Last active
January 29, 2021 12:41
-
-
Save nuryagdym/9fe9ab4b3de3d5738d6f596ecf5db374 to your computer and use it in GitHub Desktop.
Angular JSON object to query string
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
import {HttpParams} from "@angular/common/http"; | |
export class QueryStringBuilder { | |
static buildParametersFromSearch<T>(obj: T): HttpParams { | |
let params: HttpParams= new HttpParams(); | |
if (obj == null) | |
{ | |
return params; | |
} | |
return QueryStringBuilder.populateSearchParams(params, '', obj); | |
} | |
private static populateArray<T>(params: HttpParams, prefix: string, val: Array<T>): HttpParams { | |
for (let index in val) { | |
let key = prefix + '[' + index + ']'; | |
let value: any = val[index]; | |
params = QueryStringBuilder.populateSearchParams(params, key, value); | |
} | |
return params; | |
} | |
private static populateObject<T>(params: HttpParams, prefix: string, val: T): HttpParams { | |
const objectKeys = Object.keys(val) as Array<keyof T>; | |
for (let objKey of objectKeys) { | |
let value = val[objKey]; | |
let key = prefix; | |
if (prefix) { | |
key += '[' + objKey + ']'; | |
} else { | |
key += objKey; | |
} | |
params = QueryStringBuilder.populateSearchParams(params, key, value); | |
} | |
return params; | |
} | |
private static populateSearchParams<T>(params: HttpParams, key: string, value: any): HttpParams { | |
if (value instanceof Array) { | |
return QueryStringBuilder.populateArray(params, key, value); | |
} | |
else if (value instanceof Date) { | |
return params.append(key, value.toISOString()); | |
} | |
else if (value instanceof Object) { | |
return QueryStringBuilder.populateObject(params, key, value); | |
} | |
else if ('undefined' !== typeof value && null !== value){ | |
return params.append(key, value.toString()); | |
} | |
return params; | |
} | |
} |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Usage:
Posted data will be:
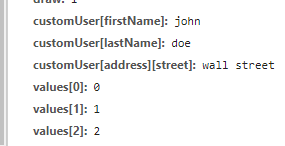
or as encoded:
customUser%5BfirstName%5D=john&customUser%5BlastName%5D=doe&customUser%5Baddress%5D%5Bstreet%5D=wall%20street&values%5B0%5D=0&values%5B1%5D=1&values%5B2%5D=2