Created
February 6, 2021 15:02
-
-
Save robsonlira/bc649c1a7b7046509958b5b95d7a9a9f to your computer and use it in GitHub Desktop.
Usuário no Menu
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
<ion-app [class.dark-theme]="dark"> | |
<ion-split-pane contentId="main-content" when="md" | |
> | |
<ion-menu contentId="main-content" menuId="main-menu" | |
type="overlay" | |
> | |
<ion-content> | |
<ion-list id="inbox-list"> | |
<ion-list-header>Menu</ion-list-header> | |
<ion-note *ngIf="user">{{ user.nome }}</ion-note> | |
<ion-note *ngIf="!user">Usuário</ion-note> | |
<ion-menu-toggle | |
auto-hide="false" | |
*ngFor="let p of appPages; let i = index" | |
> |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
export class AppComponent implements OnInit { | |
user: User = null; | |
config: Config = null; | |
loggedIn = false; | |
dark = false; | |
initializeApp() { | |
this.platform.ready().then(() => { | |
this.statusBar.styleDefault(); | |
this.splashScreen.hide(); | |
this.config = this.storage.getLocalConfig(); | |
this.dark = this.config ? this.config.dark : false; | |
this.authService.authState$.subscribe((state) => { | |
if (!state) { | |
console.log('Ouvindo authState Não logado.'); | |
} else { | |
console.log('Ouvindo authState'); | |
this.loggedIn = true; | |
this.user = this.authService.getUser(); | |
} | |
}); | |
}); | |
this.platform.backButton.subscribeWithPriority(10, (processNextHandler) => { | |
console.log('Back press handler!'); | |
if (this.location.isCurrentPathEqualTo('/folder') || this.location.isCurrentPathEqualTo('/login') ) { | |
// Mostrar o Alerta de Saida! | |
this.showExitConfirm(); | |
processNextHandler(); | |
} else { | |
// Navegue para pagina anterior | |
this.location.back(); | |
} | |
}); | |
this.platform.backButton.subscribeWithPriority(5, () => { | |
console.log('Handler called to force close!'); | |
this.alertController.getTop().then((r) => { | |
if (r) { | |
navigator['app'].exitApp(); | |
} | |
}) | |
.catch((e) => { | |
console.log(e); | |
}); | |
}); | |
} | |
ngOnInit() { | |
} | |
// When Logout Button is pressed | |
logout() { | |
this.overlayService.alert({ | |
header: 'Questão', | |
message: 'Deseja sair de sua conta?', | |
buttons: [ | |
{ | |
text: 'Não', | |
role: 'cancel', | |
handler: () => { | |
console.log('Application exit prevented!'); | |
}, | |
}, | |
{ | |
text: 'Sair', | |
handler: async () => { | |
this.loggedIn = false; | |
this.user = null; | |
await this.authService.logout(); | |
this.navCtrl.navigateRoot('/login'); | |
}, | |
}, | |
], | |
}); | |
} | |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
import { Injectable } from '@angular/core'; | |
import { | |
ActivatedRouteSnapshot, | |
CanActivate, | |
CanActivateChild, | |
RouterStateSnapshot, | |
UrlTree, | |
Router, | |
Route, | |
UrlSegment, | |
} from '@angular/router'; | |
import { Observable } from 'rxjs'; | |
import { filter, map, take, tap } from 'rxjs/operators'; | |
import { AuthService } from '../services/auth.service'; | |
@Injectable({ | |
providedIn: 'root', | |
}) | |
export class AuthGuard implements CanActivate, CanActivateChild { | |
constructor( | |
private authService: AuthService, | |
private router: Router) {} | |
canActivate(next: ActivatedRouteSnapshot, state: RouterStateSnapshot): Observable<boolean> { | |
return this.checkAuthState(state.url); | |
} | |
canActivateChild(route: ActivatedRouteSnapshot, state: RouterStateSnapshot): Observable<boolean> { | |
return this.canActivate(route, state); | |
} | |
canLoad(route: Route, segments: UrlSegment[]): Observable<boolean> { | |
const url = segments.map((s) => `/${s}`).join(''); | |
return this.checkAuthState(url).pipe(take(1)); | |
} | |
canLoad1(route: Route, segments: UrlSegment[]): Observable<boolean> { | |
const url = segments.map((s) => `/${s}`).join(''); | |
return this.authService.authState$.pipe( | |
filter(val => val !== null), // Filter out initial Behaviour subject value | |
take(1), // Otherwise the Observable doesn't complete! | |
map( isAuthenticated =>{ | |
if (isAuthenticated){ | |
return true; | |
} else { | |
this.router.navigate(['/login'], { | |
queryParams: { url }, | |
}); | |
