Last active
June 18, 2020 17:14
-
-
Save pgiu/b5bd3014fc9ce2aef2397399455b71ca to your computer and use it in GitHub Desktop.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
#!/usr/bin/python3 | |
# Plugin para mostrar el estado de aplicaciones de P13N en la barra de aplicaciones en gnome usando Argos. | |
# Le pega a olympus para traerse el estado de las aplicaciones. | |
# ¿Cómo usarlo en Ubuntu? | |
# 1. Instalar Argos | |
# https://extensions.gnome.org/extension/1176/argos/ | |
# 2. Copiar este archivo a ~/.config/argos (mantener el nombre) | |
# | |
# Para más información sobre Argos, visitar la página del proyecto: https://github.com/p-e-w/argos | |
# | |
# Usas Mac? No hay problema! | |
# => Este plugin debería ser compatible con BitBar (https://github.com/matryer/bitbar) | |
# | |
import datetime | |
import requests | |
from requests import ConnectTimeout | |
def get_emoji_for_state(state): | |
# Para más ćódigos de emojis buscar en: https://www.webfx.com/tools/emoji-cheat-sheet/ | |
if state == 'GREEN': | |
return ':sunny:' | |
if state == 'GREY': | |
return ' :cloud:' | |
if state == 'timeout': | |
return ':no_entry_sign:' | |
else: | |
return ':fire:' | |
def format_url(url): | |
return "href='" + url + "'" | |
def extract_host_name(json): | |
try: | |
# Also convert to int since update_time will be string. When comparing | |
# strings, "10" is smaller than "2". | |
return json['app_details']['app_name'] | |
except KeyError: | |
return 0 | |
def get_app_state(environment, app_name): | |
url = 'http://backoffice.despegar.com/olympus/status/all/raw?env=' + environment + '&app_name=' + app_name | |
try: | |
response = requests.get(url, verify=False, timeout=1) | |
except ConnectTimeout: | |
return app_name + ": timeout! :skull: | " + format_url(url), 'timeout' | |
parsed = response.json() | |
# print(json.dumps(parsed, indent=4, sort_keys=True)) | |
general_state = 'GREEN' | |
c = app_name + " " | |
hosts = "" | |
parsed.sort(key=lambda k: k['app_details']['host_name']) | |
for elem in parsed: | |
state = elem['data']['general'] | |
host_name = elem['app_details']['host_name'] | |
if state == 'RED': | |
general_state = state | |
hosts += "--" + get_emoji_for_state(state) + " " + host_name + " | " + format_url(url) + ' \n' | |
return get_emoji_for_state(general_state) + " " + c + "\n" + hosts, general_state | |
def main(): | |
# Agregar o remover aplicaciones de esta lista | |
apps = ['freya', 'euler-aws', 'p13n-spark-scheduler'] | |
environment = 'prod' # Otros valores posibles: beta | |
general_state = 'GREEN' | |
app_status = '' | |
for app in apps: | |
res, state = get_app_state(environment, app) | |
app_status += res + "\n" | |
if state != 'GREEN': | |
general_state = state | |
print("Olympus " + environment + " " + get_emoji_for_state(general_state)) | |
print("---") | |
print(app_status) | |
now = datetime.datetime.now().strftime("%Y-%m-%d %H:%M:%S") | |
print("Actualizado: " + now) | |
print("Click para refrescar | refresh=true") | |
if __name__ == "__main__": | |
main() |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Así se ve en funcionamiento:
Este es el mapeo de estados a emojis
Cuando no puede conectarse (vpn?) se muestra así
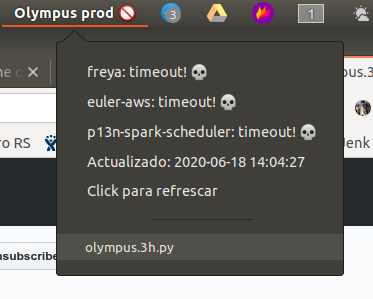