Created
November 6, 2018 16:59
-
-
Save ramytamer/d0065c56212a09fff41184aeb1e25c2a to your computer and use it in GitHub Desktop.
Command to easily compose commit message and commit it
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
#! /usr/bin/env elixir | |
defmodule Commit do | |
def process([]), do: wrong_usage() | |
def process(["--message-only"]), do: wrong_usage() | |
def process(["-m"]), do: wrong_usage() | |
def process([message_only | commit_message]) when message_only in ["--message-only", "-m"], | |
do: commit_message |> format() |> compose(true) |> commit() | |
def process(commit_message), do: commit_message |> format() |> compose() |> commit() | |
defp format(message), do: Enum.join(message, " ") | |
defp compose(message, _message_only = true), do: message | |
defp compose(message) do | |
branch = get_branch_name() | |
"[#{branch}] #{message}" | |
end | |
def get_branch_name() do | |
with {result, 0} <- System.cmd("git", ["branch"]) do | |
result | |
|> String.split("\n") | |
|> List.first() | |
|> String.split(" ") | |
|> List.last() | |
else | |
err -> exit_with_error(err) | |
end | |
end | |
defp commit(message) do | |
with {result, 0} <- System.cmd("git", ["commit", "-S", "-m", message]) do | |
exit_with_success(result) | |
else | |
{_, code} -> exit_with_error("Failed with code: #{code}", code) | |
end | |
end | |
defp exit_with_success(result) do | |
IO.puts("Done 🎉") | |
System.halt(0) | |
end | |
defp wrong_usage(), | |
do: | |
exit_with_error( | |
"Invalid arguments, usage: \n\t c COMMIT_MESSAGE \n\t c [--message-only | -m] COMMIT_MESSAGE" | |
) | |
defp exit_with_error({err, code}), do: exit_with_error(err, code) | |
defp exit_with_error(err, code \\ 1) do | |
IO.puts(:stderr, err) | |
System.halt(code) | |
end | |
end | |
System.argv() |> Commit.process() |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
This command guess the branch name by default and prepend it to the commit message unless it's expliclity passed as an option
-m
or--no-message
before the message to only commit the message without the branch name.You can have it in your system by just adding it to your
$PATH
variable.Usage example:
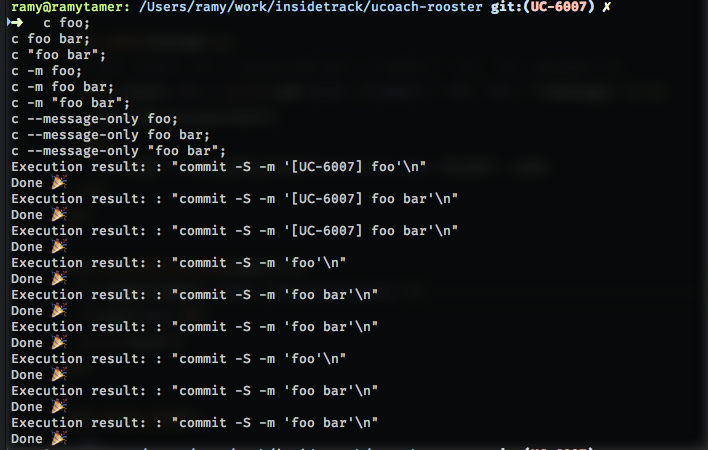