Created
March 1, 2019 16:23
-
-
Save rictorres/07e5e58a137b90546865367904d2c733 to your computer and use it in GitHub Desktop.
check deps ("success" exit code)
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
const npmCheck = require('npm-check') | |
const chalk = require('chalk') | |
const stripAnsi = require('strip-ansi') | |
const _flatten = require('lodash.flatten') | |
const _compact = require('lodash.compact') | |
const table = require('text-table') | |
const emoji = require('node-emoji') | |
function uppercaseFirstLetter(str) { | |
return str[0].toUpperCase() + str.substr(1) | |
} | |
function render(pkg, currentState) { | |
const packageName = pkg.moduleName | |
const rows = [] | |
const indent = ' ' + emoji.emojify(' ') | |
const flags = currentState.get('global') | |
? '--global' | |
: `--save${pkg.devDependency ? '-dev' : ''}` | |
const upgradeCommand = `npm install ${flags} ${packageName}@${pkg.latest}` | |
const upgradeMessage = `${chalk.green(upgradeCommand)} to go from ${pkg.installed} to ${ | |
pkg.latest | |
}` | |
// DYLAN: clean this up | |
const status = _compact( | |
_flatten([ | |
pkg.notInstalled | |
? chalk.bgRed.white.bold(emoji.emojify(' :worried: ') + ' MISSING! ') + | |
' Not installed.' | |
: '', | |
pkg.notInPackageJson | |
? chalk.bgRed.white.bold(emoji.emojify(' :worried: ') + ' PKG ERR! ') + | |
' Not in the package.json. ' + | |
pkg.notInPackageJson | |
: '', | |
pkg.pkgError && !pkg.notInstalled | |
? chalk.bgGreen.white.bold(emoji.emojify(' :worried: ') + ' PKG ERR! ') + | |
' ' + | |
chalk.red(pkg.pkgError.message) | |
: '', | |
pkg.bump && pkg.easyUpgrade | |
? [ | |
chalk.bgGreen.white.bold(emoji.emojify(' :heart_eyes: ') + ' UPDATE! ') + | |
' Your local install is out of date. ' + | |
chalk.blue.underline(pkg.homepage || ''), | |
indent + upgradeMessage, | |
] | |
: '', | |
pkg.bump && !pkg.easyUpgrade | |
? [ | |
chalk.white.bold.bgGreen( | |
pkg.bump === 'nonSemver' | |
? emoji.emojify(' :sunglasses: ') + ' new ver! '.toUpperCase() | |
: emoji.emojify(' :sunglasses: ') + | |
' ' + | |
pkg.bump.toUpperCase() + | |
' UP ' | |
) + | |
' ' + | |
uppercaseFirstLetter(pkg.bump) + | |
' update available. ' + | |
chalk.blue.underline(pkg.homepage || ''), | |
indent + upgradeMessage, | |
] | |
: '', | |
pkg.unused | |
? [ | |
chalk.black.bold.bgWhite(emoji.emojify(' :confused: ') + ' NOTUSED? ') + | |
` ${chalk.yellow(`Still using ${packageName}?`)}`, | |
indent + | |
`Depcheck did not find code similar to ${chalk.green( | |
`require('${packageName}')` | |
)} or ${chalk.green(`import from '${packageName}'`)}.`, | |
indent + | |
