Created
November 1, 2016 07:34
-
-
Save rathankalluri/d88ee0df9bb685b01b19376f578b7998 to your computer and use it in GitHub Desktop.
Draw a Flower in Python
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
import turtle | |
""" Draw a flower in Pyhton""" | |
def draw_square(square): | |
for i in range(0,2): | |
square.forward(100) | |
square.right(30) | |
square.forward(100) | |
square.right(150) | |
def draw_flower(): | |
window = turtle.Screen() | |
window.bgcolor("blue") | |
pen = turtle.Turtle() | |
pen.shape("triangle") | |
pen.color("#99FF00") | |
for i in range(0,36): | |
draw_square(pen) | |
pen.right(10) | |
for i in range(0,4): | |
pen.circle(50) | |
pen.right(90) | |
pen.right(90) | |
pen.forward(300) | |
pen.right(90) | |
draw_square(pen) | |
pen.left(180) | |
draw_square(pen) | |
pen.left(270) | |
pen.forward(200) | |
window.exitonclick() | |
draw_flower() |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
if you want colored petals use this one
import turtle
def draw_square(square):
def draw_flower():
draw_flower()
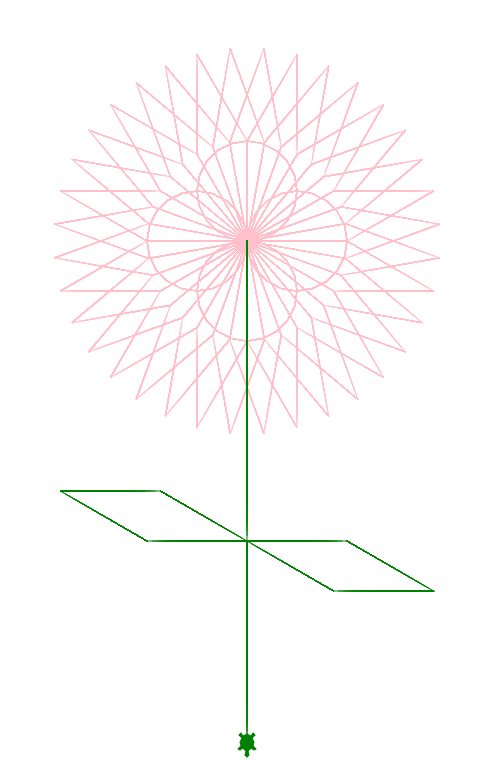