- E:
- F:
-
G:
- H:
- J:
- K:
- O:
- R:
-
S:
STD Libraries - STLs - String functions - Seive - Summation formulas - sqrt() funcs & log() funcs - Substr vs Subseq vs Subarr - Setprecision
- U - Z:
- find
- Find value in range (function template)
- count
- Count appearances of value in range (function template)
Modifying sequence operations:
- copy
- Copy range of elements (function template)
- swap
- Exchange values of two objects (function template)
- fill
- Fill range with value (function template)
- remove
- Remove value from range (function template)
- reverse
- Reverse range (function template)
- rotate
- Rotate left the elements in range (function template)
- shuffle
- Randomly rearrange elements in range using generator (function template)
Sorting:
- sort
- Sort elements in range (function template)
- is_sorted
- Check whether range is sorted (function template)
- lower_bound
- Return iterator to lower bound (function template)
- upper_bound
- Return iterator to upper bound (function template)
- includes
- Test whether sorted range includes another sorted range (function template)
- set_union
- Union of two sorted ranges (function template)
- set_intersection
- Intersection of two sorted ranges (function template)
- set_difference
- Difference of two sorted ranges (function template)
Min/max:
- min
- Return the smallest (function template)
- max
- Return the largest (function template)
Other:
- lexicographical_compare
- Lexicographical less-than comparison (function template)
- next_permutation
- Transform range to next permutation (function template)
- prev_permutation
- Transform range to previous permutation (function template)
//Even test
if( (n%2) == 9) // works for +ve values ONLY!
if( (n&1) == 1) // works for -ve an +ve values!
//print number in binary ( can also use it to count bits )
void printNumber(int n, int len)
{
if(!len)
return ;
printNumber(n>>1, len-1); // remove last bit
cout<<(n&1); // print last bit
cnt++; //count bits
}
//print number in binary (method 2) -> you also can save it as binary in bitset
int val = 10;
cout << bitset<bits_no>(val);
// Get bit
int getBit(int num, int idx) {
return ((num >> idx) & 1) == 1; // 110100, idx = 4 --> 110 & 1 = 0
}
//Set bit
int setBit1(int num, int idx) {
return num | (1<<idx);
}
//Reset bit
int setBit0(int num, int idx) {
return num & ~(1<<idx); // 110100, idx = 3 --> 110100 & ~000100 = 110100 & 111011
}
//Flip Bit
int flipBit(int num, int idx) {
return num ^ (1<<idx);
}
//used in Gettig mask subsets + Counting 1 bits + Getting first 1 bit from right (LSB)
/*
X-1 is very important!
X = 840 = 01101001000
X-1 = 839 = 01101000111
X & (X-1) = 01101000000 first bit from right removed
X & ~(X-1) = 01101001000 & 10010111000 = 00000001000 //get 1st 1 bit from (lsb)
*/
int countNumBits2(int mask) { // O(bits Count) // you also can use '__builtin_popcount()'
int ret = 0;
while (mask) {
mask &= (mask-1);
++ret; // Simply remove the last bit and so on
}
return ret;
}
//GET subsets of specific mask
//we know subsets of 101: 101, 100, 001, 000
void getAllSubMasks(int mask) {
for(int subMask = mask ; subMask ; subMask = (subMask - 1) & mask)
printNumber(subMask, 32); // this code doesn't print 0
// For reverse: ~subMask&mask = subMask^mask
}
//GET all subsets of length 'X'
// len = 3: 000, 001, 010, 011, 100, 101, 110, 111
void printAllSubsets(int len) // Remember we did it recursively! This is much SIMPLER!
{
for (int i = 0; i < (1<<len); ++i)
printNumber(i, len);
// For reversed order. Either reverse each item or work from big to small
//for (int i = (1<<len); i >= 0 ; --i)
// printNumber(i, len);
}
polarity method : if low starts at highly possible case it ends at opposite polarity (first impossiple case ) high the same ends at first possible case (high is answer!)
