Created
August 12, 2020 15:37
-
-
Save rodydavis/4f4b631f9883d73e2f875e54dae7f366 to your computer and use it in GitHub Desktop.
Flutter Slider Limit Min/Max
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
// Flutter code sample for Slider | |
// 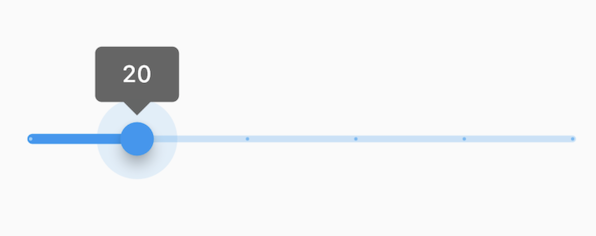 | |
// | |
// The Sliders value is part of the Stateful widget subclass to change the value | |
// setState was called. | |
// Docs: https://api.flutter.dev/flutter/material/Slider-class.html | |
import 'package:flutter/material.dart'; | |
void main() => runApp(MyApp()); | |
/// This Widget is the main application widget. | |
class MyApp extends StatelessWidget { | |
static const String _title = 'Flutter Code Sample'; | |
@override | |
Widget build(BuildContext context) { | |
return MaterialApp( | |
title: _title, | |
home: Scaffold( | |
appBar: AppBar(title: const Text(_title)), | |
body: MyStatefulWidget(), | |
), | |
); | |
} | |
} | |
class MyStatefulWidget extends StatefulWidget { | |
MyStatefulWidget({Key key}) : super(key: key); | |
@override | |
_MyStatefulWidgetState createState() => _MyStatefulWidgetState(); | |
} | |
class _MyStatefulWidgetState extends State<MyStatefulWidget> { | |
double _currentSliderValue = 20; | |
@override | |
Widget build(BuildContext context) { | |
return Slider( | |
value: _currentSliderValue, | |
min: 10, | |
max: 90, | |
label: _currentSliderValue.round().toString(), | |
onChanged: (double value) { | |
setState(() { | |
_currentSliderValue = value; | |
}); | |
}, | |
); | |
} | |
} |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment