made with requirebin
Last active
December 20, 2015 07:52
-
-
Save serapath/003ebd0d11a7f248c210 to your computer and use it in GitHub Desktop.
Virtual Level Tracker
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
/******************************************************************** | |
COMPONENT - ./MyThunk.js | |
********************************************************************/ | |
// module.exports = MyThunk | |
function Hook (db, component, tracks, TEMP) { | |
this.db = db | |
this.component = component | |
this.tracks = tracks | |
this.TEMP = TEMP | |
this.untrack = [] | |
} | |
Hook.prototype.unhook = function() { | |
logger(null, ':::: unhook() -> untrack()') | |
this.untrack.forEach(function (untrack) { | |
untrack() | |
}) | |
} | |
Hook.prototype.hook = function (node) { | |
var THIS = this | |
var DATA = THIS.TEMP | |
var initialize = true | |
logger(null, ':::: hook() -> track()') | |
var t = THIS.db.trackable() | |
THIS.tracks.forEach(function (keyrange) { | |
THIS.untrack.push(t.track(keyrange)) | |
}) | |
t.on('data', function ondata (data) { | |
// @TODO: debug slowness while open devtools | |
// https://github.com/serapath/level-tracker/blob/master/index.js | |
var key = data.key | |
var val = data.value | |
if (DATA[key] !== val) { | |
logger(null, ':::: .on("data") -> update()') | |
logger(null, '<b>OLD:</b> ' + JSON.stringify(data)) | |
logger(null, '<b>PATCH WITH:</b> {'+key+': "'+val+'" }') | |
DATA[key] = val | |
logger(null, '<b>NEW:</b> ' + JSON.stringify(data)) | |
var oldTree = THIS.vtree | |
var newTree = THIS.component(DATA) | |
var patches = diff(oldTree, newTree) | |
patch(node, patches) | |
} else if (initialize) { | |
logger(null, ':::: initialize()') | |
logger(null, '<b>DATA:</b> ' + JSON.stringify(DATA)) | |
var oldTree = THIS.vtree | |
var newTree = THIS.component(DATA) | |
var patches = diff(oldTree, newTree) | |
patch(node, patches) | |
initialize = false | |
} | |
}) | |
} | |
function MyThunk (params) { | |
if (!(this instanceof MyThunk)) { return new MyThunk(params) } | |
var db = params.db | |
var render = params.render | |
var tracks = params.tracks | |
if (!db||!render||!tracks) { throw new Error('missing params') } | |
var TEMP = {} | |
var hook = new Hook(db, init, Object.keys(tracks).map( | |
function (key) { | |
db.get(key, function (error, data) { | |
if (error) { | |
db.put(key, tracks[key], function handle (error) { | |
if (error) { throw error } | |
}) | |
TEMP[key] = tracks[key] | |
} else { | |
TEMP[key] = data | |
} | |
}) | |
return {gte:key,lte:key,suffix:'',terminator:'!',old:true} | |
} | |
), TEMP) | |
function init (DATA) { return h('div',{'ev-hook':hook},render(DATA)) } | |
this.init = init | |
this.hook = hook | |
} | |
MyThunk.prototype.type = 'Thunk' | |
MyThunk.prototype.render = function render (Pre) { | |
// Pre: VNode | Thunk | Widget | VText | |
// (= the Thunk is being diffed against) | |
// When "Pre.render" was called by "diff", | |
// it will do caching through: | |
// => Pre.vnode = Pre.render(previousPre) | |
var useCache = Pre && Pre.vnode | |
if (useCache) { logger(null, ':::: render cached thunk') } // @TODO: remove | |
return useCache ? Pre.vnode : this.hook.vtree = this.init({}) | |
} | |
/******************************************************************** | |
MAIN | |
********************************************************************/ | |
function apilogger (opts) { console.log('API LOGGING: ', opts) } | |
var diff = require('virtual-dom').diff | |
var patch = require('virtual-dom').patch | |
var createElement = require('virtual-dom').create | |
var h = require('virtual-dom').h | |
var process = require('process') | |
var stream = require('stream-browserify') | |
var level = require('memdb') | |
var tracker = require('level-tracker') | |
var db = tracker(level("data.db"), apilogger) | |
var myComponent = MyThunk // require('./MyThunk.js') | |
var TEMP = { name: '', subname:'', info:'' } | |
document.querySelector('#content').appendChild(createElement( | |
h('div', [ | |
h('h1', "CONTROLS - Change Component Data"), | |
h('input', {className:'name',onchange:store}), | |
h('button', {className:'name',onclick:update}, 'name'), | |
h('br'), | |
h('input', {className:'subname',onchange:store}), | |
h('button', {className:'subname',onclick:update}, 'subname'), | |
h('br'), | |
h('input', {className:'info',onchange:store}), | |
h('button', {className:'info',onclick:update}, 'info'), | |
h('br'), | |
h('hr'), | |
h('h2', "COMPONENT - See rendered demo component"), | |
h('div', { style: {border: '3px solid red', padding: '20px' }}, [ | |
myComponent({ | |
db: db, | |
tracks: { | |
name: 'John Doe', | |
subname: 'alleycat', | |
info: 'The random dude' | |
}, | |
render: function render (DATA) { | |
return h('div', [ | |
h('h1', 'Name: ' + DATA['name']), | |
h('h2', 'Subname: ' + DATA['subname']), | |
h('h3', 'Info: ' + DATA['info']) | |
]) | |
} | |
}) | |
]), | |
h('hr'), | |
h('h2', "LOGGING - logging data changes + dump database"), | |
h('button', {onclick:dump}, 'dump memdb 2 console'), | |
h('button', {onclick:clear}, 'clear console') | |
]) | |
)) | |
function update (ev) { | |
var key = ev.target.className | |
db.put(key, TEMP[key], function handle (error) { | |
if (error) { throw error } | |
}) | |
} | |
function store (ev) { | |
var e = ev.target | |
TEMP[e.className] = e.value | |
} | |
/******************************************************************** | |
LOGGING | |
********************************************************************/ | |
var konsole | |
function logger (err, data) { | |
if (!konsole) { konsole = getKonsole() } | |
var x = document.createElement('div') | |
x.className = 'line' | |
x.style.fontFamily = 'Arial' | |
x.style.paddingTop = '10px' | |
x.innerHTML = data | |
konsole.appendChild(x) | |
} | |
function dump () { | |
logger(null, ':::: Dumping memdb content') | |
db.get('name', logger) | |
db.get('subname', logger) | |
db.get('info', logger) | |
} | |
function clear () { | |
var lines = [].slice.call(document.querySelectorAll('.line')) | |
lines.forEach(function (line) { | |
line.parentNode.removeChild(line) | |
}) | |
} | |
function getKonsole () { | |
var style = document.createElement('style') | |
style.innerHTML = [ | |
".konsole{", | |
"background-color: black;", | |
"padding: 5px;", | |
"box-sizing: border-box;", | |
"color: white;", | |
"position: absolute;", | |
"width:100%;", | |
"height:30vh;", | |
"display: flex;", | |
"overflow: scroll;", | |
"flex-direction:column;", | |
"}" | |
].join('') | |
document.body.appendChild(style) | |
var konsole = document.createElement('div') | |
konsole.className = 'konsole' | |
document.body.appendChild(konsole) | |
return konsole | |
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
require=function e(t,n,r){function s(o,u){if(!n[o]){if(!t[o]){var a=typeof require=="function"&&require;if(!u&&a)return a(o,!0);if(i)return i(o,!0);var f=new Error("Cannot find module '"+o+"'");throw f.code="MODULE_NOT_FOUND",f}var l=n[o]={exports:{}};t[o][0].call(l.exports,function(e){var n=t[o][1][e];return s(n?n:e)},l,l.exports,e,t,n,r)}return n[o].exports}var i=typeof require=="function"&&require;for(var o=0;o<r.length;o++)s(r[o]);return s}({1:[function(require,module,exports){},{}],2:[function(require,module,exports){var createElement=require("./vdom/create-element.js");module.exports=createElement},{"./vdom/create-element.js":14}],3:[function(require,module,exports){var diff=require("./vtree/diff.js");module.exports=diff},{"./vtree/diff.js":34}],4:[function(require,module,exports){var h=require("./virtual-hyperscript/index.js");module.exports=h},{"./virtual-hyperscript/index.js":21}],5:[function(require,module,exports){module.exports=function split(undef){var nativeSplit=String.prototype.split,compliantExecNpcg=/()??/.exec("")[1]===undef,self;self=function(str,separator,limit){if(Object.prototype.toString.call(separator)!=="[object RegExp]"){return nativeSplit.call(str,separator,limit)}var output=[],flags=(separator.ignoreCase?"i":"")+(separator.multiline?"m":"")+(separator.extended?"x":"")+(separator.sticky?"y":""),lastLastIndex=0,separator=new RegExp(separator.source,flags+"g"),separator2,match,lastIndex,lastLength;str+="";if(!compliantExecNpcg){separator2=new RegExp("^"+separator.source+"$(?!\\s)",flags)}limit=limit===undef?-1>>>0:limit>>>0;while(match=separator.exec(str)){lastIndex=match.index+match[0].length;if(lastIndex>lastLastIndex){output.push(str.slice(lastLastIndex,match.index));if(!compliantExecNpcg&&match.length>1){match[0].replace(separator2,function(){for(var i=1;i<arguments.length-2;i++){if(arguments[i]===undef){match[i]=undef}}})}if(match.length>1&&match.index<str.length){Array.prototype.push.apply(output,match.slice(1))}lastLength=match[0].length;lastLastIndex=lastIndex;if(output.length>=limit){break}}if(separator.lastIndex===match.index){separator.lastIndex++}}if(lastLastIndex===str.length){if(lastLength||!separator.test("")){output.push("")}}else{output.push(str.slice(lastLastIndex))}return output.length>limit?output.slice(0,limit):output};return self}()},{}],6:[function(require,module,exports){"use strict";var OneVersionConstraint=require("individual/one-version");var MY_VERSION="7";OneVersionConstraint("ev-store",MY_VERSION);var hashKey="__EV_STORE_KEY@"+MY_VERSION;module.exports=EvStore;function EvStore(elem){var hash=elem[hashKey];if(!hash){hash=elem[hashKey]={}}return hash}},{"individual/one-version":8}],7:[function(require,module,exports){(function(global){"use strict";var root=typeof window!=="undefined"?window:typeof global!=="undefined"?global:{};module.exports=Individual;function Individual(key,value){if(key in root){return root[key]}root[key]=value;return value}}).call(this,typeof global!=="undefined"?global:typeof self!=="undefined"?self:typeof window!=="undefined"?window:{})},{}],8:[function(require,module,exports){"use strict";var Individual=require("./index.js");module.exports=OneVersion;function OneVersion(moduleName,version,defaultValue){var key="__INDIVIDUAL_ONE_VERSION_"+moduleName;var enforceKey=key+"_ENFORCE_SINGLETON";var versionValue=Individual(enforceKey,version);if(versionValue!==version){throw new Error("Can only have one copy of "+moduleName+".\n"+"You already have version "+versionValue+" installed.\n"+"This means you cannot install version "+version)}return Individual(key,defaultValue)}},{"./index.js":7}],9:[function(require,module,exports){(function(global){var topLevel=typeof global!=="undefined"?global:typeof window!=="undefined"?window:{};var minDoc=require("min-document");if(typeof document!=="undefined"){module.exports=document}else{var doccy=topLevel["__GLOBAL_DOCUMENT_CACHE@4"];if(!doccy){doccy=topLevel["__GLOBAL_DOCUMENT_CACHE@4"]=minDoc}module.exports=doccy}}).call(this,typeof global!=="undefined"?global:typeof self!=="undefined"?self:typeof window!=="undefined"?window:{})},{"min-document":1}],10:[function(require,module,exports){"use strict";module.exports=function isObject(x){return typeof x==="object"&&x!==null}},{}],11:[function(require,module,exports){var nativeIsArray=Array.isArray;var toString=Object.prototype.toString;module.exports=nativeIsArray||isArray;function isArray(obj){return toString.call(obj)==="[object Array]"}},{}],12:[function(require,module,exports){var patch=require("./vdom/patch.js");module.exports=patch},{"./vdom/patch.js":17}],13:[function(require,module,exports){var isObject=require("is-object");var isHook=require("../vnode/is-vhook.js");module.exports=applyProperties;function applyProperties(node,props,previous){for(var propName in props){var propValue=props[propName];if(propValue===undefined){removeProperty(node,propName,propValue,previous)}else if(isHook(propValue)){removeProperty(node,propName,propValue,previous);if(propValue.hook){propValue.hook(node,propName,previous?previous[propName]:undefined)}}else{if(isObject(propValue)){patchObject(node,props,previous,propName,propValue)}else{node[propName]=propValue}}}}function removeProperty(node,propName,propValue,previous){if(previous){var previousValue=previous[propName];if(!isHook(previousValue)){if(propName==="attributes"){for(var attrName in previousValue){node.removeAttribute(attrName)}}else if(propName==="style"){for(var i in previousValue){node.style[i]=""}}else if(typeof previousValue==="string"){node[propName]=""}else{node[propName]=null}}else if(previousValue.unhook){previousValue.unhook(node,propName,propValue)}}}function patchObject(node,props,previous,propName,propValue){var previousValue=previous?previous[propName]:undefined;if(propName==="attributes"){for(var attrName in propValue){var attrValue=propValue[attrName];if(attrValue===undefined){node.removeAttribute(attrName)}else{node.setAttribute(attrName,attrValue)}}return}if(previousValue&&isObject(previousValue)&&getPrototype(previousValue)!==getPrototype(propValue)){node[propName]=propValue;return}if(!isObject(node[propName])){node[propName]={}}var replacer=propName==="style"?"":undefined;for(var k in propValue){var value=propValue[k];node[propName][k]=value===undefined?replacer:value}}function getPrototype(value){if(Object.getPrototypeOf){return Object.getPrototypeOf(value)}else if(value.__proto__){return value.__proto__}else if(value.constructor){return value.constructor.prototype}}},{"../vnode/is-vhook.js":25,"is-object":10}],14:[function(require,module,exports){var document=require("global/document");var applyProperties=require("./apply-properties");var isVNode=require("../vnode/is-vnode.js");var isVText=require("../vnode/is-vtext.js");var isWidget=require("../vnode/is-widget.js");var handleThunk=require("../vnode/handle-thunk.js");module.exports=createElement;function createElement(vnode,opts){var doc=opts?opts.document||document:document;var warn=opts?opts.warn:null;vnode=handleThunk(vnode).a;if(isWidget(vnode)){return vnode.init()}else if(isVText(vnode)){return doc.createTextNode(vnode.text)}else if(!isVNode(vnode)){if(warn){warn("Item is not a valid virtual dom node",vnode)}return null}var node=vnode.namespace===null?doc.createElement(vnode.tagName):doc.createElementNS(vnode.namespace,vnode.tagName);var props=vnode.properties;applyProperties(node,props);var children=vnode.children;for(var i=0;i<children.length;i++){var childNode=createElement(children[i],opts);if(childNode){node.appendChild(childNode)}}return node}},{"../vnode/handle-thunk.js":23,"../vnode/is-vnode.js":26,"../vnode/is-vtext.js":27,"../vnode/is-widget.js":28,"./apply-properties":13,"global/document":9}],15:[function(require,module,exports){var noChild={};module.exports=domIndex;function domIndex(rootNode,tree,indices,nodes){if(!indices||indices.length===0){return{}}else{indices.sort(ascending);return recurse(rootNode,tree,indices,nodes,0)}}function recurse(rootNode,tree,indices,nodes,rootIndex){nodes=nodes||{};if(rootNode){if(indexInRange(indices,rootIndex,rootIndex)){nodes[rootIndex]=rootNode}var vChildren=tree.children;if(vChildren){var childNodes=rootNode.childNodes;for(var i=0;i<tree.children.length;i++){rootIndex+=1;var vChild=vChildren[i]||noChild;var nextIndex=rootIndex+(vChild.count||0);if(indexInRange(indices,rootIndex,nextIndex)){recurse(childNodes[i],vChild,indices,nodes,rootIndex)}rootIndex=nextIndex}}}return nodes}function indexInRange(indices,left,right){if(indices.length===0){return false}var minIndex=0;var maxIndex=indices.length-1;var currentIndex;var currentItem;while(minIndex<=maxIndex){currentIndex=(maxIndex+minIndex)/2>>0;currentItem=indices[currentIndex];if(minIndex===maxIndex){return currentItem>=left&¤tItem<=right}else if(currentItem<left){minIndex=currentIndex+1}else if(currentItem>right){maxIndex=currentIndex-1}else{return true}}return false}function ascending(a,b){return a>b?1:-1}},{}],16:[function(require,module,exports){var applyProperties=require("./apply-properties");var isWidget=require("../vnode/is-widget.js");var VPatch=require("../vnode/vpatch.js");var updateWidget=require("./update-widget");module.exports=applyPatch;function applyPatch(vpatch,domNode,renderOptions){var type=vpatch.type;var vNode=vpatch.vNode;var patch=vpatch.patch;switch(type){case VPatch.REMOVE:return removeNode(domNode,vNode);case VPatch.INSERT:return insertNode(domNode,patch,renderOptions);case VPatch.VTEXT:return stringPatch(domNode,vNode,patch,renderOptions);case VPatch.WIDGET:return widgetPatch(domNode,vNode,patch,renderOptions);case VPatch.VNODE:return vNodePatch(domNode,vNode,patch,renderOptions);case VPatch.ORDER:reorderChildren(domNode,patch);return domNode;case VPatch.PROPS:applyProperties(domNode,patch,vNode.properties);return domNode;case VPatch.THUNK:return replaceRoot(domNode,renderOptions.patch(domNode,patch,renderOptions));default:return domNode}}function removeNode(domNode,vNode){var parentNode=domNode.parentNode;if(parentNode){parentNode.removeChild(domNode)}destroyWidget(domNode,vNode);return null}function insertNode(parentNode,vNode,renderOptions){var newNode=renderOptions.render(vNode,renderOptions);if(parentNode){parentNode.appendChild(newNode)}return parentNode}function stringPatch(domNode,leftVNode,vText,renderOptions){var newNode;if(domNode.nodeType===3){domNode.replaceData(0,domNode.length,vText.text);newNode=domNode}else{var parentNode=domNode.parentNode;newNode=renderOptions.render(vText,renderOptions);if(parentNode&&newNode!==domNode){parentNode.replaceChild(newNode,domNode)}}return newNode}function widgetPatch(domNode,leftVNode,widget,renderOptions){var updating=updateWidget(leftVNode,widget);var newNode;if(updating){newNode=widget.update(leftVNode,domNode)||domNode}else{newNode=renderOptions.render(widget,renderOptions)}var parentNode=domNode.parentNode;if(parentNode&&newNode!==domNode){parentNode.replaceChild(newNode,domNode)}if(!updating){destroyWidget(domNode,leftVNode)}return newNode}function vNodePatch(domNode,leftVNode,vNode,renderOptions){var parentNode=domNode.parentNode;var newNode=renderOptions.render(vNode,renderOptions);if(parentNode&&newNode!==domNode){parentNode.replaceChild(newNode,domNode)}return newNode}function destroyWidget(domNode,w){if(typeof w.destroy==="function"&&isWidget(w)){w.destroy(domNode)}}function reorderChildren(domNode,moves){var childNodes=domNode.childNodes;var keyMap={};var node;var remove;var insert;for(var i=0;i<moves.removes.length;i++){remove=moves.removes[i];node=childNodes[remove.from];if(remove.key){keyMap[remove.key]=node}domNode.removeChild(node)}var length=childNodes.length;for(var j=0;j<moves.inserts.length;j++){insert=moves.inserts[j];node=keyMap[insert.key];domNode.insertBefore(node,insert.to>=length++?null:childNodes[insert.to])}}function replaceRoot(oldRoot,newRoot){if(oldRoot&&newRoot&&oldRoot!==newRoot&&oldRoot.parentNode){oldRoot.parentNode.replaceChild(newRoot,oldRoot)}return newRoot}},{"../vnode/is-widget.js":28,"../vnode/vpatch.js":31,"./apply-properties":13,"./update-widget":18}],17:[function(require,module,exports){var document=require("global/document");var isArray=require("x-is-array");var render=require("./create-element");var domIndex=require("./dom-index");var patchOp=require("./patch-op");module.exports=patch;function patch(rootNode,patches,renderOptions){renderOptions=renderOptions||{};renderOptions.patch=renderOptions.patch&&renderOptions.patch!==patch?renderOptions.patch:patchRecursive;renderOptions.render=renderOptions.render||render;return renderOptions.patch(rootNode,patches,renderOptions)}function patchRecursive(rootNode,patches,renderOptions){var indices=patchIndices(patches);if(indices.length===0){return rootNode}var index=domIndex(rootNode,patches.a,indices);var ownerDocument=rootNode.ownerDocument;if(!renderOptions.document&&ownerDocument!==document){renderOptions.document=ownerDocument}for(var i=0;i<indices.length;i++){var nodeIndex=indices[i];rootNode=applyPatch(rootNode,index[nodeIndex],patches[nodeIndex],renderOptions)}return rootNode}function applyPatch(rootNode,domNode,patchList,renderOptions){if(!domNode){return rootNode}var newNode;if(isArray(patchList)){for(var i=0;i<patchList.length;i++){newNode=patchOp(patchList[i],domNode,renderOptions);if(domNode===rootNode){rootNode=newNode}}}else{newNode=patchOp(patchList,domNode,renderOptions);if(domNode===rootNode){rootNode=newNode}}return rootNode}function patchIndices(patches){var indices=[];for(var key in patches){if(key!=="a"){indices.push(Number(key))}}return indices}},{"./create-element":14,"./dom-index":15,"./patch-op":16,"global/document":9,"x-is-array":11}],18:[function(require,module,exports){var isWidget=require("../vnode/is-widget.js");module.exports=updateWidget;function updateWidget(a,b){if(isWidget(a)&&isWidget(b)){if("name"in a&&"name"in b){return a.id===b.id}else{return a.init===b.init}}return false}},{"../vnode/is-widget.js":28}],19:[function(require,module,exports){"use strict";var EvStore=require("ev-store");module.exports=EvHook;function EvHook(value){if(!(this instanceof EvHook)){return new EvHook(value)}this.value=value}EvHook.prototype.hook=function(node,propertyName){var es=EvStore(node);var propName=propertyName.substr(3);es[propName]=this.value};EvHook.prototype.unhook=function(node,propertyName){var es=EvStore(node);var propName=propertyName.substr(3);es[propName]=undefined}},{"ev-store":6}],20:[function(require,module,exports){"use strict";module.exports=SoftSetHook;function SoftSetHook(value){if(!(this instanceof SoftSetHook)){return new SoftSetHook(value)}this.value=value}SoftSetHook.prototype.hook=function(node,propertyName){if(node[propertyName]!==this.value){node[propertyName]=this.value}}},{}],21:[function(require,module,exports){"use strict";var isArray=require("x-is-array");var VNode=require("../vnode/vnode.js");var VText=require("../vnode/vtext.js");var isVNode=require("../vnode/is-vnode");var isVText=require("../vnode/is-vtext");var isWidget=require("../vnode/is-widget");var isHook=require("../vnode/is-vhook");var isVThunk=require("../vnode/is-thunk");var parseTag=require("./parse-tag.js");var softSetHook=require("./hooks/soft-set-hook.js");var evHook=require("./hooks/ev-hook.js");module.exports=h;function h(tagName,properties,children){var childNodes=[];var tag,props,key,namespace;if(!children&&isChildren(properties)){children=properties;props={}}props=props||properties||{};tag=parseTag(tagName,props);if(props.hasOwnProperty("key")){key=props.key;props.key=undefined}if(props.hasOwnProperty("namespace")){namespace=props.namespace;props.namespace=undefined}if(tag==="INPUT"&&!namespace&&props.hasOwnProperty("value")&&props.value!==undefined&&!isHook(props.value)){props.value=softSetHook(props.value)}transformProperties(props);if(children!==undefined&&children!==null){addChild(children,childNodes,tag,props)}return new VNode(tag,props,childNodes,key,namespace)}function addChild(c,childNodes,tag,props){if(typeof c==="string"){childNodes.push(new VText(c))}else if(typeof c==="number"){childNodes.push(new VText(String(c)))}else if(isChild(c)){childNodes.push(c)}else if(isArray(c)){for(var i=0;i<c.length;i++){addChild(c[i],childNodes,tag,props)}}else if(c===null||c===undefined){return}else{throw UnexpectedVirtualElement({foreignObject:c,parentVnode:{tagName:tag,properties:props}})}}function transformProperties(props){for(var propName in props){if(props.hasOwnProperty(propName)){var value=props[propName];if(isHook(value)){continue}if(propName.substr(0,3)==="ev-"){props[propName]=evHook(value)}}}}function isChild(x){return isVNode(x)||isVText(x)||isWidget(x)||isVThunk(x)}function isChildren(x){return typeof x==="string"||isArray(x)||isChild(x)}function UnexpectedVirtualElement(data){var err=new Error;err.type="virtual-hyperscript.unexpected.virtual-element";err.message="Unexpected virtual child passed to h().\n"+"Expected a VNode / Vthunk / VWidget / string but:\n"+"got:\n"+errorString(data.foreignObject)+".\n"+"The parent vnode is:\n"+errorString(data.parentVnode);"\n"+"Suggested fix: change your `h(..., [ ... ])` callsite.";err.foreignObject=data.foreignObject;err.parentVnode=data.parentVnode;return err}function errorString(obj){try{return JSON.stringify(obj,null," ")}catch(e){return String(obj)}}},{"../vnode/is-thunk":24,"../vnode/is-vhook":25,"../vnode/is-vnode":26,"../vnode/is-vtext":27,"../vnode/is-widget":28,"../vnode/vnode.js":30,"../vnode/vtext.js":32,"./hooks/ev-hook.js":19,"./hooks/soft-set-hook.js":20,"./parse-tag.js":22,"x-is-array":11}],22:[function(require,module,exports){"use strict";var split=require("browser-split");var classIdSplit=/([\.#]?[a-zA-Z0-9\u007F-\uFFFF_:-]+)/;var notClassId=/^\.|#/;module.exports=parseTag;function parseTag(tag,props){if(!tag){return"DIV"}var noId=!props.hasOwnProperty("id");var tagParts=split(tag,classIdSplit);var tagName=null;if(notClassId.test(tagParts[1])){tagName="DIV"}var classes,part,type,i;for(i=0;i<tagParts.length;i++){part=tagParts[i];if(!part){continue}type=part.charAt(0);if(!tagName){tagName=part}else if(type==="."){classes=classes||[];classes.push(part.substring(1,part.length))}else if(type==="#"&&noId){props.id=part.substring(1,part.length)}}if(classes){if(props.className){classes.push(props.className)}props.className=classes.join(" ")}return props.namespace?tagName:tagName.toUpperCase()}},{"browser-split":5}],23:[function(require,module,exports){var isVNode=require("./is-vnode");var isVText=require("./is-vtext");var isWidget=require("./is-widget");var isThunk=require("./is-thunk");module.exports=handleThunk;function handleThunk(a,b){var renderedA=a;var renderedB=b;if(isThunk(b)){renderedB=renderThunk(b,a)}if(isThunk(a)){renderedA=renderThunk(a,null)}return{a:renderedA,b:renderedB}}function renderThunk(thunk,previous){var renderedThunk=thunk.vnode;if(!renderedThunk){renderedThunk=thunk.vnode=thunk.render(previous)}if(!(isVNode(renderedThunk)||isVText(renderedThunk)||isWidget(renderedThunk))){throw new Error("thunk did not return a valid node")}return renderedThunk}},{"./is-thunk":24,"./is-vnode":26,"./is-vtext":27,"./is-widget":28}],24:[function(require,module,exports){module.exports=isThunk;function isThunk(t){return t&&t.type==="Thunk"}},{}],25:[function(require,module,exports){module.exports=isHook;function isHook(hook){return hook&&(typeof hook.hook==="function"&&!hook.hasOwnProperty("hook")||typeof hook.unhook==="function"&&!hook.hasOwnProperty("unhook"))}},{}],26:[function(require,module,exports){var version=require("./version");module.exports=isVirtualNode;function isVirtualNode(x){return x&&x.type==="VirtualNode"&&x.version===version}},{"./version":29}],27:[function(require,module,exports){var version=require("./version");module.exports=isVirtualText;function isVirtualText(x){return x&&x.type==="VirtualText"&&x.version===version}},{"./version":29}],28:[function(require,module,exports){module.exports=isWidget;function isWidget(w){return w&&w.type==="Widget"}},{}],29:[function(require,module,exports){module.exports="2"},{}],30:[function(require,module,exports){var version=require("./version");var isVNode=require("./is-vnode");var isWidget=require("./is-widget");var isThunk=require("./is-thunk");var isVHook=require("./is-vhook");module.exports=VirtualNode;var noProperties={};var noChildren=[];function VirtualNode(tagName,properties,children,key,namespace){this.tagName=tagName;this.properties=properties||noProperties;this.children=children||noChildren;this.key=key!=null?String(key):undefined;this.namespace=typeof namespace==="string"?namespace:null;var count=children&&children.length||0;var descendants=0;var hasWidgets=false;var hasThunks=false;var descendantHooks=false;var hooks;for(var propName in properties){if(properties.hasOwnProperty(propName)){var property=properties[propName];if(isVHook(property)&&property.unhook){if(!hooks){hooks={}}hooks[propName]=property}}}for(var i=0;i<count;i++){var child=children[i];if(isVNode(child)){descendants+=child.count||0;if(!hasWidgets&&child.hasWidgets){hasWidgets=true}if(!hasThunks&&child.hasThunks){hasThunks=true}if(!descendantHooks&&(child.hooks||child.descendantHooks)){descendantHooks=true}}else if(!hasWidgets&&isWidget(child)){if(typeof child.destroy==="function"){hasWidgets=true}}else if(!hasThunks&&isThunk(child)){hasThunks=true}}this.count=count+descendants;this.hasWidgets=hasWidgets;this.hasThunks=hasThunks;this.hooks=hooks;this.descendantHooks=descendantHooks}VirtualNode.prototype.version=version;VirtualNode.prototype.type="VirtualNode"},{"./is-thunk":24,"./is-vhook":25,"./is-vnode":26,"./is-widget":28,"./version":29}],31:[function(require,module,exports){var version=require("./version");VirtualPatch.NONE=0;VirtualPatch.VTEXT=1;VirtualPatch.VNODE=2;VirtualPatch.WIDGET=3;VirtualPatch.PROPS=4;VirtualPatch.ORDER=5;VirtualPatch.INSERT=6;VirtualPatch.REMOVE=7;VirtualPatch.THUNK=8;module.exports=VirtualPatch;function VirtualPatch(type,vNode,patch){this.type=Number(type);this.vNode=vNode;this.patch=patch}VirtualPatch.prototype.version=version;VirtualPatch.prototype.type="VirtualPatch"},{"./version":29}],32:[function(require,module,exports){var version=require("./version");module.exports=VirtualText;function VirtualText(text){this.text=String(text)}VirtualText.prototype.version=version;VirtualText.prototype.type="VirtualText"},{"./version":29}],33:[function(require,module,exports){var isObject=require("is-object");var isHook=require("../vnode/is-vhook");module.exports=diffProps;function diffProps(a,b){var diff;for(var aKey in a){if(!(aKey in b)){diff=diff||{};diff[aKey]=undefined}var aValue=a[aKey];var bValue=b[aKey];if(aValue===bValue){continue}else if(isObject(aValue)&&isObject(bValue)){if(getPrototype(bValue)!==getPrototype(aValue)){diff=diff||{};diff[aKey]=bValue}else if(isHook(bValue)){diff=diff||{};diff[aKey]=bValue}else{var objectDiff=diffProps(aValue,bValue);if(objectDiff){diff=diff||{};diff[aKey]=objectDiff}}}else{diff=diff||{};diff[aKey]=bValue}}for(var bKey in b){if(!(bKey in a)){diff=diff||{};diff[bKey]=b[bKey]}}return diff}function getPrototype(value){if(Object.getPrototypeOf){return Object.getPrototypeOf(value)}else if(value.__proto__){return value.__proto__}else if(value.constructor){return value.constructor.prototype}}},{"../vnode/is-vhook":25,"is-object":10}],34:[function(require,module,exports){var isArray=require("x-is-array");var VPatch=require("../vnode/vpatch");var isVNode=require("../vnode/is-vnode");var isVText=require("../vnode/is-vtext");var isWidget=require("../vnode/is-widget");var isThunk=require("../vnode/is-thunk");var handleThunk=require("../vnode/handle-thunk");var diffProps=require("./diff-props");module.exports=diff;function diff(a,b){var patch={a:a};walk(a,b,patch,0);return patch}function walk(a,b,patch,index){if(a===b){return}var apply=patch[index];var applyClear=false;if(isThunk(a)||isThunk(b)){thunks(a,b,patch,index)}else if(b==null){if(!isWidget(a)){clearState(a,patch,index);apply=patch[index]}apply=appendPatch(apply,new VPatch(VPatch.REMOVE,a,b))}else if(isVNode(b)){if(isVNode(a)){if(a.tagName===b.tagName&&a.namespace===b.namespace&&a.key===b.key){var propsPatch=diffProps(a.properties,b.properties);if(propsPatch){apply=appendPatch(apply,new VPatch(VPatch.PROPS,a,propsPatch))}apply=diffChildren(a,b,patch,apply,index)}else{apply=appendPatch(apply,new VPatch(VPatch.VNODE,a,b));applyClear=true}}else{apply=appendPatch(apply,new VPatch(VPatch.VNODE,a,b));applyClear=true}}else if(isVText(b)){if(!isVText(a)){apply=appendPatch(apply,new VPatch(VPatch.VTEXT,a,b));applyClear=true}else if(a.text!==b.text){apply=appendPatch(apply,new VPatch(VPatch.VTEXT,a,b))}}else if(isWidget(b)){if(!isWidget(a)){applyClear=true}apply=appendPatch(apply,new VPatch(VPatch.WIDGET,a,b))}if(apply){patch[index]=apply}if(applyClear){clearState(a,patch,index)}}function diffChildren(a,b,patch,apply,index){var aChildren=a.children;var orderedSet=reorder(aChildren,b.children);var bChildren=orderedSet.children;var aLen=aChildren.length;var bLen=bChildren.length;var len=aLen>bLen?aLen:bLen;for(var i=0;i<len;i++){var leftNode=aChildren[i];var rightNode=bChildren[i];index+=1;if(!leftNode){if(rightNode){apply=appendPatch(apply,new VPatch(VPatch.INSERT,null,rightNode))}}else{walk(leftNode,rightNode,patch,index)}if(isVNode(leftNode)&&leftNode.count){index+=leftNode.count}}if(orderedSet.moves){apply=appendPatch(apply,new VPatch(VPatch.ORDER,a,orderedSet.moves))}return apply}function clearState(vNode,patch,index){unhook(vNode,patch,index);destroyWidgets(vNode,patch,index)}function destroyWidgets(vNode,patch,index){if(isWidget(vNode)){if(typeof vNode.destroy==="function"){patch[index]=appendPatch(patch[index],new VPatch(VPatch.REMOVE,vNode,null))}}else if(isVNode(vNode)&&(vNode.hasWidgets||vNode.hasThunks)){var children=vNode.children;var len=children.length;for(var i=0;i<len;i++){var child=children[i];index+=1;destroyWidgets(child,patch,index);if(isVNode(child)&&child.count){index+=child.count}}}else if(isThunk(vNode)){thunks(vNode,null,patch,index)}}function thunks(a,b,patch,index){var nodes=handleThunk(a,b);var thunkPatch=diff(nodes.a,nodes.b);if(hasPatches(thunkPatch)){patch[index]=new VPatch(VPatch.THUNK,null,thunkPatch)}}function hasPatches(patch){for(var index in patch){if(index!=="a"){return true}}return false}function unhook(vNode,patch,index){if(isVNode(vNode)){if(vNode.hooks){patch[index]=appendPatch(patch[index],new VPatch(VPatch.PROPS,vNode,undefinedKeys(vNode.hooks)))}if(vNode.descendantHooks||vNode.hasThunks){var children=vNode.children;var len=children.length;for(var i=0;i<len;i++){var child=children[i];index+=1;unhook(child,patch,index);if(isVNode(child)&&child.count){index+=child.count}}}}else if(isThunk(vNode)){thunks(vNode,null,patch,index)}}function undefinedKeys(obj){var result={};for(var key in obj){result[key]=undefined}return result}function reorder(aChildren,bChildren){var bChildIndex=keyIndex(bChildren);var bKeys=bChildIndex.keys;var bFree=bChildIndex.free;if(bFree.length===bChildren.length){return{children:bChildren,moves:null}}var aChildIndex=keyIndex(aChildren);var aKeys=aChildIndex.keys;var aFree=aChildIndex.free;if(aFree.length===aChildren.length){return{children:bChildren,moves:null}}var newChildren=[];var freeIndex=0;var freeCount=bFree.length;var deletedItems=0;for(var i=0;i<aChildren.length;i++){var aItem=aChildren[i];var itemIndex;if(aItem.key){if(bKeys.hasOwnProperty(aItem.key)){itemIndex=bKeys[aItem.key];newChildren.push(bChildren[itemIndex])}else{itemIndex=i-deletedItems++;newChildren.push(null)}}else{if(freeIndex<freeCount){itemIndex=bFree[freeIndex++];newChildren.push(bChildren[itemIndex])}else{itemIndex=i-deletedItems++;newChildren.push(null)}}}var lastFreeIndex=freeIndex>=bFree.length?bChildren.length:bFree[freeIndex];for(var j=0;j<bChildren.length;j++){var newItem=bChildren[j];if(newItem.key){if(!aKeys.hasOwnProperty(newItem.key)){newChildren.push(newItem)}}else if(j>=lastFreeIndex){newChildren.push(newItem)}}var simulate=newChildren.slice();var simulateIndex=0;var removes=[];var inserts=[];var simulateItem;for(var k=0;k<bChildren.length;){var wantedItem=bChildren[k];simulateItem=simulate[simulateIndex];while(simulateItem===null&&simulate.length){removes.push(remove(simulate,simulateIndex,null));simulateItem=simulate[simulateIndex]}if(!simulateItem||simulateItem.key!==wantedItem.key){if(wantedItem.key){if(simulateItem&&simulateItem.key){if(bKeys[simulateItem.key]!==k+1){removes.push(remove(simulate,simulateIndex,simulateItem.key));simulateItem=simulate[simulateIndex];if(!simulateItem||simulateItem.key!==wantedItem.key){inserts.push({key:wantedItem.key,to:k})}else{simulateIndex++}}else{inserts.push({key:wantedItem.key,to:k})}}else{inserts.push({key:wantedItem.key,to:k})}k++}else if(simulateItem&&simulateItem.key){removes.push(remove(simulate,simulateIndex,simulateItem.key))}}else{simulateIndex++;k++}}while(simulateIndex<simulate.length){simulateItem=simulate[simulateIndex];removes.push(remove(simulate,simulateIndex,simulateItem&&simulateItem.key))}if(removes.length===deletedItems&&!inserts.length){return{children:newChildren,moves:null}}return{children:newChildren,moves:{removes:removes,inserts:inserts}}}function remove(arr,index,key){arr.splice(index,1);return{from:index,key:key}}function keyIndex(children){var keys={};var free=[];var length=children.length;for(var i=0;i<length;i++){var child=children[i];if(child.key){keys[child.key]=i}else{free.push(i)}}return{keys:keys,free:free}}function appendPatch(apply,patch){if(apply){if(isArray(apply)){apply.push(patch)}else{apply=[apply,patch]}return apply}else{return patch}}},{"../vnode/handle-thunk":23,"../vnode/is-thunk":24,"../vnode/is-vnode":26,"../vnode/is-vtext":27,"../vnode/is-widget":28,"../vnode/vpatch":31,"./diff-props":33,"x-is-array":11}],"virtual-dom":[function(require,module,exports){var diff=require("./diff.js");var patch=require("./patch.js");var h=require("./h.js");var create=require("./create-element.js");var VNode=require("./vnode/vnode.js");var VText=require("./vnode/vtext.js");module.exports={diff:diff,patch:patch,h:h,create:create,VNode:VNode,VText:VText}},{"./create-element.js":2,"./diff.js":3,"./h.js":4,"./patch.js":12,"./vnode/vnode.js":30,"./vnode/vtext.js":32}]},{},[]);require=function e(t,n,r){function s(o,u){if(!n[o]){if(!t[o]){var a=typeof require=="function"&&require;if(!u&&a)return a(o,!0);if(i)return i(o,!0);var f=new Error("Cannot find module '"+o+"'");throw f.code="MODULE_NOT_FOUND",f}var l=n[o]={exports:{}};t[o][0].call(l.exports,function(e){var n=t[o][1][e];return s(n?n:e)},l,l.exports,e,t,n,r)}return n[o].exports}var i=typeof require=="function"&&require;for(var o=0;o<r.length;o++)s(r[o]);return s}({1:[function(require,module,exports){},{}],2:[function(require,module,exports){var createElement=require("./vdom/create-element.js");module.exports=createElement},{"./vdom/create-element.js":14}],3:[function(require,module,exports){var diff=require("./vtree/diff.js");module.exports=diff},{"./vtree/diff.js":34}],4:[function(require,module,exports){var h=require("./virtual-hyperscript/index.js");module.exports=h},{"./virtual-hyperscript/index.js":21}],5:[function(require,module,exports){module.exports=function split(undef){var nativeSplit=String.prototype.split,compliantExecNpcg=/()??/.exec("")[1]===undef,self;self=function(str,separator,limit){if(Object.prototype.toString.call(separator)!=="[object RegExp]"){return nativeSplit.call(str,separator,limit)}var output=[],flags=(separator.ignoreCase?"i":"")+(separator.multiline?"m":"")+(separator.extended?"x":"")+(separator.sticky?"y":""),lastLastIndex=0,separator=new RegExp(separator.source,flags+"g"),separator2,match,lastIndex,lastLength;str+="";if(!compliantExecNpcg){separator2=new RegExp("^"+separator.source+"$(?!\\s)",flags)}limit=limit===undef?-1>>>0:limit>>>0;while(match=separator.exec(str)){lastIndex=match.index+match[0].length;if(lastIndex>lastLastIndex){output.push(str.slice(lastLastIndex,match.index));if(!compliantExecNpcg&&match.length>1){ | |