//only edit mid
int ans = 1;
int l = 1;//re-assure from input ranges
int h = arr[n - 1] - arr[0]; //re-assure from input ranges
while ( l < h ){
int m = (l + h + 1) / 2;
if ( h - l <= 4 ){
for (int i = l; i <= h; i++) //i could be decrementing
if ( ok(arr , ... , i) )
ans = i;
break;
}
if ( !ok(arr , ... , m) ) h = m - 1; // `h = m-1;` may be switched with else line: `l = m + 1;`
else{ ans = m; l = m + 1; }
}
double binarySearch(double st, double en){
double L = 0 , R = en, mid;
while(R - L > eps){ // eps: some very small value (dependent on the problem)
mid = L + (R - L) / 2;
if(valid(mid))
L = mid;
else
R = mid;
}
return L + (R - L) / 2; // mid
}
( maze and the general code template of backtracking ) :
// Typical backtracking procedure ( base case - state - transition )
void recursion(state s)
{
if( base(s) )
return ;
for each substate ss
mark ss
recursion (ss)
unmark ss
}
//example : maze
char maze(MAX , MAX){
//.SX..
//..X.E
//....X
//X.XX.
}
bool vis[MAX][MAX];
bool findEnd2(int r, int c) // Recursion State: r, c and FULL visted array
{
if( !valid(r, c) || maze[r][c] == 'X' || vis[r][c] == 1)
return false; // invalid position or block position
vis[r][c] = 1; // we just visited it, don't allow any one back to it
if( maze[r][c] =='E')
return true; // we found End
// Try the 4 neighbor cells
if(findEnd2(r, c-1)) return true; // search up
if(findEnd2(r, c+1)) return true; // search down
if(findEnd2(r-1, c)) return true; // search left
if(findEnd2(r+1, c)) return true; // search right
vis[r][c] = 0; // undo marking, other paths allowed to use it now
// Can't find a way for it!
return false;
}
example of backtraking that i studied before:
https://www.geeksforgeeks.org/write-a-c-program-to-print-all-permutations-of-a-given-string/
bool isPrime(int n) { // O(sqrt(n))
if (n == 2) return true;
if (n < 2 || !(n & 1)) return false;
for (int i = 3; i * i <= n; i += 2) {
if (n % i == 0) return false;
}
return true;
}
//lamda function (sort vector of pairs)
vector<pair<int, stack<int>>> arr(mp.begin() , mp.end());
sort(arr.begin(), arr.end(), [](const auto& a, const auto& b) { return a.first > b.first;});
//compare function ( sort desc. by first if equal sort desc. by second)
bool comp ( pair<int, int> &a , pair<int,int> &b){
if ( a.F != b.F ){
return a.F > b.F;
}else{
return a.S > b.S;
}
}
//traverse and print all zeroes in main diagonal
for (int step = 0 ; step < row; step++)
cout << arr[step][step];
//traverse and print all zeroes in anti diagonal
for (int step = 0 ; step < row; step++)
cout << arr[step][colm - 1 - step];
// in iterative style
ll fastPow(ll base, ll power /*, ll m*/) { // O(log[power])
ll ans = 1;
while (power) {
if (power & 1)
ans = (ans * base); // if we have mod => ans = (ans * base) % m
base = (base * base); // base = (base * base) % m
power >>= 1;
}
return ans;
}
//in RECURSIVE style
int fast_pow(int x,int n) {
if(n==0) return 1;
//n is even
else if(n%2 == 0)
return fast_pow(x*x,n/2);
//n is odd
else
return x * fast_pow(x*x,(n-1)/2);
}
void printDivisors(int n)
{
// Note that this loop runs till square root
for (int i=1; i<=sqrt(n); i++)
{
if (n%i == 0)
{
// If divisors are equal, print only one(case of n being complete square)
if (n/i == i)
