Last active
February 22, 2022 00:38
-
-
Save santolucito/44410ed78def1b68b9994b74227f59ee to your computer and use it in GitHub Desktop.
ESP32 capacitive touch+ python
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
# this code will read the touch values from the ESP32 and echo them on the command line | |
# you could do something else more interesting with these values (e.g. visualize/sonify) | |
# pip install pyserial | |
import serial | |
# change the port as necessary by your OS | |
ser = serial.Serial('/dev/ttyS8', 115200) | |
while(True): | |
print(str(ser.readline().strip(), 'ascii')) |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
/* | |
This is an example how to use Touch Intrrerupts and read touch values | |
just touch the pins themselves to get different values | |
*/ | |
int threshold = 40; | |
bool touch1detected = false; | |
bool touch2detected = false; | |
uint8_t touch1Val = 0; | |
uint8_t touch2Val = 0; | |
void gotTouch1(){ | |
touch1detected = true; | |
touch1Val = touchRead(T2); | |
} | |
void gotTouch2(){ | |
touch2detected = true; | |
touch2Val = touchRead(T9); | |
} | |
void setup() { | |
Serial.begin(115200); | |
delay(1000); // give me time to bring up serial monitor | |
Serial.println("ESP32 Touch Interrupt Test"); | |
touchAttachInterrupt(T2, gotTouch1, threshold); | |
touchAttachInterrupt(T9, gotTouch2, threshold); | |
} | |
void loop(){ | |
if(touch1detected){ | |
touch1detected = false; | |
Serial.println(touch1Val); | |
} | |
if(touch2detected){ | |
touch2detected = false; | |
Serial.println(touch2Val); | |
} | |
} |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
pinout image for location of touch sensors on TTGO T-Display from https://raw.githubusercontent.com/Xinyuan-LilyGO/TTGO-T-Display/master/image/pinmap.jpg
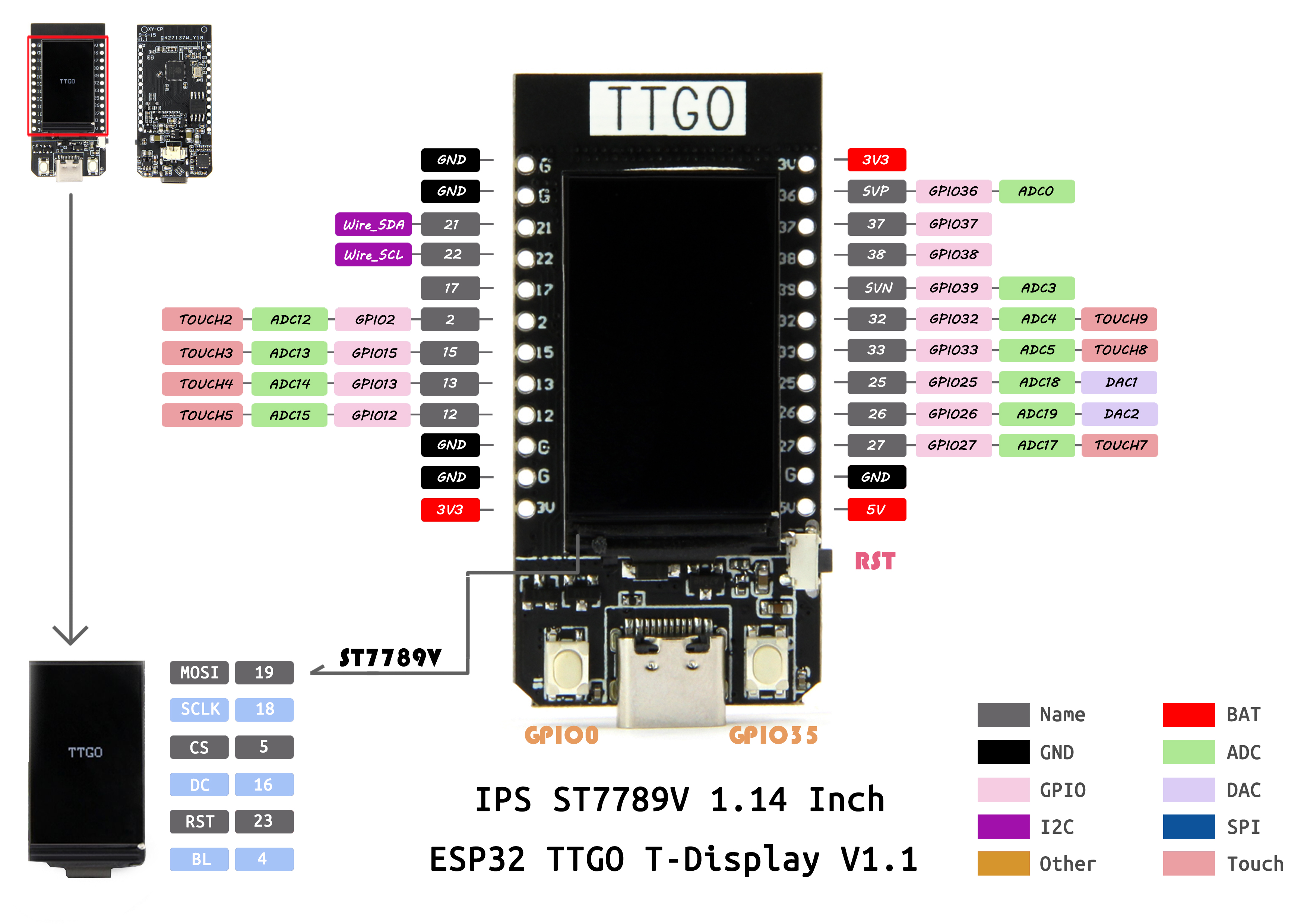
s