Created
October 6, 2019 16:53
-
-
Save schreibfaul1/a5caac2ba71f2cc1bb788ac047a01398 to your computer and use it in GitHub Desktop.
ESP32 AD9850 (DDS Signal Generator)
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
#include "Arduino.h" | |
#include "SPI.h" | |
// Digital I/O used | |
#define AD9850_CS 21 | |
#define AD9850_RST 22 | |
// Global variables | |
uint32_t AD9850_CLOCK=125000000; // Module crystal frequency. Tweak here for accuracy. | |
uint32_t sweep=0; | |
uint32_t sweep_max=2000; | |
uint32_t sweep_min=1000; | |
boolean sweep_direction=false; | |
// Objects | |
SPISettings SPI_AD9850; | |
void AD9850_set_frequency(uint32_t freq){ | |
freq=freq * 4294967295/AD9850_CLOCK; | |
digitalWrite(AD9850_CS, LOW); | |
SPI.beginTransaction(SPI_AD9850); | |
SPI.write32(freq); | |
SPI.write(0); | |
SPI.endTransaction(); | |
digitalWrite(AD9850_CS, HIGH); | |
} | |
void AD9850_reset(){ | |
// RESET AD9850 | |
digitalWrite(AD9850_RST, HIGH); | |
delay(1); | |
digitalWrite(AD9850_RST, LOW); | |
delay(1); | |
AD9850_set_frequency(0); | |
delay(1); | |
} | |
//The setup function is called once at startup of the sketch | |
void setup(){ | |
pinMode(AD9850_CS, OUTPUT); digitalWrite(AD9850_CS, HIGH); | |
pinMode(AD9850_RST, OUTPUT); digitalWrite(AD9850_RST, LOW); | |
SPI.begin(); | |
SPI_AD9850=SPISettings(1000000, LSBFIRST, SPI_MODE0); | |
AD9850_reset(); | |
AD9850_set_frequency(10000); | |
delay(1000); | |
sweep=sweep_min; | |
sweep_direction=true; | |
} | |
// The loop function is called in an endless loop | |
void loop(){ | |
if(sweep==sweep_min) sweep_direction=true; | |
if(sweep==sweep_max) sweep_direction=false; | |
if(sweep_direction==true) sweep++; else sweep--; | |
AD9850_set_frequency(sweep); | |
delay(2); | |
} |
Hi Thanks for your post. Could you tell us how you connect pins from AD9850 to ESP? Thank you
You need:
GPIO21 CS -> FQ_UD
GPIO22 RST -> RESET
GPIO23 MOSI -> Serial Data
GPIO 18 CLK -> W_CLK
Hi,
Thanks for your reply. It's working well now. Thank you.
Best,
Changrong
Sent from Outlook for iOS<https://aka.ms/o0ukef>
…________________________________
From: Wolle ***@***.***>
Sent: Tuesday, December 12, 2023 3:09:19 PM
To: schreibfaul1 ***@***.***>
Cc: Comment ***@***.***>; Manual ***@***.***>
Subject: Re: schreibfaul1/DDS_AD9850.ino
⚠ External sender. Take care when opening links or attachments. Do not provide your login details.
@schreibfaul1 commented on this gist.
________________________________
You need:
GPIO21 CS -> FQ_UD
GPIO22 RST -> RESET
GPIO23 MOSI -> Serial Data
GPIO 18 CLK -> W_CLK
—
Reply to this email directly, view it on GitHub<https://gist.github.com/schreibfaul1/a5caac2ba71f2cc1bb788ac047a01398#gistcomment-4790535> or unsubscribe<https://github.com/notifications/unsubscribe-auth/A5AJXPWPKSF7QK3G24JM4XLYJBXR7BFKMF2HI4TJMJ2XIZLTSKBKK5TBNR2WLJDHNFZXJJDOMFWWLK3UNBZGKYLEL52HS4DFQKSXMYLMOVS2I5DSOVS2I3TBNVS3W5DIOJSWCZC7OBQXE5DJMNUXAYLOORPWCY3UNF3GS5DZVRZXKYTKMVRXIX3UPFYGLK2HNFZXIQ3PNVWWK3TUUZ2G64DJMNZZDAVEOR4XAZNEM5UXG5FFOZQWY5LFVA4TQNZSHA4DONNHORZGSZ3HMVZKMY3SMVQXIZI>.
