Last active
November 15, 2017 13:24
-
-
Save ryohlan/95042f0cc4799b8759334addb390a0dd to your computer and use it in GitHub Desktop.
MaterialTextInput
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
// @flow | |
import React from 'react'; | |
import { View, TextInput, Animated } from 'react-native'; | |
type Props = { | |
placeholder: string, | |
focusColor: string, | |
unfocusColor: string, | |
secureTextEntry?: boolean, | |
textColor: string, | |
fontSize: number, | |
} | |
type State = { | |
animated: any, | |
text: string, | |
formHeight: number, | |
} | |
class MaterialTextInput extends React.Component<void, Props, State> { | |
state: State = { | |
animated: new Animated.Value(0), | |
text: '', | |
formHeight: 0, | |
} | |
startFocusInAnimation() { | |
Animated.timing(this.state.animated, { | |
toValue: 1, | |
duration: 200, | |
}).start(); | |
} | |
startFocusOutAnimation() { | |
Animated.timing(this.state.animated, { | |
toValue: 0, | |
duration: 200, | |
}).start(); | |
} | |
render() { | |
const existText = !!this.state.text; | |
return ( | |
<View style={{ paddingVertical: 8 }}> | |
<View style={{ height: 20 }} /> | |
<View style={{ justifyContent: 'center' }}> | |
<Animated.Text | |
style={{ | |
position: 'absolute', | |
left: 0, | |
lineHeight: this.props.fontSize + 4, | |
margin: 0, | |
bottom: this.state.animated.interpolate({ inputRange: [0, 1], outputRange: [existText ? this.state.formHeight : 8, this.state.formHeight] }), | |
color: this.state.animated.interpolate({ inputRange: [0, 1], outputRange: [this.props.unfocusColor, this.props.focusColor] }), | |
backgroundColor: '#00000000', | |
fontSize: this.state.animated.interpolate({ inputRange: [0, 1], outputRange: [existText ? 12 : this.props.fontSize, 12] }), | |
}} | |
>{this.props.placeholder}</Animated.Text> | |
<TextInput | |
onLayout={({ nativeEvent: { layout: { height } } }) => this.setState({ formHeight: height })} | |
onChangeText={text => this.setState({ text })} | |
onEndEditing={() => this.startFocusOutAnimation()} | |
onFocus={() => this.startFocusInAnimation()} | |
underlineColorAndroid="#00000000" | |
selectionColor={this.props.focusColor} | |
secureTextEntry={this.props.secureTextEntry} | |
style={{ | |
backgroundColor: '#00000000', | |
paddingTop: 8, | |
paddingBottom: 6, | |
paddingHorizontal: 0, | |
width: '100%', | |
fontSize: this.props.fontSize, | |
color: this.props.textColor, | |
}} | |
/> | |
</View> | |
<Animated.View | |
style={{ | |
height: 2, | |
backgroundColor: this.state.animated.interpolate({ inputRange: [0, 1], outputRange: [this.props.unfocusColor, this.props.focusColor] }), | |
width: '100%', | |
opacity: this.state.animated.interpolate({ inputRange: [0, 1], outputRange: [0.7, 1] }), | |
transform: [ | |
{ scaleY: this.state.animated.interpolate({ inputRange: [0, 1], outputRange: [0.6, 1] }) }, | |
], | |
}} | |
/> | |
</View > | |
); | |
} | |
} | |
export default MaterialTextInput; |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
iOS
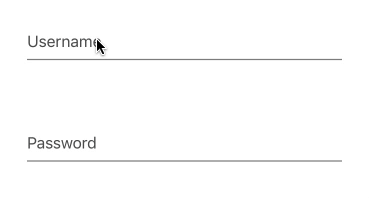
Android
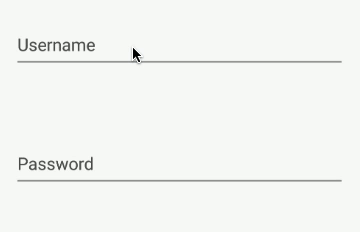