Last active
February 2, 2022 08:24
-
-
Save pexavc/c58b4df48a400460f2aa8885029ead5f to your computer and use it in GitHub Desktop.
[ Understanding Shaders ] // :: Simplex Noise
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
vec2 hash( vec2 p ) // replace this by something better | |
{ | |
p = vec2( dot(p,vec2(127.1,311.7)), dot(p,vec2(269.5,183.3)) ); | |
return -1.0 + 2.0*fract(sin(p)*43758.5453123); | |
} | |
float noise( in vec2 p ) | |
{ | |
const float K1 = 0.366025404; // (sqrt(3)-1)/2; | |
const float K2 = 0.211324865; // (3-sqrt(3))/6; | |
// It seems here we define the simplex in which we are | |
vec2 i = floor( p + (p.x+p.y)*K1 ); | |
vec2 a = p - i + (i.x+i.y)*K2; | |
// This is the fade function | |
float m = step(a.y,a.x); | |
// What happens next I have no idea.... | |
// .......................................................... | |
vec2 o = vec2 (m, 1.0-m); | |
vec2 b = a - o + K2; | |
vec2 c = a - 1.0 + 2.0*K2; | |
vec3 h = max( 0.5-vec3(dot(a,a), dot(b,b), dot(c,c) ), 0.0 ); | |
vec3 n = h*h*h*h*vec3( dot(a,hash(i+0.0)), dot(b,hash(i+o)), dot(c,hash(i+1.0))); | |
return dot( n, vec3(70.0) ); | |
} |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Almost the same effect is achieved in a simpler way.
Source: https://gamedev.ru/code/forum/?id=255209
Interesting read. Looking into Perlin noise.
Desired Effect:
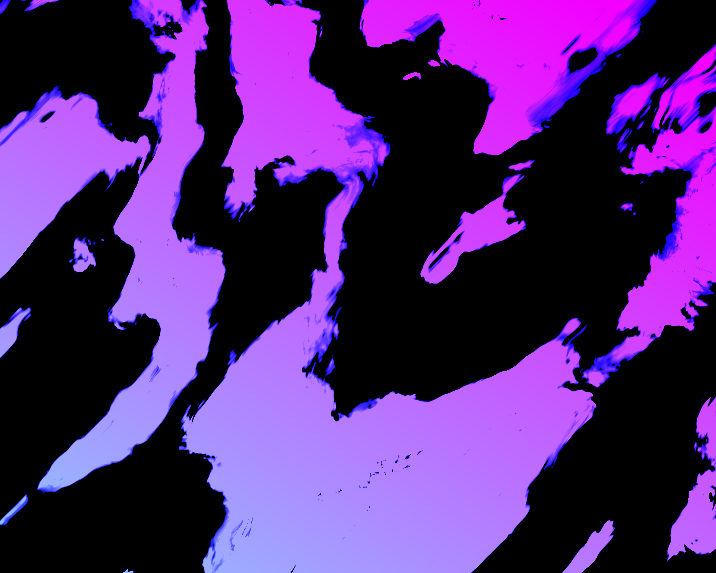