.text = "select preset"; | |
presetSelectorEl.appendChild(defaultOptionEl); | |
// attach SELECT to preset SELECT div | |
presetSelectorDiv.appendChild(presetSelectorEl); | |
// create SELECT save button | |
const savePresetBtn = document.createElement("button"); | |
savePresetBtn.innerText = "Save"; | |
// attach SELECT save button to preset SELECT div | |
presetSelectorDiv.appendChild(savePresetBtn); | |
// create SELECT delete button | |
const deletePresetBtn = document.createElement("button"); | |
deletePresetBtn.innerText = "Delete"; | |
// attach SELECT delete button to preset SELECT div | |
presetSelectorDiv.appendChild(deletePresetBtn); | |
// add SELECT options to button to presetSelectorEl | |
if (presets) { | |
presets.forEach((preset) => { | |
addOption(preset); | |
}); | |
} | |
// set new preset row with selector | |
presetSelectorRowDiv.append(presetSelectorDiv); | |
// insert new preset row above task entry area | |
document.querySelector(".form-group").prepend(presetSelectorRowDiv) | |
const handleSavePreset = () => { | |
const presetName = prompt("Enter Preset Name. Note: If preset with same name is present it will be replaced", ""); | |
if (!presetName) return; | |
const newPreset = { | |
name: presetName, | |
product: productEl.value, | |
project: projectEl.value, | |
activity: activityEl.value, | |
//NS- 10/4/2022 - add saving of time and details text | |
time: timeEl.value, | |
timeUnits: timeUnitsEl.value, | |
details: detailsEl.value, | |
capitalize: capitalYesEl.classList.contains("btn-lit") ? true : false, | |
international: internYesEl.classList.contains("btn-lit") ? true : false | |
}; | |
let newPresets = presets === null ? [] : presets.filter((_preset) => _preset.name !== newPreset.name); | |
newPresets.push(newPreset); | |
deleteOption(newPreset); | |
addOption(newPreset); | |
presets = newPresets; | |
localStorage.setItem("presets", JSON.stringify(presets)); | |
log("preset saved"); | |
}; | |
const handleDeletePreset = () => { | |
let newPresets = presets.filter((_preset) => _preset.name !== presetSelectorEl.value); | |
presets = newPresets; | |
deleteOption(); | |
localStorage.setItem("presets", JSON.stringify(presets)); | |
handlepresetChange(); | |
log("preset delete"); | |
}; | |
const handlepresetChange = () => { | |
const _selectedEl = presetSelectorEl.selectedOptions[0]; | |
//Autoselect settings | |
productEl.value = _selectedEl.product; | |
$('#product').change(); | |
setTimeout(()=>{ //wait before selecting project | |
projectEl.value = _selectedEl.project; | |
$('#project').change(); | |
setTimeout(()=>{ //wait before selecting activity | |
activityEl.value = _selectedEl.activity; | |
$('#activity').change(); | |
},100); | |
}, 100); | |
//NS- 10/4/2022 - add saving of time and details text | |
timeEl.value = _selectedEl.time; | |
timeUnitsEl.value = _selectedEl.timeUnits; | |
detailsEl.value = _selectedEl.details; | |
log(_selectedEl.product, _selectedEl.project, _selectedEl.activity); | |
if (_selectedEl.capitalize) { | |
log("capitalized"); | |
isCapital = true; | |
capitalYesEl.click(); | |
} else { | |
log("not capitalized"); | |
isCapital = false; | |
capitalNoEl.click(); | |
} | |
if (_selectedEl.international) { | |
log("international"); | |
isIntern = true; | |
internYesEl.click(); | |
} else { | |
log("not international"); | |
isIntern = false; | |
internNoEl.click(); | |
} | |
}; | |
function deleteOption(preset) { | |
let idx = -1; | |
if (preset) { | |
idx = Array.from(document.querySelector('#presetSelector').options).findIndex(p => p.text === preset.name) | |
} else { | |
idx = presetSelectorEl.options.selectedIndex; | |
} | |
if (idx > 0) { | |
presetSelectorEl.options[idx] = null; | |
} | |
} | |
function addOption(preset) { | |
const optionEl = document.createElement("option"); | |
optionEl.text = preset.name; | |
optionEl.name = preset.name; | |
optionEl.value = preset.name; | |
optionEl.product = preset.product; | |
optionEl.project = preset.project; | |
optionEl.activity = preset.activity; | |
optionEl.capitalize = preset.capitalize; | |
optionEl.international = preset.international; | |
//NS- 10/4/2022 - add saving of time and details text | |
optionEl.time = preset.time; | |
optionEl.timeUnits = preset.timeUnits; | |
optionEl.details = preset.details; | |
presetSelectorEl.appendChild(optionEl); | |
} | |
presetSelectorEl.addEventListener("change", handlepresetChange); | |
savePresetBtn.addEventListener("click", handleSavePreset); | |
deletePresetBtn.addEventListener("click", handleDeletePreset); | |
})(); |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Installation Instructions using Tampermonkey:
Navigate to Tampermonkey Dashboard
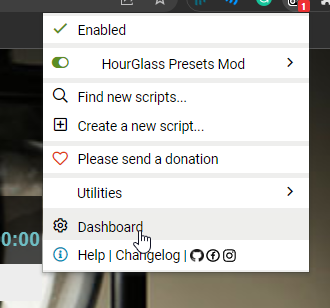
Click on Utilities tab
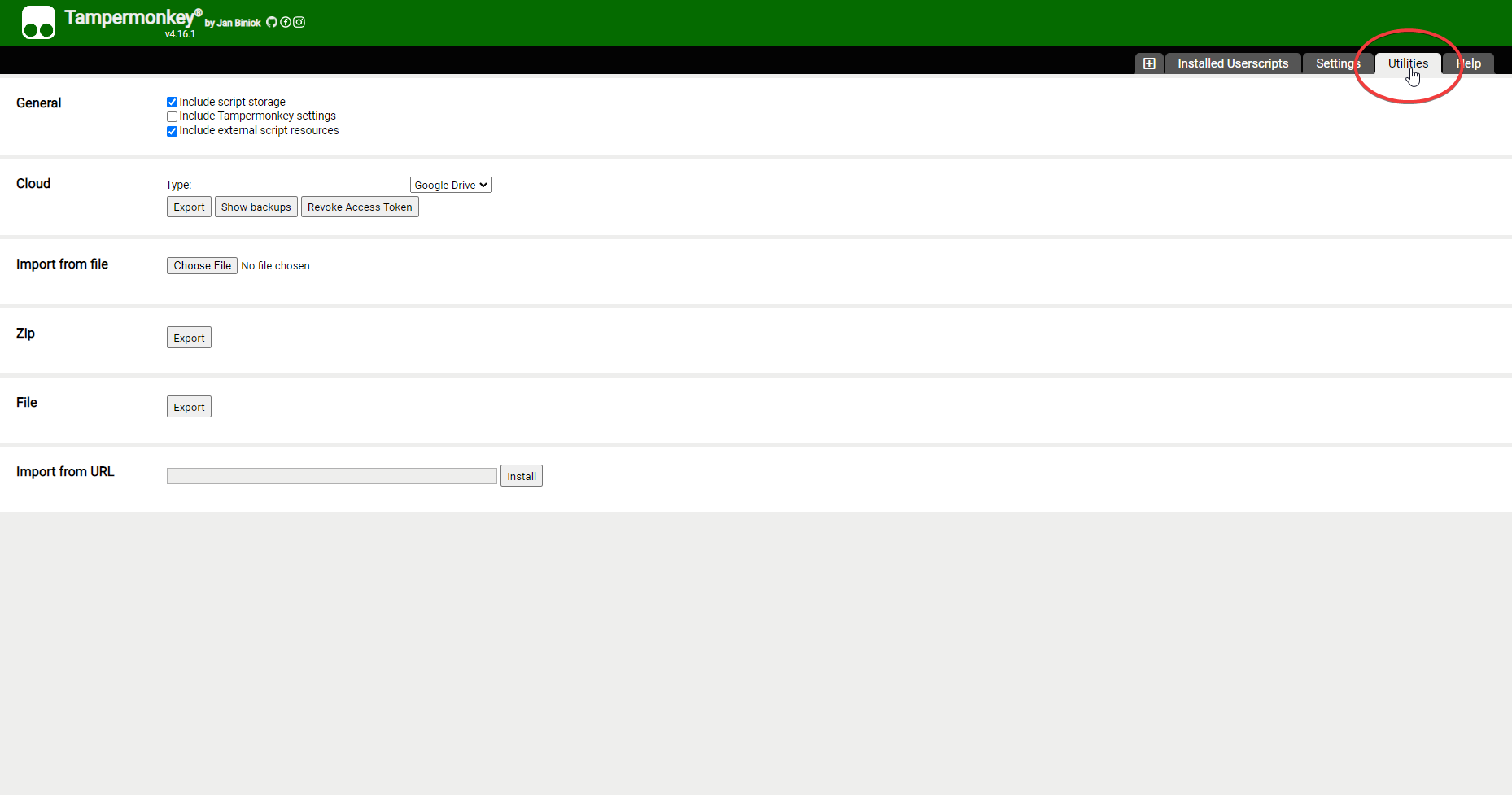
Find the Import from URL field.