match[0].replace(separator2,function(){for(var i=1;i<arguments.length-2;i++){if(arguments[i]===undef){match[i]=undef}}})}if(match.length>1&&match.index<str.length){Array.prototype.push.apply(output,match.slice(1))}lastLength=match[0].length;lastLastIndex=lastIndex;if(output.length>=limit){break}}if(separator.lastIndex===match.index){separator.lastIndex++}}if(lastLastIndex===str.length){if(lastLength||!separator.test("")){output.push("")}}else{output.push(str.slice(lastLastIndex))}return output.length>limit?output.slice(0,limit):output};return self}()},{}],6:[function(require,module,exports){"use strict";var OneVersionConstraint=require("individual/one-version");var MY_VERSION="7";OneVersionConstraint("ev-store",MY_VERSION);var hashKey="__EV_STORE_KEY@"+MY_VERSION;module.exports=EvStore;function EvStore(elem){var hash=elem[hashKey];if(!hash){hash=elem[hashKey]={}}return hash}},{"individual/one-version":8}],7:[function(require,module,exports){(function(global){"use strict";var root=typeof window!=="undefined"?window:typeof global!=="undefined"?global:{};module.exports=Individual;function Individual(key,value){if(key in root){return root[key]}root[key]=value;return value}}).call(this,typeof global!=="undefined"?global:typeof self!=="undefined"?self:typeof window!=="undefined"?window:{})},{}],8:[function(require,module,exports){"use strict";var Individual=require("./index.js");module.exports=OneVersion;function OneVersion(moduleName,version,defaultValue){var key="__INDIVIDUAL_ONE_VERSION_"+moduleName;var enforceKey=key+"_ENFORCE_SINGLETON";var versionValue=Individual(enforceKey,version);if(versionValue!==version){throw new Error("Can only have one copy of "+moduleName+".\n"+"You already have version "+versionValue+" installed.\n"+"This means you cannot install version "+version)}return Individual(key,defaultValue)}},{"./index.js":7}],9:[function(require,module,exports){(function(global){var topLevel=typeof global!=="undefined"?global:typeof window!=="undefined"?window:{};var minDoc=require("min-document");if(typeof document!=="undefined"){module.exports=document}else{var doccy=topLevel["__GLOBAL_DOCUMENT_CACHE@4"];if(!doccy){doccy=topLevel["__GLOBAL_DOCUMENT_CACHE@4"]=minDoc}module.exports=doccy}}).call(this,typeof global!=="undefined"?global:typeof self!=="undefined"?self:typeof window!=="undefined"?window:{})},{"min-document":1}],10:[function(require,module,exports){"use strict";module.exports=function isObject(x){return typeof x==="object"&&x!==null}},{}],11:[function(require,module,exports){var nativeIsArray=Array.isArray;var toString=Object.prototype.toString;module.exports=nativeIsArray||isArray;function isArray(obj){return toString.call(obj)==="[object Array]"}},{}],12:[function(require,module,exports){var patch=require("./vdom/patch.js");module.exports=patch},{"./vdom/patch.js":17}],13:[function(require,module,exports){var isObject=require("is-object");var isHook=require("../vnode/is-vhook.js");module.exports=applyProperties;function applyProperties(node,props,previous){for(var propName in props){var propValue=props[propName];if(propValue===undefined){removeProperty(node,propName,propValue,previous)}else if(isHook(propValue)){removeProperty(node,propName,propValue,previous);if(propValue.hook){propValue.hook(node,propName,previous?previous[propName]:undefined)}}else{if(isObject(propValue)){patchObject(node,props,previous,propName,propValue)}else{node[propName]=propValue}}}}function removeProperty(node,propName,propValue,previous){if(previous){var previousValue=previous[propName];if(!isHook(previousValue)){if(propName==="attributes"){for(var attrName in previousValue){node.removeAttribute(attrName)}}else if(propName==="style"){for(var i in previousValue){node.style[i]=""}}else if(typeof previousValue==="string"){node[propName]=""}else{node[propName]=null}}else if(previousValue.unhook){previousValue.unhook(node,propName,propValue)}}}function patchObject(node,props,previous,propName,propValue){var previousValue=previous?previous[propName]:undefined;if(propName==="attributes"){for(var attrName in propValue){var attrValue=propValue[attrName];if(attrValue===undefined){node.removeAttribute(attrName)}else{node.setAttribute(attrName,attrValue)}}return}if(previousValue&&isObject(previousValue)&&getPrototype(previousValue)!==getPrototype(propValue)){node[propName]=propValue;return}if(!isObject(node[propName])){node[propName]={}}var replacer=propName==="style"?"":undefined;for(var k in propValue){var value=propValue[k];node[propName][k]=value===undefined?replacer:value}}function getPrototype(value){if(Object.getPrototypeOf){return Object.getPrototypeOf(value)}else if(value.__proto__){return value.__proto__}else if(value.constructor){return value.constructor.prototype}}},{"../vnode/is-vhook.js":25,"is-object":10}],14:[function(require,module,exports){var document=require("global/document");var applyProperties=require("./apply-properties");var isVNode=require("../vnode/is-vnode.js");var isVText=require("../vnode/is-vtext.js");var isWidget=require("../vnode/is-widget.js");var handleThunk=require("../vnode/handle-thunk.js");module.exports=createElement;function createElement(vnode,opts){var doc=opts?opts.document||document:document;var warn=opts?opts.warn:null;vnode=handleThunk(vnode).a;if(isWidget(vnode)){return vnode.init()}else if(isVText(vnode)){return doc.createTextNode(vnode.text)}else if(!isVNode(vnode)){if(warn){warn("Item is not a valid virtual dom node",vnode)}return null}var node=vnode.namespace===null?doc.createElement(vnode.tagName):doc.createElementNS(vnode.namespace,vnode.tagName);var props=vnode.properties;applyProperties(node,props);var children=vnode.children;for(var i=0;i<children.length;i++){var childNode=createElement(children[i],opts);if(childNode){node.appendChild(childNode)}}return node}},{"../vnode/handle-thunk.js":23,"../vnode/is-vnode.js":26,"../vnode/is-vtext.js":27,"../vnode/is-widget.js":28,"./apply-properties":13,"global/document":9}],15:[function(require,module,exports){var noChild={};module.exports=domIndex;function domIndex(rootNode,tree,indices,nodes){if(!indices||indices.length===0){return{}}else{indices.sort(ascending);return recurse(rootNode,tree,indices,nodes,0)}}function recurse(rootNode,tree,indices,nodes,rootIndex){nodes=nodes||{};if(rootNode){if(indexInRange(indices,rootIndex,rootIndex)){nodes[rootIndex]=rootNode}var vChildren=tree.children;if(vChildren){var childNodes=rootNode.childNodes;for(var i=0;i<tree.children.length;i++){rootIndex+=1;var vChild=vChildren[i]||noChild;var nextIndex=rootIndex+(vChild.count||0);if(indexInRange(indices,rootIndex,nextIndex)){recurse(childNodes[i],vChild,indices,nodes,rootIndex)}rootIndex=nextIndex}}}return nodes}function indexInRange(indices,left,right){if(indices.length===0){return false}var minIndex=0;var maxIndex=indices.length-1;var currentIndex;var currentItem;while(minIndex<=maxIndex){currentIndex=(maxIndex+minIndex)/2>>0;currentItem=indices[currentIndex];if(minIndex===maxIndex){return currentItem>=left&¤tItem<=right}else if(currentItem<left){minIndex=currentIndex+1}else if(currentItem>right){maxIndex=currentIndex-1}else{return true}}return false}function ascending(a,b){return a>b?1:-1}},{}],16:[function(require,module,exports){var applyProperties=require("./apply-properties");var isWidget=require("../vnode/is-widget.js");var VPatch=require("../vnode/vpatch.js");var updateWidget=require("./update-widget");module.exports=applyPatch;function applyPatch(vpatch,domNode,renderOptions){var type=vpatch.type;var vNode=vpatch.vNode;var patch=vpatch.patch;switch(type){case VPatch.REMOVE:return removeNode(domNode,vNode);case VPatch.INSERT:return insertNode(domNode,patch,renderOptions);case VPatch.VTEXT:return stringPatch(domNode,vNode,patch,renderOptions);case VPatch.WIDGET:return widgetPatch(domNode,vNode,patch,renderOptions);case VPatch.VNODE:return vNodePatch(domNode,vNode,patch,renderOptions);case VPatch.ORDER:reorderChildren(domNode,patch);return domNode;case VPatch.PROPS:applyProperties(domNode,patch,vNode.properties);return domNode;case VPatch.THUNK:return replaceRoot(domNode,renderOptions.patch(domNode,patch,renderOptions));default:return domNode}}function removeNode(domNode,vNode){var parentNode=domNode.parentNode;if(parentNode){parentNode.removeChild(domNode)}destroyWidget(domNode,vNode);return null}function insertNode(parentNode,vNode,renderOptions){var newNode=renderOptions.render(vNode,renderOptions);if(parentNode){parentNode.appendChild(newNode)}return parentNode}function stringPatch(domNode,leftVNode,vText,renderOptions){var newNode;if(domNode.nodeType===3){domNode.replaceData(0,domNode.length,vText.text);newNode=domNode}else{var parentNode=domNode.parentNode;newNode=renderOptions.render(vText,renderOptions);if(parentNode&&newNode!==domNode){parentNode.replaceChild(newNode,domNode)}}return newNode}function widgetPatch(domNode,leftVNode,widget,renderOptions){var updating=updateWidget(leftVNode,widget);var newNode;if(updating){newNode=widget.update(leftVNode,domNode)||domNode}else{newNode=renderOptions.render(widget,renderOptions)}var parentNode=domNode.parentNode;if(parentNode&&newNode!==domNode){parentNode.replaceChild(newNode,domNode)}if(!updating){destroyWidget(domNode,leftVNode)}return newNode}function vNodePatch(domNode,leftVNode,vNode,renderOptions){var parentNode=domNode.parentNode;var newNode=renderOptions.render(vNode,renderOptions);if(parentNode&&newNode!==domNode){parentNode.replaceChild(newNode,domNode)}return newNode}function destroyWidget(domNode,w){if(typeof w.destroy==="function"&&isWidget(w)){w.destroy(domNode)}}function reorderChildren(domNode,moves){var childNodes=domNode.childNodes;var keyMap={};var node;var remove;var insert;for(var i=0;i<moves.removes.length;i++){remove=moves.removes[i];node=childNodes[remove.from];if(remove.key){keyMap[remove.key]=node}domNode.removeChild(node)}var length=childNodes.length;for(var j=0;j<moves.inserts.length;j++){insert=moves.inserts[j];node=keyMap[insert.key];domNode.insertBefore(node,insert.to>=length++?null:childNodes[insert.to])}}function replaceRoot(oldRoot,newRoot){if(oldRoot&&newRoot&&oldRoot!==newRoot&&oldRoot.parentNode){oldRoot.parentNode.replaceChild(newRoot,oldRoot)}return newRoot}},{"../vnode/is-widget.js":28,"../vnode/vpatch.js":31,"./apply-properties":13,"./update-widget":18}],17:[function(require,module,exports){var document=require("global/document");var isArray=require("x-is-array");var render=require("./create-element");var domIndex=require("./dom-index");var patchOp=require("./patch-op");module.exports=patch;function patch(rootNode,patches,renderOptions){renderOptions=renderOptions||{};renderOptions.patch=renderOptions.patch&&renderOptions.patch!==patch?renderOptions.patch:patchRecursive;renderOptions.render=renderOptions.render||render;return renderOptions.patch(rootNode,patches,renderOptions)}function patchRecursive(rootNode,patches,renderOptions){var indices=patchIndices(patches);if(indices.length===0){return rootNode}var index=domIndex(rootNode,patches.a,indices);var ownerDocument=rootNode.ownerDocument;if(!renderOptions.document&&ownerDocument!==document){renderOptions.document=ownerDocument}for(var i=0;i<indices.length;i++){var nodeIndex=indices[i];rootNode=applyPatch(rootNode,index[nodeIndex],patches[nodeIndex],renderOptions)}return rootNode}function applyPatch(rootNode,domNode,patchList,renderOptions){if(!domNode){return rootNode}var newNode;if(isArray(patchList)){for(var i=0;i<patchList.length;i++){newNode=patchOp(patchList[i],domNode,renderOptions);if(domNode===rootNode){rootNode=newNode}}}else{newNode=patchOp(patchList,domNode,renderOptions);if(domNode===rootNode){rootNode=newNode}}return rootNode}function patchIndices(patches){var indices=[];for(var key in patches){if(key!=="a"){indices.push(Number(key))}}return indices}},{"./create-element":14,"./dom-index":15,"./patch-op":16,"global/document":9,"x-is-array":11}],18:[function(require,module,exports){var isWidget=require("../vnode/is-widget.js");module.exports=updateWidget;function updateWidget(a,b){if(isWidget(a)&&isWidget(b)){if("name"in a&&"name"in b){return a.id===b.id}else{return a.init===b.init}}return false}},{"../vnode/is-widget.js":28}],19:[function(require,module,exports){"use strict";var EvStore=require("ev-store");module.exports=EvHook;function EvHook(value){if(!(this instanceof EvHook)){return new EvHook(value)}this.value=value}EvHook.prototype.hook=function(node,propertyName){var es=EvStore(node);var propName=propertyName.substr(3);es[propName]=this.value};EvHook.prototype.unhook=function(node,propertyName){var es=EvStore(node);var propName=propertyName.substr(3);es[propName]=undefined}},{"ev-store":6}],20:[function(require,module,exports){"use strict";module.exports=SoftSetHook;function SoftSetHook(value){if(!(this instanceof SoftSetHook)){return new SoftSetHook(value)}this.value=value}SoftSetHook.prototype.hook=function(node,propertyName){if(node[propertyName]!==this.value){node[propertyName]=this.value}}},{}],21:[function(require,module,exports){"use strict";var isArray=require("x-is-array");var VNode=require("../vnode/vnode.js");var VText=require("../vnode/vtext.js");var isVNode=require("../vnode/is-vnode");var isVText=require("../vnode/is-vtext");var isWidget=require("../vnode/is-widget");var isHook=require("../vnode/is-vhook");var isVThunk=require("../vnode/is-thunk");var parseTag=require("./parse-tag.js");var softSetHook=require("./hooks/soft-set-hook.js");var evHook=require("./hooks/ev-hook.js");module.exports=h;function h(tagName,properties,children){var childNodes=[];var tag,props,key,namespace;if(!children&&isChildren(properties)){children=properties;props={}}props=props||properties||{};tag=parseTag(tagName,props);if(props.hasOwnProperty("key")){key=props.key;props.key=undefined}if(props.hasOwnProperty("namespace")){namespace=props.namespace;props.namespace=undefined}if(tag==="INPUT"&&!namespace&&props.hasOwnProperty("value")&&props.value!==undefined&&!isHook(props.value)){props.value=softSetHook(props.value)}transformProperties(props);if(children!==undefined&&children!==null){addChild(children,childNodes,tag,props)}return new VNode(tag,props,childNodes,key,namespace)}function addChild(c,childNodes,tag,props){if(typeof c==="string"){childNodes.push(new VText(c))}else if(typeof c==="number"){childNodes.push(new VText(String(c)))}else if(isChild(c)){childNodes.push(c)}else if(isArray(c)){for(var i=0;i<c.length;i++){addChild(c[i],childNodes,tag,props)}}else if(c===null||c===undefined){return}else{throw UnexpectedVirtualElement({foreignObject:c,parentVnode:{tagName:tag,properties:props}})}}function transformProperties(props){for(var propName in props){if(props.hasOwnProperty(propName)){var value=props[propName];if(isHook(value)){continue}if(propName.substr(0,3)==="ev-"){props[propName]=evHook(value)}}}}function isChild(x){return isVNode(x)||isVText(x)||isWidget(x)||isVThunk(x)}function isChildren(x){return typeof x==="string"||isArray(x)||isChild(x)}function UnexpectedVirtualElement(data){var err=new Error;err.type="virtual-hyperscript.unexpected.virtual-element";err.message="Unexpected virtual child passed to h().\n"+"Expected a VNode / Vthunk / VWidget / string but:\n"+"got:\n"+errorString(data.foreignObject)+".\n"+"The parent vnode is:\n"+errorString(data.parentVnode);"\n"+"Suggested fix: change your `h(..., [ ... ])` callsite.";err.foreignObject=data.foreignObject;err.parentVnode=data.parentVnode;return err}function errorString(obj){try{return JSON.stringify(obj,null," ")}catch(e){return String(obj)}}},{"../vnode/is-thunk":24,"../vnode/is-vhook":25,"../vnode/is-vnode":26,"../vnode/is-vtext":27,"../vnode/is-widget":28,"../vnode/vnode.js":30,"../vnode/vtext.js":32,"./hooks/ev-hook.js":19,"./hooks/soft-set-hook.js":20,"./parse-tag.js":22,"x-is-array":11}],22:[function(require,module,exports){"use strict";var split=require("browser-split");var classIdSplit=/([\.#]?[a-zA-Z0-9\u007F-\uFFFF_:-]+)/;var notClassId=/^\.|#/;module.exports=parseTag;function parseTag(tag,props){if(!tag){return"DIV"}var noId=!props.hasOwnProperty("id");var tagParts=split(tag,classIdSplit);var tagName=null;if(notClassId.test(tagParts[1])){tagName="DIV"}var classes,part,type,i;for(i=0;i<tagParts.length;i++){part=tagParts[i];if(!part){continue}type=part.charAt(0);if(!tagName){tagName=part}else if(type==="."){classes=classes||[];classes.push(part.substring(1,part.length))}else if(type==="#"&&noId){props.id=part.substring(1,part.length)}}if(classes){if(props.className){classes.push(props.className)}props.className=classes.join(" ")}return props.namespace?tagName:tagName.toUpperCase()}},{"browser-split":5}],23:[function(require,module,exports){var isVNode=require("./is-vnode");var isVText=require("./is-vtext");var isWidget=require("./is-widget");var isThunk=require("./is-thunk");module.exports=handleThunk;function handleThunk(a,b){var renderedA=a;var renderedB=b;if(isThunk(b)){renderedB=renderThunk(b,a)}if(isThunk(a)){renderedA=renderThunk(a,null)}return{a:renderedA,b:renderedB}}function renderThunk(thunk,previous){var renderedThunk=thunk.vnode;if(!renderedThunk){renderedThunk=thunk.vnode=thunk.render(previous)}if(!(isVNode(renderedThunk)||isVText(renderedThunk)||isWidget(renderedThunk))){throw new Error("thunk did not return a valid node")}return renderedThunk}},{"./is-thunk":24,"./is-vnode":26,"./is-vtext":27,"./is-widget":28}],24:[function(require,module,exports){module.exports=isThunk;function isThunk(t){return t&&t.type==="Thunk"}},{}],25:[function(require,module,exports){module.exports=isHook;function isHook(hook){return hook&&(typeof hook.hook==="function"&&!hook.hasOwnProperty("hook")||typeof hook.unhook==="function"&&!hook.hasOwnProperty("unhook"))}},{}],26:[function(require,module,exports){var version=require("./version");module.exports=isVirtualNode;function isVirtualNode(x){return x&&x.type==="VirtualNode"&&x.version===version}},{"./version":29}],27:[function(require,module,exports){var version=require("./version");module.exports=isVirtualText;function isVirtualText(x){return x&&x.type==="VirtualText"&&x.version===version}},{"./version":29}],28:[function(require,module,exports){module.exports=isWidget;function isWidget(w){return w&&w.type==="Widget"}},{}],29:[function(require,module,exports){module.exports="2"},{}],30:[function(require,module,exports){var version=require("./version");var isVNode=require("./is-vnode");var isWidget=require("./is-widget");var isThunk=require("./is-thunk");var isVHook=require("./is-vhook");module.exports=VirtualNode;var noProperties={};var noChildren=[];function VirtualNode(tagName,properties,children,key,namespace){this.tagName=tagName;this.properties=properties||noProperties;this.children=children||noChildren;this.key=key!=null?String(key):undefined;this.namespace=typeof namespace==="string"?namespace:null;var count=children&&children.length||0;var descendants=0;var hasWidgets=false;var hasThunks=false;var descendantHooks=false;var hooks;for(var propName in properties){if(properties.hasOwnProperty(propName)){var property=properties[propName];if(isVHook(property)&&property.unhook){if(!hooks){hooks={}}hooks[propName]=property}}}for(var i=0;i<count;i++){var child=children[i];if(isVNode(child)){descendants+=child.count||0;if(!hasWidgets&&child.hasWidgets){hasWidgets=true}if(!hasThunks&&child.hasThunks){hasThunks=true}if(!descendantHooks&&(child.hooks||child.descendantHooks)){descendantHooks=true}}else if(!hasWidgets&&isWidget(child)){if(typeof child.destroy==="function"){hasWidgets=true}}else if(!hasThunks&&isThunk(child)){hasThunks=true}}this.count=count+descendants;this.hasWidgets=hasWidgets;this.hasThunks=hasThunks;this.hooks=hooks;this.descendantHooks=descendantHooks}VirtualNode.prototype.version=version;VirtualNode.prototype.type="VirtualNode"},{"./is-thunk":24,"./is-vhook":25,"./is-vnode":26,"./is-widget":28,"./version":29}],31:[function(require,module,exports){var version=require("./version");VirtualPatch.NONE=0;VirtualPatch.VTEXT=1;VirtualPatch.VNODE=2;VirtualPatch.WIDGET=3;VirtualPatch.PROPS=4;VirtualPatch.ORDER=5;VirtualPatch.INSERT=6;VirtualPatch.REMOVE=7;VirtualPatch.THUNK=8;module.exports=VirtualPatch;function VirtualPatch(type,vNode,patch){this.type=Number(type);this.vNode=vNode;this.patch=patch}VirtualPatch.prototype.version=version;VirtualPatch.prototype.type="VirtualPatch"},{"./version":29}],32:[function(require,module,exports){var version=require("./version");module.exports=VirtualText;function VirtualText(text){this.text=String(text)}VirtualText.prototype.version=version;VirtualText.prototype.type="VirtualText"},{"./version":29}],33:[function(require,module,exports){var isObject=require("is-object");var isHook=require("../vnode/is-vhook");module.exports=diffProps;function diffProps(a,b){var diff;for(var aKey in a){if(!(aKey in b)){diff=diff||{};diff[aKey]=undefined}var aValue=a[aKey];var bValue=b[aKey];if(aValue===bValue){continue}else if(isObject(aValue)&&isObject(bValue)){if(getPrototype(bValue)!==getPrototype(aValue)){diff=diff||{};diff[aKey]=bValue}else if(isHook(bValue)){diff=diff||{};diff[aKey]=bValue}else{var objectDiff=diffProps(aValue,bValue);if(objectDiff){diff=diff||{};diff[aKey]=objectDiff}}}else{diff=diff||{};diff[aKey]=bValue}}for(var bKey in b){if(!(bKey in a)){diff=diff||{};diff[bKey]=b[bKey]}}return diff}function getPrototype(value){if(Object.getPrototypeOf){return Object.getPrototypeOf(value)}else if(value.__proto__){return value.__proto__}else if(value.constructor){return value.constructor.prototype}}},{"../vnode/is-vhook":25,"is-object":10}],34:[function(require,module,exports){var isArray=require("x-is-array");var VPatch=require("../vnode/vpatch");var isVNode=require("../vnode/is-vnode");var isVText=require("../vnode/is-vtext");var isWidget=require("../vnode/is-widget");var isThunk=require("../vnode/is-thunk");var handleThunk=require("../vnode/handle-thunk");var diffProps=require("./diff-props");module.exports=diff;function diff(a,b){var patch={a:a};walk(a,b,patch,0);return patch}function walk(a,b,patch,index){if(a===b){return}var apply=patch[index];var applyClear=false;if(isThunk(a)||isThunk(b)){thunks(a,b,patch,index)}else if(b==null){if(!isWidget(a)){clearState(a,patch,index);apply=patch[index]}apply=appendPatch(apply,new VPatch(VPatch.REMOVE,a,b))}else if(isVNode(b)){if(isVNode(a)){if(a.tagName===b.tagName&&a.namespace===b.namespace&&a.key===b.key){var propsPatch=diffProps(a.properties,b.properties);if(propsPatch){apply=appendPatch(apply,new VPatch(VPatch.PROPS,a,propsPatch))}apply=diffChildren(a,b,patch,apply,index)}else{apply=appendPatch(apply,new VPatch(VPatch.VNODE,a,b));applyClear=true}}else{apply=appendPatch(apply,new VPatch(VPatch.VNODE,a,b));applyClear=true}}else if(isVText(b)){if(!isVText(a)){apply=appendPatch(apply,new VPatch(VPatch.VTEXT,a,b));applyClear=true}else if(a.text!==b.text){apply=appendPatch(apply,new VPatch(VPatch.VTEXT,a,b))}}else if(isWidget(b)){if(!isWidget(a)){applyClear=true}apply=appendPatch(apply,new VPatch(VPatch.WIDGET,a,b))}if(apply){patch[index]=apply}if(applyClear){clearState(a,patch,index)}}function diffChildren(a,b,patch,apply,index){var aChildren=a.children;var orderedSet=reorder(aChildren,b.children);var bChildren=orderedSet.children;var aLen=aChildren.length;var bLen=bChildren.length;var len=aLen>bLen?aLen:bLen;for(var i=0;i<len;i++){var leftNode=aChildren[i];var rightNode=bChildren[i];index+=1;if(!leftNode){if(rightNode){apply=appendPatch(apply,new VPatch(VPatch.INSERT,null,rightNode))}}else{walk(leftNode,rightNode,patch,index)}if(isVNode(leftNode)&&leftNode.count){index+=leftNode.count}}if(orderedSet.moves){apply=appendPatch(apply,new VPatch(VPatch.ORDER,a,orderedSet.moves))}return apply}function clearState(vNode,patch,index){unhook(vNode,patch,index);destroyWidgets(vNode,patch,index)}function destroyWidgets(vNode,patch,index){if(isWidget(vNode)){if(typeof vNode.destroy==="function"){patch[index]=appendPatch(patch[index],new VPatch(VPatch.REMOVE,vNode,null))}}else if(isVNode(vNode)&&(vNode.hasWidgets||vNode.hasThunks)){var children=vNode.children;var len=children.length;for(var i=0;i<len;i++){var child=children[i];index+=1;destroyWidgets(child,patch,index);if(isVNode(child)&&child.count){index+=child.count}}}else if(isThunk(vNode)){thunks(vNode,null,patch,index)}}function thunks(a,b,patch,index){var nodes=handleThunk(a,b);var thunkPatch=diff(nodes.a,nodes.b);if(hasPatches(thunkPatch)){patch[index]=new VPatch(VPatch.THUNK,null,thunkPatch)}}function hasPatches(patch){for(var index in patch){if(index!=="a"){return true}}return false}function unhook(vNode,patch,index){if(isVNode(vNode)){if(vNode.hooks){patch[index]=appendPatch(patch[index],new VPatch(VPatch.PROPS,vNode,undefinedKeys(vNode.hooks)))}if(vNode.descendantHooks||vNode.hasThunks){var children=vNode.children;var len=children.length;for(var i=0;i<len;i++){var child=children[i];index+=1;unhook(child,patch,index);if(isVNode(child)&&child.count){index+=child.count}}}}else if(isThunk(vNode)){thunks(vNode,null,patch,index)}}function undefinedKeys(obj){var result={};for(var key in obj){result[key]=undefined}return result}function reorder(aChildren,bChildren){var bChildIndex=keyIndex(bChildren);var bKeys=bChildIndex.keys;var bFree=bChildIndex.free;if(bFree.length===bChildren.length){return{children:bChildren,moves:null}}var aChildIndex=keyIndex(aChildren);var aKeys=aChildIndex.keys;var aFree=aChildIndex.free;if(aFree.length===aChildren.length){return{children:bChildren,moves:null}}var newChildren=[];var freeIndex=0;var freeCount=bFree.length;var deletedItems=0;for(var i=0;i<aChildren.length;i++){var aItem=aChildren[i];var itemIndex;if(aItem.key){if(bKeys.hasOwnProperty(aItem.key)){itemIndex=bKeys[aItem.key];newChildren.push(bChildren[itemIndex])}else{itemIndex=i-deletedItems++;newChildren.push(null)}}else{if(freeIndex<freeCount){itemIndex=bFree[freeIndex++];newChildren.push(bChildren[itemIndex])}else{itemIndex=i-deletedItems++;newChildren.push(null)}}}var lastFreeIndex=freeIndex>=bFree.length?bChildren.length:bFree[freeIndex];for(var j=0;j<bChildren.length;j++){var newItem=bChildren[j];if(newItem.key){if(!aKeys.hasOwnProperty(newItem.key)){newChildren.push(newItem)}}else if(j>=lastFreeIndex){newChildren.push(newItem)}}var simulate=newChildren.slice();var simulateIndex=0;var removes=[];var inserts=[];var simulateItem;for(var k=0;k<bChildren.length;){var wantedItem=bChildren[k];simulateItem=simulate[simulateIndex];while(simulateItem===null&&simulate.length){removes.push(remove(simulate,simulateIndex,null));simulateItem=simulate[simulateIndex]}if(!simulateItem||simulateItem.key!==wantedItem.key){if(wantedItem.key){if(simulateItem&&simulateItem.key){if(bKeys[simulateItem.key]!==k+1){removes.push(remove(simulate,simulateIndex,simulateItem.key));simulateItem=simulate[simulateIndex];if(!simulateItem||simulateItem.key!==wantedItem.key){inserts.push({key:wantedItem.key,to:k})}else{simulateIndex++}}else{inserts.push({key:wantedItem.key,to:k})}}else{inserts.push({key:wantedItem.key,to:k})}k++}else if(simulateItem&&simulateItem.key){removes.push(remove(simulate,simulateIndex,simulateItem.key))}}else{simulateIndex++;k++}}while(simulateIndex<simulate.length){simulateItem=simulate[simulateIndex];removes.push(remove(simulate,simulateIndex,simulateItem&&simulateItem.key))}if(removes.length===deletedItems&&!inserts.length){return{children:newChildren,moves:null}}return{children:newChildren,moves:{removes:removes,inserts:inserts}}}function remove(arr,index,key){arr.splice(index,1);return{from:index,key:key}}function keyIndex(children){var keys={};var free=[];var length=children.length;for(var i=0;i<length;i++){var child=children[i];if(child.key){keys[child.key]=i}else{free.push(i)}}return{keys:keys,free:free}}function appendPatch(apply,patch){if(apply){if(isArray(apply)){apply.push(patch)}else{apply=[apply,patch]}return apply}else{return patch}}},{"../vnode/handle-thunk":23,"../vnode/is-thunk":24,"../vnode/is-vnode":26,"../vnode/is-vtext":27,"../vnode/is-widget":28,"../vnode/vpatch":31,"./diff-props":33,"x-is-array":11}],"virtual-dom":[function(require,module,exports){var diff=require("./diff.js");var patch=require("./patch.js");var h=require("./h.js");var create=require("./create-element.js");var VNode=require("./vnode/vnode.js");var VText=require("./vnode/vtext.js");module.exports={diff:diff,patch:patch,h:h,create:create,VNode:VNode,VText:VText}},{"./create-element.js":2,"./diff.js":3,"./h.js":4,"./patch.js":12,"./vnode/vnode.js":30,"./vnode/vtext.js":32}]},{},[]);require=function e(t,n,r){function s(o,u){if(!n[o]){if(!t[o]){var a=typeof require=="function"&&require;if(!u&&a)return a(o,!0);if(i)return i(o,!0);var f=new Error("Cannot find module '"+o+"'");throw f.code="MODULE_NOT_FOUND",f}var l=n[o]={exports:{}};t[o][0].call(l.exports,function(e){var n=t[o][1][e];return s(n?n:e)},l,l.exports,e,t,n,r)}return n[o].exports}var i=typeof require=="function"&&require;for(var o=0;o<r.length;o++)s(r[o]);return s}({1:[function(require,module,exports){},{}],2:[function(require,module,exports){var createElement=require("./vdom/create-element.js");module.exports=createElement},{"./vdom/create-element.js":14}],3:[function(require,module,exports){var diff=require("./vtree/diff.js");module.exports=diff},{"./vtree/diff.js":34}],4:[function(require,module,exports){var h=require("./virtual-hyperscript/index.js");module.exports=h},{"./virtual-hyperscript/index.js":21}],5:[function(require,module,exports){module.exports=function split(undef){var nativeSplit=String.prototype.split,compliantExecNpcg=/()??/.exec("")[1]===undef,self;self=function(str,separator,limit){if(Object.prototype.toString.call(separator)!=="[object RegExp]"){return nativeSplit.call(str,separator,limit)}var output=[],flags=(separator.ignoreCase?"i":"")+(separator.multiline?"m":"")+(separator.extended?"x":"")+(separator.sticky?"y":""),lastLastIndex=0,separator=new RegExp(separator.source,flags+"g"),separator2,match,lastIndex,lastLength;str+="";if(!compliantExecNpcg){separator2=new RegExp("^"+separator.source+"$(?!\\s)",flags)}limit=limit===undef?-1>>>0:limit>>>0;while(match=separator.exec(str)){lastIndex=match.index+match[0].length;if(lastIndex>lastLastIndex){output.push(str.slice(lastLastIndex,match.index));if(!compliantExecNpcg&&match.length>1){match[0].replace(separator2,function(){for(var i=1;i<arguments.length-2;i++){if(arguments[i]===undef){match[i]=undef}}})}if(match.length>1&&match.index<str.length){Array.prototype.push.apply(output,match.slice(1))}lastLength=match[0].length;lastLastIndex=lastIndex;if(output.length>=limit){break}}if(separator.lastIndex===match.index){separator.lastIndex++}}if(lastLastIndex===str.length){if(lastLength||!separator.test("")){output.push("")}}else{output.push(str.slice(lastLastIndex))}return output.length>limit?output.slice(0,limit):output};return self}()},{}],6:[function(require,module,exports){"use strict";var OneVersionConstraint=require("individual/one-version");var MY_VERSION="7";OneVersionConstraint("ev-store",MY_VERSION);var hashKey="__EV_STORE_KEY@"+MY_VERSION;module.exports=EvStore;function EvStore(elem){var hash=elem[hashKey];if(!hash){hash=elem[hashKey]={}}return hash}},{"individual/one-version":8}],7:[function(require,module,exports){(function(global){"use strict";var root=typeof window!=="undefined"?window:typeof global!=="undefined"?global:{};module.exports=Individual;function Individual(key,value){if(key in root){return root[key]}root[key]=value;return value}}).call(this,typeof global!=="undefined"?global:typeof self!=="undefined"?self:typeof window!=="undefined"?window:{})},{}],8:[function(require,module,exports){"use strict";var Individual=require("./index.js");module.exports=OneVersion;function OneVersion(moduleName,version,defaultValue){var key="__INDIVIDUAL_ONE_VERSION_"+moduleName;var enforceKey=key+"_ENFORCE_SINGLETON";var versionValue=Individual(enforceKey,version);if(versionValue!==version){throw new Error("Can only have one copy of "+moduleName+".\n"+"You already have version "+versionValue+" installed.\n"+"This means you cannot install version "+version); | |
}return Individual(key,defaultValue)}},{"./index.js":7}],9:[function(require,module,exports){(function(global){var topLevel=typeof global!=="undefined"?global:typeof window!=="undefined"?window:{};var minDoc=require("min-document");if(typeof document!=="undefined"){module.exports=document}else{var doccy=topLevel["__GLOBAL_DOCUMENT_CACHE@4"];if(!doccy){doccy=topLevel["__GLOBAL_DOCUMENT_CACHE@4"]=minDoc}module.exports=doccy}}).call(this,typeof global!=="undefined"?global:typeof self!=="undefined"?self:typeof window!=="undefined"?window:{})},{"min-document":1}],10:[function(require,module,exports){"use strict";module.exports=function isObject(x){return typeof x==="object"&&x!==null}},{}],11:[function(require,module,exports){var nativeIsArray=Array.isArray;var toString=Object.prototype.toString;module.exports=nativeIsArray||isArray;function isArray(obj){return toString.call(obj)==="[object Array]"}},{}],12:[function(require,module,exports){var patch=require("./vdom/patch.js");module.exports=patch},{"./vdom/patch.js":17}],13:[function(require,module,exports){var isObject=require("is-object");var isHook=require("../vnode/is-vhook.js");module.exports=applyProperties;function applyProperties(node,props,previous){for(var propName in props){var propValue=props[propName];if(propValue===undefined){removeProperty(node,propName,propValue,previous)}else if(isHook(propValue)){removeProperty(node,propName,propValue,previous);if(propValue.hook){propValue.hook(node,propName,previous?previous[propName]:undefined)}}else{if(isObject(propValue)){patchObject(node,props,previous,propName,propValue)}else{node[propName]=propValue}}}}function removeProperty(node,propName,propValue,previous){if(previous){var previousValue=previous[propName];if(!isHook(previousValue)){if(propName==="attributes"){for(var attrName in previousValue){node.removeAttribute(attrName)}}else if(propName==="style"){for(var i in previousValue){node.style[i]=""}}else if(typeof previousValue==="string"){node[propName]=""}else{node[propName]=null}}else if(previousValue.unhook){previousValue.unhook(node,propName,propValue)}}}function patchObject(node,props,previous,propName,propValue){var previousValue=previous?previous[propName]:undefined;if(propName==="attributes"){for(var attrName in propValue){var attrValue=propValue[attrName];if(attrValue===undefined){node.removeAttribute(attrName)}else{node.setAttribute(attrName,attrValue)}}return}if(previousValue&&isObject(previousValue)&&getPrototype(previousValue)!==getPrototype(propValue)){node[propName]=propValue;return}if(!isObject(node[propName])){node[propName]={}}var replacer=propName==="style"?"":undefined;for(var k in propValue){var value=propValue[k];node[propName][k]=value===undefined?replacer:value}}function getPrototype(value){if(Object.getPrototypeOf){return Object.getPrototypeOf(value)}else if(value.__proto__){return value.__proto__}else if(value.constructor){return value.constructor.prototype}}},{"../vnode/is-vhook.js":25,"is-object":10}],14:[function(require,module,exports){var document=require("global/document");var applyProperties=require("./apply-properties");var isVNode=require("../vnode/is-vnode.js");var isVText=require("../vnode/is-vtext.js");var isWidget=require("../vnode/is-widget.js");var handleThunk=require("../vnode/handle-thunk.js");module.exports=createElement;function createElement(vnode,opts){var doc=opts?opts.document||document:document;var warn=opts?opts.warn:null;vnode=handleThunk(vnode).a;if(isWidget(vnode)){return vnode.init()}else if(isVText(vnode)){return doc.createTextNode(vnode.text)}else if(!isVNode(vnode)){if(warn){warn("Item is not a valid virtual dom node",vnode)}return null}var node=vnode.namespace===null?doc.createElement(vnode.tagName):doc.createElementNS(vnode.namespace,vnode.tagName);var props=vnode.properties;applyProperties(node,props);var children=vnode.children;for(var i=0;i<children.length;i++){var childNode=createElement(children[i],opts);if(childNode){node.appendChild(childNode)}}return node}},{"../vnode/handle-thunk.js":23,"../vnode/is-vnode.js":26,"../vnode/is-vtext.js":27,"../vnode/is-widget.js":28,"./apply-properties":13,"global/document":9}],15:[function(require,module,exports){var noChild={};module.exports=domIndex;function domIndex(rootNode,tree,indices,nodes){if(!indices||indices.length===0){return{}}else{indices.sort(ascending);return recurse(rootNode,tree,indices,nodes,0)}}function recurse(rootNode,tree,indices,nodes,rootIndex){nodes=nodes||{};if(rootNode){if(indexInRange(indices,rootIndex,rootIndex)){nodes[rootIndex]=rootNode}var vChildren=tree.children;if(vChildren){var childNodes=rootNode.childNodes;for(var i=0;i<tree.children.length;i++){rootIndex+=1;var vChild=vChildren[i]||noChild;var nextIndex=rootIndex+(vChild.count||0);if(indexInRange(indices,rootIndex,nextIndex)){recurse(childNodes[i],vChild,indices,nodes,rootIndex)}rootIndex=nextIndex}}}return nodes}function indexInRange(indices,left,right){if(indices.length===0){return false}var minIndex=0;var maxIndex=indices.length-1;var currentIndex;var currentItem;while(minIndex<=maxIndex){currentIndex=(maxIndex+minIndex)/2>>0;currentItem=indices[currentIndex];if(minIndex===maxIndex){return currentItem>=left&¤tItem<=right}else if(currentItem<left){minIndex=currentIndex+1}else if(currentItem>right){maxIndex=currentIndex-1}else{return true}}return false}function ascending(a,b){return a>b?1:-1}},{}],16:[function(require,module,exports){var applyProperties=require("./apply-properties");var isWidget=require("../vnode/is-widget.js");var VPatch=require("../vnode/vpatch.js");var updateWidget=require("./update-widget");module.exports=applyPatch;function applyPatch(vpatch,domNode,renderOptions){var type=vpatch.type;var vNode=vpatch.vNode;var patch=vpatch.patch;switch(type){case VPatch.REMOVE:return removeNode(domNode,vNode);case VPatch.INSERT:return insertNode(domNode,patch,renderOptions);case VPatch.VTEXT:return stringPatch(domNode,vNode,patch,renderOptions);case VPatch.WIDGET:return widgetPatch(domNode,vNode,patch,renderOptions);case VPatch.VNODE:return vNodePatch(domNode,vNode,patch,renderOptions);case VPatch.ORDER:reorderChildren(domNode,patch);return domNode;case VPatch.PROPS:applyProperties(domNode,patch,vNode.properties);return domNode;case VPatch.THUNK:return replaceRoot(domNode,renderOptions.patch(domNode,patch,renderOptions));default:return domNode}}function removeNode(domNode,vNode){var parentNode=domNode.parentNode;if(parentNode){parentNode.removeChild(domNode)}destroyWidget(domNode,vNode);return null}function insertNode(parentNode,vNode,renderOptions){var newNode=renderOptions.render(vNode,renderOptions);if(parentNode){parentNode.appendChild(newNode)}return parentNode}function stringPatch(domNode,leftVNode,vText,renderOptions){var newNode;if(domNode.nodeType===3){domNode.replaceData(0,domNode.length,vText.text);newNode=domNode}else{var parentNode=domNode.parentNode;newNode=renderOptions.render(vText,renderOptions);if(parentNode&&newNode!==domNode){parentNode.replaceChild(newNode,domNode)}}return newNode}function widgetPatch(domNode,leftVNode,widget,renderOptions){var updating=updateWidget(leftVNode,widget);var newNode;if(updating){newNode=widget.update(leftVNode,domNode)||domNode}else{newNode=renderOptions.render(widget,renderOptions)}var parentNode=domNode.parentNode;if(parentNode&&newNode!==domNode){parentNode.replaceChild(newNode,domNode)}if(!updating){destroyWidget(domNode,leftVNode)}return newNode}function vNodePatch(domNode,leftVNode,vNode,renderOptions){var parentNode=domNode.parentNode;var newNode=renderOptions.render(vNode,renderOptions);if(parentNode&&newNode!