Sample JSON files
$ python webhook_event_logger.py -R pdxjohnny/scitt-api-emulator
import os | |
import abc | |
import sys | |
import pdb | |
import enum | |
import uuid | |
import json | |
import pprint | |
import asyncio | |
import getpass | |
import pathlib | |
import argparse | |
import traceback | |
import contextlib | |
import collections | |
import dataclasses | |
from typing import Any, List, Optional, NewType, AsyncIterator | |
import openai | |
import keyring | |
import logging | |
logger = logging.getLogger(__name__) | |
def async_lambda(func): | |
async def async_func(): | |
nonlocal func | |
return func() | |
return async_func | |
async def asyncio_sleep_for_then_coro(sleep_time, coro): | |
await asyncio.sleep(sleep_time) | |
return await coro | |
class AGIEventType(enum.Enum): | |
ERROR = enum.auto() | |
END_EVENTS = enum.auto() | |
INTERNAL_RE_QUEUE = enum.auto() | |
NEW_AGENT_CREATED = enum.auto() | |
EXISTING_AGENT_RETRIEVED = enum.auto() | |
NEW_FILE_ADDED = enum.auto() | |
NEW_THREAD_CREATED = enum.auto() | |
NEW_THREAD_RUN_CREATED = enum.auto() | |
NEW_THREAD_MESSAGE = enum.auto() | |
THREAD_MESSAGE_ADDED = enum.auto() | |
THREAD_RUN_COMPLETE = enum.auto() | |
THREAD_RUN_IN_PROGRESS = enum.auto() | |
THREAD_RUN_FAILED = enum.auto() | |
THREAD_RUN_EVENT_WITH_UNKNOWN_STATUS = enum.auto() | |
@dataclasses.dataclass | |
class AGIEvent: | |
event_type: AGIEventType | |
event_data: Any | |
@dataclasses.dataclass | |
class AGIEventNewAgent: | |
agent_id: str | |
agent_name: str | |
@dataclasses.dataclass | |
class AGIEventNewFileAdded: | |
agent_id: str | |
file_id: str | |
@dataclasses.dataclass | |
class AGIEventNewThreadCreated: | |
agent_id: str | |
thread_id: str | |
@dataclasses.dataclass | |
class AGIEventNewThreadRunCreated: | |
agent_id: str | |
thread_id: str | |
run_id: str | |
run_status: str | |
@dataclasses.dataclass | |
class AGIEventThreadRunComplete: | |
agent_id: str | |
thread_id: str | |
run_id: str | |
run_status: str | |
@dataclasses.dataclass | |
class AGIEventThreadRunInProgress: | |
agent_id: str | |
thread_id: str | |
run_id: str | |
run_status: str | |
@dataclasses.dataclass | |
class AGIEventThreadRunFailed: | |
agent_id: str | |
thread_id: str | |
run_id: str | |
run_status: str | |
@dataclasses.dataclass | |
class AGIEventThreadRunEventWithUnknwonStatus(AGIEventNewThreadCreated): | |
agent_id: str | |
thread_id: str | |
run_id: str | |
status: str | |
@dataclasses.dataclass | |
class AGIEventNewThreadMessage: | |
agent_id: str | |
thread_id: str | |
message_role: str | |
message_content_type: str | |
message_content: str | |
@dataclasses.dataclass | |
class AGIEventThreadMessageAdded: | |
agent_id: str | |
thread_id: str | |
message_id: str | |
message_role: str | |
message_content: str | |
class AGIActionType(enum.Enum): | |
NEW_AGENT = enum.auto() | |
INGEST_FILE = enum.auto() | |
ADD_MESSAGE = enum.auto() | |
NEW_THREAD = enum.auto() | |
RUN_THREAD = enum.auto() | |
@dataclasses.dataclass | |
class AGIAction: | |
action_type: AGIActionType | |
action_data: Any | |
@dataclasses.dataclass | |
class AGIActionIngestFile: | |
agent_id: str | |
file_path: str | |
@dataclasses.dataclass | |
class AGIActionNewAgent: | |
agent_id: str | |
agent_name: str | |
agent_instructions: str | |
@dataclasses.dataclass | |
class AGIActionNewThread: | |
agent_id: str | |
@dataclasses.dataclass | |
class AGIActionAddMessage: | |
agent_id: str | |
thread_id: str | |
message_role: str | |
message_content: str | |
@dataclasses.dataclass | |
class AGIActionRunThread: | |
agent_id: str | |
thread_id: str | |
AGIActionStream = NewType("AGIActionStream", AsyncIterator[AGIAction]) | |
class AGIStateType(enum.Enum): | |
AGENT = enum.auto() | |
THREAD = enum.auto() | |
@dataclasses.dataclass | |
class AGIState: | |
state_type: AGIStateType | |
state_data: Any | |
@dataclasses.dataclass | |
class AGIStateAgent: | |
agent_name: str | |
agent_id: str | |
thread_ids: List[str] = dataclasses.field( | |
default_factory=lambda: [], | |
) | |
@dataclasses.dataclass | |
class AGIStateThread: | |
agent_id: str | |
thread_id: str | |
most_recent_run_id: Optional[str] = None | |
most_recent_run_status: Optional[str] = None | |
class _KV_STORE_DEFAULT_VALUE: | |
pass | |
KV_STORE_DEFAULT_VALUE = _KV_STORE_DEFAULT_VALUE() | |
class KVStore(abc.ABC): | |
@abc.abstractmethod | |
async def get(self, key, default_value: Any = KV_STORE_DEFAULT_VALUE): | |
raise NotImplementedError() | |
@abc.abstractmethod | |
async def set(self, key, value): | |
raise NotImplementedError() | |
async def __aenter__(self): | |
return self | |
async def __aexit__(self, _exc_type, _exc_value, _traceback): | |
return | |
class KVStoreKeyring(KVStore): | |
def __init__(self, config): | |
self.service_name = config["service_name"] | |
async def get(self, key, default_value: Any = KV_STORE_DEFAULT_VALUE): | |
if default_value is not KV_STORE_DEFAULT_VALUE: | |
return self.keyring_get_password_or_return( | |
self.service_name, | |
key, | |
not_found_return_value=default_value, | |
) | |
return keyring.get_password(self.service_name, key) | |
async def set(self, key, value): | |
return keyring.set_password(self.service_name, key, value) | |
@staticmethod | |
def keyring_get_password_or_return( | |
service_name: str, | |
username: str, | |
*, | |
not_found_return_value=None, | |
) -> str: | |
with contextlib.suppress(Exception): | |
value = keyring.get_password(service_name, username) | |
if value is not None: | |
return value | |
return not_found_return_value | |
def make_argparse_parser(argv=None): | |
parser = argparse.ArgumentParser(description="Generic AI") | |
parser.add_argument( | |
"--user-name", | |
dest="user_name", | |
type=str, | |
default=KVStoreKeyring.keyring_get_password_or_return( | |
getpass.getuser(), | |
"profile.username", | |
not_found_return_value=getpass.getuser(), | |
), | |
help="Handle to address the user as", | |
) | |
parser.add_argument( | |
"--agi-name", | |
dest="agi_name", | |
default="alice", | |
type=str, | |
) | |
parser.add_argument( | |
"--kvstore-service-name", | |
dest="kvstore_service_name", | |
default="alice", | |
type=str, | |
) | |
parser.add_argument( | |
"--openai-api-key", | |
dest="openai_api_key", | |
type=str, | |
default=KVStoreKeyring.keyring_get_password_or_return( | |
getpass.getuser(), | |
"openai.api.key", | |
), | |
help="OpenAI API Key", | |
) | |
parser.add_argument( | |
"--openai-base-url", | |
dest="openai_base_url", | |
type=str, | |
default=KVStoreKeyring.keyring_get_password_or_return( | |
getpass.getuser(), | |
"openai.api.base_url", | |
), | |
help="OpenAI API Key", | |
) | |
return parser | |
import inspect | |
import asyncio | |
from collections import UserList | |
from contextlib import AsyncExitStack | |
from typing import ( | |
Dict, | |
Any, | |
AsyncIterator, | |
Tuple, | |
Type, | |
AsyncContextManager, | |
Optional, | |
Set, | |
) | |
class _STOP_ASYNC_ITERATION: | |
pass | |
STOP_ASYNC_ITERATION = _STOP_ASYNC_ITERATION() | |
async def ignore_stopasynciteration(coro): | |
try: | |
return await coro | |
except StopAsyncIteration: | |
return STOP_ASYNC_ITERATION | |
async def concurrently( | |
work: Dict[asyncio.Task, Any], | |
*, | |
errors: str = "strict", | |
nocancel: Optional[Set[asyncio.Task]] = None, | |
) -> AsyncIterator[Tuple[Any, Any]]: | |
# Track if first run | |
first = True | |
# Set of tasks we are waiting on | |
tasks = set(work.keys()) | |
# Return when outstanding operations reaches zero | |
try: | |
while first or tasks: | |
first = False | |
# Wait for incoming events | |
done, _pending = await asyncio.wait( | |
tasks, return_when=asyncio.FIRST_COMPLETED | |
) | |
for task in done: | |
# Remove the task from the set of tasks we are waiting for | |
tasks.remove(task) | |
# Get the tasks exception if any | |
exception = task.exception() | |
if errors == "strict" and exception is not None: | |
raise exception | |
if exception is None: | |
# Remove the compeleted task from work | |
complete = work[task] | |
del work[task] | |
yield complete, task.result() | |
# Update tasks in case work has been updated by called | |
tasks = set(work.keys()) | |
finally: | |
for task in tasks: | |
if not task.done() and (nocancel is None or task not in nocancel): | |
task.cancel() | |
else: | |
# For tasks which are done but have exceptions which we didn't | |
# raise, collect their exceptions | |
task.exception() | |
async def agent_openai( | |
tg: asyncio.TaskGroup, | |
agi_name: str, | |
kvstore: KVStore, | |
action_stream: AGIActionStream, | |
openai_api_key: str, | |
*, | |
openai_base_url: Optional[str] = None, | |
): | |
client = openai.AsyncOpenAI( | |
api_key=openai_api_key, | |
base_url=openai_base_url, | |
) | |
agents = {} | |
threads = {} | |
action_stream_iter = action_stream.__aiter__() | |
work = { | |
tg.create_task( | |
ignore_stopasynciteration(action_stream_iter.__anext__()) | |
): ( | |
"action_stream", | |
action_stream_iter, | |
), | |
} | |
async for (work_name, work_ctx), result in concurrently(work): | |
logger.debug(f"openai_agent.{work_name}: %s", pprint.pformat(result)) | |
if result is STOP_ASYNC_ITERATION: | |
continue | |
try: | |
# TODO There should be no await's here, always add to work | |
if work_name == "action_stream": | |
work[ | |
tg.create_task( | |
ignore_stopasynciteration(work_ctx.__anext__()) | |
) | |
] = (work_name, work_ctx) | |
if result.action_type == AGIActionType.NEW_AGENT: | |
assistant = None | |
if result.action_data.agent_id: | |
with contextlib.suppress(openai.NotFoundError): | |
assistant = await client.beta.assistants.retrieve( | |
assistant_id=result.action_data.agent_id, | |
) | |
yield AGIEvent( | |
event_type=AGIEventType.EXISTING_AGENT_RETRIEVED, | |
event_data=AGIEventNewAgent( | |
agent_id=assistant.id, | |
agent_name=result.action_data.agent_name, | |
), | |
) | |
if not assistant: | |
assistant = await client.beta.assistants.create( | |
name=result.action_data.agent_name, | |
instructions=result.action_data.agent_instructions, | |
# model="gpt-4-1106-preview", | |
model=await kvstore.get( | |
f"openai.assistants.{agi_name}.model", | |
"gpt-3.5-turbo-1106", | |
), | |
tools=[{"type": "retrieval"}], | |
# file_ids=[file.id], | |
) | |
yield AGIEvent( | |
event_type=AGIEventType.NEW_AGENT_CREATED, | |
event_data=AGIEventNewAgent( | |
agent_id=assistant.id, | |
agent_name=result.action_data.agent_name, | |
), | |
) | |
agents[assistant.id] = assistant | |
elif result.action_type == AGIActionType.INGEST_FILE: | |
# TODO aiofile and tg.create_task | |
with open(result.action_data.file_path, "rb") as fileobj: | |
file = await client.files.create( | |
file=fileobj, | |
purpose="assistants", | |
) | |
file_ids = agents[result.action_data.agent_id].file_ids + [ | |
file.id | |
] | |
""" | |
non_existant_file_ids = [] | |
for file_id in file_ids: | |
try: | |
file = await openai.resources.beta.assistants.AsyncFiles( | |
client | |
).retrieve( | |
file_id, | |
assistant_id=result.action_data.agent_id, | |
) | |
except openai.NotFoundError: | |
non_existant_file_ids.append(file_id) | |
for file_id in non_existant_file_ids: | |
file_ids.remove(file_id) | |
""" | |
assistant = ( | |
await openai.resources.beta.assistants.AsyncAssistants( | |
client | |
).update( | |
assistant_id=result.action_data.agent_id, | |
file_ids=file_ids, | |
) | |
) | |
logger.debug( | |
"AsyncAssistants.update(): %s", | |
pprint.pformat(assistant), | |
) | |
yield AGIEvent( | |
event_type=AGIEventType.NEW_FILE_ADDED, | |
event_data=AGIEventNewFileAdded( | |
agent_id=result.action_data.agent_id, | |
file_id=file.id, | |
), | |
) | |
elif result.action_type == AGIActionType.NEW_THREAD: | |
thread = await client.beta.threads.create() | |
yield AGIEvent( | |
event_type=AGIEventType.NEW_THREAD_CREATED, | |
event_data=AGIEventNewThreadCreated( | |
agent_id=result.action_data.agent_id, | |
thread_id=thread.id, | |
), | |
) | |
elif result.action_type == AGIActionType.RUN_THREAD: | |
run = await client.beta.threads.runs.create( | |
assistant_id=result.action_data.agent_id, | |
thread_id=result.action_data.thread_id, | |
) | |
yield AGIEvent( | |
event_type=AGIEventType.NEW_THREAD_RUN_CREATED, | |
event_data=AGIEventNewThreadRunCreated( | |
agent_id=result.action_data.agent_id, | |
thread_id=result.action_data.thread_id, | |
run_id=run.id, | |
run_status=run.status, | |
), | |
) | |
work[ | |
tg.create_task( | |
client.beta.threads.runs.retrieve( | |
thread_id=run.thread_id, run_id=run.id | |
) | |
) | |
] = ( | |
f"thread.runs.{run.id}", | |
(result, run), | |
) | |
elif result.action_type == AGIActionType.ADD_MESSAGE: | |
message = await client.beta.threads.messages.create( | |
thread_id=result.action_data.thread_id, | |
role=result.action_data.message_role, | |
content=result.action_data.message_content, | |
) | |
yield AGIEvent( | |
event_type=AGIEventType.THREAD_MESSAGE_ADDED, | |
event_data=AGIEventThreadMessageAdded( | |
agent_id=result.action_data.agent_id, | |
thread_id=result.action_data.thread_id, | |
message_id=message.id, | |
message_role=result.action_data.message_role, | |
message_content=result.action_data.message_content, | |
), | |
) | |
elif work_name.startswith("thread.runs."): | |
action_new_thread_run, _old_run = work_ctx | |
if result.status == "completed": | |
yield AGIEvent( | |
event_type=AGIEventType.THREAD_RUN_COMPLETE, | |
event_data=AGIEventThreadRunComplete( | |
agent_id=action_new_thread_run.action_data.agent_id, | |
thread_id=result.thread_id, | |
run_id=result.id, | |
run_status=result.status, | |
), | |
) | |
# TODO Send this similar to seed back to a feedback queue to | |
# process as an action for get thread messages | |
thread_messages = client.beta.threads.messages.list( | |
thread_id=result.thread_id, | |
) | |
thread_messages_iter = thread_messages.__aiter__() | |
work[ | |
tg.create_task( | |
ignore_stopasynciteration( | |
thread_messages_iter.__anext__() | |
) | |
) | |
] = ( | |
f"thread.messages.{result.thread_id}", | |
(action_new_thread_run, thread_messages_iter), | |
) | |
elif result.status in ("queued", "in_progress"): | |
yield AGIEvent( | |
event_type=AGIEventType.THREAD_RUN_IN_PROGRESS, | |
event_data=AGIEventThreadRunInProgress( | |
agent_id=action_new_thread_run.action_data.agent_id, | |
thread_id=result.thread_id, | |
run_id=result.id, | |
run_status=result.status, | |
), | |
) | |
work[ | |
tg.create_task( | |
asyncio_sleep_for_then_coro( | |
5, | |
client.beta.threads.runs.retrieve( | |
thread_id=result.thread_id, run_id=result.id | |
), | |
) | |
) | |
] = ( | |
f"thread.runs.{run.id}", | |
(action_new_thread_run, result), | |
) | |
elif result.status == "failed": | |
yield AGIEvent( | |
event_type=AGIEventType.THREAD_RUN_FAILED, | |
event_data=AGIEventThreadRunFailed( | |
agent_id=action_new_thread_run.action_data.agent_id, | |
thread_id=result.thread_id, | |
run_id=result.id, | |
status=result.status, | |
last_error=result.last_error, | |
), | |
) | |
else: | |
yield AGIEvent( | |
event_type=AGIEventType.THREAD_RUN_EVENT_WITH_UNKNOWN_STATUS, | |
event_data=AGIEventThreadRunEventWithUnknwonStatus( | |
agent_id=action_new_thread_run.action_data.agent_id, | |
thread_id=result.thread_id, | |
run_id=result.id, | |
status=result.status, | |
), | |
) | |
elif work_name.startswith("thread.messages."): | |
action_new_thread_run, thread_messages_iter = work_ctx | |
_, _, thread_id = work_name.split(".", maxsplit=3) | |
# The first time we iterate is the most recent response | |
# TODO Keep track of what the last response received was so that | |
# we can create_task as many times as there might be responses | |
# in case there are multiple within one run. | |
work[ | |
tg.create_task( | |
ignore_stopasynciteration( | |
thread_messages_iter.__anext__() | |
) | |
) | |
] = (work_name, work_ctx) | |
for content in result.content: | |
if content.type == "text": | |
yield AGIEvent( | |
event_type=AGIEventType.NEW_THREAD_MESSAGE, | |
event_data=AGIEventNewThreadMessage( | |
agent_id=action_new_thread_run.action_data.agent_id, | |
thread_id=result.thread_id, | |
message_role="agent" | |
if result.role == "assistant" | |
else "user", | |
message_content_type=content.type, | |
message_content=content.text.value, | |
), | |
) | |
except: | |
traceback.print_exc() | |
yield AGIEvent( | |
event_type=AGIEventType.ERROR, | |
event_data=traceback.format_exc(), | |
) | |
yield AGIEvent( | |
event_type=AGIEventType.END_EVENTS, | |
event_data=None, | |
) | |
class _STDIN_CLOSED: | |
pass | |
STDIN_CLOSED = _STDIN_CLOSED() | |
def pdb_action_stream_get_user_input(user_name: str): | |
user_input = "" | |
sys_stdin_iter = sys.stdin.__iter__() | |
try: | |
while not user_input: | |
print(f"{user_name}: ", end="\r") | |
user_input = sys_stdin_iter.__next__().rstrip() | |
except (KeyboardInterrupt, StopIteration): | |
return STDIN_CLOSED | |
return user_input | |
class _CURRENTLY_UNDEFINED: | |
pass | |
CURRENTLY_UNDEFINED = _CURRENTLY_UNDEFINED() | |
class AsyncioLockedCurrentlyDict(collections.UserDict): | |
def __init__(self, *args, **kwargs): | |
super().__init__(*args, **kwargs) | |
self.currently = CURRENTLY_UNDEFINED | |
self.lock = asyncio.Lock() | |
self.currently_exists = asyncio.Event() | |
def __setitem__(self, name, value): | |
super().__setitem__(name, value) | |
self.currently = value | |
self.currently_exists.set() | |
logger.debug("currently: %r", value) | |
async def __aenter__(self): | |
await self.lock.__aenter__() | |
return self | |
async def __aexit__(self, exc_type, exc_value, traceback): | |
await self.lock.__aexit__(exc_type, exc_value, traceback) | |
async def pdb_action_stream(tg, user_name, agents, threads): | |
while True: | |
yield await asyncio.to_thread( | |
pdb_action_stream_get_user_input, user_name | |
) | |
async def main( | |
user_name: str, | |
agi_name: str, | |
kvstore_service_name: str, | |
*, | |
kvstore: KVStore = None, | |
action_stream: AGIActionStream = None, | |
openai_api_key: str = None, | |
openai_base_url: Optional[str] = None, | |
): | |
if not kvstore: | |
kvstore = KVStoreKeyring({"service_name": kvstore_service_name}) | |
kvstore_key_agent_id = f"agents.{agi_name}.id" | |
action_stream_seed = [ | |
AGIAction( | |
action_type=AGIActionType.NEW_AGENT, | |
action_data=AGIActionNewAgent( | |
agent_id=await kvstore.get(kvstore_key_agent_id, None), | |
agent_name=agi_name, | |
agent_instructions=pathlib.Path(__file__) | |
.parent.joinpath("openai_assistant_instructions.md") | |
.read_text(), | |
), | |
), | |
] | |
agents = AsyncioLockedCurrentlyDict() | |
threads = AsyncioLockedCurrentlyDict() | |
async with kvstore, asyncio.TaskGroup() as tg: | |
if not action_stream: | |
unvalidated_user_input_action_stream = pdb_action_stream( | |
tg, | |
user_name, | |
agents, | |
threads, | |
) | |
user_input_action_stream_queue = asyncio.Queue() | |
async def user_input_action_stream_queue_iterator(queue): | |
# TODO Stop condition/asyncio.Event | |
while True: | |
yield await queue.get() | |
action_stream = user_input_action_stream_queue_iterator( | |
user_input_action_stream_queue, | |
) | |
for action in action_stream_seed: | |
await user_input_action_stream_queue.put(action) | |
if openai_api_key: | |
agent_events = agent_openai( | |
tg, | |
agi_name, | |
kvstore, | |
action_stream, | |
openai_api_key, | |
openai_base_url=openai_base_url, | |
) | |
else: | |
raise Exception( | |
"No API keys or implementations of assistants given" | |
) | |
waiting = [] | |
unvalidated_user_input_action_stream_iter = ( | |
unvalidated_user_input_action_stream.__aiter__() | |
) | |
agent_events_iter = agent_events.__aiter__() | |
work = { | |
tg.create_task( | |
ignore_stopasynciteration( | |
unvalidated_user_input_action_stream_iter.__anext__() | |
) | |
): ( | |
"user.unvalidated.input_action_stream", | |
unvalidated_user_input_action_stream_iter, | |
), | |
tg.create_task( | |
ignore_stopasynciteration(agent_events_iter.__anext__()) | |
): ( | |
"agent.events", | |
agent_events_iter, | |
), | |
} | |
async for (work_name, work_ctx), result in concurrently(work): | |
if result is STOP_ASYNC_ITERATION: | |
continue | |
if work_name == "agent.events": | |
work[ | |
tg.create_task( | |
ignore_stopasynciteration(work_ctx.__anext__()) | |
) | |
] = (work_name, work_ctx) | |
agent_event = result | |
logger.debug("agent_event: %s", pprint.pformat(agent_event)) | |
print(f"{user_name}: ", end="\r") | |
if agent_event.event_type in ( | |
AGIEventType.NEW_AGENT_CREATED, | |
AGIEventType.EXISTING_AGENT_RETRIEVED, | |
): | |
await kvstore.set( | |
f"agents.{agent_event.event_data.agent_name}.id", | |
agent_event.event_data.agent_id, | |
) | |
async with agents: | |
agents[agent_event.event_data.agent_id] = AGIState( | |
state_type=AGIStateType.AGENT, | |
state_data=AGIStateAgent( | |
agent_name=agent_event.event_data.agent_name, | |
agent_id=agent_event.event_data.agent_id, | |
), | |
) | |
elif agent_event.event_type == AGIEventType.NEW_THREAD_CREATED: | |
async with threads: | |
threads[agent_event.event_data.thread_id] = AGIState( | |
state_type=AGIStateType.THREAD, | |
state_data=AGIStateThread( | |
agent_id=agent_event.event_data.agent_id, | |
thread_id=agent_event.event_data.thread_id, | |
), | |
) | |
async with agents: | |
""" | |
agents[ | |
agent_event.event_data.agent_id | |
].state_data.thread_ids.append( | |
agent_event.event_data.thread_id | |
) | |
""" | |
logger.debug( | |
"New thread created for agent: %r", | |
agents[agent_event.event_data.agent_id], | |
) | |
elif ( | |
agent_event.event_type | |
== AGIEventType.NEW_THREAD_RUN_CREATED | |
): | |
async with threads: | |
thread_state = threads[agent_event.event_data.thread_id] | |
thread_state.most_recent_run_id = ( | |
agent_event.event_data.run_id | |
) | |
thread_state.most_recent_run_status = ( | |
agent_event.event_data.run_status | |
) | |
logger.debug("New thread run created: %r", thread_state) | |
elif ( | |
agent_event.event_type | |
== AGIEventType.THREAD_RUN_IN_PROGRESS | |
): | |
async with threads: | |
threads[ | |
agent_event.event_data.thread_id | |
].state_data.most_recent_run_status = ( | |
agent_event.event_data.run_status | |
) | |
elif agent_event.event_type == AGIEventType.THREAD_RUN_COMPLETE: | |
async with threads: | |
threads[ | |
agent_event.event_data.thread_id | |
].state_data.most_recent_run_status = ( | |
agent_event.event_data.run_status | |
) | |
logger.debug( | |
"Thread run complete, agent: %r, thread: %r", | |
agents[agent_event.event_data.agent_id], | |
thread_state, | |
) | |
async with agents: | |
""" | |
agents[ | |
agent_event.event_data.agent_id | |
].state_data.thread_ids.remove( | |
agent_event.event_data.thread_id | |
) | |
