Commands
bower i
pulp build --to app.js
Versions
- Pulp version 12.3.0
- purs version 0.12.0
{ | |
"name": "ps", | |
"ignore": [ | |
"**/.*", | |
"node_modules", | |
"bower_components", | |
"output" | |
], | |
"dependencies": { | |
"purescript-prelude": "^4.1.0", | |
"purescript-console": "^4.1.0", | |
"purescript-effect": "^2.0.0", | |
"purescript-canvas": "^4.0.0", | |
"purescript-signal": "^10.1.0" | |
}, | |
"devDependencies": { | |
"purescript-psci-support": "^4.0.0" | |
} | |
} |
<!DOCTYPE html> | |
<html lang="en"> | |
<head> | |
<meta charset="UTF-8"> | |
<meta name="viewport" content="width=device-width, initial-scale=1.0"> | |
<meta http-equiv="X-UA-Compatible" content="ie=edge"> | |
<title>Document</title> | |
</head> | |
<body> | |
<canvas id="scene" width="800" height="800"></canvas> | |
<script src="app.js"></script> | |
</body> | |
</html> |
module Main where | |
import Prelude | |
import Effect (Effect) | |
import Data.Maybe (Maybe(Just, Nothing)) | |
import Graphics.Canvas as C | |
import Signal (foldp, runSignal) | |
import Signal.DOM (animationFrame) | |
main :: Effect Unit | |
main = do | |
mcanvas <- C.getCanvasElementById "scene" | |
case mcanvas of | |
Just canvas -> do | |
context <- C.getContext2D canvas | |
frames <- animationFrame | |
let game = foldp (const update) initialState frames | |
runSignal (render context <$> game) | |
Nothing -> do | |
pure unit | |
type State = | |
{ x :: Number | |
, step :: Number | |
} | |
initialState :: State | |
initialState = | |
{ x: 0.0 | |
, step: 10.0 | |
} | |
scene :: | |
{ x :: Number | |
, y :: Number | |
, width :: Number | |
, height :: Number | |
, boxSize :: Number | |
} | |
scene = | |
{ x: 0.0 | |
, y: 0.0 | |
, width: 800.0 | |
, height: 800.0 | |
, boxSize: 25.0 | |
} | |
update :: State -> State | |
update state = | |
if state.x + scene.boxSize > scene.width then | |
{ x: scene.width - scene.boxSize | |
, step: -state.step | |
} | |
else if state.x < scene.x then | |
{ x: scene.x | |
, step: -state.step | |
} | |
else | |
{ x: state.x + state.step | |
, step: state.step | |
} | |
render :: C.Context2D -> State -> Effect Unit | |
render context state = do | |
clearCanvas context | |
drawRect context state | |
pure unit | |
clearCanvas :: C.Context2D -> Effect Unit | |
clearCanvas ctx = do | |
C.setFillStyle ctx "#000000" | |
C.fillRect ctx { x: 0.0, y: 0.0, width: scene.width, height: scene.height } | |
pure unit | |
drawRect :: C.Context2D -> State -> Effect Unit | |
drawRect ctx state = do | |
C.setFillStyle ctx "#0088DD" | |
C.fillRect ctx | |
{ x: state.x | |
, y: scene.height / 2.0 | |
, width: scene.boxSize | |
, height: scene.boxSize | |
} | |
pure unit |
Forked and some fixed to adapt PureScript version 0.12.0.
Forked form: https://gist.github.com/soupi/76103c45fc00a6c1478e
Captured GIF (Actual appearance is more smooth)
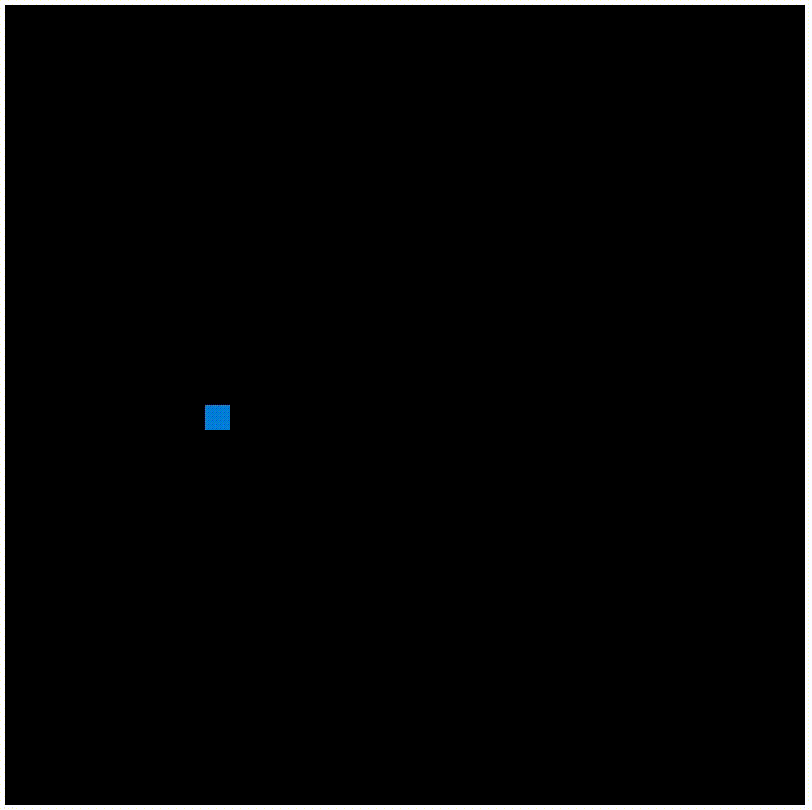