==domNode){parentNode.replaceChild(newNode,domNode)}return newNode}function destroyWidget(domNode,w){if(typeof w.destroy==="function"&&isWidget(w)){w.destroy(domNode)}}function reorderChildren(domNode,moves){var childNodes=domNode.childNodes;var keyMap={};var node;var remove;var insert;for(var i=0;i<moves.removes.length;i++){remove=moves.removes[i];node=childNodes[remove.from];if(remove.key){keyMap[remove.key]=node}domNode.removeChild(node)}var length=childNodes.length;for(var j=0;j<moves.inserts.length;j++){insert=moves.inserts[j];node=keyMap[insert.key];domNode.insertBefore(node,insert.to>=length++?null:childNodes[insert.to])}}function replaceRoot(oldRoot,newRoot){if(oldRoot&&newRoot&&oldRoot!==newRoot&&oldRoot.parentNode){oldRoot.parentNode.replaceChild(newRoot,oldRoot)}return newRoot}},{"../vnode/is-widget.js":28,"../vnode/vpatch.js":31,"./apply-properties":13,"./update-widget":18}],17:[function(require,module,exports){var document=require("global/document");var isArray=require("x-is-array");var render=require("./create-element");var domIndex=require("./dom-index");var patchOp=require("./patch-op");module.exports=patch;function patch(rootNode,patches,renderOptions){renderOptions=renderOptions||{};renderOptions.patch=renderOptions.patch&&renderOptions.patch!==patch?renderOptions.patch:patchRecursive;renderOptions.render=renderOptions.render||render;return renderOptions.patch(rootNode,patches,renderOptions)}function patchRecursive(rootNode,patches,renderOptions){var indices=patchIndices(patches);if(indices.length===0){return rootNode}var index=domIndex(rootNode,patches.a,indices);var ownerDocument=rootNode.ownerDocument;if(!renderOptions.document&&ownerDocument!==document){renderOptions.document=ownerDocument}for(var i=0;i<indices.length;i++){var nodeIndex=indices[i];rootNode=applyPatch(rootNode,index[nodeIndex],patches[nodeIndex],renderOptions)}return rootNode}function applyPatch(rootNode,domNode,patchList,renderOptions){if(!domNode){return rootNode}var newNode;if(isArray(patchList)){for(var i=0;i<patchList.length;i++){newNode=patchOp(patchList[i],domNode,renderOptions);if(domNode===rootNode){rootNode=newNode}}}else{newNode=patchOp(patchList,domNode,renderOptions);if(domNode===rootNode){rootNode=newNode}}return rootNode}function patchIndices(patches){var indices=[];for(var key in patches){if(key!=="a"){indices.push(Number(key))}}return indices}},{"./create-element":14,"./dom-index":15,"./patch-op":16,"global/document":9,"x-is-array":11}],18:[function(require,module,exports){var isWidget=require("../vnode/is-widget.js");module.exports=updateWidget;function updateWidget(a,b){if(isWidget(a)&&isWidget(b)){if("name"in a&&"name"in b){return a.id===b.id}else{return a.init===b.init}}return false}},{"../vnode/is-widget.js":28}],19:[function(require,module,exports){"use strict";var EvStore=require("ev-store");module.exports=EvHook;function EvHook(value){if(!(this instanceof EvHook)){return new EvHook(value)}this.value=value}EvHook.prototype.hook=function(node,propertyName){var es=EvStore(node);var propName=propertyName.substr(3);es[propName]=this.value};EvHook.prototype.unhook=function(node,propertyName){var es=EvStore(node);var propName=propertyName.substr(3);es[propName]=undefined}},{"ev-store":6}],20:[function(require,module,exports){"use strict";module.exports=SoftSetHook;function SoftSetHook(value){if(!(this instanceof SoftSetHook)){return new SoftSetHook(value)}this.value=value}SoftSetHook.prototype.hook=function(node,propertyName){if(node[propertyName]!==this.value){node[propertyName]=this.value}}},{}],21:[function(require,module,exports){"use strict";var isArray=require("x-is-array");var VNode=require("../vnode/vnode.js");var VText=require("../vnode/vtext.js");var isVNode=require("../vnode/is-vnode");var isVText=require("../vnode/is-vtext");var isWidget=require("../vnode/is-widget");var isHook=require("../vnode/is-vhook");var isVThunk=require("../vnode/is-thunk");var parseTag=require("./parse-tag.js");var softSetHook=require("./hooks/soft-set-hook.js");var evHook=require("./hooks/ev-hook.js");module.exports=h;function h(tagName,properties,children){var childNodes=[];var tag,props,key,namespace;if(!children&&isChildren(properties)){children=properties;props={}}props=props||properties||{};tag=parseTag(tagName,props);if(props.hasOwnProperty("key")){key=props.key;props.key=undefined}if(props.hasOwnProperty("namespace")){namespace=props.namespace;props.namespace=undefined}if(tag==="INPUT"&&!namespace&&props.hasOwnProperty("value")&&props.value!==undefined&&!isHook(props.value)){props.value=softSetHook(props.value)}transformProperties(props);if(children!==undefined&&children!==null){addChild(children,childNodes,tag,props)}return new VNode(tag,props,childNodes,key,namespace)}function addChild(c,childNodes,tag,props){if(typeof c==="string"){childNodes.push(new VText(c))}else if(typeof c==="number"){childNodes.push(new VText(String(c)))}else if(isChild(c)){childNodes.push(c)}else if(isArray(c)){for(var i=0;i<c.length;i++){addChild(c[i],childNodes,tag,props)}}else if(c===null||c===undefined){return}else{throw UnexpectedVirtualElement({foreignObject:c,parentVnode:{tagName:tag,properties:props}})}}function transformProperties(props){for(var propName in props){if(props.hasOwnProperty(propName)){var value=props[propName];if(isHook(value)){continue}if(propName.substr(0,3)==="ev-"){props[propName]=evHook(value)}}}}function isChild(x){return isVNode(x)||isVText(x)||isWidget(x)||isVThunk(x)}function isChildren(x){return typeof x==="string"||isArray(x)||isChild(x)}function UnexpectedVirtualElement(data){var err=new Error;err.type="virtual-hyperscript.unexpected.virtual-element";err.message="Unexpected virtual child passed to h().\n"+"Expected a VNode / Vthunk / VWidget / string but:\n"+"got:\n"+errorString(data.foreignObject)+".\n"+"The parent vnode is:\n"+errorString(data.parentVnode);"\n"+"Suggested fix: change your `h(..., [ ... ])` callsite.";err.foreignObject=data.foreignObject;err.parentVnode=data.parentVnode;return err}function errorString(obj){try{return JSON.stringify(obj,null," ")}catch(e){return String(obj)}}},{"../vnode/is-thunk":24,"../vnode/is-vhook":25,"../vnode/is-vnode":26,"../vnode/is-vtext":27,"../vnode/is-widget":28,"../vnode/vnode.js":30,"../vnode/vtext.js":32,"./hooks/ev-hook.js":19,"./hooks/soft-set-hook.js":20,"./parse-tag.js":22,"x-is-array":11}],22:[function(require,module,exports){"use strict";var split=require("browser-split");var classIdSplit=/([\.#]?[a-zA-Z0-9\u007F-\uFFFF_:-]+)/;var notClassId=/^\.|#/;module.exports=parseTag;function parseTag(tag,props){if(!tag){return"DIV"}var noId=!props.hasOwnProperty("id");var tagParts=split(tag,classIdSplit);var tagName=null;if(notClassId.test(tagParts[1])){tagName="DIV"}var classes,part,type,i;for(i=0;i<tagParts.length;i++){part=tagParts[i];if(!part){continue}type=part.charAt(0);if(!tagName){tagName=part}else if(type==="."){classes=classes||[];classes.push(part.substring(1,part.length))}else if(type==="#"&&noId){props.id=part.substring(1,part.length)}}if(classes){if(props.className){classes.push(props.className)}props.className=classes.join(" ")}return props.namespace?tagName:tagName.toUpperCase()}},{"browser-split":5}],23:[function(require,module,exports){var isVNode=require("./is-vnode");var isVText=require("./is-vtext");var isWidget=require("./is-widget");var isThunk=require("./is-thunk");module.exports=handleThunk;function handleThunk(a,b){var renderedA=a;var renderedB=b;if(isThunk(b)){renderedB=renderThunk(b,a)}if(isThunk(a)){renderedA=renderThunk(a,null)}return{a:renderedA,b:renderedB}}function renderThunk(thunk,previous){var renderedThunk=thunk.vnode;if(!renderedThunk){renderedThunk=thunk.vnode=thunk.render(previous)}if(!(isVNode(renderedThunk)||isVText(renderedThunk)||isWidget(renderedThunk))){throw new Error("thunk did not return a valid node")}return renderedThunk}},{"./is-thunk":24,"./is-vnode":26,"./is-vtext":27,"./is-widget":28}],24:[function(require,module,exports){module.exports=isThunk;function isThunk(t){return t&&t.type==="Thunk"}},{}],25:[function(require,module,exports){module.exports=isHook;function isHook(hook){return hook&&(typeof hook.hook==="function"&&!hook.hasOwnProperty("hook")||typeof hook.unhook==="function"&&!hook.hasOwnProperty("unhook"))}},{}],26:[function(require,module,exports){var version=require("./version");module.exports=isVirtualNode;function isVirtualNode(x){return x&&x.type==="VirtualNode"&&x.version===version}},{"./version":29}],27:[function(require,module,exports){var version=require("./version");module.exports=isVirtualText;function isVirtualText(x){return x&&x.type==="VirtualText"&&x.version===version}},{"./version":29}],28:[function(require,module,exports){module.exports=isWidget;function isWidget(w){return w&&w.type==="Widget"}},{}],29:[function(require,module,exports){module.exports="2"},{}],30:[function(require,module,exports){var version=require("./version");var isVNode=require("./is-vnode");var isWidget=require("./is-widget");var isThunk=require("./is-thunk");var isVHook=require("./is-vhook");module.exports=VirtualNode;var noProperties={};var noChildren=[];function VirtualNode(tagName,properties,children,key,namespace){this.tagName=tagName;this.properties=properties||noProperties;this.children=children||noChildren;this.key=key!=null?String(key):undefined;this.namespace=typeof namespace==="string"?namespace:null;var count=children&&children.length||0;var descendants=0;var hasWidgets=false;var hasThunks=false;var descendantHooks=false;var hooks;for(var propName in properties){if(properties.hasOwnProperty(propName)){var property=properties[propName];if(isVHook(property)&&property.unhook){if(!hooks){hooks={}}hooks[propName]=property}}}for(var i=0;i<count;i++){var child=children[i];if(isVNode(child)){descendants+=child.count||0;if(!hasWidgets&&child.hasWidgets){hasWidgets=true}if(!hasThunks&&child.hasThunks){hasThunks=true}if(!descendantHooks&&(child.hooks||child.descendantHooks)){descendantHooks=true}}else if(!hasWidgets&&isWidget(child)){if(typeof child.destroy==="function"){hasWidgets=true}}else if(!hasThunks&&isThunk(child)){hasThunks=true}}this.count=count+descendants;this.hasWidgets=hasWidgets;this.hasThunks=hasThunks;this.hooks=hooks;this.descendantHooks=descendantHooks}VirtualNode.prototype.version=version;VirtualNode.prototype.type="VirtualNode"},{"./is-thunk":24,"./is-vhook":25,"./is-vnode":26,"./is-widget":28,"./version":29}],31:[function(require,module,exports){var version=require("./version");VirtualPatch.NONE=0;VirtualPatch.VTEXT=1;VirtualPatch.VNODE=2;VirtualPatch.WIDGET=3;VirtualPatch.PROPS=4;VirtualPatch.ORDER=5;VirtualPatch.INSERT=6;VirtualPatch.REMOVE=7;VirtualPatch.THUNK=8;module.exports=VirtualPatch;function VirtualPatch(type,vNode,patch){this.type=Number(type);this.vNode=vNode;this.patch=patch}VirtualPatch.prototype.version=version;VirtualPatch.prototype.type="VirtualPatch"},{"./version":29}],32:[function(require,module,exports){var version=require("./version");module.exports=VirtualText;function VirtualText(text){this.text=String(text)}VirtualText.prototype.version=version;VirtualText.prototype.type="VirtualText"},{"./version":29}],33:[function(require,module,exports){var isObject=require("is-object");var isHook=require("../vnode/is-vhook");module.exports=diffProps;function diffProps(a,b){var diff;for(var aKey in a){if(!(aKey in b)){diff=diff||{};diff[aKey]=undefined}var aValue=a[aKey];var bValue=b[aKey];if(aValue===bValue){continue}else if(isObject(aValue)&&isObject(bValue)){if(getPrototype(bValue)!==getPrototype(aValue)){diff=diff||{};diff[aKey]=bValue}else if(isHook(bValue)){diff=diff||{};diff[aKey]=bValue}else{var objectDiff=diffProps(aValue,bValue);if(objectDiff){diff=diff||{};diff[aKey]=objectDiff}}}else{diff=diff||{};diff[aKey]=bValue}}for(var bKey in b){if(!(bKey in a)){diff=diff||{};diff[bKey]=b[bKey]}}return diff}function getPrototype(value){if(Object.getPrototypeOf){return Object.getPrototypeOf(value)}else if(value.__proto__){return value.__proto__}else if(value.constructor){return value.constructor.prototype}}},{"../vnode/is-vhook":25,"is-object":10}],34:[function(require,module,exports){var isArray=require("x-is-array");var VPatch=require("../vnode/vpatch");var isVNode=require("../vnode/is-vnode");var isVText=require("../vnode/is-vtext");var isWidget=require("../vnode/is-widget");var isThunk=require("../vnode/is-thunk");var handleThunk=require("../vnode/handle-thunk");var diffProps=require("./diff-props");module.exports=diff;function diff(a,b){var patch={a:a};walk(a,b,patch,0);return patch}function walk(a,b,patch,index){if(a===b){return}var apply=patch[index];var applyClear=false;if(isThunk(a)||isThunk(b)){thunks(a,b,patch,index)}else if(b==null){if(!isWidget(a)){clearState(a,patch,index);apply=patch[index]}apply=appendPatch(apply,new VPatch(VPatch.REMOVE,a,b))}else if(isVNode(b)){if(isVNode(a)){if(a.tagName===b.tagName&&a.namespace===b.namespace&&a.key===b.key){var propsPatch=diffProps(a.properties,b.properties);if(propsPatch){apply=appendPatch(apply,new VPatch(VPatch.PROPS,a,propsPatch))}apply=diffChildren(a,b,patch,apply,index)}else{apply=appendPatch(apply,new VPatch(VPatch.VNODE,a,b));applyClear=true}}else{apply=appendPatch(apply,new VPatch(VPatch.VNODE,a,b));applyClear=true}}else if(isVText(b)){if(!isVText(a)){apply=appendPatch(apply,new VPatch(VPatch.VTEXT,a,b));applyClear=true}else if(a.text!==b.text){apply=appendPatch(apply,new VPatch(VPatch.VTEXT,a,b))}}else if(isWidget(b)){if(!isWidget(a)){applyClear=true}apply=appendPatch(apply,new VPatch(VPatch.WIDGET,a,b))}if(apply){patch[index]=apply}if(applyClear){clearState(a,patch,index)}}function diffChildren(a,b,patch,apply,index){var aChildren=a.children;var orderedSet=reorder(aChildren,b.children);var bChildren=orderedSet.children;var aLen=aChildren.length;var bLen=bChildren.length;var len=aLen>bLen?aLen:bLen;for(var i=0;i<len;i++){var leftNode=aChildren[i];var rightNode=bChildren[i];index+=1;if(!leftNode){if(rightNode){apply=appendPatch(apply,new VPatch(VPatch.INSERT,null,rightNode))}}else{walk(leftNode,rightNode,patch,index)}if(isVNode(leftNode)&&leftNode.count){index+=leftNode.count}}if(orderedSet.moves){apply=appendPatch(apply,new VPatch(VPatch.ORDER,a,orderedSet.moves))}return apply}function clearState(vNode,patch,index){unhook(vNode,patch,index);destroyWidgets(vNode,patch,index)}function destroyWidgets(vNode,patch,index){if(isWidget(vNode)){if(typeof vNode.destroy==="function"){patch[index]=appendPatch(patch[index],new VPatch(VPatch.REMOVE,vNode,null))}}else if(isVNode(vNode)&&(vNode.hasWidgets||vNode.hasThunks)){var children=vNode.children;var len=children.length;for(var i=0;i<len;i++){var child=children[i];index+=1;destroyWidgets(child,patch,index);if(isVNode(child)&&child.count){index+=child.count}}}else if(isThunk(vNode)){thunks(vNode,null,patch,index)}}function thunks(a,b,patch,index){var nodes=handleThunk(a,b);var thunkPatch=diff(nodes.a,nodes.b);if(hasPatches(thunkPatch)){patch[index]=new VPatch(VPatch.THUNK,null,thunkPatch)}}function hasPatches(patch){for(var index in patch){if(index!=="a"){return true}}return false}function unhook(vNode,patch,index){if(isVNode(vNode)){if(vNode.hooks){patch[index]=appendPatch(patch[index],new VPatch(VPatch.PROPS,vNode,undefinedKeys(vNode.hooks)))}if(vNode.descendantHooks||vNode.hasThunks){var children=vNode.children;var len=children.length;for(var i=0;i<len;i++){var child=children[i];index+=1;unhook(child,patch,index);if(isVNode(child)&&child.count){index+=child.count}}}}else if(isThunk(vNode)){thunks(vNode,null,patch,index)}}function undefinedKeys(obj){var result={};for(var key in obj){result[key]=undefined}return result}function reorder(aChildren,bChildren){var bChildIndex=keyIndex(bChildren);var bKeys=bChildIndex.keys;var bFree=bChildIndex.free;if(bFree.length===bChildren.length){return{children:bChildren,moves:null}}var aChildIndex=keyIndex(aChildren);var aKeys=aChildIndex.keys;var aFree=aChildIndex.free;if(aFree.length===aChildren.length){return{children:bChildren,moves:null}}var newChildren=[];var freeIndex=0;var freeCount=bFree.length;var deletedItems=0;for(var i=0;i<aChildren.length;i++){var aItem=aChildren[i];var itemIndex;if(aItem.key){if(bKeys.hasOwnProperty(aItem.key)){itemIndex=bKeys[aItem.key];newChildren.push(bChildren[itemIndex])}else{itemIndex=i-deletedItems++;newChildren.push(null)}}else{if(freeIndex<freeCount){itemIndex=bFree[freeIndex++];newChildren.push(bChildren[itemIndex])}else{itemIndex=i-deletedItems++;newChildren.push(null)}}}var lastFreeIndex=freeIndex>=bFree.length?bChildren.length:bFree[freeIndex];for(var j=0;j<bChildren.length;j++){var newItem=bChildren[j];if(newItem.key){if(!aKeys.hasOwnProperty(newItem.key)){newChildren.push(newItem)}}else if(j>=lastFreeIndex){newChildren.push(newItem)}}var simulate=newChildren.slice();var simulateIndex=0;var removes=[];var inserts=[];var simulateItem;for(var k=0;k<bChildren.length;){var wantedItem=bChildren[k];simulateItem=simulate[simulateIndex];while(simulateItem===null&&simulate.length){removes.push(remove(simulate,simulateIndex,null));simulateItem=simulate[simulateIndex]}if(!simulateItem||simulateItem.key!==wantedItem.key){if(wantedItem.key){if(simulateItem&&simulateItem.key){if(bKeys[simulateItem.key]!==k+1){removes.push(remove(simulate,simulateIndex,simulateItem.key));simulateItem=simulate[simulateIndex];if(!simulateItem||simulateItem.key!==wantedItem.key){inserts.push({key:wantedItem.key,to:k})}else{simulateIndex++}}else{inserts.push({key:wantedItem.key,to:k})}}else{inserts.push({key:wantedItem.key,to:k})}k++}else if(simulateItem&&simulateItem.key){removes.push(remove(simulate,simulateIndex,simulateItem.key))}}else{simulateIndex++;k++}}while(simulateIndex<simulate.length){simulateItem=simulate[simulateIndex];removes.push(remove(simulate,simulateIndex,simulateItem&&simulateItem.key))}if(removes.length===deletedItems&&!inserts.length){return{children:newChildren,moves:null}}return{children:newChildren,moves:{removes:removes,inserts:inserts}}}function remove(arr,index,key){arr.splice(index,1);return{from:index,key:key}}function keyIndex(children){var keys={};var free=[];var length=children.length;for(var i=0;i<length;i++){var child=children[i];if(child.key){keys[child.key]=i}else{free.push(i)}}return{keys:keys,free:free}}function appendPatch(apply,patch){if(apply){if(isArray(apply)){apply.push(patch)}else{apply=[apply,patch]}return apply}else{return patch}}},{"../vnode/handle-thunk":23,"../vnode/is-thunk":24,"../vnode/is-vnode":26,"../vnode/is-vtext":27,"../vnode/is-widget":28,"../vnode/vpatch":31,"./diff-props":33,"x-is-array":11}],"virtual-dom":[function(require,module,exports){var diff=require("./diff.js");var patch=require("./patch.js");var h=require("./h.js");var create=require("./create-element.js");var VNode=require("./vnode/vnode.js");var VText=require("./vnode/vtext.js");module.exports={diff:diff,patch:patch,h:h,create:create,VNode:VNode,VText:VText}},{"./create-element.js":2,"./diff.js":3,"./h.js":4,"./patch.js":12,"./vnode/vnode.js":30,"./vnode/vtext.js":32}]},{},[]);require=function e(t,n,r){function s(o,u){if(!n[o]){if(!t[o]){var a=typeof require=="function"&&require;if(!u&&a)return a(o,!0);if(i)return i(o,!0);var f=new Error("Cannot find module '"+o+"'");throw f.code="MODULE_NOT_FOUND",f}var l=n[o]={exports:{}};t[o][0].call(l.exports,function(e){var n=t[o][1][e];return s(n?n:e)},l,l.exports,e,t,n,r)}return n[o].exports}var i=typeof require=="function"&&require;for(var o=0;o<r.length;o++)s(r[o]);return s}({1:[function(require,module,exports){},{}],2:[function(require,module,exports){var createElement=require("./vdom/create-element.js");module.exports=createElement},{"./vdom/create-element.js":14}],3:[function(require,module,exports){var diff=require("./vtree/diff.js");module.exports=diff},{"./vtree/diff.js":34}],4:[function(require,module,exports){var h=require("./virtual-hyperscript/index.js");module.exports=h},{"./virtual-hyperscript/index.js":21}],5:[function(require,module,exports){module.exports=function split(undef){var nativeSplit=String.prototype.split,compliantExecNpcg=/()??/.exec("")[1]===undef,self;self=function(str,separator,limit){if(Object.prototype.toString.call(separator)!=="[object RegExp]"){return nativeSplit.call(str,separator,limit)}var output=[],flags=(separator.ignoreCase?"i":"")+(separator.multiline?"m":"")+(separator.extended?"x":"")+(separator.sticky?"y":""),lastLastIndex=0,separator=new RegExp(separator.source,flags+"g"),separator2,match,lastIndex,lastLength;str+="";if(!compliantExecNpcg){separator2=new RegExp("^"+separator.source+"$(?!\\s)",flags)}limit=limit===undef?-1>>>0:limit>>>0;while(match=separator.exec(str)){lastIndex=match.index+match[0].length;if(lastIndex>lastLastIndex){output.push(str.slice(lastLastIndex,match.index));if(!compliantExecNpcg&&match.length>1){match[0].replace(separator2,function(){for(var i=1;i<arguments.length-2;i++){if(arguments[i]===undef){match[i]=undef}}})}if(match.length>1&&match.index<str.length){Array.prototype.push.apply(output,match.slice(1))}lastLength=match[0].length;lastLastIndex=lastIndex;if(output.length>=limit){break}}if(separator.lastIndex===match.index){separator.lastIndex++}}if(lastLastIndex===str.length){if(lastLength||!separator.test("")){output.push("")}}else{output.push(str.slice(lastLastIndex))}return output.length>limit?output.slice(0,limit):output};return self}()},{}],6:[function(require,module,exports){"use strict";var OneVersionConstraint=require("individual/one-version");var MY_VERSION="7";OneVersionConstraint("ev-store",MY_VERSION);var hashKey="__EV_STORE_KEY@"+MY_VERSION;module.exports=EvStore;function EvStore(elem){var hash=elem[hashKey];if(!hash){hash=elem[hashKey]={}}return hash}},{"individual/one-version":8}],7:[function(require,module,exports){(function(global){"use strict";var root=typeof window!=="undefined"?window:typeof global!=="undefined"?global:{};module.exports=Individual;function Individual(key,value){if(key in root){return root[key]}root[key]=value;return value}}).call(this,typeof global!=="undefined"?global:typeof self!=="undefined"?self:typeof window!=="undefined"?window:{})},{}],8:[function(require,module,exports){"use strict";var Individual=require("./index.js");module.exports=OneVersion;function OneVersion(moduleName,version,defaultValue){var key="__INDIVIDUAL_ONE_VERSION_"+moduleName;var enforceKey=key+"_ENFORCE_SINGLETON";var versionValue=Individual(enforceKey,version);if(versionValue!==version){throw new Error("Can only have one copy of "+moduleName+".\n"+"You already have version "+versionValue+" installed.\n"+"This means you cannot install version "+version)}return Individual(key,defaultValue)}},{"./index.js":7}],9:[function(require,module,exports){(function(global){var topLevel=typeof global!=="undefined"?global:typeof window!=="undefined"?window:{};var minDoc=require("min-document");if(typeof document!=="undefined"){module.exports=document}else{var doccy=topLevel["__GLOBAL_DOCUMENT_CACHE@4"];if(!doccy){doccy=topLevel["__GLOBAL_DOCUMENT_CACHE@4"]=minDoc}module.exports=doccy}}).call(this,typeof global!=="undefined"?global:typeof self!=="undefined"?self:typeof window!=="undefined"?window:{})},{"min-document":1}],10:[function(require,module,exports){"use strict";module.exports=function isObject(x){return typeof x==="object"&&x!==null}},{}],11:[function(require,module,exports){var nativeIsArray=Array.isArray;var toString=Object.prototype.toString;module.exports=nativeIsArray||isArray;function isArray(obj){return toString.call(obj)==="[object Array]"}},{}],12:[function(require,module,exports){var patch=require("./vdom/patch.js");module.exports=patch},{"./vdom/patch.js":17}],13:[function(require,module,exports){var isObject=require("is-object");var isHook=require("../vnode/is-vhook.js");module.exports=applyProperties;function applyProperties(node,props,previous){for(var propName in props){var propValue=props[propName];if(propValue===undefined){removeProperty(node,propName,propValue,previous)}else if(isHook(propValue)){removeProperty(node,propName,propValue,previous);if(propValue.hook){propValue.hook(node,propName,previous?previous[propName]:undefined)}}else{if(isObject(propValue)){patchObject(node,props,previous,propName,propValue)}else{node[propName]=propValue}}}}function removeProperty(node,propName,propValue,previous){if(previous){var previousValue=previous[propName];if(!isHook(previousValue)){ | |
if(propName==="attributes"){for(var attrName in previousValue){node.removeAttribute(attrName)}}else if(propName==="style"){for(var i in previousValue){node.style[i]=""}}else if(typeof previousValue==="string"){node[propName]=""}else{node[propName]=null}}else if(previousValue.unhook){previousValue.unhook(node,propName,propValue)}}}function patchObject(node,props,previous,propName,propValue){var previousValue=previous?previous[propName]:undefined;if(propName==="attributes"){for(var attrName in propValue){var attrValue=propValue[attrName];if(attrValue===undefined){node.removeAttribute(attrName)}else{node.setAttribute(attrName,attrValue)}}return}if(previousValue&&isObject(previousValue)&&getPrototype(previousValue)!==getPrototype(propValue)){node[propName]=propValue;return}if(!isObject(node[propName])){node[propName]={}}var replacer=propName==="style"?"":undefined;for(var k in propValue){var value=propValue[k];node[propName][k]=value===undefined?replacer:value}}function getPrototype(value){if(Object.getPrototypeOf){return Object.getPrototypeOf(value)}else if(value.__proto__){return value.__proto__}else if(value.constructor){return value.constructor.prototype}}},{"../vnode/is-vhook.js":25,"is-object":10}],14:[function(require,module,exports){var document=require("global/document");var applyProperties=require("./apply-properties");var isVNode=require("../vnode/is-vnode.js");var isVText=require("../vnode/is-vtext.js");var isWidget=require("../vnode/is-widget.js");var handleThunk=require("../vnode/handle-thunk.js");module.exports=createElement;function createElement(vnode,opts){var doc=opts?opts.document||document:document;var warn=opts?opts.warn:null;vnode=handleThunk(vnode).a;if(isWidget(vnode)){return vnode.init()}else if(isVText(vnode)){return doc.createTextNode(vnode.text)}else if(!isVNode(vnode)){if(warn){warn("Item is not a valid virtual dom node",vnode)}return null}var node=vnode.namespace===null?doc.createElement(vnode.tagName):doc.createElementNS(vnode.namespace,vnode.tagName);var props=vnode.properties;applyProperties(node,props);var children=vnode.children;for(var i=0;i<children.length;i++){var childNode=createElement(children[i],opts);if(childNode){node.appendChild(childNode)}}return node}},{"../vnode/handle-thunk.js":23,"../vnode/is-vnode.js":26,"../vnode/is-vtext.js":27,"../vnode/is-widget.js":28,"./apply-properties":13,"global/document":9}],15:[function(require,module,exports){var noChild={};module.exports=domIndex;function domIndex(rootNode,tree,indices,nodes){if(!indices||indices.length===0){return{}}else{indices.sort(ascending);return recurse(rootNode,tree,indices,nodes,0)}}function recurse(rootNode,tree,indices,nodes,rootIndex){nodes=nodes||{};if(rootNode){if(indexInRange(indices,rootIndex,rootIndex)){nodes[rootIndex]=rootNode}var vChildren=tree.children;if(vChildren){var childNodes=rootNode.childNodes;for(var i=0;i<tree.children.length;i++){rootIndex+=1;var vChild=vChildren[i]||noChild;var nextIndex=rootIndex+(vChild.count||0);if(indexInRange(indices,rootIndex,nextIndex)){recurse(childNodes[i],vChild,indices,nodes,rootIndex)}rootIndex=nextIndex}}}return nodes}function indexInRange(indices,left,right){if(indices.length===0){return false}var minIndex=0;var maxIndex=indices.length-1;var currentIndex;var currentItem;while(minIndex<=maxIndex){currentIndex=(maxIndex+minIndex)/2>>0;currentItem=indices[currentIndex];if(minIndex===maxIndex){return currentItem>=left&¤tItem<=right}else if(currentItem<left){minIndex=currentIndex+1}else if(currentItem>right){maxIndex=currentIndex-1}else{return true}}return false}function ascending(a,b){return a>b?1:-1}},{}],16:[function(require,module,exports){var applyProperties=require("./apply-properties");var isWidget=require("../vnode/is-widget.js");var VPatch=require("../vnode/vpatch.js");var updateWidget=require("./update-widget");module.exports=applyPatch;function applyPatch(vpatch,domNode,renderOptions){var type=vpatch.type;var vNode=vpatch.vNode;var patch=vpatch.patch;switch(type){case VPatch.REMOVE:return removeNode(domNode,vNode);case VPatch.INSERT:return insertNode(domNode,patch,renderOptions);case VPatch.VTEXT:return stringPatch(domNode,vNode,patch,renderOptions);case VPatch.WIDGET:return widgetPatch(domNode,vNode,patch,renderOptions);case VPatch.VNODE:return vNodePatch(domNode,vNode,patch,renderOptions);case VPatch.ORDER:reorderChildren(domNode,patch);return domNode;case VPatch.PROPS:applyProperties(domNode,patch,vNode.properties);return domNode;case VPatch.THUNK:return replaceRoot(domNode,renderOptions.patch(domNode,patch,renderOptions));default:return domNode}}function removeNode(domNode,vNode){var parentNode=domNode.parentNode;if(parentNode){parentNode.removeChild(domNode)}destroyWidget(domNode,vNode);return null}function insertNode(parentNode,vNode,renderOptions){var newNode=renderOptions.render(vNode,renderOptions);if(parentNode){parentNode.appendChild(newNode)}return parentNode}function stringPatch(domNode,leftVNode,vText,renderOptions){var newNode;if(domNode.nodeType===3){domNode.replaceData(0,domNode.length,vText.text);newNode=domNode}else{var parentNode=domNode.parentNode;newNode=renderOptions.render(vText,renderOptions);if(parentNode&&newNode!==domNode){parentNode.replaceChild(newNode,domNode)}}return newNode}function widgetPatch(domNode,leftVNode,widget,renderOptions){var updating=updateWidget(leftVNode,widget);var newNode;if(updating){newNode=widget.update(leftVNode,domNode)||domNode}else{newNode=renderOptions.render(widget,renderOptions)}var parentNode=domNode.parentNode;if(parentNode&&newNode!==domNode){parentNode.replaceChild(newNode,domNode)}if(!updating){destroyWidget(domNode,leftVNode)}return newNode}function vNodePatch(domNode,leftVNode,vNode,renderOptions){var parentNode=domNode.parentNode;var newNode=renderOptions.render(vNode,renderOptions);if(parentNode&&newNode!==domNode){parentNode.replaceChild(newNode,domNode)}return newNode}function destroyWidget(domNode,w){if(typeof w.destroy==="function"&&isWidget(w)){w.destroy(domNode)}}function reorderChildren(domNode,moves){var childNodes=domNode.childNodes;var keyMap={};var node;var remove;var insert;for(var i=0;i<moves.removes.length;i++){remove=moves.removes[i];node=childNodes[remove.from];if(remove.key){keyMap[remove.key]=node}domNode.removeChild(node)}var length=childNodes.length;for(var j=0;j<moves.inserts.length;j++){insert=moves.inserts[j];node=keyMap[insert.key];domNode.insertBefore(node,insert.to>=length++?null:childNodes[insert.to])}}function replaceRoot(oldRoot,newRoot){if(oldRoot&&newRoot&&oldRoot!==newRoot&&oldRoot.parentNode){oldRoot.parentNode.replaceChild(newRoot,oldRoot)}return newRoot}},{"../vnode/is-widget.js":28,"../vnode/vpatch.js":31,"./apply-properties":13,"./update-widget":18}],17:[function(require,module,exports){var document=require("global/document");var isArray=require("x-is-array");var render=require("./create-element");var domIndex=require("./dom-index");var patchOp=require("./patch-op");module.exports=patch;function patch(rootNode,patches,renderOptions){renderOptions=renderOptions||{};renderOptions.patch=renderOptions.patch&&renderOptions.patch!==patch?renderOptions.patch:patchRecursive;renderOptions.render=renderOptions.render||render;return renderOptions.patch(rootNode,patches,renderOptions)}function patchRecursive(rootNode,patches,renderOptions){var indices=patchIndices(patches);if(indices.length===0){return rootNode}var index=domIndex(rootNode,patches.a,indices);var ownerDocument=rootNode.ownerDocument;if(!renderOptions.document&&ownerDocument!==document){renderOptions.document=ownerDocument}for(var i=0;i<indices.length;i++){var nodeIndex=indices[i];rootNode=applyPatch(rootNode,index[nodeIndex],patches[nodeIndex],renderOptions)}return rootNode}function applyPatch(rootNode,domNode,patchList,renderOptions){if(!domNode){return rootNode}var newNode;if(isArray(patchList)){for(var i=0;i<patchList.length;i++){newNode=patchOp(patchList[i],domNode,renderOptions);if(domNode===rootNode){rootNode=newNode}}}else{newNode=patchOp(patchList,domNode,renderOptions);if(domNode===rootNode){rootNode=newNode}}return rootNode}function patchIndices(patches){var indices=[];for(var key in patches){if(key!=="a"){indices.push(Number(key))}}return indices}},{"./create-element":14,"./dom-index":15,"./patch-op":16,"global/document":9,"x-is-array":11}],18:[function(require,module,exports){var isWidget=require("../vnode/is-widget.js");module.exports=updateWidget;function updateWidget(a,b){if(isWidget(a)&&isWidget(b)){if("name"in a&&"name"in b){return a.id===b.id}else{return a.init===b.init}}return false}},{"../vnode/is-widget.js":28}],19:[function(require,module,exports){"use strict";var EvStore=require("ev-store");module.exports=EvHook;function EvHook(value){if(!(this instanceof EvHook)){return new EvHook(value)}this.value=value}EvHook.prototype.hook=function(node,propertyName){var es=EvStore(node);var propName=propertyName.substr(3);es[propName]=this.value};EvHook.prototype.unhook=function(node,propertyName){var es=EvStore(node);var propName=propertyName.substr(3);es[propName]=undefined}},{"ev-store":6}],20:[function(require,module,exports){"use strict";module.exports=SoftSetHook;function SoftSetHook(value){if(!(this instanceof SoftSetHook)){return new SoftSetHook(value)}this.value=value}SoftSetHook.prototype.hook=function(node,propertyName){if(node[propertyName]!==this.value){node[propertyName]=this.value}}},{}],21:[function(require,module,exports){"use strict";var isArray=require("x-is-array");var VNode=require("../vnode/vnode.js");var VText=require("../vnode/vtext.js");var isVNode=require("../vnode/is-vnode");var isVText=require("../vnode/is-vtext");var isWidget=require("../vnode/is-widget");var isHook=require("../vnode/is-vhook");var isVThunk=require("../vnode/is-thunk");var parseTag=require("./parse-tag.js");var softSetHook=require("./hooks/soft-set-hook.js");var evHook=require("./hooks/ev-hook.js");module.exports=h;function h(tagName,properties,children){var childNodes=[];var tag,props,key,namespace;if(!children&&isChildren(properties)){children=properties;props={}}props=props||properties||{};tag=parseTag(tagName,props);if(props.hasOwnProperty("key")){key=props.key;props.key=undefined}if(props.hasOwnProperty("namespace")){namespace=props.namespace;props.namespace=undefined}if(tag==="INPUT"&&!namespace&&props.hasOwnProperty("value")&&props.value!==undefined&&!isHook(props.value)){props.value=softSetHook(props.value)}transformProperties(props);if(children!==undefined&&children!==null){addChild(children,childNodes,tag,props)}return new VNode(tag,props,childNodes,key,namespace)}function addChild(c,childNodes,tag,props){if(typeof c==="string"){childNodes.push(new VText(c))}else if(typeof c==="number"){childNodes.push(new VText(String(c)))}else if(isChild(c)){childNodes.push(c)}else if(isArray(c)){for(var i=0;i<c.length;i++){addChild(c[i],childNodes,tag,props)}}else if(c===null||c===undefined){return}else{throw UnexpectedVirtualElement({foreignObject:c,parentVnode:{tagName:tag,properties:props}})}}function transformProperties(props){for(var propName in props){if(props.hasOwnProperty(propName)){var value=props[propName];if(isHook(value)){continue}if(propName.substr(0,3)==="ev-"){props[propName]=evHook(value)}}}}function isChild(x){return isVNode(x)||isVText(x)||isWidget(x)||isVThunk(x)}function isChildren(x){return typeof x==="string"||isArray(x)||isChild(x)}function UnexpectedVirtualElement(data){var err=new Error;err.type="virtual-hyperscript.unexpected.virtual-element";err.message="Unexpected virtual child passed to h().\n"+"Expected a VNode / Vthunk / VWidget / string but:\n"+"got:\n"+errorString(data.foreignObject)+".\n"+"The parent vnode is:\n"+errorString(data.parentVnode);"\n"+"Suggested fix: change your `h(..., [ ... ])` callsite.";err.foreignObject=data.foreignObject;err.parentVnode=data.parentVnode;return err}function errorString(obj){try{return JSON.stringify(obj,null," ")}catch(e){return String(obj)}}},{"../vnode/is-thunk":24,"../vnode/is-vhook":25,"../vnode/is-vnode":26,"../vnode/is-vtext":27,"../vnode/is-widget":28,"../vnode/vnode.js":30,"../vnode/vtext.js":32,"./hooks/ev-hook.js":19,"./hooks/soft-set-hook.js":20,"./parse-tag.js":22,"x-is-array":11}],22:[function(require,module,exports){"use strict";var split=require("browser-split");var classIdSplit=/([\.#]?[a-zA-Z0-9\u007F-\uFFFF_:-]+)/;var notClassId=/^\.|#/;module.exports=parseTag;function parseTag(tag,props){if(!tag){return"DIV"}var noId=!props.hasOwnProperty("id");var tagParts=split(tag,classIdSplit);var tagName=null;if(notClassId.test(tagParts[1])){tagName="DIV"}var classes,part,type,i;for(i=0;i<tagParts.length;i++){part=tagParts[i];if(!part){continue}type=part.charAt(0);if(!tagName){tagName=part}else if(type==="."){classes=classes||[];classes.push(part.substring(1,part.length))}else if(type==="#"&&noId){props.id=part.substring(1,part.length)}}if(classes){if(props.className){classes.push(props.className)}props.className=classes.join(" ")}return props.namespace?tagName:tagName.toUpperCase()}},{"browser-split":5}],23:[function(require,module,exports){var isVNode=require("./is-vnode");var isVText=require("./is-vtext");var isWidget=require("./is-widget");var isThunk=require("./is-thunk");module.exports=handleThunk;function handleThunk(a,b){var renderedA=a;var renderedB=b;if(isThunk(b)){renderedB=renderThunk(b,a)}if(isThunk(a)){renderedA=renderThunk(a,null)}return{a:renderedA,b:renderedB}}function renderThunk(thunk,previous){var renderedThunk=thunk.vnode;if(!renderedThunk){renderedThunk=thunk.vnode=thunk.render(previous)}if(!(isVNode(renderedThunk)||isVText(renderedThunk)||isWidget(renderedThunk))){throw new Error("thunk did not return a valid node")}return renderedThunk}},{"./is-thunk":24,"./is-vnode":26,"./is-vtext":27,"./is-widget":28}],24:[function(require,module,exports){module.exports=isThunk;function isThunk(t){return t&&t.type==="Thunk"}},{}],25:[function(require,module,exports){module.exports=isHook;function isHook(hook){return hook&&(typeof hook.hook==="function"&&!hook.hasOwnProperty("hook")||typeof hook.unhook==="function"&&!hook.hasOwnProperty("unhook"))}},{}],26:[function(require,module,exports){var version=require("./version");module.exports=isVirtualNode;function isVirtualNode(x){return x&&x.type==="VirtualNode"&&x.version===version}},{"./version":29}],27:[function(require,module,exports){var version=require("./version");module.exports=isVirtualText;function isVirtualText(x){return x&&x.type==="VirtualText"&&x.version===version}},{"./version":29}],28:[function(require,module,exports){module.exports=isWidget;function isWidget(w){return w&&w.type==="Widget"}},{}],29:[function(require,module,exports){module.exports="2"},{}],30:[function(require,module,exports){var version=require("./version");var isVNode=require("./is-vnode");var isWidget=require("./is-widget");var isThunk=require("./is-thunk");var isVHook=require("./is-vhook");module.exports=VirtualNode;var noProperties={};var noChildren=[];function VirtualNode(tagName,properties,children,key,namespace){this.tagName=tagName;this.properties=properties||noProperties;this.children=children||noChildren;this.key=key!