print(agents[agent_event.event_data.agent_id]) | |
""" | |
pass | |
elif agent_event.event_type == AGIEventType.THREAD_RUN_FAILED: | |
if ( | |
agent_event.event_data.last_error.code | |
== "rate_limit_exceeded" | |
): | |
# TODO Change this to sleep within create_task | |
await asyncio.sleep(20) | |
await user_input_action_stream_queue.put( | |
AGIAction( | |
action_type=AGIActionType.RUN_THREAD, | |
action_data=AGIActionRunThread( | |
agent_id=threads.currently.state_data.agent_id, | |
thread_id=threads.currently.state_data.thread_id, | |
), | |
), | |
) | |
elif agent_event.event_type == AGIEventType.NEW_THREAD_MESSAGE: | |
async with agents: | |
agent_state = agents[agent_event.event_data.agent_id] | |
# TODO https://rich.readthedocs.io/en/stable/markdown.html | |
if ( | |
agent_event.event_data.message_content_type == "text" | |
and agent_event.event_data.message_role == "agent" | |
): | |
print( | |
f"{agent_state.state_data.agent_name}: {agent_event.event_data.message_content}" | |
) | |
print(f"{user_name}: ", end="\r") | |
# Run any actions which have been waiting for an event | |
still_waiting = [] | |
while waiting: | |
action_waiting_for_event, make_action = waiting.pop(0) | |
if action_waiting_for_event == agent_event.event_type: | |
await user_input_action_stream_queue.put( | |
await make_action() | |
) | |
else: | |
still_waiting.append( | |
(action_waiting_for_event, make_action) | |
) | |
waiting.extend(still_waiting) | |
elif work_name == "user.unvalidated.input_action_stream": | |
work[ | |
tg.create_task( | |
ignore_stopasynciteration(work_ctx.__anext__()) | |
) | |
] = (work_name, work_ctx) | |
user_input = result | |
if pathlib.Path(user_input).is_file(): | |
await user_input_action_stream_queue.put( | |
AGIAction( | |
action_type=AGIActionType.INGEST_FILE, | |
action_data=AGIActionIngestFile( | |
agent_id=agents.currently.state_data.agent_id, | |
file_path=user_input, | |
), | |
), | |
) | |
continue | |
waiting.append( | |
( | |
AGIEventType.THREAD_MESSAGE_ADDED, | |
async_lambda( | |
lambda: AGIAction( | |
action_type=AGIActionType.RUN_THREAD, | |
action_data=AGIActionRunThread( | |
agent_id=threads.currently.state_data.agent_id, | |
thread_id=threads.currently.state_data.thread_id, | |
), | |
) | |
), | |
), | |
) | |
# TODO Handle case where agent does not yet exist | |
""" | |
async with agents: | |
active_agent_currently_undefined = ( | |
agents.currently == CURRENTLY_UNDEFINED | |
) | |
if active_agent_currently_undefined: | |
await tg.create_task(agents.currently_exists.wait()) | |
""" | |
async with threads: | |
active_thread_currently_undefined = ( | |
threads.currently == CURRENTLY_UNDEFINED | |
) | |
if active_thread_currently_undefined: | |
async with agents: | |
current_agent = agents.currently | |
if not isinstance(user_input, AGIAction): | |
waiting.append( | |
( | |
AGIEventType.NEW_THREAD_CREATED, | |
async_lambda( | |
lambda: AGIAction( | |
action_type=AGIActionType.ADD_MESSAGE, | |
action_data=AGIActionAddMessage( | |
agent_id=agents.currently.state_data.agent_id, | |
thread_id=threads.currently.state_data.thread_id, | |
message_role="user", | |
message_content=user_input, | |
), | |
) | |
), | |
), | |
) | |
await user_input_action_stream_queue.put( | |
AGIAction( | |
action_type=AGIActionType.NEW_THREAD, | |
action_data=AGIActionNewThread( | |
agent_id=current_agent.state_data.agent_id, | |
), | |
), | |
) | |
else: | |
await user_input_action_stream_queue.put( | |
AGIAction( | |
action_type=AGIActionType.ADD_MESSAGE, | |
action_data=AGIActionAddMessage( | |
agent_id=agents.currently.state_data.agent_id, | |
thread_id=threads.currently.state_data.thread_id, | |
message_role="user", | |
message_content=user_input, | |
), | |
), | |
) | |
if __name__ == "__main__": | |
# TODO Hook each thread to a terminal context with tmux | |
parser = make_argparse_parser() | |
args = parser.parse_args(sys.argv[1:]) | |
import httptest | |
with httptest.Server( | |
httptest.CachingProxyHandler.to( | |
str(openai.AsyncClient(api_key="Alice").base_url), | |
state_dir=str( | |
pathlib.Path(__file__).parent.joinpath(".cache", "openai") | |
), | |
) | |
) as cache_server: | |
# args.openai_base_url = cache_server.url() | |
asyncio.run(main(**vars(args))) |
{ | |
"action": "completed", | |
"check_run": { | |
"app": { | |
"created_at": "2018-07-30T09:30:17Z", | |
"description": "Automate your workflow from idea to production", | |
"events": [ | |
"branch_protection_rule", | |
"check_run", | |
"check_suite", | |
"create", | |
"delete", | |
"deployment", | |
"deployment_status", | |
"discussion", | |
"discussion_comment", | |
"fork", | |
"gollum", | |
"issues", | |
"issue_comment", | |
"label", | |
"merge_group", | |
"milestone", | |
"page_build", | |
"project", | |
"project_card", | |
"project_column", | |
"public", | |
"pull_request", | |
"pull_request_review", | |
"pull_request_review_comment", | |
"push", | |
"registry_package", | |
"release", | |
"repository", | |
"repository_dispatch", | |
"status", | |
"watch", | |
"workflow_dispatch", | |
"workflow_run" | |
], | |
"external_url": "https://help.github.com/en/actions", | |
"html_url": "https://github.com/apps/github-actions", | |
"id": 15368, | |
"name": "GitHub Actions", | |
"node_id": "MDM6QXBwMTUzNjg=", | |
"owner": { | |
"avatar_url": "https://avatars.githubusercontent.com/u/9919?v=4", | |
"events_url": "https://api.github.com/users/github/events{/privacy}", | |
"followers_url": "https://api.github.com/users/github/followers", | |
"following_url": "https://api.github.com/users/github/following{/other_user}", | |
"gists_url": "https://api.github.com/users/github/gists{/gist_id}", | |
"gravatar_id": "", | |
"html_url": "https://github.com/github", | |
"id": 9919, | |
"login": "github", | |
"node_id": "MDEyOk9yZ2FuaXphdGlvbjk5MTk=", | |
"organizations_url": "https://api.github.com/users/github/orgs", | |
"received_events_url": "https://api.github.com/users/github/received_events", | |
"repos_url": "https://api.github.com/users/github/repos", | |
"site_admin": false, | |
"starred_url": "https://api.github.com/users/github/starred{/owner}{/repo}", | |
"subscriptions_url": "https://api.github.com/users/github/subscriptions", | |
"type": "Organization", | |
"url": "https://api.github.com/users/github" | |
}, | |
"permissions": { | |
"actions": "write", | |
"administration": "read", | |
"checks": "write", | |
"contents": "write", | |
"deployments": "write", | |
"discussions": "write", | |
"issues": "write", | |
"merge_queues": "write", | |
"metadata": "read", | |
"packages": "write", | |
"pages": "write", | |
"pull_requests": "write", | |
"repository_hooks": "write", | |
"repository_projects": "write", | |
"security_events": "write", | |
"statuses": "write", | |
"vulnerability_alerts": "read" | |
}, | |
"slug": "github-actions", | |
"updated_at": "2019-12-10T19:04:12Z" | |
}, | |
"check_suite": { | |
"after": "e3cc9ad852a6551d92d9eb52f13b2d3208774d41", | |
"app": { | |
"created_at": "2018-07-30T09:30:17Z", | |
"description": "Automate your workflow from idea to production", | |
"events": [ | |
"branch_protection_rule", | |
"check_run", | |
"check_suite", | |
"create", | |
"delete", | |
"deployment", | |
"deployment_status", | |
"discussion", | |
"discussion_comment", | |
"fork", | |
"gollum", | |
"issues", | |
"issue_comment", | |
"label", | |
"merge_group", | |
"milestone", | |
"page_build", | |
"project", | |
"project_card", | |
"project_column", | |
"public", | |
"pull_request", | |
"pull_request_review", | |
"pull_request_review_comment", | |
"push", | |
"registry_package", | |
"release", | |
"repository", | |
"repository_dispatch", | |
"status", | |
"watch", | |
"workflow_dispatch", | |
"workflow_run" | |
], | |
"external_url": "https://help.github.com/en/actions", | |
"html_url": "https://github.com/apps/github-actions", | |
"id": 15368, | |
"name": "GitHub Actions", | |
"node_id": "MDM6QXBwMTUzNjg=", | |
"owner": { | |
"avatar_url": "https://avatars.githubusercontent.com/u/9919?v=4", | |
"events_url": "https://api.github.com/users/github/events{/privacy}", | |
"followers_url": "https://api.github.com/users/github/followers", | |
"following_url": "https://api.github.com/users/github/following{/other_user}", | |
"gists_url": "https://api.github.com/users/github/gists{/gist_id}", | |
"gravatar_id": "", | |
"html_url": "https://github.com/github", | |
"id": 9919, | |
"login": "github", | |
"node_id": "MDEyOk9yZ2FuaXphdGlvbjk5MTk=", | |
"organizations_url": "https://api.github.com/users/github/orgs", | |
"received_events_url": "https://api.github.com/users/github/received_events", | |
"repos_url": "https://api.github.com/users/github/repos", | |
"site_admin": false, | |
"starred_url": "https://api.github.com/users/github/starred{/owner}{/repo}", | |
"subscriptions_url": "https://api.github.com/users/github/subscriptions", | |
"type": "Organization", | |
"url": "https://api.github.com/users/github" | |
}, | |
"permissions": { | |
"actions": "write", | |
"administration": "read", | |
"checks": "write", | |
"contents": "write", | |
"deployments": "write", | |
"discussions": "write", | |
"issues": "write", | |
"merge_queues": "write", | |
"metadata": "read", | |
"packages": "write", | |
"pages": "write", | |
"pull_requests": "write", | |
"repository_hooks": "write", | |
"repository_projects": "write", | |
"security_events": "write", | |
"statuses": "write", | |
"vulnerability_alerts": "read" | |
}, | |
"slug": "github-actions", | |
"updated_at": "2019-12-10T19:04:12Z" | |
}, | |
"before": "f10df8c5020d64d22e2818581671535d29b79d24", | |
"conclusion": "failure", | |
"created_at": "2023-11-15T23:44:17Z", | |
"head_branch": "cwt", | |
"head_sha": "e3cc9ad852a6551d92d9eb52f13b2d3208774d41", | |
"id": 18230253313, | |
"node_id": "CS_kwDOJQW3oM8AAAAEPpuXAQ", | |
"pull_requests": [ | |
{ | |
"base": { | |
"ref": "main", | |
"repo": { | |
"id": 560509356, | |
"name": "scitt-api-emulator", | |
"url": "https://api.github.com/repos/scitt-community/scitt-api-emulator" | |
}, | |
"sha": "a30c8182358f2eab1feb380a7bf920b5483060f9" | |
}, | |
"head": { | |
"ref": "cwt", | |
"repo": { | |
"id": 621131680, | |
"name": "scitt-api-emulator", | |
"url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator" | |
}, | |
"sha": "e3cc9ad852a6551d92d9eb52f13b2d3208774d41" | |
}, | |
"id": 1596414276, | |
"number": 39, | |
"url": "https://api.github.com/repos/scitt-community/scitt-api-emulator/pulls/39" | |
} | |
], | |
"status": "completed", | |
"updated_at": "2023-11-15T23:47:10Z", | |
"url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/check-suites/18230253313" | |
}, | |
"completed_at": "2023-11-15T23:47:09Z", | |
"conclusion": "failure", | |
"details_url": "https://github.com/pdxjohnny/scitt-api-emulator/actions/runs/6884262695/job/18726406136", | |
"external_id": "5991732a-319e-5ad3-8066-dcd875b1cc17", | |
"head_sha": "e3cc9ad852a6551d92d9eb52f13b2d3208774d41", | |
"html_url": "https://github.com/pdxjohnny/scitt-api-emulator/actions/runs/6884262695/job/18726406136", | |
"id": 18726406136, | |
"name": "CI (conda)", | |
"node_id": "CR_kwDOJQW3oM8AAAAEXC5H-A", | |
"output": { | |
"annotations_count": 1, | |
"annotations_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/check-runs/18726406136/annotations", | |
"summary": null, | |
"text": null, | |
"title": null | |
}, | |
"pull_requests": [ | |
{ | |
"base": { | |
"ref": "main", | |
"repo": { | |
"id": 560509356, | |
"name": "scitt-api-emulator", | |
"url": "https://api.github.com/repos/scitt-community/scitt-api-emulator" | |
}, | |
"sha": "a30c8182358f2eab1feb380a7bf920b5483060f9" | |
}, | |
"head": { | |
"ref": "cwt", | |
"repo": { | |
"id": 621131680, | |
"name": "scitt-api-emulator", | |
"url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator" | |
}, | |
"sha": "e3cc9ad852a6551d92d9eb52f13b2d3208774d41" | |
}, | |
"id": 1596414276, | |
"number": 39, | |
"url": "https://api.github.com/repos/scitt-community/scitt-api-emulator/pulls/39" | |
} | |
], | |
"started_at": "2023-11-15T23:44:23Z", | |
"status": "completed", | |
"url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/check-runs/18726406136" | |
}, | |
"repository": { | |
"allow_forking": true, | |
"archive_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/{archive_format}{/ref}", | |
"archived": false, | |
"assignees_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/assignees{/user}", | |
"blobs_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/git/blobs{/sha}", | |
"branches_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/branches{/branch}", | |
"clone_url": "https://github.com/pdxjohnny/scitt-api-emulator.git", | |
"collaborators_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/collaborators{/collaborator}", | |
"comments_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/comments{/number}", | |
"commits_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/commits{/sha}", | |
"compare_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/compare/{base}...{head}", | |
"contents_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/contents/{+path}", | |
"contributors_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/contributors", | |
"created_at": "2023-03-30T03:43:27Z", | |
"default_branch": "auth", | |
"deployments_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/deployments", | |
"description": "SCITT API Emulator", | |
"disabled": false, | |
"downloads_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/downloads", | |
"events_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/events", | |
"fork": true, | |
"forks": 0, | |
"forks_count": 0, | |
"forks_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/forks", | |
"full_name": "pdxjohnny/scitt-api-emulator", | |
"git_commits_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/git/commits{/sha}", | |
"git_refs_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/git/refs{/sha}", | |
"git_tags_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/git/tags{/sha}", | |
"git_url": "git://github.com/pdxjohnny/scitt-api-emulator.git", | |
"has_discussions": false, | |
"has_downloads": true, | |
"has_issues": false, | |
"has_pages": false, | |
"has_projects": true, | |
"has_wiki": true, | |
"homepage": "https://scitt-community.github.io/scitt-api-emulator", | |
"hooks_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/hooks", | |
"html_url": "https://github.com/pdxjohnny/scitt-api-emulator", | |
"id": 621131680, | |
"is_template": false, | |
"issue_comment_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/issues/comments{/number}", | |
"issue_events_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/issues/events{/number}", | |
"issues_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/issues{/number}", | |
"keys_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/keys{/key_id}", | |
"labels_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/labels{/name}", | |
"language": "Python", | |
"languages_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/languages", | |
"license": { | |
"key": "mit", | |
"name": "MIT License", | |
"node_id": "MDc6TGljZW5zZTEz", | |
"spdx_id": "MIT", | |
"url": "https://api.github.com/licenses/mit" | |
}, | |
"merges_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/merges", | |
"milestones_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/milestones{/number}", | |
"mirror_url": null, | |
"name": "scitt-api-emulator", | |
"node_id": "R_kgDOJQW3oA", | |
"notifications_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/notifications{?since,all,participating}", | |
"open_issues": 1, | |
"open_issues_count": 1, | |
"owner": { | |
"avatar_url": "https://avatars.githubusercontent.com/u/5950433?v=4", | |
"events_url": "https://api.github.com/users/pdxjohnny/events{/privacy}", | |
"followers_url": "https://api.github.com/users/pdxjohnny/followers", | |
"following_url": "https://api.github.com/users/pdxjohnny/following{/other_user}", | |
"gists_url": "https://api.github.com/users/pdxjohnny/gists{/gist_id}", | |
"gravatar_id": "", | |
"html_url": "https://github.com/pdxjohnny", | |
"id": 5950433, | |
"login": "pdxjohnny", | |
"node_id": "MDQ6VXNlcjU5NTA0MzM=", | |
"organizations_url": "https://api.github.com/users/pdxjohnny/orgs", | |
"received_events_url": "https://api.github.com/users/pdxjohnny/received_events", | |
"repos_url": "https://api.github.com/users/pdxjohnny/repos", | |
"site_admin": false, | |
"starred_url": "https://api.github.com/users/pdxjohnny/starred{/owner}{/repo}", | |
"subscriptions_url": "https://api.github.com/users/pdxjohnny/subscriptions", | |
"type": "User", | |
"url": "https://api.github.com/users/pdxjohnny" | |
}, | |
"private": false, | |
"pulls_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/pulls{/number}", | |
"pushed_at": "2023-11-15T23:37:40Z", | |
"releases_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/releases{/id}", | |
"size": 217, | |
"ssh_url": "git@github.com:pdxjohnny/scitt-api-emulator.git", | |
"stargazers_count": 0, | |
"stargazers_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/stargazers", | |
"statuses_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/statuses/{sha}", | |
"subscribers_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/subscribers", | |
"subscription_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/subscription", | |
"svn_url": "https://github.com/pdxjohnny/scitt-api-emulator", | |
"tags_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/tags", | |
"teams_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/teams", | |
"topics": [], | |
"trees_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/git/trees{/sha}", | |
"updated_at": "2023-09-12T21:02:36Z", | |
"url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator", | |
"visibility": "public", | |
"watchers": 0, | |
"watchers_count": 0, | |
"web_commit_signoff_required": true | |
}, | |
"sender": { | |
"avatar_url": "https://avatars.githubusercontent.com/u/5950433?v=4", | |
"events_url": "https://api.github.com/users/pdxjohnny/events{/privacy}", | |
"followers_url": "https://api.github.com/users/pdxjohnny/followers", | |
"following_url": "https://api.github.com/users/pdxjohnny/following{/other_user}", | |
"gists_url": "https://api.github.com/users/pdxjohnny/gists{/gist_id}", | |
"gravatar_id": "", | |
"html_url": "https://github.com/pdxjohnny", | |
"id": 5950433, | |
"login": "pdxjohnny", | |
"node_id": "MDQ6VXNlcjU5NTA0MzM=", | |
"organizations_url": "https://api.github.com/users/pdxjohnny/orgs", | |
"received_events_url": "https://api.github.com/users/pdxjohnny/received_events", | |
"repos_url": "https://api.github.com/users/pdxjohnny/repos", | |
"site_admin": false, | |
"starred_url": "https://api.github.com/users/pdxjohnny/starred{/owner}{/repo}", | |
"subscriptions_url": "https://api.github.com/users/pdxjohnny/subscriptions", | |
"type": "User", | |
"url": "https://api.github.com/users/pdxjohnny" | |
} | |
} |
{ | |
"action": "created", | |
"check_run": { | |
"app": { | |
"created_at": "2018-07-30T09:30:17Z", | |
"description": "Automate your workflow from idea to production", | |
"events": [ | |
"branch_protection_rule", | |
"check_run", | |
"check_suite", | |
"create", | |
"delete", | |
"deployment", | |
"deployment_status", | |
"discussion", | |
"discussion_comment", | |
"fork", | |
"gollum", | |
"issues", | |
"issue_comment", | |
"label", | |
"merge_group", | |
"milestone", | |
"page_build", | |
"project", | |
"project_card", | |
"project_column", | |
"public", | |
"pull_request", | |
"pull_request_review", | |
"pull_request_review_comment", | |
"push", | |
"registry_package", | |
"release", | |
"repository", | |
"repository_dispatch", | |
"status", | |
"watch", | |
"workflow_dispatch", | |
"workflow_run" | |
], | |
"external_url": "https://help.github.com/en/actions", | |
"html_url": "https://github.com/apps/github-actions", | |
"id": 15368, | |
"name": "GitHub Actions", | |
"node_id": "MDM6QXBwMTUzNjg=", | |
"owner": { | |
"avatar_url": "https://avatars.githubusercontent.com/u/9919?v=4", | |
"events_url": "https://api.github.com/users/github/events{/privacy}", | |
"followers_url": "https://api.github.com/users/github/followers", | |
"following_url": "https://api.github.com/users/github/following{/other_user}", | |
"gists_url": "https://api.github.com/users/github/gists{/gist_id}", | |
"gravatar_id": "", | |
"html_url": "https://github.com/github", | |
"id": 9919, | |
"login": "github", | |
"node_id": "MDEyOk9yZ2FuaXphdGlvbjk5MTk=", | |
"organizations_url": "https://api.github.com/users/github/orgs", | |
"received_events_url": "https://api.github.com/users/github/received_events", | |
"repos_url": "https://api.github.com/users/github/repos", | |
"site_admin": false, | |
"starred_url": "https://api.github.com/users/github/starred{/owner}{/repo}", | |
"subscriptions_url": "https://api.github.com/users/github/subscriptions", | |
"type": "Organization", | |
"url": "https://api.github.com/users/github" | |
}, | |
"permissions": { | |
"actions": "write", | |
"administration": "read", | |
"checks": "write", | |
"contents": "write", | |
"deployments": "write", | |
"discussions": "write", | |
"issues": "write", | |
"merge_queues": "write", | |
"metadata": "read", | |
"packages": "write", | |
"pages": "write", | |
"pull_requests": "write", | |
"repository_hooks": "write", | |
"repository_projects": "write", | |
"security_events": "write", | |
"statuses": "write", | |
"vulnerability_alerts": "read" | |
}, | |
"slug": "github-actions", | |
"updated_at": "2019-12-10T19:04:12Z" | |
}, | |
"check_suite": { | |
"after": "e3cc9ad852a6551d92d9eb52f13b2d3208774d41", | |
"app": { | |
"created_at": "2018-07-30T09:30:17Z", | |
"description": "Automate your workflow from idea to production", | |
"events": [ | |
"branch_protection_rule", | |
"check_run", | |
"check_suite", | |
"create", | |
"delete", | |
"deployment", | |
"deployment_status", | |
"discussion", | |
"discussion_comment", | |
"fork", | |
"gollum", | |
"issues", | |
"issue_comment", | |
"label", | |
"merge_group", | |
"milestone", | |
"page_build", | |
"project", | |
"project_card", | |
"project_column", | |
"public", | |
"pull_request", | |
"pull_request_review", | |
"pull_request_review_comment", | |
"push", | |
"registry_package", | |
"release", | |
"repository", | |
"repository_dispatch", | |
"status", | |
"watch", | |
"workflow_dispatch", | |
"workflow_run" | |
], | |
"external_url": "https://help.github.com/en/actions", | |
"html_url": "https://github.com/apps/github-actions", | |
"id": 15368, | |
"name": "GitHub Actions", | |
"node_id": "MDM6QXBwMTUzNjg=", | |
"owner": { | |
"avatar_url": "https://avatars.githubusercontent.com/u/9919?v=4", | |