Paste the gist RAW link and click Install

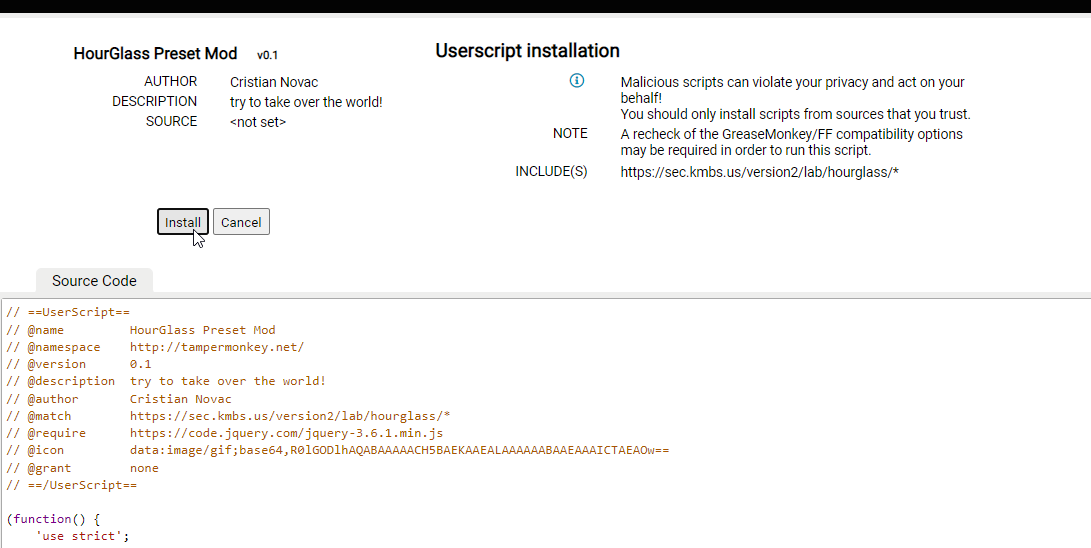
https://gist.github.com/no-vac/f211c5fc20157d909fcac279e82639ac/raw/HourGlass%2520Preset%2520Mod
You should now be able to see the installed script in the "Installed Scripts" tab.