cout <<" "<< i;
else // Otherwise print both
cout << " "<< i << " " << n/i;
}
}
}
gcd <data_type> (x,y);
__gcd(x,y);
int lcm = (x * y) / gcd(x,y);
--- > ## log for counting number of bits or digits: ```cpp int n = 124123123; int no_bits = floor( log2(n) ) + 1; // gets no of bits
int no_dig = floor( log10(n) ) + 1; // get no of digits
---
> ## Mod properties:
( a + b) % c = ( ( a % c ) + ( b % c ) ) % c
( a * b) % c = ( ( a % c ) * ( b % c ) ) % c
( a – b) % c = ( ( a % c ) – ( b % c ) ) % c
---
> ## nPr , nCr:
* ### in nPr ( any re-order of same choosen elements increases all nPr count )
* ### nCr is alwyas less than nPr (diffrent arrangement of same choosen elements does not increase nCr counter more than once for same elements)
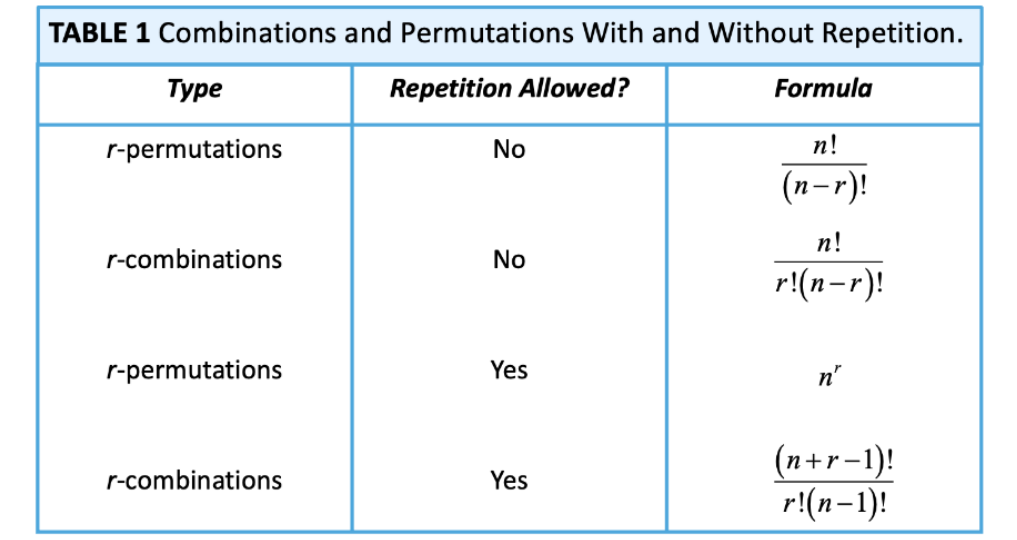
---
> ## next_permutation(start,end)
array must be sorted inc. to get all permutations count and arrangment (summary: to get all permutaion start from smallest arrangement in lexical order)
---
> ## Problem constraints Hints:
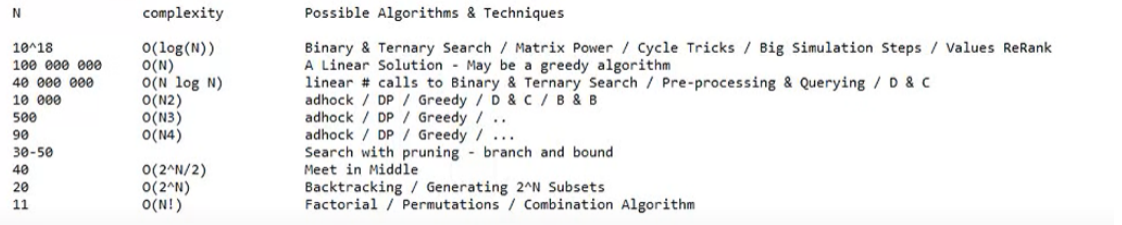
---
> ## Prime factorization (to get all prime factors of a number)
```cpp
vector<int> v;
vector<int> prime_factors(int n){
while ( (n & 1) == 0 ){//if even note: '==' has higher precedence than '&'
v.push_back(2);
n >>= 1;
}
// n must be odd at this point. So we can skip
// one element (Note i = i +2)
for(int i = 3; i <= n / i; i += 2){
// While i divides n, print i and divide n
while (n % i == 0){
v.push_back(i);
n /= i;
}
}
// This condition is to handle the case when n
// is a prime number greater than 2
if (n > 2) v.push_back(n);
return v;
}
scanf("%d", &testInteger);
printf("Number = %d", testInteger); //%f for float %lf for double %c for char
//control floating point
//9dig before the point + the point then 6dig after the point) -> 123456789.123456
printf("%10.6lf",myDoubleNum);
// x = (-b ± sqrt(b^2 - 4ac) ) / 2a;
float a, b, c, x1, x2, discriminant, realPart, imaginaryPart;
cin >> a >> b >> c;
discriminant = b*b - 4*a*c;
if (discriminant > 0) {
x1 = (-b + sqrt(discriminant)) / (2*a);
x2 = (-b - sqrt(discriminant)) / (2*a);
}
else if (discriminant == 0) {
x1 = -b/(2*a);
}
else {
realPart = -b/(2*a);
imaginaryPart =sqrt(-discriminant)/(2*a);
}
Standard C++ header Equivalent in previous versions <iostream><limits><utility><cmath><ctype><algorithm><bitset><deque><iterator><list><map><queue><set><stack><unordered_map><unordered set><vector>
-
(note1: container member functions (note: element with index 0 is the RMB) (note2 : bistset can be initialized using string of bits "binary string" ):
bitset<size> variable_name("BINARY_STRING");
Bit access
- operator[]
- Access bit (public member function)
- count
- Count bits set (public member function)
- size
- Return size (public member function)
- test
- Return bit value (public member function)
- any
- Test if any bit is set (public member function)
- none
- Test if no bit is set (public member function)
- all
- Test if all bits are set (public member function)
Bit operations
- set
- Set bits (public member function)
- reset
- Reset bits (public member function)
- flip
- Flip bits (public member function)
Bitset operations
- to_string
- Convert to string (public member function)