"events_url": "https://api.github.com/users/github/events{/privacy}", | |
"followers_url": "https://api.github.com/users/github/followers", | |
"following_url": "https://api.github.com/users/github/following{/other_user}", | |
"gists_url": "https://api.github.com/users/github/gists{/gist_id}", | |
"gravatar_id": "", | |
"html_url": "https://github.com/github", | |
"id": 9919, | |
"login": "github", | |
"node_id": "MDEyOk9yZ2FuaXphdGlvbjk5MTk=", | |
"organizations_url": "https://api.github.com/users/github/orgs", | |
"received_events_url": "https://api.github.com/users/github/received_events", | |
"repos_url": "https://api.github.com/users/github/repos", | |
"site_admin": false, | |
"starred_url": "https://api.github.com/users/github/starred{/owner}{/repo}", | |
"subscriptions_url": "https://api.github.com/users/github/subscriptions", | |
"type": "Organization", | |
"url": "https://api.github.com/users/github" | |
}, | |
"permissions": { | |
"actions": "write", | |
"administration": "read", | |
"checks": "write", | |
"contents": "write", | |
"deployments": "write", | |
"discussions": "write", | |
"issues": "write", | |
"merge_queues": "write", | |
"metadata": "read", | |
"packages": "write", | |
"pages": "write", | |
"pull_requests": "write", | |
"repository_hooks": "write", | |
"repository_projects": "write", | |
"security_events": "write", | |
"statuses": "write", | |
"vulnerability_alerts": "read" | |
}, | |
"slug": "github-actions", | |
"updated_at": "2019-12-10T19:04:12Z" | |
}, | |
"before": "f10df8c5020d64d22e2818581671535d29b79d24", | |
"conclusion": null, | |
"created_at": "2023-11-15T23:44:17Z", | |
"head_branch": "cwt", | |
"head_sha": "e3cc9ad852a6551d92d9eb52f13b2d3208774d41", | |
"id": 18230253313, | |
"node_id": "CS_kwDOJQW3oM8AAAAEPpuXAQ", | |
"pull_requests": [ | |
{ | |
"base": { | |
"ref": "main", | |
"repo": { | |
"id": 560509356, | |
"name": "scitt-api-emulator", | |
"url": "https://api.github.com/repos/scitt-community/scitt-api-emulator" | |
}, | |
"sha": "a30c8182358f2eab1feb380a7bf920b5483060f9" | |
}, | |
"head": { | |
"ref": "cwt", | |
"repo": { | |
"id": 621131680, | |
"name": "scitt-api-emulator", | |
"url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator" | |
}, | |
"sha": "e3cc9ad852a6551d92d9eb52f13b2d3208774d41" | |
}, | |
"id": 1596414276, | |
"number": 39, | |
"url": "https://api.github.com/repos/scitt-community/scitt-api-emulator/pulls/39" | |
} | |
], | |
"status": "queued", | |
"updated_at": "2023-11-15T23:44:17Z", | |
"url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/check-suites/18230253313" | |
}, | |
"completed_at": null, | |
"conclusion": null, | |
"details_url": "https://github.com/pdxjohnny/scitt-api-emulator/actions/runs/6884262695/job/18726406244", | |
"external_id": "c5ad7ac8-bcdc-5ad7-e04f-c98593680465", | |
"head_sha": "e3cc9ad852a6551d92d9eb52f13b2d3208774d41", | |
"html_url": "https://github.com/pdxjohnny/scitt-api-emulator/actions/runs/6884262695/job/18726406244", | |
"id": 18726406244, | |
"name": "CI (venv) (3.8)", | |
"node_id": "CR_kwDOJQW3oM8AAAAEXC5IZA", | |
"output": { | |
"annotations_count": 0, | |
"annotations_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/check-runs/18726406244/annotations", | |
"summary": null, | |
"text": null, | |
"title": null | |
}, | |
"pull_requests": [ | |
{ | |
"base": { | |
"ref": "main", | |
"repo": { | |
"id": 560509356, | |
"name": "scitt-api-emulator", | |
"url": "https://api.github.com/repos/scitt-community/scitt-api-emulator" | |
}, | |
"sha": "a30c8182358f2eab1feb380a7bf920b5483060f9" | |
}, | |
"head": { | |
"ref": "cwt", | |
"repo": { | |
"id": 621131680, | |
"name": "scitt-api-emulator", | |
"url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator" | |
}, | |
"sha": "e3cc9ad852a6551d92d9eb52f13b2d3208774d41" | |
}, | |
"id": 1596414276, | |
"number": 39, | |
"url": "https://api.github.com/repos/scitt-community/scitt-api-emulator/pulls/39" | |
} | |
], | |
"started_at": "2023-11-15T23:44:19Z", | |
"status": "queued", | |
"url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/check-runs/18726406244" | |
}, | |
"repository": { | |
"allow_forking": true, | |
"archive_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/{archive_format}{/ref}", | |
"archived": false, | |
"assignees_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/assignees{/user}", | |
"blobs_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/git/blobs{/sha}", | |
"branches_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/branches{/branch}", | |
"clone_url": "https://github.com/pdxjohnny/scitt-api-emulator.git", | |
"collaborators_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/collaborators{/collaborator}", | |
"comments_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/comments{/number}", | |
"commits_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/commits{/sha}", | |
"compare_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/compare/{base}...{head}", | |
"contents_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/contents/{+path}", | |
"contributors_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/contributors", | |
"created_at": "2023-03-30T03:43:27Z", | |
"default_branch": "auth", | |
"deployments_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/deployments", | |
"description": "SCITT API Emulator", | |
"disabled": false, | |
"downloads_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/downloads", | |
"events_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/events", | |
"fork": true, | |
"forks": 0, | |
"forks_count": 0, | |
"forks_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/forks", | |
"full_name": "pdxjohnny/scitt-api-emulator", | |
"git_commits_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/git/commits{/sha}", | |
"git_refs_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/git/refs{/sha}", | |
"git_tags_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/git/tags{/sha}", | |
"git_url": "git://github.com/pdxjohnny/scitt-api-emulator.git", | |
"has_discussions": false, | |
"has_downloads": true, | |
"has_issues": false, | |
"has_pages": false, | |
"has_projects": true, | |
"has_wiki": true, | |
"homepage": "https://scitt-community.github.io/scitt-api-emulator", | |
"hooks_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/hooks", | |
"html_url": "https://github.com/pdxjohnny/scitt-api-emulator", | |
"id": 621131680, | |
"is_template": false, | |
"issue_comment_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/issues/comments{/number}", | |
"issue_events_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/issues/events{/number}", | |
"issues_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/issues{/number}", | |
"keys_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/keys{/key_id}", | |
"labels_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/labels{/name}", | |
"language": "Python", | |
"languages_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/languages", | |
"license": { | |
"key": "mit", | |
"name": "MIT License", | |
"node_id": "MDc6TGljZW5zZTEz", | |
"spdx_id": "MIT", | |
"url": "https://api.github.com/licenses/mit" | |
}, | |
"merges_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/merges", | |
"milestones_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/milestones{/number}", | |
"mirror_url": null, | |
"name": "scitt-api-emulator", | |
"node_id": "R_kgDOJQW3oA", | |
"notifications_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/notifications{?since,all,participating}", | |
"open_issues": 1, | |
"open_issues_count": 1, | |
"owner": { | |
"avatar_url": "https://avatars.githubusercontent.com/u/5950433?v=4", | |
"events_url": "https://api.github.com/users/pdxjohnny/events{/privacy}", | |
"followers_url": "https://api.github.com/users/pdxjohnny/followers", | |
"following_url": "https://api.github.com/users/pdxjohnny/following{/other_user}", | |
"gists_url": "https://api.github.com/users/pdxjohnny/gists{/gist_id}", | |
"gravatar_id": "", | |
"html_url": "https://github.com/pdxjohnny", | |
"id": 5950433, | |
"login": "pdxjohnny", | |
"node_id": "MDQ6VXNlcjU5NTA0MzM=", | |
"organizations_url": "https://api.github.com/users/pdxjohnny/orgs", | |
"received_events_url": "https://api.github.com/users/pdxjohnny/received_events", | |
"repos_url": "https://api.github.com/users/pdxjohnny/repos", | |
"site_admin": false, | |
"starred_url": "https://api.github.com/users/pdxjohnny/starred{/owner}{/repo}", | |
"subscriptions_url": "https://api.github.com/users/pdxjohnny/subscriptions", | |
"type": "User", | |
"url": "https://api.github.com/users/pdxjohnny" | |
}, | |
"private": false, | |
"pulls_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/pulls{/number}", | |
"pushed_at": "2023-11-15T23:37:40Z", | |
"releases_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/releases{/id}", | |
"size": 217, | |
"ssh_url": "git@github.com:pdxjohnny/scitt-api-emulator.git", | |
"stargazers_count": 0, | |
"stargazers_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/stargazers", | |
"statuses_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/statuses/{sha}", | |
"subscribers_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/subscribers", | |
"subscription_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/subscription", | |
"svn_url": "https://github.com/pdxjohnny/scitt-api-emulator", | |
"tags_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/tags", | |
"teams_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/teams", | |
"topics": [], | |
"trees_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/git/trees{/sha}", | |
"updated_at": "2023-09-12T21:02:36Z", | |
"url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator", | |
"visibility": "public", | |
"watchers": 0, | |
"watchers_count": 0, | |
"web_commit_signoff_required": true | |
}, | |
"sender": { | |
"avatar_url": "https://avatars.githubusercontent.com/u/5950433?v=4", | |
"events_url": "https://api.github.com/users/pdxjohnny/events{/privacy}", | |
"followers_url": "https://api.github.com/users/pdxjohnny/followers", | |
"following_url": "https://api.github.com/users/pdxjohnny/following{/other_user}", | |
"gists_url": "https://api.github.com/users/pdxjohnny/gists{/gist_id}", | |
"gravatar_id": "", | |
"html_url": "https://github.com/pdxjohnny", | |
"id": 5950433, | |
"login": "pdxjohnny", | |
"node_id": "MDQ6VXNlcjU5NTA0MzM=", | |
"organizations_url": "https://api.github.com/users/pdxjohnny/orgs", | |
"received_events_url": "https://api.github.com/users/pdxjohnny/received_events", | |
"repos_url": "https://api.github.com/users/pdxjohnny/repos", | |
"site_admin": false, | |
"starred_url": "https://api.github.com/users/pdxjohnny/starred{/owner}{/repo}", | |
"subscriptions_url": "https://api.github.com/users/pdxjohnny/subscriptions", | |
"type": "User", | |
"url": "https://api.github.com/users/pdxjohnny" | |
} | |
} |
{ | |
"action": "completed", | |
"check_suite": { | |
"after": "e3cc9ad852a6551d92d9eb52f13b2d3208774d41", | |
"app": { | |
"created_at": "2018-07-30T09:30:17Z", | |
"description": "Automate your workflow from idea to production", | |
"events": [ | |
"branch_protection_rule", | |
"check_run", | |
"check_suite", | |
"create", | |
"delete", | |
"deployment", | |
"deployment_status", | |
"discussion", | |
"discussion_comment", | |
"fork", | |
"gollum", | |
"issues", | |
"issue_comment", | |
"label", | |
"merge_group", | |
"milestone", | |
"page_build", | |
"project", | |
"project_card", | |
"project_column", | |
"public", | |
"pull_request", | |
"pull_request_review", | |
"pull_request_review_comment", | |
"push", | |
"registry_package", | |
"release", | |
"repository", | |
"repository_dispatch", | |
"status", | |
"watch", | |
"workflow_dispatch", | |
"workflow_run" | |
], | |
"external_url": "https://help.github.com/en/actions", | |
"html_url": "https://github.com/apps/github-actions", | |
"id": 15368, | |
"name": "GitHub Actions", | |
"node_id": "MDM6QXBwMTUzNjg=", | |
"owner": { | |
"avatar_url": "https://avatars.githubusercontent.com/u/9919?v=4", | |
"events_url": "https://api.github.com/users/github/events{/privacy}", | |
"followers_url": "https://api.github.com/users/github/followers", | |
"following_url": "https://api.github.com/users/github/following{/other_user}", | |
"gists_url": "https://api.github.com/users/github/gists{/gist_id}", | |
"gravatar_id": "", | |
"html_url": "https://github.com/github", | |
"id": 9919, | |
"login": "github", | |
"node_id": "MDEyOk9yZ2FuaXphdGlvbjk5MTk=", | |
"organizations_url": "https://api.github.com/users/github/orgs", | |
"received_events_url": "https://api.github.com/users/github/received_events", | |
"repos_url": "https://api.github.com/users/github/repos", | |
"site_admin": false, | |
"starred_url": "https://api.github.com/users/github/starred{/owner}{/repo}", | |
"subscriptions_url": "https://api.github.com/users/github/subscriptions", | |
"type": "Organization", | |
"url": "https://api.github.com/users/github" | |
}, | |
"permissions": { | |
"actions": "write", | |
"administration": "read", | |
"checks": "write", | |
"contents": "write", | |
"deployments": "write", | |
"discussions": "write", | |
"issues": "write", | |
"merge_queues": "write", | |
"metadata": "read", | |
"packages": "write", | |
"pages": "write", | |
"pull_requests": "write", | |
"repository_hooks": "write", | |
"repository_projects": "write", | |
"security_events": "write", | |
"statuses": "write", | |
"vulnerability_alerts": "read" | |
}, | |
"slug": "github-actions", | |
"updated_at": "2019-12-10T19:04:12Z" | |
}, | |
"before": "f10df8c5020d64d22e2818581671535d29b79d24", | |
"check_runs_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/check-suites/18230253313/check-runs", | |
"conclusion": "failure", | |
"created_at": "2023-11-15T23:44:17Z", | |
"head_branch": "cwt", | |
"head_commit": { | |
"author": { | |
"email": "johnandersenpdx@gmail.com", | |
"name": "John Andersen" | |
}, | |
"committer": { | |
"email": "johnandersenpdx@gmail.com", | |
"name": "John Andersen" | |
}, | |
"id": "e3cc9ad852a6551d92d9eb52f13b2d3208774d41", | |
"message": "IN PROGRESS: did helpers: ecdsa p-384 key loading\n\nSigned-off-by: John Andersen <johnandersenpdx@gmail.com>", | |
"timestamp": "2023-11-15T23:37:36Z", | |
"tree_id": "70268469668b1cbff7e4684528217af7a54d66bb" | |
}, | |
"head_sha": "e3cc9ad852a6551d92d9eb52f13b2d3208774d41", | |
"id": 18230253313, | |
"latest_check_runs_count": 3, | |
"node_id": "CS_kwDOJQW3oM8AAAAEPpuXAQ", | |
"pull_requests": [ | |
{ | |
"base": { | |
"ref": "main", | |
"repo": { | |
"id": 560509356, | |
"name": "scitt-api-emulator", | |
"url": "https://api.github.com/repos/scitt-community/scitt-api-emulator" | |
}, | |
"sha": "a30c8182358f2eab1feb380a7bf920b5483060f9" | |
}, | |
"head": { | |
"ref": "cwt", | |
"repo": { | |
"id": 621131680, | |
"name": "scitt-api-emulator", | |
"url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator" | |
}, | |
"sha": "e3cc9ad852a6551d92d9eb52f13b2d3208774d41" | |
}, | |
"id": 1596414276, | |
"number": 39, | |
"url": "https://api.github.com/repos/scitt-community/scitt-api-emulator/pulls/39" | |
} | |
], | |
"rerequestable": true, | |
"runs_rerequestable": false, | |
"status": "completed", | |
"updated_at": "2023-11-15T23:47:10Z", | |
"url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/check-suites/18230253313" | |
}, | |
"repository": { | |
"allow_forking": true, | |
"archive_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/{archive_format}{/ref}", | |
"archived": false, | |
"assignees_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/assignees{/user}", | |
"blobs_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/git/blobs{/sha}", | |
"branches_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/branches{/branch}", | |
"clone_url": "https://github.com/pdxjohnny/scitt-api-emulator.git", | |
"collaborators_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/collaborators{/collaborator}", | |
"comments_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/comments{/number}", | |
"commits_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/commits{/sha}", | |
"compare_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/compare/{base}...{head}", | |
"contents_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/contents/{+path}", | |
"contributors_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/contributors", | |
"created_at": "2023-03-30T03:43:27Z", | |
"default_branch": "auth", | |
"deployments_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/deployments", | |
"description": "SCITT API Emulator", | |
"disabled": false, | |
"downloads_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/downloads", | |
"events_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/events", | |
"fork": true, | |
"forks": 0, | |
"forks_count": 0, | |
"forks_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/forks", | |
"full_name": "pdxjohnny/scitt-api-emulator", | |
"git_commits_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/git/commits{/sha}", | |
"git_refs_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/git/refs{/sha}", | |
"git_tags_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/git/tags{/sha}", | |
"git_url": "git://github.com/pdxjohnny/scitt-api-emulator.git", | |
"has_discussions": false, | |
"has_downloads": true, | |
"has_issues": false, | |
"has_pages": false, | |
"has_projects": true, | |
"has_wiki": true, | |
"homepage": "https://scitt-community.github.io/scitt-api-emulator", | |
"hooks_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/hooks", | |
"html_url": "https://github.com/pdxjohnny/scitt-api-emulator", | |
"id": 621131680, | |
"is_template": false, | |
"issue_comment_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/issues/comments{/number}", | |
"issue_events_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/issues/events{/number}", | |
"issues_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/issues{/number}", | |
"keys_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/keys{/key_id}", | |
"labels_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/labels{/name}", | |
"language": "Python", | |
"languages_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/languages", | |
"license": { | |
"key": "mit", | |
"name": "MIT License", | |
"node_id": "MDc6TGljZW5zZTEz", | |
"spdx_id": "MIT", | |
"url": "https://api.github.com/licenses/mit" | |
}, | |
"merges_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/merges", | |
"milestones_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/milestones{/number}", | |
"mirror_url": null, | |
"name": "scitt-api-emulator", | |
"node_id": "R_kgDOJQW3oA", | |
"notifications_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/notifications{?since,all,participating}", | |
"open_issues": 1, | |
"open_issues_count": 1, | |
"owner": { | |
"avatar_url": "https://avatars.githubusercontent.com/u/5950433?v=4", | |
"events_url": "https://api.github.com/users/pdxjohnny/events{/privacy}", | |
"followers_url": "https://api.github.com/users/pdxjohnny/followers", | |
"following_url": "https://api.github.com/users/pdxjohnny/following{/other_user}", | |
"gists_url": "https://api.github.com/users/pdxjohnny/gists{/gist_id}", | |
"gravatar_id": "", | |
"html_url": "https://github.com/pdxjohnny", | |
"id": 5950433, | |
"login": "pdxjohnny", | |
"node_id": "MDQ6VXNlcjU5NTA0MzM=", | |
"organizations_url": "https://api.github.com/users/pdxjohnny/orgs", | |
"received_events_url": "https://api.github.com/users/pdxjohnny/received_events", | |
"repos_url": "https://api.github.com/users/pdxjohnny/repos", | |
"site_admin": false, | |
"starred_url": "https://api.github.com/users/pdxjohnny/starred{/owner}{/repo}", | |
"subscriptions_url": "https://api.github.com/users/pdxjohnny/subscriptions", | |
"type": "User", | |
"url": "https://api.github.com/users/pdxjohnny" | |
}, | |
"private": false, | |
"pulls_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/pulls{/number}", | |
"pushed_at": "2023-11-15T23:37:40Z", | |
"releases_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/releases{/id}", | |
"size": 217, | |
"ssh_url": "git@github.com:pdxjohnny/scitt-api-emulator.git", | |
"stargazers_count": 0, | |
"stargazers_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/stargazers", | |
"statuses_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/statuses/{sha}", | |
"subscribers_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/subscribers", | |
"subscription_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/subscription", | |
"svn_url": "https://github.com/pdxjohnny/scitt-api-emulator", | |
"tags_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/tags", | |
"teams_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/teams", | |
"topics": [], | |
"trees_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/git/trees{/sha}", | |
"updated_at": "2023-09-12T21:02:36Z", | |
"url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator", | |
"visibility": "public", | |
"watchers": 0, | |
"watchers_count": 0, | |
"web_commit_signoff_required": true | |
}, | |
"sender": { | |
"avatar_url": "https://avatars.githubusercontent.com/u/5950433?v=4", | |
"events_url": "https://api.github.com/users/pdxjohnny/events{/privacy}", | |
"followers_url": "https://api.github.com/users/pdxjohnny/followers", | |
"following_url": "https://api.github.com/users/pdxjohnny/following{/other_user}", | |
"gists_url": "https://api.github.com/users/pdxjohnny/gists{/gist_id}", | |
"gravatar_id": "", | |
"html_url": "https://github.com/pdxjohnny", | |
"id": 5950433, | |
"login": "pdxjohnny", | |
"node_id": "MDQ6VXNlcjU5NTA0MzM=", | |
"organizations_url": "https://api.github.com/users/pdxjohnny/orgs", | |
"received_events_url": "https://api.github.com/users/pdxjohnny/received_events", | |
"repos_url": "https://api.github.com/users/pdxjohnny/repos", | |
"site_admin": false, | |
"starred_url": "https://api.github.com/users/pdxjohnny/starred{/owner}{/repo}", | |
"subscriptions_url": "https://api.github.com/users/pdxjohnny/subscriptions", | |
"type": "User", | |
"url": "https://api.github.com/users/pdxjohnny" | |
} | |
} |
{ | |
"action": "edited", | |
"changes": { | |
"body": { | |
