Last active
April 16, 2021 06:23
-
-
Save oradkovsky/ed9924a0d007ed10be44d244becb862b to your computer and use it in GitHub Desktop.
Glide shadow transformation
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
/** | |
* Quick and hacky way, tested under SDK19 and newer. | |
* How to use (key: in conjunction with CircleCrop): | |
* <code>transform(new CircleCrop(), new CircleShadowTransformation())</code> | |
*/ | |
public class CircleShadowTransformation extends BitmapTransformation { | |
private static final int SHADOW_RADIUS = 10; | |
private static final String ID = "a.b.c.transformations.CircleShadowTransformation"; | |
private static final byte[] ID_BYTES = ID.getBytes(Charset.forName(STRING_CHARSET_NAME)); | |
@Override | |
protected Bitmap transform(BitmapPool pool, Bitmap sourceBitmap, int outWidth, int outHeight) { | |
int size = sourceBitmap.getWidth(); | |
Bitmap outputBitmap = Bitmap.createBitmap( | |
size + SHADOW_RADIUS * 2, | |
size + SHADOW_RADIUS * 2, | |
Bitmap.Config.ARGB_8888); | |
Canvas outputCanvas = new Canvas(outputBitmap); | |
Paint shadow = new Paint(Paint.ANTI_ALIAS_FLAG); | |
shadow.setShadowLayer(SHADOW_RADIUS, 0, 0, Color.BLACK); | |
outputCanvas.drawCircle( | |
(size + SHADOW_RADIUS * 2) / 2, | |
(size + SHADOW_RADIUS * 2) / 2, | |
(size) / 2, | |
shadow); | |
outputCanvas.drawBitmap(sourceBitmap, SHADOW_RADIUS, SHADOW_RADIUS, null); | |
return outputBitmap; | |
} | |
@Override | |
public boolean equals(Object obj) { | |
return obj instanceof CircleShadowTransformation; | |
} | |
@Override | |
public int hashCode() { | |
return ID.hashCode(); | |
} | |
@Override | |
public void updateDiskCacheKey(MessageDigest messageDigest) { | |
messageDigest.update(ID_BYTES); | |
} | |
} |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Here is the sample (simple ImageView, 100x100dp)
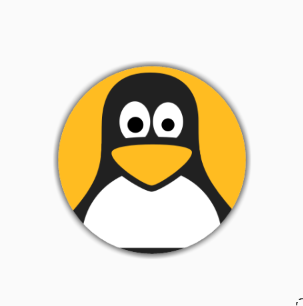