Last active
April 28, 2023 08:33
-
-
Save oOtroyOo/da4583fb87782f6d7b082f079151ee5b to your computer and use it in GitHub Desktop.
Unity Editor - Draw a button on Project Window and Find asset by GUID
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
using System; | |
using System.IO; | |
using System.Linq; | |
using System.Reflection; | |
using GameFramework.Base; | |
using NUnit.Framework; | |
using UnityEditor; | |
using UnityEngine; | |
using UnityEngine.UIElements; | |
public class ProjectFindByGuid | |
{ | |
private static Type tProjectBrowser = Type.GetType("UnityEditor.ProjectBrowser,UnityEditor.dll"); | |
private static FieldInfo m_SearchFieldText = tProjectBrowser.GetField("m_SearchFieldText", BindingFlags.Instance | BindingFlags.NonPublic); | |
private static IMGUIContainer _container = new IMGUIContainer(OnGUI); | |
private static EditorWindow projectWindow; | |
[InitializeOnLoadMethod] | |
public static void OnLoad() | |
{ | |
AssemblyReloadEvents.afterAssemblyReload += Reload; | |
Reload(); | |
} | |
private static void Reload() | |
{ | |
EditorApplication.update -= Update; | |
EditorApplication.update += Update; | |
} | |
private static void Update() | |
{ | |
if (EditorApplication.isCompiling) | |
{ | |
EditorApplication.update -= Update; | |
return; | |
} | |
if (EditorWindow.focusedWindow != null && EditorWindow.focusedWindow.GetType() == tProjectBrowser) | |
{ | |
projectWindow = EditorWindow.focusedWindow; | |
if (!projectWindow.rootVisualElement.parent.hierarchy.Children().Contains(_container)) | |
{ | |
projectWindow.rootVisualElement.parent.hierarchy.Insert(1, _container); | |
_container.StretchToParentSize(); | |
} | |
} | |
if (projectWindow != null & !string.IsNullOrEmpty(errorMsg)) | |
{ | |
projectWindow.Repaint(); | |
} | |
} | |
private static GUIContent btnTitle; | |
private static GUIStyle btnStyle; | |
private static GUIStyle errorStyle; | |
private static string errorMsg; | |
private static double errorMsgStartTime; | |
static void OnGUI() | |
{ | |
if (projectWindow == null) | |
{ | |
return; | |
} | |
btnTitle = btnTitle ?? new GUIContent("搜索GUID", | |
EditorGUIUtility.IconContent("d_search_icon").image); | |
btnStyle = btnStyle ?? new GUIStyle(GUI.skin.button); | |
var calcSize = GUI.skin.button.CalcSize(btnTitle); | |
Rect rect = new Rect(new Vector2(0, 22 - calcSize.y), calcSize); | |
float xMin = 45; | |
float xRight = 415; | |
if (Screen.width < xMin + xRight) | |
{ | |
rect.x = xMin; | |
} | |
else | |
{ | |
rect.x = Screen.width - xRight; | |
} | |
var value = (string)m_SearchFieldText.GetValue(projectWindow); | |
if (!string.IsNullOrEmpty(value)) | |
{ | |
if (GUI.Button(rect, btnTitle)) | |
{ | |
var assetPath = AssetDatabase.GUIDToAssetPath(value); | |
if (!string.IsNullOrEmpty(assetPath)) | |
{ | |
GUI.FocusControl(null); | |
var asset = AssetDatabase.LoadMainAssetAtPath(assetPath); | |
EditorGUIUtility.PingObject(asset); | |
Selection.activeObject = asset; | |
} | |
else | |
{ | |
errorMsg = $"未找到GUID=[{value}] 的资源,请在搜索框输入正确的GUID"; | |
errorMsgStartTime = EditorApplication.timeSinceStartup; | |
Debug.LogError(errorMsg); | |
} | |
} | |
} | |
if (!string.IsNullOrEmpty(errorMsg)) | |
{ | |
var lerp = Mathf.Clamp01(1 - (float)(EditorApplication.timeSinceStartup - errorMsgStartTime - 1)); | |
if (lerp <= 0) | |
{ | |
errorMsg = null; | |
return; | |
} | |
errorStyle = errorStyle ?? "OL Ping"; | |
Color color = Color.white; | |
color.a = lerp; | |
GUI.color = color; | |
GUI.backgroundColor = color; | |
rect.y += 40; | |
rect.size = errorStyle.CalcSize(new GUIContent(errorMsg)); | |
GUI.Label(rect, errorMsg, errorStyle); | |
} | |
GUI.backgroundColor = Color.white; | |
GUI.color = Color.white; | |
} | |
} |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Not Found:
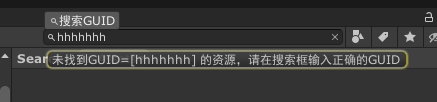