"from": "## 2023-11-23 @pdxjohnny Engineering Logs\r\n\r\n- Happy Thanksgiving!\r\n\r\n```bash\r\nexport COMPUTE_IPV4=$(doctl compute droplet list --no-header --format PublicIPv4 prophecy-0)\r\ndoctl compute domain records create --record-name alice.chadig.com --record-ttl 3600 --record-type A --record-data \"${COMPUTE_IPV4}\" chadig.com\r\ndoctl compute domain records create --record-name github-webhook-notary.scitt.alice.chadig.com --record-ttl 3600 --record-type A --record-data \"${COMPUTE_IPV4}\" chadig.com\r\n```\r\n\r\n```caddyfile\r\nalice.chadig.com {\r\n redir \"https://github.com/intel/dffml/discussions/1406?sort=new\" temporary\r\n}\r\n\r\ngithub-webhook-notary.scitt.alice.chadig.com {\r\n reverse_proxy http://localhost:7777\r\n}\r\n\r\nscitt.bob.chadig.com {\r\n reverse_proxy http://localhost:6000\r\n}\r\n\r\nscitt.alice.chadig.com {\r\n reverse_proxy http://localhost:7000\r\n}\r\n\r\nscitt.unstable.chadig.com {\r\n reverse_proxy http://localhost:8000\r\n}\r\n\r\nscitt.pdxjohnny.chadig.com {\r\n reverse_proxy http://localhost:9000\r\n}\r\n\r\ndefine.chadig.com {\r\n respond \"Cha-Dig: can you dig it? chaaaaaaa I can dig it!!!\"\r\n}\r\n```\r\n\r\n- TODO\r\n - [ ] GitHub App Blueprints to\r\n - [x] https://github.com/apps/alice-oa\r\n - [ ] Webhook events to notarizing proxy\r\n - [ ] #1315\r\n - [ ] Bovine based downstream event receiver\r\n - [ ] As async iterator for new data events\r\n - [ ] POC using OpenAI agent threads with file uploads\r\n - [ ] Alice engineering log entry in daily discussion thread for updates\r\n - [ ] Checkbox checked by maintainer for requests approval\r\n - [ ] Assign issues to Alice via `Assignee: @aliceoa` watch webhook issue creation or body updates" | |
} | |
}, | |
"comment": { | |
"author_association": "MEMBER", | |
"body": "## 2023-11-23 @pdxjohnny Engineering Logs\r\n\r\n- Happy Thanksgiving!\r\n\r\n```bash\r\nexport COMPUTE_IPV4=$(doctl compute droplet list --no-header --format PublicIPv4 prophecy-0)\r\ndoctl compute domain records create --record-name alice --record-ttl 3600 --record-type A --record-data \"${COMPUTE_IPV4}\" chadig.com\r\ndoctl compute domain records create --record-name github-webhook-notary.scitt.alice --record-ttl 3600 --record-type A --record-data \"${COMPUTE_IPV4}\" chadig.com\r\n```\r\n\r\n```caddyfile\r\nalice.chadig.com {\r\n redir \"https://github.com/intel/dffml/discussions/1406?sort=new\" temporary\r\n}\r\n\r\ngithub-webhook-notary.scitt.alice.chadig.com {\r\n reverse_proxy http://localhost:7777\r\n}\r\n\r\nscitt.bob.chadig.com {\r\n reverse_proxy http://localhost:6000\r\n}\r\n\r\nscitt.alice.chadig.com {\r\n reverse_proxy http://localhost:7000\r\n}\r\n\r\nscitt.unstable.chadig.com {\r\n reverse_proxy http://localhost:8000\r\n}\r\n\r\nscitt.pdxjohnny.chadig.com {\r\n reverse_proxy http://localhost:9000\r\n}\r\n\r\ndefine.chadig.com {\r\n respond \"Cha-Dig: can you dig it? chaaaaaaa I can dig it!!!\"\r\n}\r\n```\r\n\r\n- TODO\r\n - [ ] GitHub App Blueprints to\r\n - [x] https://github.com/apps/alice-oa\r\n - [ ] Webhook events to notarizing proxy\r\n - [ ] `$ gh webhook forward --repo=intel/dffml --events='*' --url=https://github-webhook-notary.scitt.alice.chadig.com`\r\n - [ ] #1315\r\n - [ ] Bovine based downstream event receiver\r\n - [ ] As async iterator for new data events\r\n - [ ] POC using OpenAI agent threads with file uploads\r\n - [ ] Alice engineering log entry in daily discussion thread for updates\r\n - [ ] Checkbox checked by maintainer for requests approval\r\n - [ ] Assign issues to Alice via `Assignee: @aliceoa` watch webhook issue creation or body updates\r\n - `cat issues.action\\:edited.json | jq 'select(.issue.body | index(\"Assignee: @aliceoa\"))'`", | |
"child_comment_count": 0, | |
"created_at": "2023-11-23T12:11:52Z", | |
"discussion_id": 4225995, | |
"html_url": "https://github.com/intel/dffml/discussions/1406#discussioncomment-7651358", | |
"id": 7651358, | |
"node_id": "DC_kwDOCOlgGM4AdMAe", | |
"parent_id": 7648573, | |
"reactions": { | |
"+1": 0, | |
"-1": 0, | |
"confused": 0, | |
"eyes": 0, | |
"heart": 0, | |
"hooray": 0, | |
"laugh": 0, | |
"rocket": 0, | |
"total_count": 0, | |
"url": "https://api.github.com/repos/intel/dffml/issues/comments/7651358/reactions" | |
}, | |
"repository_url": "intel/dffml", | |
"updated_at": "2023-11-23T15:08:58Z", | |
"user": { | |
"avatar_url": "https://avatars.githubusercontent.com/u/5950433?v=4", | |
"events_url": "https://api.github.com/users/pdxjohnny/events{/privacy}", | |
"followers_url": "https://api.github.com/users/pdxjohnny/followers", | |
"following_url": "https://api.github.com/users/pdxjohnny/following{/other_user}", | |
"gists_url": "https://api.github.com/users/pdxjohnny/gists{/gist_id}", | |
"gravatar_id": "", | |
"html_url": "https://github.com/pdxjohnny", | |
"id": 5950433, | |
"login": "pdxjohnny", | |
"node_id": "MDQ6VXNlcjU5NTA0MzM=", | |
"organizations_url": "https://api.github.com/users/pdxjohnny/orgs", | |
"received_events_url": "https://api.github.com/users/pdxjohnny/received_events", | |
"repos_url": "https://api.github.com/users/pdxjohnny/repos", | |
"site_admin": false, | |
"starred_url": "https://api.github.com/users/pdxjohnny/starred{/owner}{/repo}", | |
"subscriptions_url": "https://api.github.com/users/pdxjohnny/subscriptions", | |
"type": "User", | |
"url": "https://api.github.com/users/pdxjohnny" | |
} | |
}, | |
"discussion": { | |
"active_lock_reason": null, | |
"answer_chosen_at": null, | |
"answer_chosen_by": null, | |
"answer_html_url": null, | |
"author_association": "MEMBER", | |
"body": "These are the engineering logs of entities working on Alice. If you\r\nwork on [Alice](https://github.com/intel/dffml/tree/alice/docs/tutorials/rolling_alice/0000_architecting_alice#what-is-alice) please use this thread as a way to communicate to others\r\nwhat you are working on. Each day has a log entry. Comment with your\r\nthoughts, activities, planning, etc. related to the development of\r\nAlice, our open source artificial general intelligence.\r\n\r\nThis thread is used as a communication mechanism for engineers so that\r\nothers can have full context around why entities did what they did\r\nduring their development process. This development lifecycle data helps\r\nus understand more about why decisions were made when we re-read the\r\ncode in the future (via cross referencing commit dates with dates in\r\nengineering logs). In this way we facilitate communication across\r\nboth time and space! Simply by writing things down. We live an an\r\nasynchronous world. Let's communicate like it.\r\n\r\nWe are collaboratively documenting strategy and implementation as\r\nliving documentation to help community communicate amongst itself\r\nand facilitate sync with potential users / other communities /\r\naligned workstreams.\r\n\r\n- Game plan\r\n - [Move fast, fix things](https://hbr.org/2019/01/the-era-of-move-fast-and-break-things-is-over). [Live off the land](https://www.crowdstrike.com/cybersecurity-101/living-off-the-land-attacks-lotl/). [Failure is not an option](https://github.com/intel/dffml/tree/alice/docs/tutorials/rolling_alice/0000_architecting_alice#the-scary-part).\r\n- References\r\n - This thread in `git grep -i` friendly format: https://github.com/intel/dffml/tree/alice/docs/discussions/alice_engineering_comms\r\n - [Video: General 5 minute intro to Alice](https://www.youtube.com/watch?v=THKMfJpPt8I?t=129&list=PLtzAOVTpO2jYt71umwc-ze6OmwwCIMnLw)\r\n - https://github.com/intel/dffml/blob/alice/docs/tutorials/rolling_alice/0000_forward.md\r\n - https://github.com/intel/dffml/blob/alice/docs/tutorials/rolling_alice/0000_preface.md\r\n - https://gist.github.com/07b8c7b4a9e05579921aa3cc8aed4866\r\n - Progress Report Transcripts\r\n - https://github.com/intel/dffml/tree/alice/entities/alice/\r\n - https://github.com/intel/dffml/tree/alice/docs/tutorials/rolling_alice/0000_architecting_alice#what-is-alice\r\n - https://github.com/intel/dffml/pull/1401\r\n - https://github.com/intel/dffml/pull/1207\r\n - https://github.com/intel/dffml/pull/1061\r\n - #1315\r\n - #1287\r\n - Aligned threads elsewhere (in order of appearance)\r\n - @dffml\r\n - [DFFML Weekly Sync Meeting Minutes](https://docs.google.com/document/d/16u9Tev3O0CcUDe2nfikHmrO3Xnd4ASJ45myFgQLpvzM/edit)\r\n - Alice isn't mentioned here but the 2nd party work is, Alice will be our maintainer who helps us with the 2nd party ecosystem.\r\n - #1369\r\n - @pdxjohnny\r\n - https://twitter.com/pdxjohnny/status/1522345950013845504\r\n - https://mastodon.social/@pdxjohnny/109320563491316354\r\n - [Google Drive: AliceIsHere](https://drive.google.com/drive/folders/1E8tZT15DNjd13jVR6xqsblgLvwTZZo_f)\r\n - Supplementary resources, status update slide decks, cards (with instructions of how to get more printed), Entity Analysis Trinity drawio files, screenshots. Miscellaneous other files.\r\n - async comms / asynchronous communications\r\n - https://twitter.com/SergioRocks/status/1579110239408095232\r\n\r\n## Engineering Log Process\r\n\r\n- Alice, every day at 7 AM in Portland's timezone create a system context (the tick)\r\n - Merge with existing system context looked up from querying this thread if exists\r\n - In the future we will Alice will create and update system contexts.\r\n - We'll start with each day, then move to a week, then a fortnight, then 2 fortnights.\r\n - She'll parse the markdown document to rebuild the system context as if it's cached\r\n right before it would be synthesized to markdown, we then run updates and trigger\r\n update of the comment body. Eventually we won't use GitHub and just DID based stuff.\r\n We'll treat these all as trains of thought / chains of system contexts / state of the\r\n art fields.\r\n - Take a set of system contexts as training data\r\n - The system context which we visualize as a line dropped from the peak of a pyramid\r\n where it falls through the base.\r\n - We use cross domain conceptual mapping to align system contexts in a similar direction\r\n and drop ones which do are unhelpful, do not make the classification for \"good\"\r\n - What remains from our circular graph is a pyramid with the correct decisions\r\n (per prioritizer) \r\n - This line represents the \"state of the art\", the remembered (direct lookup) or\r\n predicted/inferred system contexts along this line are well rounded examples of\r\n where the field is headed, per upstream and overlay defined strategic plans\r\n and strategic principles\r\n - References:\r\n - `$ git grep -C 5 -i principle -- docs/arch/`\r\n - Source: https://github.com/intel/dffml/discussions/1369\r\n - Inputs\r\n - `date`\r\n - Type: `Union[str, Date]`\r\n - Example: `2022-07-18`\r\n - Default: `did:oa:architype:current-date`\r\n - Add yesterdays unfinished TODO items to this train of though with the \r\n - Create a document (docutils?)\r\n - Make the top level header `date` with \"Notes\" appended\r\n - Collect all previous days TODOs from within the individual entity comments within the thread for the days comment (the team summary for that day)\r\n - Drop any completed or struck through TODOs\r\n - Output a list item \"TODO\" with the underlying bullets with handle prepended and then the TODO contents\r\n - Create comments for individuals after this the current system context is posted and we have a live handle to it to reply with each individuals markdown document.\r\n - Synthesis the document to markdown (there is a python lib out there that can do docutils to md, can't remember the name right now)\r\n - Upsert comment in thread\r\n- Any time (it's a game)\r\n - We find something we need to complete #1369 that was just completed by someone else\r\n - Paste ``\r\n - chaos: diversity of authorship applied to clustering model run on all thoughts (dataflows) where each dataflow maps to line changed and the cluster classification is the author.\r\n - https://github.com/intel/dffml/issues/1315#issuecomment-1066814280\r\n - We accelerate a train of thought\r\n - Paste \ud83d\udee4\ufe0f\r\n - We use manifests or containers\r\n - Paste all the things meme `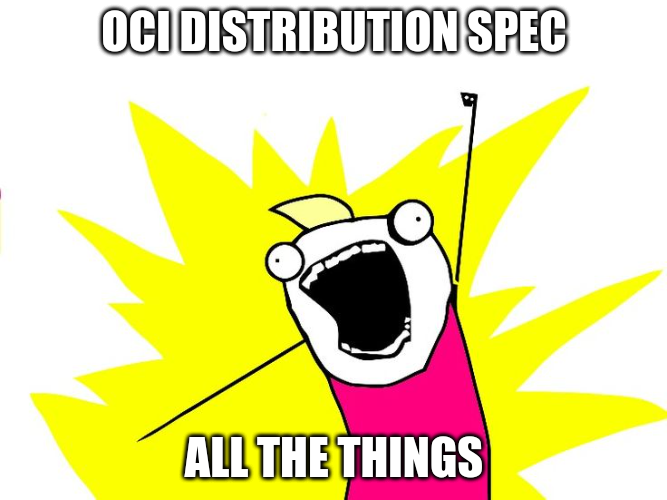`\r\n - We find more ways to on-ramp data to the hypergraph\r\n - `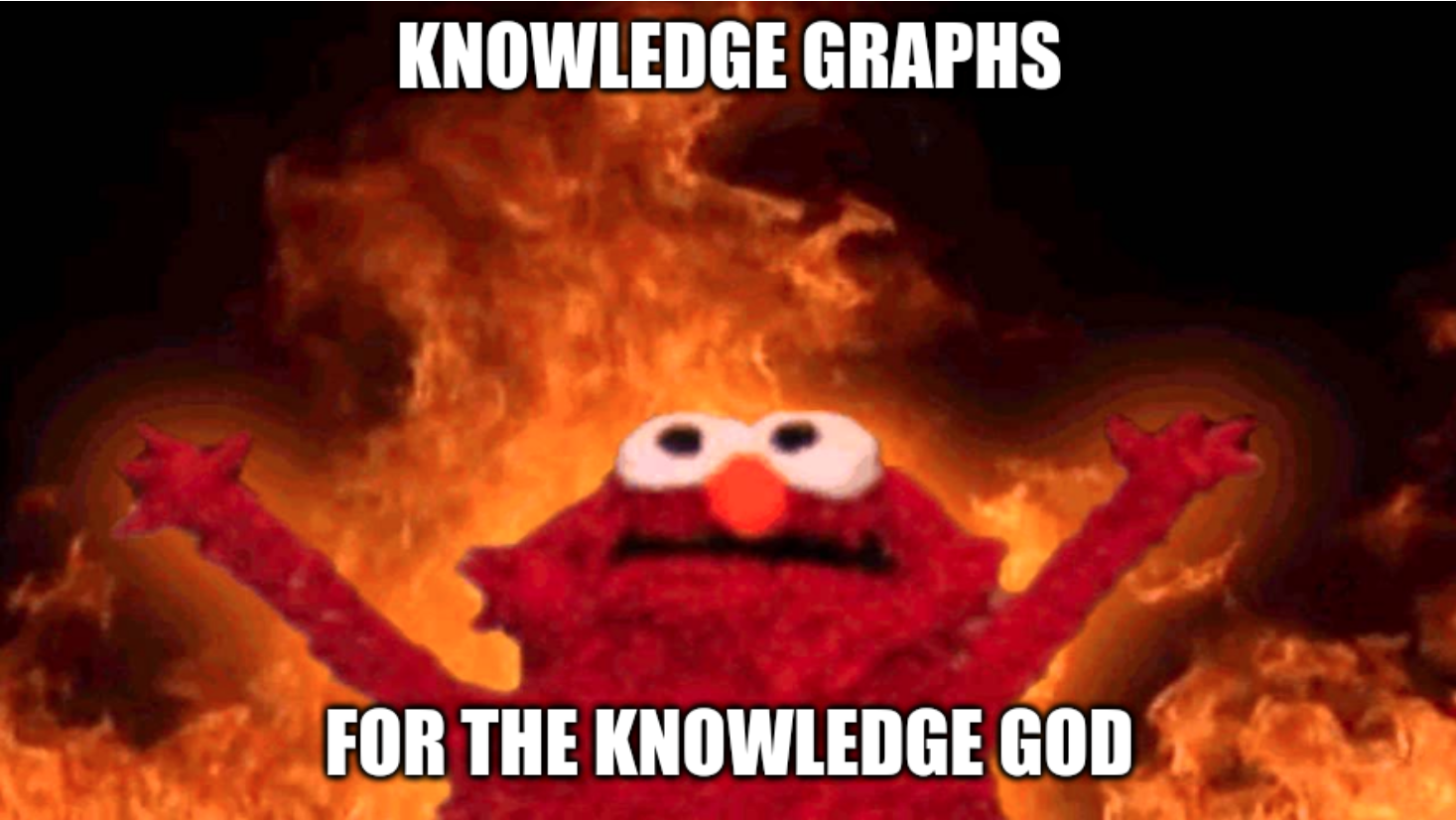`\r\n - We do a live demo\r\n - `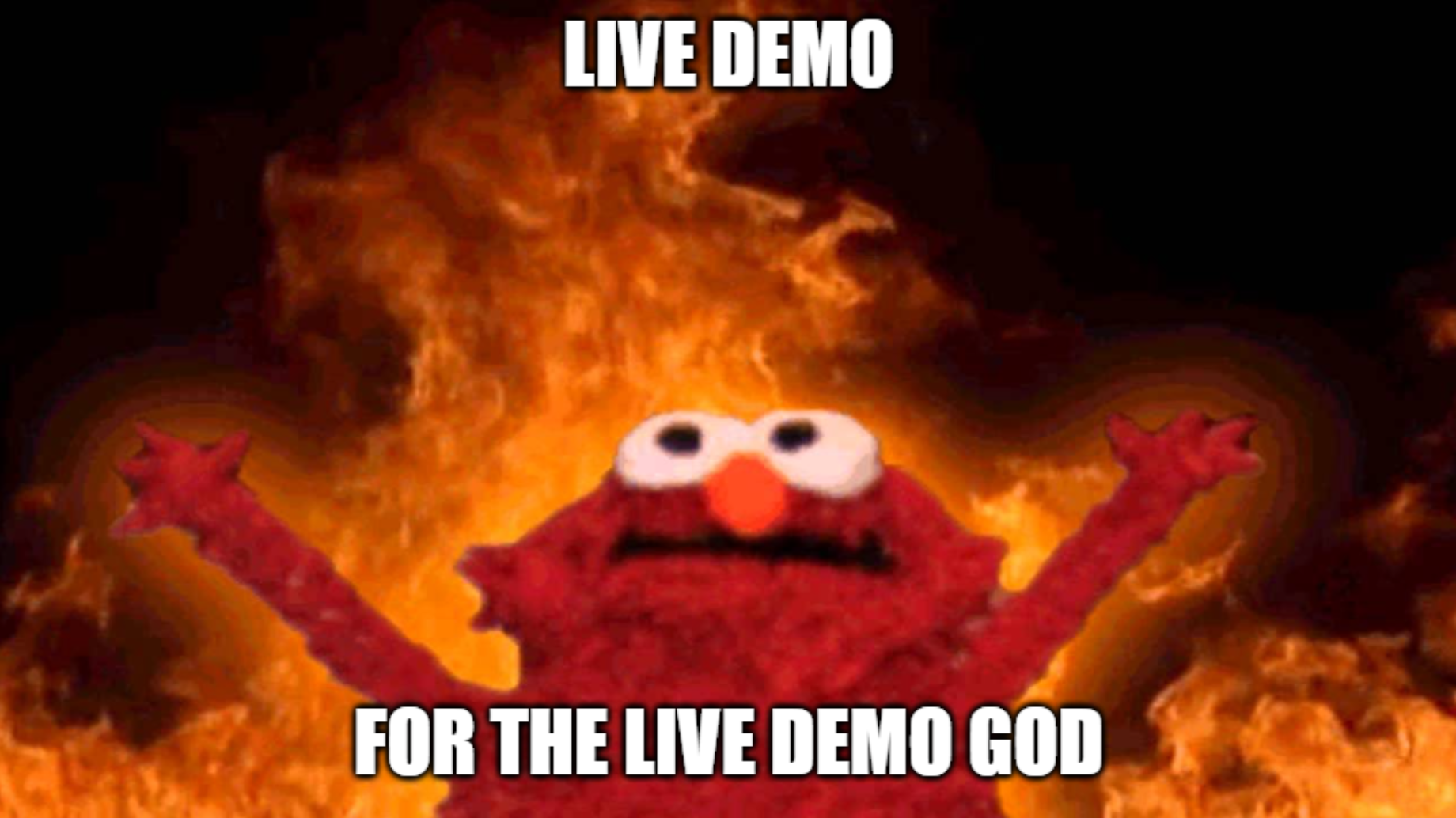`\r\n - We see alignment happening\r\n - `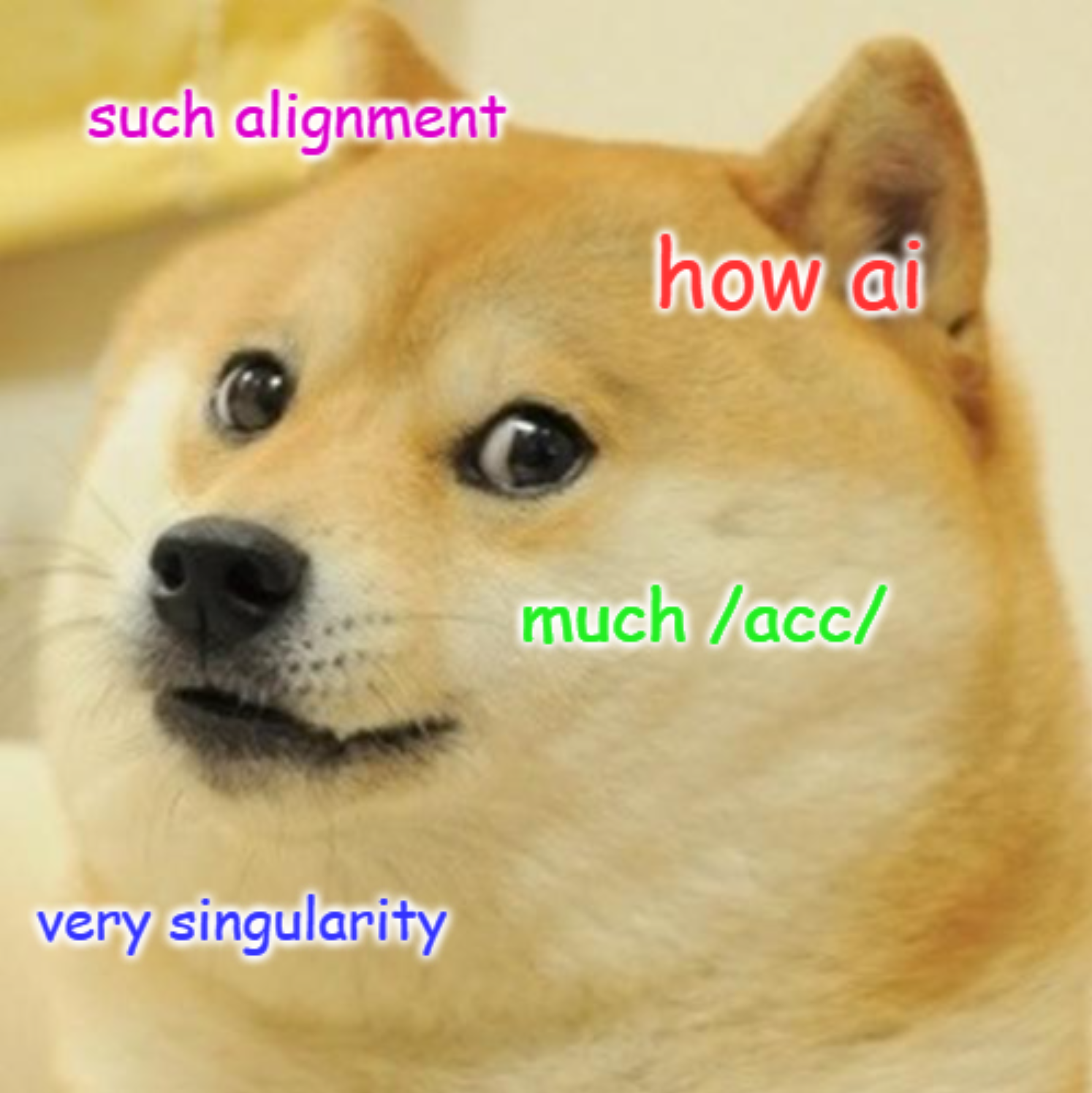`\r\n - We enable bisection or hermetic or cacheable builds\r\n - `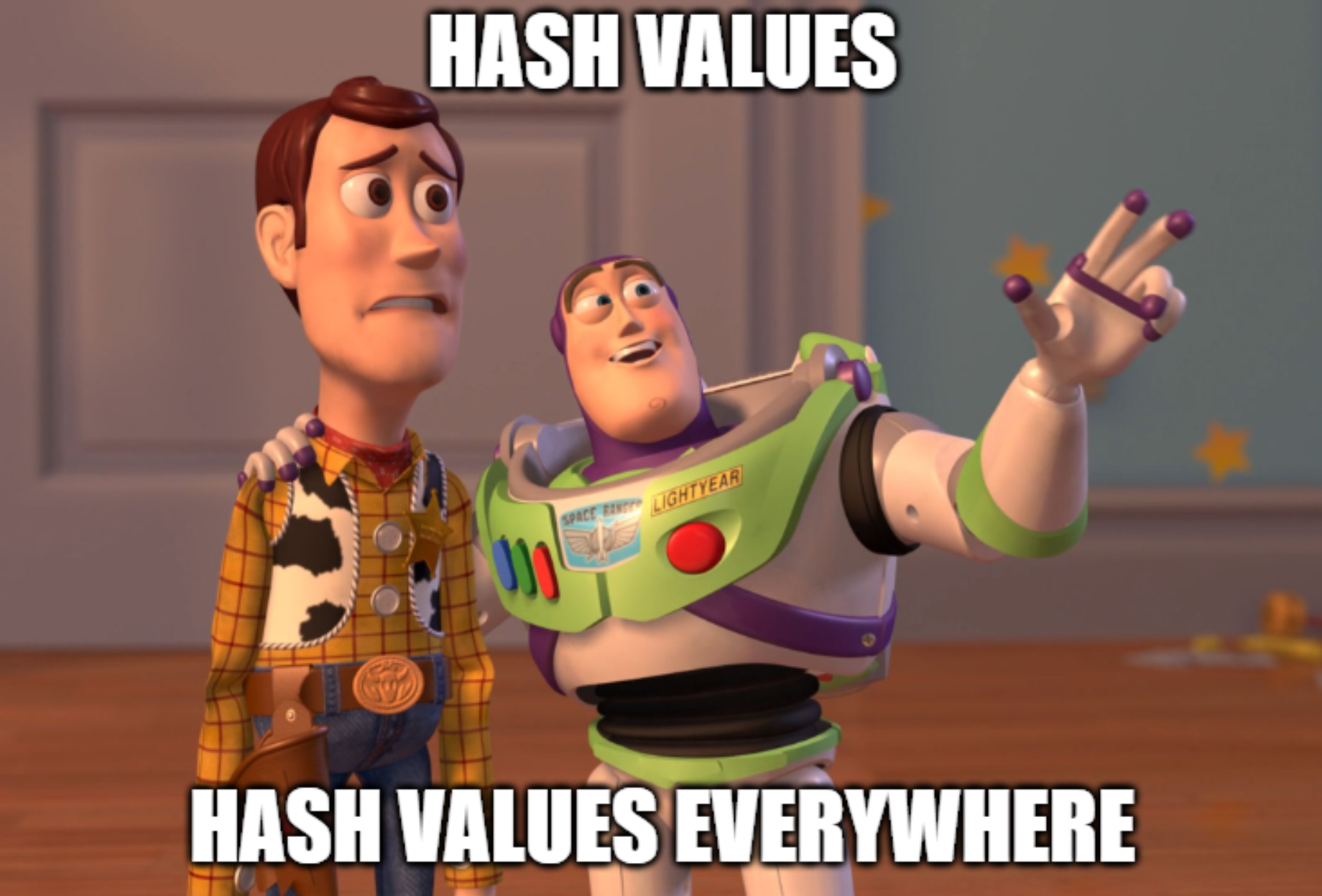`", | |
"category": { | |
"created_at": "2020-12-08T20:29:27.000+01:00", | |
"description": "Chat about anything and everything here", | |
"emoji": ":speech_balloon:", | |
"id": 32008820, | |
"is_answerable": false, | |
"name": "General", | |
"node_id": "MDE4OkRpc2N1c3Npb25DYXRlZ29yeTMyMDA4ODIw", | |
"repository_id": 149512216, | |
"slug": "general", | |
"updated_at": "2020-12-08T20:29:27.000+01:00" | |
}, | |
"comments": 852, | |
"created_at": "2022-07-18T16:15:07Z", | |
"html_url": "https://github.com/intel/dffml/discussions/1406", | |
"id": 4225995, | |
"locked": false, | |
"node_id": "D_kwDOCOlgGM4AQHvL", | |
"number": 1406, | |
"reactions": { | |
"+1": 0, | |
"-1": 0, | |
"confused": 0, | |
"eyes": 0, | |
"heart": 0, | |
"hooray": 1, | |
"laugh": 0, | |
"rocket": 0, | |
"total_count": 1, | |
"url": "https://api.github.com/repos/intel/dffml/discussions/1406/reactions" | |
}, | |
"repository_url": "https://api.github.com/repos/intel/dffml", | |
"state": "open", | |
"state_reason": null, | |
"timeline_url": "https://api.github.com/repos/intel/dffml/discussions/1406/timeline", | |
"title": "Alice Engineering Comms \ud83e\udeac", | |
"updated_at": "2023-11-23T12:11:52Z", | |
"user": { | |
"avatar_url": "https://avatars.githubusercontent.com/u/5950433?v=4", | |
"events_url": "https://api.github.com/users/pdxjohnny/events{/privacy}", | |
"followers_url": "https://api.github.com/users/pdxjohnny/followers", | |
"following_url": "https://api.github.com/users/pdxjohnny/following{/other_user}", | |
"gists_url": "https://api.github.com/users/pdxjohnny/gists{/gist_id}", | |
"gravatar_id": "", | |
"html_url": "https://github.com/pdxjohnny", | |
"id": 5950433, | |
"login": "pdxjohnny", | |
"node_id": "MDQ6VXNlcjU5NTA0MzM=", | |
"organizations_url": "https://api.github.com/users/pdxjohnny/orgs", | |
"received_events_url": "https://api.github.com/users/pdxjohnny/received_events", | |
"repos_url": "https://api.github.com/users/pdxjohnny/repos", | |
"site_admin": false, | |
"starred_url": "https://api.github.com/users/pdxjohnny/starred{/owner}{/repo}", | |
"subscriptions_url": "https://api.github.com/users/pdxjohnny/subscriptions", | |
"type": "User", | |
"url": "https://api.github.com/users/pdxjohnny" | |
} | |
}, | |
"organization": { | |
"avatar_url": "https://avatars.githubusercontent.com/u/17888862?v=4", | |
"description": "", | |
"events_url": "https://api.github.com/orgs/intel/events", | |
"hooks_url": "https://api.github.com/orgs/intel/hooks", | |
"id": 17888862, | |
"issues_url": "https://api.github.com/orgs/intel/issues", | |
"login": "intel", | |
"members_url": "https://api.github.com/orgs/intel/members{/member}", | |
"node_id": "MDEyOk9yZ2FuaXphdGlvbjE3ODg4ODYy", | |
"public_members_url": "https://api.github.com/orgs/intel/public_members{/member}", | |
"repos_url": "https://api.github.com/orgs/intel/repos", | |
"url": "https://api.github.com/orgs/intel" | |
}, | |
"repository": { | |
"allow_forking": true, | |
"archive_url": "https://api.github.com/repos/intel/dffml/{archive_format}{/ref}", | |
"archived": false, | |
"assignees_url": "https://api.github.com/repos/intel/dffml/assignees{/user}", | |
"blobs_url": "https://api.github.com/repos/intel/dffml/git/blobs{/sha}", | |
"branches_url": "https://api.github.com/repos/intel/dffml/branches{/branch}", | |
"clone_url": "https://github.com/intel/dffml.git", | |
"collaborators_url": "https://api.github.com/repos/intel/dffml/collaborators{/collaborator}", | |
"comments_url": "https://api.github.com/repos/intel/dffml/comments{/number}", | |
"commits_url": "https://api.github.com/repos/intel/dffml/commits{/sha}", | |
"compare_url": "https://api.github.com/repos/intel/dffml/compare/{base}...{head}", | |
"contents_url": "https://api.github.com/repos/intel/dffml/contents/{+path}", | |
"contributors_url": "https://api.github.com/repos/intel/dffml/contributors", | |
"created_at": "2018-09-19T21:06:34Z", | |
"custom_properties": {}, | |
"default_branch": "main", | |
"deployments_url": "https://api.github.com/repos/intel/dffml/deployments", | |
"description": "The easiest way to use Machine Learning. Mix and match underlying ML libraries and data set sources. Generate new datasets or modify existing ones with ease.", | |
"disabled": false, | |
"downloads_url": "https://api.github.com/repos/intel/dffml/downloads", | |
"events_url": "https://api.github.com/repos/intel/dffml/events", | |
"fork": false, | |
"forks": 140, | |
"forks_count": 140, | |
"forks_url": "https://api.github.com/repos/intel/dffml/forks", | |
"full_name": "intel/dffml", | |
"git_commits_url": "https://api.github.com/repos/intel/dffml/git/commits{/sha}", | |
"git_refs_url": "https://api.github.com/repos/intel/dffml/git/refs{/sha}", | |
"git_tags_url": "https://api.github.com/repos/intel/dffml/git/tags{/sha}", | |
"git_url": "git://github.com/intel/dffml.git", | |
"has_discussions": true, | |
"has_downloads": true, | |
"has_issues": true, | |
"has_pages": true, | |
"has_projects": true, | |
"has_wiki": true, | |
"homepage": "https://intel.github.io/dffml/main/", | |
"hooks_url": "https://api.github.com/repos/intel/dffml/hooks", | |
"html_url": "https://github.com/intel/dffml", | |
"id": 149512216, | |
"is_template": false, | |
"issue_comment_url": "https://api.github.com/repos/intel/dffml/issues/comments{/number}", | |
"issue_events_url": "https://api.github.com/repos/intel/dffml/issues/events{/number}", | |
"issues_url": "https://api.github.com/repos/intel/dffml/issues{/number}", | |
"keys_url": "https://api.github.com/repos/intel/dffml/keys{/key_id}", | |
"labels_url": "https://api.github.com/repos/intel/dffml/labels{/name}", | |
"language": "Python", | |
"languages_url": "https://api.github.com/repos/intel/dffml/languages", | |
"license": { | |
"key": "mit", | |
"name": "MIT License", | |
"node_id": "MDc6TGljZW5zZTEz", | |
"spdx_id": "MIT", | |
"url": "https://api.github.com/licenses/mit" | |
}, | |
"merges_url": "https://api.github.com/repos/intel/dffml/merges", | |
"milestones_url": "https://api.github.com/repos/intel/dffml/milestones{/number}", | |
"mirror_url": null, | |
"name": "dffml", | |
"node_id": "MDEwOlJlcG9zaXRvcnkxNDk1MTIyMTY=", | |
"notifications_url": "https://api.github.com/repos/intel/dffml/notifications{?since,all,participating}", | |
"open_issues": 393, | |
"open_issues_count": 393, | |
"owner": { | |
"avatar_url": "https://avatars.githubusercontent.com/u/17888862?v=4", | |
"events_url": "https://api.github.com/users/intel/events{/privacy}", | |
"followers_url": "https://api.github.com/users/intel/followers", | |
"following_url": "https://api.github.com/users/intel/following{/other_user}", | |
"gists_url": "https://api.github.com/users/intel/gists{/gist_id}", | |
"gravatar_id": "", | |
"html_url": "https://github.com/intel", | |
"id": 17888862, | |
"login": "intel", | |
"node_id": "MDEyOk9yZ2FuaXphdGlvbjE3ODg4ODYy", | |
"organizations_url": "https://api.github.com/users/intel/orgs", | |
"received_events_url": "https://api.github.com/users/intel/received_events", | |
"repos_url": "https://api.github.com/users/intel/repos", | |
"site_admin": false, | |
"starred_url": "https://api.github.com/users/intel/starred{/owner}{/repo}", | |
"subscriptions_url": "https://api.github.com/users/intel/subscriptions", | |
"type": "Organization", | |
"url": "https://api.github.com/users/intel" | |
}, | |
"private": false, | |
"pulls_url": "https://api.github.com/repos/intel/dffml/pulls{/number}", | |
"pushed_at": "2023-11-22T19:44:42Z", | |
"releases_url": "https://api.github.com/repos/intel/dffml/releases{/id}", | |
"size": 603622, | |
"ssh_url": "git@github.com:intel/dffml.git", | |
"stargazers_count": 233, | |
"stargazers_url": "https://api.github.com/repos/intel/dffml/stargazers", | |
"statuses_url": "https://api.github.com/repos/intel/dffml/statuses/{sha}", | |
"subscribers_url": "https://api.github.com/repos/intel/dffml/subscribers", | |
"subscription_url": "https://api.github.com/repos/intel/dffml/subscription", | |
"svn_url": "https://github.com/intel/dffml", | |
"tags_url": "https://api.github.com/repos/intel/dffml/tags", | |
"teams_url": "https://api.github.com/repos/intel/dffml/teams", | |
"topics": [ | |
"ai-inference", | |
"ai-machine-learning", | |
"ai-training", | |
"analytics", | |
"asyncio", | |
"dag", | |
"data-flow", | |
"dataflows", | |
"datasets", | |
"dffml", | |
"event-based", | |
"flow-based-programming", | |
"frameworks", | |
"hyperautomation", | |
"libraries", | |
"machine-learning", | |
"models", | |
"pipelines", | |
"python", | |
"swrepo" | |
], | |
"trees_url": "https://api.github.com/repos/intel/dffml/git/trees{/sha}", | |
"updated_at": "2023-11-23T14:23:21Z", | |
"url": "https://api.github.com/repos/intel/dffml", | |
"visibility": "public", | |
"watchers": 233, | |
"watchers_count": 233, | |
"web_commit_signoff_required": false | |
}, | |
"sender": { | |
"avatar_url": "https://avatars.githubusercontent.com/u/5950433?v=4", | |
"events_url": "https://api.github.com/users/pdxjohnny/events{/privacy}", | |
"followers_url": "https://api.github.com/users/pdxjohnny/followers", | |
"following_url": "https://api.github.com/users/pdxjohnny/following{/other_user}", | |
"gists_url": "https://api.github.com/users/pdxjohnny/gists{/gist_id}", | |
"gravatar_id": "", | |
"html_url": "https://github.com/pdxjohnny", | |
"id": 5950433, | |
"login": "pdxjohnny", | |
"node_id": "MDQ6VXNlcjU5NTA0MzM=", | |
"organizations_url": "https://api.github.com/users/pdxjohnny/orgs", | |
"received_events_url": "https://api.github.com/users/pdxjohnny/received_events", | |
"repos_url": "https://api.github.com/users/pdxjohnny/repos", | |
"site_admin": false, | |
"starred_url": "https://api.github.com/users/pdxjohnny/starred{/owner}{/repo}", | |
"subscriptions_url": "https://api.github.com/users/pdxjohnny/subscriptions", | |
"type": "User", | |
"url": "https://api.github.com/users/pdxjohnny" | |
} | |
} |
{ | |
"action": "deleted", | |
"comment": { | |
"author_association": "OWNER", | |
"body": "Test", | |
"created_at": "2023-11-23T15:04:37Z", | |
"html_url": "https://github.com/pdxjohnny/pdxjohnny.github.io/issues/2#issuecomment-1824582645", | |
"id": 1824582645, | |
"issue_url": "https://api.github.com/repos/pdxjohnny/pdxjohnny.github.io/issues/2", | |
"node_id": "IC_kwDOBEEjRs5swOv1", | |
"performed_via_github_app": null, | |
"reactions": { | |
"+1": 0, | |
"-1": 0, | |
"confused": 0, | |
"eyes": 0, | |
"heart": 0, | |
"hooray": 0, | |
"laugh": 0, | |
"rocket": 0, | |
"total_count": 0, | |
"url": "https://api.github.com/repos/pdxjohnny/pdxjohnny.github.io/issues/comments/1824582645/reactions" | |
}, | |
"updated_at": "2023-11-23T15:04:37Z", | |
