The scope of a variable is the region of the program in which you can directly access the variable:
if (true) {
let x = 123;
}
Here, the scope of x
is the then-block of this if-then-else statement.
A closure is a function plus the connection to the variables that existed at its birth place:
function funcFactory(value) {
return function () {
return value;
};
}
const func = funcFactory('abc');
func(); // 'abc'
func(); // 'abc'
funcFactory
returns a closure. Because the closure still has the connection to the variables at its birth place, it can still access the variable value
(even though it escaped its scope).
extending a little bit on that, the inner functions have access to their local variables and variables of all enclosing scopes (inner functions have broader access than outer functions). JS keeps track on which scope does a given variable come from (that's where shadowing kicks in). This is also reflected in devtools/debugger:
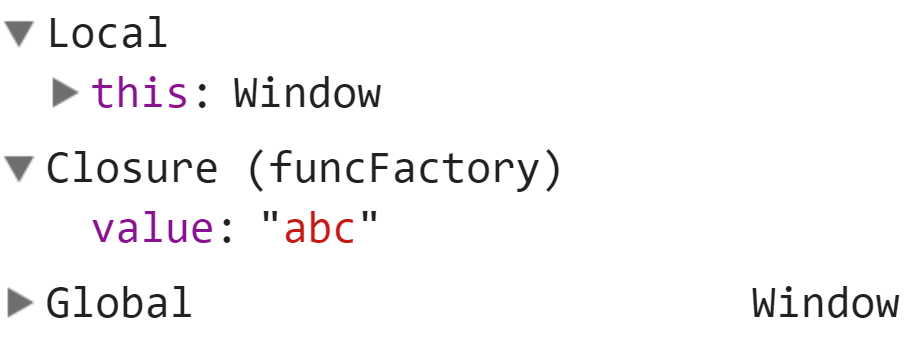
(the breakpoint is set at
return value;
line)