-
-
Save petertwise/26611bbcfc63f9c7e3a7dc45e9145133 to your computer and use it in GitHub Desktop.
<?php | |
/** | |
* Create a Google Calendar "add to calendar" link. | |
* | |
* This function is convienient because it does not require an API connection. | |
* Note that this only allows for adding a single event. | |
* The data does not have to exist already on any Google Calendar anywhere. | |
* This just adds your event data to the end-users GCal one item at a time. | |
* See https://stackoverflow.com/a/19867654/947370 for a full explaination of | |
* the Google Calendar URL structure. | |
* | |
* @author Peter Wise / Square Candy Design | |
* @link https://stackoverflow.com/a/19867654/947370 | |
* | |
* @param string $name The main title of the event | |
* @param string $startdate The start date and time in any format that strtotime can digest | |
* @param string $enddate The end date and time in any format that strtotime can digest | |
* @param string $description The longer description text of the event | |
* @param string $location The event location - any text google maps can parse as a single point | |
* @param bool $allday Is the event "All Day" with no times displayed? | |
* @param string $linktext The text of the link. Defaults to 'Add to gCal'. | |
* @param array $classes An array of classes to add to the link element. | |
* @return string An HTML link to add the event | |
*/ | |
function squarecandy_add_to_gcal( | |
$name, | |
$startdate, | |
$enddate = false, | |
$description = false, | |
$location = false, | |
$allday = false, | |
$linktext = 'Add to gCal', | |
$classes = array('gcal-button, button') | |
) { | |
// calculate the start and end dates, convert to ISO format | |
if ($allday) { | |
$startdate = date('Ymd',strtotime($startdate)); | |
} | |
else { | |
$startdate = date('Ymd\THis',strtotime($startdate)); | |
} | |
if ($enddate && !empty($enddate) && strlen($enddate) > 2) { | |
if ($allday) { | |
$enddate = date('Ymd',strtotime($enddate . ' + 1 day')); | |
} | |
else { | |
$enddate = date('Ymd\THis',strtotime($enddate)); | |
} | |
} | |
else { | |
$enddate = date('Ymd\THis',strtotime($startdate . ' + 2 hours')); | |
} | |
// build the url | |
$url = 'http://www.google.com/calendar/event?action=TEMPLATE'; | |
$url .= '&text=' . rawurlencode($name); | |
$url .= '&dates=' . $startdate . '/' . $enddate; | |
if ($description) { | |
$url .= '&details=' . rawurlencode($description); | |
} | |
if ($location) { | |
$url .= '&location=' . rawurlencode($location); | |
} | |
// build the link output | |
$output = '<a href="' . $url . '" class="' . implode(' ',$classes) . '">'.$linktext.'</a>'; | |
return $output; | |
} | |
/******************** | |
* | |
* Example Usage: | |
* | |
* echo squarecandy_add_to_gcal('Example Event', 'June 30, 2017 8:00pm'); | |
* echo squarecandy_add_to_gcal('Example Event', 'June 30, 2017 8:00pm', 'July 2, 2017 10:00am', 'This is my detailed event description', '1600 Pennsylvania Ave NW, Washington, DC 20500'); | |
* echo squarecandy_add_to_gcal('Example Event', 'June 30, 2017', 'July 2, 2017', 'This is my detailed event description', '1600 Pennsylvania Ave NW, Washington, DC 20500', true, 'gCal+', array('my-custom-class') ); | |
* | |
*/ | |
?> |
@nmj18txstate - see the example usage section in the comments at the bottom of the file. The function doesn't print anything to the screen by itself. You must call it with the details of your particular event within your own custom code.
Hi!
do you know what the attribute for the 'reminder' or 'notification' option is? I would love to be able to set up at least one notification setting.
Thank you!
@vanesa - unfortunately I think you might need to use the Google API v3 for that, which requires connecting with an API key or other authentication and is overwhelmingly more annoying than just setting up a url...
Is there anything like this that you can provide a complete iCal instead of a single event?
@dawogfather - nope, you need to go for the Calendar API to get that.
Nice and thanks for sharing, but would benefit from including the timezone (cz
) parameter.
Please find the below screenshots, i executed the function in netbeans and in my webbrowser but i'm getting no output on screen. Please help me figure out the problem. Am i missing something apart from this ?
