Last active
October 20, 2020 22:09
-
-
Save odahcam/727d46d072f237c19952cd5987946ee0 to your computer and use it in GitHub Desktop.
Scripts for Building and Testing pure TypeScript applications.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
{ | |
"main": "./cjs/index.js", | |
"module": "./esm/index.js", | |
"typings": "./esm/index.d.ts", | |
"esnext": "./esm/index.js", | |
"scripts": { | |
"clean": "yarn shx rm -rf dist output", | |
"test": "yarn test:build && yarn test:run", | |
"test:build": "tsc --build tsconfig.test.json", | |
"test:run": "mocha -r tsconfig-paths/register --timeout 200000 output/tests/**/*.spec.js", | |
"cover": "nyc --reporter=lcov --reporter=text yarn test", | |
"build": "yarn clean && yarn build:es2015 && yarn build:esm && yarn build:cjs && yarn build:umd && yarn build:umd:min && yarn build:copy", | |
"build:es2015": "tsc --build tsconfig.lib-es2015.json", | |
"build:esm": "tsc --build tsconfig.lib-esm.json", | |
"build:cjs": "tsc --build tsconfig.lib-cjs.json", | |
"build:umd": "rollup dist/esm/index.js --format umd --name ZXingLibrary --sourcemap --file dist/umd/zxing-library.js", | |
"build:umd:min": "cd dist/umd && terser --compress --mangle --source-map --screw-ie8 --comments -o zxing-library.min.js -- zxing-library.js && gzip zxing-library.min.js -c > zxing-library.min.js.gz", | |
"build:copy": "cp README.md dist && cp package.json dist && cp LICENSE dist", | |
"shx": "./node_modules/.bin/shx", | |
"tsc": "./node_modules/.bin/tsc", | |
} | |
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
{ | |
"compilerOptions": { | |
"baseUrl": "./", | |
"module": "commonjs", | |
"target": "es5", | |
"moduleResolution": "node", | |
"lib": [ | |
"es7", | |
"dom" | |
], | |
"alwaysStrict": true, | |
"noImplicitAny": false, | |
"sourceMap": true, | |
"declaration": true, | |
"preserveConstEnums": true, | |
"downlevelIteration": true, | |
"paths": { | |
"@zxing/library": [ | |
"./dist" | |
], | |
"@zxing/library/*": [ | |
"./dist/*" | |
] | |
} | |
}, | |
"include": [ | |
"src/**/*.ts" | |
] | |
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
{ | |
"extends": "./tsconfig.json", | |
"compilerOptions": { | |
"module": "commonjs", | |
"target": "es5", | |
"outDir": "dist/cjs" | |
}, | |
"exclude": [ | |
"src/test" | |
] | |
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
{ | |
"extends": "./tsconfig.json", | |
"compilerOptions": { | |
"module": "es2015", | |
"target": "es2015", | |
"outDir": "dist/es2015" | |
}, | |
"exclude": [ | |
"src/test" | |
] | |
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
{ | |
"extends": "./tsconfig.json", | |
"compilerOptions": { | |
"module": "es2015", | |
"target": "es5", | |
"outDir": "dist/esm" | |
}, | |
"exclude": [ | |
"src/test" | |
] | |
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
{ | |
"extends": "./tsconfig.json", | |
"compilerOptions": { | |
"target": "es6", | |
"module": "commonjs", | |
"moduleResolution": "node", | |
"lib": [ | |
"es7", | |
"dom" | |
], | |
"outDir": "output/tests" | |
}, | |
"include": [ | |
"src/test/**/*.ts" | |
], | |
"exclude": [ | |
"node_modules", | |
"src/core" | |
] | |
} |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
TypeScript outputs some polyfill function declarations checking for stuff inside
this
, which Rollup rewrites to undefined, since the UMD scopethis
points to the function scope itself.Using this knowledge, I've changed the
context
(which is theglobal
context orthis
the source is trying to achieve) option inrollup.config.js
so the library can find the window context and check for stuff it needs:context: '(globalThis || window || undefined)'
.Aand the result:
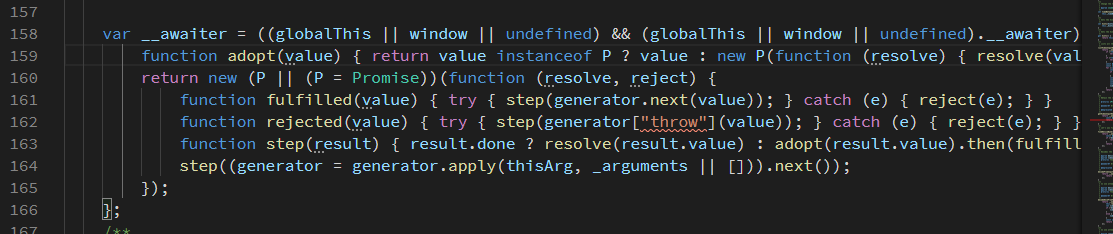
Looks awful doesn't it? 😄
The important part is that it works.
I'll be including the
rollup.config.js
file here once it is ready.