Last active
September 30, 2019 06:25
-
-
Save phlippieb/8a250129fd11cbe0f7902981d612a0e8 to your computer and use it in GitHub Desktop.
Extremely simple sign in with apple test app
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
/* | |
In anticipation of iOS 13, I'm implementing Sign in with Apple. | |
To get started, I just made a simple VC that brings up the auth stuff, without even handling the result. | |
When running it in a simulator and completing the sign in process, the process appears to be broken: | |
- The auth controller is not dismissed | |
- `ASAuthorizationController`'s delegate method `authorizationController:didCompleteWithAuthorization` is not called | |
The code below is intended as a simple copy+paste to make problem replication easier: | |
1. Create a new XCode project | |
2. Choose the "Single View App" template | |
3. Choose to use StoryBoards (not SwiftUI) | |
4. (Project name, location, etc doesn't matter) | |
5. In the project's settings, under the "Signing and Capabilities" tab, add the "Sign in with Apple" capability to your target. | |
6. Replace the contents of ViewController.swift with the code below. | |
7. Add breakpoints where indicated (at the two delegate methods). | |
*/ | |
import UIKit | |
import AuthenticationServices | |
class ViewController: UIViewController { | |
override func viewDidLoad() { | |
super.viewDidLoad() | |
self.view.backgroundColor = .white | |
let signInButton = ASAuthorizationAppleIDButton() | |
signInButton.addTarget(self, action: #selector(onSignIn), for: .touchUpInside) | |
self.view.addSubview(signInButton) | |
signInButton.translatesAutoresizingMaskIntoConstraints = false | |
signInButton.centerXAnchor.constraint(equalTo: self.view.centerXAnchor).isActive = true | |
signInButton.centerYAnchor.constraint(equalTo: self.view.centerYAnchor).isActive = true | |
} | |
@objc private func onSignIn() { | |
let request = ASAuthorizationAppleIDProvider().createRequest() | |
request.requestedScopes = [.fullName] | |
let controller = ASAuthorizationController( | |
authorizationRequests: [request]) | |
controller.delegate = self | |
controller.presentationContextProvider = self | |
controller.performRequests() | |
} | |
} | |
extension ViewController: ASAuthorizationControllerDelegate { | |
func authorizationController(controller: ASAuthorizationController, didCompleteWithError error: Error) { | |
print() // <-- Put a breakpoint here | |
} | |
func authorizationController(controller: ASAuthorizationController, didCompleteWithAuthorization authorization: ASAuthorization) { | |
print() // <-- Put a breakpoint here | |
} | |
} | |
extension ViewController: ASAuthorizationControllerPresentationContextProviding { | |
func presentationAnchor(for controller: ASAuthorizationController) -> ASPresentationAnchor { | |
return self.view.window! | |
} | |
} | |
/* | |
Now, when you run the app, tap on the "sign-in" button, and follow the sign-in process. | |
After you complete the process and enter your password, the auth controller will stay on-screen, and your breakpoints won't get hit. | |
Environment info | |
---------------- | |
- MacOS: Mojave 10.14.6 (18G95) | |
- XCode: Version 11.0 (11A419c) | |
- Simulator: Version 11.0 (SimulatorApp-912.1 SimulatorKit-570.3 CoreSimulator-681.5.1) | |
- Simulator iOS: 13.0 (17A577) | |
*/ |
Update: Deploying this app to a real device (not a simulator) resulted in the flow actually working.
- On a simulator, the final step of signing in is where you enter your password (as pictured in the screenshot above)
- On my phone, I didn't get the password option; instead, the phone went to face ID, and completed the flow successfully
This leads me to suspect that the iOS 13 Beta (at least the one on the simulator in the XCode 11 Beta) has a buggy Apple ID auth flow; hopefully this will be fixed in time for the public release of iOS 13!
This sign in with Apple flow seems fine now in iOS 13.1 by the simulator.
This sign in with Apple flow seems fine now in iOS 13.1 by the simulator.
