Created
December 19, 2022 09:40
-
-
Save rlevchenko/04b5efbc5f4923f65fcef703a4c01320 to your computer and use it in GitHub Desktop.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
## Blog Post: https://rlevchenko.com/2022/12/19/python-coding-fizzbuzz-challenge/ | |
import matplotlib.pyplot as plt | |
import colorama | |
from colorama import Fore, Back, Style | |
colorama.init() | |
def fizz_buzz(x,y): | |
"""Python version of popular Fizz Buzz task""" | |
fb = 0 ; b = 0; f = 0; rest = 0 # start values | |
fb_type = ['fizzbuzz','fizz','buzz','rest'] # plot labels | |
fb_colors = ['r','y','c','g'] # plot colors | |
fb_explode = [0.2, 0.1, 0.1, 0.1] # plot fraction of the radius | |
try: | |
for n in range(x,y): | |
if ((n % 3 == 0) and (n % 5 == 0)): | |
fb += 1 | |
print(Fore.RED + f"Found FizzBuzz: {n}") | |
elif n % 3 ==0: | |
f += 1 | |
print(Fore.WHITE + f"Found Fizz: {n}") | |
elif n % 5 ==0: | |
b += 1 | |
print(Fore.GREEN + f"Found Buzz: {n}") | |
else: | |
rest += 1 | |
print(Style.BRIGHT + f"The rest is {n}") | |
print(Style.RESET_ALL) | |
fb_array = [fb, f, b, rest] | |
plt.pie(fb_array, colors = fb_colors, explode = fb_explode, shadow = True, radius = 1.1, autopct = '%1.1f%%') # form a pie | |
plt.legend(fb_type,loc='upper right') # show legend | |
plt.show() # show a pie | |
except: | |
print(Style.BRIGHT + Fore.RED + "You provided wrong x and y") | |
print(Style.RESET_ALL) | |
finally: | |
print(Style.BRIGHT + Fore.GREEN + "Author: github.com/rlevchenko") | |
print(Style.RESET_ALL) |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Result:
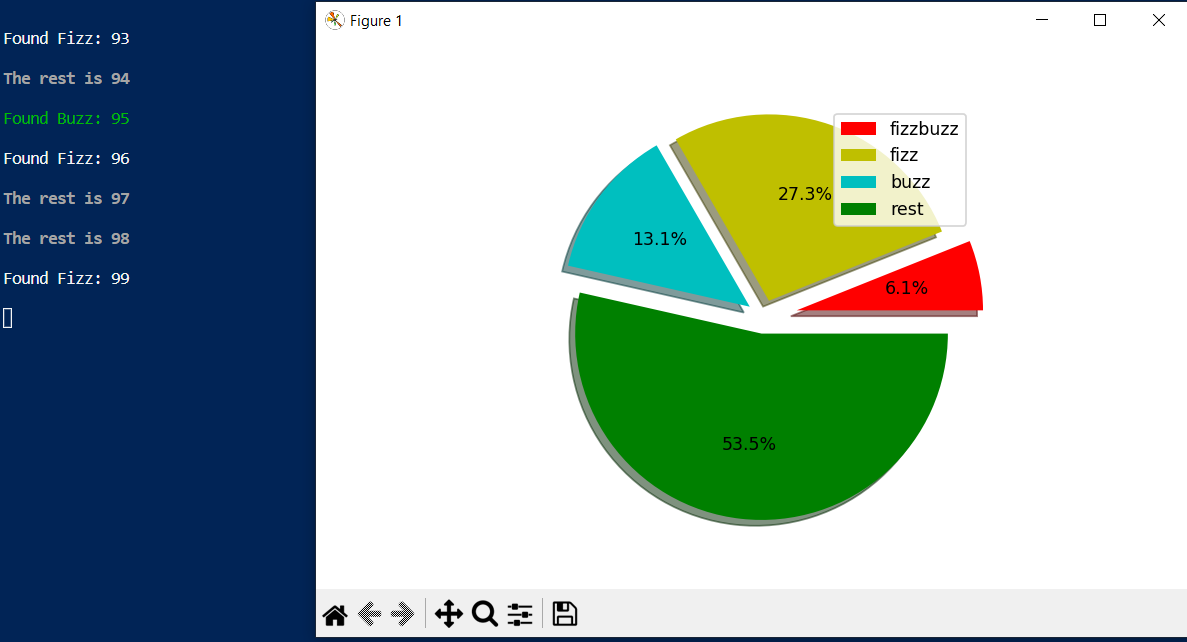