Last active
February 8, 2021 02:04
-
-
Save phpenterprise/101679894fc15c51c628c299df56a64a to your computer and use it in GitHub Desktop.
Configure Python as a service in AWS EC2
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
#!/bin/bash | |
# | |
# custom-service Start up custom-service | |
# | |
# chkconfig: 2345 55 25 | |
# description: the custom service (Python) | |
# | |
# processname: custom-service | |
# Source function library | |
. /etc/init.d/functions | |
# set the python script name | |
SNAME=custom-service.py | |
# the path directory and name of the python program (warning: do not change $SNAME var) | |
PROG=/var/www/python/$SNAME | |
# start function | |
start() { | |
#check the daemon status first | |
if [ -f /var/lock/subsys/$SNAME ] | |
then | |
echo "$SNAME is already started!" | |
exit 0; | |
else | |
echo $"Starting $SNAME ..." | |
nohup python $PROG & | |
[ $? -eq 0 ] && touch /var/lock/subsys/$SNAME | |
echo $"$SNAME started." | |
exit 0; | |
fi | |
} | |
#stop function | |
stop() { | |
echo "Stopping $SNAME ..." | |
pid=`ps -ef | grep "$PROG" | grep -v 'grep' | awk '{ print $2 }' | head -1` | |
if [ "$pid" != "" ]; then | |
echo "Kill proccess " + $pid | |
kill $pid | |
rm -rf /var/lock/subsys/$SNAME | |
else | |
echo "$SNAME is stoped." | |
rm -rf /var/lock/subsys/$SNAME | |
fi | |
exit 0; | |
} | |
case "$1" in | |
start) | |
start | |
;; | |
stop) | |
stop | |
;; | |
reload|restart) | |
stop | |
start | |
;; | |
status) | |
pid=`ps -ef | grep "$PROG" | grep -v 'grep' | awk '{ print $2 }' | head -1` | |
if [ "$pid" = "" ]; then | |
echo "$SNAME is stoped. " | |
else | |
echo "$SNAME (pid $pid) is running..." | |
fi | |
;; | |
*) | |
echo $"\nUsage: $0 {start|stop|restart|status}" | |
exit 1 | |
esac |
sudo chmod +x /etc/init.d/custom-service
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Configure a Python as a service in AWS EC2
After much unsuccessful research to set up a Python API written on custom port 8080 to run on Amazon's Linux AMI operating system (AWS), I decided to solve this dilemma and share the solution with all of you.
Fact 1
There is nothing in the official documentation on Amazon AWS talking about it. I have worked with Linux versions such as Centos 6/7, RedHat and Debian and had not faced such difficulty.
Fact 2
This version of Amazon is my favorite because it is compact and stable with no pre-installed plugins and unnecessary tools. Because of this, a great deal needs to be implemented in hand or an auxiliary tool for managing a specific service installed. In this case what we want is to add third party services (in Python) auto bootable on start.
Fact 3:
This version of the Linux operating system does not have the "systemd" or "systemctl" modules and cannot be installed because they do not exist in the repositories of recent versions of AMI on AWS.
Solution
I did a thorough analysis of the startup directories used by other programs to reverse engineer how they created the services and enabled automatic startup in system reboot.
Installation
1. create custom service file
$ sudo nano /etc/init.d/custom-service
2. copy the contents of the file above
Paste with the right mouse button
3. remove the .sh extension if you downloaded the file
$ mv custom-service.sh custom-service
4. override service configuration, Python file paths and directory
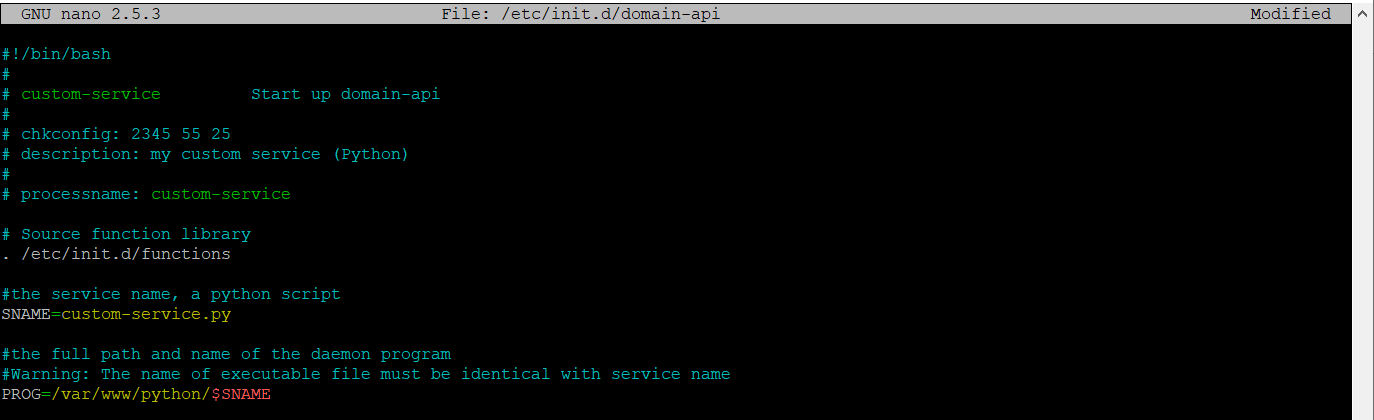
5. save (CTRL + O) and exit NANO editor (CTRL + X)

6. start the service by running the command
$ sudo service custom-service start
If Python file is OK it will run perfectly
7. check if the service is active (running)
$ sudo service custom-service status
Test the core of the service or if it's an HTTP API, test access in your browser
8. To stop the service, type the command below.
$ sudo service custom-service stop
Setting up autostart at boot
1. Put the service on startup
$ sudo chkconfig custom-service on
2. Test the existence of the service
$ sudo chkconfig --list custom-service
If it appears as "ON" is installed correctly.
Okay, I hope it helped you. Leave your comments if successful.