Last active
August 20, 2022 10:57
-
-
Save rBrenick/f192194f0499c14cd4580649be3717f0 to your computer and use it in GitHub Desktop.
progress bar convenience class for Maya
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
__author__ = "RichardBrenick@gmail.com" | |
__created__ = "2022-08-11" | |
from maya import cmds | |
class ProgressBar(object): | |
""" | |
Convenience class for showing a progress bar in Maya | |
---- simple example | |
for i in range(20): | |
ProgressBar("Waiting for something", total=20) | |
time.sleep(0.3) | |
---- little more complex example | |
example_list = range(20) | |
for i in example_list: | |
ProgressBar("ProgressTitle", total=len(example_list), status="example: {}".format(i), wide=True) | |
time.sleep(0.3) | |
""" | |
PROGRESS_BARS = {} | |
def __new__(cls, title, total, status="", wide=True, *args, **kwargs): | |
if ProgressBar.PROGRESS_BARS.get(title): | |
current_amount = cmds.progressWindow(query=True, progress=True) | |
new_amount = current_amount + 1 | |
if new_amount < total: | |
cmds.progressWindow(edit=True, progress=new_amount, status=status) | |
return None | |
if new_amount == total: | |
cmds.progressWindow(endProgress=True) | |
ProgressBar.PROGRESS_BARS.pop(title) | |
return None | |
return super(ProgressBar, cls).__new__(cls, *args, **kwargs) | |
def __init__(self, title, total, status="", wide=True): | |
cmds.progressWindow(endProgress=True) | |
if wide: | |
init_status = " " * 250 | |
else: | |
init_status = status | |
cmds.progressWindow(title=title, progress=1, maxValue=total, status=init_status, isInterruptable=False) | |
cmds.progressWindow(edit=True, status=status) | |
ProgressBar.PROGRESS_BARS[title] = self | |
if __name__ == "__main__": | |
import time | |
example_list = range(100) | |
for i in example_list: | |
ProgressBar("Progress is being made...", total=len(example_list)) | |
time.sleep(0.03) | |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Example
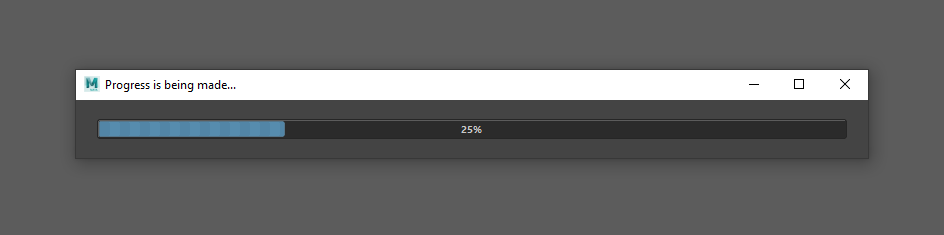