Last active
May 25, 2023 10:15
-
-
Save samuelkarani/19daede737e66121592f3da0937737a4 to your computer and use it in GitHub Desktop.
Simple React "cache" api function. No clearing strategies e.g. LRU etc. Just simple interval clearing. Official version is currently available ONLY in react canaries and RSC environment i.e. “react-server” exports condition. Depends on object-hash package to create hash key from arguments and optional additional key.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
import objectHash from "object-hash"; | |
const cacheTimeout = 60 * 60 * 1000 | |
let store: Record<string, unknown> = {}; | |
export function cache<A extends unknown[], B = unknown>( | |
fn: (...args: A) => Promise<B>, | |
key?: string // optional key for guaranteed uniqueness | |
) { | |
return async (...args: A) => { | |
const hash = objectHash([...args, key]); | |
if (hash in store) { | |
return store[hash] as B; | |
} | |
const response = await fn(...args); | |
store[hash] = response; | |
return response; | |
}; | |
} | |
export function clear() { | |
store = {}; | |
} | |
setTimeout(clear, cacheTimeout); | |
// usage | |
async function fetchData() { | |
const response = await fetch("http://example.com/movies.json"); | |
const data = await response.json(); | |
return data | |
} | |
cache(fetchData, "movies"); // normal request | |
cache(fetchData, "movies"); // no request - uses cache |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
I asked Dan Abramov (official React spokesman) about the
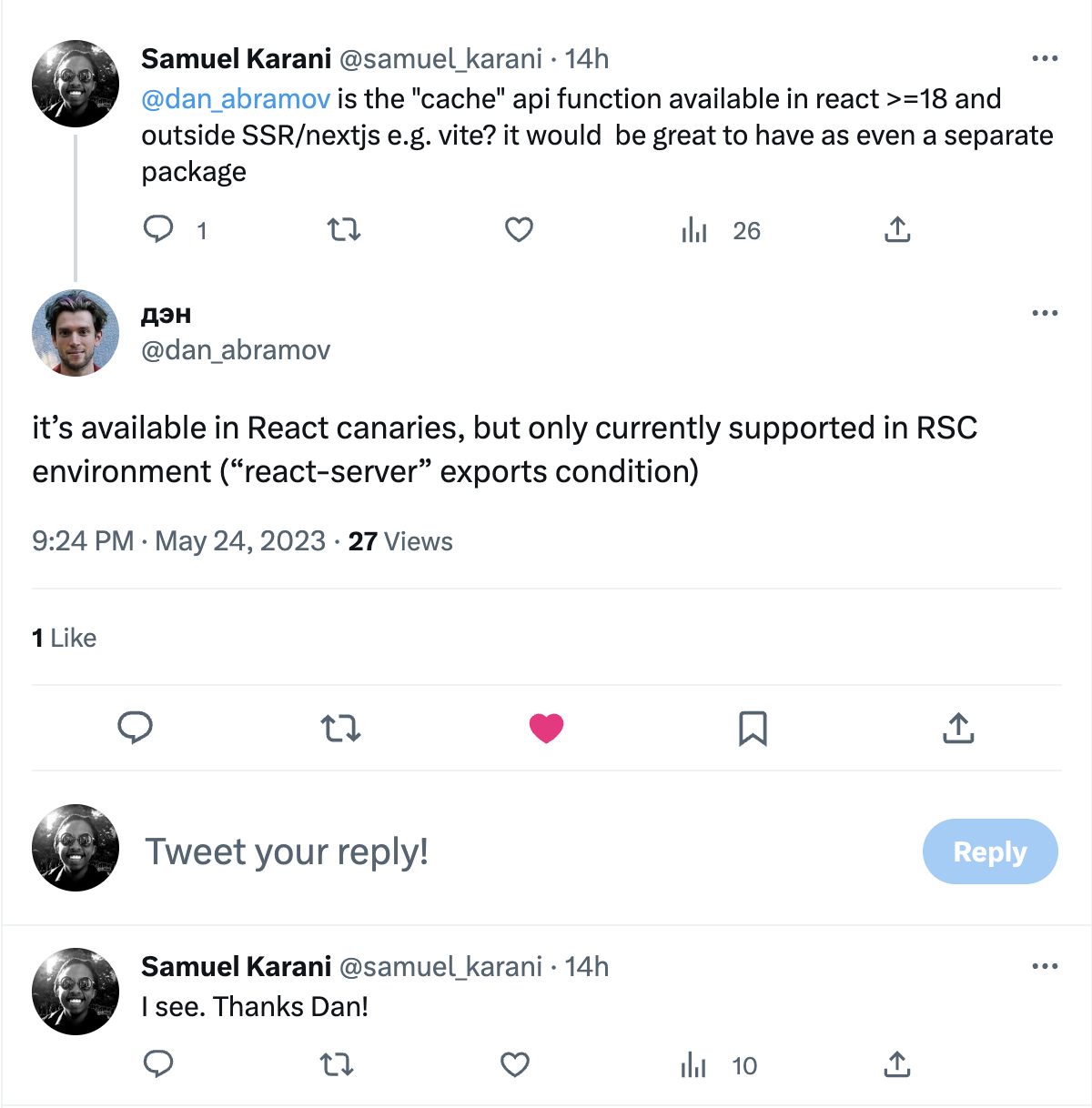
cache
function availability and created this snippet for anyone's benefit.