Created
January 15, 2022 16:46
-
-
Save oliverfoggin/7e2c5ab5e6973aa52187b66b776f1db6 to your computer and use it in GitHub Desktop.
Collection view reload data only reloading items once each...
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
import UIKit | |
class MyCollectionViewCell: UICollectionViewCell { | |
let label: UILabel = UILabel() | |
override init(frame: CGRect) { | |
super.init(frame: frame) | |
contentView.addSubview(label) | |
label.translatesAutoresizingMaskIntoConstraints = false | |
label.centerYAnchor.constraint(equalTo: contentView.centerYAnchor).isActive = true | |
label.centerXAnchor.constraint(equalTo: contentView.centerXAnchor).isActive = true | |
} | |
required init?(coder: NSCoder) { | |
fatalError("init(coder:) has not been implemented") | |
} | |
} | |
class ViewController: UIViewController, UICollectionViewDelegate, UICollectionViewDataSource { | |
let items: [Int] = Array(1...10) | |
@IBOutlet weak var collectionView: UICollectionView! | |
override func viewDidLoad() { | |
super.viewDidLoad() | |
// Do any additional setup after loading the view. | |
collectionView.register(MyCollectionViewCell.self, forCellWithReuseIdentifier: "cell") | |
navigationItem.rightBarButtonItem = UIBarButtonItem(barButtonSystemItem: .refresh, target: self, action: #selector(reload)) | |
} | |
@objc func reload() { | |
print("Reload") | |
collectionView.reloadData() // logs out "Reload" and then reloads the items, causing each item to log out from the `cellForItemAt` function | |
} | |
func collectionView(_ collectionView: UICollectionView, numberOfItemsInSection section: Int) -> Int { | |
items.count | |
} | |
func collectionView(_ collectionView: UICollectionView, cellForItemAt indexPath: IndexPath) -> UICollectionViewCell { | |
let cell = collectionView.dequeueReusableCell(withReuseIdentifier: "cell", for: indexPath) as! MyCollectionViewCell | |
let item = items[indexPath.item] | |
print(item) // Logs out 1-10 (items from the array) | |
cell.label.text = "\(item)" | |
return cell | |
} | |
} |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Here is the collection view...
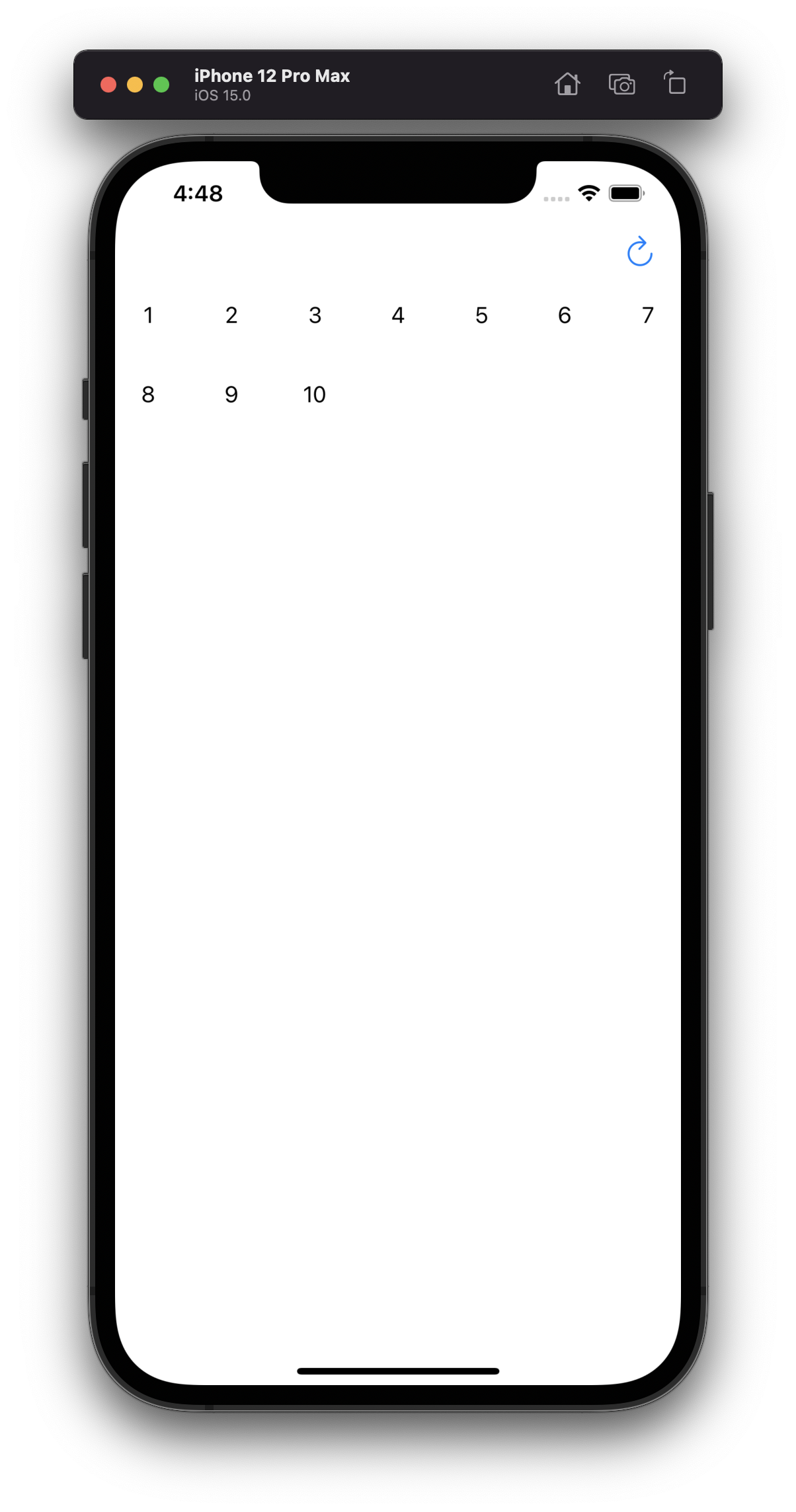
Here is the log. As you can see, each "Reload" then only logs 1...10 once each.