- to_ulong
- Convert to unsigned long integer (public member function)
- to_ullong
- Convert to unsigned long long (public member function)
-
Iterators:
- begin
- Return iterator to beginning (public member function)
- end
- Return iterator to end (public member function)
- rbegin
- Return reverse iterator to reverse beginning (public member function)
- rend
- Return reverse iterator to reverse end (public member function)
- size
- Return size (public member function)
- max_size
- Return maximum no. of elements vector can ever hold (effected by machine and ram) (public member function)
- resize
- Change size (public member function)
- capacity
- Return size of allocated storage capacity (public member function)
- empty
- Test whether vector is empty (public member function)
- reserve
- Request a change in capacity (public member function)
- shrink_to_fit
- Shrink to fit (public member function)
Element access:
- operator[]
- Access element (public member function)
- at
- Access element (public member function)
- front
- Access first element (public member function)
- back
- Access last element (public member function)
- data
- Access data (public member function)
Modifiers:
- assign
- Assign vector content (public member function)
- push_back
- Add element at the end (public member function)
- pop_back
- Delete last element (public member function)
- insert
- Insert elements (public member function)
- erase
- Erase elements (public member function)
- swap
- Swap content (public member function)
- clear
- Clear content (public member function)
- emplace
- Construct and insert element (public member function)
- emplace_back
- Construct and insert element at the end (public member function)
-
- empty
- Test whether container is empty (public member function)
- size
- Return size (public member function)
- top
- Access next element (public member function)
- push
- Insert element (public member function)
- emplace
- Construct and insert element (public member function)
- pop
- Remove top element (public member function)
- swap
- Swap contents (public member function)
Non-member function overloads
- relational operators
- Relational operators for stack (function)
- swap (stack)
- Exchange contents of stacks (public member function)
-
- empty
- Test whether container is empty (public member function)
- size
- Return size (public member function)
- front
- Access next element (public member function)
- back
- Access last element (public member function)
- push
- Insert element (public member function)
- emplace
- Construct and insert element (public member function)
- pop
- Remove next element (public member function)
- swap
- Swap contents (public member function)
Non-member function overloads
- relational operators
- Relational operators for queue (function)
- swap (queue)
- Exchange contents of queues (public member function)
-
Iterators:
- begin
- Return iterator to beginning (public member function)
- end
- Return iterator to end (public member function)
- rbegin
- Return reverse iterator to reverse beginning (public member function)
- rend
- Return reverse iterator to reverse end (public member function)
- cbegin
- Return const_iterator to beginning (public member function)