=null?String(key):undefined;this.namespace=typeof namespace==="string"?namespace:null;var count=children&&children.length||0;var descendants=0;var hasWidgets=false;var hasThunks=false;var descendantHooks=false;var hooks;for(var propName in properties){if(properties.hasOwnProperty(propName)){var property=properties[propName];if(isVHook(property)&&property.unhook){if(!hooks){hooks={}}hooks[propName]=property}}}for(var i=0;i<count;i++){var child=children[i];if(isVNode(child)){descendants+=child.count||0;if(!hasWidgets&&child.hasWidgets){hasWidgets=true}if(!hasThunks&&child.hasThunks){hasThunks=true}if(!descendantHooks&&(child.hooks||child.descendantHooks)){descendantHooks=true}}else if(!hasWidgets&&isWidget(child)){if(typeof child.destroy==="function"){hasWidgets=true}}else if(!hasThunks&&isThunk(child)){hasThunks=true}}this.count=count+descendants;this.hasWidgets=hasWidgets;this.hasThunks=hasThunks;this.hooks=hooks;this.descendantHooks=descendantHooks}VirtualNode.prototype.version=version;VirtualNode.prototype.type="VirtualNode"},{"./is-thunk":24,"./is-vhook":25,"./is-vnode":26,"./is-widget":28,"./version":29}],31:[function(require,module,exports){var version=require("./version");VirtualPatch.NONE=0;VirtualPatch.VTEXT=1;VirtualPatch.VNODE=2;VirtualPatch.WIDGET=3;VirtualPatch.PROPS=4;VirtualPatch.ORDER=5;VirtualPatch.INSERT=6;VirtualPatch.REMOVE=7;VirtualPatch.THUNK=8;module.exports=VirtualPatch;function VirtualPatch(type,vNode,patch){this.type=Number(type);this.vNode=vNode;this.patch=patch}VirtualPatch.prototype.version=version;VirtualPatch.prototype.type="VirtualPatch"},{"./version":29}],32:[function(require,module,exports){var version=require("./version");module.exports=VirtualText;function VirtualText(text){this.text=String(text)}VirtualText.prototype.version=version;VirtualText.prototype.type="VirtualText"},{"./version":29}],33:[function(require,module,exports){var isObject=require("is-object");var isHook=require("../vnode/is-vhook");module.exports=diffProps;function diffProps(a,b){var diff;for(var aKey in a){if(!(aKey in b)){diff=diff||{};diff[aKey]=undefined}var aValue=a[aKey];var bValue=b[aKey];if(aValue===bValue){continue}else if(isObject(aValue)&&isObject(bValue)){if(getPrototype(bValue)!==getPrototype(aValue)){diff=diff||{};diff[aKey]=bValue}else if(isHook(bValue)){diff=diff||{};diff[aKey]=bValue}else{var objectDiff=diffProps(aValue,bValue);if(objectDiff){diff=diff||{};diff[aKey]=objectDiff}}}else{diff=diff||{};diff[aKey]=bValue}}for(var bKey in b){if(!(bKey in a)){diff=diff||{};diff[bKey]=b[bKey]}}return diff}function getPrototype(value){if(Object.getPrototypeOf){return Object.getPrototypeOf(value)}else if(value.__proto__){return value.__proto__}else if(value.constructor){return value.constructor.prototype}}},{"../vnode/is-vhook":25,"is-object":10}],34:[function(require,module,exports){var isArray=require("x-is-array");var VPatch=require("../vnode/vpatch");var isVNode=require("../vnode/is-vnode");var isVText=require("../vnode/is-vtext");var isWidget=require("../vnode/is-widget");var isThunk=require("../vnode/is-thunk");var handleThunk=require("../vnode/handle-thunk");var diffProps=require("./diff-props");module.exports=diff;function diff(a,b){var patch={a:a};walk(a,b,patch,0);return patch}function walk(a,b,patch,index){if(a===b){return}var apply=patch[index];var applyClear=false;if(isThunk(a)||isThunk(b)){thunks(a,b,patch,index)}else if(b==null){if(!isWidget(a)){clearState(a,patch,index);apply=patch[index]}apply=appendPatch(apply,new VPatch(VPatch.REMOVE,a,b))}else if(isVNode(b)){if(isVNode(a)){if(a.tagName===b.tagName&&a.namespace===b.namespace&&a.key===b.key){var propsPatch=diffProps(a.properties,b.properties);if(propsPatch){apply=appendPatch(apply,new VPatch(VPatch.PROPS,a,propsPatch))}apply=diffChildren(a,b,patch,apply,index)}else{apply=appendPatch(apply,new VPatch(VPatch.VNODE,a,b));applyClear=true}}else{apply=appendPatch(apply,new VPatch(VPatch.VNODE,a,b));applyClear=true}}else if(isVText(b)){if(!isVText(a)){apply=appendPatch(apply,new VPatch(VPatch.VTEXT,a,b));applyClear=true}else if(a.text!==b.text){apply=appendPatch(apply,new VPatch(VPatch.VTEXT,a,b))}}else if(isWidget(b)){if(!isWidget(a)){applyClear=true}apply=appendPatch(apply,new VPatch(VPatch.WIDGET,a,b))}if(apply){patch[index]=apply}if(applyClear){clearState(a,patch,index)}}function diffChildren(a,b,patch,apply,index){var aChildren=a.children;var orderedSet=reorder(aChildren,b.children);var bChildren=orderedSet.children;var aLen=aChildren.length;var bLen=bChildren.length;var len=aLen>bLen?aLen:bLen;for(var i=0;i<len;i++){var leftNode=aChildren[i];var rightNode=bChildren[i];index+=1;if(!leftNode){if(rightNode){apply=appendPatch(apply,new VPatch(VPatch.INSERT,null,rightNode))}}else{walk(leftNode,rightNode,patch,index)}if(isVNode(leftNode)&&leftNode.count){index+=leftNode.count}}if(orderedSet.moves){apply=appendPatch(apply,new VPatch(VPatch.ORDER,a,orderedSet.moves))}return apply}function clearState(vNode,patch,index){unhook(vNode,patch,index);destroyWidgets(vNode,patch,index)}function destroyWidgets(vNode,patch,index){if(isWidget(vNode)){if(typeof vNode.destroy==="function"){patch[index]=appendPatch(patch[index],new VPatch(VPatch.REMOVE,vNode,null))}}else if(isVNode(vNode)&&(vNode.hasWidgets||vNode.hasThunks)){var children=vNode.children;var len=children.length;for(var i=0;i<len;i++){var child=children[i];index+=1;destroyWidgets(child,patch,index);if(isVNode(child)&&child.count){index+=child.count}}}else if(isThunk(vNode)){thunks(vNode,null,patch,index)}}function thunks(a,b,patch,index){var nodes=handleThunk(a,b);var thunkPatch=diff(nodes.a,nodes.b);if(hasPatches(thunkPatch)){patch[index]=new VPatch(VPatch.THUNK,null,thunkPatch)}}function hasPatches(patch){for(var index in patch){if(index!=="a"){return true}}return false}function unhook(vNode,patch,index){if(isVNode(vNode)){if(vNode.hooks){patch[index]=appendPatch(patch[index],new VPatch(VPatch.PROPS,vNode,undefinedKeys(vNode.hooks)))}if(vNode.descendantHooks||vNode.hasThunks){var children=vNode.children;var len=children.length;for(var i=0;i<len;i++){var child=children[i];index+=1;unhook(child,patch,index);if(isVNode(child)&&child.count){index+=child.count}}}}else if(isThunk(vNode)){thunks(vNode,null,patch,index)}}function undefinedKeys(obj){var result={};for(var key in obj){result[key]=undefined}return result}function reorder(aChildren,bChildren){var bChildIndex=keyIndex(bChildren);var bKeys=bChildIndex.keys;var bFree=bChildIndex.free;if(bFree.length===bChildren.length){return{children:bChildren,moves:null}}var aChildIndex=keyIndex(aChildren);var aKeys=aChildIndex.keys;var aFree=aChildIndex.free;if(aFree.length===aChildren.length){return{children:bChildren,moves:null}}var newChildren=[];var freeIndex=0;var freeCount=bFree.length;var deletedItems=0;for(var i=0;i<aChildren.length;i++){var aItem=aChildren[i];var itemIndex;if(aItem.key){if(bKeys.hasOwnProperty(aItem.key)){itemIndex=bKeys[aItem.key];newChildren.push(bChildren[itemIndex])}else{itemIndex=i-deletedItems++;newChildren.push(null)}}else{if(freeIndex<freeCount){itemIndex=bFree[freeIndex++];newChildren.push(bChildren[itemIndex])}else{itemIndex=i-deletedItems++;newChildren.push(null)}}}var lastFreeIndex=freeIndex>=bFree.length?bChildren.length:bFree[freeIndex];for(var j=0;j<bChildren.length;j++){var newItem=bChildren[j];if(newItem.key){if(!aKeys.hasOwnProperty(newItem.key)){newChildren.push(newItem)}}else if(j>=lastFreeIndex){newChildren.push(newItem)}}var simulate=newChildren.slice();var simulateIndex=0;var removes=[];var inserts=[];var simulateItem;for(var k=0;k<bChildren.length;){var wantedItem=bChildren[k];simulateItem=simulate[simulateIndex];while(simulateItem===null&&simulate.length){removes.push(remove(simulate,simulateIndex,null));simulateItem=simulate[simulateIndex]}if(!simulateItem||simulateItem.key!==wantedItem.key){if(wantedItem.key){if(simulateItem&&simulateItem.key){if(bKeys[simulateItem.key]!==k+1){removes.push(remove(simulate,simulateIndex,simulateItem.key));simulateItem=simulate[simulateIndex];if(!simulateItem||simulateItem.key!==wantedItem.key){inserts.push({key:wantedItem.key,to:k})}else{simulateIndex++}}else{inserts.push({key:wantedItem.key,to:k})}}else{inserts.push({key:wantedItem.key,to:k})}k++}else if(simulateItem&&simulateItem.key){removes.push(remove(simulate,simulateIndex,simulateItem.key))}}else{simulateIndex++;k++}}while(simulateIndex<simulate.length){simulateItem=simulate[simulateIndex];removes.push(remove(simulate,simulateIndex,simulateItem&&simulateItem.key))}if(removes.length===deletedItems&&!inserts.length){return{children:newChildren,moves:null}}return{children:newChildren,moves:{removes:removes,inserts:inserts}}}function remove(arr,index,key){arr.splice(index,1);return{from:index,key:key}}function keyIndex(children){var keys={};var free=[];var length=children.length;for(var i=0;i<length;i++){var child=children[i];if(child.key){keys[child.key]=i}else{free.push(i)}}return{keys:keys,free:free}}function appendPatch(apply,patch){if(apply){if(isArray(apply)){apply.push(patch)}else{apply=[apply,patch]}return apply}else{return patch}}},{"../vnode/handle-thunk":23,"../vnode/is-thunk":24,"../vnode/is-vnode":26,"../vnode/is-vtext":27,"../vnode/is-widget":28,"../vnode/vpatch":31,"./diff-props":33,"x-is-array":11}],"virtual-dom":[function(require,module,exports){var diff=require("./diff.js");var patch=require("./patch.js");var h=require("./h.js");var create=require("./create-element.js");var VNode=require("./vnode/vnode.js");var VText=require("./vnode/vtext.js");module.exports={diff:diff,patch:patch,h:h,create:create,VNode:VNode,VText:VText}},{"./create-element.js":2,"./diff.js":3,"./h.js":4,"./patch.js":12,"./vnode/vnode.js":30,"./vnode/vtext.js":32}]},{},[]);require=function e(t,n,r){function s(o,u){if(!n[o]){if(!t[o]){var a=typeof require=="function"&&require;if(!u&&a)return a(o,!0);if(i)return i(o,!0);var f=new Error("Cannot find module '"+o+"'");throw f.code="MODULE_NOT_FOUND",f}var l=n[o]={exports:{}};t[o][0].call(l.exports,function(e){var n=t[o][1][e];return s(n?n:e)},l,l.exports,e,t,n,r)}return n[o].exports}var i=typeof require=="function"&&require;for(var o=0;o<r.length;o++)s(r[o]);return s}({process:[function(require,module,exports){var process=module.exports={};var queue=[];var draining=false;var currentQueue;var queueIndex=-1;function cleanUpNextTick(){draining=false;if(currentQueue.length){queue=currentQueue.concat(queue)}else{queueIndex=-1}if(queue.length){drainQueue()}}function drainQueue(){if(draining){return}var timeout=setTimeout(cleanUpNextTick);draining=true;var len=queue.length;while(len){currentQueue=queue;queue=[];while(++queueIndex<len){if(currentQueue){currentQueue[queueIndex].run()}}queueIndex=-1;len=queue.length}currentQueue=null;draining=false;clearTimeout(timeout)}process.nextTick=function(fun){var args=new Array(arguments.length-1);if(arguments.length>1){for(var i=1;i<arguments.length;i++){args[i-1]=arguments[i]}}queue.push(new Item(fun,args));if(queue.length===1&&!draining){setTimeout(drainQueue,0)}};function Item(fun,array){this.fun=fun;this.array=array}Item.prototype.run=function(){this.fun.apply(null,this.array)};process.title="browser";process.browser=true;process.env={};process.argv=[];process.version="";process.versions={};function noop(){}process.on=noop;process.addListener=noop;process.once=noop;process.off=noop;process.removeListener=noop;process.removeAllListeners=noop;process.emit=noop;process.binding=function(name){throw new Error("process.binding is not supported")};process.cwd=function(){return"/"};process.chdir=function(dir){throw new Error("process.chdir is not supported")};process.umask=function(){return 0}},{}]},{},[]);require=function e(t,n,r){function s(o,u){if(!n[o]){if(!t[o]){var a=typeof require=="function"&&require;if(!u&&a)return a(o,!0);if(i)return i(o,!0);var f=new Error("Cannot find module '"+o+"'");throw f.code="MODULE_NOT_FOUND",f}var l=n[o]={exports:{}};t[o][0].call(l.exports,function(e){var n=t[o][1][e];return s(n?n:e)},l,l.exports,e,t,n,r)}return n[o].exports}var i=typeof require=="function"&&require;for(var o=0;o<r.length;o++)s(r[o]);return s}({1:[function(require,module,exports){},{}],2:[function(require,module,exports){var base64=require("base64-js");var ieee754=require("ieee754");var isArray=require("is-array");exports.Buffer=Buffer;exports.SlowBuffer=SlowBuffer;exports.INSPECT_MAX_BYTES=50;Buffer.poolSize=8192;var kMaxLength=1073741823;var rootParent={};Buffer.TYPED_ARRAY_SUPPORT=function(){try{var buf=new ArrayBuffer(0);var arr=new Uint8Array(buf);arr.foo=function(){return 42};return 42===arr.foo()&&typeof arr.subarray==="function"&&new Uint8Array(1).subarray(1,1).byteLength===0}catch(e){return false}}();function Buffer(subject,encoding,noZero){if(!(this instanceof Buffer))return new Buffer(subject,encoding,noZero);var type=typeof subject;var length;if(type==="number")length=subject>0?subject>>>0:0;else if(type==="string"){length=Buffer.byteLength(subject,encoding)}else if(type==="object"&&subject!==null){if(subject.type==="Buffer"&&isArray(subject.data))subject=subject.data;length=+subject.length>0?Math.floor(+subject.length):0}else throw new TypeError("must start with number, buffer, array or string");if(length>kMaxLength)throw new RangeError("Attempt to allocate Buffer larger than maximum "+"size: 0x"+kMaxLength.toString(16)+" bytes");var buf;if(Buffer.TYPED_ARRAY_SUPPORT){buf=Buffer._augment(new Uint8Array(length))}else{buf=this;buf.length=length;buf._isBuffer=true}var i;if(Buffer.TYPED_ARRAY_SUPPORT&&typeof subject.byteLength==="number"){buf._set(subject)}else if(isArrayish(subject)){if(Buffer.isBuffer(subject)){for(i=0;i<length;i++)buf[i]=subject.readUInt8(i)}else{for(i=0;i<length;i++)buf[i]=(subject[i]%256+256)%256}}else if(type==="string"){buf.write(subject,0,encoding)}else if(type==="number"&&!Buffer.TYPED_ARRAY_SUPPORT&&!noZero){for(i=0;i<length;i++){buf[i]=0}}if(length>0&&length<=Buffer.poolSize)buf.parent=rootParent;return buf}function SlowBuffer(subject,encoding,noZero){if(!(this instanceof SlowBuffer))return new SlowBuffer(subject,encoding,noZero);var buf=new Buffer(subject,encoding,noZero);delete buf.parent;return buf}Buffer.isBuffer=function(b){return!!(b!=null&&b._isBuffer)};Buffer.compare=function(a,b){if(!Buffer.isBuffer(a)||!Buffer.isBuffer(b))throw new TypeError("Arguments must be Buffers");var x=a.length;var y=b.length;for(var i=0,len=Math.min(x,y);i<len&&a[i]===b[i];i++){}if(i!==len){x=a[i];y=b[i]}if(x<y)return-1;if(y<x)return 1;return 0};Buffer.isEncoding=function(encoding){switch(String(encoding).toLowerCase()){case"hex":case"utf8":case"utf-8":case"ascii":case"binary":case"base64":case"raw":case"ucs2":case"ucs-2":case"utf16le":case"utf-16le":return true;default:return false}};Buffer.concat=function(list,totalLength){if(!isArray(list))throw new TypeError("Usage: Buffer.concat(list[, length])");if(list.length===0){return new Buffer(0)}else if(list.length===1){return list[0]}var i;if(totalLength===undefined){totalLength=0;for(i=0;i<list.length;i++){totalLength+=list[i].length}}var buf=new Buffer(totalLength);var pos=0;for(i=0;i<list.length;i++){var item=list[i];item.copy(buf,pos);pos+=item.length}return buf};Buffer.byteLength=function(str,encoding){var ret;str=str+"";switch(encoding||"utf8"){case"ascii":case"binary":case"raw":ret=str.length;break;case"ucs2":case"ucs-2":case"utf16le":case"utf-16le":ret=str.length*2;break;case"hex":ret=str.length>>>1;break;case"utf8":case"utf-8":ret=utf8ToBytes(str).length;break;case"base64":ret=base64ToBytes(str).length;break;default:ret=str.length}return ret};Buffer.prototype.length=undefined;Buffer.prototype.parent=undefined;Buffer.prototype.toString=function(encoding,start,end){var loweredCase=false;start=start>>>0;end=end===undefined||end===Infinity?this.length:end>>>0;if(!encoding)encoding="utf8";if(start<0)start=0;if(end>this.length)end=this.length;if(end<=start)return"";while(true){switch(encoding){case"hex":return hexSlice(this,start,end);case"utf8":case"utf-8":return utf8Slice(this,start,end);case"ascii":return asciiSlice(this,start,end);case"binary":return binarySlice(this,start,end);case"base64":return base64Slice(this,start,end);case"ucs2":case"ucs-2":case"utf16le":case"utf-16le":return utf16leSlice(this,start,end);default:if(loweredCase)throw new TypeError("Unknown encoding: "+encoding);encoding=(encoding+"").toLowerCase();loweredCase=true}}};Buffer.prototype.equals=function(b){if(!Buffer.isBuffer(b))throw new TypeError("Argument must be a Buffer");return Buffer.compare(this,b)===0};Buffer.prototype.inspect=function(){var str="";var max=exports.INSPECT_MAX_BYTES;if(this.length>0){str=this.toString("hex",0,max).match(/.{2}/g).join(" ");if(this.length>max)str+=" ... "}return"<Buffer "+str+">"};Buffer.prototype.compare=function(b){if(!Buffer.isBuffer(b))throw new TypeError("Argument must be a Buffer"); | |
return Buffer.compare(this,b)};Buffer.prototype.get=function(offset){console.log(".get() is deprecated. Access using array indexes instead.");return this.readUInt8(offset)};Buffer.prototype.set=function(v,offset){console.log(".set() is deprecated. Access using array indexes instead.");return this.writeUInt8(v,offset)};function hexWrite(buf,string,offset,length){offset=Number(offset)||0;var remaining=buf.length-offset;if(!length){length=remaining}else{length=Number(length);if(length>remaining){length=remaining}}var strLen=string.length;if(strLen%2!==0)throw new Error("Invalid hex string");if(length>strLen/2){length=strLen/2}for(var i=0;i<length;i++){var byte=parseInt(string.substr(i*2,2),16);if(isNaN(byte))throw new Error("Invalid hex string");buf[offset+i]=byte}return i}function utf8Write(buf,string,offset,length){var charsWritten=blitBuffer(utf8ToBytes(string,buf.length-offset),buf,offset,length);return charsWritten}function asciiWrite(buf,string,offset,length){var charsWritten=blitBuffer(asciiToBytes(string),buf,offset,length);return charsWritten}function binaryWrite(buf,string,offset,length){return asciiWrite(buf,string,offset,length)}function base64Write(buf,string,offset,length){var charsWritten=blitBuffer(base64ToBytes(string),buf,offset,length);return charsWritten}function utf16leWrite(buf,string,offset,length){var charsWritten=blitBuffer(utf16leToBytes(string,buf.length-offset),buf,offset,length,2);return charsWritten}Buffer.prototype.write=function(string,offset,length,encoding){if(isFinite(offset)){if(!isFinite(length)){encoding=length;length=undefined}}else{var swap=encoding;encoding=offset;offset=length;length=swap}offset=Number(offset)||0;if(length<0||offset<0||offset>this.length)throw new RangeError("attempt to write outside buffer bounds");var remaining=this.length-offset;if(!length){length=remaining}else{length=Number(length);if(length>remaining){length=remaining}}encoding=String(encoding||"utf8").toLowerCase();var ret;switch(encoding){case"hex":ret=hexWrite(this,string,offset,length);break;case"utf8":case"utf-8":ret=utf8Write(this,string,offset,length);break;case"ascii":ret=asciiWrite(this,string,offset,length);break;case"binary":ret=binaryWrite(this,string,offset,length);break;case"base64":ret=base64Write(this,string,offset,length);break;case"ucs2":case"ucs-2":case"utf16le":case"utf-16le":ret=utf16leWrite(this,string,offset,length);break;default:throw new TypeError("Unknown encoding: "+encoding)}return ret};Buffer.prototype.toJSON=function(){return{type:"Buffer",data:Array.prototype.slice.call(this._arr||this,0)}};function base64Slice(buf,start,end){if(start===0&&end===buf.length){return base64.fromByteArray(buf)}else{return base64.fromByteArray(buf.slice(start,end))}}function utf8Slice(buf,start,end){var res="";var tmp="";end=Math.min(buf.length,end);for(var i=start;i<end;i++){if(buf[i]<=127){res+=decodeUtf8Char(tmp)+String.fromCharCode(buf[i]);tmp=""}else{tmp+="%"+buf[i].toString(16)}}return res+decodeUtf8Char(tmp)}function asciiSlice(buf,start,end){var ret="";end=Math.min(buf.length,end);for(var i=start;i<end;i++){ret+=String.fromCharCode(buf[i]&127)}return ret}function binarySlice(buf,start,end){var ret="";end=Math.min(buf.length,end);for(var i=start;i<end;i++){ret+=String.fromCharCode(buf[i])}return ret}function hexSlice(buf,start,end){var len=buf.length;if(!start||start<0)start=0;if(!end||end<0||end>len)end=len;var out="";for(var i=start;i<end;i++){out+=toHex(buf[i])}return out}function utf16leSlice(buf,start,end){var bytes=buf.slice(start,end);var res="";for(var i=0;i<bytes.length;i+=2){res+=String.fromCharCode(bytes[i]+bytes[i+1]*256)}return res}Buffer.prototype.slice=function(start,end){var len=this.length;start=~~start;end=end===undefined?len:~~end;if(start<0){start+=len;if(start<0)start=0}else if(start>len){start=len}if(end<0){end+=len;if(end<0)end=0}else if(end>len){end=len}if(end<start)end=start;var newBuf;if(Buffer.TYPED_ARRAY_SUPPORT){newBuf=Buffer._augment(this.subarray(start,end))}else{var sliceLen=end-start;newBuf=new Buffer(sliceLen,undefined,true);for(var i=0;i<sliceLen;i++){newBuf[i]=this[i+start]}}if(newBuf.length)newBuf.parent=this.parent||this;return newBuf};function checkOffset(offset,ext,length){if(offset%1!==0||offset<0)throw new RangeError("offset is not uint");if(offset+ext>length)throw new RangeError("Trying to access beyond buffer length")}Buffer.prototype.readUIntLE=function(offset,byteLength,noAssert){offset=offset>>>0;byteLength=byteLength>>>0;if(!noAssert)checkOffset(offset,byteLength,this.length);var val=this[offset];var mul=1;var i=0;while(++i<byteLength&&(mul*=256))val+=this[offset+i]*mul;return val};Buffer.prototype.readUIntBE=function(offset,byteLength,noAssert){offset=offset>>>0;byteLength=byteLength>>>0;if(!noAssert)checkOffset(offset,byteLength,this.length);var val=this[offset+--byteLength];var mul=1;while(byteLength>0&&(mul*=256))val+=this[offset+--byteLength]*mul;return val};Buffer.prototype.readUInt8=function(offset,noAssert){if(!noAssert)checkOffset(offset,1,this.length);return this[offset]};Buffer.prototype.readUInt16LE=function(offset,noAssert){if(!noAssert)checkOffset(offset,2,this.length);return this[offset]|this[offset+1]<<8};Buffer.prototype.readUInt16BE=function(offset,noAssert){if(!noAssert)checkOffset(offset,2,this.length);return this[offset]<<8|this[offset+1]};Buffer.prototype.readUInt32LE=function(offset,noAssert){if(!noAssert)checkOffset(offset,4,this.length);return(this[offset]|this[offset+1]<<8|this[offset+2]<<16)+this[offset+3]*16777216};Buffer.prototype.readUInt32BE=function(offset,noAssert){if(!noAssert)checkOffset(offset,4,this.length);return this[offset]*16777216+(this[offset+1]<<16|this[offset+2]<<8|this[offset+3])};Buffer.prototype.readIntLE=function(offset,byteLength,noAssert){offset=offset>>>0;byteLength=byteLength>>>0;if(!noAssert)checkOffset(offset,byteLength,this.length);var val=this[offset];var mul=1;var i=0;while(++i<byteLength&&(mul*=256))val+=this[offset+i]*mul;mul*=128;if(val>=mul)val-=Math.pow(2,8*byteLength);return val};Buffer.prototype.readIntBE=function(offset,byteLength,noAssert){offset=offset>>>0;byteLength=byteLength>>>0;if(!noAssert)checkOffset(offset,byteLength,this.length);var i=byteLength;var mul=1;var val=this[offset+--i];while(i>0&&(mul*=256))val+=this[offset+--i]*mul;mul*=128;if(val>=mul)val-=Math.pow(2,8*byteLength);return val};Buffer.prototype.readInt8=function(offset,noAssert){if(!noAssert)checkOffset(offset,1,this.length);if(!(this[offset]&128))return this[offset];return(255-this[offset]+1)*-1};Buffer.prototype.readInt16LE=function(offset,noAssert){if(!noAssert)checkOffset(offset,2,this.length);var val=this[offset]|this[offset+1]<<8;return val&32768?val|4294901760:val};Buffer.prototype.readInt16BE=function(offset,noAssert){if(!noAssert)checkOffset(offset,2,this.length);var val=this[offset+1]|this[offset]<<8;return val&32768?val|4294901760:val};Buffer.prototype.readInt32LE=function(offset,noAssert){if(!noAssert)checkOffset(offset,4,this.length);return this[offset]|this[offset+1]<<8|this[offset+2]<<16|this[offset+3]<<24};Buffer.prototype.readInt32BE=function(offset,noAssert){if(!noAssert)checkOffset(offset,4,this.length);return this[offset]<<24|this[offset+1]<<16|this[offset+2]<<8|this[offset+3]};Buffer.prototype.readFloatLE=function(offset,noAssert){if(!noAssert)checkOffset(offset,4,this.length);return ieee754.read(this,offset,true,23,4)};Buffer.prototype.readFloatBE=function(offset,noAssert){if(!noAssert)checkOffset(offset,4,this.length);return ieee754.read(this,offset,false,23,4)};Buffer.prototype.readDoubleLE=function(offset,noAssert){if(!noAssert)checkOffset(offset,8,this.length);return ieee754.read(this,offset,true,52,8)};Buffer.prototype.readDoubleBE=function(offset,noAssert){if(!noAssert)checkOffset(offset,8,this.length);return ieee754.read(this,offset,false,52,8)};function checkInt(buf,value,offset,ext,max,min){if(!Buffer.isBuffer(buf))throw new TypeError("buffer must be a Buffer instance");if(value>max||value<min)throw new RangeError("value is out of bounds");if(offset+ext>buf.length)throw new RangeError("index out of range")}Buffer.prototype.writeUIntLE=function(value,offset,byteLength,noAssert){value=+value;offset=offset>>>0;byteLength=byteLength>>>0;if(!noAssert)checkInt(this,value,offset,byteLength,Math.pow(2,8*byteLength),0);var mul=1;var i=0;this[offset]=value&255;while(++i<byteLength&&(mul*=256))this[offset+i]=value/mul>>>0&255;return offset+byteLength};Buffer.prototype.writeUIntBE=function(value,offset,byteLength,noAssert){value=+value;offset=offset>>>0;byteLength=byteLength>>>0;if(!noAssert)checkInt(this,value,offset,byteLength,Math.pow(2,8*byteLength),0);var i=byteLength-1;var mul=1;this[offset+i]=value&255;while(--i>=0&&(mul*=256))this[offset+i]=value/mul>>>0&255;return offset+byteLength};Buffer.prototype.writeUInt8=function(value,offset,noAssert){value=+value;offset=offset>>>0;if(!noAssert)checkInt(this,value,offset,1,255,0);if(!Buffer.TYPED_ARRAY_SUPPORT)value=Math.floor(value);this[offset]=value;return offset+1};function objectWriteUInt16(buf,value,offset,littleEndian){if(value<0)value=65535+value+1;for(var i=0,j=Math.min(buf.length-offset,2);i<j;i++){buf[offset+i]=(value&255<<8*(littleEndian?i:1-i))>>>(littleEndian?i:1-i)*8}}Buffer.prototype.writeUInt16LE=function(value,offset,noAssert){value=+value;offset=offset>>>0;if(!noAssert)checkInt(this,value,offset,2,65535,0);if(Buffer.TYPED_ARRAY_SUPPORT){this[offset]=value;this[offset+1]=value>>>8}else objectWriteUInt16(this,value,offset,true);return offset+2};Buffer.prototype.writeUInt16BE=function(value,offset,noAssert){value=+value;offset=offset>>>0;if(!noAssert)checkInt(this,value,offset,2,65535,0);if(Buffer.TYPED_ARRAY_SUPPORT){this[offset]=value>>>8;this[offset+1]=value}else objectWriteUInt16(this,value,offset,false);return offset+2};function objectWriteUInt32(buf,value,offset,littleEndian){if(value<0)value=4294967295+value+1;for(var i=0,j=Math.min(buf.length-offset,4);i<j;i++){buf[offset+i]=value>>>(littleEndian?i:3-i)*8&255}}Buffer.prototype.writeUInt32LE=function(value,offset,noAssert){value=+value;offset=offset>>>0;if(!noAssert)checkInt(this,value,offset,4,4294967295,0);if(Buffer.TYPED_ARRAY_SUPPORT){this[offset+3]=value>>>24;this[offset+2]=value>>>16;this[offset+1]=value>>>8;this[offset]=value}else objectWriteUInt32(this,value,offset,true);return offset+4};Buffer.prototype.writeUInt32BE=function(value,offset,noAssert){value=+value;offset=offset>>>0;if(!noAssert)checkInt(this,value,offset,4,4294967295,0);if(Buffer.TYPED_ARRAY_SUPPORT){this[offset]=value>>>24;this[offset+1]=value>>>16;this[offset+2]=value>>>8;this[offset+3]=value}else objectWriteUInt32(this,value,offset,false);return offset+4};Buffer.prototype.writeIntLE=function(value,offset,byteLength,noAssert){value=+value;offset=offset>>>0;if(!noAssert){checkInt(this,value,offset,byteLength,Math.pow(2,8*byteLength-1)-1,-Math.pow(2,8*byteLength-1))}var i=0;var mul=1;var sub=value<0?1:0;this[offset]=value&255;while(++i<byteLength&&(mul*=256))this[offset+i]=(value/mul>>0)-sub&255;return offset+byteLength};Buffer.prototype.writeIntBE=function(value,offset,byteLength,noAssert){value=+value;offset=offset>>>0;if(!noAssert){checkInt(this,value,offset,byteLength,Math.pow(2,8*byteLength-1)-1,-Math.pow(2,8*byteLength-1))}var i=byteLength-1;var mul=1;var sub=value<0?1:0;this[offset+i]=value&255;while(--i>=0&&(mul*=256))this[offset+i]=(value/mul>>0)-sub&255;return offset+byteLength};Buffer.prototype.writeInt8=function(value,offset,noAssert){value=+value;offset=offset>>>0;if(!noAssert)checkInt(this,value,offset,1,127,-128);if(!Buffer.TYPED_ARRAY_SUPPORT)value=Math.floor(value);if(value<0)value=255+value+1;this[offset]=value;return offset+1};Buffer.prototype.writeInt16LE=function(value,offset,noAssert){value=+value;offset=offset>>>0;if(!noAssert)checkInt(this,value,offset,2,32767,-32768);if(Buffer.TYPED_ARRAY_SUPPORT){this[offset]=value;this[offset+1]=value>>>8}else objectWriteUInt16(this,value,offset,true);return offset+2};Buffer.prototype.writeInt16BE=function(value,offset,noAssert){value=+value;offset=offset>>>0;if(!noAssert)checkInt(this,value,offset,2,32767,-32768);if(Buffer.TYPED_ARRAY_SUPPORT){this[offset]=value>>>8;this[offset+1]=value}else objectWriteUInt16(this,value,offset,false);return offset+2};Buffer.prototype.writeInt32LE=function(value,offset,noAssert){value=+value;offset=offset>>>0;if(!noAssert)checkInt(this,value,offset,4,2147483647,-2147483648);if(Buffer.TYPED_ARRAY_SUPPORT){this[offset]=value;this[offset+1]=value>>>8;this[offset+2]=value>>>16;this[offset+3]=value>>>24}else objectWriteUInt32(this,value,offset,true);return offset+4};Buffer.prototype.writeInt32BE=function(value,offset,noAssert){value=+value;offset=offset>>>0;if(!noAssert)checkInt(this,value,offset,4,2147483647,-2147483648);if(value<0)value=4294967295+value+1;if(Buffer.TYPED_ARRAY_SUPPORT){this[offset]=value>>>24;this[offset+1]=value>>>16;this[offset+2]=value>>>8;this[offset+3]=value}else objectWriteUInt32(this,value,offset,false);return offset+4};function checkIEEE754(buf,value,offset,ext,max,min){if(value>max||value<min)throw new RangeError("value is out of bounds");if(offset+ext>buf.length)throw new RangeError("index out of range");if(offset<0)throw new RangeError("index out of range")}function writeFloat(buf,value,offset,littleEndian,noAssert){if(!noAssert)checkIEEE754(buf,value,offset,4,3.4028234663852886e38,-3.4028234663852886e38);ieee754.write(buf,value,offset,littleEndian,23,4);return offset+4}Buffer.prototype.writeFloatLE=function(value,offset,noAssert){return writeFloat(this,value,offset,true,noAssert)};Buffer.prototype.writeFloatBE=function(value,offset,noAssert){return writeFloat(this,value,offset,false,noAssert)};function writeDouble(buf,value,offset,littleEndian,noAssert){if(!noAssert)checkIEEE754(buf,value,offset,8,1.7976931348623157e308,-1.7976931348623157e308);ieee754.write(buf,value,offset,littleEndian,52,8);return offset+8}Buffer.prototype.writeDoubleLE=function(value,offset,noAssert){return writeDouble(this,value,offset,true,noAssert)};Buffer.prototype.writeDoubleBE=function(value,offset,noAssert){return writeDouble(this,value,offset,false,noAssert)};Buffer.prototype.copy=function(target,target_start,start,end){var source=this;if(!start)start=0;if(!end&&end!==0)end=this.length;if(target_start>=target.length)target_start=target.length;if(!target_start)target_start=0;if(end>0&&end<start)end=start;if(end===start)return 0;if(target.length===0||source.length===0)return 0;if(target_start<0)throw new RangeError("targetStart out of bounds");if(start<0||start>=source.length)throw new RangeError("sourceStart out of bounds");if(end<0)throw new RangeError("sourceEnd out of bounds");if(end>this.length)end=this.length;if(target.length-target_start<end-start)end=target.length-target_start+start;var len=end-start;if(len<1e3||!Buffer.TYPED_ARRAY_SUPPORT){for(var i=0;i<len;i++){target[i+target_start]=this[i+start]}}else{target._set(this.subarray(start,start+len),target_start)}return len};Buffer.prototype.fill=function(value,start,end){if(!value)value=0;if(!start)start=0;if(!end)end=this.length;if(end<start)throw new RangeError("end < start");if(end===start)return;if(this.length===0)return;if(start<0||start>=this.length)throw new RangeError("start out of bounds");if(end<0||end>this.length)throw new RangeError("end out of bounds");var i;if(typeof value==="number"){for(i=start;i<end;i++){this[i]=value}}else{var bytes=utf8ToBytes(value.toString());var len=bytes.length;for(i=start;i<end;i++){this[i]=bytes[i%len]}}return this};Buffer.prototype.toArrayBuffer=function(){if(typeof Uint8Array!=="undefined"){if(Buffer.TYPED_ARRAY_SUPPORT){return new Buffer(this).buffer}else{var buf=new Uint8Array(this.length);for(var i=0,len=buf.length;i<len;i+=1){buf[i]=this[i]}return buf.buffer}}else{throw new TypeError("Buffer.toArrayBuffer not supported in this browser")}};var BP=Buffer.prototype;Buffer._augment=function(arr){arr.constructor=Buffer;arr._isBuffer=true;arr._get=arr.get;arr._set=arr.set;arr.get=BP.get;arr.set=BP.set;arr.write=BP.write;arr.toString=BP.toString;arr.toLocaleString=BP.toString;arr.toJSON=BP.toJSON;arr.equals=BP.equals;arr.compare=BP.compare;arr.copy=BP.copy;arr.slice=BP.slice;arr.readUIntLE=BP.readUIntLE;arr.readUIntBE=BP.readUIntBE;arr.readUInt8=BP.readUInt8;arr.readUInt16LE=BP.readUInt16LE;arr.readUInt16BE=BP.readUInt16BE;arr.readUInt32LE=BP.readUInt32LE;arr.readUInt32BE=BP.readUInt32BE;arr.readIntLE=BP.readIntLE;arr.readIntBE=BP.readIntBE;arr.readInt8=BP.readInt8;arr.readInt16LE=BP.readInt16LE;arr.readInt16BE=BP.readInt16BE;arr.readInt32LE=BP.readInt32LE;arr.readInt32BE=BP.readInt32BE;arr.readFloatLE=BP.readFloatLE;arr.readFloatBE=BP.readFloatBE;arr.readDoubleLE=BP.readDoubleLE;arr.readDoubleBE=BP.readDoubleBE;arr.writeUInt8=BP.writeUInt8;arr.writeUIntLE=BP.writeUIntLE;arr.writeUIntBE=BP.writeUIntBE;arr.writeUInt16LE=BP.writeUInt16LE;arr.writeUInt16BE=BP.writeUInt16BE;arr.writeUInt32LE=BP.writeUInt32LE;arr.writeUInt32BE=BP.writeUInt32BE;arr.writeIntLE=BP.writeIntLE;arr.writeIntBE=BP.writeIntBE;arr.writeInt8=BP.writeInt8;arr.writeInt16LE=BP.writeInt16LE;arr.writeInt16BE=BP.writeInt16BE;arr.writeInt32LE=BP.writeInt32LE;arr.writeInt32BE=BP.writeInt32BE;arr.writeFloatLE=BP.writeFloatLE;arr.writeFloatBE=BP.writeFloatBE;arr.writeDoubleLE=BP.writeDoubleLE;arr.writeDoubleBE=BP.writeDoubleBE;arr.fill=BP.fill;arr.inspect=BP.inspect;arr.toArrayBuffer=BP.toArrayBuffer;return arr};var INVALID_BASE64_RE=/[^+\/0-9A-z\-]/g;function base64clean(str){str=stringtrim(str).replace(INVALID_BASE64_RE,"");if(str.length<2)return"";while(str.length%4!==0){str=str+"="}return str}function stringtrim(str){if(str.trim)return str.trim();return str.replace(/^\s+|\s+$/g,"")}function isArrayish(subject){return isArray(subject)||Buffer.isBuffer(subject)||subject&&typeof subject==="object"&&typeof subject.length==="number"}function toHex(n){if(n<16)return"0"+n.toString(16);return n.toString(16)}function utf8ToBytes(string,units){var codePoint,length=string.length;var leadSurrogate=null;units=units||Infinity;var bytes=[];var i=0;for(;i<length;i++){codePoint=string.charCodeAt(i);if(codePoint>55295&&codePoint<57344){if(leadSurrogate){if(codePoint<56320){if((units-=3)>-1)bytes.push(239,191,189);leadSurrogate=codePoint;continue}else{codePoint=leadSurrogate-55296<<10|codePoint-56320|65536;leadSurrogate=null}}else{if(codePoint>56319){if((units-=3)>-1)bytes.push(239,191,189);continue}else if(i+1===length){if((units-=3)>-1)bytes.push(239,191,189);continue}else{leadSurrogate=codePoint;continue}}}else if(leadSurrogate){if((units-=3)>-1)bytes.push(239,191,189);leadSurrogate=null}if(codePoint<128){if((units-=1)<0)break;bytes.push(codePoint)}else if(codePoint<2048){if((units-=2)<0)break;bytes.push(codePoint>>6|192,codePoint&63|128)}else if(codePoint<65536){if((units-=3)<0)break;bytes.push(codePoint>>12|224,codePoint>>6&63|128,codePoint&63|128)}else if(codePoint<2097152){if((units-=4)<0)break;bytes.push(codePoint>>18|240,codePoint>>12&63|128,codePoint>>6&63|128,codePoint&63|128)}else{throw new Error("Invalid code point")}}return bytes}function asciiToBytes(str){var byteArray=[];for(var i=0;i<str.length;i++){byteArray.push(str.charCodeAt(i)&255)}return byteArray}function utf16leToBytes(str,units){var c,hi,lo;var byteArray=[];for(var i=0;i<str.length;i++){if((units-=2)<0)break;c=str.charCodeAt(i);hi=c>>8;lo=c%256;byteArray.push(lo);byteArray.push(hi)}return byteArray}function base64ToBytes(str){return base64.toByteArray(base64clean(str))}function blitBuffer(src,dst,offset,length,unitSize){if(unitSize)length-=length%unitSize;for(var i=0;i<length;i++){if(i+offset>=dst.length||i>=src.length)break;dst[i+offset]=src[i]}return i}function decodeUtf8Char(str){try{return decodeURIComponent(str)}catch(err){return String.fromCharCode(65533)}}},{"base64-js":3,ieee754:4,"is-array":5}],3:[function(require,module,exports){var lookup="ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789+/";(function(exports){"use strict";var Arr=typeof Uint8Array!=="undefined"?Uint8Array:Array;var PLUS="+".charCodeAt(0);var SLASH="/".charCodeAt(0);var NUMBER="0".charCodeAt(0);var LOWER="a".charCodeAt(0);var UPPER="A".charCodeAt(0);var PLUS_URL_SAFE="-".charCodeAt(0);var SLASH_URL_SAFE="_".charCodeAt(0);function decode(elt){var code=elt.charCodeAt(0);if(code===PLUS||code===PLUS_URL_SAFE)return 62;if(code===SLASH||code===SLASH_URL_SAFE)return 63;if(code<NUMBER)return-1;if(code<NUMBER+10)return code-NUMBER+26+26;if(code<UPPER+26)return code-UPPER;if(code<LOWER+26)return code-LOWER+26}function b64ToByteArray(b64){var i,j,l,tmp,placeHolders,arr;if(b64.length%4>0){throw new Error("Invalid string. Length must be a multiple of 4")}var len=b64.length;placeHolders="="===b64.charAt(len-2)?2:"="===b64.charAt(len-1)?1:0;arr=new Arr(b64.length*3/4-placeHolders);l=placeHolders>0?b64.length-4:b64.length;var L=0;function push(v){arr[L++]=v}for(i=0,j=0;i<l;i+=4,j+=3){tmp=decode(b64.charAt(i))<<18|decode(b64.charAt(i+1))<<12|decode(b64.charAt(i+2))<<6|decode(b64.charAt(i+3));push((tmp&16711680)>>16);push((tmp&65280)>>8);push(tmp&255)}if(placeHolders===2){tmp=decode(b64.charAt(i))<<2|decode(b64.charAt(i+1))>>4;push(tmp&255)}else if(placeHolders===1){tmp=decode(b64.charAt(i))<<10|decode(b64.charAt(i+1))<<4|decode(b64.charAt(i+2))>>2;push(tmp>>8&255);push(tmp&255)}return arr}function uint8ToBase64(uint8){var i,extraBytes=uint8.length%3,output="",temp,length;function encode(num){return lookup.charAt(num)}function tripletToBase64(num){return encode(num>>18&63)+encode(num>>12&63)+encode(num>>6&63)+encode(num&63)}for(i=0,length=uint8.length-extraBytes;i<length;i+=3){temp=(uint8[i]<<16)+(uint8[i+1]<<8)+uint8[i+2];output+=tripletToBase64(temp)}switch(extraBytes){case 1:temp=uint8[uint8.length-1];output+=encode(temp>>2);output+=encode(temp<<4&63);output+="==";break;case 2:temp=(uint8[uint8.length-2]<<8)+uint8[uint8.length-1];output+=encode(temp>>10);output+=encode(temp>>4&63);output+=encode(temp<<2&63);output+="=";break}return output}exports.toByteArray=b64ToByteArray;exports.fromByteArray=uint8ToBase64})(typeof exports==="undefined"?this.base64js={}:exports)},{}],4:[function(require,module,exports){exports.read=function(buffer,offset,isLE,mLen,nBytes){var e,m,eLen=nBytes*8-mLen-1,eMax=(1<<eLen)-1,eBias=eMax>>1,nBits=-7,i=isLE?nBytes-1:0,d=isLE?