Agreed 👍
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
This is the screen where you get stuck:
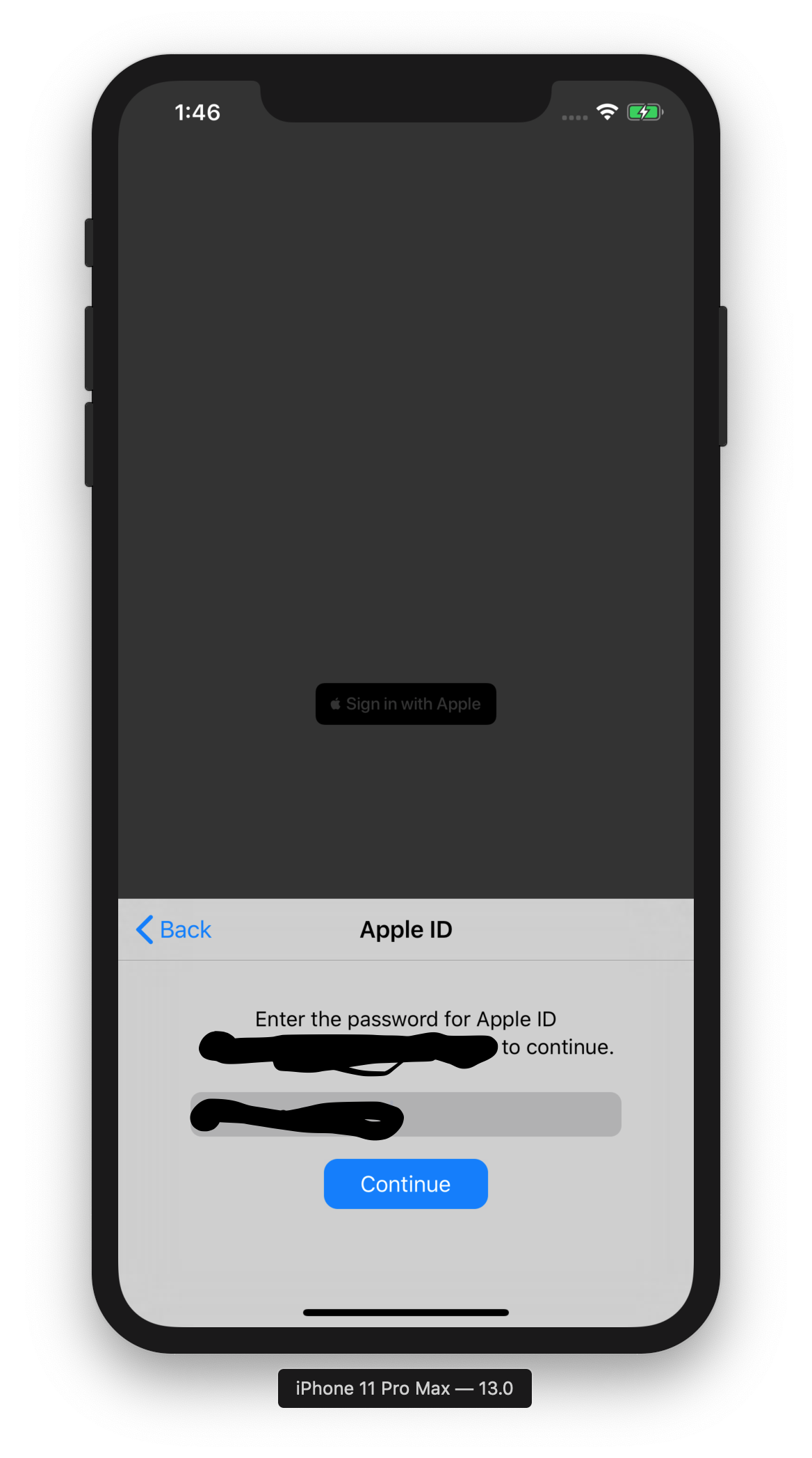