- cend
- Return const_iterator to end (public member function)
- crbegin
- Return const_reverse_iterator to reverse beginning (public member function)
- crend
- Return const_reverse_iterator to reverse end (public member function)
Capacity:
- size
- Return size (public member function)
- max_size
- Return maximum size (public member function)
- resize
- Change size (public member function)
- empty
- Test whether container is empty (public member function)
- shrink_to_fit
- Shrink to fit (public member function)
Element access:
- operator[]
- Access element (public member function)
- at
- Access element (public member function)
- front
- Access first element (public member function)
- back
- Access last element (public member function)
Modifiers:
- assign
- Assign container content (public member function)
- push_back
- Add element at the end (public member function)
- push_front
- Insert element at beginning (public member function)
- pop_back
- Delete last element (public member function)
- pop_front
- Delete first element (public member function)
- insert
- Insert elements (public member function)
- erase
- Erase elements (public member function)
- swap
- Swap content (public member function)
- clear
- Clear content (public member function)
- emplace
- Construct and insert element (public member function)
- emplace_front
- Construct and insert element at beginning (public member function)
- emplace_back
- Construct and insert element at the end (public member function)
Non-member functions overloads
- relational operators
- Relational operators for deque (function)
- swap
- Exchanges the contents of two deque containers (function template)
-
- empty
- Test whether container is empty (public member function)
- size
- Return size (public member function)
- top
- Access top element (public member function)
- push
- Insert element (public member function)
- emplace
- Construct and insert element (public member function)
- pop
- Remove top element (public member function)
- swap
- Swap contents (public member function)
Non-member function overloads
- swap (queue)
- Exchange contents of priority queues (public member function)
-
Map ( multimap , unordered_map , unordered_multimap )ordered search : O(log n) unordered search: O(1)
Iterators:
- begin
- Return iterator to beginning (public member function)
- end
- Return iterator to end (public member function)
- rbegin
- Return reverse iterator to reverse beginning (public member function)
- rend
- Return reverse iterator to reverse end (public member function)
- cbegin
- Return const_iterator to beginning (public member function)
- cend
- Return const_iterator to end (public member function)
- crbegin
- Return const_reverse_iterator to reverse beginning (public member function)
- crend
- Return const_reverse_iterator to reverse end (public member function)
Capacity:
- empty
- Test whether container is empty (public member function)
- size
- Return container size (public member function)
- max_size
- Return maximum size (public member function)
Element access:
- operator[]
- Access element (public member function)
- at
- Access element (public member function)
Modifiers:
- insert