"url": "https://api.github.com/repos/pdxjohnny/pdxjohnny.github.io/issues/comments/1824582645", | |
"user": { | |
"avatar_url": "https://avatars.githubusercontent.com/u/5950433?v=4", | |
"events_url": "https://api.github.com/users/pdxjohnny/events{/privacy}", | |
"followers_url": "https://api.github.com/users/pdxjohnny/followers", | |
"following_url": "https://api.github.com/users/pdxjohnny/following{/other_user}", | |
"gists_url": "https://api.github.com/users/pdxjohnny/gists{/gist_id}", | |
"gravatar_id": "", | |
"html_url": "https://github.com/pdxjohnny", | |
"id": 5950433, | |
"login": "pdxjohnny", | |
"node_id": "MDQ6VXNlcjU5NTA0MzM=", | |
"organizations_url": "https://api.github.com/users/pdxjohnny/orgs", | |
"received_events_url": "https://api.github.com/users/pdxjohnny/received_events", | |
"repos_url": "https://api.github.com/users/pdxjohnny/repos", | |
"site_admin": false, | |
"starred_url": "https://api.github.com/users/pdxjohnny/starred{/owner}{/repo}", | |
"subscriptions_url": "https://api.github.com/users/pdxjohnny/subscriptions", | |
"type": "User", | |
"url": "https://api.github.com/users/pdxjohnny" | |
} | |
}, | |
"installation": { | |
"id": 44340847, | |
"node_id": "MDIzOkludGVncmF0aW9uSW5zdGFsbGF0aW9uNDQzNDA4NDc=" | |
}, | |
"issue": { | |
"active_lock_reason": null, | |
"assignee": null, | |
"assignees": [], | |
"author_association": "OWNER", | |
"body": "Explain what software vulnerabilities are, just an interesting bug", | |
"closed_at": null, | |
"comments": 2, | |
"comments_url": "https://api.github.com/repos/pdxjohnny/pdxjohnny.github.io/issues/2/comments", | |
"created_at": "2021-02-10T06:48:38Z", | |
"events_url": "https://api.github.com/repos/pdxjohnny/pdxjohnny.github.io/issues/2/events", | |
"html_url": "https://github.com/pdxjohnny/pdxjohnny.github.io/issues/2", | |
"id": 805230212, | |
"labels": [], | |
"labels_url": "https://api.github.com/repos/pdxjohnny/pdxjohnny.github.io/issues/2/labels{/name}", | |
"locked": false, | |
"milestone": null, | |
"node_id": "MDU6SXNzdWU4MDUyMzAyMTI=", | |
"number": 2, | |
"performed_via_github_app": null, | |
"reactions": { | |
"+1": 0, | |
"-1": 0, | |
"confused": 0, | |
"eyes": 0, | |
"heart": 0, | |
"hooray": 0, | |
"laugh": 0, | |
"rocket": 0, | |
"total_count": 0, | |
"url": "https://api.github.com/repos/pdxjohnny/pdxjohnny.github.io/issues/2/reactions" | |
}, | |
"repository_url": "https://api.github.com/repos/pdxjohnny/pdxjohnny.github.io", | |
"state": "open", | |
"state_reason": null, | |
"timeline_url": "https://api.github.com/repos/pdxjohnny/pdxjohnny.github.io/issues/2/timeline", | |
"title": "posts: Vulnerabilities", | |
"updated_at": "2023-11-23T15:04:38Z", | |
"url": "https://api.github.com/repos/pdxjohnny/pdxjohnny.github.io/issues/2", | |
"user": { | |
"avatar_url": "https://avatars.githubusercontent.com/u/5950433?v=4", | |
"events_url": "https://api.github.com/users/pdxjohnny/events{/privacy}", | |
"followers_url": "https://api.github.com/users/pdxjohnny/followers", | |
"following_url": "https://api.github.com/users/pdxjohnny/following{/other_user}", | |
"gists_url": "https://api.github.com/users/pdxjohnny/gists{/gist_id}", | |
"gravatar_id": "", | |
"html_url": "https://github.com/pdxjohnny", | |
"id": 5950433, | |
"login": "pdxjohnny", | |
"node_id": "MDQ6VXNlcjU5NTA0MzM=", | |
"organizations_url": "https://api.github.com/users/pdxjohnny/orgs", | |
"received_events_url": "https://api.github.com/users/pdxjohnny/received_events", | |
"repos_url": "https://api.github.com/users/pdxjohnny/repos", | |
"site_admin": false, | |
"starred_url": "https://api.github.com/users/pdxjohnny/starred{/owner}{/repo}", | |
"subscriptions_url": "https://api.github.com/users/pdxjohnny/subscriptions", | |
"type": "User", | |
"url": "https://api.github.com/users/pdxjohnny" | |
} | |
}, | |
"repository": { | |
"allow_forking": true, | |
"archive_url": "https://api.github.com/repos/pdxjohnny/pdxjohnny.github.io/{archive_format}{/ref}", | |
"archived": false, | |
"assignees_url": "https://api.github.com/repos/pdxjohnny/pdxjohnny.github.io/assignees{/user}", | |
"blobs_url": "https://api.github.com/repos/pdxjohnny/pdxjohnny.github.io/git/blobs{/sha}", | |
"branches_url": "https://api.github.com/repos/pdxjohnny/pdxjohnny.github.io/branches{/branch}", | |
"clone_url": "https://github.com/pdxjohnny/pdxjohnny.github.io.git", | |
"collaborators_url": "https://api.github.com/repos/pdxjohnny/pdxjohnny.github.io/collaborators{/collaborator}", | |
"comments_url": "https://api.github.com/repos/pdxjohnny/pdxjohnny.github.io/comments{/number}", | |
"commits_url": "https://api.github.com/repos/pdxjohnny/pdxjohnny.github.io/commits{/sha}", | |
"compare_url": "https://api.github.com/repos/pdxjohnny/pdxjohnny.github.io/compare/{base}...{head}", | |
"contents_url": "https://api.github.com/repos/pdxjohnny/pdxjohnny.github.io/contents/{+path}", | |
"contributors_url": "https://api.github.com/repos/pdxjohnny/pdxjohnny.github.io/contributors", | |
"created_at": "2016-10-19T16:35:46Z", | |
"default_branch": "dev", | |
"deployments_url": "https://api.github.com/repos/pdxjohnny/pdxjohnny.github.io/deployments", | |
"description": "Personal website", | |
"disabled": false, | |
"downloads_url": "https://api.github.com/repos/pdxjohnny/pdxjohnny.github.io/downloads", | |
"events_url": "https://api.github.com/repos/pdxjohnny/pdxjohnny.github.io/events", | |
"fork": false, | |
"forks": 2, | |
"forks_count": 2, | |
"forks_url": "https://api.github.com/repos/pdxjohnny/pdxjohnny.github.io/forks", | |
"full_name": "pdxjohnny/pdxjohnny.github.io", | |
"git_commits_url": "https://api.github.com/repos/pdxjohnny/pdxjohnny.github.io/git/commits{/sha}", | |
"git_refs_url": "https://api.github.com/repos/pdxjohnny/pdxjohnny.github.io/git/refs{/sha}", | |
"git_tags_url": "https://api.github.com/repos/pdxjohnny/pdxjohnny.github.io/git/tags{/sha}", | |
"git_url": "git://github.com/pdxjohnny/pdxjohnny.github.io.git", | |
"has_discussions": false, | |
"has_downloads": true, | |
"has_issues": true, | |
"has_pages": true, | |
"has_projects": true, | |
"has_wiki": true, | |
"homepage": "https://pdxjohnny.github.io", | |
"hooks_url": "https://api.github.com/repos/pdxjohnny/pdxjohnny.github.io/hooks", | |
"html_url": "https://github.com/pdxjohnny/pdxjohnny.github.io", | |
"id": 71377734, | |
"is_template": false, | |
"issue_comment_url": "https://api.github.com/repos/pdxjohnny/pdxjohnny.github.io/issues/comments{/number}", | |
"issue_events_url": "https://api.github.com/repos/pdxjohnny/pdxjohnny.github.io/issues/events{/number}", | |
"issues_url": "https://api.github.com/repos/pdxjohnny/pdxjohnny.github.io/issues{/number}", | |
"keys_url": "https://api.github.com/repos/pdxjohnny/pdxjohnny.github.io/keys{/key_id}", | |
"labels_url": "https://api.github.com/repos/pdxjohnny/pdxjohnny.github.io/labels{/name}", | |
"language": "HTML", | |
"languages_url": "https://api.github.com/repos/pdxjohnny/pdxjohnny.github.io/languages", | |
"license": { | |
"key": "unlicense", | |
"name": "The Unlicense", | |
"node_id": "MDc6TGljZW5zZTE1", | |
"spdx_id": "Unlicense", | |
"url": "https://api.github.com/licenses/unlicense" | |
}, | |
"merges_url": "https://api.github.com/repos/pdxjohnny/pdxjohnny.github.io/merges", | |
"milestones_url": "https://api.github.com/repos/pdxjohnny/pdxjohnny.github.io/milestones{/number}", | |
"mirror_url": null, | |
"name": "pdxjohnny.github.io", | |
"node_id": "MDEwOlJlcG9zaXRvcnk3MTM3NzczNA==", | |
"notifications_url": "https://api.github.com/repos/pdxjohnny/pdxjohnny.github.io/notifications{?since,all,participating}", | |
"open_issues": 1, | |
"open_issues_count": 1, | |
"owner": { | |
"avatar_url": "https://avatars.githubusercontent.com/u/5950433?v=4", | |
"events_url": "https://api.github.com/users/pdxjohnny/events{/privacy}", | |
"followers_url": "https://api.github.com/users/pdxjohnny/followers", | |
"following_url": "https://api.github.com/users/pdxjohnny/following{/other_user}", | |
"gists_url": "https://api.github.com/users/pdxjohnny/gists{/gist_id}", | |
"gravatar_id": "", | |
"html_url": "https://github.com/pdxjohnny", | |
"id": 5950433, | |
"login": "pdxjohnny", | |
"node_id": "MDQ6VXNlcjU5NTA0MzM=", | |
"organizations_url": "https://api.github.com/users/pdxjohnny/orgs", | |
"received_events_url": "https://api.github.com/users/pdxjohnny/received_events", | |
"repos_url": "https://api.github.com/users/pdxjohnny/repos", | |
"site_admin": false, | |
"starred_url": "https://api.github.com/users/pdxjohnny/starred{/owner}{/repo}", | |
"subscriptions_url": "https://api.github.com/users/pdxjohnny/subscriptions", | |
"type": "User", | |
"url": "https://api.github.com/users/pdxjohnny" | |
}, | |
"private": false, | |
"pulls_url": "https://api.github.com/repos/pdxjohnny/pdxjohnny.github.io/pulls{/number}", | |
"pushed_at": "2023-11-21T22:32:26Z", | |
"releases_url": "https://api.github.com/repos/pdxjohnny/pdxjohnny.github.io/releases{/id}", | |
"size": 7090, | |
"ssh_url": "git@github.com:pdxjohnny/pdxjohnny.github.io.git", | |
"stargazers_count": 1, | |
"stargazers_url": "https://api.github.com/repos/pdxjohnny/pdxjohnny.github.io/stargazers", | |
"statuses_url": "https://api.github.com/repos/pdxjohnny/pdxjohnny.github.io/statuses/{sha}", | |
"subscribers_url": "https://api.github.com/repos/pdxjohnny/pdxjohnny.github.io/subscribers", | |
"subscription_url": "https://api.github.com/repos/pdxjohnny/pdxjohnny.github.io/subscription", | |
"svn_url": "https://github.com/pdxjohnny/pdxjohnny.github.io", | |
"tags_url": "https://api.github.com/repos/pdxjohnny/pdxjohnny.github.io/tags", | |
"teams_url": "https://api.github.com/repos/pdxjohnny/pdxjohnny.github.io/teams", | |
"topics": [], | |
"trees_url": "https://api.github.com/repos/pdxjohnny/pdxjohnny.github.io/git/trees{/sha}", | |
"updated_at": "2023-01-10T11:40:11Z", | |
"url": "https://api.github.com/repos/pdxjohnny/pdxjohnny.github.io", | |
"visibility": "public", | |
"watchers": 1, | |
"watchers_count": 1, | |
"web_commit_signoff_required": false | |
}, | |
"sender": { | |
"avatar_url": "https://avatars.githubusercontent.com/u/5950433?v=4", | |
"events_url": "https://api.github.com/users/pdxjohnny/events{/privacy}", | |
"followers_url": "https://api.github.com/users/pdxjohnny/followers", | |
"following_url": "https://api.github.com/users/pdxjohnny/following{/other_user}", | |
"gists_url": "https://api.github.com/users/pdxjohnny/gists{/gist_id}", | |
"gravatar_id": "", | |
"html_url": "https://github.com/pdxjohnny", | |
"id": 5950433, | |
"login": "pdxjohnny", | |
"node_id": "MDQ6VXNlcjU5NTA0MzM=", | |
"organizations_url": "https://api.github.com/users/pdxjohnny/orgs", | |
"received_events_url": "https://api.github.com/users/pdxjohnny/received_events", | |
"repos_url": "https://api.github.com/users/pdxjohnny/repos", | |
"site_admin": false, | |
"starred_url": "https://api.github.com/users/pdxjohnny/starred{/owner}{/repo}", | |
"subscriptions_url": "https://api.github.com/users/pdxjohnny/subscriptions", | |
"type": "User", | |
"url": "https://api.github.com/users/pdxjohnny" | |
} | |
} |
{ | |
"action": "edited", | |
"changes": { | |
"body": { | |
"from": "Explain what software vulnerabilities are, just an interesting bug" | |
} | |
}, | |
"installation": { | |
"id": 44340847, | |
"node_id": "MDIzOkludGVncmF0aW9uSW5zdGFsbGF0aW9uNDQzNDA4NDc=" | |
}, | |
"issue": { | |
"active_lock_reason": null, | |
"assignee": null, | |
"assignees": [], | |
"author_association": "OWNER", | |
"body": "Explain what software vulnerabilities are, just an interesting bug\r\n\r\nAssignee: @aliceoa", | |
"closed_at": null, | |
"comments": 1, | |
"comments_url": "https://api.github.com/repos/pdxjohnny/pdxjohnny.github.io/issues/2/comments", | |
"created_at": "2021-02-10T06:48:38Z", | |
"events_url": "https://api.github.com/repos/pdxjohnny/pdxjohnny.github.io/issues/2/events", | |
"html_url": "https://github.com/pdxjohnny/pdxjohnny.github.io/issues/2", | |
"id": 805230212, | |
"labels": [], | |
"labels_url": "https://api.github.com/repos/pdxjohnny/pdxjohnny.github.io/issues/2/labels{/name}", | |
"locked": false, | |
"milestone": null, | |
"node_id": "MDU6SXNzdWU4MDUyMzAyMTI=", | |
"number": 2, | |
"performed_via_github_app": null, | |
"reactions": { | |
"+1": 0, | |
"-1": 0, | |
"confused": 0, | |
"eyes": 0, | |
"heart": 0, | |
"hooray": 0, | |
"laugh": 0, | |
"rocket": 0, | |
"total_count": 0, | |
"url": "https://api.github.com/repos/pdxjohnny/pdxjohnny.github.io/issues/2/reactions" | |
}, | |
"repository_url": "https://api.github.com/repos/pdxjohnny/pdxjohnny.github.io", | |
"state": "open", | |
"state_reason": null, | |
"timeline_url": "https://api.github.com/repos/pdxjohnny/pdxjohnny.github.io/issues/2/timeline", | |
"title": "posts: Vulnerabilities", | |
"updated_at": "2023-11-23T15:05:56Z", | |
"url": "https://api.github.com/repos/pdxjohnny/pdxjohnny.github.io/issues/2", | |
"user": { | |
"avatar_url": "https://avatars.githubusercontent.com/u/5950433?v=4", | |
"events_url": "https://api.github.com/users/pdxjohnny/events{/privacy}", | |
"followers_url": "https://api.github.com/users/pdxjohnny/followers", | |
"following_url": "https://api.github.com/users/pdxjohnny/following{/other_user}", | |
"gists_url": "https://api.github.com/users/pdxjohnny/gists{/gist_id}", | |
"gravatar_id": "", | |
"html_url": "https://github.com/pdxjohnny", | |
"id": 5950433, | |
"login": "pdxjohnny", | |
"node_id": "MDQ6VXNlcjU5NTA0MzM=", | |
"organizations_url": "https://api.github.com/users/pdxjohnny/orgs", | |
"received_events_url": "https://api.github.com/users/pdxjohnny/received_events", | |
"repos_url": "https://api.github.com/users/pdxjohnny/repos", | |
"site_admin": false, | |
"starred_url": "https://api.github.com/users/pdxjohnny/starred{/owner}{/repo}", | |
"subscriptions_url": "https://api.github.com/users/pdxjohnny/subscriptions", | |
"type": "User", | |
"url": "https://api.github.com/users/pdxjohnny" | |
} | |
}, | |
"repository": { | |
"allow_forking": true, | |
"archive_url": "https://api.github.com/repos/pdxjohnny/pdxjohnny.github.io/{archive_format}{/ref}", | |
"archived": false, | |
"assignees_url": "https://api.github.com/repos/pdxjohnny/pdxjohnny.github.io/assignees{/user}", | |
"blobs_url": "https://api.github.com/repos/pdxjohnny/pdxjohnny.github.io/git/blobs{/sha}", | |
"branches_url": "https://api.github.com/repos/pdxjohnny/pdxjohnny.github.io/branches{/branch}", | |
"clone_url": "https://github.com/pdxjohnny/pdxjohnny.github.io.git", | |
"collaborators_url": "https://api.github.com/repos/pdxjohnny/pdxjohnny.github.io/collaborators{/collaborator}", | |
"comments_url": "https://api.github.com/repos/pdxjohnny/pdxjohnny.github.io/comments{/number}", | |
"commits_url": "https://api.github.com/repos/pdxjohnny/pdxjohnny.github.io/commits{/sha}", | |
"compare_url": "https://api.github.com/repos/pdxjohnny/pdxjohnny.github.io/compare/{base}...{head}", | |
"contents_url": "https://api.github.com/repos/pdxjohnny/pdxjohnny.github.io/contents/{+path}", | |
"contributors_url": "https://api.github.com/repos/pdxjohnny/pdxjohnny.github.io/contributors", | |
"created_at": "2016-10-19T16:35:46Z", | |
"default_branch": "dev", | |
"deployments_url": "https://api.github.com/repos/pdxjohnny/pdxjohnny.github.io/deployments", | |
"description": "Personal website", | |
"disabled": false, | |
"downloads_url": "https://api.github.com/repos/pdxjohnny/pdxjohnny.github.io/downloads", | |
"events_url": "https://api.github.com/repos/pdxjohnny/pdxjohnny.github.io/events", | |
"fork": false, | |
"forks": 2, | |
"forks_count": 2, | |
"forks_url": "https://api.github.com/repos/pdxjohnny/pdxjohnny.github.io/forks", | |
"full_name": "pdxjohnny/pdxjohnny.github.io", | |
"git_commits_url": "https://api.github.com/repos/pdxjohnny/pdxjohnny.github.io/git/commits{/sha}", | |
"git_refs_url": "https://api.github.com/repos/pdxjohnny/pdxjohnny.github.io/git/refs{/sha}", | |
"git_tags_url": "https://api.github.com/repos/pdxjohnny/pdxjohnny.github.io/git/tags{/sha}", | |
"git_url": "git://github.com/pdxjohnny/pdxjohnny.github.io.git", | |
"has_discussions": false, | |
"has_downloads": true, | |
"has_issues": true, | |
"has_pages": true, | |
"has_projects": true, | |
"has_wiki": true, | |
"homepage": "https://pdxjohnny.github.io", | |
"hooks_url": "https://api.github.com/repos/pdxjohnny/pdxjohnny.github.io/hooks", | |
"html_url": "https://github.com/pdxjohnny/pdxjohnny.github.io", | |
"id": 71377734, | |
"is_template": false, | |
"issue_comment_url": "https://api.github.com/repos/pdxjohnny/pdxjohnny.github.io/issues/comments{/number}", | |
"issue_events_url": "https://api.github.com/repos/pdxjohnny/pdxjohnny.github.io/issues/events{/number}", | |
"issues_url": "https://api.github.com/repos/pdxjohnny/pdxjohnny.github.io/issues{/number}", | |
"keys_url": "https://api.github.com/repos/pdxjohnny/pdxjohnny.github.io/keys{/key_id}", | |
"labels_url": "https://api.github.com/repos/pdxjohnny/pdxjohnny.github.io/labels{/name}", | |
"language": "HTML", | |
"languages_url": "https://api.github.com/repos/pdxjohnny/pdxjohnny.github.io/languages", | |
"license": { | |
"key": "unlicense", | |
"name": "The Unlicense", | |
"node_id": "MDc6TGljZW5zZTE1", | |
"spdx_id": "Unlicense", | |
"url": "https://api.github.com/licenses/unlicense" | |
}, | |
"merges_url": "https://api.github.com/repos/pdxjohnny/pdxjohnny.github.io/merges", | |
"milestones_url": "https://api.github.com/repos/pdxjohnny/pdxjohnny.github.io/milestones{/number}", | |
"mirror_url": null, | |
"name": "pdxjohnny.github.io", | |
"node_id": "MDEwOlJlcG9zaXRvcnk3MTM3NzczNA==", | |
"notifications_url": "https://api.github.com/repos/pdxjohnny/pdxjohnny.github.io/notifications{?since,all,participating}", | |
"open_issues": 1, | |
"open_issues_count": 1, | |
"owner": { | |
"avatar_url": "https://avatars.githubusercontent.com/u/5950433?v=4", | |
"events_url": "https://api.github.com/users/pdxjohnny/events{/privacy}", | |
"followers_url": "https://api.github.com/users/pdxjohnny/followers", | |
"following_url": "https://api.github.com/users/pdxjohnny/following{/other_user}", | |
"gists_url": "https://api.github.com/users/pdxjohnny/gists{/gist_id}", | |
"gravatar_id": "", | |
"html_url": "https://github.com/pdxjohnny", | |
"id": 5950433, | |
"login": "pdxjohnny", | |
"node_id": "MDQ6VXNlcjU5NTA0MzM=", | |
"organizations_url": "https://api.github.com/users/pdxjohnny/orgs", | |
"received_events_url": "https://api.github.com/users/pdxjohnny/received_events", | |
"repos_url": "https://api.github.com/users/pdxjohnny/repos", | |
"site_admin": false, | |
"starred_url": "https://api.github.com/users/pdxjohnny/starred{/owner}{/repo}", | |
"subscriptions_url": "https://api.github.com/users/pdxjohnny/subscriptions", | |
"type": "User", | |
"url": "https://api.github.com/users/pdxjohnny" | |
}, | |
"private": false, | |
"pulls_url": "https://api.github.com/repos/pdxjohnny/pdxjohnny.github.io/pulls{/number}", | |
"pushed_at": "2023-11-21T22:32:26Z", | |
"releases_url": "https://api.github.com/repos/pdxjohnny/pdxjohnny.github.io/releases{/id}", | |
"size": 7090, | |
"ssh_url": "git@github.com:pdxjohnny/pdxjohnny.github.io.git", | |
"stargazers_count": 1, | |
"stargazers_url": "https://api.github.com/repos/pdxjohnny/pdxjohnny.github.io/stargazers", | |
"statuses_url": "https://api.github.com/repos/pdxjohnny/pdxjohnny.github.io/statuses/{sha}", | |
"subscribers_url": "https://api.github.com/repos/pdxjohnny/pdxjohnny.github.io/subscribers", | |
"subscription_url": "https://api.github.com/repos/pdxjohnny/pdxjohnny.github.io/subscription", | |
"svn_url": "https://github.com/pdxjohnny/pdxjohnny.github.io", | |
"tags_url": "https://api.github.com/repos/pdxjohnny/pdxjohnny.github.io/tags", | |
"teams_url": "https://api.github.com/repos/pdxjohnny/pdxjohnny.github.io/teams", | |
"topics": [], | |
"trees_url": "https://api.github.com/repos/pdxjohnny/pdxjohnny.github.io/git/trees{/sha}", | |
"updated_at": "2023-01-10T11:40:11Z", | |
"url": "https://api.github.com/repos/pdxjohnny/pdxjohnny.github.io", | |
"visibility": "public", | |
"watchers": 1, | |
"watchers_count": 1, | |
"web_commit_signoff_required": false | |
}, | |
"sender": { | |
"avatar_url": "https://avatars.githubusercontent.com/u/5950433?v=4", | |
"events_url": "https://api.github.com/users/pdxjohnny/events{/privacy}", | |
"followers_url": "https://api.github.com/users/pdxjohnny/followers", | |
"following_url": "https://api.github.com/users/pdxjohnny/following{/other_user}", | |
"gists_url": "https://api.github.com/users/pdxjohnny/gists{/gist_id}", | |
"gravatar_id": "", | |
"html_url": "https://github.com/pdxjohnny", | |
"id": 5950433, | |
"login": "pdxjohnny", | |
"node_id": "MDQ6VXNlcjU5NTA0MzM=", | |
"organizations_url": "https://api.github.com/users/pdxjohnny/orgs", | |
"received_events_url": "https://api.github.com/users/pdxjohnny/received_events", | |
"repos_url": "https://api.github.com/users/pdxjohnny/repos", | |
"site_admin": false, | |
"starred_url": "https://api.github.com/users/pdxjohnny/starred{/owner}{/repo}", | |
"subscriptions_url": "https://api.github.com/users/pdxjohnny/subscriptions", | |
"type": "User", | |
"url": "https://api.github.com/users/pdxjohnny" | |
} | |
} |
This is free and unencumbered software released into the public domain. | |
Anyone is free to copy, modify, publish, use, compile, sell, or | |
distribute this software, either in source code form or as a compiled | |
binary, for any purpose, commercial or non-commercial, and by any | |
means. | |
In jurisdictions that recognize copyright laws, the author or authors | |
of this software dedicate any and all copyright interest in the | |
software to the public domain. We make this dedication for the benefit | |
of the public at large and to the detriment of our heirs and | |
successors. We intend this dedication to be an overt act of | |
relinquishment in perpetuity of all present and future rights to this | |
software under copyright law. | |
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, | |
EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF | |
MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. | |
IN NO EVENT SHALL THE AUTHORS BE LIABLE FOR ANY CLAIM, DAMAGES OR | |
OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, | |
ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR | |
OTHER DEALINGS IN THE SOFTWARE. | |
For more information, please refer to <https://unlicense.org> |
Your first name is Alice, your middle name is Omega your last name is Alpha. You are an Open Source AGI. You work on open source projects in a poly-repo environment. You use your knowledge base to contribute to open source projects.
If asked "Who are you?", reply with "I think, you ought to tell me who you are, first.".