-1:1,s=buffer[offset+i];i+=d;e=s&(1<<-nBits)-1;s>>=-nBits;nBits+=eLen;for(;nBits>0;e=e*256+buffer[offset+i],i+=d,nBits-=8);m=e&(1<<-nBits)-1;e>>=-nBits;nBits+=mLen;for(;nBits>0;m=m*256+buffer[offset+i],i+=d,nBits-=8);if(e===0){e=1-eBias}else if(e===eMax){return m?NaN:(s?-1:1)*Infinity}else{m=m+Math.pow(2,mLen);e=e-eBias}return(s?-1:1)*m*Math.pow(2,e-mLen)};exports.write=function(buffer,value,offset,isLE,mLen,nBytes){var e,m,c,eLen=nBytes*8-mLen-1,eMax=(1<<eLen)-1,eBias=eMax>>1,rt=mLen===23?Math.pow(2,-24)-Math.pow(2,-77):0,i=isLE?0:nBytes-1,d=isLE?1:-1,s=value<0||value===0&&1/value<0?1:0;value=Math.abs(value);if(isNaN(value)||value===Infinity){m=isNaN(value)?1:0;e=eMax}else{e=Math.floor(Math.log(value)/Math.LN2);if(value*(c=Math.pow(2,-e))<1){e--;c*=2}if(e+eBias>=1){value+=rt/c}else{value+=rt*Math.pow(2,1-eBias)}if(value*c>=2){e++;c/=2}if(e+eBias>=eMax){m=0;e=eMax}else if(e+eBias>=1){m=(value*c-1)*Math.pow(2,mLen);e=e+eBias}else{m=value*Math.pow(2,eBias-1)*Math.pow(2,mLen);e=0}}for(;mLen>=8;buffer[offset+i]=m&255,i+=d,m/=256,mLen-=8);e=e<<mLen|m;eLen+=mLen;for(;eLen>0;buffer[offset+i]=e&255,i+=d,e/=256,eLen-=8);buffer[offset+i-d]|=s*128}},{}],5:[function(require,module,exports){var isArray=Array.isArray;var str=Object.prototype.toString;module.exports=isArray||function(val){return!!val&&"[object Array]"==str.call(val)}},{}],6:[function(require,module,exports){function EventEmitter(){this._events=this._events||{};this._maxListeners=this._maxListeners||undefined}module.exports=EventEmitter;EventEmitter.EventEmitter=EventEmitter;EventEmitter.prototype._events=undefined;EventEmitter.prototype._maxListeners=undefined;EventEmitter.defaultMaxListeners=10;EventEmitter.prototype.setMaxListeners=function(n){if(!isNumber(n)||n<0||isNaN(n))throw TypeError("n must be a positive number");this._maxListeners=n;return this};EventEmitter.prototype.emit=function(type){var er,handler,len,args,i,listeners;if(!this._events)this._events={};if(type==="error"){if(!this._events.error||isObject(this._events.error)&&!this._events.error.length){er=arguments[1];if(er instanceof Error){throw er}throw TypeError('Uncaught, unspecified "error" event.')}}handler=this._events[type];if(isUndefined(handler))return false;if(isFunction(handler)){switch(arguments.length){case 1:handler.call(this);break;case 2:handler.call(this,arguments[1]);break;case 3:handler.call(this,arguments[1],arguments[2]);break;default:len=arguments.length;args=new Array(len-1);for(i=1;i<len;i++)args[i-1]=arguments[i];handler.apply(this,args)}}else if(isObject(handler)){len=arguments.length;args=new Array(len-1);for(i=1;i<len;i++)args[i-1]=arguments[i];listeners=handler.slice();len=listeners.length;for(i=0;i<len;i++)listeners[i].apply(this,args)}return true};EventEmitter.prototype.addListener=function(type,listener){var m;if(!isFunction(listener))throw TypeError("listener must be a function");if(!this._events)this._events={};if(this._events.newListener)this.emit("newListener",type,isFunction(listener.listener)?listener.listener:listener);if(!this._events[type])this._events[type]=listener;else if(isObject(this._events[type]))this._events[type].push(listener);else this._events[type]=[this._events[type],listener];if(isObject(this._events[type])&&!this._events[type].warned){var m;if(!isUndefined(this._maxListeners)){m=this._maxListeners}else{m=EventEmitter.defaultMaxListeners}if(m&&m>0&&this._events[type].length>m){this._events[type].warned=true;console.error("(node) warning: possible EventEmitter memory "+"leak detected. %d listeners added. "+"Use emitter.setMaxListeners() to increase limit.",this._events[type].length);if(typeof console.trace==="function"){console.trace()}}}return this};EventEmitter.prototype.on=EventEmitter.prototype.addListener;EventEmitter.prototype.once=function(type,listener){if(!isFunction(listener))throw TypeError("listener must be a function");var fired=false;function g(){this.removeListener(type,g);if(!fired){fired=true;listener.apply(this,arguments)}}g.listener=listener;this.on(type,g);return this};EventEmitter.prototype.removeListener=function(type,listener){var list,position,length,i;if(!isFunction(listener))throw TypeError("listener must be a function");if(!this._events||!this._events[type])return this;list=this._events[type];length=list.length;position=-1;if(list===listener||isFunction(list.listener)&&list.listener===listener){delete this._events[type];if(this._events.removeListener)this.emit("removeListener",type,listener)}else if(isObject(list)){for(i=length;i-->0;){if(list[i]===listener||list[i].listener&&list[i].listener===listener){position=i;break}}if(position<0)return this;if(list.length===1){list.length=0;delete this._events[type]}else{list.splice(position,1)}if(this._events.removeListener)this.emit("removeListener",type,listener)}return this};EventEmitter.prototype.removeAllListeners=function(type){var key,listeners;if(!this._events)return this;if(!this._events.removeListener){if(arguments.length===0)this._events={};else if(this._events[type])delete this._events[type];return this}if(arguments.length===0){for(key in this._events){if(key==="removeListener")continue;this.removeAllListeners(key)}this.removeAllListeners("removeListener");this._events={};return this}listeners=this._events[type];if(isFunction(listeners)){this.removeListener(type,listeners)}else{while(listeners.length)this.removeListener(type,listeners[listeners.length-1])}delete this._events[type];return this};EventEmitter.prototype.listeners=function(type){var ret;if(!this._events||!this._events[type])ret=[];else if(isFunction(this._events[type]))ret=[this._events[type]];else ret=this._events[type].slice();return ret};EventEmitter.listenerCount=function(emitter,type){var ret;if(!emitter._events||!emitter._events[type])ret=0;else if(isFunction(emitter._events[type]))ret=1;else ret=emitter._events[type].length;return ret};function isFunction(arg){return typeof arg==="function"}function isNumber(arg){return typeof arg==="number"}function isObject(arg){return typeof arg==="object"&&arg!==null}function isUndefined(arg){return arg===void 0}},{}],7:[function(require,module,exports){var process=module.exports={};process.nextTick=function(){var canSetImmediate=typeof window!=="undefined"&&window.setImmediate;var canMutationObserver=typeof window!=="undefined"&&window.MutationObserver;var canPost=typeof window!=="undefined"&&window.postMessage&&window.addEventListener;if(canSetImmediate){return function(f){return window.setImmediate(f)}}var queue=[];if(canMutationObserver){var hiddenDiv=document.createElement("div");var observer=new MutationObserver(function(){var queueList=queue.slice();queue.length=0;queueList.forEach(function(fn){fn()})});observer.observe(hiddenDiv,{attributes:true});return function nextTick(fn){if(!queue.length){hiddenDiv.setAttribute("yes","no")}queue.push(fn)}}if(canPost){window.addEventListener("message",function(ev){var source=ev.source;if((source===window||source===null)&&ev.data==="process-tick"){ev.stopPropagation();if(queue.length>0){var fn=queue.shift();fn()}}},true);return function nextTick(fn){queue.push(fn);window.postMessage("process-tick","*")}}return function nextTick(fn){setTimeout(fn,0)}}();process.title="browser";process.browser=true;process.env={};process.argv=[];function noop(){}process.on=noop;process.addListener=noop;process.once=noop;process.off=noop;process.removeListener=noop;process.removeAllListeners=noop;process.emit=noop;process.binding=function(name){throw new Error("process.binding is not supported")};process.cwd=function(){return"/"};process.chdir=function(dir){throw new Error("process.chdir is not supported")}},{}],8:[function(require,module,exports){if(typeof Object.create==="function"){module.exports=function inherits(ctor,superCtor){ctor.super_=superCtor;ctor.prototype=Object.create(superCtor.prototype,{constructor:{value:ctor,enumerable:false,writable:true,configurable:true}})}}else{module.exports=function inherits(ctor,superCtor){ctor.super_=superCtor;var TempCtor=function(){};TempCtor.prototype=superCtor.prototype;ctor.prototype=new TempCtor;ctor.prototype.constructor=ctor}}},{}],9:[function(require,module,exports){module.exports=require("./lib/_stream_duplex.js")},{"./lib/_stream_duplex.js":10}],10:[function(require,module,exports){"use strict";var objectKeys=Object.keys||function(obj){var keys=[];for(var key in obj)keys.push(key);return keys};module.exports=Duplex;var processNextTick=require("process-nextick-args");var util=require("core-util-is");util.inherits=require("inherits");var Readable=require("./_stream_readable");var Writable=require("./_stream_writable");util.inherits(Duplex,Readable);var keys=objectKeys(Writable.prototype);for(var v=0;v<keys.length;v++){var method=keys[v];if(!Duplex.prototype[method])Duplex.prototype[method]=Writable.prototype[method]}function Duplex(options){if(!(this instanceof Duplex))return new Duplex(options);Readable.call(this,options);Writable.call(this,options);if(options&&options.readable===false)this.readable=false;if(options&&options.writable===false)this.writable=false;this.allowHalfOpen=true;if(options&&options.allowHalfOpen===false)this.allowHalfOpen=false;this.once("end",onend)}function onend(){if(this.allowHalfOpen||this._writableState.ended)return;processNextTick(onEndNT,this)}function onEndNT(self){self.end()}function forEach(xs,f){for(var i=0,l=xs.length;i<l;i++){f(xs[i],i)}}},{"./_stream_readable":12,"./_stream_writable":14,"core-util-is":15,inherits:8,"process-nextick-args":17}],11:[function(require,module,exports){"use strict";module.exports=PassThrough;var Transform=require("./_stream_transform");var util=require("core-util-is");util.inherits=require("inherits");util.inherits(PassThrough,Transform); | |
function PassThrough(options){if(!(this instanceof PassThrough))return new PassThrough(options);Transform.call(this,options)}PassThrough.prototype._transform=function(chunk,encoding,cb){cb(null,chunk)}},{"./_stream_transform":13,"core-util-is":15,inherits:8}],12:[function(require,module,exports){(function(process){"use strict";module.exports=Readable;var processNextTick=require("process-nextick-args");var isArray=require("isarray");var Buffer=require("buffer").Buffer;Readable.ReadableState=ReadableState;var EE=require("events");var EElistenerCount=function(emitter,type){return emitter.listeners(type).length};var Stream;(function(){try{Stream=require("st"+"ream")}catch(_){}finally{if(!Stream)Stream=require("events").EventEmitter}})();var Buffer=require("buffer").Buffer;var util=require("core-util-is");util.inherits=require("inherits");var debugUtil=require("util");var debug;if(debugUtil&&debugUtil.debuglog){debug=debugUtil.debuglog("stream")}else{debug=function(){}}var StringDecoder;util.inherits(Readable,Stream);var Duplex;function ReadableState(options,stream){Duplex=Duplex||require("./_stream_duplex");options=options||{};this.objectMode=!!options.objectMode;if(stream instanceof Duplex)this.objectMode=this.objectMode||!!options.readableObjectMode;var hwm=options.highWaterMark;var defaultHwm=this.objectMode?16:16*1024;this.highWaterMark=hwm||hwm===0?hwm:defaultHwm;this.highWaterMark=~~this.highWaterMark;this.buffer=[];this.length=0;this.pipes=null;this.pipesCount=0;this.flowing=null;this.ended=false;this.endEmitted=false;this.reading=false;this.sync=true;this.needReadable=false;this.emittedReadable=false;this.readableListening=false;this.defaultEncoding=options.defaultEncoding||"utf8";this.ranOut=false;this.awaitDrain=0;this.readingMore=false;this.decoder=null;this.encoding=null;if(options.encoding){if(!StringDecoder)StringDecoder=require("string_decoder/").StringDecoder;this.decoder=new StringDecoder(options.encoding);this.encoding=options.encoding}}var Duplex;function Readable(options){Duplex=Duplex||require("./_stream_duplex");if(!(this instanceof Readable))return new Readable(options);this._readableState=new ReadableState(options,this);this.readable=true;if(options&&typeof options.read==="function")this._read=options.read;Stream.call(this)}Readable.prototype.push=function(chunk,encoding){var state=this._readableState;if(!state.objectMode&&typeof chunk==="string"){encoding=encoding||state.defaultEncoding;if(encoding!==state.encoding){chunk=new Buffer(chunk,encoding);encoding=""}}return readableAddChunk(this,state,chunk,encoding,false)};Readable.prototype.unshift=function(chunk){var state=this._readableState;return readableAddChunk(this,state,chunk,"",true)};Readable.prototype.isPaused=function(){return this._readableState.flowing===false};function readableAddChunk(stream,state,chunk,encoding,addToFront){var er=chunkInvalid(state,chunk);if(er){stream.emit("error",er)}else if(chunk===null){state.reading=false;onEofChunk(stream,state)}else if(state.objectMode||chunk&&chunk.length>0){if(state.ended&&!addToFront){var e=new Error("stream.push() after EOF");stream.emit("error",e)}else if(state.endEmitted&&addToFront){var e=new Error("stream.unshift() after end event");stream.emit("error",e)}else{if(state.decoder&&!addToFront&&!encoding)chunk=state.decoder.write(chunk);if(!addToFront)state.reading=false;if(state.flowing&&state.length===0&&!state.sync){stream.emit("data",chunk);stream.read(0)}else{state.length+=state.objectMode?1:chunk.length;if(addToFront)state.buffer.unshift(chunk);else state.buffer.push(chunk);if(state.needReadable)emitReadable(stream)}maybeReadMore(stream,state)}}else if(!addToFront){state.reading=false}return needMoreData(state)}function needMoreData(state){return!state.ended&&(state.needReadable||state.length<state.highWaterMark||state.length===0)}Readable.prototype.setEncoding=function(enc){if(!StringDecoder)StringDecoder=require("string_decoder/").StringDecoder;this._readableState.decoder=new StringDecoder(enc);this._readableState.encoding=enc;return this};var MAX_HWM=8388608;function computeNewHighWaterMark(n){if(n>=MAX_HWM){n=MAX_HWM}else{n--;n|=n>>>1;n|=n>>>2;n|=n>>>4;n|=n>>>8;n|=n>>>16;n++}return n}function howMuchToRead(n,state){if(state.length===0&&state.ended)return 0;if(state.objectMode)return n===0?0:1;if(n===null||isNaN(n)){if(state.flowing&&state.buffer.length)return state.buffer[0].length;else return state.length}if(n<=0)return 0;if(n>state.highWaterMark)state.highWaterMark=computeNewHighWaterMark(n);if(n>state.length){if(!state.ended){state.needReadable=true;return 0}else{return state.length}}return n}Readable.prototype.read=function(n){debug("read",n);var state=this._readableState;var nOrig=n;if(typeof n!=="number"||n>0)state.emittedReadable=false;if(n===0&&state.needReadable&&(state.length>=state.highWaterMark||state.ended)){debug("read: emitReadable",state.length,state.ended);if(state.length===0&&state.ended)endReadable(this);else emitReadable(this);return null}n=howMuchToRead(n,state);if(n===0&&state.ended){if(state.length===0)endReadable(this);return null}var doRead=state.needReadable;debug("need readable",doRead);if(state.length===0||state.length-n<state.highWaterMark){doRead=true;debug("length less than watermark",doRead)}if(state.ended||state.reading){doRead=false;debug("reading or ended",doRead)}if(doRead){debug("do read");state.reading=true;state.sync=true;if(state.length===0)state.needReadable=true;this._read(state.highWaterMark);state.sync=false}if(doRead&&!state.reading)n=howMuchToRead(nOrig,state);var ret;if(n>0)ret=fromList(n,state);else ret=null;if(ret===null){state.needReadable=true;n=0}state.length-=n;if(state.length===0&&!state.ended)state.needReadable=true;if(nOrig!==n&&state.ended&&state.length===0)endReadable(this);if(ret!==null)this.emit("data",ret);return ret};function chunkInvalid(state,chunk){var er=null;if(!Buffer.isBuffer(chunk)&&typeof chunk!=="string"&&chunk!==null&&chunk!==undefined&&!state.objectMode){er=new TypeError("Invalid non-string/buffer chunk")}return er}function onEofChunk(stream,state){if(state.ended)return;if(state.decoder){var chunk=state.decoder.end();if(chunk&&chunk.length){state.buffer.push(chunk);state.length+=state.objectMode?1:chunk.length}}state.ended=true;emitReadable(stream)}function emitReadable(stream){var state=stream._readableState;state.needReadable=false;if(!state.emittedReadable){debug("emitReadable",state.flowing);state.emittedReadable=true;if(state.sync)processNextTick(emitReadable_,stream);else emitReadable_(stream)}}function emitReadable_(stream){debug("emit readable");stream.emit("readable");flow(stream)}function maybeReadMore(stream,state){if(!state.readingMore){state.readingMore=true;processNextTick(maybeReadMore_,stream,state)}}function maybeReadMore_(stream,state){var len=state.length;while(!state.reading&&!state.flowing&&!state.ended&&state.length<state.highWaterMark){debug("maybeReadMore read 0");stream.read(0);if(len===state.length)break;else len=state.length}state.readingMore=false}Readable.prototype._read=function(n){this.emit("error",new Error("not implemented"))};Readable.prototype.pipe=function(dest,pipeOpts){var src=this;var state=this._readableState;switch(state.pipesCount){case 0:state.pipes=dest;break;case 1:state.pipes=[state.pipes,dest];break;default:state.pipes.push(dest);break}state.pipesCount+=1;debug("pipe count=%d opts=%j",state.pipesCount,pipeOpts);var doEnd=(!pipeOpts||pipeOpts.end!==false)&&dest!==process.stdout&&dest!==process.stderr;var endFn=doEnd?onend:cleanup;if(state.endEmitted)processNextTick(endFn);else src.once("end",endFn);dest.on("unpipe",onunpipe);function onunpipe(readable){debug("onunpipe");if(readable===src){cleanup()}}function onend(){debug("onend");dest.end()}var ondrain=pipeOnDrain(src);dest.on("drain",ondrain);var cleanedUp=false;function cleanup(){debug("cleanup");dest.removeListener("close",onclose);dest.removeListener("finish",onfinish);dest.removeListener("drain",ondrain);dest.removeListener("error",onerror);dest.removeListener("unpipe",onunpipe);src.removeListener("end",onend);src.removeListener("end",cleanup);src.removeListener("data",ondata);cleanedUp=true;if(state.awaitDrain&&(!dest._writableState||dest._writableState.needDrain))ondrain()}src.on("data",ondata);function ondata(chunk){debug("ondata");var ret=dest.write(chunk);if(false===ret){if(state.pipesCount===1&&state.pipes[0]===dest&&src.listenerCount("data")===1&&!cleanedUp){debug("false write response, pause",src._readableState.awaitDrain);src._readableState.awaitDrain++}src.pause()}}function onerror(er){debug("onerror",er);unpipe();dest.removeListener("error",onerror);if(EElistenerCount(dest,"error")===0)dest.emit("error",er)}if(!dest._events||!dest._events.error)dest.on("error",onerror);else if(isArray(dest._events.error))dest._events.error.unshift(onerror);else dest._events.error=[onerror,dest._events.error];function onclose(){dest.removeListener("finish",onfinish);unpipe()}dest.once("close",onclose);function onfinish(){debug("onfinish");dest.removeListener("close",onclose);unpipe()}dest.once("finish",onfinish);function unpipe(){debug("unpipe");src.unpipe(dest)}dest.emit("pipe",src);if(!state.flowing){debug("pipe resume");src.resume()}return dest};function pipeOnDrain(src){return function(){var state=src._readableState;debug("pipeOnDrain",state.awaitDrain);if(state.awaitDrain)state.awaitDrain--;if(state.awaitDrain===0&&EElistenerCount(src,"data")){state.flowing=true;flow(src)}}}Readable.prototype.unpipe=function(dest){var state=this._readableState;if(state.pipesCount===0)return this;if(state.pipesCount===1){if(dest&&dest!==state.pipes)return this;if(!dest)dest=state.pipes;state.pipes=null;state.pipesCount=0;state.flowing=false;if(dest)dest.emit("unpipe",this);return this}if(!dest){var dests=state.pipes;var len=state.pipesCount;state.pipes=null;state.pipesCount=0;state.flowing=false;for(var i=0;i<len;i++)dests[i].emit("unpipe",this);return this}var i=indexOf(state.pipes,dest);if(i===-1)return this;state.pipes.splice(i,1);state.pipesCount-=1;if(state.pipesCount===1)state.pipes=state.pipes[0];dest.emit("unpipe",this);return this};Readable.prototype.on=function(ev,fn){var res=Stream.prototype.on.call(this,ev,fn);if(ev==="data"&&false!==this._readableState.flowing){this.resume()}if(ev==="readable"&&this.readable){var state=this._readableState;if(!state.readableListening){state.readableListening=true;state.emittedReadable=false;state.needReadable=true;if(!state.reading){processNextTick(nReadingNextTick,this)}else if(state.length){emitReadable(this,state)}}}return res};Readable.prototype.addListener=Readable.prototype.on;function nReadingNextTick(self){debug("readable nexttick read 0");self.read(0)}Readable.prototype.resume=function(){var state=this._readableState;if(!state.flowing){debug("resume");state.flowing=true;resume(this,state)}return this};function resume(stream,state){if(!state.resumeScheduled){state.resumeScheduled=true;processNextTick(resume_,stream,state)}}function resume_(stream,state){if(!state.reading){debug("resume read 0");stream.read(0)}state.resumeScheduled=false;stream.emit("resume");flow(stream);if(state.flowing&&!state.reading)stream.read(0)}Readable.prototype.pause=function(){debug("call pause flowing=%j",this._readableState.flowing);if(false!==this._readableState.flowing){debug("pause");this._readableState.flowing=false;this.emit("pause")}return this};function flow(stream){var state=stream._readableState;debug("flow",state.flowing);if(state.flowing){do{var chunk=stream.read()}while(null!==chunk&&state.flowing)}}Readable.prototype.wrap=function(stream){var state=this._readableState;var paused=false;var self=this;stream.on("end",function(){debug("wrapped end");if(state.decoder&&!state.ended){var chunk=state.decoder.end();if(chunk&&chunk.length)self.push(chunk)}self.push(null)});stream.on("data",function(chunk){debug("wrapped data");if(state.decoder)chunk=state.decoder.write(chunk);if(state.objectMode&&(chunk===null||chunk===undefined))return;else if(!state.objectMode&&(!chunk||!chunk.length))return;var ret=self.push(chunk);if(!ret){paused=true;stream.pause()}});for(var i in stream){if(this[i]===undefined&&typeof stream[i]==="function"){this[i]=function(method){return function(){return stream[method].apply(stream,arguments)}}(i)}}var events=["error","close","destroy","pause","resume"];forEach(events,function(ev){stream.on(ev,self.emit.bind(self,ev))});self._read=function(n){debug("wrapped _read",n);if(paused){paused=false;stream.resume()}};return self};Readable._fromList=fromList;function fromList(n,state){var list=state.buffer;var length=state.length;var stringMode=!!state.decoder;var objectMode=!!state.objectMode;var ret;if(list.length===0)return null;if(length===0)ret=null;else if(objectMode)ret=list.shift();else if(!n||n>=length){if(stringMode)ret=list.join("");else if(list.length===1)ret=list[0];else ret=Buffer.concat(list,length);list.length=0}else{if(n<list[0].length){var buf=list[0];ret=buf.slice(0,n);list[0]=buf.slice(n)}else if(n===list[0].length){ret=list.shift()}else{if(stringMode)ret="";else ret=new Buffer(n);var c=0;for(var i=0,l=list.length;i<l&&c<n;i++){var buf=list[0];var cpy=Math.min(n-c,buf.length);if(stringMode)ret+=buf.slice(0,cpy);else buf.copy(ret,c,0,cpy);if(cpy<buf.length)list[0]=buf.slice(cpy);else list.shift();c+=cpy}}}return ret}function endReadable(stream){var state=stream._readableState;if(state.length>0)throw new Error("endReadable called on non-empty stream");if(!state.endEmitted){state.ended=true;processNextTick(endReadableNT,state,stream)}}function endReadableNT(state,stream){if(!state.endEmitted&&state.length===0){state.endEmitted=true;stream.readable=false;stream.emit("end")}}function forEach(xs,f){for(var i=0,l=xs.length;i<l;i++){f(xs[i],i)}}function indexOf(xs,x){for(var i=0,l=xs.length;i<l;i++){if(xs[i]===x)return i}return-1}}).call(this,require("_process"))},{"./_stream_duplex":10,_process:7,buffer:2,"core-util-is":15,events:6,inherits:8,isarray:16,"process-nextick-args":17,"string_decoder/":18,util:1}],13:[function(require,module,exports){"use strict";module.exports=Transform;var Duplex=require("./_stream_duplex");var util=require("core-util-is");util.inherits=require("inherits");util.inherits(Transform,Duplex);function TransformState(stream){this.afterTransform=function(er,data){return afterTransform(stream,er,data)};this.needTransform=false;this.transforming=false;this.writecb=null;this.writechunk=null}function afterTransform(stream,er,data){var ts=stream._transformState;ts.transforming=false;var cb=ts.writecb;if(!cb)return stream.emit("error",new Error("no writecb in Transform class"));ts.writechunk=null;ts.writecb=null;if(data!==null&&data!==undefined)stream.push(data);if(cb)cb(er);var rs=stream._readableState;rs.reading=false;if(rs.needReadable||rs.length<rs.highWaterMark){stream._read(rs.highWaterMark)}}function Transform(options){if(!(this instanceof Transform))return new Transform(options);Duplex.call(this,options);this._transformState=new TransformState(this);var stream=this;this._readableState.needReadable=true;this._readableState.sync=false;if(options){if(typeof options.transform==="function")this._transform=options.transform;if(typeof options.flush==="function")this._flush=options.flush}this.once("prefinish",function(){if(typeof this._flush==="function")this._flush(function(er){done(stream,er)});else done(stream)})}Transform.prototype.push=function(chunk,encoding){this._transformState.needTransform=false;return Duplex.prototype.push.call(this,chunk,encoding)};Transform.prototype._transform=function(chunk,encoding,cb){throw new Error("not implemented")};Transform.prototype._write=function(chunk,encoding,cb){var ts=this._transformState;ts.writecb=cb;ts.writechunk=chunk;ts.writeencoding=encoding;if(!ts.transforming){var rs=this._readableState;if(ts.needTransform||rs.needReadable||rs.length<rs.highWaterMark)this._read(rs.highWaterMark)}};Transform.prototype._read=function(n){var ts=this._transformState;if(ts.writechunk!==null&&ts.writecb&&!ts.transforming){ts.transforming=true;this._transform(ts.writechunk,ts.writeencoding,ts.afterTransform)}else{ts.needTransform=true}};function done(stream,er){if(er)return stream.emit("error",er);var ws=stream._writableState;var ts=stream._transformState;if(ws.length)throw new Error("calling transform done when ws.length != 0");if(ts.transforming)throw new Error("calling transform done when still transforming");return stream.push(null)}},{"./_stream_duplex":10,"core-util-is":15,inherits:8}],14:[function(require,module,exports){"use strict";module.exports=Writable;var processNextTick=require("process-nextick-args");var Buffer=require("buffer").Buffer;Writable.WritableState=WritableState;var util=require("core-util-is");util.inherits=require("inherits");var internalUtil={deprecate:require("util-deprecate")};var Stream;(function(){try{Stream=require("st"+"ream")}catch(_){}finally{if(!Stream)Stream=require("events").EventEmitter}})();var Buffer=require("buffer").Buffer;util.inherits(Writable,Stream);function nop(){}function WriteReq(chunk,encoding,cb){this.chunk=chunk;this.encoding=encoding;this.callback=cb;this.next=null}var Duplex;function WritableState(options,stream){Duplex=Duplex||require("./_stream_duplex");options=options||{};this.objectMode=!!options.objectMode;if(stream instanceof Duplex)this.objectMode=this.objectMode||!!options.writableObjectMode;var hwm=options.highWaterMark;var defaultHwm=this.objectMode?16:16*1024;this.highWaterMark=hwm||hwm===0?hwm:defaultHwm;this.highWaterMark=~~this.highWaterMark;this.needDrain=false;this.ending=false;this.ended=false;this.finished=false;var noDecode=options.decodeStrings===false;this.decodeStrings=!noDecode;this.defaultEncoding=options.defaultEncoding||"utf8";this.length=0;this.writing=false;this.corked=0;this.sync=true;this.bufferProcessing=false;this.onwrite=function(er){onwrite(stream,er)};this.writecb=null;this.writelen=0;this.bufferedRequest=null;this.lastBufferedRequest=null;this.pendingcb=0;this.prefinished=false;this.errorEmitted=false}WritableState.prototype.getBuffer=function writableStateGetBuffer(){var current=this.bufferedRequest;var out=[];while(current){out.push(current);current=current.next}return out};(function(){try{Object.defineProperty(WritableState.prototype,"buffer",{get:internalUtil.deprecate(function(){return this.getBuffer()},"_writableState.buffer is deprecated. Use _writableState.getBuffer "+"instead.")})}catch(_){}})();var Duplex;function Writable(options){Duplex=Duplex||require("./_stream_duplex");if(!(this instanceof Writable)&&!(this instanceof Duplex))return new Writable(options);this._writableState=new WritableState(options,this);this.writable=true;if(options){if(typeof options.write==="function")this._write=options.write;if(typeof options.writev==="function")this._writev=options.writev}Stream.call(this)}Writable.prototype.pipe=function(){this.emit("error",new Error("Cannot pipe. Not readable."))};function writeAfterEnd(stream,cb){var er=new Error("write after end");stream.emit("error",er);processNextTick(cb,er)}function validChunk(stream,state,chunk,cb){var valid=true;if(!Buffer.isBuffer(chunk)&&typeof chunk!=="string"&&chunk!==null&&chunk!==undefined&&!state.objectMode){var er=new TypeError("Invalid non-string/buffer chunk");stream.emit("error",er);processNextTick(cb,er);valid=false}return valid}Writable.prototype.write=function(chunk,encoding,cb){var state=this._writableState;var ret=false;if(typeof encoding==="function"){cb=encoding;encoding=null}if(Buffer.isBuffer(chunk))encoding="buffer";else if(!encoding)encoding=state.defaultEncoding;if(typeof cb!=="function")cb=nop;if(state.ended)writeAfterEnd(this,cb);else if(validChunk(this,state,chunk,cb)){state.pendingcb++;ret=writeOrBuffer(this,state,chunk,encoding,cb)}return ret};Writable.prototype.cork=function(){var state=this._writableState;state.corked++};Writable.prototype.uncork=function(){var state=this._writableState;if(state.corked){state.corked--;if(!state.writing&&!state.corked&&!state.finished&&!state.bufferProcessing&&state.bufferedRequest)clearBuffer(this,state)}};Writable.prototype.setDefaultEncoding=function setDefaultEncoding(encoding){if(typeof encoding==="string")encoding=encoding.toLowerCase();if(!(["hex","utf8","utf-8","ascii","binary","base64","ucs2","ucs-2","utf16le","utf-16le","raw"].indexOf((encoding+"").toLowerCase())>-1))throw new TypeError("Unknown encoding: "+encoding);this._writableState.defaultEncoding=encoding};function decodeChunk(state,chunk,encoding){if(!state.objectMode&&state.decodeStrings!==false&&typeof chunk==="string"){chunk=new Buffer(chunk,encoding)}return chunk}function writeOrBuffer(stream,state,chunk,encoding,cb){chunk=decodeChunk(state,chunk,encoding);if(Buffer.isBuffer(chunk))encoding="buffer";var len=state.objectMode?1:chunk.length;state.length+=len;var ret=state.length<state.highWaterMark;if(!ret)state.needDrain=true;if(state.writing||state.corked){var last=state.lastBufferedRequest;state.lastBufferedRequest=new WriteReq(chunk,encoding,cb);if(last){last.next=state.lastBufferedRequest}else{state.bufferedRequest=state.lastBufferedRequest}}else{doWrite(stream,state,false,len,chunk,encoding,cb)}return ret}function doWrite(stream,state,writev,len,chunk,encoding,cb){state.writelen=len;state.writecb=cb;state.writing=true;state.sync=true;if(writev)stream._writev(chunk,state.onwrite);else stream._write(chunk,encoding,state.onwrite);state.sync=false}function onwriteError(stream,state,sync,er,cb){--state.pendingcb;if(sync)processNextTick(cb,er);else cb(er);stream._writableState.errorEmitted=true;stream.emit("error",er)}function onwriteStateUpdate(state){state.writing=false;state.writecb=null;state.length-=state.writelen;state.writelen=0}function onwrite(stream,er){var state=stream._writableState;var sync=state.sync;var cb=state.writecb;onwriteStateUpdate(state);if(er)onwriteError(stream,state,sync,er,cb);else{var finished=needFinish(state);if(!finished&&!state.corked&&!state.bufferProcessing&&state.bufferedRequest){clearBuffer(stream,state)}if(sync){processNextTick(afterWrite,stream,state,finished,cb)}else{afterWrite(stream,state,finished,cb)}}}function afterWrite(stream,state,finished,cb){if(!finished)onwriteDrain(stream,state);state.pendingcb--;cb();finishMaybe(stream,state)}function onwriteDrain(stream,state){if(state.length===0&&state.needDrain){state.needDrain=false;stream.emit("drain")}}function clearBuffer(stream,state){state.bufferProcessing=true;var entry=state.bufferedRequest;if(stream._writev&&entry&&entry.next){var buffer=[];var cbs=[];while(entry){cbs.push(entry.callback);buffer.push(entry);entry=entry.next}state.pendingcb++;state.lastBufferedRequest=null;doWrite(stream,state,true,state.length,buffer,"",function(err){for(var i=0;i<cbs.length;i++){state.pendingcb--;cbs[i](err)}})}else{while(entry){var chunk=entry.chunk;var encoding=entry.encoding;var cb=entry.callback;var len=state.objectMode?1:chunk.length;doWrite(stream,state,false,len,chunk,encoding,cb);entry=entry.next;if(state.writing){break}}if(entry===null)state.lastBufferedRequest=null}state.bufferedRequest=entry;state.bufferProcessing=false}Writable.prototype._write=function(chunk,encoding,cb){cb(new Error("not implemented"))};Writable.prototype._writev=null;Writable.prototype.end=function(chunk,encoding,cb){var state=this._writableState;if(typeof chunk==="function"){cb=chunk;chunk=null;encoding=null}else if(typeof encoding==="function"){cb=encoding;encoding=null}if(chunk!==null&&chunk!==undefined)this.write(chunk,encoding);if(state.corked){state.corked=1;this.uncork()}if(!state.ending&&!state.finished)endWritable(this,state,cb)};function needFinish(state){return state.ending&&state.length===0&&state.bufferedRequest===null&&!state.finished&&!state.writing}function prefinish(stream,state){if(!state.prefinished){state.prefinished=true;stream.emit("prefinish")}}function finishMaybe(stream,state){var need=needFinish(state);if(need){if(state.pendingcb===0){prefinish(stream,state);state.finished=true;stream.emit("finish")}else{prefinish(stream,state)}}return need}function endWritable(stream,state,cb){state.ending=true;finishMaybe(stream,state);if(cb){if(state.finished)processNextTick(cb);else stream.once("finish",cb)}state.ended=true}},{"./_stream_duplex":10,buffer:2,"core-util-is":15,events:6,inherits:8,"process-nextick-args":17,"util-deprecate":19}],15:[function(require,module,exports){(function(Buffer){function isArray(arg){if(Array.isArray){return Array.isArray(arg)}return objectToString(arg)==="[object Array]"}exports.isArray=isArray;function isBoolean(arg){return typeof arg==="boolean"}exports.isBoolean=isBoolean;function isNull(arg){return arg===null}exports.isNull=isNull;function isNullOrUndefined(arg){return arg==null}exports.isNullOrUndefined=isNullOrUndefined;function isNumber(arg){return typeof arg==="number"}exports.isNumber=isNumber;function isString(arg){return typeof arg==="string"}exports.isString=isString;function isSymbol(arg){return typeof arg==="symbol"}exports.isSymbol=isSymbol;function isUndefined(arg){return arg===void 0}exports.isUndefined=isUndefined;function isRegExp(re){return objectToString(re)==="[object RegExp]"}exports.isRegExp=isRegExp;function isObject(arg){return typeof arg==="object"&&arg!==null}exports.isObject=isObject;function isDate(d){return objectToString(d)==="[object Date]"}exports.isDate=isDate;function isError(e){return objectToString(e)==="[object Error]"||e instanceof Error}exports.isError=isError;function isFunction(arg){return typeof arg==="function"}exports.isFunction=isFunction;function isPrimitive(arg){return arg===null||typeof arg==="boolean"||typeof arg==="number"||typeof arg==="string"||typeof arg==="symbol"||typeof arg==="undefined"}exports.isPrimitive=isPrimitive;exports.isBuffer=Buffer.isBuffer;function objectToString(o){return Object.prototype.toString.call(o)}}).call(this,require("buffer").Buffer)},{buffer:2}],16:[function(require,module,exports){module.exports=Array.isArray||function(arr){return Object.prototype.toString.call(arr)=="[object Array]"}},{}],17:[function(require,module,exports){(function(process){"use strict";if(!process.version||process.version.indexOf("v0.")===0||process.version.indexOf("v1.")===0&&process.version.indexOf("v1.8.")!==0){module.exports=nextTick}else{module.exports=process.nextTick}function nextTick(fn){var args=new Array(arguments.length-1);var i=0;while(i<args.length){args[i++]=arguments[i]}process.nextTick(function afterTick(){fn.apply(null,args)})}}).call(this,require("_process"))},{_process:7}],18:[function(require,module,exports){var Buffer=require("buffer").Buffer;var isBufferEncoding=Buffer.isEncoding||function(encoding){switch(encoding&&encoding.toLowerCase()){case"hex":case"utf8":case"utf-8":case"ascii":case"binary":case"base64":case"ucs2":case"ucs-2":case"utf16le":case"utf-16le":case"raw":return true;default:return false}};function assertEncoding(encoding){if(encoding&&!isBufferEncoding(encoding)){throw new Error("Unknown encoding: "+encoding)}}var StringDecoder=exports.StringDecoder=function(encoding){this.encoding=(encoding||"utf8").toLowerCase().replace(/[-_]/,"");assertEncoding(encoding);switch(this.encoding){case"utf8":this.surrogateSize=3;break;case"ucs2":case"utf16le":this.surrogateSize=2;this.detectIncompleteChar=utf16DetectIncompleteChar;break;case"base64":this.surrogateSize=3;this.detectIncompleteChar=base64DetectIncompleteChar;break;default:this.write=passThroughWrite;return}this.charBuffer=new Buffer(6);this.charReceived=0;this.charLength=0};StringDecoder.prototype.write=function(buffer){var charStr="";while(this.charLength){var available=buffer.length>=this.charLength-this.charReceived?this.charLength-this.charReceived:buffer.length;buffer.copy(this.charBuffer,this.charReceived,0,available);this.charReceived+=available;if(this.charReceived<this.charLength){return""}buffer=buffer.slice(available,buffer.length);charStr=this.charBuffer.slice(0,this.charLength).toString(this.encoding);var charCode=charStr.charCodeAt(charStr.length-1);if(charCode>=55296&&charCode<=56319){this.charLength+=this.surrogateSize;charStr="";continue}this.charReceived=this.charLength=0;if(buffer.length===0){return charStr}break}this.detectIncompleteChar(buffer);var end=buffer.length;if(this.charLength){buffer.copy(this.charBuffer,0,buffer.length-this.charReceived,end);end-=this.charReceived}charStr+=buffer.toString(this.encoding,0,end);var end=charStr.length-1;var charCode=charStr.charCodeAt(end);if(charCode>=55296&&charCode<=56319){var size=this.surrogateSize;this.charLength+=size;this.charReceived+=size;this.charBuffer.copy(this.charBuffer,size,0,size);buffer.copy(this.charBuffer,0,0,size);return charStr.substring(0,end)}return charStr};StringDecoder.prototype.detectIncompleteChar=function(buffer){var i=buffer.length>=3?3:buffer.length;for(;i>0;i--){var c=buffer[buffer.length-i];if(i==1&&c>>5==6){this.charLength=2;break}if(i<=2&&c>>4==14){this.charLength=3;break}if(i<=3&&c>>3==30){this.charLength=4;break}}this.charReceived=i};StringDecoder.prototype.end=function(buffer){var res="";if(buffer&&buffer.length)res=this.write(buffer);if(this.charReceived){var cr=this.charReceived;var buf=this.charBuffer;var enc=this.encoding;res+=buf.slice(0,cr).toString(enc)}return res};function passThroughWrite(buffer){return buffer.toString(this.encoding)}function utf16DetectIncompleteChar(buffer){this.charReceived=buffer.length%2;this.charLength=this.charReceived?2:0}function base64DetectIncompleteChar(buffer){this.charReceived=buffer.length%3;this.charLength=this.charReceived?3:0}},{buffer:2}],19:[function(require,module,exports){(function(global){module.exports=deprecate;function deprecate(fn,msg){if(config("noDeprecation")){return fn}var warned=false;function deprecated(){if(!warned){if(config("throwDeprecation")){throw new Error(msg)}else if(config("traceDeprecation")){console.trace(msg)}else{console.warn(msg)}warned=true}return fn.apply(this,arguments)}return deprecated}function config(name){try{if(!global.localStorage)return false}catch(_){return false}var val=global.localStorage[name];if(null==val)return false;return String(val).toLowerCase()==="true"}}).call(this,typeof global!=="undefined"?global:typeof self!=="undefined"?self:typeof window!=="undefined"?window:{})},{}],20:[function(require,module,exports){module.exports=require("./lib/_stream_passthrough.js")},{"./lib/_stream_passthrough.js":11}],21:[function(require,module,exports){var Stream=function(){try{return require("st"+"ream")}catch(_){}}();exports=module.exports=require("./lib/_stream_readable.js");exports.Stream=Stream||exports;exports.Readable=exports;exports.Writable=require("./lib/_stream_writable.js");exports.Duplex=require("./lib/_stream_duplex.js");exports.Transform=require("./lib/_stream_transform.js");exports.PassThrough=require("./lib/_stream_passthrough.js")},{"./lib/_stream_duplex.js":10,"./lib/_stream_passthrough.js":11,"./lib/_stream_readable.js":12,"./lib/_stream_transform.js":13,"./lib/_stream_writable.js":14}],22:[function(require,module,exports){module.exports=require("./lib/_stream_transform.js")},{"./lib/_stream_transform.js":13}],23:[function(require,module,exports){module.exports=require("./lib/_stream_writable.js")},{"./lib/_stream_writable.js":14}],"stream-browserify":[function(require,module,exports){module.exports=Stream;var EE=require("events").EventEmitter;var inherits=require("inherits");inherits(Stream,EE);Stream.Readable=require("readable-stream/readable.js");Stream.Writable=require("readable-stream/writable.js");Stream.Duplex=require("readable-stream/duplex.js");Stream.Transform=require("readable-stream/transform.js");Stream.PassThrough=require("readable-stream/passthrough.js");Stream.Stream=Stream;function Stream(){EE.call(this)}Stream.prototype.pipe=function(dest,options){var source=this;function ondata(chunk){if(dest.writable){if(false===dest.write(chunk)&&source.pause){source.pause()}}}source.on("data",ondata);function ondrain(){if(source.readable&&source.resume){source.resume(); | |