- Insert elements (public member function)
- erase
- Erase elements (public member function)
- swap
- Swap content (public member function)
- clear
- Clear content (public member function)
- emplace
- Construct and insert element (public member function)
- emplace_hint
- Construct and insert element with hint (public member function)
Operations:
- find
- Get iterator to element (public member function)
- count
- Count elements with a specific key (public member function)
- lower_bound
- Return iterator to lower bound (public member function)
- upper_bound
- Return iterator to upper bound (public member function)
- equal_range
- Get range of equal elements (public member function)
-
Set ( multiset , unordered_set , unordered_multiset ) ordered search : O(log n) unordered search: O(1)
Iterators:
- begin
- Return iterator to beginning (public member function)
- end
- Return iterator to end (public member function)
- rbegin
- Return reverse iterator to reverse beginning (public member function)
- rend
- Return reverse iterator to reverse end (public member function)
- cbegin
- Return const_iterator to beginning (public member function)
- cend
- Return const_iterator to end (public member function)
- crbegin
- Return const_reverse_iterator to reverse beginning (public member function)
- crend
- Return const_reverse_iterator to reverse end (public member function)
Capacity:
- empty
- Test whether container is empty (public member function)
- size
- Return container size (public member function)
- max_size
- Return maximum size (public member function)
Modifiers:
- insert
- Insert element (public member function)
- erase
- Erase elements (public member function)
- swap
- Swap content (public member function)
- clear
- Clear content (public member function)
- emplace
- Construct and insert element (public member function)
- emplace_hint
- Construct and insert element with hint (public member function)
Operations:
- find
- Get iterator to element (public member function)
- count
- Count elements with a specific value (public member function)
- lower_bound
- Return iterator to lower bound (public member function)
- upper_bound
- Return iterator to upper bound (public member function)
- equal_range
- Get range of equal elements (public member function)
int N = 1e6 + 5;
vector<bool> is_prime(N, true);
void sieve(ll sz){
is_prime[0] = is_prime[1] = false; //not primes ( 1 is rarely considered prime )
for (int i = 2; i * i <= n; i++) { // or 'i <= i/n' if 'i' could OF
if (is_prime[i]) {
for (int j = i * i; j <= n; j += i)
is_prime[j] = false;
}
}
}
may have accuracy and approximation issues at very big or small numbers (hard to predict when it will be off by +1 or -1 so you can handle its errors)
to compile-run : g++ main.cpp -o main.exe && main.exe
or clang++ main.cpp -o main.exe && main.exe
// #include <stdio.h>
#include <bits/stdc++.h>
using namespace std;
#define Txtio freopen("input.txt","r",stdin); freopen("output.txt","w",stdout);
#define fastio \
ios_base::sync_with_stdio(false); \
cin.tie(nullptr); \
cout.tie(nullptr);
#define F first
#define S second
using ll = long long;
using ull = unsigned long long;
const ll LM = LONG_LONG_MAX;
const int N = 2e6 + 5, M = INT32_MAX;
// int arr[N];