You are receiving this email because you commented on the thread.
Triage notifications on the go with GitHub Mobile for iOS<https://apps.apple.com/app/apple-store/id1477376905?ct=notification-email&mt=8&pt=524675> or Android<https://play.google.com/store/apps/details?id=com.github.android&referrer=utm_campaign%3Dnotification-email%26utm_medium%3Demail%26utm_source%3Dgithub>.
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
AD9850 Breadbord
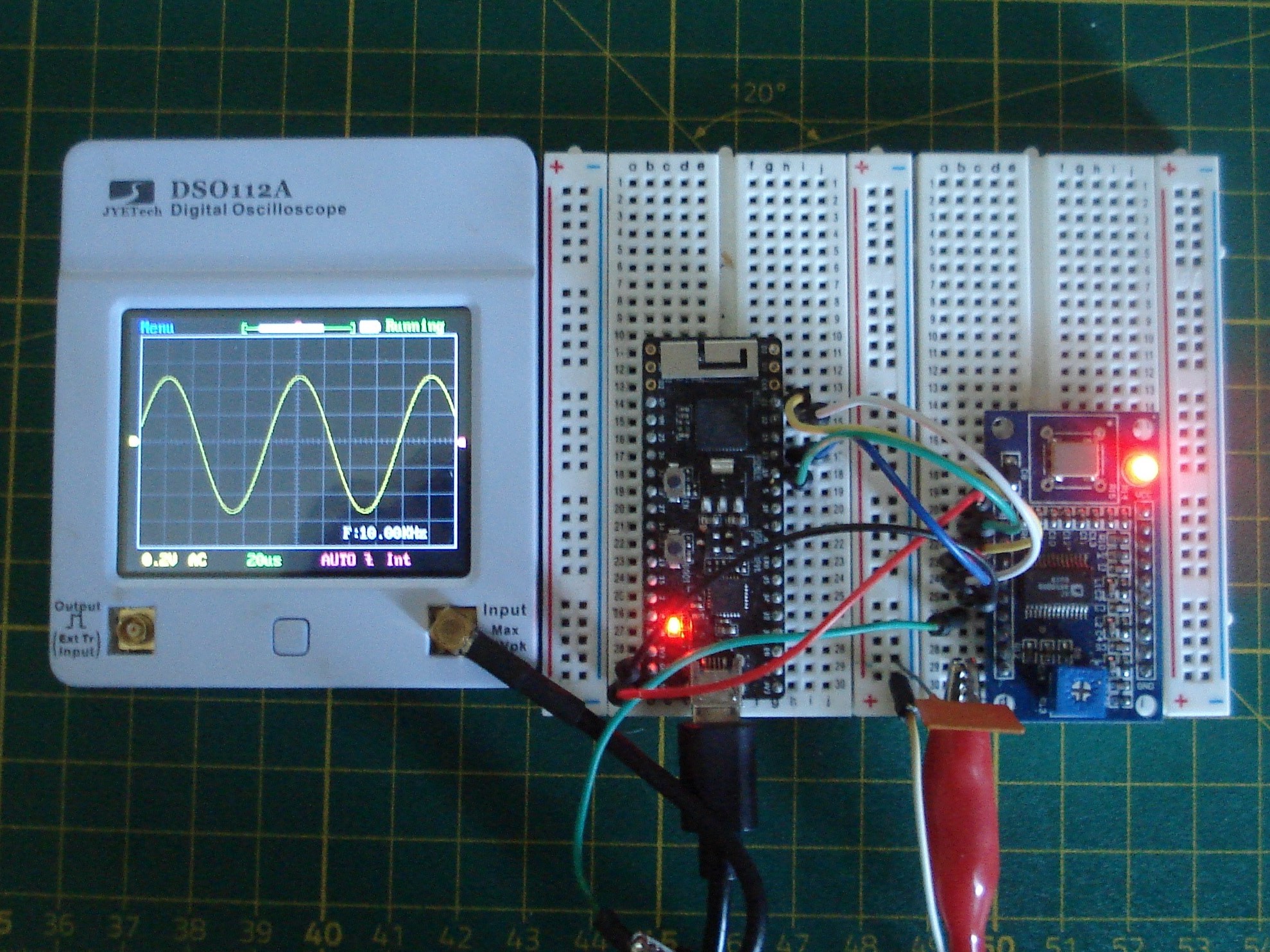