Created
September 20, 2020 20:30
-
-
Save prof3ssorSt3v3/98e8466a2f2be4f6c538958309f77381 to your computer and use it in GitHub Desktop.
Working with Select, Option, and Optgroup
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
<!DOCTYPE html> | |
<html lang="en"> | |
<head> | |
<meta charset="UTF-8" /> | |
<meta name="viewport" content="width=device-width, initial-scale=1.0" /> | |
<title>Document</title> | |
<style> | |
/* Default styles */ | |
*, | |
*::after, | |
*::before { | |
padding: 0; | |
margin: 0; | |
box-sizing: border-box; | |
} | |
html { | |
font-size: 20px; | |
line-height: 1.6; | |
font-family: -apple-system, BlinkMacSystemFont, 'Segoe UI', Roboto, | |
Oxygen, Ubuntu, Cantarell, 'Open Sans', 'Helvetica Neue', sans-serif; | |
} | |
header { | |
margin: 2rem auto; | |
} | |
h1 { | |
padding: 0rem 2rem; | |
color: #333; | |
font-family: 'Raleway', sans-serif; | |
} | |
/* Form styles */ | |
form { | |
margin: 2rem auto; | |
padding: 1rem; | |
} | |
.formBox { | |
display: grid; | |
grid-template-columns: 1fr 3fr; | |
gap: 1rem; | |
margin: 2rem 0; | |
} | |
label { | |
font-size: 1rem; | |
padding: 0.2rem 0; | |
font-weight: 300; | |
color: #777; | |
text-align: right; | |
} | |
select, | |
input, | |
button, | |
option, | |
optgroup, | |
textarea { | |
font-size: 1rem; | |
font-weight: 500; | |
color: #555; | |
padding: 0.2rem 0.5rem; | |
margin: 0 1rem 0 0; | |
} | |
optgroup { | |
color: #999; | |
font-weight: 200; | |
padding-left: 0.2rem; | |
} | |
.buttons button { | |
grid-column-start: 2; | |
grid-column-end: 3; | |
background-color: #333; | |
color: #eee; | |
} | |
</style> | |
</head> | |
<body> | |
<header><h1>Select, Options, OptGroup</h1></header> | |
<form action="#" name="myForm" id="myForm"> | |
<div class="formBox"> | |
<label for="thing">Username</label> | |
<input type="email" id="thing" name="thing" /> | |
</div> | |
<div class="formBox"> | |
<label for="flavours">Favourite Flavours</label> | |
<select id="flavours" name="flavours" multiple> | |
<optgroup label="Maybe"> | |
<option value="dust">Dust</option> | |
<option value="beer" selected>Beer</option> | |
<option value="cheese">Cheese</option> | |
</optgroup> | |
<optgroup label="Maybe Not"> | |
<option value="cat">Cat</option> | |
<option value="rust">Rust</option> | |
<option value="octopus">Octopus</option> | |
</optgroup> | |
</select> | |
</div> | |
<div class="formBox buttons"> | |
<button id="btnSend">Send</button> | |
</div> | |
</form> | |
<script> | |
document.addEventListener('DOMContentLoaded', () => { | |
document | |
.getElementById('flavours') | |
.addEventListener('input', handleSelect); | |
document.getElementById('thing').addEventListener('input', handleData); | |
}); | |
function handleSelect(ev) { | |
let select = ev.target; //document.getElementById('flavours'); | |
console.log(select.value); | |
let choices = []; | |
// for (let i = 0; i < select.selectedOptions.length; i++) { | |
// choices.push(select.selectedOptions[i].value); | |
// } | |
choices = [].map.call(select.selectedOptions, (option) => option.value); | |
console.log(choices); | |
} | |
function handleData(ev) { | |
let theInput = ev.target; | |
console.log(theInput.value, typeof theInput.value); | |
} | |
</script> | |
</body> | |
</html> |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Hello, i need help to fill an online form. I am using autofill extension from google. It does well but skips two field. Is there any solution?
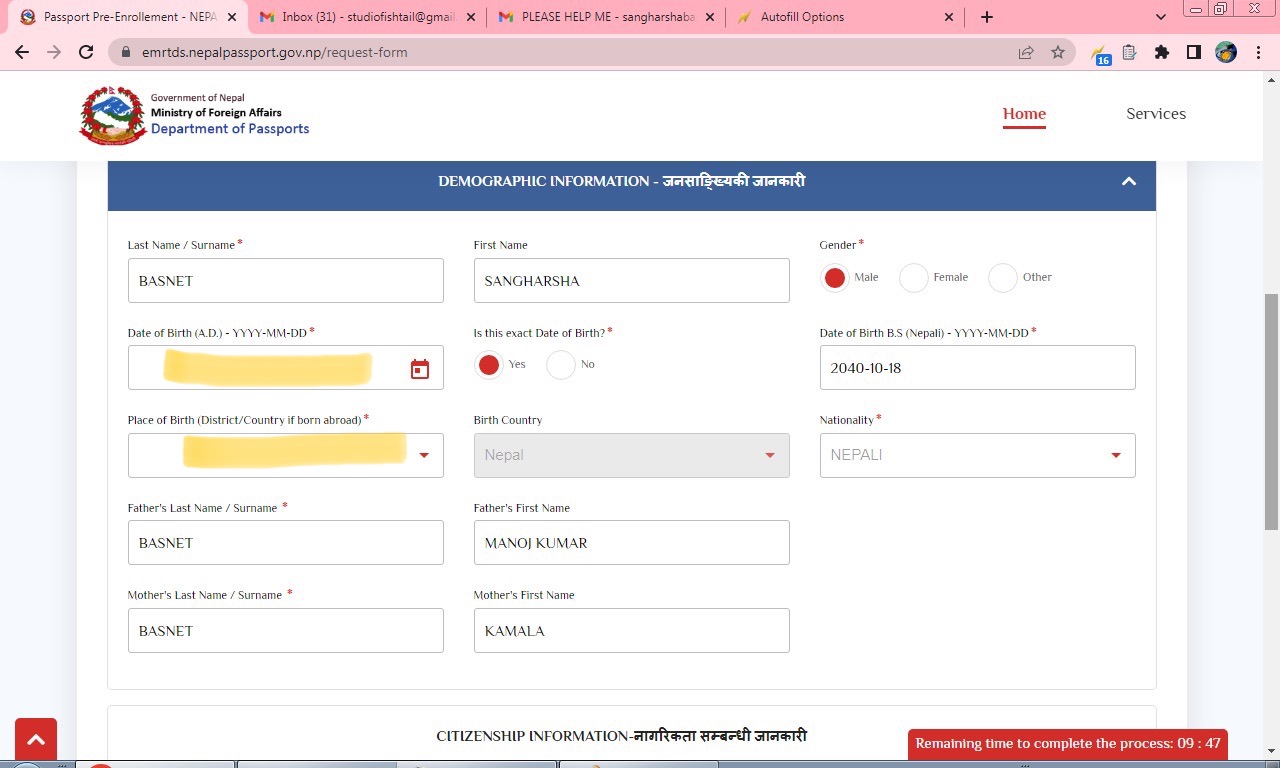