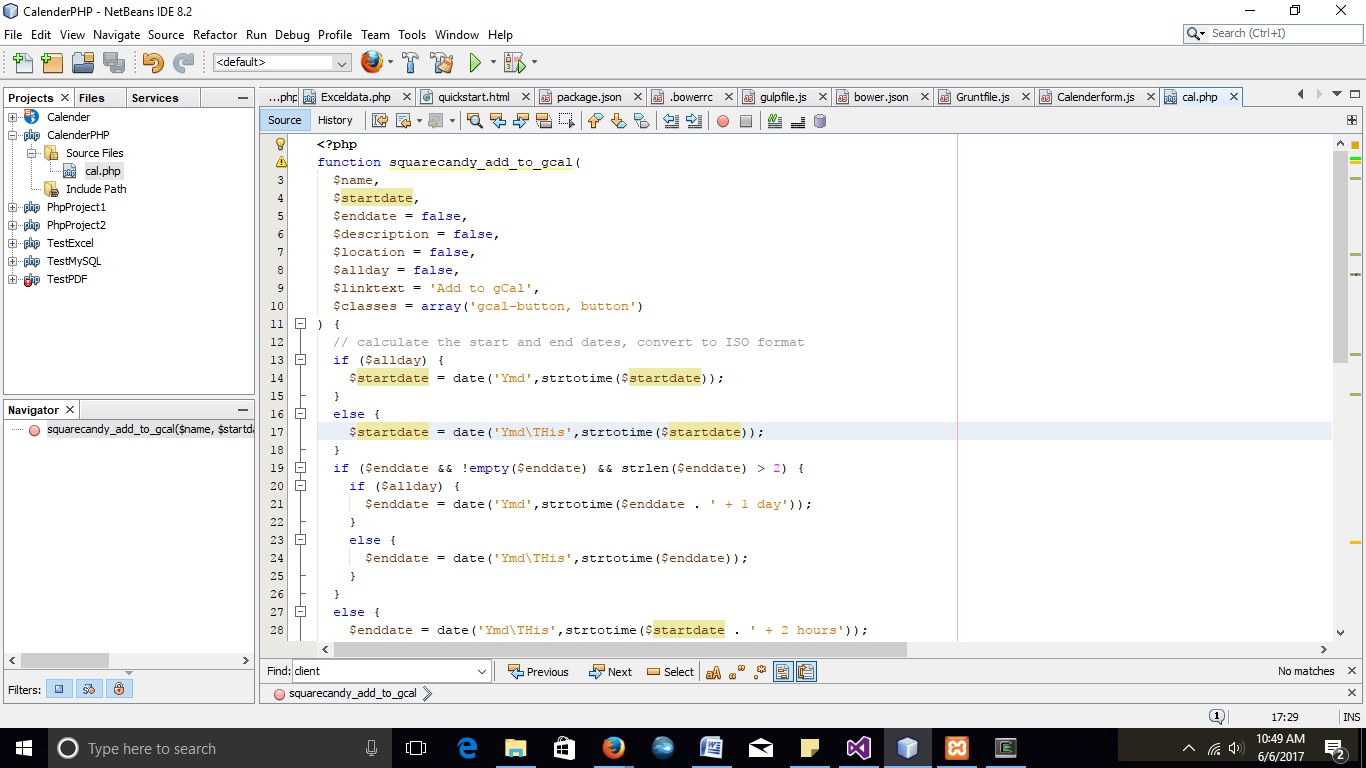
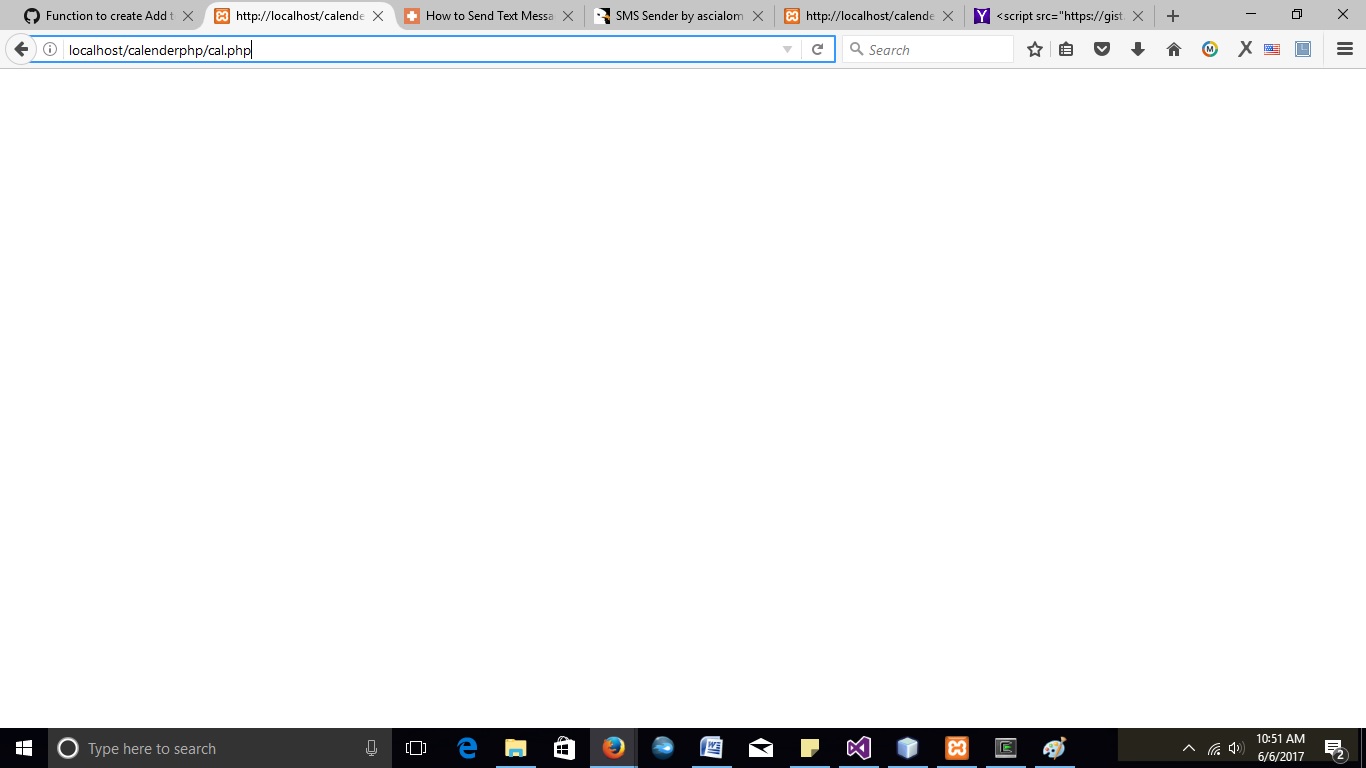