`Check your code before removing as depcheck isn't able to foresee all ways dependencies can be used.`, | |
indent + `Use ${chalk.green('--skip-unused')} to skip this check.`, | |
indent + | |
`To remove this package: ${chalk.green( | |
`npm uninstall --save${ | |
pkg.devDependency ? '-dev' : '' | |
} ${packageName}` | |
)}`, | |
] | |
: '', | |
pkg.mismatch && !pkg.bump | |
? chalk.bgRed.yellow.bold(emoji.emojify(' :interrobang: ') + ' MISMATCH ') + | |
' Installed version does not match package.json. ' + | |
pkg.installed + | |
' ≠ ' + | |
pkg.packageJson | |
: '', | |
pkg.regError | |
? chalk.bgRed.white.bold(emoji.emojify(' :no_entry: ') + ' NPM ERR! ') + | |
' ' + | |
chalk.red(pkg.regError) | |
: '', | |
]) | |
) | |
if (!status.length) { | |
return false | |
} | |
rows.push([chalk.yellow(packageName), status.shift()]) | |
while (status.length) { | |
rows.push([' ', status.shift()]) | |
} | |
rows.push([' ']) | |
return rows | |
} | |
function outputConsole(currentState) { | |
const packages = currentState.get('packages') | |
const rows = packages | |
.reduce((acc, pkg) => { | |
return acc.concat(render(pkg, currentState)) | |
}, []) | |
.filter(Boolean) | |
if (rows.length) { | |
const renderedTable = table(rows, { | |
stringLength: s => stripAnsi(s).length, | |
}) | |
console.log('') | |
console.log(renderedTable) | |
console.log( | |
`Use ${chalk.green( | |
`npm-check -${currentState.get('global') ? 'g' : ''}u` | |
)} for interactive update.` | |
) | |
process.exitCode = 1 | |
} else { | |
console.log( | |
`${emoji.emojify(':heart: ')}Your modules look ${chalk.bold( | |
'amazing' | |
)}. Keep up the great work.${emoji.emojify(' :heart:')}` | |
) | |
process.exitCode = 0 | |
} | |
} | |
npmCheck({ | |
skipUnused: true, | |
ignoreDev: true, | |
}).then(currentState => { | |
outputConsole(currentState) | |
process.exit(0) | |
}) |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
{ | |
"name": "i-will-update-my-deps-later", | |
"version": "1.0.0", | |
"description": "", | |
"private": true, | |
"license": "UNLICENSED", | |
"author": "New10", | |
"engines": { | |
"node": "8.x.x" | |
}, | |
"scripts": { | |
"check:dependencies": "node ./check-deps.js" | |
}, | |
"husky": { | |
"hooks": { | |
"pre-push": "npm run check:dependencies" | |
} | |
}, | |
"dependencies": {}, | |
"devDependencies": { | |
"chalk": "^2.4.2", | |
"husky": "^1.3.1", | |
"lodash.compact": "^3.0.1", | |
"lodash.flatten": "^4.4.0", | |
"node-emoji": "^1.10.0", | |
"npm-check": "^5.9.0", | |
"pre-push": "^0.1.1", | |
"strip-ansi": "^5.0.0" | |
} | |
} |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
before:
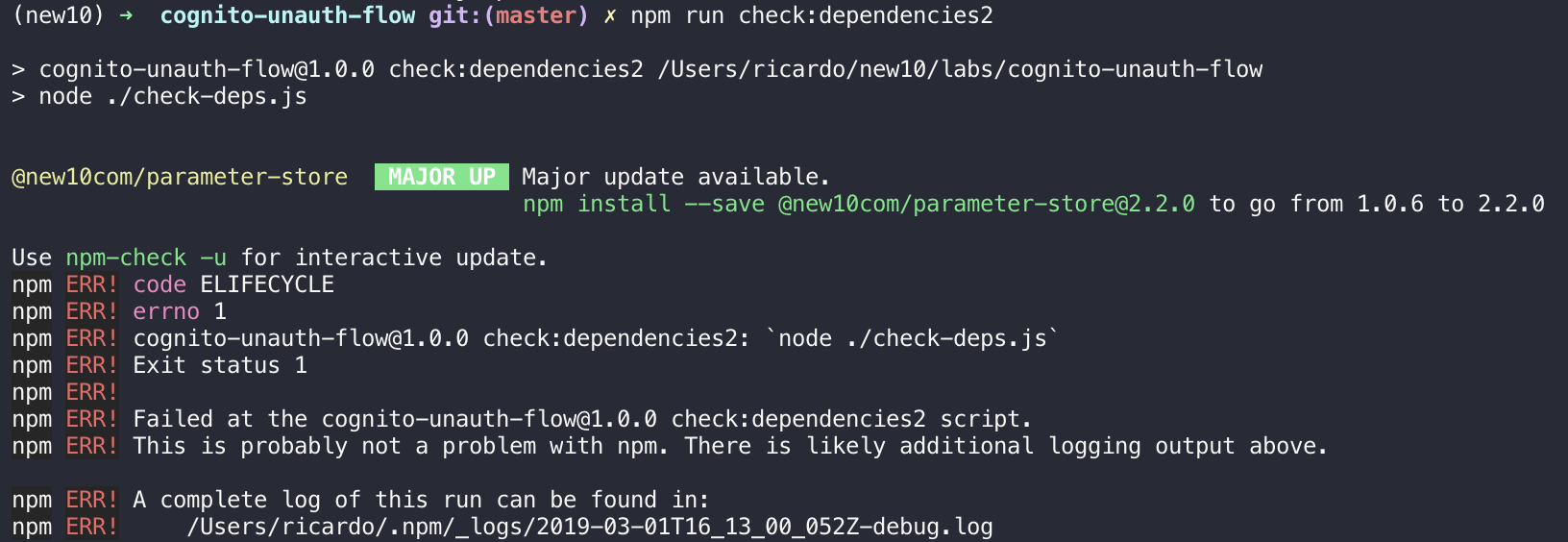
after (with this script):