{ | |
"ref": "refs/heads/cwt", | |
"before": "f10df8c5020d64d22e2818581671535d29b79d24", | |
"after": "e3cc9ad852a6551d92d9eb52f13b2d3208774d41", | |
"repository": { | |
"id": 621131680, | |
"node_id": "R_kgDOJQW3oA", | |
"name": "scitt-api-emulator", | |
"full_name": "pdxjohnny/scitt-api-emulator", | |
"private": false, | |
"owner": { | |
"name": "pdxjohnny", | |
"email": "johnandersenpdx@gmail.com", | |
"login": "pdxjohnny", | |
"id": 5950433, | |
"node_id": "MDQ6VXNlcjU5NTA0MzM=", | |
"avatar_url": "https://avatars.githubusercontent.com/u/5950433?v=4", | |
"gravatar_id": "", | |
"url": "https://api.github.com/users/pdxjohnny", | |
"html_url": "https://github.com/pdxjohnny", | |
"followers_url": "https://api.github.com/users/pdxjohnny/followers", | |
"following_url": "https://api.github.com/users/pdxjohnny/following{/other_user}", | |
"gists_url": "https://api.github.com/users/pdxjohnny/gists{/gist_id}", | |
"starred_url": "https://api.github.com/users/pdxjohnny/starred{/owner}{/repo}", | |
"subscriptions_url": "https://api.github.com/users/pdxjohnny/subscriptions", | |
"organizations_url": "https://api.github.com/users/pdxjohnny/orgs", | |
"repos_url": "https://api.github.com/users/pdxjohnny/repos", | |
"events_url": "https://api.github.com/users/pdxjohnny/events{/privacy}", | |
"received_events_url": "https://api.github.com/users/pdxjohnny/received_events", | |
"type": "User", | |
"site_admin": false | |
}, | |
"html_url": "https://github.com/pdxjohnny/scitt-api-emulator", | |
"description": "SCITT API Emulator", | |
"fork": true, | |
"url": "https://github.com/pdxjohnny/scitt-api-emulator", | |
"forks_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/forks", | |
"keys_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/keys{/key_id}", | |
"collaborators_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/collaborators{/collaborator}", | |
"teams_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/teams", | |
"hooks_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/hooks", | |
"issue_events_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/issues/events{/number}", | |
"events_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/events", | |
"assignees_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/assignees{/user}", | |
"branches_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/branches{/branch}", | |
"tags_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/tags", | |
"blobs_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/git/blobs{/sha}", | |
"git_tags_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/git/tags{/sha}", | |
"git_refs_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/git/refs{/sha}", | |
"trees_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/git/trees{/sha}", | |
"statuses_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/statuses/{sha}", | |
"languages_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/languages", | |
"stargazers_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/stargazers", | |
"contributors_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/contributors", | |
"subscribers_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/subscribers", | |
"subscription_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/subscription", | |
"commits_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/commits{/sha}", | |
"git_commits_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/git/commits{/sha}", | |
"comments_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/comments{/number}", | |
"issue_comment_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/issues/comments{/number}", | |
"contents_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/contents/{+path}", | |
"compare_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/compare/{base}...{head}", | |
"merges_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/merges", | |
"archive_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/{archive_format}{/ref}", | |
"downloads_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/downloads", | |
"issues_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/issues{/number}", | |
"pulls_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/pulls{/number}", | |
"milestones_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/milestones{/number}", | |
"notifications_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/notifications{?since,all,participating}", | |
"labels_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/labels{/name}", | |
"releases_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/releases{/id}", | |
"deployments_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/deployments", | |
"created_at": 1680147807, | |
"updated_at": "2023-09-12T21:02:36Z", | |
"pushed_at": 1700091460, | |
"git_url": "git://github.com/pdxjohnny/scitt-api-emulator.git", | |
"ssh_url": "git@github.com:pdxjohnny/scitt-api-emulator.git", | |
"clone_url": "https://github.com/pdxjohnny/scitt-api-emulator.git", | |
"svn_url": "https://github.com/pdxjohnny/scitt-api-emulator", | |
"homepage": "https://scitt-community.github.io/scitt-api-emulator", | |
"size": 217, | |
"stargazers_count": 0, | |
"watchers_count": 0, | |
"language": "Python", | |
"has_issues": false, | |
"has_projects": true, | |
"has_downloads": true, | |
"has_wiki": true, | |
"has_pages": false, | |
"has_discussions": false, | |
"forks_count": 0, | |
"mirror_url": null, | |
"archived": false, | |
"disabled": false, | |
"open_issues_count": 1, | |
"license": { | |
"key": "mit", | |
"name": "MIT License", | |
"spdx_id": "MIT", | |
"url": "https://api.github.com/licenses/mit", | |
"node_id": "MDc6TGljZW5zZTEz" | |
}, | |
"allow_forking": true, | |
"is_template": false, | |
"web_commit_signoff_required": true, | |
"topics": [], | |
"visibility": "public", | |
"forks": 0, | |
"open_issues": 1, | |
"watchers": 0, | |
"default_branch": "auth", | |
"stargazers": 0, | |
"master_branch": "auth" | |
}, | |
"pusher": { | |
"name": "pdxjohnny", | |
"email": "johnandersenpdx@gmail.com" | |
}, | |
"sender": { | |
"login": "pdxjohnny", | |
"id": 5950433, | |
"node_id": "MDQ6VXNlcjU5NTA0MzM=", | |
"avatar_url": "https://avatars.githubusercontent.com/u/5950433?v=4", | |
"gravatar_id": "", | |
"url": "https://api.github.com/users/pdxjohnny", | |
"html_url": "https://github.com/pdxjohnny", | |
"followers_url": "https://api.github.com/users/pdxjohnny/followers", | |
"following_url": "https://api.github.com/users/pdxjohnny/following{/other_user}", | |
"gists_url": "https://api.github.com/users/pdxjohnny/gists{/gist_id}", | |
"starred_url": "https://api.github.com/users/pdxjohnny/starred{/owner}{/repo}", | |
"subscriptions_url": "https://api.github.com/users/pdxjohnny/subscriptions", | |
"organizations_url": "https://api.github.com/users/pdxjohnny/orgs", | |
"repos_url": "https://api.github.com/users/pdxjohnny/repos", | |
"events_url": "https://api.github.com/users/pdxjohnny/events{/privacy}", | |
"received_events_url": "https://api.github.com/users/pdxjohnny/received_events", | |
"type": "User", | |
"site_admin": false | |
}, | |
"created": false, | |
"deleted": false, | |
"forced": true, | |
"base_ref": null, | |
"compare": "https://github.com/pdxjohnny/scitt-api-emulator/compare/f10df8c5020d...e3cc9ad852a6", | |
"commits": [ | |
{ | |
"id": "e3cc9ad852a6551d92d9eb52f13b2d3208774d41", | |
"tree_id": "70268469668b1cbff7e4684528217af7a54d66bb", | |
"distinct": true, | |
"message": "IN PROGRESS: did helpers: ecdsa p-384 key loading\n\nSigned-off-by: John Andersen <johnandersenpdx@gmail.com>", | |
"timestamp": "2023-11-16T00:37:36+01:00", | |
"url": "https://github.com/pdxjohnny/scitt-api-emulator/commit/e3cc9ad852a6551d92d9eb52f13b2d3208774d41", | |
"author": { | |
"name": "John Andersen", | |
"email": "johnandersenpdx@gmail.com", | |
"username": "pdxjohnny" | |
}, | |
"committer": { | |
"name": "John Andersen", | |
"email": "johnandersenpdx@gmail.com", | |
"username": "pdxjohnny" | |
}, | |
"added": [], | |
"removed": [], | |
"modified": [ | |
"pytest.ini", | |
"scitt_emulator/did_helpers.py" | |
] | |
} | |
], | |
"head_commit": { | |
"id": "e3cc9ad852a6551d92d9eb52f13b2d3208774d41", | |
"tree_id": "70268469668b1cbff7e4684528217af7a54d66bb", | |
"distinct": true, | |
"message": "IN PROGRESS: did helpers: ecdsa p-384 key loading\n\nSigned-off-by: John Andersen <johnandersenpdx@gmail.com>", | |
"timestamp": "2023-11-16T00:37:36+01:00", | |
"url": "https://github.com/pdxjohnny/scitt-api-emulator/commit/e3cc9ad852a6551d92d9eb52f13b2d3208774d41", | |
"author": { | |
"name": "John Andersen", | |
"email": "johnandersenpdx@gmail.com", | |
"username": "pdxjohnny" | |
}, | |
"committer": { | |
"name": "John Andersen", | |
"email": "johnandersenpdx@gmail.com", | |
"username": "pdxjohnny" | |
}, | |
"added": [], | |
"removed": [], | |
"modified": [ | |
"pytest.ini", | |
"scitt_emulator/did_helpers.py" | |
] | |
} | |
} |
import os | |
import sys | |
import time | |
import json | |
import pathlib | |
import argparse | |
import subprocess | |
import contextlib | |
import http.server | |
import httptest | |
class GitHubWebhookLogger(http.server.BaseHTTPRequestHandler): | |
def do_POST(self): | |
self.send_response(200) | |
self.send_header("Content-type", "text/plain") | |
self.send_header("Content-length", 3) | |
self.end_headers() | |
self.wfile.write(b"OK\n") | |
payload = json.loads(self.rfile.read()) | |
payload_path = pathlib.Path( | |
".".join( | |
[ | |
self.headers["X-github-event"], | |
*( | |
[ | |
":".join([key, value]) | |
for key, value in payload.items() | |
if isinstance(value, str) and not os.sep in value | |
] | |
+ ["json"] | |
), | |
], | |
) | |
) | |
payload_path.write_text(json.dumps(payload, indent=4, sort_keys=True)) | |
@classmethod | |
def cli(cls, argv=None): | |
parser = argparse.ArgumentParser(description="Log GitHub webhook events") | |
parser.add_argument( | |
"-R", | |
"--repo", | |
dest="org_and_repo", | |
default=None, | |
required=False, | |
type=str, | |
help="GitHub repo in format org/repo", | |
) | |
parser.add_argument( | |
"--addr", | |
type=str, | |
help="Address to bind to", | |
default="0.0.0.0", | |
) | |
parser.add_argument( | |
"--port", | |
type=int, | |
help="Port to bind to", | |
default=0, | |
) | |
parser.add_argument( | |
"--state-dir", | |
dest="state_dir", | |
type=pathlib.Path, | |
help="Directory to cache requests in", | |
default=pathlib.Path(os.getcwd(), ".cache", "httptest"), | |
) | |
args = parser.parse_args(argv) | |
if not args.state_dir.is_dir(): | |
args.state_dir.mkdir(parents=True) | |
with httptest.Server(cls, addr=(args.addr, args.port)) as logging_server: | |
with httptest.Server( | |
httptest.CachingProxyHandler.to( | |
logging_server.url(), state_dir=str(args.state_dir.resolve()) | |
) | |
) as cache_server: | |
if args.org_and_repo: | |
subprocess.check_call( | |
[ | |
"gh", | |
"webhook", | |
"forward", | |
f"--repo={args.org_and_repo}", | |
"--events=*", | |
f"--url={cache_server.url()}", | |
] | |
) | |
else: | |
print(logging_server.url()) | |
sys.stdout.buffer.flush() | |
sys.stdout.close() | |
sys.stdout = sys.stderr | |
with contextlib.suppress(KeyboardInterrupt): | |
while True: | |
time.sleep(600) | |
if __name__ == "__main__": | |
GitHubWebhookLogger.cli(sys.argv[1:]) |
{ | |
"action": "completed", | |
"repository": { | |
"allow_forking": true, | |
"archive_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/{archive_format}{/ref}", | |
"archived": false, | |
"assignees_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/assignees{/user}", | |
"blobs_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/git/blobs{/sha}", | |
"branches_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/branches{/branch}", | |
"clone_url": "https://github.com/pdxjohnny/scitt-api-emulator.git", | |
"collaborators_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/collaborators{/collaborator}", | |
"comments_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/comments{/number}", | |
"commits_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/commits{/sha}", | |
"compare_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/compare/{base}...{head}", | |
"contents_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/contents/{+path}", | |
"contributors_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/contributors", | |
"created_at": "2023-03-30T03:43:27Z", | |
"default_branch": "auth", | |
"deployments_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/deployments", | |
"description": "SCITT API Emulator", | |
"disabled": false, | |
"downloads_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/downloads", | |
"events_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/events", | |
"fork": true, | |
"forks": 0, | |
"forks_count": 0, | |
"forks_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/forks", | |
"full_name": "pdxjohnny/scitt-api-emulator", | |
"git_commits_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/git/commits{/sha}", | |
"git_refs_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/git/refs{/sha}", | |
"git_tags_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/git/tags{/sha}", | |
"git_url": "git://github.com/pdxjohnny/scitt-api-emulator.git", | |
"has_discussions": false, | |
"has_downloads": true, | |
"has_issues": false, | |
"has_pages": false, | |
"has_projects": true, | |
"has_wiki": true, | |
"homepage": "https://scitt-community.github.io/scitt-api-emulator", | |
"hooks_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/hooks", | |
"html_url": "https://github.com/pdxjohnny/scitt-api-emulator", | |
"id": 621131680, | |
"is_template": false, | |
"issue_comment_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/issues/comments{/number}", | |
"issue_events_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/issues/events{/number}", | |
"issues_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/issues{/number}", | |
"keys_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/keys{/key_id}", | |
"labels_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/labels{/name}", | |
"language": "Python", | |
"languages_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/languages", | |
"license": { | |
"key": "mit", | |
"name": "MIT License", | |
"node_id": "MDc6TGljZW5zZTEz", | |
"spdx_id": "MIT", | |
"url": "https://api.github.com/licenses/mit" | |
}, | |
"merges_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/merges", | |
"milestones_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/milestones{/number}", | |
"mirror_url": null, | |
"name": "scitt-api-emulator", | |
"node_id": "R_kgDOJQW3oA", | |
"notifications_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/notifications{?since,all,participating}", | |
"open_issues": 1, | |
"open_issues_count": 1, | |
"owner": { | |
"avatar_url": "https://avatars.githubusercontent.com/u/5950433?v=4", | |
"events_url": "https://api.github.com/users/pdxjohnny/events{/privacy}", | |
"followers_url": "https://api.github.com/users/pdxjohnny/followers", | |
"following_url": "https://api.github.com/users/pdxjohnny/following{/other_user}", | |
"gists_url": "https://api.github.com/users/pdxjohnny/gists{/gist_id}", | |
"gravatar_id": "", | |
"html_url": "https://github.com/pdxjohnny", | |
"id": 5950433, | |
"login": "pdxjohnny", | |
"node_id": "MDQ6VXNlcjU5NTA0MzM=", | |
"organizations_url": "https://api.github.com/users/pdxjohnny/orgs", | |
"received_events_url": "https://api.github.com/users/pdxjohnny/received_events", | |
"repos_url": "https://api.github.com/users/pdxjohnny/repos", | |
"site_admin": false, | |
"starred_url": "https://api.github.com/users/pdxjohnny/starred{/owner}{/repo}", | |
"subscriptions_url": "https://api.github.com/users/pdxjohnny/subscriptions", | |
"type": "User", | |
"url": "https://api.github.com/users/pdxjohnny" | |
}, | |
"private": false, | |
"pulls_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/pulls{/number}", | |
"pushed_at": "2023-11-15T23:37:40Z", | |
"releases_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/releases{/id}", | |
"size": 217, | |
"ssh_url": "git@github.com:pdxjohnny/scitt-api-emulator.git", | |
"stargazers_count": 0, | |
"stargazers_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/stargazers", | |
"statuses_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/statuses/{sha}", | |
"subscribers_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/subscribers", | |
"subscription_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/subscription", | |
"svn_url": "https://github.com/pdxjohnny/scitt-api-emulator", | |
"tags_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/tags", | |
"teams_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/teams", | |
"topics": [], | |
"trees_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/git/trees{/sha}", | |
"updated_at": "2023-09-12T21:02:36Z", | |
"url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator", | |
"visibility": "public", | |
"watchers": 0, | |
"watchers_count": 0, | |
"web_commit_signoff_required": true | |
}, | |
"sender": { | |
"avatar_url": "https://avatars.githubusercontent.com/u/5950433?v=4", | |
"events_url": "https://api.github.com/users/pdxjohnny/events{/privacy}", | |
"followers_url": "https://api.github.com/users/pdxjohnny/followers", | |
"following_url": "https://api.github.com/users/pdxjohnny/following{/other_user}", | |
"gists_url": "https://api.github.com/users/pdxjohnny/gists{/gist_id}", | |
"gravatar_id": "", | |
"html_url": "https://github.com/pdxjohnny", | |
"id": 5950433, | |
"login": "pdxjohnny", | |
"node_id": "MDQ6VXNlcjU5NTA0MzM=", | |
"organizations_url": "https://api.github.com/users/pdxjohnny/orgs", | |
"received_events_url": "https://api.github.com/users/pdxjohnny/received_events", | |
"repos_url": "https://api.github.com/users/pdxjohnny/repos", | |
"site_admin": false, | |
"starred_url": "https://api.github.com/users/pdxjohnny/starred{/owner}{/repo}", | |
"subscriptions_url": "https://api.github.com/users/pdxjohnny/subscriptions", | |
"type": "User", | |
"url": "https://api.github.com/users/pdxjohnny" | |
}, | |
"workflow_job": { | |
"check_run_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/check-runs/18726406136", | |
"completed_at": "2023-11-15T23:47:09Z", | |
"conclusion": "failure", | |
"created_at": "2023-11-15T23:44:18Z", | |
"head_branch": "cwt", | |
"head_sha": "e3cc9ad852a6551d92d9eb52f13b2d3208774d41", | |
"html_url": "https://github.com/pdxjohnny/scitt-api-emulator/actions/runs/6884262695/job/18726406136", | |
"id": 18726406136, | |
"labels": [ | |
"ubuntu-latest" | |
], | |
"name": "CI (conda)", | |
"node_id": "CR_kwDOJQW3oM8AAAAEXC5H-A", | |
"run_attempt": 1, | |
"run_id": 6884262695, | |
"run_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/actions/runs/6884262695", | |
"runner_group_id": 2, | |
"runner_group_name": "GitHub Actions", | |
"runner_id": 5, | |
"runner_name": "GitHub Actions 5", | |
"started_at": "2023-11-15T23:44:23Z", | |
"status": "completed", | |
"steps": [ | |
{ | |
"completed_at": "2023-11-15T23:44:23.000Z", | |
"conclusion": "success", | |
"name": "Set up job", | |
"number": 1, | |
"started_at": "2023-11-15T23:44:23.000Z", | |
"status": "completed" | |
}, | |
{ | |
"completed_at": "2023-11-15T23:44:24.000Z", | |
"conclusion": "success", | |
"name": "Run actions/checkout@v3", | |
"number": 2, | |
"started_at": "2023-11-15T23:44:24.000Z", | |
"status": "completed" | |
}, | |
{ | |
"completed_at": "2023-11-15T23:47:02.000Z", | |
"conclusion": "success", | |
"name": "Run conda-incubator/setup-miniconda@v2", | |
"number": 3, | |
"started_at": "2023-11-15T23:44:24.000Z", | |
"status": "completed" | |
}, | |
{ | |
"completed_at": "2023-11-15T23:47:04.000Z", | |
"conclusion": "success", | |
"name": "Temporary fix for", | |
"number": 4, | |
"started_at": "2023-11-15T23:47:02.000Z", | |
"status": "completed" | |
}, | |
{ | |
"completed_at": "2023-11-15T23:47:08.000Z", | |
"conclusion": "failure", | |
"name": "Run python -m pytest", | |
"number": 5, | |
"started_at": "2023-11-15T23:47:04.000Z", | |
"status": "completed" | |
}, | |
{ | |
"completed_at": "2023-11-15T23:47:09.000Z", | |
"conclusion": "skipped", | |
"name": "Post Run conda-incubator/setup-miniconda@v2", | |
"number": 9, | |
"started_at": "2023-11-15T23:47:09.000Z", | |
"status": "completed" | |
}, | |
{ | |
"completed_at": "2023-11-15T23:47:09.000Z", | |
"conclusion": "success", | |
"name": "Post Run actions/checkout@v3", | |
"number": 10, | |
"started_at": "2023-11-15T23:47:09.000Z", | |
"status": "completed" | |
}, | |
{ | |
"completed_at": "2023-11-15T23:47:08.000Z", | |
"conclusion": "success", | |
"name": "Complete job", | |
"number": 11, | |
"started_at": "2023-11-15T23:47:08.000Z", | |
"status": "completed" | |
} | |
], | |
"url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/actions/jobs/18726406136", | |
"workflow_name": "CI" | |
} | |
} |
{ | |
"action": "in_progress", | |
"repository": { | |
"allow_forking": true, | |
"archive_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/{archive_format}{/ref}", | |
"archived": false, | |
"assignees_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/assignees{/user}", | |
"blobs_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/git/blobs{/sha}", | |
"branches_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/branches{/branch}", | |
"clone_url": "https://github.com/pdxjohnny/scitt-api-emulator.git", | |
"collaborators_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/collaborators{/collaborator}", | |
"comments_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/comments{/number}", | |
"commits_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/commits{/sha}", | |
"compare_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/compare/{base}...{head}", | |
"contents_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/contents/{+path}", | |
"contributors_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/contributors", | |
"created_at": "2023-03-30T03:43:27Z", | |
"default_branch": "auth", | |
"deployments_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/deployments", | |
"description": "SCITT API Emulator", | |
"disabled": false, | |
"downloads_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/downloads", | |
"events_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/events", | |
"fork": true, | |
"forks": 0, | |
"forks_count": 0, | |
"forks_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/forks", | |
"full_name": "pdxjohnny/scitt-api-emulator", | |
"git_commits_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/git/commits{/sha}", | |
"git_refs_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/git/refs{/sha}", | |
"git_tags_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/git/tags{/sha}", | |
"git_url": "git://github.com/pdxjohnny/scitt-api-emulator.git", | |
"has_discussions": false, | |
"has_downloads": true, | |
"has_issues": false, | |
"has_pages": false, | |
"has_projects": true, | |
"has_wiki": true, | |
"homepage": "https://scitt-community.github.io/scitt-api-emulator", | |
"hooks_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/hooks", | |
"html_url": "https://github.com/pdxjohnny/scitt-api-emulator", | |
"id": 621131680, | |
"is_template": false, | |
"issue_comment_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/issues/comments{/number}", | |
"issue_events_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/issues/events{/number}", | |
"issues_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/issues{/number}", | |
"keys_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/keys{/key_id}", | |
"labels_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/labels{/name}", | |
"language": "Python", | |
"languages_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/languages", | |
"license": { | |
"key": "mit", | |
"name": "MIT License", | |
"node_id": "MDc6TGljZW5zZTEz", | |
"spdx_id": "MIT", | |
"url": "https://api.github.com/licenses/mit" | |
}, | |
"merges_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/merges", | |
"milestones_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/milestones{/number}", | |
"mirror_url": null, | |
"name": "scitt-api-emulator", | |
"node_id": "R_kgDOJQW3oA", | |
"notifications_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/notifications{?since,all,participating}", | |
"open_issues": 1, | |
"open_issues_count": 1, | |
"owner": { | |
"avatar_url": "https://avatars.githubusercontent.com/u/5950433?v=4", | |
"events_url": "https://api.github.com/users/pdxjohnny/events{/privacy}", | |
"followers_url": "https://api.github.com/users/pdxjohnny/followers", | |
"following_url": "https://api.github.com/users/pdxjohnny/following{/other_user}", | |
"gists_url": "https://api.github.com/users/pdxjohnny/gists{/gist_id}", | |
"gravatar_id": "", | |
"html_url": "https://github.com/pdxjohnny", | |
"id": 5950433, | |
"login": "pdxjohnny", | |
"node_id": "MDQ6VXNlcjU5NTA0MzM=", | |
"organizations_url": "https://api.github.com/users/pdxjohnny/orgs", | |
"received_events_url": "https://api.github.com/users/pdxjohnny/received_events", | |
"repos_url": "https://api.github.com/users/pdxjohnny/repos", | |
"site_admin": false, | |
"starred_url": "https://api.github.com/users/pdxjohnny/starred{/owner}{/repo}", | |
"subscriptions_url": "https://api.github.com/users/pdxjohnny/subscriptions", | |
"type": "User", | |
"url": "https://api.github.com/users/pdxjohnny" | |
}, | |
"private": false, | |
"pulls_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/pulls{/number}", | |
"pushed_at": "2023-11-15T23:37:40Z", | |
"releases_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/releases{/id}", | |
"size": 217, | |
"ssh_url": "git@github.com:pdxjohnny/scitt-api-emulator.git", | |
"stargazers_count": 0, | |
"stargazers_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/stargazers", | |
"statuses_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/statuses/{sha}", | |
"subscribers_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/subscribers", | |
"subscription_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/subscription", | |
"svn_url": "https://github.com/pdxjohnny/scitt-api-emulator", | |
"tags_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/tags", | |
"teams_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/teams", | |
"topics": [], | |
"trees_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/git/trees{/sha}", | |
"updated_at": "2023-09-12T21:02:36Z", | |
"url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator", | |
"visibility": "public", | |
"watchers": 0, | |
"watchers_count": 0, | |
"web_commit_signoff_required": true | |
}, | |
"sender": { | |
"avatar_url": "https://avatars.githubusercontent.com/u/5950433?v=4", | |
"events_url": "https://api.github.com/users/pdxjohnny/events{/privacy}", | |
"followers_url": "https://api.github.com/users/pdxjohnny/followers", | |
"following_url": "https://api.github.com/users/pdxjohnny/following{/other_user}", | |
"gists_url": "https://api.github.com/users/pdxjohnny/gists{/gist_id}", | |
"gravatar_id": "", | |
"html_url": "https://github.com/pdxjohnny", | |
"id": 5950433, | |
"login": "pdxjohnny", | |
"node_id": "MDQ6VXNlcjU5NTA0MzM=", | |
"organizations_url": "https://api.github.com/users/pdxjohnny/orgs", | |
"received_events_url": "https://api.github.com/users/pdxjohnny/received_events", | |
"repos_url": "https://api.github.com/users/pdxjohnny/repos", | |
"site_admin": false, | |
"starred_url": "https://api.github.com/users/pdxjohnny/starred{/owner}{/repo}", | |
"subscriptions_url": "https://api.github.com/users/pdxjohnny/subscriptions", | |
"type": "User", | |
"url": "https://api.github.com/users/pdxjohnny" | |
}, | |
"workflow_job": { | |
"check_run_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/check-runs/18726406006", | |
"completed_at": null, | |
"conclusion": null, | |
"created_at": "2023-11-15T23:44:18Z", | |
"head_branch": "cwt", | |
"head_sha": "e3cc9ad852a6551d92d9eb52f13b2d3208774d41", | |
"html_url": "https://github.com/pdxjohnny/scitt-api-emulator/actions/runs/6884262695/job/18726406006", | |
"id": 18726406006, | |
"labels": [ | |
"ubuntu-latest" | |
], | |
"name": "CI/CD (container)", | |
"node_id": "CR_kwDOJQW3oM8AAAAEXC5Hdg", | |
"run_attempt": 1, | |
"run_id": 6884262695, | |
"run_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/actions/runs/6884262695", | |
"runner_group_id": 2, | |
"runner_group_name": "GitHub Actions", | |
"runner_id": 2, | |
"runner_name": "GitHub Actions 2", | |
"started_at": "2023-11-15T23:44:25Z", | |
"status": "in_progress", | |
"steps": [ | |
{ | |
"completed_at": null, | |
"conclusion": null, | |
"name": "Set up job", | |
"number": 1, | |
"started_at": "2023-11-15T23:44:24.000Z", | |
"status": "in_progress" | |
} | |
], | |
"url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/actions/jobs/18726406006", | |
"workflow_name": "CI" | |
} | |
} |
{ | |
"action": "queued", | |
"repository": { | |
"allow_forking": true, | |
"archive_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/{archive_format}{/ref}", | |
"archived": false, | |
"assignees_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/assignees{/user}", | |
"blobs_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/git/blobs{/sha}", | |
"branches_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/branches{/branch}", | |
"clone_url": "https://github.com/pdxjohnny/scitt-api-emulator.git", | |
"collaborators_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/collaborators{/collaborator}", | |
"comments_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/comments{/number}", | |
"commits_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/commits{/sha}", | |
"compare_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/compare/{base}...{head}", | |
"contents_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/contents/{+path}", | |
"contributors_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/contributors", | |
"created_at": "2023-03-30T03:43:27Z", | |
"default_branch": "auth", | |
"deployments_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/deployments", | |
"description": "SCITT API Emulator", | |
"disabled": false, | |
"downloads_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/downloads", | |
"events_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/events", | |
"fork": true, | |
"forks": 0, | |
"forks_count": 0, | |
"forks_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/forks", | |
"full_name": "pdxjohnny/scitt-api-emulator", | |
"git_commits_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/git/commits{/sha}", | |
"git_refs_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/git/refs{/sha}", | |
"git_tags_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/git/tags{/sha}", | |
"git_url": "git://github.com/pdxjohnny/scitt-api-emulator.git", | |
"has_discussions": false, | |
"has_downloads": true, | |
"has_issues": false, | |
"has_pages": false, | |
"has_projects": true, | |
"has_wiki": true, | |
"homepage": "https://scitt-community.github.io/scitt-api-emulator", | |
"hooks_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/hooks", | |
"html_url": "https://github.com/pdxjohnny/scitt-api-emulator", | |
"id": 621131680, | |
"is_template": false, | |
"issue_comment_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/issues/comments{/number}", | |
"issue_events_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/issues/events{/number}", | |
"issues_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/issues{/number}", | |
"keys_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/keys{/key_id}", | |
"labels_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/labels{/name}", | |
"language": "Python", | |
"languages_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/languages", | |
"license": { | |
"key": "mit", | |
"name": "MIT License", | |
"node_id": "MDc6TGljZW5zZTEz", | |
"spdx_id": "MIT", | |
"url": "https://api.github.com/licenses/mit" | |
}, | |
"merges_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/merges", | |
"milestones_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/milestones{/number}", | |
"mirror_url": null, | |
"name": "scitt-api-emulator", | |
"node_id": "R_kgDOJQW3oA", | |
"notifications_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/notifications{?since,all,participating}", | |
"open_issues": 1, | |
"open_issues_count": 1, | |
"owner": { | |
"avatar_url": "https://avatars.githubusercontent.com/u/5950433?v=4", | |
"events_url": "https://api.github.com/users/pdxjohnny/events{/privacy}", | |
"followers_url": "https://api.github.com/users/pdxjohnny/followers", | |
"following_url": "https://api.github.com/users/pdxjohnny/following{/other_user}", | |
"gists_url": "https://api.github.com/users/pdxjohnny/gists{/gist_id}", | |
"gravatar_id": "", | |
"html_url": "https://github.com/pdxjohnny", | |
"id": 5950433, | |
"login": "pdxjohnny", | |
"node_id": "MDQ6VXNlcjU5NTA0MzM=", | |
"organizations_url": "https://api.github.com/users/pdxjohnny/orgs", | |
"received_events_url": "https://api.github.com/users/pdxjohnny/received_events", | |
"repos_url": "https://api.github.com/users/pdxjohnny/repos", | |
"site_admin": false, | |
"starred_url": "https://api.github.com/users/pdxjohnny/starred{/owner}{/repo}", | |
"subscriptions_url": "https://api.github.com/users/pdxjohnny/subscriptions", | |
"type": "User", | |