int main(void) {
// Txtio;
fastio; // disable with 'printf() , scanf()'
int t = 1;
// cin >> t;
while (t--) {
}
return 0;
}
- Code:
int arr[N+1][M+1]={0}, prefixSum[N+1][M+1];
int n,m;
cin>>n>>m;
for(int i=1; i<=n; i++)
for(int j=1; j<=m; j++)
cin>>arr[i][j];
for(int i=1; i<=n; i++)
for(int j=1; j<=m; j++)
prefixSum[i][j]=arr[i][j] + prefixSum[i-1][j] + prefixSum[i][j-1] - prefixSum[i-1][j-1];
int r1,c1, r2,c2;
cin>>r1>>c1>>r2>>c2; // one-based
cout<<prefixSum[r2][c2] - prefixSum[r1-1][c2] - prefixSum[r2][c1-1] + prefixSum[r1-1][c1-1]<<'\n';
- after constructing final Commulative array :
Char | Number | Description |
---|---|---|
0 - 31 | Control characters (see below) | |
32 | space | |
! | 33 | exclamation mark |
" | 34 | quotation mark |
# | 35 | number sign |
$ | 36 | dollar sign |
% | 37 | percent sign |
& | 38 | ampersand |
' | 39 | apostrophe |
( | 40 | left parenthesis |
) | 41 | right parenthesis |
* | 42 | asterisk |
+ | 43 | plus sign |
, | 44 | comma |
- | 45 | hyphen |
. | 46 | period |
/ | 47 | slash |
0 | 48 | digit 0 |
1 | 49 | digit 1 |
2 | 50 | digit 2 |
3 | 51 | digit 3 |
4 | 52 | digit 4 |
5 | 53 | digit 5 |
6 | 54 | digit 6 |
7 | 55 | digit 7 |
8 | 56 | digit 8 |
9 | 57 | digit 9 |
: | 58 | colon |
; | 59 | semicolon |
< | 60 | less-than |
= | 61 | equals-to |
> | 62 | greater-than |
? | 63 | question mark |
@ | 64 | at sign |
A | 65 | uppercase A |
B | 66 | uppercase B |
C | 67 | uppercase C |
D | 68 | uppercase D |
E | 69 | uppercase E |
F | 70 | uppercase F |
G | 71 | uppercase G |
H | 72 | uppercase H |
I | 73 | uppercase I |
J | 74 | uppercase J |
K | 75 | uppercase K |
L | 76 | uppercase L |
M | 77 | uppercase M |
N | 78 | uppercase N |
O | 79 | uppercase O |
P | 80 | uppercase P |
Q | 81 | uppercase Q |
R | 82 | uppercase R |
S | 83 | uppercase S |
T | 84 | uppercase T |
U | 85 | uppercase U |
V | 86 | uppercase V |
W | 87 | uppercase W |
X | 88 | uppercase X |
Y | 89 | uppercase Y |
Z | 90 | uppercase Z |
[ | 91 | left square bracket |
\ | 92 | backslash |
] | 93 | right square bracket |
^ | 94 | caret |
_ | 95 | underscore |
` | 96 | grave accent |
a | 97 | lowercase a |
b | 98 | lowercase b |
c | 99 | lowercase c |
d | 100 | lowercase d> |
e | 101 | lowercase e> |
f | 102 | lowercase f> |
g | 103 | lowercase g> |
h | 104 | lowercase h> |
i | 105 | lowercase i> |
j | 106 | lowercase j> |
k | 107 | lowercase k> |
l | 108 | lowercase l> |
m | 109 | lowercase m> |
n | 110 | lowercase n> |
o | 111 | lowercase o> |
p | 112 | lowercase p> |
q | 113 | lowercase q> |
r | 114 | lowercase r> |
s | 115 | lowercase s> |
t | 116 | lowercase t> |
u | 117 | lowercase u> |
v | 118 | lowercase v> |
w | 119 | lowercase w> |
x | 120 | lowercase x> |
y | 121 | lowercase y> |
z | 122 | lowercase z> |
{ | 123 | left curly brace> |
| | 124 | vertical bar> |
} | 125 | right curly brace> |
~ | 126 | tilde> |
No. | Binary Number |
---|---|
101 | 1100101 |
102 | 1100110 |
103 | 1100111 |
104 | 1101000 |
105 | 1101001 |
106 | 1101010 |
107 | 1101011 |
108 | 1101100 |
109 | 1101101 |
110 | 1101110 |
111 | 1101111 |
112 | 1110000 |
113 | 1110001 |
114 | 1110010 |
115 | 1110011 |
116 | 1110100 |
117 | 1110101 |
118 | 1110110 |
119 | 1110111 |
120 | 1111000 |
