Last active
March 5, 2020 00:08
-
-
Save rngtm/5e20236cf20bfa6c45fb94664e49b6cb to your computer and use it in GitHub Desktop.
時間 <->フレーム数 の相互変換ツール
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
using UnityEngine; | |
using UnityEditor; | |
namespace Tools | |
{ | |
public class TimeFrameConverter : EditorWindow | |
{ | |
static private Color darkColor = new Color(216f , 222f , 226f, 255f) / 255f; | |
static private GUIStyle rowStyle = null; | |
static private GUIStyle valueBoxStyle = null; | |
[SerializeField] private float inputTime = 0f; | |
[SerializeField] private int inputFrameCount24 = 0; | |
[SerializeField] private int inputFrameCount30 = 0; | |
[SerializeField] private int inputFrameCount60 = 0; | |
static readonly private GUILayoutOption[] labelFieldLayoutOption = new GUILayoutOption[] | |
{ | |
GUILayout.Width(105f) | |
}; | |
static readonly private GUILayoutOption[] valueFieldLayoutOption = new GUILayoutOption[] | |
{ | |
GUILayout.Width(64f), | |
}; | |
/// <summary> | |
/// GUIStyle作成 | |
/// </summary> | |
private void CreateStylesIfNull() | |
{ | |
rowStyle = rowStyle ?? new GUIStyle(); | |
rowStyle.margin.left = 4; | |
rowStyle.margin.bottom = 2; | |
valueBoxStyle = valueBoxStyle ?? new GUIStyle(GUI.skin.box); | |
valueBoxStyle.margin = new RectOffset(0, 0, 0, 0); | |
} | |
/// <summary> | |
/// ウィンドウを開く | |
/// </summary> | |
[MenuItem("Tools/Time Frame Converter")] | |
static void Open() | |
{ | |
EditorWindow window = ScriptableObject.CreateInstance<TimeFrameConverter>(); | |
window.Show(); | |
} | |
private void OnGUI() | |
{ | |
CreateStylesIfNull(); | |
GUILayout.Space(3f); | |
using (new EditorGUILayout.HorizontalScope(rowStyle)) | |
{ | |
DrawInputTime(ref inputTime); | |
DrawTimeAsFrameCount(inputTime, 24); | |
DrawTimeAsFrameCount(inputTime, 60); | |
} | |
using (new EditorGUILayout.HorizontalScope(rowStyle)) | |
{ | |
DrawInputFrameCount(ref inputFrameCount24, 24); | |
DrawFrameCountAsTime(inputFrameCount24, 24); | |
} | |
using (new EditorGUILayout.HorizontalScope(rowStyle)) | |
{ | |
DrawInputFrameCount(ref inputFrameCount30, 30); | |
DrawFrameCountAsTime(inputFrameCount30, 30); | |
} | |
using (new EditorGUILayout.HorizontalScope(rowStyle)) | |
{ | |
DrawInputFrameCount(ref inputFrameCount60, 60); | |
DrawFrameCountAsTime(inputFrameCount60, 60); | |
} | |
} | |
/// <summary> | |
/// 秒数の入力フィールド | |
/// </summary> | |
static private void DrawInputTime(ref float time) | |
{ | |
//using (new EditorGUILayout.VerticalScope(valueBoxStyle, GUILayout.Width(240f))) | |
using (new EditorGUILayout.HorizontalScope(valueBoxStyle)) | |
{ | |
EditorGUILayout.LabelField("Time[sec]", labelFieldLayoutOption); | |
time = EditorGUILayout.FloatField(time, valueFieldLayoutOption); | |
} | |
} | |
/// <summary> | |
/// フレーム数の入力フィールド | |
/// </summary> | |
static private void DrawInputFrameCount(ref int frameCount, int frameRate) | |
{ | |
using (new EditorGUILayout.HorizontalScope(valueBoxStyle)) | |
{ | |
EditorGUILayout.LabelField($"Frames[{frameRate}fps]", labelFieldLayoutOption); | |
frameCount = EditorGUILayout.IntField(frameCount, valueFieldLayoutOption); | |
} | |
} | |
/// <summary> | |
/// 秒数をフレーム数換算で表示 | |
/// </summary> | |
static private void DrawTimeAsFrameCount(float time, int frameRate) | |
{ | |
var defaultColor = GUI.backgroundColor; | |
GUI.backgroundColor = darkColor; | |
using (new EditorGUILayout.HorizontalScope(valueBoxStyle)) | |
{ | |
EditorGUILayout.LabelField($"Frames[{frameRate}fps]", labelFieldLayoutOption); | |
EditorGUILayout.FloatField(time * frameRate, valueFieldLayoutOption); | |
} | |
GUI.backgroundColor = defaultColor; | |
} | |
/// <summary> | |
/// フレーム数を秒数換算表示 | |
/// </summary> | |
static private void DrawFrameCountAsTime(int frameCount, int frameRate) | |
{ | |
var defaultColor = GUI.backgroundColor; | |
GUI.backgroundColor = darkColor; | |
using (new EditorGUILayout.HorizontalScope(valueBoxStyle)) | |
{ | |
EditorGUILayout.LabelField($"Time[sec]", labelFieldLayoutOption); | |
// フレーム数を秒数へ変換して表示 | |
EditorGUILayout.FloatField((float)frameCount / frameRate, valueFieldLayoutOption); | |
} | |
GUI.backgroundColor = defaultColor; | |
} | |
} | |
} |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
画面上部から Tools -> Time Frame Converterを選択するとツールが起動します。
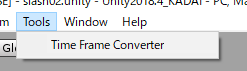