"url": "https://api.github.com/users/pdxjohnny" | |
}, | |
"private": false, | |
"pulls_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/pulls{/number}", | |
"pushed_at": "2023-11-15T23:37:40Z", | |
"releases_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/releases{/id}", | |
"size": 217, | |
"ssh_url": "git@github.com:pdxjohnny/scitt-api-emulator.git", | |
"stargazers_count": 0, | |
"stargazers_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/stargazers", | |
"statuses_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/statuses/{sha}", | |
"subscribers_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/subscribers", | |
"subscription_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/subscription", | |
"svn_url": "https://github.com/pdxjohnny/scitt-api-emulator", | |
"tags_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/tags", | |
"teams_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/teams", | |
"topics": [], | |
"trees_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/git/trees{/sha}", | |
"updated_at": "2023-09-12T21:02:36Z", | |
"url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator", | |
"visibility": "public", | |
"watchers": 0, | |
"watchers_count": 0, | |
"web_commit_signoff_required": true | |
}, | |
"sender": { | |
"avatar_url": "https://avatars.githubusercontent.com/u/5950433?v=4", | |
"events_url": "https://api.github.com/users/pdxjohnny/events{/privacy}", | |
"followers_url": "https://api.github.com/users/pdxjohnny/followers", | |
"following_url": "https://api.github.com/users/pdxjohnny/following{/other_user}", | |
"gists_url": "https://api.github.com/users/pdxjohnny/gists{/gist_id}", | |
"gravatar_id": "", | |
"html_url": "https://github.com/pdxjohnny", | |
"id": 5950433, | |
"login": "pdxjohnny", | |
"node_id": "MDQ6VXNlcjU5NTA0MzM=", | |
"organizations_url": "https://api.github.com/users/pdxjohnny/orgs", | |
"received_events_url": "https://api.github.com/users/pdxjohnny/received_events", | |
"repos_url": "https://api.github.com/users/pdxjohnny/repos", | |
"site_admin": false, | |
"starred_url": "https://api.github.com/users/pdxjohnny/starred{/owner}{/repo}", | |
"subscriptions_url": "https://api.github.com/users/pdxjohnny/subscriptions", | |
"type": "User", | |
"url": "https://api.github.com/users/pdxjohnny" | |
}, | |
"workflow_job": { | |
"check_run_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/check-runs/18726406244", | |
"completed_at": null, | |
"conclusion": null, | |
"created_at": "2023-11-15T23:44:19Z", | |
"head_branch": "cwt", | |
"head_sha": "e3cc9ad852a6551d92d9eb52f13b2d3208774d41", | |
"html_url": "https://github.com/pdxjohnny/scitt-api-emulator/actions/runs/6884262695/job/18726406244", | |
"id": 18726406244, | |
"labels": [ | |
"ubuntu-latest" | |
], | |
"name": "CI (venv) (3.8)", | |
"node_id": "CR_kwDOJQW3oM8AAAAEXC5IZA", | |
"run_attempt": 1, | |
"run_id": 6884262695, | |
"run_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/actions/runs/6884262695", | |
"runner_group_id": null, | |
"runner_group_name": null, | |
"runner_id": null, | |
"runner_name": null, | |
"started_at": "2023-11-15T23:44:19Z", | |
"status": "queued", | |
"steps": [], | |
"url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/actions/jobs/18726406244", | |
"workflow_name": "CI" | |
} | |
} |
{ | |
"action": "completed", | |
"repository": { | |
"allow_forking": true, | |
"archive_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/{archive_format}{/ref}", | |
"archived": false, | |
"assignees_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/assignees{/user}", | |
"blobs_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/git/blobs{/sha}", | |
"branches_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/branches{/branch}", | |
"clone_url": "https://github.com/pdxjohnny/scitt-api-emulator.git", | |
"collaborators_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/collaborators{/collaborator}", | |
"comments_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/comments{/number}", | |
"commits_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/commits{/sha}", | |
"compare_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/compare/{base}...{head}", | |
"contents_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/contents/{+path}", | |
"contributors_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/contributors", | |
"created_at": "2023-03-30T03:43:27Z", | |
"default_branch": "auth", | |
"deployments_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/deployments", | |
"description": "SCITT API Emulator", | |
"disabled": false, | |
"downloads_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/downloads", | |
"events_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/events", | |
"fork": true, | |
"forks": 0, | |
"forks_count": 0, | |
"forks_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/forks", | |
"full_name": "pdxjohnny/scitt-api-emulator", | |
"git_commits_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/git/commits{/sha}", | |
"git_refs_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/git/refs{/sha}", | |
"git_tags_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/git/tags{/sha}", | |
"git_url": "git://github.com/pdxjohnny/scitt-api-emulator.git", | |
"has_discussions": false, | |
"has_downloads": true, | |
"has_issues": false, | |
"has_pages": false, | |
"has_projects": true, | |
"has_wiki": true, | |
"homepage": "https://scitt-community.github.io/scitt-api-emulator", | |
"hooks_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/hooks", | |
"html_url": "https://github.com/pdxjohnny/scitt-api-emulator", | |
"id": 621131680, | |
"is_template": false, | |
"issue_comment_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/issues/comments{/number}", | |
"issue_events_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/issues/events{/number}", | |
"issues_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/issues{/number}", | |
"keys_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/keys{/key_id}", | |
"labels_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/labels{/name}", | |
"language": "Python", | |
"languages_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/languages", | |
"license": { | |
"key": "mit", | |
"name": "MIT License", | |
"node_id": "MDc6TGljZW5zZTEz", | |
"spdx_id": "MIT", | |
"url": "https://api.github.com/licenses/mit" | |
}, | |
"merges_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/merges", | |
"milestones_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/milestones{/number}", | |
"mirror_url": null, | |
"name": "scitt-api-emulator", | |
"node_id": "R_kgDOJQW3oA", | |
"notifications_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/notifications{?since,all,participating}", | |
"open_issues": 1, | |
"open_issues_count": 1, | |
"owner": { | |
"avatar_url": "https://avatars.githubusercontent.com/u/5950433?v=4", | |
"events_url": "https://api.github.com/users/pdxjohnny/events{/privacy}", | |
"followers_url": "https://api.github.com/users/pdxjohnny/followers", | |
"following_url": "https://api.github.com/users/pdxjohnny/following{/other_user}", | |
"gists_url": "https://api.github.com/users/pdxjohnny/gists{/gist_id}", | |
"gravatar_id": "", | |
"html_url": "https://github.com/pdxjohnny", | |
"id": 5950433, | |
"login": "pdxjohnny", | |
"node_id": "MDQ6VXNlcjU5NTA0MzM=", | |
"organizations_url": "https://api.github.com/users/pdxjohnny/orgs", | |
"received_events_url": "https://api.github.com/users/pdxjohnny/received_events", | |
"repos_url": "https://api.github.com/users/pdxjohnny/repos", | |
"site_admin": false, | |
"starred_url": "https://api.github.com/users/pdxjohnny/starred{/owner}{/repo}", | |
"subscriptions_url": "https://api.github.com/users/pdxjohnny/subscriptions", | |
"type": "User", | |
"url": "https://api.github.com/users/pdxjohnny" | |
}, | |
"private": false, | |
"pulls_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/pulls{/number}", | |
"pushed_at": "2023-11-15T23:37:40Z", | |
"releases_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/releases{/id}", | |
"size": 217, | |
"ssh_url": "git@github.com:pdxjohnny/scitt-api-emulator.git", | |
"stargazers_count": 0, | |
"stargazers_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/stargazers", | |
"statuses_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/statuses/{sha}", | |
"subscribers_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/subscribers", | |
"subscription_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/subscription", | |
"svn_url": "https://github.com/pdxjohnny/scitt-api-emulator", | |
"tags_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/tags", | |
"teams_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/teams", | |
"topics": [], | |
"trees_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/git/trees{/sha}", | |
"updated_at": "2023-09-12T21:02:36Z", | |
"url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator", | |
"visibility": "public", | |
"watchers": 0, | |
"watchers_count": 0, | |
"web_commit_signoff_required": true | |
}, | |
"sender": { | |
"avatar_url": "https://avatars.githubusercontent.com/u/5950433?v=4", | |
"events_url": "https://api.github.com/users/pdxjohnny/events{/privacy}", | |
"followers_url": "https://api.github.com/users/pdxjohnny/followers", | |
"following_url": "https://api.github.com/users/pdxjohnny/following{/other_user}", | |
"gists_url": "https://api.github.com/users/pdxjohnny/gists{/gist_id}", | |
"gravatar_id": "", | |
"html_url": "https://github.com/pdxjohnny", | |
"id": 5950433, | |
"login": "pdxjohnny", | |
"node_id": "MDQ6VXNlcjU5NTA0MzM=", | |
"organizations_url": "https://api.github.com/users/pdxjohnny/orgs", | |
"received_events_url": "https://api.github.com/users/pdxjohnny/received_events", | |
"repos_url": "https://api.github.com/users/pdxjohnny/repos", | |
"site_admin": false, | |
"starred_url": "https://api.github.com/users/pdxjohnny/starred{/owner}{/repo}", | |
"subscriptions_url": "https://api.github.com/users/pdxjohnny/subscriptions", | |
"type": "User", | |
"url": "https://api.github.com/users/pdxjohnny" | |
}, | |
"workflow": { | |
"badge_url": "https://github.com/pdxjohnny/scitt-api-emulator/workflows/CI/badge.svg", | |
"created_at": "2023-03-30T04:49:33.000Z", | |
"html_url": "https://github.com/pdxjohnny/scitt-api-emulator/blob/auth/.github/workflows/ci.yml", | |
"id": 52784377, | |
"name": "CI", | |
"node_id": "W_kwDOJQW3oM4DJWz5", | |
"path": ".github/workflows/ci.yml", | |
"state": "active", | |
"updated_at": "2023-03-30T04:49:33.000Z", | |
"url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/actions/workflows/52784377" | |
}, | |
"workflow_run": { | |
"actor": { | |
"avatar_url": "https://avatars.githubusercontent.com/u/5950433?v=4", | |
"events_url": "https://api.github.com/users/pdxjohnny/events{/privacy}", | |
"followers_url": "https://api.github.com/users/pdxjohnny/followers", | |
"following_url": "https://api.github.com/users/pdxjohnny/following{/other_user}", | |
"gists_url": "https://api.github.com/users/pdxjohnny/gists{/gist_id}", | |
"gravatar_id": "", | |
"html_url": "https://github.com/pdxjohnny", | |
"id": 5950433, | |
"login": "pdxjohnny", | |
"node_id": "MDQ6VXNlcjU5NTA0MzM=", | |
"organizations_url": "https://api.github.com/users/pdxjohnny/orgs", | |
"received_events_url": "https://api.github.com/users/pdxjohnny/received_events", | |
"repos_url": "https://api.github.com/users/pdxjohnny/repos", | |
"site_admin": false, | |
"starred_url": "https://api.github.com/users/pdxjohnny/starred{/owner}{/repo}", | |
"subscriptions_url": "https://api.github.com/users/pdxjohnny/subscriptions", | |
"type": "User", | |
"url": "https://api.github.com/users/pdxjohnny" | |
}, | |
"artifacts_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/actions/runs/6884262695/artifacts", | |
"cancel_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/actions/runs/6884262695/cancel", | |
"check_suite_id": 18230253313, | |
"check_suite_node_id": "CS_kwDOJQW3oM8AAAAEPpuXAQ", | |
"check_suite_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/check-suites/18230253313", | |
"conclusion": "failure", | |
"created_at": "2023-11-15T23:44:17Z", | |
"display_title": "CI", | |
"event": "workflow_dispatch", | |
"head_branch": "cwt", | |
"head_commit": { | |
"author": { | |
"email": "johnandersenpdx@gmail.com", | |
"name": "John Andersen" | |
}, | |
"committer": { | |
"email": "johnandersenpdx@gmail.com", | |
"name": "John Andersen" | |
}, | |
"id": "e3cc9ad852a6551d92d9eb52f13b2d3208774d41", | |
"message": "IN PROGRESS: did helpers: ecdsa p-384 key loading\n\nSigned-off-by: John Andersen <johnandersenpdx@gmail.com>", | |
"timestamp": "2023-11-15T23:37:36Z", | |
"tree_id": "70268469668b1cbff7e4684528217af7a54d66bb" | |
}, | |
"head_repository": { | |
"archive_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/{archive_format}{/ref}", | |
"assignees_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/assignees{/user}", | |
"blobs_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/git/blobs{/sha}", | |
"branches_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/branches{/branch}", | |
"collaborators_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/collaborators{/collaborator}", | |
"comments_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/comments{/number}", | |
"commits_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/commits{/sha}", | |
"compare_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/compare/{base}...{head}", | |
"contents_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/contents/{+path}", | |
"contributors_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/contributors", | |
"deployments_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/deployments", | |
"description": "SCITT API Emulator", | |
"downloads_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/downloads", | |
"events_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/events", | |
"fork": true, | |
"forks_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/forks", | |
"full_name": "pdxjohnny/scitt-api-emulator", | |
"git_commits_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/git/commits{/sha}", | |
"git_refs_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/git/refs{/sha}", | |
"git_tags_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/git/tags{/sha}", | |
"hooks_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/hooks", | |
"html_url": "https://github.com/pdxjohnny/scitt-api-emulator", | |
"id": 621131680, | |
"issue_comment_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/issues/comments{/number}", | |
"issue_events_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/issues/events{/number}", | |
"issues_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/issues{/number}", | |
"keys_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/keys{/key_id}", | |
"labels_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/labels{/name}", | |
"languages_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/languages", | |
"merges_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/merges", | |
"milestones_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/milestones{/number}", | |
"name": "scitt-api-emulator", | |
"node_id": "R_kgDOJQW3oA", | |
"notifications_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/notifications{?since,all,participating}", | |
"owner": { | |
"avatar_url": "https://avatars.githubusercontent.com/u/5950433?v=4", | |
"events_url": "https://api.github.com/users/pdxjohnny/events{/privacy}", | |
"followers_url": "https://api.github.com/users/pdxjohnny/followers", | |
"following_url": "https://api.github.com/users/pdxjohnny/following{/other_user}", | |
"gists_url": "https://api.github.com/users/pdxjohnny/gists{/gist_id}", | |
"gravatar_id": "", | |
"html_url": "https://github.com/pdxjohnny", | |
"id": 5950433, | |
"login": "pdxjohnny", | |
"node_id": "MDQ6VXNlcjU5NTA0MzM=", | |
"organizations_url": "https://api.github.com/users/pdxjohnny/orgs", | |
"received_events_url": "https://api.github.com/users/pdxjohnny/received_events", | |
"repos_url": "https://api.github.com/users/pdxjohnny/repos", | |
"site_admin": false, | |
"starred_url": "https://api.github.com/users/pdxjohnny/starred{/owner}{/repo}", | |
"subscriptions_url": "https://api.github.com/users/pdxjohnny/subscriptions", | |
"type": "User", | |
"url": "https://api.github.com/users/pdxjohnny" | |
}, | |
"private": false, | |
"pulls_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/pulls{/number}", | |
"releases_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/releases{/id}", | |
"stargazers_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/stargazers", | |
"statuses_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/statuses/{sha}", | |
"subscribers_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/subscribers", | |
"subscription_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/subscription", | |
"tags_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/tags", | |
"teams_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/teams", | |
"trees_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/git/trees{/sha}", | |
"url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator" | |
}, | |
"head_sha": "e3cc9ad852a6551d92d9eb52f13b2d3208774d41", | |
"html_url": "https://github.com/pdxjohnny/scitt-api-emulator/actions/runs/6884262695", | |
"id": 6884262695, | |
"jobs_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/actions/runs/6884262695/jobs", | |
"logs_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/actions/runs/6884262695/logs", | |
"name": "CI", | |
"node_id": "WFR_kwLOJQW3oM8AAAABmlWDJw", | |
"path": ".github/workflows/ci.yml", | |
"previous_attempt_url": null, | |
"pull_requests": [ | |
{ | |
"base": { | |
"ref": "main", | |
"repo": { | |
"id": 560509356, | |
"name": "scitt-api-emulator", | |
"url": "https://api.github.com/repos/scitt-community/scitt-api-emulator" | |
}, | |
"sha": "a30c8182358f2eab1feb380a7bf920b5483060f9" | |
}, | |
"head": { | |
"ref": "cwt", | |
"repo": { | |
"id": 621131680, | |
"name": "scitt-api-emulator", | |
"url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator" | |
}, | |
"sha": "e3cc9ad852a6551d92d9eb52f13b2d3208774d41" | |
}, | |
"id": 1596414276, | |
"number": 39, | |
"url": "https://api.github.com/repos/scitt-community/scitt-api-emulator/pulls/39" | |
} | |
], | |
"referenced_workflows": [], | |
"repository": { | |
"archive_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/{archive_format}{/ref}", | |
"assignees_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/assignees{/user}", | |
"blobs_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/git/blobs{/sha}", | |
"branches_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/branches{/branch}", | |
"collaborators_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/collaborators{/collaborator}", | |
"comments_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/comments{/number}", | |
"commits_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/commits{/sha}", | |
"compare_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/compare/{base}...{head}", | |
"contents_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/contents/{+path}", | |
"contributors_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/contributors", | |
"deployments_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/deployments", | |
"description": "SCITT API Emulator", | |
"downloads_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/downloads", | |
"events_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/events", | |
"fork": true, | |
"forks_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/forks", | |
"full_name": "pdxjohnny/scitt-api-emulator", | |
"git_commits_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/git/commits{/sha}", | |
"git_refs_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/git/refs{/sha}", | |
"git_tags_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/git/tags{/sha}", | |
"hooks_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/hooks", | |
"html_url": "https://github.com/pdxjohnny/scitt-api-emulator", | |
"id": 621131680, | |
"issue_comment_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/issues/comments{/number}", | |
"issue_events_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/issues/events{/number}", | |
"issues_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/issues{/number}", | |
"keys_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/keys{/key_id}", | |
"labels_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/labels{/name}", | |
"languages_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/languages", | |
"merges_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/merges", | |
"milestones_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/milestones{/number}", | |
"name": "scitt-api-emulator", | |
"node_id": "R_kgDOJQW3oA", | |
"notifications_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/notifications{?since,all,participating}", | |
"owner": { | |
"avatar_url": "https://avatars.githubusercontent.com/u/5950433?v=4", | |
"events_url": "https://api.github.com/users/pdxjohnny/events{/privacy}", | |
"followers_url": "https://api.github.com/users/pdxjohnny/followers", | |
"following_url": "https://api.github.com/users/pdxjohnny/following{/other_user}", | |
"gists_url": "https://api.github.com/users/pdxjohnny/gists{/gist_id}", | |
"gravatar_id": "", | |
"html_url": "https://github.com/pdxjohnny", | |
"id": 5950433, | |
"login": "pdxjohnny", | |
"node_id": "MDQ6VXNlcjU5NTA0MzM=", | |
"organizations_url": "https://api.github.com/users/pdxjohnny/orgs", | |
"received_events_url": "https://api.github.com/users/pdxjohnny/received_events", | |
"repos_url": "https://api.github.com/users/pdxjohnny/repos", | |
"site_admin": false, | |
"starred_url": "https://api.github.com/users/pdxjohnny/starred{/owner}{/repo}", | |
"subscriptions_url": "https://api.github.com/users/pdxjohnny/subscriptions", | |
"type": "User", | |
"url": "https://api.github.com/users/pdxjohnny" | |
}, | |
"private": false, | |
"pulls_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/pulls{/number}", | |
"releases_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/releases{/id}", | |
"stargazers_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/stargazers", | |
"statuses_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/statuses/{sha}", | |
"subscribers_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/subscribers", | |
"subscription_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/subscription", | |
"tags_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/tags", | |
"teams_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/teams", | |
"trees_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/git/trees{/sha}", | |
"url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator" | |
}, | |
"rerun_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/actions/runs/6884262695/rerun", | |
"run_attempt": 1, | |
"run_number": 34, | |
"run_started_at": "2023-11-15T23:44:17Z", | |
"status": "completed", | |
"triggering_actor": { | |
"avatar_url": "https://avatars.githubusercontent.com/u/5950433?v=4", | |
"events_url": "https://api.github.com/users/pdxjohnny/events{/privacy}", | |
"followers_url": "https://api.github.com/users/pdxjohnny/followers", | |
"following_url": "https://api.github.com/users/pdxjohnny/following{/other_user}", | |
"gists_url": "https://api.github.com/users/pdxjohnny/gists{/gist_id}", | |
"gravatar_id": "", | |
"html_url": "https://github.com/pdxjohnny", | |
"id": 5950433, | |
"login": "pdxjohnny", | |
"node_id": "MDQ6VXNlcjU5NTA0MzM=", | |
"organizations_url": "https://api.github.com/users/pdxjohnny/orgs", | |
"received_events_url": "https://api.github.com/users/pdxjohnny/received_events", | |
"repos_url": "https://api.github.com/users/pdxjohnny/repos", | |
"site_admin": false, | |
"starred_url": "https://api.github.com/users/pdxjohnny/starred{/owner}{/repo}", | |
"subscriptions_url": "https://api.github.com/users/pdxjohnny/subscriptions", | |
"type": "User", | |
"url": "https://api.github.com/users/pdxjohnny" | |
}, | |
"updated_at": "2023-11-15T23:47:10Z", | |
"url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/actions/runs/6884262695", | |
"workflow_id": 52784377, | |
"workflow_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/actions/workflows/52784377" | |
} | |
} |
{ | |
"action": "in_progress", | |
"repository": { | |
"allow_forking": true, | |
"archive_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/{archive_format}{/ref}", | |
"archived": false, | |
"assignees_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/assignees{/user}", | |
"blobs_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/git/blobs{/sha}", | |
"branches_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/branches{/branch}", | |
"clone_url": "https://github.com/pdxjohnny/scitt-api-emulator.git", | |
"collaborators_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/collaborators{/collaborator}", | |
"comments_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/comments{/number}", | |
"commits_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/commits{/sha}", | |
"compare_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/compare/{base}...{head}", | |
"contents_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/contents/{+path}", | |
"contributors_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/contributors", | |
"created_at": "2023-03-30T03:43:27Z", | |
"default_branch": "auth", | |
"deployments_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/deployments", | |
"description": "SCITT API Emulator", | |
"disabled": false, | |
"downloads_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/downloads", | |
"events_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/events", | |
"fork": true, | |
"forks": 0, | |
"forks_count": 0, | |
"forks_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/forks", | |
"full_name": "pdxjohnny/scitt-api-emulator", | |
"git_commits_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/git/commits{/sha}", | |
"git_refs_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/git/refs{/sha}", | |
"git_tags_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/git/tags{/sha}", | |
"git_url": "git://github.com/pdxjohnny/scitt-api-emulator.git", | |
"has_discussions": false, | |
"has_downloads": true, | |
"has_issues": false, | |
"has_pages": false, | |
"has_projects": true, | |
"has_wiki": true, | |
"homepage": "https://scitt-community.github.io/scitt-api-emulator", | |
"hooks_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/hooks", | |
"html_url": "https://github.com/pdxjohnny/scitt-api-emulator", | |
"id": 621131680, | |
"is_template": false, | |
"issue_comment_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/issues/comments{/number}", | |
"issue_events_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/issues/events{/number}", | |
"issues_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/issues{/number}", | |
"keys_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/keys{/key_id}", | |
"labels_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/labels{/name}", | |
"language": "Python", | |
"languages_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/languages", | |
"license": { | |
"key": "mit", | |
"name": "MIT License", | |
"node_id": "MDc6TGljZW5zZTEz", | |
"spdx_id": "MIT", | |
"url": "https://api.github.com/licenses/mit" | |
}, | |
"merges_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/merges", | |
"milestones_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/milestones{/number}", | |
"mirror_url": null, | |
"name": "scitt-api-emulator", | |
"node_id": "R_kgDOJQW3oA", | |
"notifications_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/notifications{?since,all,participating}", | |
"open_issues": 1, | |
"open_issues_count": 1, | |
"owner": { | |
"avatar_url": "https://avatars.githubusercontent.com/u/5950433?v=4", | |
"events_url": "https://api.github.com/users/pdxjohnny/events{/privacy}", | |
"followers_url": "https://api.github.com/users/pdxjohnny/followers", | |
"following_url": "https://api.github.com/users/pdxjohnny/following{/other_user}", | |
"gists_url": "https://api.github.com/users/pdxjohnny/gists{/gist_id}", | |
"gravatar_id": "", | |
"html_url": "https://github.com/pdxjohnny", | |
"id": 5950433, | |
"login": "pdxjohnny", | |
"node_id": "MDQ6VXNlcjU5NTA0MzM=", | |
"organizations_url": "https://api.github.com/users/pdxjohnny/orgs", | |
"received_events_url": "https://api.github.com/users/pdxjohnny/received_events", | |
"repos_url": "https://api.github.com/users/pdxjohnny/repos", | |
"site_admin": false, | |
"starred_url": "https://api.github.com/users/pdxjohnny/starred{/owner}{/repo}", | |
"subscriptions_url": "https://api.github.com/users/pdxjohnny/subscriptions", | |
"type": "User", | |
"url": "https://api.github.com/users/pdxjohnny" | |
}, | |
"private": false, | |
"pulls_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/pulls{/number}", | |
"pushed_at": "2023-11-15T23:37:40Z", | |
"releases_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/releases{/id}", | |
"size": 217, | |
"ssh_url": "git@github.com:pdxjohnny/scitt-api-emulator.git", | |
"stargazers_count": 0, | |
"stargazers_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/stargazers", | |
"statuses_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/statuses/{sha}", | |
"subscribers_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/subscribers", | |
"subscription_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/subscription", | |
"svn_url": "https://github.com/pdxjohnny/scitt-api-emulator", | |
"tags_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/tags", | |
"teams_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/teams", | |
"topics": [], | |
"trees_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/git/trees{/sha}", | |
"updated_at": "2023-09-12T21:02:36Z", | |
"url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator", | |
"visibility": "public", | |
"watchers": 0, | |
"watchers_count": 0, | |
"web_commit_signoff_required": true | |
}, | |
"sender": { | |
"avatar_url": "https://avatars.githubusercontent.com/u/5950433?v=4", | |
"events_url": "https://api.github.com/users/pdxjohnny/events{/privacy}", | |
"followers_url": "https://api.github.com/users/pdxjohnny/followers", | |
"following_url": "https://api.github.com/users/pdxjohnny/following{/other_user}", | |
"gists_url": "https://api.github.com/users/pdxjohnny/gists{/gist_id}", | |
"gravatar_id": "", | |
"html_url": "https://github.com/pdxjohnny", | |
"id": 5950433, | |
"login": "pdxjohnny", | |
"node_id": "MDQ6VXNlcjU5NTA0MzM=", | |
"organizations_url": "https://api.github.com/users/pdxjohnny/orgs", | |
"received_events_url": "https://api.github.com/users/pdxjohnny/received_events", | |
"repos_url": "https://api.github.com/users/pdxjohnny/repos", | |
"site_admin": false, | |
"starred_url": "https://api.github.com/users/pdxjohnny/starred{/owner}{/repo}", | |
"subscriptions_url": "https://api.github.com/users/pdxjohnny/subscriptions", | |
"type": "User", | |
"url": "https://api.github.com/users/pdxjohnny" | |
}, | |
"workflow": { | |
"badge_url": "https://github.com/pdxjohnny/scitt-api-emulator/workflows/CI/badge.svg", | |
"created_at": "2023-03-30T04:49:33.000Z", | |
"html_url": "https://github.com/pdxjohnny/scitt-api-emulator/blob/auth/.github/workflows/ci.yml", | |
"id": 52784377, | |
"name": "CI", | |
"node_id": "W_kwDOJQW3oM4DJWz5", | |
"path": ".github/workflows/ci.yml", | |
"state": "active", | |
"updated_at": "2023-03-30T04:49:33.000Z", | |
"url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/actions/workflows/52784377" | |
}, | |
"workflow_run": { | |
"actor": { | |
"avatar_url": "https://avatars.githubusercontent.com/u/5950433?v=4", | |
"events_url": "https://api.github.com/users/pdxjohnny/events{/privacy}", | |
"followers_url": "https://api.github.com/users/pdxjohnny/followers", | |
"following_url": "https://api.github.com/users/pdxjohnny/following{/other_user}", | |
"gists_url": "https://api.github.com/users/pdxjohnny/gists{/gist_id}", | |
"gravatar_id": "", | |
"html_url": "https://github.com/pdxjohnny", | |
"id": 5950433, | |
"login": "pdxjohnny", | |
"node_id": "MDQ6VXNlcjU5NTA0MzM=", | |
"organizations_url": "https://api.github.com/users/pdxjohnny/orgs", | |
"received_events_url": "https://api.github.com/users/pdxjohnny/received_events", | |
"repos_url": "https://api.github.com/users/pdxjohnny/repos", | |
"site_admin": false, | |
"starred_url": "https://api.github.com/users/pdxjohnny/starred{/owner}{/repo}", | |
"subscriptions_url": "https://api.github.com/users/pdxjohnny/subscriptions", | |
"type": "User", | |
"url": "https://api.github.com/users/pdxjohnny" | |
}, | |
"artifacts_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/actions/runs/6884262695/artifacts", | |
"cancel_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/actions/runs/6884262695/cancel", | |
"check_suite_id": 18230253313, | |
"check_suite_node_id": "CS_kwDOJQW3oM8AAAAEPpuXAQ", | |
"check_suite_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/check-suites/18230253313", | |
"conclusion": null, | |
"created_at": "2023-11-15T23:44:17Z", | |
"display_title": "CI", | |
"event": "workflow_dispatch", | |