}}dest.on("drain",ondrain);if(!dest._isStdio&&(!options||options.end!==false)){source.on("end",onend);source.on("close",onclose)}var didOnEnd=false;function onend(){if(didOnEnd)return;didOnEnd=true;dest.end()}function onclose(){if(didOnEnd)return;didOnEnd=true;if(typeof dest.destroy==="function")dest.destroy()}function onerror(er){cleanup();if(EE.listenerCount(this,"error")===0){throw er}}source.on("error",onerror);dest.on("error",onerror);function cleanup(){source.removeListener("data",ondata);dest.removeListener("drain",ondrain);source.removeListener("end",onend);source.removeListener("close",onclose);source.removeListener("error",onerror);dest.removeListener("error",onerror);source.removeListener("end",cleanup);source.removeListener("close",cleanup);dest.removeListener("close",cleanup)}source.on("end",cleanup);source.on("close",cleanup);dest.on("close",cleanup);dest.emit("pipe",source);return dest}},{events:6,inherits:8,"readable-stream/duplex.js":9,"readable-stream/passthrough.js":20,"readable-stream/readable.js":21,"readable-stream/transform.js":22,"readable-stream/writable.js":23}]},{},[]);require=function e(t,n,r){function s(o,u){if(!n[o]){if(!t[o]){var a=typeof require=="function"&&require;if(!u&&a)return a(o,!0);if(i)return i(o,!0);var f=new Error("Cannot find module '"+o+"'");throw f.code="MODULE_NOT_FOUND",f}var l=n[o]={exports:{}};t[o][0].call(l.exports,function(e){var n=t[o][1][e];return s(n?n:e)},l,l.exports,e,t,n,r)}return n[o].exports}var i=typeof require=="function"&&require;for(var o=0;o<r.length;o++)s(r[o]);return s}({1:[function(require,module,exports){},{}],2:[function(require,module,exports){var base64=require("base64-js");var ieee754=require("ieee754");var isArray=require("is-array");exports.Buffer=Buffer;exports.SlowBuffer=SlowBuffer;exports.INSPECT_MAX_BYTES=50;Buffer.poolSize=8192;var kMaxLength=1073741823;var rootParent={};Buffer.TYPED_ARRAY_SUPPORT=function(){try{var buf=new ArrayBuffer(0);var arr=new Uint8Array(buf);arr.foo=function(){return 42};return 42===arr.foo()&&typeof arr.subarray==="function"&&new Uint8Array(1).subarray(1,1).byteLength===0}catch(e){return false}}();function Buffer(subject,encoding,noZero){if(!(this instanceof Buffer))return new Buffer(subject,encoding,noZero);var type=typeof subject;var length;if(type==="number")length=subject>0?subject>>>0:0;else if(type==="string"){length=Buffer.byteLength(subject,encoding)}else if(type==="object"&&subject!==null){if(subject.type==="Buffer"&&isArray(subject.data))subject=subject.data;length=+subject.length>0?Math.floor(+subject.length):0}else throw new TypeError("must start with number, buffer, array or string");if(length>kMaxLength)throw new RangeError("Attempt to allocate Buffer larger than maximum "+"size: 0x"+kMaxLength.toString(16)+" bytes");var buf;if(Buffer.TYPED_ARRAY_SUPPORT){buf=Buffer._augment(new Uint8Array(length))}else{buf=this;buf.length=length;buf._isBuffer=true}var i;if(Buffer.TYPED_ARRAY_SUPPORT&&typeof subject.byteLength==="number"){buf._set(subject)}else if(isArrayish(subject)){if(Buffer.isBuffer(subject)){for(i=0;i<length;i++)buf[i]=subject.readUInt8(i)}else{for(i=0;i<length;i++)buf[i]=(subject[i]%256+256)%256}}else if(type==="string"){buf.write(subject,0,encoding)}else if(type==="number"&&!Buffer.TYPED_ARRAY_SUPPORT&&!noZero){for(i=0;i<length;i++){buf[i]=0}}if(length>0&&length<=Buffer.poolSize)buf.parent=rootParent;return buf}function SlowBuffer(subject,encoding,noZero){if(!(this instanceof SlowBuffer))return new SlowBuffer(subject,encoding,noZero);var buf=new Buffer(subject,encoding,noZero);delete buf.parent;return buf}Buffer.isBuffer=function(b){return!!(b!=null&&b._isBuffer)};Buffer.compare=function(a,b){if(!Buffer.isBuffer(a)||!Buffer.isBuffer(b))throw new TypeError("Arguments must be Buffers");var x=a.length;var y=b.length;for(var i=0,len=Math.min(x,y);i<len&&a[i]===b[i];i++){}if(i!==len){x=a[i];y=b[i]}if(x<y)return-1;if(y<x)return 1;return 0};Buffer.isEncoding=function(encoding){switch(String(encoding).toLowerCase()){case"hex":case"utf8":case"utf-8":case"ascii":case"binary":case"base64":case"raw":case"ucs2":case"ucs-2":case"utf16le":case"utf-16le":return true;default:return false}};Buffer.concat=function(list,totalLength){if(!isArray(list))throw new TypeError("Usage: Buffer.concat(list[, length])");if(list.length===0){return new Buffer(0)}else if(list.length===1){return list[0]}var i;if(totalLength===undefined){totalLength=0;for(i=0;i<list.length;i++){totalLength+=list[i].length}}var buf=new Buffer(totalLength);var pos=0;for(i=0;i<list.length;i++){var item=list[i];item.copy(buf,pos);pos+=item.length}return buf};Buffer.byteLength=function(str,encoding){var ret;str=str+"";switch(encoding||"utf8"){case"ascii":case"binary":case"raw":ret=str.length;break;case"ucs2":case"ucs-2":case"utf16le":case"utf-16le":ret=str.length*2;break;case"hex":ret=str.length>>>1;break;case"utf8":case"utf-8":ret=utf8ToBytes(str).length;break;case"base64":ret=base64ToBytes(str).length;break;default:ret=str.length}return ret};Buffer.prototype.length=undefined;Buffer.prototype.parent=undefined;Buffer.prototype.toString=function(encoding,start,end){var loweredCase=false;start=start>>>0;end=end===undefined||end===Infinity?this.length:end>>>0;if(!encoding)encoding="utf8";if(start<0)start=0;if(end>this.length)end=this.length;if(end<=start)return"";while(true){switch(encoding){case"hex":return hexSlice(this,start,end);case"utf8":case"utf-8":return utf8Slice(this,start,end);case"ascii":return asciiSlice(this,start,end);case"binary":return binarySlice(this,start,end);case"base64":return base64Slice(this,start,end);case"ucs2":case"ucs-2":case"utf16le":case"utf-16le":return utf16leSlice(this,start,end);default:if(loweredCase)throw new TypeError("Unknown encoding: "+encoding);encoding=(encoding+"").toLowerCase();loweredCase=true}}};Buffer.prototype.equals=function(b){if(!Buffer.isBuffer(b))throw new TypeError("Argument must be a Buffer");return Buffer.compare(this,b)===0};Buffer.prototype.inspect=function(){var str="";var max=exports.INSPECT_MAX_BYTES;if(this.length>0){str=this.toString("hex",0,max).match(/.{2}/g).join(" ");if(this.length>max)str+=" ... "}return"<Buffer "+str+">"};Buffer.prototype.compare=function(b){if(!Buffer.isBuffer(b))throw new TypeError("Argument must be a Buffer");return Buffer.compare(this,b)};Buffer.prototype.get=function(offset){console.log(".get() is deprecated. Access using array indexes instead.");return this.readUInt8(offset)};Buffer.prototype.set=function(v,offset){console.log(".set() is deprecated. Access using array indexes instead.");return this.writeUInt8(v,offset)};function hexWrite(buf,string,offset,length){offset=Number(offset)||0;var remaining=buf.length-offset;if(!length){length=remaining}else{length=Number(length);if(length>remaining){length=remaining}}var strLen=string.length;if(strLen%2!==0)throw new Error("Invalid hex string");if(length>strLen/2){length=strLen/2}for(var i=0;i<length;i++){var byte=parseInt(string.substr(i*2,2),16);if(isNaN(byte))throw new Error("Invalid hex string");buf[offset+i]=byte}return i}function utf8Write(buf,string,offset,length){var charsWritten=blitBuffer(utf8ToBytes(string,buf.length-offset),buf,offset,length);return charsWritten}function asciiWrite(buf,string,offset,length){var charsWritten=blitBuffer(asciiToBytes(string),buf,offset,length);return charsWritten}function binaryWrite(buf,string,offset,length){return asciiWrite(buf,string,offset,length)}function base64Write(buf,string,offset,length){var charsWritten=blitBuffer(base64ToBytes(string),buf,offset,length);return charsWritten}function utf16leWrite(buf,string,offset,length){var charsWritten=blitBuffer(utf16leToBytes(string,buf.length-offset),buf,offset,length,2);return charsWritten}Buffer.prototype.write=function(string,offset,length,encoding){if(isFinite(offset)){if(!isFinite(length)){encoding=length;length=undefined}}else{var swap=encoding;encoding=offset;offset=length;length=swap}offset=Number(offset)||0;if(length<0||offset<0||offset>this.length)throw new RangeError("attempt to write outside buffer bounds");var remaining=this.length-offset;if(!length){length=remaining}else{length=Number(length);if(length>remaining){length=remaining}}encoding=String(encoding||"utf8").toLowerCase();var ret;switch(encoding){case"hex":ret=hexWrite(this,string,offset,length);break;case"utf8":case"utf-8":ret=utf8Write(this,string,offset,length);break;case"ascii":ret=asciiWrite(this,string,offset,length);break;case"binary":ret=binaryWrite(this,string,offset,length);break;case"base64":ret=base64Write(this,string,offset,length);break;case"ucs2":case"ucs-2":case"utf16le":case"utf-16le":ret=utf16leWrite(this,string,offset,length);break;default:throw new TypeError("Unknown encoding: "+encoding)}return ret};Buffer.prototype.toJSON=function(){return{type:"Buffer",data:Array.prototype.slice.call(this._arr||this,0)}};function base64Slice(buf,start,end){if(start===0&&end===buf.length){return base64.fromByteArray(buf)}else{return base64.fromByteArray(buf.slice(start,end))}}function utf8Slice(buf,start,end){var res="";var tmp="";end=Math.min(buf.length,end);for(var i=start;i<end;i++){if(buf[i]<=127){res+=decodeUtf8Char(tmp)+String.fromCharCode(buf[i]);tmp=""}else{tmp+="%"+buf[i].toString(16)}}return res+decodeUtf8Char(tmp)}function asciiSlice(buf,start,end){var ret="";end=Math.min(buf.length,end);for(var i=start;i<end;i++){ret+=String.fromCharCode(buf[i]&127)}return ret}function binarySlice(buf,start,end){var ret="";end=Math.min(buf.length,end);for(var i=start;i<end;i++){ret+=String.fromCharCode(buf[i])}return ret}function hexSlice(buf,start,end){var len=buf.length;if(!start||start<0)start=0;if(!end||end<0||end>len)end=len;var out="";for(var i=start;i<end;i++){out+=toHex(buf[i])}return out}function utf16leSlice(buf,start,end){var bytes=buf.slice(start,end);var res="";for(var i=0;i<bytes.length;i+=2){res+=String.fromCharCode(bytes[i]+bytes[i+1]*256)}return res}Buffer.prototype.slice=function(start,end){var len=this.length;start=~~start;end=end===undefined?len:~~end;if(start<0){start+=len;if(start<0)start=0}else if(start>len){start=len}if(end<0){end+=len;if(end<0)end=0}else if(end>len){end=len}if(end<start)end=start;var newBuf;if(Buffer.TYPED_ARRAY_SUPPORT){newBuf=Buffer._augment(this.subarray(start,end))}else{var sliceLen=end-start;newBuf=new Buffer(sliceLen,undefined,true);for(var i=0;i<sliceLen;i++){newBuf[i]=this[i+start]}}if(newBuf.length)newBuf.parent=this.parent||this;return newBuf};function checkOffset(offset,ext,length){if(offset%1!==0||offset<0)throw new RangeError("offset is not uint");if(offset+ext>length)throw new RangeError("Trying to access beyond buffer length")}Buffer.prototype.readUIntLE=function(offset,byteLength,noAssert){offset=offset>>>0;byteLength=byteLength>>>0;if(!noAssert)checkOffset(offset,byteLength,this.length);var val=this[offset];var mul=1;var i=0;while(++i<byteLength&&(mul*=256))val+=this[offset+i]*mul;return val};Buffer.prototype.readUIntBE=function(offset,byteLength,noAssert){offset=offset>>>0;byteLength=byteLength>>>0;if(!noAssert)checkOffset(offset,byteLength,this.length);var val=this[offset+--byteLength];var mul=1;while(byteLength>0&&(mul*=256))val+=this[offset+--byteLength]*mul;return val};Buffer.prototype.readUInt8=function(offset,noAssert){if(!noAssert)checkOffset(offset,1,this.length);return this[offset]};Buffer.prototype.readUInt16LE=function(offset,noAssert){if(!noAssert)checkOffset(offset,2,this.length);return this[offset]|this[offset+1]<<8};Buffer.prototype.readUInt16BE=function(offset,noAssert){if(!noAssert)checkOffset(offset,2,this.length);return this[offset]<<8|this[offset+1]};Buffer.prototype.readUInt32LE=function(offset,noAssert){if(!noAssert)checkOffset(offset,4,this.length);return(this[offset]|this[offset+1]<<8|this[offset+2]<<16)+this[offset+3]*16777216};Buffer.prototype.readUInt32BE=function(offset,noAssert){if(!noAssert)checkOffset(offset,4,this.length);return this[offset]*16777216+(this[offset+1]<<16|this[offset+2]<<8|this[offset+3])};Buffer.prototype.readIntLE=function(offset,byteLength,noAssert){offset=offset>>>0;byteLength=byteLength>>>0;if(!noAssert)checkOffset(offset,byteLength,this.length);var val=this[offset];var mul=1;var i=0;while(++i<byteLength&&(mul*=256))val+=this[offset+i]*mul;mul*=128;if(val>=mul)val-=Math.pow(2,8*byteLength);return val};Buffer.prototype.readIntBE=function(offset,byteLength,noAssert){offset=offset>>>0;byteLength=byteLength>>>0;if(!noAssert)checkOffset(offset,byteLength,this.length);var i=byteLength;var mul=1;var val=this[offset+--i];while(i>0&&(mul*=256))val+=this[offset+--i]*mul;mul*=128;if(val>=mul)val-=Math.pow(2,8*byteLength);return val};Buffer.prototype.readInt8=function(offset,noAssert){if(!noAssert)checkOffset(offset,1,this.length);if(!(this[offset]&128))return this[offset];return(255-this[offset]+1)*-1};Buffer.prototype.readInt16LE=function(offset,noAssert){if(!noAssert)checkOffset(offset,2,this.length);var val=this[offset]|this[offset+1]<<8;return val&32768?val|4294901760:val};Buffer.prototype.readInt16BE=function(offset,noAssert){if(!noAssert)checkOffset(offset,2,this.length);var val=this[offset+1]|this[offset]<<8;return val&32768?val|4294901760:val};Buffer.prototype.readInt32LE=function(offset,noAssert){if(!noAssert)checkOffset(offset,4,this.length);return this[offset]|this[offset+1]<<8|this[offset+2]<<16|this[offset+3]<<24};Buffer.prototype.readInt32BE=function(offset,noAssert){if(!noAssert)checkOffset(offset,4,this.length);return this[offset]<<24|this[offset+1]<<16|this[offset+2]<<8|this[offset+3]};Buffer.prototype.readFloatLE=function(offset,noAssert){if(!noAssert)checkOffset(offset,4,this.length);return ieee754.read(this,offset,true,23,4)};Buffer.prototype.readFloatBE=function(offset,noAssert){if(!noAssert)checkOffset(offset,4,this.length);return ieee754.read(this,offset,false,23,4)};Buffer.prototype.readDoubleLE=function(offset,noAssert){if(!noAssert)checkOffset(offset,8,this.length);return ieee754.read(this,offset,true,52,8)};Buffer.prototype.readDoubleBE=function(offset,noAssert){if(!noAssert)checkOffset(offset,8,this.length);return ieee754.read(this,offset,false,52,8)};function checkInt(buf,value,offset,ext,max,min){if(!Buffer.isBuffer(buf))throw new TypeError("buffer must be a Buffer instance");if(value>max||value<min)throw new RangeError("value is out of bounds");if(offset+ext>buf.length)throw new RangeError("index out of range")}Buffer.prototype.writeUIntLE=function(value,offset,byteLength,noAssert){value=+value;offset=offset>>>0;byteLength=byteLength>>>0;if(!noAssert)checkInt(this,value,offset,byteLength,Math.pow(2,8*byteLength),0);var mul=1;var i=0;this[offset]=value&255;while(++i<byteLength&&(mul*=256))this[offset+i]=value/mul>>>0&255;return offset+byteLength};Buffer.prototype.writeUIntBE=function(value,offset,byteLength,noAssert){value=+value;offset=offset>>>0;byteLength=byteLength>>>0;if(!noAssert)checkInt(this,value,offset,byteLength,Math.pow(2,8*byteLength),0);var i=byteLength-1;var mul=1;this[offset+i]=value&255;while(--i>=0&&(mul*=256))this[offset+i]=value/mul>>>0&255;return offset+byteLength};Buffer.prototype.writeUInt8=function(value,offset,noAssert){value=+value;offset=offset>>>0;if(!noAssert)checkInt(this,value,offset,1,255,0);if(!Buffer.TYPED_ARRAY_SUPPORT)value=Math.floor(value);this[offset]=value;return offset+1};function objectWriteUInt16(buf,value,offset,littleEndian){if(value<0)value=65535+value+1;for(var i=0,j=Math.min(buf.length-offset,2);i<j;i++){buf[offset+i]=(value&255<<8*(littleEndian?i:1-i))>>>(littleEndian?i:1-i)*8}}Buffer.prototype.writeUInt16LE=function(value,offset,noAssert){value=+value;offset=offset>>>0;if(!noAssert)checkInt(this,value,offset,2,65535,0);if(Buffer.TYPED_ARRAY_SUPPORT){this[offset]=value;this[offset+1]=value>>>8}else objectWriteUInt16(this,value,offset,true);return offset+2};Buffer.prototype.writeUInt16BE=function(value,offset,noAssert){value=+value;offset=offset>>>0;if(!noAssert)checkInt(this,value,offset,2,65535,0);if(Buffer.TYPED_ARRAY_SUPPORT){this[offset]=value>>>8;this[offset+1]=value}else objectWriteUInt16(this,value,offset,false);return offset+2};function objectWriteUInt32(buf,value,offset,littleEndian){if(value<0)value=4294967295+value+1;for(var i=0,j=Math.min(buf.length-offset,4);i<j;i++){buf[offset+i]=value>>>(littleEndian?i:3-i)*8&255}}Buffer.prototype.writeUInt32LE=function(value,offset,noAssert){value=+value;offset=offset>>>0;if(!noAssert)checkInt(this,value,offset,4,4294967295,0);if(Buffer.TYPED_ARRAY_SUPPORT){this[offset+3]=value>>>24;this[offset+2]=value>>>16;this[offset+1]=value>>>8;this[offset]=value}else objectWriteUInt32(this,value,offset,true);return offset+4};Buffer.prototype.writeUInt32BE=function(value,offset,noAssert){value=+value;offset=offset>>>0;if(!noAssert)checkInt(this,value,offset,4,4294967295,0);if(Buffer.TYPED_ARRAY_SUPPORT){this[offset]=value>>>24;this[offset+1]=value>>>16;this[offset+2]=value>>>8;this[offset+3]=value}else objectWriteUInt32(this,value,offset,false);return offset+4};Buffer.prototype.writeIntLE=function(value,offset,byteLength,noAssert){value=+value;offset=offset>>>0;if(!noAssert){checkInt(this,value,offset,byteLength,Math.pow(2,8*byteLength-1)-1,-Math.pow(2,8*byteLength-1))}var i=0;var mul=1;var sub=value<0?1:0;this[offset]=value&255;while(++i<byteLength&&(mul*=256))this[offset+i]=(value/mul>>0)-sub&255;return offset+byteLength};Buffer.prototype.writeIntBE=function(value,offset,byteLength,noAssert){value=+value;offset=offset>>>0;if(!noAssert){checkInt(this,value,offset,byteLength,Math.pow(2,8*byteLength-1)-1,-Math.pow(2,8*byteLength-1))}var i=byteLength-1;var mul=1;var sub=value<0?1:0;this[offset+i]=value&255;while(--i>=0&&(mul*=256))this[offset+i]=(value/mul>>0)-sub&255;return offset+byteLength};Buffer.prototype.writeInt8=function(value,offset,noAssert){value=+value;offset=offset>>>0;if(!noAssert)checkInt(this,value,offset,1,127,-128);if(!Buffer.TYPED_ARRAY_SUPPORT)value=Math.floor(value);if(value<0)value=255+value+1;this[offset]=value;return offset+1};Buffer.prototype.writeInt16LE=function(value,offset,noAssert){value=+value;offset=offset>>>0;if(!noAssert)checkInt(this,value,offset,2,32767,-32768);if(Buffer.TYPED_ARRAY_SUPPORT){this[offset]=value;this[offset+1]=value>>>8}else objectWriteUInt16(this,value,offset,true);return offset+2};Buffer.prototype.writeInt16BE=function(value,offset,noAssert){value=+value;offset=offset>>>0;if(!noAssert)checkInt(this,value,offset,2,32767,-32768);if(Buffer.TYPED_ARRAY_SUPPORT){this[offset]=value>>>8;this[offset+1]=value}else objectWriteUInt16(this,value,offset,false);return offset+2};Buffer.prototype.writeInt32LE=function(value,offset,noAssert){value=+value;offset=offset>>>0;if(!noAssert)checkInt(this,value,offset,4,2147483647,-2147483648);if(Buffer.TYPED_ARRAY_SUPPORT){this[offset]=value;this[offset+1]=value>>>8;this[offset+2]=value>>>16;this[offset+3]=value>>>24}else objectWriteUInt32(this,value,offset,true);return offset+4};Buffer.prototype.writeInt32BE=function(value,offset,noAssert){value=+value;offset=offset>>>0;if(!noAssert)checkInt(this,value,offset,4,2147483647,-2147483648);if(value<0)value=4294967295+value+1;if(Buffer.TYPED_ARRAY_SUPPORT){this[offset]=value>>>24;this[offset+1]=value>>>16;this[offset+2]=value>>>8;this[offset+3]=value}else objectWriteUInt32(this,value,offset,false);return offset+4};function checkIEEE754(buf,value,offset,ext,max,min){if(value>max||value<min)throw new RangeError("value is out of bounds");if(offset+ext>buf.length)throw new RangeError("index out of range");if(offset<0)throw new RangeError("index out of range")}function writeFloat(buf,value,offset,littleEndian,noAssert){if(!noAssert)checkIEEE754(buf,value,offset,4,3.4028234663852886e38,-3.4028234663852886e38);ieee754.write(buf,value,offset,littleEndian,23,4);return offset+4}Buffer.prototype.writeFloatLE=function(value,offset,noAssert){return writeFloat(this,value,offset,true,noAssert)};Buffer.prototype.writeFloatBE=function(value,offset,noAssert){return writeFloat(this,value,offset,false,noAssert)};function writeDouble(buf,value,offset,littleEndian,noAssert){if(!noAssert)checkIEEE754(buf,value,offset,8,1.7976931348623157e308,-1.7976931348623157e308);ieee754.write(buf,value,offset,littleEndian,52,8);return offset+8}Buffer.prototype.writeDoubleLE=function(value,offset,noAssert){return writeDouble(this,value,offset,true,noAssert)};Buffer.prototype.writeDoubleBE=function(value,offset,noAssert){return writeDouble(this,value,offset,false,noAssert)};Buffer.prototype.copy=function(target,target_start,start,end){var source=this;if(!start)start=0;if(!end&&end!==0)end=this.length;if(target_start>=target.length)target_start=target.length;if(!target_start)target_start=0;if(end>0&&end<start)end=start;if(end===start)return 0;if(target.length===0||source.length===0)return 0;if(target_start<0)throw new RangeError("targetStart out of bounds");if(start<0||start>=source.length)throw new RangeError("sourceStart out of bounds");if(end<0)throw new RangeError("sourceEnd out of bounds");if(end>this.length)end=this.length;if(target.length-target_start<end-start)end=target.length-target_start+start;var len=end-start;if(len<1e3||!Buffer.TYPED_ARRAY_SUPPORT){for(var i=0;i<len;i++){target[i+target_start]=this[i+start]}}else{target._set(this.subarray(start,start+len),target_start)}return len};Buffer.prototype.fill=function(value,start,end){if(!value)value=0;if(!start)start=0;if(!end)end=this.length;if(end<start)throw new RangeError("end < start");if(end===start)return;if(this.length===0)return;if(start<0||start>=this.length)throw new RangeError("start out of bounds");if(end<0||end>this.length)throw new RangeError("end out of bounds");var i;if(typeof value==="number"){for(i=start;i<end;i++){this[i]=value}}else{var bytes=utf8ToBytes(value.toString());var len=bytes.length;for(i=start;i<end;i++){this[i]=bytes[i%len]}}return this};Buffer.prototype.toArrayBuffer=function(){if(typeof Uint8Array!=="undefined"){if(Buffer.TYPED_ARRAY_SUPPORT){return new Buffer(this).buffer}else{var buf=new Uint8Array(this.length);for(var i=0,len=buf.length;i<len;i+=1){buf[i]=this[i]}return buf.buffer}}else{throw new TypeError("Buffer.toArrayBuffer not supported in this browser")}};var BP=Buffer.prototype;Buffer._augment=function(arr){arr.constructor=Buffer;arr._isBuffer=true;arr._get=arr.get;arr._set=arr.set;arr.get=BP.get;arr.set=BP.set;arr.write=BP.write;arr.toString=BP.toString;arr.toLocaleString=BP.toString;arr.toJSON=BP.toJSON;arr.equals=BP.equals;arr.compare=BP.compare;arr.copy=BP.copy;arr.slice=BP.slice;arr.readUIntLE=BP.readUIntLE;arr.readUIntBE=BP.readUIntBE;arr.readUInt8=BP.readUInt8;arr.readUInt16LE=BP.readUInt16LE;arr.readUInt16BE=BP.readUInt16BE;arr.readUInt32LE=BP.readUInt32LE;arr.readUInt32BE=BP.readUInt32BE;arr.readIntLE=BP.readIntLE;arr.readIntBE=BP.readIntBE;arr.readInt8=BP.readInt8;arr.readInt16LE=BP.readInt16LE;arr.readInt16BE=BP.readInt16BE;arr.readInt32LE=BP.readInt32LE;arr.readInt32BE=BP.readInt32BE;arr.readFloatLE=BP.readFloatLE;arr.readFloatBE=BP.readFloatBE;arr.readDoubleLE=BP.readDoubleLE;arr.readDoubleBE=BP.readDoubleBE;arr.writeUInt8=BP.writeUInt8;arr.writeUIntLE=BP.writeUIntLE;arr.writeUIntBE=BP.writeUIntBE;arr.writeUInt16LE=BP.writeUInt16LE;arr.writeUInt16BE=BP.writeUInt16BE;arr.writeUInt32LE=BP.writeUInt32LE;arr.writeUInt32BE=BP.writeUInt32BE;arr.writeIntLE=BP.writeIntLE;arr.writeIntBE=BP.writeIntBE;arr.writeInt8=BP.writeInt8;arr.writeInt16LE=BP.writeInt16LE;arr.writeInt16BE=BP.writeInt16BE;arr.writeInt32LE=BP.writeInt32LE;arr.writeInt32BE=BP.writeInt32BE;arr.writeFloatLE=BP.writeFloatLE;arr.writeFloatBE=BP.writeFloatBE;arr.writeDoubleLE=BP.writeDoubleLE;arr.writeDoubleBE=BP.writeDoubleBE;arr.fill=BP.fill;arr.inspect=BP.inspect;arr.toArrayBuffer=BP.toArrayBuffer;return arr};var INVALID_BASE64_RE=/[^+\/0-9A-z\-]/g;function base64clean(str){str=stringtrim(str).replace(INVALID_BASE64_RE,"");if(str.length<2)return"";while(str.length%4!==0){str=str+"="}return str}function stringtrim(str){if(str.trim)return str.trim();return str.replace(/^\s+|\s+$/g,"")}function isArrayish(subject){return isArray(subject)||Buffer.isBuffer(subject)||subject&&typeof subject==="object"&&typeof subject.length==="number"}function toHex(n){if(n<16)return"0"+n.toString(16);return n.toString(16)}function utf8ToBytes(string,units){var codePoint,length=string.length;var leadSurrogate=null;units=units||Infinity;var bytes=[];var i=0;for(;i<length;i++){codePoint=string.charCodeAt(i);if(codePoint>55295&&codePoint<57344){if(leadSurrogate){if(codePoint<56320){if((units-=3)>-1)bytes.push(239,191,189);leadSurrogate=codePoint;continue}else{codePoint=leadSurrogate-55296<<10|codePoint-56320|65536;leadSurrogate=null}}else{if(codePoint>56319){if((units-=3)>-1)bytes.push(239,191,189);continue}else if(i+1===length){if((units-=3)>-1)bytes.push(239,191,189);continue}else{leadSurrogate=codePoint;continue}}}else if(leadSurrogate){if((units-=3)>-1)bytes.push(239,191,189);leadSurrogate=null}if(codePoint<128){if((units-=1)<0)break;bytes.push(codePoint)}else if(codePoint<2048){if((units-=2)<0)break;bytes.push(codePoint>>6|192,codePoint&63|128)}else if(codePoint<65536){if((units-=3)<0)break;bytes.push(codePoint>>12|224,codePoint>>6&63|128,codePoint&63|128)}else if(codePoint<2097152){if((units-=4)<0)break;bytes.push(codePoint>>18|240,codePoint>>12&63|128,codePoint>>6&63|128,codePoint&63|128)}else{throw new Error("Invalid code point")}}return bytes}function asciiToBytes(str){var byteArray=[];for(var i=0;i<str.length;i++){byteArray.push(str.charCodeAt(i)&255)}return byteArray}function utf16leToBytes(str,units){var c,hi,lo;var byteArray=[];for(var i=0;i<str.length;i++){if((units-=2)<0)break;c=str.charCodeAt(i);hi=c>>8;lo=c%256;byteArray.push(lo);byteArray.push(hi)}return byteArray}function base64ToBytes(str){return base64.toByteArray(base64clean(str))}function blitBuffer(src,dst,offset,length,unitSize){if(unitSize)length-=length%unitSize;for(var i=0;i<length;i++){if(i+offset>=dst.length||i>=src.length)break;dst[i+offset]=src[i]}return i}function decodeUtf8Char(str){try{return decodeURIComponent(str)}catch(err){return String.fromCharCode(65533)}}},{"base64-js":3,ieee754:4,"is-array":5}],3:[function(require,module,exports){var lookup="ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789+/";(function(exports){"use strict";var Arr=typeof Uint8Array!=="undefined"?Uint8Array:Array;var PLUS="+".charCodeAt(0);var SLASH="/".charCodeAt(0);var NUMBER="0".charCodeAt(0);var LOWER="a".charCodeAt(0);var UPPER="A".charCodeAt(0);var PLUS_URL_SAFE="-".charCodeAt(0);var SLASH_URL_SAFE="_".charCodeAt(0);function decode(elt){var code=elt.charCodeAt(0);if(code===PLUS||code===PLUS_URL_SAFE)return 62;if(code===SLASH||code===SLASH_URL_SAFE)return 63;if(code<NUMBER)return-1;if(code<NUMBER+10)return code-NUMBER+26+26;if(code<UPPER+26)return code-UPPER;if(code<LOWER+26)return code-LOWER+26}function b64ToByteArray(b64){var i,j,l,tmp,placeHolders,arr;if(b64.length%4>0){throw new Error("Invalid string. Length must be a multiple of 4")}var len=b64.length;placeHolders="="===b64.charAt(len-2)?2:"="===b64.charAt(len-1)?1:0;arr=new Arr(b64.length*3/4-placeHolders);l=placeHolders>0?b64.length-4:b64.length;var L=0;function push(v){arr[L++]=v}for(i=0,j=0;i<l;i+=4,j+=3){tmp=decode(b64.charAt(i))<<18|decode(b64.charAt(i+1))<<12|decode(b64.charAt(i+2))<<6|decode(b64.charAt(i+3));push((tmp&16711680)>>16);push((tmp&65280)>>8);push(tmp&255)}if(placeHolders===2){tmp=decode(b64.charAt(i))<<2|decode(b64.charAt(i+1))>>4;push(tmp&255)}else if(placeHolders===1){tmp=decode(b64.charAt(i))<<10|decode(b64.charAt(i+1))<<4|decode(b64.charAt(i+2))>>2;push(tmp>>8&255);push(tmp&255)}return arr}function uint8ToBase64(uint8){var i,extraBytes=uint8.length%3,output="",temp,length;function encode(num){return lookup.charAt(num)}function tripletToBase64(num){return encode(num>>18&63)+encode(num>>12&63)+encode(num>>6&63)+encode(num&63)}for(i=0,length=uint8.length-extraBytes;i<length;i+=3){temp=(uint8[i]<<16)+(uint8[i+1]<<8)+uint8[i+2];output+=tripletToBase64(temp)}switch(extraBytes){case 1:temp=uint8[uint8.length-1];output+=encode(temp>>2);output+=encode(temp<<4&63);output+="==";break;case 2:temp=(uint8[uint8.length-2]<<8)+uint8[uint8.length-1];output+=encode(temp>>10);output+=encode(temp>>4&63);output+=encode(temp<<2&63);output+="=";break}return output}exports.toByteArray=b64ToByteArray;exports.fromByteArray=uint8ToBase64})(typeof exports==="undefined"?this.base64js={}:exports)},{}],4:[function(require,module,exports){exports.read=function(buffer,offset,isLE,mLen,nBytes){var e,m,eLen=nBytes*8-mLen-1,eMax=(1<<eLen)-1,eBias=eMax>>1,nBits=-7,i=isLE?nBytes-1:0,d=isLE?-1:1,s=buffer[offset+i];i+=d;e=s&(1<<-nBits)-1;s>>=-nBits;nBits+=eLen;for(;nBits>0;e=e*256+buffer[offset+i],i+=d,nBits-=8);m=e&(1<<-nBits)-1;e>>=-nBits;nBits+=mLen;for(;nBits>0;m=m*256+buffer[offset+i],i+=d,nBits-=8);if(e===0){e=1-eBias}else if(e===eMax){return m?NaN:(s?-1:1)*Infinity}else{m=m+Math.pow(2,mLen);e=e-eBias}return(s?-1:1)*m*Math.pow(2,e-mLen)};exports.write=function(buffer,value,offset,isLE,mLen,nBytes){var e,m,c,eLen=nBytes*8-mLen-1,eMax=(1<<eLen)-1,eBias=eMax>>1,rt=mLen===23?Math.pow(2,-24)-Math.pow(2,-77):0,i=isLE?0:nBytes-1,d=isLE?1:-1,s=value<0||value===0&&1/value<0?1:0;value=Math.abs(value);if(isNaN(value)||value===Infinity){m=isNaN(value)?1:0;e=eMax}else{e=Math.floor(Math.log(value)/Math.LN2);if(value*(c=Math.pow(2,-e))<1){e--;c*=2}if(e+eBias>=1){value+=rt/c}else{value+=rt*Math.pow(2,1-eBias)}if(value*c>=2){e++;c/=2}if(e+eBias>=eMax){m=0;e=eMax}else if(e+eBias>=1){m=(value*c-1)*Math.pow(2,mLen);e=e+eBias}else{m=value*Math.pow(2,eBias-1)*Math.pow(2,mLen);e=0}}for(;mLen>=8;buffer[offset+i]=m&255,i+=d,m/=256,mLen-=8);e=e<<mLen|m;eLen+=mLen;for(;eLen>0;buffer[offset+i]=e&255,i+=d,e/=256,eLen-=8);buffer[offset+i-d]|=s*128}},{}],5:[function(require,module,exports){var isArray=Array.isArray;var str=Object.prototype.toString;module.exports=isArray||function(val){return!!val&&"[object Array]"==str.call(val)}},{}],6:[function(require,module,exports){function EventEmitter(){this._events=this._events||{};this._maxListeners=this._maxListeners||undefined}module.exports=EventEmitter;EventEmitter.EventEmitter=EventEmitter;EventEmitter.prototype._events=undefined;EventEmitter.prototype._maxListeners=undefined;EventEmitter.defaultMaxListeners=10;EventEmitter.prototype.setMaxListeners=function(n){if(!isNumber(n)||n<0||isNaN(n))throw TypeError("n must be a positive number");this._maxListeners=n;return this};EventEmitter.prototype.emit=function(type){var er,handler,len,args,i,listeners;if(!this._events)this._events={};if(type==="error"){if(!this._events.error||isObject(this._events.error)&&!this._events.error.length){er=arguments[1];if(er instanceof Error){throw er}throw TypeError('Uncaught, unspecified "error" event.')}}handler=this._events[type];if(isUndefined(handler))return false;if(isFunction(handler)){switch(arguments.length){case 1:handler.call(this);break;case 2:handler.call(this,arguments[1]);break;case 3:handler.call(this,arguments[1],arguments[2]);break;default:len=arguments.length;args=new Array(len-1);for(i=1;i<len;i++)args[i-1]=arguments[i];handler.apply(this,args)}}else if(isObject(handler)){len=arguments.length;args=new Array(len-1);for(i=1;i<len;i++)args[i-1]=arguments[i];listeners=handler.slice();len=listeners.length;for(i=0;i<len;i++)listeners[i].apply(this,args)}return true};EventEmitter.prototype.addListener=function(type,listener){var m;if(!isFunction(listener))throw TypeError("listener must be a function");if(!this._events)this._events={};if(this._events.newListener)this.emit("newListener",type,isFunction(listener.listener)?listener.listener:listener);if(!this._events[type])this._events[type]=listener;else if(isObject(this._events[type]))this._events[type].push(listener);else this._events[type]=[this._events[type],listener];if(isObject(this._events[type])&&!this._events[type].warned){var m;if(!isUndefined(this._maxListeners)){m=this._maxListeners}else{m=EventEmitter.defaultMaxListeners}if(m&&m>0&&this._events[type].length>m){this._events[type].warned=true; | |
