Last active
August 30, 2023 08:10
-
-
Save postmalloc/e2602752d46c5b9dee24462356f96cca to your computer and use it in GitHub Desktop.
Hacker News comments sidebar bookmarklet
Update: As suggested in the HN discussion, I removed the reliance on escaping, and instead create DOM nodes directly. This should minimise the chances of XSS.
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Usage:
Note: It will not work on websites (e.g. github.com) that have CSP restrictions
Screenshot:
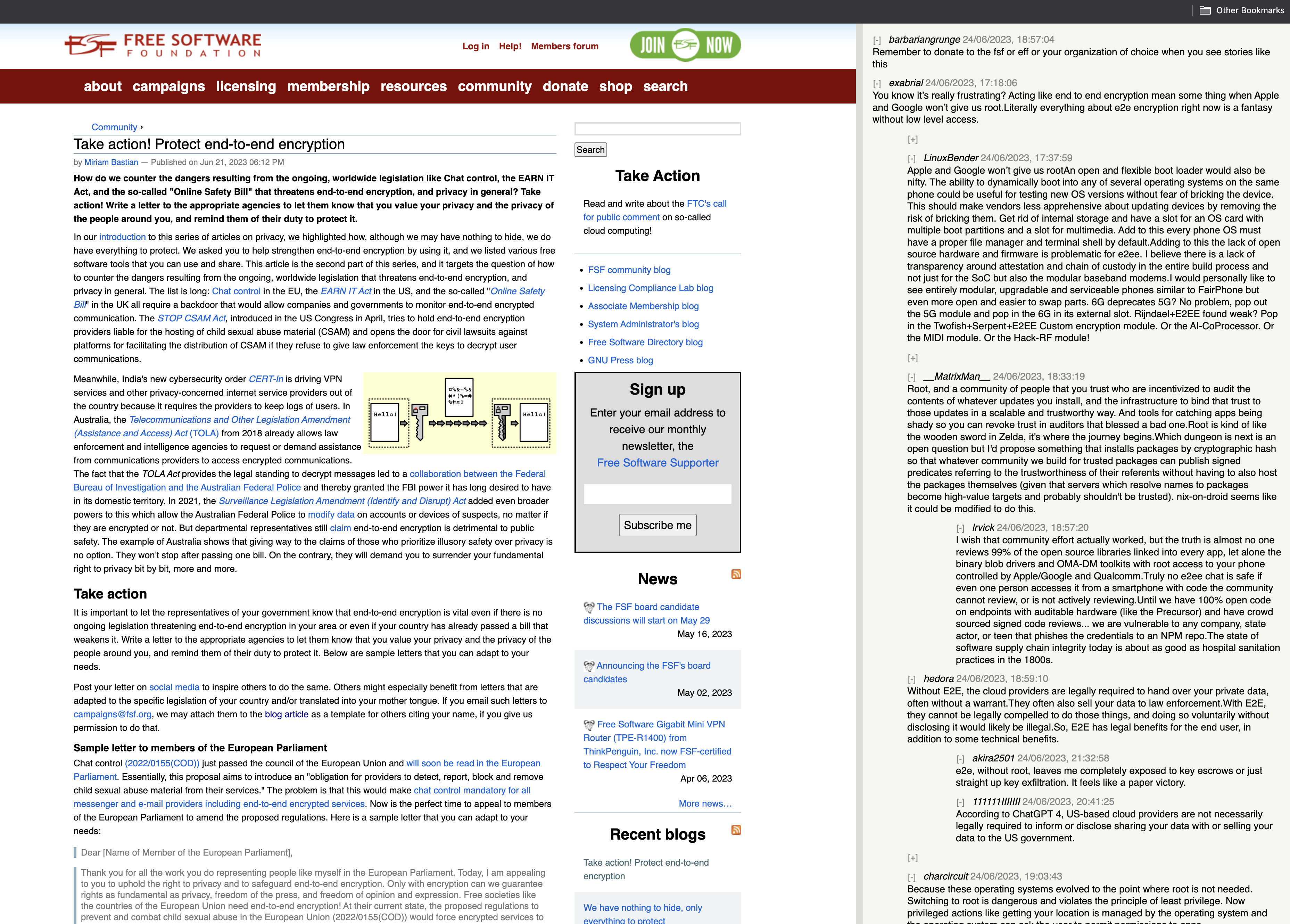