"head_branch": "cwt", | |
"head_commit": { | |
"author": { | |
"email": "johnandersenpdx@gmail.com", | |
"name": "John Andersen" | |
}, | |
"committer": { | |
"email": "johnandersenpdx@gmail.com", | |
"name": "John Andersen" | |
}, | |
"id": "e3cc9ad852a6551d92d9eb52f13b2d3208774d41", | |
"message": "IN PROGRESS: did helpers: ecdsa p-384 key loading\n\nSigned-off-by: John Andersen <johnandersenpdx@gmail.com>", | |
"timestamp": "2023-11-15T23:37:36Z", | |
"tree_id": "70268469668b1cbff7e4684528217af7a54d66bb" | |
}, | |
"head_repository": { | |
"archive_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/{archive_format}{/ref}", | |
"assignees_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/assignees{/user}", | |
"blobs_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/git/blobs{/sha}", | |
"branches_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/branches{/branch}", | |
"collaborators_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/collaborators{/collaborator}", | |
"comments_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/comments{/number}", | |
"commits_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/commits{/sha}", | |
"compare_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/compare/{base}...{head}", | |
"contents_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/contents/{+path}", | |
"contributors_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/contributors", | |
"deployments_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/deployments", | |
"description": "SCITT API Emulator", | |
"downloads_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/downloads", | |
"events_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/events", | |
"fork": true, | |
"forks_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/forks", | |
"full_name": "pdxjohnny/scitt-api-emulator", | |
"git_commits_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/git/commits{/sha}", | |
"git_refs_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/git/refs{/sha}", | |
"git_tags_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/git/tags{/sha}", | |
"hooks_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/hooks", | |
"html_url": "https://github.com/pdxjohnny/scitt-api-emulator", | |
"id": 621131680, | |
"issue_comment_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/issues/comments{/number}", | |
"issue_events_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/issues/events{/number}", | |
"issues_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/issues{/number}", | |
"keys_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/keys{/key_id}", | |
"labels_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/labels{/name}", | |
"languages_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/languages", | |
"merges_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/merges", | |
"milestones_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/milestones{/number}", | |
"name": "scitt-api-emulator", | |
"node_id": "R_kgDOJQW3oA", | |
"notifications_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/notifications{?since,all,participating}", | |
"owner": { | |
"avatar_url": "https://avatars.githubusercontent.com/u/5950433?v=4", | |
"events_url": "https://api.github.com/users/pdxjohnny/events{/privacy}", | |
"followers_url": "https://api.github.com/users/pdxjohnny/followers", | |
"following_url": "https://api.github.com/users/pdxjohnny/following{/other_user}", | |
"gists_url": "https://api.github.com/users/pdxjohnny/gists{/gist_id}", | |
"gravatar_id": "", | |
"html_url": "https://github.com/pdxjohnny", | |
"id": 5950433, | |
"login": "pdxjohnny", | |
"node_id": "MDQ6VXNlcjU5NTA0MzM=", | |
"organizations_url": "https://api.github.com/users/pdxjohnny/orgs", | |
"received_events_url": "https://api.github.com/users/pdxjohnny/received_events", | |
"repos_url": "https://api.github.com/users/pdxjohnny/repos", | |
"site_admin": false, | |
"starred_url": "https://api.github.com/users/pdxjohnny/starred{/owner}{/repo}", | |
"subscriptions_url": "https://api.github.com/users/pdxjohnny/subscriptions", | |
"type": "User", | |
"url": "https://api.github.com/users/pdxjohnny" | |
}, | |
"private": false, | |
"pulls_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/pulls{/number}", | |
"releases_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/releases{/id}", | |
"stargazers_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/stargazers", | |
"statuses_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/statuses/{sha}", | |
"subscribers_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/subscribers", | |
"subscription_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/subscription", | |
"tags_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/tags", | |
"teams_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/teams", | |
"trees_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/git/trees{/sha}", | |
"url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator" | |
}, | |
"head_sha": "e3cc9ad852a6551d92d9eb52f13b2d3208774d41", | |
"html_url": "https://github.com/pdxjohnny/scitt-api-emulator/actions/runs/6884262695", | |
"id": 6884262695, | |
"jobs_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/actions/runs/6884262695/jobs", | |
"logs_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/actions/runs/6884262695/logs", | |
"name": "CI", | |
"node_id": "WFR_kwLOJQW3oM8AAAABmlWDJw", | |
"path": ".github/workflows/ci.yml", | |
"previous_attempt_url": null, | |
"pull_requests": [ | |
{ | |
"base": { | |
"ref": "main", | |
"repo": { | |
"id": 560509356, | |
"name": "scitt-api-emulator", | |
"url": "https://api.github.com/repos/scitt-community/scitt-api-emulator" | |
}, | |
"sha": "a30c8182358f2eab1feb380a7bf920b5483060f9" | |
}, | |
"head": { | |
"ref": "cwt", | |
"repo": { | |
"id": 621131680, | |
"name": "scitt-api-emulator", | |
"url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator" | |
}, | |
"sha": "e3cc9ad852a6551d92d9eb52f13b2d3208774d41" | |
}, | |
"id": 1596414276, | |
"number": 39, | |
"url": "https://api.github.com/repos/scitt-community/scitt-api-emulator/pulls/39" | |
} | |
], | |
"referenced_workflows": [], | |
"repository": { | |
"archive_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/{archive_format}{/ref}", | |
"assignees_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/assignees{/user}", | |
"blobs_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/git/blobs{/sha}", | |
"branches_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/branches{/branch}", | |
"collaborators_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/collaborators{/collaborator}", | |
"comments_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/comments{/number}", | |
"commits_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/commits{/sha}", | |
"compare_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/compare/{base}...{head}", | |
"contents_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/contents/{+path}", | |
"contributors_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/contributors", | |
"deployments_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/deployments", | |
"description": "SCITT API Emulator", | |
"downloads_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/downloads", | |
"events_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/events", | |
"fork": true, | |
"forks_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/forks", | |
"full_name": "pdxjohnny/scitt-api-emulator", | |
"git_commits_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/git/commits{/sha}", | |
"git_refs_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/git/refs{/sha}", | |
"git_tags_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/git/tags{/sha}", | |
"hooks_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/hooks", | |
"html_url": "https://github.com/pdxjohnny/scitt-api-emulator", | |
"id": 621131680, | |
"issue_comment_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/issues/comments{/number}", | |
"issue_events_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/issues/events{/number}", | |
"issues_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/issues{/number}", | |
"keys_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/keys{/key_id}", | |
"labels_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/labels{/name}", | |
"languages_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/languages", | |
"merges_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/merges", | |
"milestones_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/milestones{/number}", | |
"name": "scitt-api-emulator", | |
"node_id": "R_kgDOJQW3oA", | |
"notifications_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/notifications{?since,all,participating}", | |
"owner": { | |
"avatar_url": "https://avatars.githubusercontent.com/u/5950433?v=4", | |
"events_url": "https://api.github.com/users/pdxjohnny/events{/privacy}", | |
"followers_url": "https://api.github.com/users/pdxjohnny/followers", | |
"following_url": "https://api.github.com/users/pdxjohnny/following{/other_user}", | |
"gists_url": "https://api.github.com/users/pdxjohnny/gists{/gist_id}", | |
"gravatar_id": "", | |
"html_url": "https://github.com/pdxjohnny", | |
"id": 5950433, | |
"login": "pdxjohnny", | |
"node_id": "MDQ6VXNlcjU5NTA0MzM=", | |
"organizations_url": "https://api.github.com/users/pdxjohnny/orgs", | |
"received_events_url": "https://api.github.com/users/pdxjohnny/received_events", | |
"repos_url": "https://api.github.com/users/pdxjohnny/repos", | |
"site_admin": false, | |
"starred_url": "https://api.github.com/users/pdxjohnny/starred{/owner}{/repo}", | |
"subscriptions_url": "https://api.github.com/users/pdxjohnny/subscriptions", | |
"type": "User", | |
"url": "https://api.github.com/users/pdxjohnny" | |
}, | |
"private": false, | |
"pulls_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/pulls{/number}", | |
"releases_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/releases{/id}", | |
"stargazers_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/stargazers", | |
"statuses_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/statuses/{sha}", | |
"subscribers_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/subscribers", | |
"subscription_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/subscription", | |
"tags_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/tags", | |
"teams_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/teams", | |
"trees_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/git/trees{/sha}", | |
"url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator" | |
}, | |
"rerun_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/actions/runs/6884262695/rerun", | |
"run_attempt": 1, | |
"run_number": 34, | |
"run_started_at": "2023-11-15T23:44:17Z", | |
"status": "in_progress", | |
"triggering_actor": { | |
"avatar_url": "https://avatars.githubusercontent.com/u/5950433?v=4", | |
"events_url": "https://api.github.com/users/pdxjohnny/events{/privacy}", | |
"followers_url": "https://api.github.com/users/pdxjohnny/followers", | |
"following_url": "https://api.github.com/users/pdxjohnny/following{/other_user}", | |
"gists_url": "https://api.github.com/users/pdxjohnny/gists{/gist_id}", | |
"gravatar_id": "", | |
"html_url": "https://github.com/pdxjohnny", | |
"id": 5950433, | |
"login": "pdxjohnny", | |
"node_id": "MDQ6VXNlcjU5NTA0MzM=", | |
"organizations_url": "https://api.github.com/users/pdxjohnny/orgs", | |
"received_events_url": "https://api.github.com/users/pdxjohnny/received_events", | |
"repos_url": "https://api.github.com/users/pdxjohnny/repos", | |
"site_admin": false, | |
"starred_url": "https://api.github.com/users/pdxjohnny/starred{/owner}{/repo}", | |
"subscriptions_url": "https://api.github.com/users/pdxjohnny/subscriptions", | |
"type": "User", | |
"url": "https://api.github.com/users/pdxjohnny" | |
}, | |
"updated_at": "2023-11-15T23:44:25Z", | |
"url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/actions/runs/6884262695", | |
"workflow_id": 52784377, | |
"workflow_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/actions/workflows/52784377" | |
} | |
} |
{ | |
"action": "requested", | |
"repository": { | |
"allow_forking": true, | |
"archive_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/{archive_format}{/ref}", | |
"archived": false, | |
"assignees_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/assignees{/user}", | |
"blobs_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/git/blobs{/sha}", | |
"branches_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/branches{/branch}", | |
"clone_url": "https://github.com/pdxjohnny/scitt-api-emulator.git", | |
"collaborators_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/collaborators{/collaborator}", | |
"comments_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/comments{/number}", | |
"commits_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/commits{/sha}", | |
"compare_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/compare/{base}...{head}", | |
"contents_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/contents/{+path}", | |
"contributors_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/contributors", | |
"created_at": "2023-03-30T03:43:27Z", | |
"default_branch": "auth", | |
"deployments_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/deployments", | |
"description": "SCITT API Emulator", | |
"disabled": false, | |
"downloads_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/downloads", | |
"events_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/events", | |
"fork": true, | |
"forks": 0, | |
"forks_count": 0, | |
"forks_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/forks", | |
"full_name": "pdxjohnny/scitt-api-emulator", | |
"git_commits_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/git/commits{/sha}", | |
"git_refs_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/git/refs{/sha}", | |
"git_tags_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/git/tags{/sha}", | |
"git_url": "git://github.com/pdxjohnny/scitt-api-emulator.git", | |
"has_discussions": false, | |
"has_downloads": true, | |
"has_issues": false, | |
"has_pages": false, | |
"has_projects": true, | |
"has_wiki": true, | |
"homepage": "https://scitt-community.github.io/scitt-api-emulator", | |
"hooks_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/hooks", | |
"html_url": "https://github.com/pdxjohnny/scitt-api-emulator", | |
"id": 621131680, | |
"is_template": false, | |
"issue_comment_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/issues/comments{/number}", | |
"issue_events_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/issues/events{/number}", | |
"issues_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/issues{/number}", | |
"keys_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/keys{/key_id}", | |
"labels_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/labels{/name}", | |
"language": "Python", | |
"languages_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/languages", | |
"license": { | |
"key": "mit", | |
"name": "MIT License", | |
"node_id": "MDc6TGljZW5zZTEz", | |
"spdx_id": "MIT", | |
"url": "https://api.github.com/licenses/mit" | |
}, | |
"merges_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/merges", | |
"milestones_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/milestones{/number}", | |
"mirror_url": null, | |
"name": "scitt-api-emulator", | |
"node_id": "R_kgDOJQW3oA", | |
"notifications_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/notifications{?since,all,participating}", | |
"open_issues": 1, | |
"open_issues_count": 1, | |
"owner": { | |
"avatar_url": "https://avatars.githubusercontent.com/u/5950433?v=4", | |
"events_url": "https://api.github.com/users/pdxjohnny/events{/privacy}", | |
"followers_url": "https://api.github.com/users/pdxjohnny/followers", | |
"following_url": "https://api.github.com/users/pdxjohnny/following{/other_user}", | |
"gists_url": "https://api.github.com/users/pdxjohnny/gists{/gist_id}", | |
"gravatar_id": "", | |
"html_url": "https://github.com/pdxjohnny", | |
"id": 5950433, | |
"login": "pdxjohnny", | |
"node_id": "MDQ6VXNlcjU5NTA0MzM=", | |
"organizations_url": "https://api.github.com/users/pdxjohnny/orgs", | |
"received_events_url": "https://api.github.com/users/pdxjohnny/received_events", | |
"repos_url": "https://api.github.com/users/pdxjohnny/repos", | |
"site_admin": false, | |
"starred_url": "https://api.github.com/users/pdxjohnny/starred{/owner}{/repo}", | |
"subscriptions_url": "https://api.github.com/users/pdxjohnny/subscriptions", | |
"type": "User", | |
"url": "https://api.github.com/users/pdxjohnny" | |
}, | |
"private": false, | |
"pulls_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/pulls{/number}", | |
"pushed_at": "2023-11-15T23:37:40Z", | |
"releases_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/releases{/id}", | |
"size": 217, | |
"ssh_url": "git@github.com:pdxjohnny/scitt-api-emulator.git", | |
"stargazers_count": 0, | |
"stargazers_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/stargazers", | |
"statuses_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/statuses/{sha}", | |
"subscribers_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/subscribers", | |
"subscription_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/subscription", | |
"svn_url": "https://github.com/pdxjohnny/scitt-api-emulator", | |
"tags_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/tags", | |
"teams_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/teams", | |
"topics": [], | |
"trees_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/git/trees{/sha}", | |
"updated_at": "2023-09-12T21:02:36Z", | |
"url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator", | |
"visibility": "public", | |
"watchers": 0, | |
"watchers_count": 0, | |
"web_commit_signoff_required": true | |
}, | |
"sender": { | |
"avatar_url": "https://avatars.githubusercontent.com/u/5950433?v=4", | |
"events_url": "https://api.github.com/users/pdxjohnny/events{/privacy}", | |
"followers_url": "https://api.github.com/users/pdxjohnny/followers", | |
"following_url": "https://api.github.com/users/pdxjohnny/following{/other_user}", | |
"gists_url": "https://api.github.com/users/pdxjohnny/gists{/gist_id}", | |
"gravatar_id": "", | |
"html_url": "https://github.com/pdxjohnny", | |
"id": 5950433, | |
"login": "pdxjohnny", | |
"node_id": "MDQ6VXNlcjU5NTA0MzM=", | |
"organizations_url": "https://api.github.com/users/pdxjohnny/orgs", | |
"received_events_url": "https://api.github.com/users/pdxjohnny/received_events", | |
"repos_url": "https://api.github.com/users/pdxjohnny/repos", | |
"site_admin": false, | |
"starred_url": "https://api.github.com/users/pdxjohnny/starred{/owner}{/repo}", | |
"subscriptions_url": "https://api.github.com/users/pdxjohnny/subscriptions", | |
"type": "User", | |
"url": "https://api.github.com/users/pdxjohnny" | |
}, | |
"workflow": { | |
"badge_url": "https://github.com/pdxjohnny/scitt-api-emulator/workflows/CI/badge.svg", | |
"created_at": "2023-03-30T04:49:33.000Z", | |
"html_url": "https://github.com/pdxjohnny/scitt-api-emulator/blob/auth/.github/workflows/ci.yml", | |
"id": 52784377, | |
"name": "CI", | |
"node_id": "W_kwDOJQW3oM4DJWz5", | |
"path": ".github/workflows/ci.yml", | |
"state": "active", | |
"updated_at": "2023-03-30T04:49:33.000Z", | |
"url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/actions/workflows/52784377" | |
}, | |
"workflow_run": { | |
"actor": { | |
"avatar_url": "https://avatars.githubusercontent.com/u/5950433?v=4", | |
"events_url": "https://api.github.com/users/pdxjohnny/events{/privacy}", | |
"followers_url": "https://api.github.com/users/pdxjohnny/followers", | |
"following_url": "https://api.github.com/users/pdxjohnny/following{/other_user}", | |
"gists_url": "https://api.github.com/users/pdxjohnny/gists{/gist_id}", | |
"gravatar_id": "", | |
"html_url": "https://github.com/pdxjohnny", | |
"id": 5950433, | |
"login": "pdxjohnny", | |
"node_id": "MDQ6VXNlcjU5NTA0MzM=", | |
"organizations_url": "https://api.github.com/users/pdxjohnny/orgs", | |
"received_events_url": "https://api.github.com/users/pdxjohnny/received_events", | |
"repos_url": "https://api.github.com/users/pdxjohnny/repos", | |
"site_admin": false, | |
"starred_url": "https://api.github.com/users/pdxjohnny/starred{/owner}{/repo}", | |
"subscriptions_url": "https://api.github.com/users/pdxjohnny/subscriptions", | |
"type": "User", | |
"url": "https://api.github.com/users/pdxjohnny" | |
}, | |
"artifacts_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/actions/runs/6884262695/artifacts", | |
"cancel_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/actions/runs/6884262695/cancel", | |
"check_suite_id": 18230253313, | |
"check_suite_node_id": "CS_kwDOJQW3oM8AAAAEPpuXAQ", | |
"check_suite_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/check-suites/18230253313", | |
"conclusion": null, | |
"created_at": "2023-11-15T23:44:17Z", | |
"display_title": "CI", | |
"event": "workflow_dispatch", | |
"head_branch": "cwt", | |
"head_commit": { | |
"author": { | |
"email": "johnandersenpdx@gmail.com", | |
"name": "John Andersen" | |
}, | |
"committer": { | |
"email": "johnandersenpdx@gmail.com", | |
"name": "John Andersen" | |
}, | |
"id": "e3cc9ad852a6551d92d9eb52f13b2d3208774d41", | |
"message": "IN PROGRESS: did helpers: ecdsa p-384 key loading\n\nSigned-off-by: John Andersen <johnandersenpdx@gmail.com>", | |
"timestamp": "2023-11-15T23:37:36Z", | |
"tree_id": "70268469668b1cbff7e4684528217af7a54d66bb" | |
}, | |
"head_repository": { | |
"archive_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/{archive_format}{/ref}", | |
"assignees_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/assignees{/user}", | |
"blobs_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/git/blobs{/sha}", | |
"branches_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/branches{/branch}", | |
"collaborators_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/collaborators{/collaborator}", | |
"comments_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/comments{/number}", | |
"commits_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/commits{/sha}", | |
"compare_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/compare/{base}...{head}", | |
"contents_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/contents/{+path}", | |
"contributors_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/contributors", | |
"deployments_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/deployments", | |
"description": "SCITT API Emulator", | |
"downloads_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/downloads", | |
"events_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/events", | |
"fork": true, | |
"forks_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/forks", | |
"full_name": "pdxjohnny/scitt-api-emulator", | |
"git_commits_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/git/commits{/sha}", | |
"git_refs_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/git/refs{/sha}", | |
"git_tags_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/git/tags{/sha}", | |
"hooks_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/hooks", | |
"html_url": "https://github.com/pdxjohnny/scitt-api-emulator", | |
"id": 621131680, | |
"issue_comment_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/issues/comments{/number}", | |
"issue_events_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/issues/events{/number}", | |
"issues_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/issues{/number}", | |
"keys_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/keys{/key_id}", | |
"labels_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/labels{/name}", | |
"languages_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/languages", | |
"merges_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/merges", | |
"milestones_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/milestones{/number}", | |
"name": "scitt-api-emulator", | |
"node_id": "R_kgDOJQW3oA", | |
"notifications_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/notifications{?since,all,participating}", | |
"owner": { | |
"avatar_url": "https://avatars.githubusercontent.com/u/5950433?v=4", | |
"events_url": "https://api.github.com/users/pdxjohnny/events{/privacy}", | |
"followers_url": "https://api.github.com/users/pdxjohnny/followers", | |
"following_url": "https://api.github.com/users/pdxjohnny/following{/other_user}", | |
"gists_url": "https://api.github.com/users/pdxjohnny/gists{/gist_id}", | |
"gravatar_id": "", | |
"html_url": "https://github.com/pdxjohnny", | |
"id": 5950433, | |
"login": "pdxjohnny", | |
"node_id": "MDQ6VXNlcjU5NTA0MzM=", | |
"organizations_url": "https://api.github.com/users/pdxjohnny/orgs", | |
"received_events_url": "https://api.github.com/users/pdxjohnny/received_events", | |
"repos_url": "https://api.github.com/users/pdxjohnny/repos", | |
"site_admin": false, | |
"starred_url": "https://api.github.com/users/pdxjohnny/starred{/owner}{/repo}", | |
"subscriptions_url": "https://api.github.com/users/pdxjohnny/subscriptions", | |
"type": "User", | |
"url": "https://api.github.com/users/pdxjohnny" | |
}, | |
"private": false, | |
"pulls_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/pulls{/number}", | |
"releases_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/releases{/id}", | |
"stargazers_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/stargazers", | |
"statuses_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/statuses/{sha}", | |
"subscribers_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/subscribers", | |
"subscription_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/subscription", | |
"tags_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/tags", | |
"teams_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/teams", | |
"trees_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/git/trees{/sha}", | |
"url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator" | |
}, | |
"head_sha": "e3cc9ad852a6551d92d9eb52f13b2d3208774d41", | |
"html_url": "https://github.com/pdxjohnny/scitt-api-emulator/actions/runs/6884262695", | |
"id": 6884262695, | |
"jobs_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/actions/runs/6884262695/jobs", | |
"logs_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/actions/runs/6884262695/logs", | |
"name": "CI", | |
"node_id": "WFR_kwLOJQW3oM8AAAABmlWDJw", | |
"path": ".github/workflows/ci.yml", | |
"previous_attempt_url": null, | |
"pull_requests": [ | |
{ | |
"base": { | |
"ref": "main", | |
"repo": { | |
"id": 560509356, | |
"name": "scitt-api-emulator", | |
"url": "https://api.github.com/repos/scitt-community/scitt-api-emulator" | |
}, | |
"sha": "a30c8182358f2eab1feb380a7bf920b5483060f9" | |
}, | |
"head": { | |
"ref": "cwt", | |
"repo": { | |
"id": 621131680, | |
"name": "scitt-api-emulator", | |
"url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator" | |
}, | |
"sha": "e3cc9ad852a6551d92d9eb52f13b2d3208774d41" | |
}, | |
"id": 1596414276, | |
"number": 39, | |
"url": "https://api.github.com/repos/scitt-community/scitt-api-emulator/pulls/39" | |
} | |
], | |
"referenced_workflows": [], | |
"repository": { | |
"archive_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/{archive_format}{/ref}", | |
"assignees_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/assignees{/user}", | |
"blobs_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/git/blobs{/sha}", | |
"branches_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/branches{/branch}", | |
"collaborators_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/collaborators{/collaborator}", | |
"comments_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/comments{/number}", | |
"commits_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/commits{/sha}", | |
"compare_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/compare/{base}...{head}", | |
"contents_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/contents/{+path}", | |
"contributors_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/contributors", | |
"deployments_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/deployments", | |
"description": "SCITT API Emulator", | |
"downloads_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/downloads", | |
"events_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/events", | |
"fork": true, | |
"forks_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/forks", | |
"full_name": "pdxjohnny/scitt-api-emulator", | |
"git_commits_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/git/commits{/sha}", | |
"git_refs_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/git/refs{/sha}", | |
"git_tags_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/git/tags{/sha}", | |
"hooks_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/hooks", | |
"html_url": "https://github.com/pdxjohnny/scitt-api-emulator", | |
"id": 621131680, | |
"issue_comment_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/issues/comments{/number}", | |
"issue_events_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/issues/events{/number}", | |
"issues_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/issues{/number}", | |
"keys_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/keys{/key_id}", | |
"labels_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/labels{/name}", | |
"languages_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/languages", | |
"merges_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/merges", | |
"milestones_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/milestones{/number}", | |
"name": "scitt-api-emulator", | |
"node_id": "R_kgDOJQW3oA", | |
"notifications_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/notifications{?since,all,participating}", | |
"owner": { | |
"avatar_url": "https://avatars.githubusercontent.com/u/5950433?v=4", | |
"events_url": "https://api.github.com/users/pdxjohnny/events{/privacy}", | |
"followers_url": "https://api.github.com/users/pdxjohnny/followers", | |
"following_url": "https://api.github.com/users/pdxjohnny/following{/other_user}", | |
"gists_url": "https://api.github.com/users/pdxjohnny/gists{/gist_id}", | |
"gravatar_id": "", | |
"html_url": "https://github.com/pdxjohnny", | |
"id": 5950433, | |
"login": "pdxjohnny", | |
"node_id": "MDQ6VXNlcjU5NTA0MzM=", | |
"organizations_url": "https://api.github.com/users/pdxjohnny/orgs", | |
"received_events_url": "https://api.github.com/users/pdxjohnny/received_events", | |
"repos_url": "https://api.github.com/users/pdxjohnny/repos", | |
"site_admin": false, | |
"starred_url": "https://api.github.com/users/pdxjohnny/starred{/owner}{/repo}", | |
"subscriptions_url": "https://api.github.com/users/pdxjohnny/subscriptions", | |
"type": "User", | |
"url": "https://api.github.com/users/pdxjohnny" | |
}, | |
"private": false, | |
"pulls_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/pulls{/number}", | |
"releases_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/releases{/id}", | |
"stargazers_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/stargazers", | |
"statuses_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/statuses/{sha}", | |
"subscribers_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/subscribers", | |
"subscription_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/subscription", | |
"tags_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/tags", | |
"teams_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/teams", | |
"trees_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/git/trees{/sha}", | |
"url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator" | |
}, | |
"rerun_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/actions/runs/6884262695/rerun", | |
"run_attempt": 1, | |
"run_number": 34, | |
"run_started_at": "2023-11-15T23:44:17Z", | |
"status": "queued", | |
"triggering_actor": { | |
"avatar_url": "https://avatars.githubusercontent.com/u/5950433?v=4", | |
"events_url": "https://api.github.com/users/pdxjohnny/events{/privacy}", | |
"followers_url": "https://api.github.com/users/pdxjohnny/followers", | |
"following_url": "https://api.github.com/users/pdxjohnny/following{/other_user}", | |
"gists_url": "https://api.github.com/users/pdxjohnny/gists{/gist_id}", | |
"gravatar_id": "", | |
"html_url": "https://github.com/pdxjohnny", | |
"id": 5950433, | |
"login": "pdxjohnny", | |
"node_id": "MDQ6VXNlcjU5NTA0MzM=", | |
"organizations_url": "https://api.github.com/users/pdxjohnny/orgs", | |
"received_events_url": "https://api.github.com/users/pdxjohnny/received_events", | |
"repos_url": "https://api.github.com/users/pdxjohnny/repos", | |
"site_admin": false, | |
"starred_url": "https://api.github.com/users/pdxjohnny/starred{/owner}{/repo}", | |
"subscriptions_url": "https://api.github.com/users/pdxjohnny/subscriptions", | |
"type": "User", | |
"url": "https://api.github.com/users/pdxjohnny" | |
}, | |
"updated_at": "2023-11-15T23:44:17Z", | |
"url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/actions/runs/6884262695", | |
"workflow_id": 52784377, | |
"workflow_url": "https://api.github.com/repos/pdxjohnny/scitt-api-emulator/actions/workflows/52784377" | |
} | |
} |