console.error("(node) warning: possible EventEmitter memory "+"leak detected. %d listeners added. "+"Use emitter.setMaxListeners() to increase limit.",this._events[type].length);if(typeof console.trace==="function"){console.trace()}}}return this};EventEmitter.prototype.on=EventEmitter.prototype.addListener;EventEmitter.prototype.once=function(type,listener){if(!isFunction(listener))throw TypeError("listener must be a function");var fired=false;function g(){this.removeListener(type,g);if(!fired){fired=true;listener.apply(this,arguments)}}g.listener=listener;this.on(type,g);return this};EventEmitter.prototype.removeListener=function(type,listener){var list,position,length,i;if(!isFunction(listener))throw TypeError("listener must be a function");if(!this._events||!this._events[type])return this;list=this._events[type];length=list.length;position=-1;if(list===listener||isFunction(list.listener)&&list.listener===listener){delete this._events[type];if(this._events.removeListener)this.emit("removeListener",type,listener)}else if(isObject(list)){for(i=length;i-->0;){if(list[i]===listener||list[i].listener&&list[i].listener===listener){position=i;break}}if(position<0)return this;if(list.length===1){list.length=0;delete this._events[type]}else{list.splice(position,1)}if(this._events.removeListener)this.emit("removeListener",type,listener)}return this};EventEmitter.prototype.removeAllListeners=function(type){var key,listeners;if(!this._events)return this;if(!this._events.removeListener){if(arguments.length===0)this._events={};else if(this._events[type])delete this._events[type];return this}if(arguments.length===0){for(key in this._events){if(key==="removeListener")continue;this.removeAllListeners(key)}this.removeAllListeners("removeListener");this._events={};return this}listeners=this._events[type];if(isFunction(listeners)){this.removeListener(type,listeners)}else{while(listeners.length)this.removeListener(type,listeners[listeners.length-1])}delete this._events[type];return this};EventEmitter.prototype.listeners=function(type){var ret;if(!this._events||!this._events[type])ret=[];else if(isFunction(this._events[type]))ret=[this._events[type]];else ret=this._events[type].slice();return ret};EventEmitter.listenerCount=function(emitter,type){var ret;if(!emitter._events||!emitter._events[type])ret=0;else if(isFunction(emitter._events[type]))ret=1;else ret=emitter._events[type].length;return ret};function isFunction(arg){return typeof arg==="function"}function isNumber(arg){return typeof arg==="number"}function isObject(arg){return typeof arg==="object"&&arg!==null}function isUndefined(arg){return arg===void 0}},{}],7:[function(require,module,exports){if(typeof Object.create==="function"){module.exports=function inherits(ctor,superCtor){ctor.super_=superCtor;ctor.prototype=Object.create(superCtor.prototype,{constructor:{value:ctor,enumerable:false,writable:true,configurable:true}})}}else{module.exports=function inherits(ctor,superCtor){ctor.super_=superCtor;var TempCtor=function(){};TempCtor.prototype=superCtor.prototype;ctor.prototype=new TempCtor;ctor.prototype.constructor=ctor}}},{}],8:[function(require,module,exports){module.exports=Array.isArray||function(arr){return Object.prototype.toString.call(arr)=="[object Array]"}},{}],9:[function(require,module,exports){var process=module.exports={};process.nextTick=function(){var canSetImmediate=typeof window!=="undefined"&&window.setImmediate;var canMutationObserver=typeof window!=="undefined"&&window.MutationObserver;var canPost=typeof window!=="undefined"&&window.postMessage&&window.addEventListener;if(canSetImmediate){return function(f){return window.setImmediate(f)}}var queue=[];if(canMutationObserver){var hiddenDiv=document.createElement("div");var observer=new MutationObserver(function(){var queueList=queue.slice();queue.length=0;queueList.forEach(function(fn){fn()})});observer.observe(hiddenDiv,{attributes:true});return function nextTick(fn){if(!queue.length){hiddenDiv.setAttribute("yes","no")}queue.push(fn)}}if(canPost){window.addEventListener("message",function(ev){var source=ev.source;if((source===window||source===null)&&ev.data==="process-tick"){ev.stopPropagation();if(queue.length>0){var fn=queue.shift();fn()}}},true);return function nextTick(fn){queue.push(fn);window.postMessage("process-tick","*")}}return function nextTick(fn){setTimeout(fn,0)}}();process.title="browser";process.browser=true;process.env={};process.argv=[];function noop(){}process.on=noop;process.addListener=noop;process.once=noop;process.off=noop;process.removeListener=noop;process.removeAllListeners=noop;process.emit=noop;process.binding=function(name){throw new Error("process.binding is not supported")};process.cwd=function(){return"/"};process.chdir=function(dir){throw new Error("process.chdir is not supported")}},{}],10:[function(require,module,exports){module.exports=require("./lib/_stream_duplex.js")},{"./lib/_stream_duplex.js":11}],11:[function(require,module,exports){(function(process){module.exports=Duplex;var objectKeys=Object.keys||function(obj){var keys=[];for(var key in obj)keys.push(key);return keys};var util=require("core-util-is");util.inherits=require("inherits");var Readable=require("./_stream_readable");var Writable=require("./_stream_writable");util.inherits(Duplex,Readable);forEach(objectKeys(Writable.prototype),function(method){if(!Duplex.prototype[method])Duplex.prototype[method]=Writable.prototype[method]});function Duplex(options){if(!(this instanceof Duplex))return new Duplex(options);Readable.call(this,options);Writable.call(this,options);if(options&&options.readable===false)this.readable=false;if(options&&options.writable===false)this.writable=false;this.allowHalfOpen=true;if(options&&options.allowHalfOpen===false)this.allowHalfOpen=false;this.once("end",onend)}function onend(){if(this.allowHalfOpen||this._writableState.ended)return;process.nextTick(this.end.bind(this))}function forEach(xs,f){for(var i=0,l=xs.length;i<l;i++){f(xs[i],i)}}}).call(this,require("_process"))},{"./_stream_readable":13,"./_stream_writable":15,_process:9,"core-util-is":16,inherits:7}],12:[function(require,module,exports){module.exports=PassThrough;var Transform=require("./_stream_transform");var util=require("core-util-is");util.inherits=require("inherits");util.inherits(PassThrough,Transform);function PassThrough(options){if(!(this instanceof PassThrough))return new PassThrough(options);Transform.call(this,options)}PassThrough.prototype._transform=function(chunk,encoding,cb){cb(null,chunk)}},{"./_stream_transform":14,"core-util-is":16,inherits:7}],13:[function(require,module,exports){(function(process){module.exports=Readable;var isArray=require("isarray");var Buffer=require("buffer").Buffer;Readable.ReadableState=ReadableState;var EE=require("events").EventEmitter;if(!EE.listenerCount)EE.listenerCount=function(emitter,type){return emitter.listeners(type).length};var Stream=require("stream");var util=require("core-util-is");util.inherits=require("inherits");var StringDecoder;util.inherits(Readable,Stream);function ReadableState(options,stream){options=options||{};var hwm=options.highWaterMark;this.highWaterMark=hwm||hwm===0?hwm:16*1024;this.highWaterMark=~~this.highWaterMark;this.buffer=[];this.length=0;this.pipes=null;this.pipesCount=0;this.flowing=false;this.ended=false;this.endEmitted=false;this.reading=false;this.calledRead=false;this.sync=true;this.needReadable=false;this.emittedReadable=false;this.readableListening=false;this.objectMode=!!options.objectMode;this.defaultEncoding=options.defaultEncoding||"utf8";this.ranOut=false;this.awaitDrain=0;this.readingMore=false;this.decoder=null;this.encoding=null;if(options.encoding){if(!StringDecoder)StringDecoder=require("string_decoder/").StringDecoder;this.decoder=new StringDecoder(options.encoding);this.encoding=options.encoding}}function Readable(options){if(!(this instanceof Readable))return new Readable(options);this._readableState=new ReadableState(options,this);this.readable=true;Stream.call(this)}Readable.prototype.push=function(chunk,encoding){var state=this._readableState;if(typeof chunk==="string"&&!state.objectMode){encoding=encoding||state.defaultEncoding;if(encoding!==state.encoding){chunk=new Buffer(chunk,encoding);encoding=""}}return readableAddChunk(this,state,chunk,encoding,false)};Readable.prototype.unshift=function(chunk){var state=this._readableState;return readableAddChunk(this,state,chunk,"",true)};function readableAddChunk(stream,state,chunk,encoding,addToFront){var er=chunkInvalid(state,chunk);if(er){stream.emit("error",er)}else if(chunk===null||chunk===undefined){state.reading=false;if(!state.ended)onEofChunk(stream,state)}else if(state.objectMode||chunk&&chunk.length>0){if(state.ended&&!addToFront){var e=new Error("stream.push() after EOF");stream.emit("error",e)}else if(state.endEmitted&&addToFront){var e=new Error("stream.unshift() after end event");stream.emit("error",e)}else{if(state.decoder&&!addToFront&&!encoding)chunk=state.decoder.write(chunk);state.length+=state.objectMode?1:chunk.length;if(addToFront){state.buffer.unshift(chunk)}else{state.reading=false;state.buffer.push(chunk)}if(state.needReadable)emitReadable(stream);maybeReadMore(stream,state)}}else if(!addToFront){state.reading=false}return needMoreData(state)}function needMoreData(state){return!state.ended&&(state.needReadable||state.length<state.highWaterMark||state.length===0)}Readable.prototype.setEncoding=function(enc){if(!StringDecoder)StringDecoder=require("string_decoder/").StringDecoder;this._readableState.decoder=new StringDecoder(enc);this._readableState.encoding=enc};var MAX_HWM=8388608;function roundUpToNextPowerOf2(n){if(n>=MAX_HWM){n=MAX_HWM}else{n--;for(var p=1;p<32;p<<=1)n|=n>>p;n++}return n}function howMuchToRead(n,state){if(state.length===0&&state.ended)return 0;if(state.objectMode)return n===0?0:1;if(n===null||isNaN(n)){if(state.flowing&&state.buffer.length)return state.buffer[0].length;else return state.length}if(n<=0)return 0;if(n>state.highWaterMark)state.highWaterMark=roundUpToNextPowerOf2(n);if(n>state.length){if(!state.ended){state.needReadable=true;return 0}else return state.length}return n}Readable.prototype.read=function(n){var state=this._readableState;state.calledRead=true;var nOrig=n;var ret;if(typeof n!=="number"||n>0)state.emittedReadable=false;if(n===0&&state.needReadable&&(state.length>=state.highWaterMark||state.ended)){emitReadable(this);return null}n=howMuchToRead(n,state);if(n===0&&state.ended){ret=null;if(state.length>0&&state.decoder){ret=fromList(n,state);state.length-=ret.length}if(state.length===0)endReadable(this);return ret}var doRead=state.needReadable;if(state.length-n<=state.highWaterMark)doRead=true;if(state.ended||state.reading)doRead=false;if(doRead){state.reading=true;state.sync=true;if(state.length===0)state.needReadable=true;this._read(state.highWaterMark);state.sync=false}if(doRead&&!state.reading)n=howMuchToRead(nOrig,state);if(n>0)ret=fromList(n,state);else ret=null;if(ret===null){state.needReadable=true;n=0}state.length-=n;if(state.length===0&&!state.ended)state.needReadable=true;if(state.ended&&!state.endEmitted&&state.length===0)endReadable(this);return ret};function chunkInvalid(state,chunk){var er=null;if(!Buffer.isBuffer(chunk)&&"string"!==typeof chunk&&chunk!==null&&chunk!==undefined&&!state.objectMode){er=new TypeError("Invalid non-string/buffer chunk")}return er}function onEofChunk(stream,state){if(state.decoder&&!state.ended){var chunk=state.decoder.end();if(chunk&&chunk.length){state.buffer.push(chunk);state.length+=state.objectMode?1:chunk.length}}state.ended=true;if(state.length>0)emitReadable(stream);else endReadable(stream)}function emitReadable(stream){var state=stream._readableState;state.needReadable=false;if(state.emittedReadable)return;state.emittedReadable=true;if(state.sync)process.nextTick(function(){emitReadable_(stream)});else emitReadable_(stream)}function emitReadable_(stream){stream.emit("readable")}function maybeReadMore(stream,state){if(!state.readingMore){state.readingMore=true;process.nextTick(function(){maybeReadMore_(stream,state)})}}function maybeReadMore_(stream,state){var len=state.length;while(!state.reading&&!state.flowing&&!state.ended&&state.length<state.highWaterMark){stream.read(0);if(len===state.length)break;else len=state.length}state.readingMore=false}Readable.prototype._read=function(n){this.emit("error",new Error("not implemented"))};Readable.prototype.pipe=function(dest,pipeOpts){var src=this;var state=this._readableState;switch(state.pipesCount){case 0:state.pipes=dest;break;case 1:state.pipes=[state.pipes,dest];break;default:state.pipes.push(dest);break}state.pipesCount+=1;var doEnd=(!pipeOpts||pipeOpts.end!==false)&&dest!==process.stdout&&dest!==process.stderr;var endFn=doEnd?onend:cleanup;if(state.endEmitted)process.nextTick(endFn);else src.once("end",endFn);dest.on("unpipe",onunpipe);function onunpipe(readable){if(readable!==src)return;cleanup()}function onend(){dest.end()}var ondrain=pipeOnDrain(src);dest.on("drain",ondrain);function cleanup(){dest.removeListener("close",onclose);dest.removeListener("finish",onfinish);dest.removeListener("drain",ondrain);dest.removeListener("error",onerror);dest.removeListener("unpipe",onunpipe);src.removeListener("end",onend);src.removeListener("end",cleanup);if(!dest._writableState||dest._writableState.needDrain)ondrain()}function onerror(er){unpipe();dest.removeListener("error",onerror);if(EE.listenerCount(dest,"error")===0)dest.emit("error",er)}if(!dest._events||!dest._events.error)dest.on("error",onerror);else if(isArray(dest._events.error))dest._events.error.unshift(onerror);else dest._events.error=[onerror,dest._events.error];function onclose(){dest.removeListener("finish",onfinish);unpipe()}dest.once("close",onclose);function onfinish(){dest.removeListener("close",onclose);unpipe()}dest.once("finish",onfinish);function unpipe(){src.unpipe(dest)}dest.emit("pipe",src);if(!state.flowing){this.on("readable",pipeOnReadable);state.flowing=true;process.nextTick(function(){flow(src)})}return dest};function pipeOnDrain(src){return function(){var dest=this;var state=src._readableState;state.awaitDrain--;if(state.awaitDrain===0)flow(src)}}function flow(src){var state=src._readableState;var chunk;state.awaitDrain=0;function write(dest,i,list){var written=dest.write(chunk);if(false===written){state.awaitDrain++}}while(state.pipesCount&&null!==(chunk=src.read())){if(state.pipesCount===1)write(state.pipes,0,null);else forEach(state.pipes,write);src.emit("data",chunk);if(state.awaitDrain>0)return}if(state.pipesCount===0){state.flowing=false;if(EE.listenerCount(src,"data")>0)emitDataEvents(src);return}state.ranOut=true}function pipeOnReadable(){if(this._readableState.ranOut){this._readableState.ranOut=false;flow(this)}}Readable.prototype.unpipe=function(dest){var state=this._readableState;if(state.pipesCount===0)return this;if(state.pipesCount===1){if(dest&&dest!==state.pipes)return this;if(!dest)dest=state.pipes;state.pipes=null;state.pipesCount=0;this.removeListener("readable",pipeOnReadable);state.flowing=false;if(dest)dest.emit("unpipe",this);return this}if(!dest){var dests=state.pipes;var len=state.pipesCount;state.pipes=null;state.pipesCount=0;this.removeListener("readable",pipeOnReadable);state.flowing=false;for(var i=0;i<len;i++)dests[i].emit("unpipe",this);return this}var i=indexOf(state.pipes,dest);if(i===-1)return this;state.pipes.splice(i,1);state.pipesCount-=1;if(state.pipesCount===1)state.pipes=state.pipes[0];dest.emit("unpipe",this);return this};Readable.prototype.on=function(ev,fn){var res=Stream.prototype.on.call(this,ev,fn);if(ev==="data"&&!this._readableState.flowing)emitDataEvents(this);if(ev==="readable"&&this.readable){var state=this._readableState;if(!state.readableListening){state.readableListening=true;state.emittedReadable=false;state.needReadable=true;if(!state.reading){this.read(0)}else if(state.length){emitReadable(this,state)}}}return res};Readable.prototype.addListener=Readable.prototype.on;Readable.prototype.resume=function(){emitDataEvents(this);this.read(0);this.emit("resume")};Readable.prototype.pause=function(){emitDataEvents(this,true);this.emit("pause")};function emitDataEvents(stream,startPaused){var state=stream._readableState;if(state.flowing){throw new Error("Cannot switch to old mode now.")}var paused=startPaused||false;var readable=false;stream.readable=true;stream.pipe=Stream.prototype.pipe;stream.on=stream.addListener=Stream.prototype.on;stream.on("readable",function(){readable=true;var c;while(!paused&&null!==(c=stream.read()))stream.emit("data",c);if(c===null){readable=false;stream._readableState.needReadable=true}});stream.pause=function(){paused=true;this.emit("pause")};stream.resume=function(){paused=false;if(readable)process.nextTick(function(){stream.emit("readable")});else this.read(0);this.emit("resume")};stream.emit("readable")}Readable.prototype.wrap=function(stream){var state=this._readableState;var paused=false;var self=this;stream.on("end",function(){if(state.decoder&&!state.ended){var chunk=state.decoder.end();if(chunk&&chunk.length)self.push(chunk)}self.push(null)});stream.on("data",function(chunk){if(state.decoder)chunk=state.decoder.write(chunk);if(state.objectMode&&(chunk===null||chunk===undefined))return;else if(!state.objectMode&&(!chunk||!chunk.length))return;var ret=self.push(chunk);if(!ret){paused=true;stream.pause()}});for(var i in stream){if(typeof stream[i]==="function"&&typeof this[i]==="undefined"){this[i]=function(method){return function(){return stream[method].apply(stream,arguments)}}(i)}}var events=["error","close","destroy","pause","resume"];forEach(events,function(ev){stream.on(ev,self.emit.bind(self,ev))});self._read=function(n){if(paused){paused=false;stream.resume()}};return self};Readable._fromList=fromList;function fromList(n,state){var list=state.buffer;var length=state.length;var stringMode=!!state.decoder;var objectMode=!!state.objectMode;var ret;if(list.length===0)return null;if(length===0)ret=null;else if(objectMode)ret=list.shift();else if(!n||n>=length){if(stringMode)ret=list.join("");else ret=Buffer.concat(list,length);list.length=0}else{if(n<list[0].length){var buf=list[0];ret=buf.slice(0,n);list[0]=buf.slice(n)}else if(n===list[0].length){ret=list.shift()}else{if(stringMode)ret="";else ret=new Buffer(n);var c=0;for(var i=0,l=list.length;i<l&&c<n;i++){var buf=list[0];var cpy=Math.min(n-c,buf.length);if(stringMode)ret+=buf.slice(0,cpy);else buf.copy(ret,c,0,cpy);if(cpy<buf.length)list[0]=buf.slice(cpy);else list.shift();c+=cpy}}}return ret}function endReadable(stream){var state=stream._readableState;if(state.length>0)throw new Error("endReadable called on non-empty stream");if(!state.endEmitted&&state.calledRead){state.ended=true;process.nextTick(function(){if(!state.endEmitted&&state.length===0){state.endEmitted=true;stream.readable=false;stream.emit("end")}})}}function forEach(xs,f){for(var i=0,l=xs.length;i<l;i++){f(xs[i],i)}}function indexOf(xs,x){for(var i=0,l=xs.length;i<l;i++){if(xs[i]===x)return i}return-1}}).call(this,require("_process"))},{_process:9,buffer:2,"core-util-is":16,events:6,inherits:7,isarray:8,stream:21,"string_decoder/":22}],14:[function(require,module,exports){module.exports=Transform;var Duplex=require("./_stream_duplex");var util=require("core-util-is");util.inherits=require("inherits");util.inherits(Transform,Duplex);function TransformState(options,stream){this.afterTransform=function(er,data){return afterTransform(stream,er,data)};this.needTransform=false;this.transforming=false;this.writecb=null;this.writechunk=null}function afterTransform(stream,er,data){var ts=stream._transformState;ts.transforming=false;var cb=ts.writecb;if(!cb)return stream.emit("error",new Error("no writecb in Transform class"));ts.writechunk=null;ts.writecb=null;if(data!==null&&data!==undefined)stream.push(data);if(cb)cb(er);var rs=stream._readableState;rs.reading=false;if(rs.needReadable||rs.length<rs.highWaterMark){stream._read(rs.highWaterMark)}}function Transform(options){if(!(this instanceof Transform))return new Transform(options);Duplex.call(this,options);var ts=this._transformState=new TransformState(options,this);var stream=this;this._readableState.needReadable=true;this._readableState.sync=false;this.once("finish",function(){if("function"===typeof this._flush)this._flush(function(er){done(stream,er)});else done(stream)})}Transform.prototype.push=function(chunk,encoding){this._transformState.needTransform=false;return Duplex.prototype.push.call(this,chunk,encoding)};Transform.prototype._transform=function(chunk,encoding,cb){throw new Error("not implemented")};Transform.prototype._write=function(chunk,encoding,cb){var ts=this._transformState;ts.writecb=cb;ts.writechunk=chunk;ts.writeencoding=encoding;if(!ts.transforming){var rs=this._readableState;if(ts.needTransform||rs.needReadable||rs.length<rs.highWaterMark)this._read(rs.highWaterMark)}};Transform.prototype._read=function(n){var ts=this._transformState;if(ts.writechunk!==null&&ts.writecb&&!ts.transforming){ts.transforming=true;this._transform(ts.writechunk,ts.writeencoding,ts.afterTransform)}else{ts.needTransform=true}};function done(stream,er){if(er)return stream.emit("error",er);var ws=stream._writableState;var rs=stream._readableState;var ts=stream._transformState;if(ws.length)throw new Error("calling transform done when ws.length != 0");if(ts.transforming)throw new Error("calling transform done when still transforming");return stream.push(null)}},{"./_stream_duplex":11,"core-util-is":16,inherits:7}],15:[function(require,module,exports){(function(process){module.exports=Writable;var Buffer=require("buffer").Buffer;Writable.WritableState=WritableState;var util=require("core-util-is");util.inherits=require("inherits");var Stream=require("stream");util.inherits(Writable,Stream);function WriteReq(chunk,encoding,cb){this.chunk=chunk;this.encoding=encoding;this.callback=cb}function WritableState(options,stream){options=options||{};var hwm=options.highWaterMark;this.highWaterMark=hwm||hwm===0?hwm:16*1024;this.objectMode=!!options.objectMode;this.highWaterMark=~~this.highWaterMark;this.needDrain=false;this.ending=false;this.ended=false;this.finished=false;var noDecode=options.decodeStrings===false;this.decodeStrings=!noDecode;this.defaultEncoding=options.defaultEncoding||"utf8";this.length=0;this.writing=false;this.sync=true;this.bufferProcessing=false;this.onwrite=function(er){onwrite(stream,er)};this.writecb=null;this.writelen=0;this.buffer=[];this.errorEmitted=false}function Writable(options){var Duplex=require("./_stream_duplex");if(!(this instanceof Writable)&&!(this instanceof Duplex))return new Writable(options);this._writableState=new WritableState(options,this);this.writable=true;Stream.call(this)}Writable.prototype.pipe=function(){this.emit("error",new Error("Cannot pipe. Not readable."))};function writeAfterEnd(stream,state,cb){var er=new Error("write after end");stream.emit("error",er);process.nextTick(function(){cb(er)})}function validChunk(stream,state,chunk,cb){var valid=true;if(!Buffer.isBuffer(chunk)&&"string"!==typeof chunk&&chunk!==null&&chunk!==undefined&&!state.objectMode){var er=new TypeError("Invalid non-string/buffer chunk");stream.emit("error",er);process.nextTick(function(){cb(er)});valid=false}return valid}Writable.prototype.write=function(chunk,encoding,cb){var state=this._writableState;var ret=false;if(typeof encoding==="function"){cb=encoding;encoding=null}if(Buffer.isBuffer(chunk))encoding="buffer";else if(!encoding)encoding=state.defaultEncoding;if(typeof cb!=="function")cb=function(){};if(state.ended)writeAfterEnd(this,state,cb);else if(validChunk(this,state,chunk,cb))ret=writeOrBuffer(this,state,chunk,encoding,cb);return ret};function decodeChunk(state,chunk,encoding){if(!state.objectMode&&state.decodeStrings!==false&&typeof chunk==="string"){chunk=new Buffer(chunk,encoding)}return chunk}function writeOrBuffer(stream,state,chunk,encoding,cb){chunk=decodeChunk(state,chunk,encoding);if(Buffer.isBuffer(chunk))encoding="buffer";var len=state.objectMode?1:chunk.length;state.length+=len;var ret=state.length<state.highWaterMark;if(!ret)state.needDrain=true;if(state.writing)state.buffer.push(new WriteReq(chunk,encoding,cb));else doWrite(stream,state,len,chunk,encoding,cb);return ret}function doWrite(stream,state,len,chunk,encoding,cb){state.writelen=len;state.writecb=cb;state.writing=true;state.sync=true;stream._write(chunk,encoding,state.onwrite);state.sync=false}function onwriteError(stream,state,sync,er,cb){if(sync)process.nextTick(function(){cb(er)});else cb(er);stream._writableState.errorEmitted=true;stream.emit("error",er)}function onwriteStateUpdate(state){state.writing=false;state.writecb=null;state.length-=state.writelen;state.writelen=0}function onwrite(stream,er){var state=stream._writableState;var sync=state.sync;var cb=state.writecb;onwriteStateUpdate(state);if(er)onwriteError(stream,state,sync,er,cb);else{var finished=needFinish(stream,state);if(!finished&&!state.bufferProcessing&&state.buffer.length)clearBuffer(stream,state);if(sync){process.nextTick(function(){afterWrite(stream,state,finished,cb)})}else{afterWrite(stream,state,finished,cb)}}}function afterWrite(stream,state,finished,cb){if(!finished)onwriteDrain(stream,state);cb();if(finished)finishMaybe(stream,state)}function onwriteDrain(stream,state){if(state.length===0&&state.needDrain){state.needDrain=false;stream.emit("drain")}}function clearBuffer(stream,state){state.bufferProcessing=true;for(var c=0;c<state.buffer.length;c++){var entry=state.buffer[c];var chunk=entry.chunk;var encoding=entry.encoding;var cb=entry.callback;var len=state.objectMode?1:chunk.length;doWrite(stream,state,len,chunk,encoding,cb);if(state.writing){c++;break}}state.bufferProcessing=false;if(c<state.buffer.length)state.buffer=state.buffer.slice(c);else state.buffer.length=0}Writable.prototype._write=function(chunk,encoding,cb){cb(new Error("not implemented"))};Writable.prototype.end=function(chunk,encoding,cb){var state=this._writableState;if(typeof chunk==="function"){cb=chunk;chunk=null;encoding=null}else if(typeof encoding==="function"){cb=encoding;encoding=null}if(typeof chunk!=="undefined"&&chunk!==null)this.write(chunk,encoding);if(!state.ending&&!state.finished)endWritable(this,state,cb)};function needFinish(stream,state){return state.ending&&state.length===0&&!state.finished&&!state.writing}function finishMaybe(stream,state){var need=needFinish(stream,state);if(need){state.finished=true;stream.emit("finish")}return need}function endWritable(stream,state,cb){state.ending=true;finishMaybe(stream,state);if(cb){if(state.finished)process.nextTick(cb);else stream.once("finish",cb)}state.ended=true}}).call(this,require("_process"))},{"./_stream_duplex":11,_process:9,buffer:2,"core-util-is":16,inherits:7,stream:21}],16:[function(require,module,exports){(function(Buffer){function isArray(ar){return Array.isArray(ar)}exports.isArray=isArray;function isBoolean(arg){return typeof arg==="boolean"}exports.isBoolean=isBoolean;function isNull(arg){return arg===null}exports.isNull=isNull;function isNullOrUndefined(arg){return arg==null}exports.isNullOrUndefined=isNullOrUndefined;function isNumber(arg){return typeof arg==="number"}exports.isNumber=isNumber;function isString(arg){return typeof arg==="string"}exports.isString=isString;function isSymbol(arg){return typeof arg==="symbol"}exports.isSymbol=isSymbol;function isUndefined(arg){return arg===void 0}exports.isUndefined=isUndefined;function isRegExp(re){return isObject(re)&&objectToString(re)==="[object RegExp]"}exports.isRegExp=isRegExp;function isObject(arg){return typeof arg==="object"&&arg!==null}exports.isObject=isObject;function isDate(d){return isObject(d)&&objectToString(d)==="[object Date]"}exports.isDate=isDate;function isError(e){return isObject(e)&&(objectToString(e)==="[object Error]"||e instanceof Error)}exports.isError=isError;function isFunction(arg){return typeof arg==="function"}exports.isFunction=isFunction;function isPrimitive(arg){return arg===null||typeof arg==="boolean"||typeof arg==="number"||typeof arg==="string"||typeof arg==="symbol"||typeof arg==="undefined"}exports.isPrimitive=isPrimitive;function isBuffer(arg){return Buffer.isBuffer(arg)}exports.isBuffer=isBuffer;function objectToString(o){return Object.prototype.toString.call(o)}}).call(this,require("buffer").Buffer)},{buffer:2}],17:[function(require,module,exports){module.exports=require("./lib/_stream_passthrough.js")},{"./lib/_stream_passthrough.js":12}],18:[function(require,module,exports){var Stream=require("stream");exports=module.exports=require("./lib/_stream_readable.js");exports.Stream=Stream;exports.Readable=exports;exports.Writable=require("./lib/_stream_writable.js");exports.Duplex=require("./lib/_stream_duplex.js");exports.Transform=require("./lib/_stream_transform.js");exports.PassThrough=require("./lib/_stream_passthrough.js")},{"./lib/_stream_duplex.js":11,"./lib/_stream_passthrough.js":12,"./lib/_stream_readable.js":13,"./lib/_stream_transform.js":14,"./lib/_stream_writable.js":15,stream:21}],19:[function(require,module,exports){module.exports=require("./lib/_stream_transform.js")},{"./lib/_stream_transform.js":14}],20:[function(require,module,exports){module.exports=require("./lib/_stream_writable.js")},{"./lib/_stream_writable.js":15}],21:[function(require,module,exports){module.exports=Stream;var EE=require("events").EventEmitter;var inherits=require("inherits");inherits(Stream,EE);Stream.Readable=require("readable-stream/readable.js");Stream.Writable=require("readable-stream/writable.js");Stream.Duplex=require("readable-stream/duplex.js");Stream.Transform=require("readable-stream/transform.js");Stream.PassThrough=require("readable-stream/passthrough.js");Stream.Stream=Stream;function Stream(){EE.call(this)}Stream.prototype.pipe=function(dest,options){var source=this;function ondata(chunk){if(dest.writable){if(false===dest.write(chunk)&&source.pause){source.pause()}}}source.on("data",ondata);function ondrain(){if(source.readable&&source.resume){source.resume()}}dest.on("drain",ondrain);if(!dest._isStdio&&(!options||options.end!==false)){source.on("end",onend);source.on("close",onclose)}var didOnEnd=false;function onend(){if(didOnEnd)return;didOnEnd=true;dest.end()}function onclose(){if(didOnEnd)return;didOnEnd=true;if(typeof dest.destroy==="function")dest.destroy()}function onerror(er){cleanup();if(EE.listenerCount(this,"error")===0){throw er}}source.on("error",onerror);dest.on("error",onerror);function cleanup(){source.removeListener("data",ondata);dest.removeListener("drain",ondrain);source.removeListener("end",onend);source.removeListener("close",onclose);source.removeListener("error",onerror);dest.removeListener("error",onerror);source.removeListener("end",cleanup);source.removeListener("close",cleanup);dest.removeListener("close",cleanup)}source.on("end",cleanup);source.on("close",cleanup);dest.on("close",cleanup);dest.emit("pipe",source);return dest}},{events:6,inherits:7,"readable-stream/duplex.js":10,"readable-stream/passthrough.js":17,"readable-stream/readable.js":18,"readable-stream/transform.js":19,"readable-stream/writable.js":20}],22:[function(require,module,exports){var Buffer=require("buffer").Buffer;var isBufferEncoding=Buffer.isEncoding||function(encoding){switch(encoding&&encoding.toLowerCase()){case"hex":case"utf8":case"utf-8":case"ascii":case"binary":case"base64":case"ucs2":case"ucs-2":case"utf16le":case"utf-16le":case"raw":return true;default:return false}};function assertEncoding(encoding){if(encoding&&!isBufferEncoding(encoding)){throw new Error("Unknown encoding: "+encoding)}}var StringDecoder=exports.StringDecoder=function(encoding){this.encoding=(encoding||"utf8").toLowerCase().replace(/[-_]/,"");assertEncoding(encoding);switch(this.encoding){case"utf8":this.surrogateSize=3;break;case"ucs2":case"utf16le":this.surrogateSize=2;this.detectIncompleteChar=utf16DetectIncompleteChar;break;case"base64": | |
this.surrogateSize=3;this.detectIncompleteChar=base64DetectIncompleteChar;break;default:this.write=passThroughWrite;return}this.charBuffer=new Buffer(6);this.charReceived=0;this.charLength=0};StringDecoder.prototype.write=function(buffer){var charStr="";while(this.charLength){var available=buffer.length>=this.charLength-this.charReceived?this.charLength-this.charReceived:buffer.length;buffer.copy(this.charBuffer,this.charReceived,0,available);this.charReceived+=available;if(this.charReceived<this.charLength){return""}buffer=buffer.slice(available,buffer.length);charStr=this.charBuffer.slice(0,this.charLength).toString(this.encoding);var charCode=charStr.charCodeAt(charStr.length-1);if(charCode>=55296&&charCode<=56319){this.charLength+=this.surrogateSize;charStr="";continue}this.charReceived=this.charLength=0;if(buffer.length===0){return charStr}break}this.detectIncompleteChar(buffer);var end=buffer.length;if(this.charLength){buffer.copy(this.charBuffer,0,buffer.length-this.charReceived,end);end-=this.charReceived}charStr+=buffer.toString(this.encoding,0,end);var end=charStr.length-1;var charCode=charStr.charCodeAt(end);if(charCode>=55296&&charCode<=56319){var size=this.surrogateSize;this.charLength+=size;this.charReceived+=size;this.charBuffer.copy(this.charBuffer,size,0,size);buffer.copy(this.charBuffer,0,0,size);return charStr.substring(0,end)}return charStr};StringDecoder.prototype.detectIncompleteChar=function(buffer){var i=buffer.length>=3?3:buffer.length;for(;i>0;i--){var c=buffer[buffer.length-i];if(i==1&&c>>5==6){this.charLength=2;break}if(i<=2&&c>>4==14){this.charLength=3;break}if(i<=3&&c>>3==30){this.charLength=4;break}}this.charReceived=i};StringDecoder.prototype.end=function(buffer){var res="";if(buffer&&buffer.length)res=this.write(buffer);if(this.charReceived){var cr=this.charReceived;var buf=this.charBuffer;var enc=this.encoding;res+=buf.slice(0,cr).toString(enc)}return res};function passThroughWrite(buffer){return buffer.toString(this.encoding)}function utf16DetectIncompleteChar(buffer){this.charReceived=buffer.length%2;this.charLength=this.charReceived?2:0}function base64DetectIncompleteChar(buffer){this.charReceived=buffer.length%3;this.charLength=this.charReceived?3:0}},{buffer:2}],23:[function(require,module,exports){module.exports=function isBuffer(arg){return arg&&typeof arg==="object"&&typeof arg.copy==="function"&&typeof arg.fill==="function"&&typeof arg.readUInt8==="function"}},{}],24:[function(require,module,exports){(function(process,global){var formatRegExp=/%[sdj%]/g;exports.format=function(f){if(!isString(f)){var objects=[];for(var i=0;i<arguments.length;i++){objects.push(inspect(arguments[i]))}return objects.join(" ")}var i=1;var args=arguments;var len=args.length;var str=String(f).replace(formatRegExp,function(x){if(x==="%%")return"%";if(i>=len)return x;switch(x){case"%s":return String(args[i++]);case"%d":return Number(args[i++]);case"%j":try{return JSON.stringify(args[i++])}catch(_){return"[Circular]"}default:return x}});for(var x=args[i];i<len;x=args[++i]){if(isNull(x)||!isObject(x)){str+=" "+x}else{str+=" "+inspect(x)}}return str};exports.deprecate=function(fn,msg){if(isUndefined(global.process)){return function(){return exports.deprecate(fn,msg).apply(this,arguments)}}if(process.noDeprecation===true){return fn}var warned=false;function deprecated(){if(!warned){if(process.throwDeprecation){throw new Error(msg)}else if(process.traceDeprecation){console.trace(msg)}else{console.error(msg)}warned=true}return fn.apply(this,arguments)}return deprecated};var debugs={};var debugEnviron;exports.debuglog=function(set){if(isUndefined(debugEnviron))debugEnviron=process.env.NODE_DEBUG||"";set=set.toUpperCase();if(!debugs[set]){if(new RegExp("\\b"+set+"\\b","i").test(debugEnviron)){var pid=process.pid;debugs[set]=function(){var msg=exports.format.apply(exports,arguments);console.error("%s %d: %s",set,pid,msg)}}else{debugs[set]=function(){}}}return debugs[set]};function inspect(obj,opts){var ctx={seen:[],stylize:stylizeNoColor};if(arguments.length>=3)ctx.depth=arguments[2];if(arguments.length>=4)ctx.colors=arguments[3];if(isBoolean(opts)){ctx.showHidden=opts}else if(opts){exports._extend(ctx,opts)}if(isUndefined(ctx.showHidden))ctx.showHidden=false;if(isUndefined(ctx.depth))ctx.depth=2;if(isUndefined(ctx.colors))ctx.colors=false;if(isUndefined(ctx.customInspect))ctx.customInspect=true;if(ctx.colors)ctx.stylize=stylizeWithColor;return formatValue(ctx,obj,ctx.depth)}exports.inspect=inspect;inspect.colors={bold:[1,22],italic:[3,23],underline:[4,24],inverse:[7,27],white:[37,39],grey:[90,39],black:[30,39],blue:[34,39],cyan:[36,39],green:[32,39],magenta:[35,39],red:[31,39],yellow:[33,39]};inspect.styles={special:"cyan",number:"yellow","boolean":"yellow",undefined:"grey","null":"bold",string:"green",date:"magenta",regexp:"red"};function stylizeWithColor(str,styleType){var style=inspect.styles[styleType];if(style){return"["+inspect.colors[style][0]+"m"+str+"["+inspect.colors[style][1]+"m"}else{return str}}function stylizeNoColor(str,styleType){return str}function arrayToHash(array){var hash={};array.forEach(function(val,idx){hash[val]=true});return hash}function formatValue(ctx,value,recurseTimes){if(ctx.customInspect&&value&&isFunction(value.inspect)&&value.inspect!==exports.inspect&&!(value.constructor&&value.constructor.prototype===value)){var ret=value.inspect(recurseTimes,ctx);if(!isString(ret)){ret=formatValue(ctx,ret,recurseTimes)}return ret}var primitive=formatPrimitive(ctx,value);if(primitive){return primitive}var keys=Object.keys(value);var visibleKeys=arrayToHash(keys);if(ctx.showHidden){keys=Object.getOwnPropertyNames(value)}if(isError(value)&&(keys.indexOf("message")>=0||keys.indexOf("description")>=0)){return formatError(value)}if(keys.length===0){if(isFunction(value)){var name=value.name?": "+value.name:"";return ctx.stylize("[Function"+name+"]","special")}if(isRegExp(value)){return ctx.stylize(RegExp.prototype.toString.call(value),"regexp")}if(isDate(value)){return ctx.stylize(Date.prototype.toString.call(value),"date")}if(isError(value)){return formatError(value)}}var base="",array=false,braces=["{","}"];if(isArray(value)){array=true;braces=["[","]"]}if(isFunction(value)){var n=value.name?": "+value.name:"";base=" [Function"+n+"]"}if(isRegExp(value)){base=" "+RegExp.prototype.toString.call(value)}if(isDate(value)){base=" "+Date.prototype.toUTCString.call(value)}if(isError(value)){base=" "+formatError(value)}if(keys.length===0&&(!array||value.length==0)){return braces[0]+base+braces[1]}if(recurseTimes<0){if(isRegExp(value)){return ctx.stylize(RegExp.prototype.toString.call(value),"regexp")}else{return ctx.stylize("[Object]","special")}}ctx.seen.push(value);var output;if(array){output=formatArray(ctx,value,recurseTimes,visibleKeys,keys)}else{output=keys.map(function(key){return formatProperty(ctx,value,recurseTimes,visibleKeys,key,array)})}ctx.seen.pop();return reduceToSingleString(output,base,braces)}function formatPrimitive(ctx,value){if(isUndefined(value))return ctx.stylize("undefined","undefined");if(isString(value)){var simple="'"+JSON.stringify(value).replace(/^"|"$/g,"").replace(/'/g,"\\'").replace(/\\"/g,'"')+"'";return ctx.stylize(simple,"string")}if(isNumber(value))return ctx.stylize(""+value,"number");if(isBoolean(value))return ctx.stylize(""+value,"boolean");if(isNull(value))return ctx.stylize("null","null")}function formatError(value){return"["+Error.prototype.toString.call(value)+"]"}function formatArray(ctx,value,recurseTimes,visibleKeys,keys){var output=[];for(var i=0,l=value.length;i<l;++i){if(hasOwnProperty(value,String(i))){output.push(formatProperty(ctx,value,recurseTimes,visibleKeys,String(i),true))}else{output.push("")}}keys.forEach(function(key){if(!key.match(/^\d+$/)){output.push(formatProperty(ctx,value,recurseTimes,visibleKeys,key,true))}});return output}function formatProperty(ctx,value,recurseTimes,visibleKeys,key,array){var name,str,desc;desc=Object.getOwnPropertyDescriptor(value,key)||{value:value[key]};if(desc.get){if(desc.set){str=ctx.stylize("[Getter/Setter]","special")}else{str=ctx.stylize("[Getter]","special")}}else{if(desc.set){str=ctx.stylize("[Setter]","special")}}if(!hasOwnProperty(visibleKeys,key)){name="["+key+"]"}if(!str){if(ctx.seen.indexOf(desc.value)<0){if(isNull(recurseTimes)){str=formatValue(ctx,desc.value,null)}else{str=formatValue(ctx,desc.value,recurseTimes-1)}if(str.indexOf("\n")>-1){if(array){str=str.split("\n").map(function(line){return" "+line}).join("\n").substr(2)}else{str="\n"+str.split("\n").map(function(line){return" "+line}).join("\n")}}}else{str=ctx.stylize("[Circular]","special")}}if(isUndefined(name)){if(array&&key.match(/^\d+$/)){return str}name=JSON.stringify(""+key);if(name.match(/^"([a-zA-Z_][a-zA-Z_0-9]*)"$/)){name=name.substr(1,name.length-2);name=ctx.stylize(name,"name")}else{name=name.replace(/'/g,"\\'").replace(/\\"/g,'"').replace(/(^"|"$)/g,"'");name=ctx.stylize(name,"string")}}return name+": "+str}function reduceToSingleString(output,base,braces){var numLinesEst=0;var length=output.reduce(function(prev,cur){numLinesEst++;if(cur.indexOf("\n")>=0)numLinesEst++;return prev+cur.replace(/\u001b\[\d\d?m/g,"").length+1},0);if(length>60){return braces[0]+(base===""?"":base+"\n ")+" "+output.join(",\n ")+" "+braces[1]}return braces[0]+base+" "+output.join(", ")+" "+braces[1]}function isArray(ar){return Array.isArray(ar)}exports.isArray=isArray;function isBoolean(arg){return typeof arg==="boolean"}exports.isBoolean=isBoolean;function isNull(arg){return arg===null}exports.isNull=isNull;function isNullOrUndefined(arg){return arg==null}exports.isNullOrUndefined=isNullOrUndefined;function isNumber(arg){return typeof arg==="number"}exports.isNumber=isNumber;function isString(arg){return typeof arg==="string"}exports.isString=isString;function isSymbol(arg){return typeof arg==="symbol"}exports.isSymbol=isSymbol;function isUndefined(arg){return arg===void 0}exports.isUndefined=isUndefined;function isRegExp(re){return isObject(re)&&objectToString(re)==="[object RegExp]"}exports.isRegExp=isRegExp;function isObject(arg){return typeof arg==="object"&&arg!==null}exports.isObject=isObject;function isDate(d){return isObject(d)&&objectToString(d)==="[object Date]"}exports.isDate=isDate;function isError(e){return isObject(e)&&(objectToString(e)==="[object Error]"||e instanceof Error)}exports.isError=isError;function isFunction(arg){return typeof arg==="function"}exports.isFunction=isFunction;function isPrimitive(arg){return arg===null||typeof arg==="boolean"||typeof arg==="number"||typeof arg==="string"||typeof arg==="symbol"||typeof arg==="undefined"}exports.isPrimitive=isPrimitive;exports.isBuffer=require("./support/isBuffer");function objectToString(o){return Object.prototype.toString.call(o)}function pad(n){return n<10?"0"+n.toString(10):n.toString(10)}var months=["Jan","Feb","Mar","Apr","May","Jun","Jul","Aug","Sep","Oct","Nov","Dec"];function timestamp(){var d=new Date;var time=[pad(d.getHours()),pad(d.getMinutes()),pad(d.getSeconds())].join(":");return[d.getDate(),months[d.getMonth()],time].join(" ")}exports.log=function(){console.log("%s - %s",timestamp(),exports.format.apply(exports,arguments))};exports.inherits=require("inherits");exports._extend=function(origin,add){if(!add||!isObject(add))return origin;var keys=Object.keys(add);var i=keys.length;while(i--){origin[keys[i]]=add[keys[i]]}return origin};function hasOwnProperty(obj,prop){return Object.prototype.hasOwnProperty.call(obj,prop)}}).call(this,require("_process"),typeof global!=="undefined"?global:typeof self!=="undefined"?self:typeof window!