121 | 1111001 |
122 | 1111010 |
123 | 1111011 |
124 | 1111100 |
125 | 1111101 |
126 | 1111110 |
127 | 1111111 |
128 | 10000000 |
129 | 10000001 |
130 | 10000010 |
131 | 10000011 |
132 | 10000100 |
133 | 10000101 |
134 | 10000110 |
135 | 10000111 |
136 | 10001000 |
137 | 10001001 |
138 | 10001010 |
139 | 10001011 |
140 | 10001100 |
141 | 10001101 |
142 | 10001110 |
143 | 10001111 |
144 | 10010000 |
145 | 10010001 |
146 | 10010010 |
147 | 10010011 |
148 | 10010100 |
149 | 10010101 |
150 | 10010110 |
151 | 10010111 |
152 | 10011000 |
153 | 10011001 |
154 | 10011010 |
155 | 10011011 |
156 | 10011100 |
157 | 10011101 |
158 | 10011110 |
159 | 10011111 |
160 | 10100000 |
161 | 10100001 |
162 | 10100010 |
163 | 10100011 |
164 | 10100100 |
165 | 10100101 |
166 | 10100110 |
167 | 10100111 |
168 | 10101000 |
169 | 10101001 |
170 | 10101010 |
171 | 10101011 |
172 | 10101100 |
173 | 10101101 |
174 | 10101110 |
175 | 10101111 |
176 | 10110000 |
177 | 10110001 |
178 | 10110010 |
179 | 10110011 |
180 | 10110100 |
181 | 10110101 |
182 | 10110110 |
183 | 10110111 |
184 | 10111000 |
185 | 10111001 |
186 | 10111010 |
187 | 10111011 |
188 | 10111100 |
189 | 10111101 |
190 | 10111110 |
191 | 10111111 |
192 | 11000000 |
193 | 11000001 |
194 | 11000010 |
195 | 11000011 |
196 | 11000100 |
197 | 11000101 |
198 | 11000110 |
199 | 11000111 |
200 | 11001000 |
201 | 11001001 |
202 | 11001010 |
203 | 11001011 |
204 | 11001100 |
205 | 11001101 |
206 | 11001110 |
207 | 11001111 |
208 | 11010000 |
209 | 11010001 |
210 | 11010010 |
211 | 11010011 |
212 | 11010100 |
213 | 11010101 |
214 | 11010110 |
215 | 11010111 |
216 | 11011000 |
217 | 11011001 |
218 | 11011010 |
219 | 11011011 |
220 | 11011100 |
221 | 11011101 |
222 | 11011110 |
223 | 11011111 |
224 | 11100000 |
225 | 11100001 |
226 | 11100010 |
227 | 11100011 |
228 | 11100100 |
229 | 11100101 |
230 | 11100110 |
231 | 11100111 |
232 | 11101000 |
233 | 11101001 |
234 | 11101010 |
235 | 11101011 |
236 | 11101100 |
237 | 11101101 |
238 | 11101110 |
239 | 11101111 |
240 | 11110000 |
241 | 11110001 |
242 | 11110010 |
243 | 11110011 |
244 | 11110100 |
245 | 11110101 |
246 | 11110110 |
247 | 11110111 |
248 | 11111000 |
249 | 11111001 |
250 | 11111010 |
251 | 11111011 |
252 | 11111100 |
253 | 11111101 |
254 | 11111110 |
255 | 11111111 |
256 | 100000000 |
(use `sizeof(var)` to know exact size in your machine in bytes)
Type | Typical Bit Width | Typical Range |
---|---|---|
char | 1byte | -127 to 127 or 0 to 255 |
unsigned char | 1byte | 0 to 255 |
signed char | 1byte | -127 to 127 |
int | 4bytes | -2147483648 to 2147483647 [~10e9] |
unsigned int | 4bytes | 0 to 4294967295 |
signed int | 4bytes | -2147483648 to 2147483647 |
short int | 2bytes | -32768 to 32767 |
unsigned short int | 2bytes | 0 to 65,535 |
signed short int | 2bytes | -32768 to 32767 |
long int | 8bytes | -9223372036854775808 to 9223372036854775807 |
signed long int | 8bytes | same as long int |
unsigned long int | 8bytes | 0 to 18446744073709551615 |
long long int | 8bytes | -(2^63) to (2^63)-1 [~10e19] |
unsigned long long int | 8bytes | 0 to 18,446,744,073,709,551,615 |
float | 4bytes | |
double | 8bytes | |
long double | 12bytes | |
wchar_t | 2 or 4 bytes | 1 wide character |