Navigating to the hourglass page (or reloading it if you have it open) you should now see the preset buttons and the script working
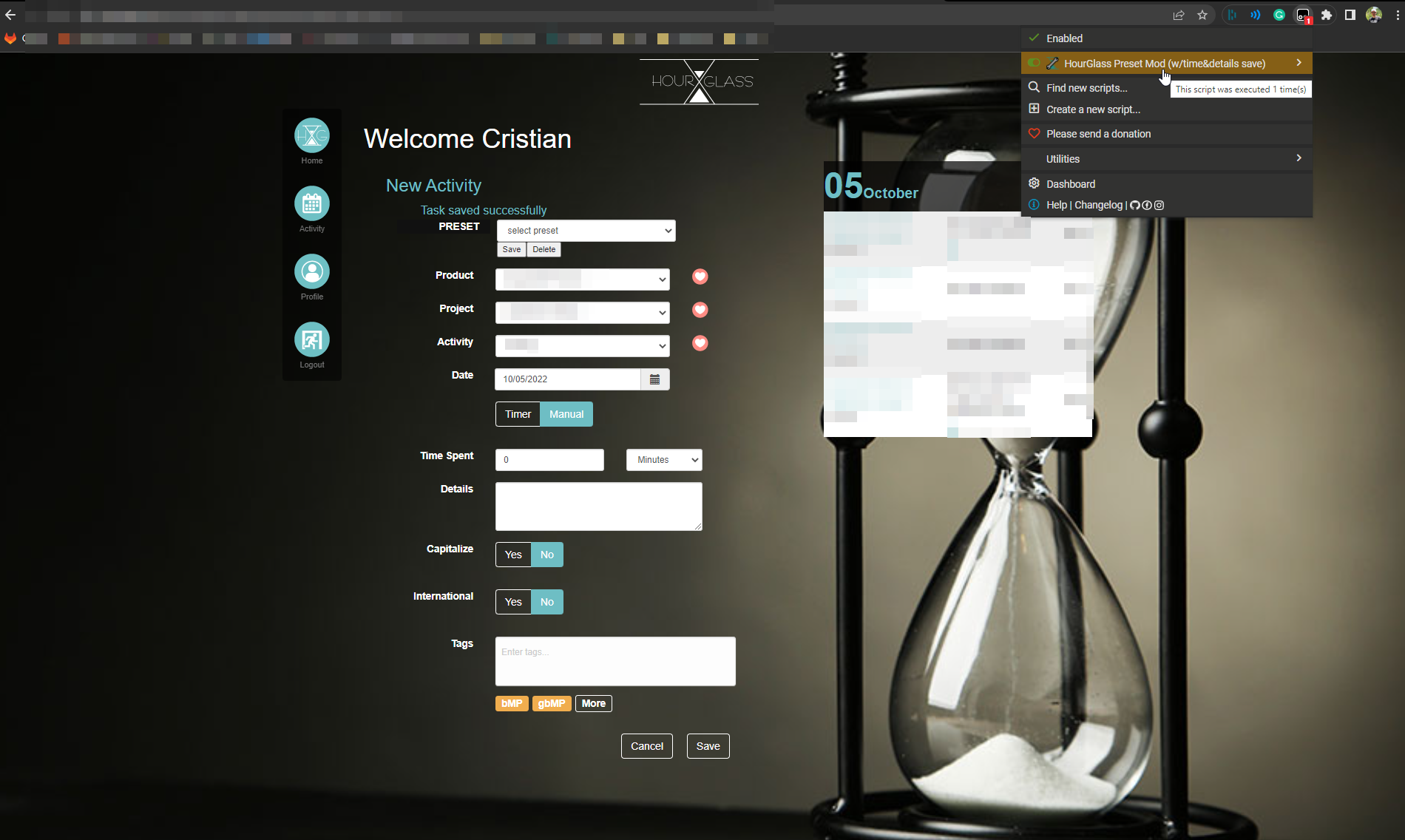
Updating Script
Tampermonkey by default automatically checks for script updates once a day, but if you want to force check for update you can go to Utilities > Check for userscript updates
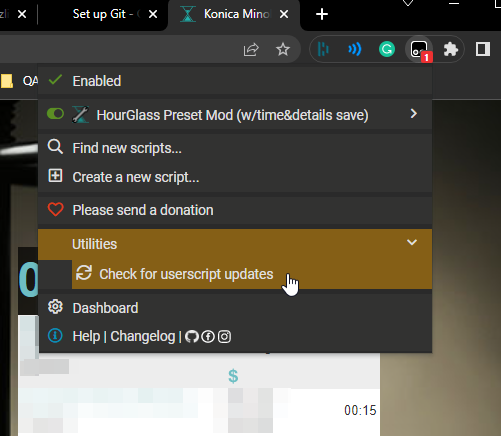