return false; | |
} | |
} ) | |
); | |
} | |
private checkAuthState(redirect: string): Observable<boolean> { | |
return this.authService.isAuthenticated.pipe( | |
tap((is) => { | |
if (!is) { | |
this.router.navigate(['/login'], { | |
queryParams: { redirect }, | |
}); | |
} | |
}) | |
); | |
} | |
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
import { environment } from './../../../environments/environment'; | |
import { Injectable } from '@angular/core'; | |
import { HttpClient, HttpParams, HttpHeaders } from '@angular/common/http'; | |
import { Observable, of, throwError, BehaviorSubject } from 'rxjs'; | |
import { StorageService } from './storage.service'; | |
import { User } from 'src/app/models/user'; | |
import { CredenciaisDTO } from 'src/app/models/credenciais.dto'; | |
import { API_CONFIG } from 'src/app/config/api.config'; | |
@Injectable({ | |
providedIn: 'root', | |
}) | |
export class AuthService { | |
authState$: BehaviorSubject<boolean> = new BehaviorSubject(null); | |
url: string; | |
constructor(private http: HttpClient, private storage: StorageService) { | |
this.montaUrl(); | |
this.checkToken(); | |
} | |
montaUrl() { | |
const localConf = this.storage.getLocalConfig(); | |
if (localConf && localConf.url) { | |
this.url = `${localConf.url}${API_CONFIG.regra}`; | |
} else { | |
this.url = `${environment.apiUrl}${API_CONFIG.regra}`; | |
} | |
} | |
checkToken() { | |
const user = this.storage.getLocalUser(); | |
if (user && user.token) { | |
this.authState$.next(true); | |
} | |
} | |
// Esta sendo utilizado no auth.guard | |
get isAuthenticated(): Observable<boolean> { | |
return this.authState$; | |
} | |
getUser(): User { | |
return this.storage.getLocalUser(); | |
} | |
authenticated(): boolean { | |
return !this.getUser() == null; | |
} | |
authenticate(creds: CredenciaisDTO): Observable<any> { | |
// Transforma objeto em String | |
const dados = JSON.stringify(creds); | |
const data = `token=${API_CONFIG.tokenDefault} | |
&metodo=${API_CONFIG.pathToAutenticate} | |
¶metros=${dados}`; | |
return this.post(data); | |
} | |
forgot(creds: CredenciaisDTO): Observable<any> { | |
// Transforma objeto em String | |
const dados = JSON.stringify(creds); | |
const data = `token=${API_CONFIG.tokenDefault} | |
&metodo=${API_CONFIG.pathToForgotLogin} | |
¶metros=${dados}`; | |
return this.post(data); | |
} | |
reset(creds: CredenciaisDTO): Observable<any> { | |
// Transforma objeto em String | |
const dados = JSON.stringify(creds); | |
const data = `token=${API_CONFIG.tokenDefault} | |
&metodo=${API_CONFIG.pathToResetPassword} | |
¶metros=${dados}`; | |
return this.post(data); | |
} | |
post(dados: any): Observable<any> { | |
const options = { | |
headers: new HttpHeaders({ | |
'Content-Type': 'application/x-www-form-urlencoded', | |
}), | |
}; | |
//debugger; | |
return this.http.post(this.url, dados, options); | |
} | |
successLogin(ctoken: string, cnome: string, cemail: string) { | |
const user: User = { | |
nome: cnome, | |
email: cemail, | |
adm: '', | |
tipoExpiracao: '', | |
token: ctoken, | |
cliente: '', | |
}; | |
this.authState$.next(true); | |
this.storage.setLocalUser(user); | |
} | |
logout(): Promise<void> { | |
this.authState$.next(false); | |
this.storage.setLocalUser(null); | |
return null; | |
} | |
} |
Correção do problema no auth.service
this.authState$.next(true);
this.storage.setLocalUser(user);
só inverter a ordem ou seja primeiro o storage para armazenar o usuário
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
O menu deve apresentar o nome do usuário se ele estiver logado, ocorre que após o login o nome não é mostrado, se eu der um refresh na pagina ai sim ele aparece.
Quando eu implementei isto eu coloquei um (atributo ou propriedade ) authState$: BehaviorSubject no serviço de autenticação
e no app.component eu coloquei um ouvinte this.authService.authState$.subscribe((state) =>
o que pode estar errado segue imagem logo apos o login, notem que não mostra o nome do usuário?
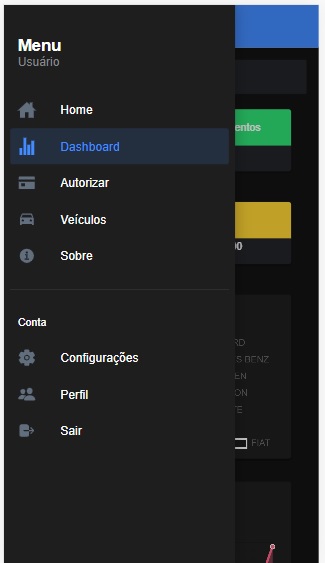
quando dou um refresh ou quando saio do app sem me deslogar e volto o nome aparece conforme esta nesta outra imagem
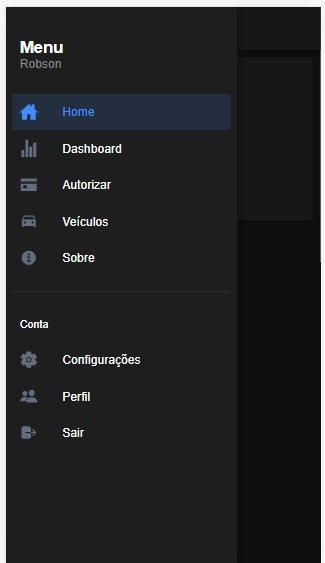