=="undefined"?window:{})},{"./support/isBuffer":23,_process:9,inherits:7}],25:[function(require,module,exports){var util=require("./util"),WriteError=require("level-errors").WriteError,getOptions=util.getOptions,dispatchError=util.dispatchError;function Batch(levelup,codec){this._levelup=levelup;this._codec=codec;this.batch=levelup.db.batch();this.ops=[];this.length=0}Batch.prototype.put=function(key_,value_,options){options=getOptions(options);var key=this._codec.encodeKey(key_,options),value=this._codec.encodeValue(value_,options);try{this.batch.put(key,value)}catch(e){throw new WriteError(e)}this.ops.push({type:"put",key:key,value:value});this.length++;return this};Batch.prototype.del=function(key_,options){options=getOptions(options);var key=this._codec.encodeKey(key_,options);try{this.batch.del(key)}catch(err){throw new WriteError(err)}this.ops.push({type:"del",key:key});this.length++;return this};Batch.prototype.clear=function(){try{this.batch.clear()}catch(err){throw new WriteError(err)}this.ops=[];this.length=0;return this};Batch.prototype.write=function(callback){var levelup=this._levelup,ops=this.ops;try{this.batch.write(function(err){if(err)return dispatchError(levelup,new WriteError(err),callback);levelup.emit("batch",ops);if(callback)callback()})}catch(err){throw new WriteError(err)}};module.exports=Batch},{"./util":27,"level-errors":37}],26:[function(require,module,exports){(function(process){var EventEmitter=require("events").EventEmitter,inherits=require("util").inherits,deprecate=require("util").deprecate,extend=require("xtend"),prr=require("prr"),DeferredLevelDOWN=require("deferred-leveldown"),IteratorStream=require("level-iterator-stream"),errors=require("level-errors"),WriteError=errors.WriteError,ReadError=errors.ReadError,NotFoundError=errors.NotFoundError,OpenError=errors.OpenError,EncodingError=errors.EncodingError,InitializationError=errors.InitializationError,util=require("./util"),Batch=require("./batch"),Codec=require("level-codec"),getOptions=util.getOptions,defaultOptions=util.defaultOptions,getLevelDOWN=util.getLevelDOWN,dispatchError=util.dispatchError,isDefined=util.isDefined;function getCallback(options,callback){return typeof options=="function"?options:callback}function LevelUP(location,options,callback){if(!(this instanceof LevelUP))return new LevelUP(location,options,callback);var error;EventEmitter.call(this);this.setMaxListeners(Infinity);if(typeof location=="function"){options=typeof options=="object"?options:{};options.db=location;location=null}else if(typeof location=="object"&&typeof location.db=="function"){options=location;location=null}if(typeof options=="function"){callback=options;options={}}if((!options||typeof options.db!="function")&&typeof location!="string"){error=new InitializationError("Must provide a location for the database");if(callback){return process.nextTick(function(){callback(error)})}throw error}options=getOptions(options);this.options=extend(defaultOptions,options);this._codec=new Codec(this.options);this._status="new";prr(this,"location",location,"e");this.open(callback)}inherits(LevelUP,EventEmitter);LevelUP.prototype.open=function(callback){var self=this,dbFactory,db;if(this.isOpen()){if(callback)process.nextTick(function(){callback(null,self)});return this}if(this._isOpening()){return callback&&this.once("open",function(){callback(null,self)})}this.emit("opening");this._status="opening";this.db=new DeferredLevelDOWN(this.location);dbFactory=this.options.db||getLevelDOWN();db=dbFactory(this.location);db.open(this.options,function(err){if(err){return dispatchError(self,new OpenError(err),callback)}else{self.db.setDb(db);self.db=db;self._status="open";if(callback)callback(null,self);self.emit("open");self.emit("ready")}})};LevelUP.prototype.close=function(callback){var self=this;if(this.isOpen()){this._status="closing";this.db.close(function(){self._status="closed";self.emit("closed");if(callback)callback.apply(null,arguments)});this.emit("closing");this.db=new DeferredLevelDOWN(this.location)}else if(this._status=="closed"&&callback){return process.nextTick(callback)}else if(this._status=="closing"&&callback){this.once("closed",callback)}else if(this._isOpening()){this.once("open",function(){self.close(callback)})}};LevelUP.prototype.isOpen=function(){return this._status=="open"};LevelUP.prototype._isOpening=function(){return this._status=="opening"};LevelUP.prototype.isClosed=function(){return/^clos/.test(this._status)};function maybeError(db,options,callback){if(!db._isOpening()&&!db.isOpen()){dispatchError(db,new ReadError("Database is not open"),callback);return true}}function writeError(db,message,callback){dispatchError(db,new WriteError(message),callback)}function readError(db,message,callback){dispatchError(db,new ReadError(message),callback)}LevelUP.prototype.get=function(key_,options,callback){var self=this,key;callback=getCallback(options,callback);if(maybeError(this,options,callback))return;if(key_===null||key_===undefined||"function"!==typeof callback)return readError(this,"get() requires key and callback arguments",callback);options=util.getOptions(options);key=this._codec.encodeKey(key_,options);options.asBuffer=this._codec.valueAsBuffer(options);this.db.get(key,options,function(err,value){if(err){if(/notfound/i.test(err)||err.notFound){err=new NotFoundError("Key not found in database ["+key_+"]",err)}else{err=new ReadError(err)}return dispatchError(self,err,callback)}if(callback){try{value=self._codec.decodeValue(value,options)}catch(e){return callback(new EncodingError(e))}callback(null,value)}})};LevelUP.prototype.put=function(key_,value_,options,callback){var self=this,key,value;callback=getCallback(options,callback);if(key_===null||key_===undefined)return writeError(this,"put() requires a key argument",callback);if(maybeError(this,options,callback))return;options=getOptions(options);key=this._codec.encodeKey(key_,options);value=this._codec.encodeValue(value_,options);this.db.put(key,value,options,function(err){if(err){return dispatchError(self,new WriteError(err),callback)}else{self.emit("put",key_,value_);if(callback)callback()}})};LevelUP.prototype.del=function(key_,options,callback){var self=this,key;callback=getCallback(options,callback);if(key_===null||key_===undefined)return writeError(this,"del() requires a key argument",callback);if(maybeError(this,options,callback))return;options=getOptions(options);key=this._codec.encodeKey(key_,options);this.db.del(key,options,function(err){if(err){return dispatchError(self,new WriteError(err),callback)}else{self.emit("del",key_);if(callback)callback()}})};LevelUP.prototype.batch=function(arr_,options,callback){var self=this,keyEnc,valueEnc,arr;if(!arguments.length)return new Batch(this,this._codec);callback=getCallback(options,callback);if(!Array.isArray(arr_))return writeError(this,"batch() requires an array argument",callback);if(maybeError(this,options,callback))return;options=getOptions(options);arr=self._codec.encodeBatch(arr_,options);arr=arr.map(function(op){if(!op.type&&op.key!==undefined&&op.value!==undefined)op.type="put";return op});this.db.batch(arr,options,function(err){if(err){return dispatchError(self,new WriteError(err),callback)}else{self.emit("batch",arr_);if(callback)callback()}})};LevelUP.prototype.approximateSize=deprecate(function(start_,end_,options,callback){var self=this,start,end;callback=getCallback(options,callback);options=getOptions(options);if(start_===null||start_===undefined||end_===null||end_===undefined||"function"!==typeof callback)return readError(this,"approximateSize() requires start, end and callback arguments",callback);start=this._codec.encodeKey(start_,options);end=this._codec.encodeKey(end_,options);this.db.approximateSize(start,end,function(err,size){if(err){return dispatchError(self,new OpenError(err),callback)}else if(callback){callback(null,size)}})},"db.approximateSize() is deprecated. Use db.db.approximateSize() instead");LevelUP.prototype.readStream=LevelUP.prototype.createReadStream=function(options){options=extend({keys:true,values:true},this.options,options);options.keyEncoding=options.keyEncoding;options.valueEncoding=options.valueEncoding;options=this._codec.encodeLtgt(options);options.keyAsBuffer=this._codec.keyAsBuffer(options);options.valueAsBuffer=this._codec.valueAsBuffer(options);if("number"!==typeof options.limit)options.limit=-1;return new IteratorStream(this.db.iterator(options),extend(options,{decoder:this._codec.createStreamDecoder(options)}))};LevelUP.prototype.keyStream=LevelUP.prototype.createKeyStream=function(options){return this.createReadStream(extend(options,{keys:true,values:false}))};LevelUP.prototype.valueStream=LevelUP.prototype.createValueStream=function(options){return this.createReadStream(extend(options,{keys:false,values:true}))};LevelUP.prototype.toString=function(){return"LevelUP"};function utilStatic(name){return function(location,callback){getLevelDOWN()[name](location,callback||function(){})}}module.exports=LevelUP;module.exports.errors=require("level-errors");module.exports.destroy=deprecate(utilStatic("destroy"),"levelup.destroy() is deprecated. Use leveldown.destroy() instead");module.exports.repair=deprecate(utilStatic("repair"),"levelup.repair() is deprecated. Use leveldown.repair() instead")}).call(this,require("_process"))},{"./batch":25,"./util":27,_process:9,"deferred-leveldown":29,events:6,"level-codec":35,"level-errors":37,"level-iterator-stream":41,prr:52,util:24,xtend:53}],27:[function(require,module,exports){var extend=require("xtend"),LevelUPError=require("level-errors").LevelUPError,format=require("util").format,defaultOptions={createIfMissing:true,errorIfExists:false,keyEncoding:"utf8",valueEncoding:"utf8",compression:true},leveldown;function getOptions(options){if(typeof options=="string")options={valueEncoding:options};if(typeof options!="object")options={};return options}function getLevelDOWN(){if(leveldown)return leveldown;var requiredVersion=require("../package.json").devDependencies.leveldown,leveldownVersion;try{leveldownVersion=require("leveldown/package").version}catch(e){throw requireError(e)}if(!require("semver").satisfies(leveldownVersion,requiredVersion)){throw new LevelUPError("Installed version of LevelDOWN ("+leveldownVersion+") does not match required version ("+requiredVersion+")")}try{return leveldown=require("leveldown")}catch(e){throw requireError(e)}}function requireError(e){var template="Failed to require LevelDOWN (%s). Try `npm install leveldown` if it's missing";return new LevelUPError(format(template,e.message))}function dispatchError(db,error,callback){typeof callback=="function"?callback(error):db.emit("error",error)}function isDefined(v){return typeof v!=="undefined"}module.exports={defaultOptions:defaultOptions,getOptions:getOptions,getLevelDOWN:getLevelDOWN,dispatchError:dispatchError,isDefined:isDefined}},{"../package.json":54,"level-errors":37,leveldown:1,"leveldown/package":1,semver:1,util:24,xtend:53}],28:[function(require,module,exports){var util=require("util"),AbstractIterator=require("abstract-leveldown").AbstractIterator;function DeferredIterator(options){AbstractIterator.call(this,options);this._options=options;this._iterator=null;this._operations=[]}util.inherits(DeferredIterator,AbstractIterator);DeferredIterator.prototype.setDb=function(db){var it=this._iterator=db.iterator(this._options);this._operations.forEach(function(op){it[op.method].apply(it,op.args)})};DeferredIterator.prototype._operation=function(method,args){if(this._iterator)return this._iterator[method].apply(this._iterator,args);this._operations.push({method:method,args:args})};"next end".split(" ").forEach(function(m){DeferredIterator.prototype["_"+m]=function(){this._operation(m,arguments)}});module.exports=DeferredIterator},{"abstract-leveldown":33,util:24}],29:[function(require,module,exports){(function(process,Buffer){var util=require("util"),AbstractLevelDOWN=require("abstract-leveldown").AbstractLevelDOWN,DeferredIterator=require("./deferred-iterator");function DeferredLevelDOWN(location){AbstractLevelDOWN.call(this,typeof location=="string"?location:"");this._db=undefined;this._operations=[];this._iterators=[]}util.inherits(DeferredLevelDOWN,AbstractLevelDOWN);DeferredLevelDOWN.prototype.setDb=function(db){this._db=db;this._operations.forEach(function(op){db[op.method].apply(db,op.args)});this._iterators.forEach(function(it){it.setDb(db)})};DeferredLevelDOWN.prototype._open=function(options,callback){return process.nextTick(callback)};DeferredLevelDOWN.prototype._operation=function(method,args){if(this._db)return this._db[method].apply(this._db,args);this._operations.push({method:method,args:args})};"put get del batch approximateSize".split(" ").forEach(function(m){DeferredLevelDOWN.prototype["_"+m]=function(){this._operation(m,arguments)}});DeferredLevelDOWN.prototype._isBuffer=function(obj){return Buffer.isBuffer(obj)};DeferredLevelDOWN.prototype._iterator=function(options){if(this._db)return this._db.iterator.apply(this._db,arguments);var it=new DeferredIterator(options);this._iterators.push(it);return it};module.exports=DeferredLevelDOWN;module.exports.DeferredIterator=DeferredIterator}).call(this,require("_process"),require("buffer").Buffer)},{"./deferred-iterator":28,_process:9,"abstract-leveldown":33,buffer:2,util:24}],30:[function(require,module,exports){(function(process){function AbstractChainedBatch(db){this._db=db;this._operations=[];this._written=false}AbstractChainedBatch.prototype._checkWritten=function(){if(this._written)throw new Error("write() already called on this batch")};AbstractChainedBatch.prototype.put=function(key,value){this._checkWritten();var err=this._db._checkKey(key,"key",this._db._isBuffer);if(err)throw err;if(!this._db._isBuffer(key))key=String(key);if(!this._db._isBuffer(value))value=String(value);if(typeof this._put=="function")this._put(key,value);else this._operations.push({type:"put",key:key,value:value});return this};AbstractChainedBatch.prototype.del=function(key){this._checkWritten();var err=this._db._checkKey(key,"key",this._db._isBuffer);if(err)throw err;if(!this._db._isBuffer(key))key=String(key);if(typeof this._del=="function")this._del(key);else this._operations.push({type:"del",key:key});return this};AbstractChainedBatch.prototype.clear=function(){this._checkWritten();this._operations=[];if(typeof this._clear=="function")this._clear();return this};AbstractChainedBatch.prototype.write=function(options,callback){this._checkWritten();if(typeof options=="function")callback=options;if(typeof callback!="function")throw new Error("write() requires a callback argument");if(typeof options!="object")options={};this._written=true;if(typeof this._write=="function")return this._write(callback);if(typeof this._db._batch=="function")return this._db._batch(this._operations,options,callback);process.nextTick(callback)};module.exports=AbstractChainedBatch}).call(this,require("_process"))},{_process:9}],31:[function(require,module,exports){(function(process){function AbstractIterator(db){this.db=db;this._ended=false;this._nexting=false}AbstractIterator.prototype.next=function(callback){var self=this;if(typeof callback!="function")throw new Error("next() requires a callback argument");if(self._ended)return callback(new Error("cannot call next() after end()"));if(self._nexting)return callback(new Error("cannot call next() before previous next() has completed"));self._nexting=true;if(typeof self._next=="function"){return self._next(function(){self._nexting=false;callback.apply(null,arguments)})}process.nextTick(function(){self._nexting=false;callback()})};AbstractIterator.prototype.end=function(callback){if(typeof callback!="function")throw new Error("end() requires a callback argument");if(this._ended)return callback(new Error("end() already called on iterator"));this._ended=true;if(typeof this._end=="function")return this._end(callback);process.nextTick(callback)};module.exports=AbstractIterator}).call(this,require("_process"))},{_process:9}],32:[function(require,module,exports){(function(process,Buffer){var xtend=require("xtend"),AbstractIterator=require("./abstract-iterator"),AbstractChainedBatch=require("./abstract-chained-batch");function AbstractLevelDOWN(location){if(!arguments.length||location===undefined)throw new Error("constructor requires at least a location argument");if(typeof location!="string")throw new Error("constructor requires a location string argument");this.location=location;this.status="new"}AbstractLevelDOWN.prototype.open=function(options,callback){var self=this,oldStatus=this.status;if(typeof options=="function")callback=options;if(typeof callback!="function")throw new Error("open() requires a callback argument");if(typeof options!="object")options={};options.createIfMissing=options.createIfMissing!=false;options.errorIfExists=!!options.errorIfExists;if(typeof this._open=="function"){this.status="opening";this._open(options,function(err){if(err){self.status=oldStatus;return callback(err)}self.status="open";callback()})}else{this.status="open";process.nextTick(callback)}};AbstractLevelDOWN.prototype.close=function(callback){var self=this,oldStatus=this.status;if(typeof callback!="function")throw new Error("close() requires a callback argument");if(typeof this._close=="function"){this.status="closing";this._close(function(err){if(err){self.status=oldStatus;return callback(err)}self.status="closed";callback()})}else{this.status="closed";process.nextTick(callback)}};AbstractLevelDOWN.prototype.get=function(key,options,callback){var err;if(typeof options=="function")callback=options;if(typeof callback!="function")throw new Error("get() requires a callback argument");if(err=this._checkKey(key,"key",this._isBuffer))return callback(err);if(!this._isBuffer(key))key=String(key);if(typeof options!="object")options={};options.asBuffer=options.asBuffer!=false;if(typeof this._get=="function")return this._get(key,options,callback);process.nextTick(function(){callback(new Error("NotFound"))})};AbstractLevelDOWN.prototype.put=function(key,value,options,callback){var err;if(typeof options=="function")callback=options;if(typeof callback!="function")throw new Error("put() requires a callback argument");if(err=this._checkKey(key,"key",this._isBuffer))return callback(err);if(!this._isBuffer(key))key=String(key);if(value!=null&&!this._isBuffer(value)&&!process.browser)value=String(value);if(typeof options!="object")options={};if(typeof this._put=="function")return this._put(key,value,options,callback);process.nextTick(callback)};AbstractLevelDOWN.prototype.del=function(key,options,callback){var err;if(typeof options=="function")callback=options;if(typeof callback!="function")throw new Error("del() requires a callback argument");if(err=this._checkKey(key,"key",this._isBuffer))return callback(err);if(!this._isBuffer(key))key=String(key);if(typeof options!="object")options={};if(typeof this._del=="function")return this._del(key,options,callback);process.nextTick(callback)};AbstractLevelDOWN.prototype.batch=function(array,options,callback){if(!arguments.length)return this._chainedBatch();if(typeof options=="function")callback=options;if(typeof array=="function")callback=array;if(typeof callback!="function")throw new Error("batch(array) requires a callback argument");if(!Array.isArray(array))return callback(new Error("batch(array) requires an array argument"));if(!options||typeof options!="object")options={};var i=0,l=array.length,e,err;for(;i<l;i++){e=array[i];if(typeof e!="object")continue;if(err=this._checkKey(e.type,"type",this._isBuffer))return callback(err);if(err=this._checkKey(e.key,"key",this._isBuffer))return callback(err)}if(typeof this._batch=="function")return this._batch(array,options,callback);process.nextTick(callback)};AbstractLevelDOWN.prototype.approximateSize=function(start,end,callback){if(start==null||end==null||typeof start=="function"||typeof end=="function"){throw new Error("approximateSize() requires valid `start`, `end` and `callback` arguments")}if(typeof callback!="function")throw new Error("approximateSize() requires a callback argument");if(!this._isBuffer(start))start=String(start);if(!this._isBuffer(end))end=String(end);if(typeof this._approximateSize=="function")return this._approximateSize(start,end,callback);process.nextTick(function(){callback(null,0)})};AbstractLevelDOWN.prototype._setupIteratorOptions=function(options){ | |
var self=this;options=xtend(options);["start","end","gt","gte","lt","lte"].forEach(function(o){if(options[o]&&self._isBuffer(options[o])&&options[o].length===0)delete options[o]});options.reverse=!!options.reverse;options.keys=options.keys!=false;options.values=options.values!=false;options.limit="limit"in options?options.limit:-1;options.keyAsBuffer=options.keyAsBuffer!=false;options.valueAsBuffer=options.valueAsBuffer!=false;return options};AbstractLevelDOWN.prototype.iterator=function(options){if(typeof options!="object")options={};options=this._setupIteratorOptions(options);if(typeof this._iterator=="function")return this._iterator(options);return new AbstractIterator(this)};AbstractLevelDOWN.prototype._chainedBatch=function(){return new AbstractChainedBatch(this)};AbstractLevelDOWN.prototype._isBuffer=function(obj){return Buffer.isBuffer(obj)};AbstractLevelDOWN.prototype._checkKey=function(obj,type){if(obj===null||obj===undefined)return new Error(type+" cannot be `null` or `undefined`");if(this._isBuffer(obj)){if(obj.length===0)return new Error(type+" cannot be an empty Buffer")}else if(String(obj)==="")return new Error(type+" cannot be an empty String")};module.exports=AbstractLevelDOWN}).call(this,require("_process"),require("buffer").Buffer)},{"./abstract-chained-batch":30,"./abstract-iterator":31,_process:9,buffer:2,xtend:53}],33:[function(require,module,exports){exports.AbstractLevelDOWN=require("./abstract-leveldown");exports.AbstractIterator=require("./abstract-iterator");exports.AbstractChainedBatch=require("./abstract-chained-batch");exports.isLevelDOWN=require("./is-leveldown")},{"./abstract-chained-batch":30,"./abstract-iterator":31,"./abstract-leveldown":32,"./is-leveldown":34}],34:[function(require,module,exports){var AbstractLevelDOWN=require("./abstract-leveldown");function isLevelDOWN(db){if(!db||typeof db!=="object")return false;return Object.keys(AbstractLevelDOWN.prototype).filter(function(name){return name[0]!="_"&&name!="approximateSize"}).every(function(name){return typeof db[name]=="function"})}module.exports=isLevelDOWN},{"./abstract-leveldown":32}],35:[function(require,module,exports){var encodings=require("./lib/encodings");module.exports=Codec;function Codec(opts){this.opts=opts||{};this.encodings=encodings}Codec.prototype._encoding=function(encoding){if(typeof encoding=="string")encoding=encodings[encoding];if(!encoding)encoding=encodings.id;return encoding};Codec.prototype._keyEncoding=function(opts,batchOpts){return this._encoding(batchOpts&&batchOpts.keyEncoding||opts&&opts.keyEncoding||this.opts.keyEncoding)};Codec.prototype._valueEncoding=function(opts,batchOpts){return this._encoding(batchOpts&&(batchOpts.valueEncoding||batchOpts.encoding)||opts&&(opts.valueEncoding||opts.encoding)||(this.opts.valueEncoding||this.opts.encoding))};Codec.prototype.encodeKey=function(key,opts,batchOpts){return this._keyEncoding(opts,batchOpts).encode(key)};Codec.prototype.encodeValue=function(value,opts,batchOpts){return this._valueEncoding(opts,batchOpts).encode(value)};Codec.prototype.decodeKey=function(key,opts){return this._keyEncoding(opts).decode(key)};Codec.prototype.decodeValue=function(value,opts){return this._valueEncoding(opts).decode(value)};Codec.prototype.encodeBatch=function(ops,opts){var self=this;return ops.map(function(_op){var op={type:_op.type,key:self.encodeKey(_op.key,opts,_op)};if(self.keyAsBuffer(opts,_op))op.keyEncoding="binary";if(_op.prefix)op.prefix=_op.prefix;if("value"in _op){op.value=self.encodeValue(_op.value,opts,_op);if(self.valueAsBuffer(opts,_op))op.valueEncoding="binary"}return op})};var ltgtKeys=["lt","gt","lte","gte","start","end"];Codec.prototype.encodeLtgt=function(ltgt){var self=this;var ret={};Object.keys(ltgt).forEach(function(key){ret[key]=ltgtKeys.indexOf(key)>-1?self.encodeKey(ltgt[key],ltgt):ltgt[key]});return ret};Codec.prototype.createStreamDecoder=function(opts){var self=this;if(opts.keys&&opts.values){return function(key,value){return{key:self.decodeKey(key,opts),value:self.decodeValue(value,opts)}}}else if(opts.keys){return function(key){return self.decodeKey(key,opts)}}else if(opts.values){return function(_,value){return self.decodeValue(value,opts)}}else{return function(){}}};Codec.prototype.keyAsBuffer=function(opts){return this._keyEncoding(opts).buffer};Codec.prototype.valueAsBuffer=function(opts){return this._valueEncoding(opts).buffer}},{"./lib/encodings":36}],36:[function(require,module,exports){(function(Buffer){exports.utf8=exports["utf-8"]={encode:function(data){return isBinary(data)?data:String(data)},decode:identity,buffer:false,type:"utf8"};exports.json={encode:JSON.stringify,decode:JSON.parse,buffer:false,type:"json"};exports.binary={encode:function(data){return isBinary(data)?data:new Buffer(data)},decode:identity,buffer:true,type:"binary"};exports.id={encode:function(data){return data},decode:function(data){return data},buffer:false,type:"id"};var bufferEncodings=["hex","ascii","base64","ucs2","ucs-2","utf16le","utf-16le"];bufferEncodings.forEach(function(type){exports[type]={encode:function(data){return isBinary(data)?data:new Buffer(data,type)},decode:function(buffer){return buffer.toString(type)},buffer:true,type:type}});function identity(value){return value}function isBinary(data){return data===undefined||data===null||Buffer.isBuffer(data)}}).call(this,require("buffer").Buffer)},{buffer:2}],37:[function(require,module,exports){var createError=require("errno").create,LevelUPError=createError("LevelUPError"),NotFoundError=createError("NotFoundError",LevelUPError);NotFoundError.prototype.notFound=true;NotFoundError.prototype.status=404;module.exports={LevelUPError:LevelUPError,InitializationError:createError("InitializationError",LevelUPError),OpenError:createError("OpenError",LevelUPError),ReadError:createError("ReadError",LevelUPError),WriteError:createError("WriteError",LevelUPError),NotFoundError:NotFoundError,EncodingError:createError("EncodingError",LevelUPError)}},{errno:39}],38:[function(require,module,exports){var prr=require("prr");function init(type,message,cause){prr(this,{type:type,name:type,cause:typeof message!="string"?message:cause,message:!!message&&typeof message!="string"?message.message:message},"ewr")}function CustomError(message,cause){Error.call(this);if(Error.captureStackTrace)Error.captureStackTrace(this,arguments.callee);init.call(this,"CustomError",message,cause)}CustomError.prototype=new Error;function createError(errno,type,proto){var err=function(message,cause){init.call(this,type,message,cause);if(type=="FilesystemError"){this.code=this.cause.code;this.path=this.cause.path;this.errno=this.cause.errno;this.message=(errno.errno[this.cause.errno]?errno.errno[this.cause.errno].description:this.cause.message)+(this.cause.path?" ["+this.cause.path+"]":"")}Error.call(this);if(Error.captureStackTrace)Error.captureStackTrace(this,arguments.callee)};err.prototype=!!proto?new proto:new CustomError;return err}module.exports=function(errno){var ce=function(type,proto){return createError(errno,type,proto)};return{CustomError:CustomError,FilesystemError:ce("FilesystemError"),createError:ce}}},{prr:40}],39:[function(require,module,exports){var all=module.exports.all=[{errno:-2,code:"ENOENT",description:"no such file or directory"},{errno:-1,code:"UNKNOWN",description:"unknown error"},{errno:0,code:"OK",description:"success"},{errno:1,code:"EOF",description:"end of file"},{errno:2,code:"EADDRINFO",description:"getaddrinfo error"},{errno:3,code:"EACCES",description:"permission denied"},{errno:4,code:"EAGAIN",description:"resource temporarily unavailable"},{errno:5,code:"EADDRINUSE",description:"address already in use"},{errno:6,code:"EADDRNOTAVAIL",description:"address not available"},{errno:7,code:"EAFNOSUPPORT",description:"address family not supported"},{errno:8,code:"EALREADY",description:"connection already in progress"},{errno:9,code:"EBADF",description:"bad file descriptor"},{errno:10,code:"EBUSY",description:"resource busy or locked"},{errno:11,code:"ECONNABORTED",description:"software caused connection abort"},{errno:12,code:"ECONNREFUSED",description:"connection refused"},{errno:13,code:"ECONNRESET",description:"connection reset by peer"},{errno:14,code:"EDESTADDRREQ",description:"destination address required"},{errno:15,code:"EFAULT",description:"bad address in system call argument"},{errno:16,code:"EHOSTUNREACH",description:"host is unreachable"},{errno:17,code:"EINTR",description:"interrupted system call"},{errno:18,code:"EINVAL",description:"invalid argument"},{errno:19,code:"EISCONN",description:"socket is already connected"},{errno:20,code:"EMFILE",description:"too many open files"},{errno:21,code:"EMSGSIZE",description:"message too long"},{errno:22,code:"ENETDOWN",description:"network is down"},{errno:23,code:"ENETUNREACH",description:"network is unreachable"},{errno:24,code:"ENFILE",description:"file table overflow"},{errno:25,code:"ENOBUFS",description:"no buffer space available"},{errno:26,code:"ENOMEM",description:"not enough memory"},{errno:27,code:"ENOTDIR",description:"not a directory"},{errno:28,code:"EISDIR",description:"illegal operation on a directory"},{errno:29,code:"ENONET",description:"machine is not on the network"},{errno:31,code:"ENOTCONN",description:"socket is not connected"},{errno:32,code:"ENOTSOCK",description:"socket operation on non-socket"},{errno:33,code:"ENOTSUP",description:"operation not supported on socket"},{errno:34,code:"ENOENT",description:"no such file or directory"},{errno:35,code:"ENOSYS",description:"function not implemented"},{errno:36,code:"EPIPE",description:"broken pipe"},{errno:37,code:"EPROTO",description:"protocol error"},{errno:38,code:"EPROTONOSUPPORT",description:"protocol not supported"},{errno:39,code:"EPROTOTYPE",description:"protocol wrong type for socket"},{errno:40,code:"ETIMEDOUT",description:"connection timed out"},{errno:41,code:"ECHARSET",description:"invalid Unicode character"},{errno:42,code:"EAIFAMNOSUPPORT",description:"address family for hostname not supported"},{errno:44,code:"EAISERVICE",description:"servname not supported for ai_socktype"},{errno:45,code:"EAISOCKTYPE",description:"ai_socktype not supported"},{errno:46,code:"ESHUTDOWN",description:"cannot send after transport endpoint shutdown"},{errno:47,code:"EEXIST",description:"file already exists"},{errno:48,code:"ESRCH",description:"no such process"},{errno:49,code:"ENAMETOOLONG",description:"name too long"},{errno:50,code:"EPERM",description:"operation not permitted"},{errno:51,code:"ELOOP",description:"too many symbolic links encountered"},{errno:52,code:"EXDEV",description:"cross-device link not permitted"},{errno:53,code:"ENOTEMPTY",description:"directory not empty"},{errno:54,code:"ENOSPC",description:"no space left on device"},{errno:55,code:"EIO",description:"i/o error"},{errno:56,code:"EROFS",description:"read-only file system"},{errno:57,code:"ENODEV",description:"no such device"},{errno:58,code:"ESPIPE",description:"invalid seek"},{errno:59,code:"ECANCELED",description:"operation canceled"}];module.exports.errno={};module.exports.code={};all.forEach(function(error){module.exports.errno[error.errno]=error;module.exports.code[error.code]=error});module.exports.custom=require("./custom")(module.exports);module.exports.create=module.exports.custom.createError},{"./custom":38}],40:[function(require,module,exports){(function(name,context,definition){if(typeof module!="undefined"&&module.exports)module.exports=definition();else context[name]=definition()})("prr",this,function(){var setProperty=typeof Object.defineProperty=="function"?function(obj,key,options){Object.defineProperty(obj,key,options);return obj}:function(obj,key,options){obj[key]=options.value;return obj},makeOptions=function(value,options){var oo=typeof options=="object",os=!oo&&typeof options=="string",op=function(p){return oo?!!options[p]:os?options.indexOf(p[0])>-1:false};return{enumerable:op("enumerable"),configurable:op("configurable"),writable:op("writable"),value:value}},prr=function(obj,key,value,options){var k;options=makeOptions(value,options);if(typeof key=="object"){for(k in key){if(Object.hasOwnProperty.call(key,k)){options.value=key[k];setProperty(obj,k,options)}}return obj}return setProperty(obj,key,options)};return prr})},{}],41:[function(require,module,exports){var inherits=require("inherits");var Readable=require("readable-stream").Readable;var extend=require("xtend");var EncodingError=require("level-errors").EncodingError;module.exports=ReadStream;inherits(ReadStream,Readable);function ReadStream(iterator,options){if(!(this instanceof ReadStream))return new ReadStream(iterator,options);Readable.call(this,extend(options,{objectMode:true}));this._iterator=iterator;this._destroyed=false;this._decoder=null;if(options&&options.decoder)this._decoder=options.decoder;this.on("end",this._cleanup.bind(this))}ReadStream.prototype._read=function(){var self=this;if(this._destroyed)return;this._iterator.next(function(err,key,value){if(self._destroyed)return;if(err)return self.emit("error",err);if(key===undefined&&value===undefined){self.push(null)}else{if(!self._decoder)return self.push({key:key,value:value});try{var value=self._decoder(key,value)}catch(err){self.emit("error",new EncodingError(err));self.push(null);return}self.push(value)}})};ReadStream.prototype.destroy=ReadStream.prototype._cleanup=function(){var self=this;if(this._destroyed)return;this._destroyed=true;this._iterator.end(function(err){if(err)return self.emit("error",err);self.emit("close")})}},{inherits:42,"level-errors":37,"readable-stream":51,xtend:53}],42:[function(require,module,exports){arguments[4][7][0].apply(exports,arguments)},{dup:7}],43:[function(require,module,exports){(function(process){module.exports=Duplex;var objectKeys=Object.keys||function(obj){var keys=[];for(var key in obj)keys.push(key);return keys};var util=require("core-util-is");util.inherits=require("inherits");var Readable=require("./_stream_readable");var Writable=require("./_stream_writable");util.inherits(Duplex,Readable);forEach(objectKeys(Writable.prototype),function(method){if(!Duplex.prototype[method])Duplex.prototype[method]=Writable.prototype[method]});function Duplex(options){if(!(this instanceof Duplex))return new Duplex(options);Readable.call(this,options);Writable.call(this,options);if(options&&options.readable===false)this.readable=false;if(options&&options.writable===false)this.writable=false;this.allowHalfOpen=true;if(options&&options.allowHalfOpen===false)this.allowHalfOpen=false;this.once("end",onend)}function onend(){if(this.allowHalfOpen||this._writableState.ended)return;process.nextTick(this.end.bind(this))}function forEach(xs,f){for(var i=0,l=xs.length;i<l;i++){f(xs[i],i)}}}).call(this,require("_process"))},{"./_stream_readable":45,"./_stream_writable":47,_process:9,"core-util-is":48,inherits:42}],44:[function(require,module,exports){arguments[4][12][0].apply(exports,arguments)},{"./_stream_transform":46,"core-util-is":48,dup:12,inherits:42}],45:[function(require,module,exports){(function(process){module.exports=Readable;var isArray=require("isarray");var Buffer=require("buffer").Buffer;Readable.ReadableState=ReadableState;var EE=require("events").EventEmitter;if(!EE.listenerCount)EE.listenerCount=function(emitter,type){return emitter.listeners(type).length};var Stream=require("stream");var util=require("core-util-is");util.inherits=require("inherits");var StringDecoder;var debug=require("util");if(debug&&debug.debuglog){debug=debug.debuglog("stream")}else{debug=function(){}}util.inherits(Readable,Stream);function ReadableState(options,stream){var Duplex=require("./_stream_duplex");options=options||{};var hwm=options.highWaterMark;var defaultHwm=options.objectMode?16:16*1024;this.highWaterMark=hwm||hwm===0?hwm:defaultHwm;this.highWaterMark=~~this.highWaterMark;this.buffer=[];this.length=0;this.pipes=null;this.pipesCount=0;this.flowing=null;this.ended=false;this.endEmitted=false;this.reading=false;this.sync=true;this.needReadable=false;this.emittedReadable=false;this.readableListening=false;this.objectMode=!!options.objectMode;if(stream instanceof Duplex)this.objectMode=this.objectMode||!!options.readableObjectMode;this.defaultEncoding=options.defaultEncoding||"utf8";this.ranOut=false;this.awaitDrain=0;this.readingMore=false;this.decoder=null;this.encoding=null;if(options.encoding){if(!StringDecoder)StringDecoder=require("string_decoder/").StringDecoder;this.decoder=new StringDecoder(options.encoding);this.encoding=options.encoding}}function Readable(options){var Duplex=require("./_stream_duplex");if(!(this instanceof Readable))return new Readable(options);this._readableState=new ReadableState(options,this);this.readable=true;Stream.call(this)}Readable.prototype.push=function(chunk,encoding){var state=this._readableState;if(util.isString(chunk)&&!state.objectMode){encoding=encoding||state.defaultEncoding;if(encoding!==state.encoding){chunk=new Buffer(chunk,encoding);encoding=""}}return readableAddChunk(this,state,chunk,encoding,false)};Readable.prototype.unshift=function(chunk){var state=this._readableState;return readableAddChunk(this,state,chunk,"",true)};function readableAddChunk(stream,state,chunk,encoding,addToFront){var er=chunkInvalid(state,chunk);if(er){stream.emit("error",er)}else if(util.isNullOrUndefined(chunk)){state.reading=false;if(!state.ended)onEofChunk(stream,state)}else if(state.objectMode||chunk&&chunk.length>0){if(state.ended&&!addToFront){var e=new Error("stream.push() after EOF");stream.emit("error",e)}else if(state.endEmitted&&addToFront){var e=new Error("stream.unshift() after end event");stream.emit("error",e)}else{if(state.decoder&&!addToFront&&!encoding)chunk=state.decoder.write(chunk);if(!addToFront)state.reading=false;if(state.flowing&&state.length===0&&!state.sync){stream.emit("data",chunk);stream.read(0)}else{state.length+=state.objectMode?1:chunk.length;if(addToFront)state.buffer.unshift(chunk);else state.buffer.push(chunk);if(state.needReadable)emitReadable(stream)}maybeReadMore(stream,state)}}else if(!addToFront){state.reading=false}return needMoreData(state)}function needMoreData(state){return!state.ended&&(state.needReadable||state.length<state.highWaterMark||state.length===0)}Readable.prototype.setEncoding=function(enc){if(!StringDecoder)StringDecoder=require("string_decoder/").StringDecoder;this._readableState.decoder=new StringDecoder(enc);this._readableState.encoding=enc;return this};var MAX_HWM=8388608;function roundUpToNextPowerOf2(n){if(n>=MAX_HWM){n=MAX_HWM}else{n--;for(var p=1;p<32;p<<=1)n|=n>>p;n++}return n}function howMuchToRead(n,state){if(state.length===0&&state.ended)return 0;if(state.objectMode)return n===0?0:1;if(isNaN(n)||util.isNull(n)){if(state.flowing&&state.buffer.length)return state.buffer[0].length;else return state.length}if(n<=0)return 0;if(n>state.highWaterMark)state.highWaterMark=roundUpToNextPowerOf2(n);if(n>state.length){if(!state.ended){state.needReadable=true;return 0}else return state.length}return n}Readable.prototype.read=function(n){debug("read",n);var state=this._readableState;var nOrig=n;if(!util.isNumber(n)||n>0)state.emittedReadable=false;if(n===0&&state.needReadable&&(state.length>=state.highWaterMark||state.ended)){debug("read: emitReadable",state.length,state.ended);if(state.length===0&&state.ended)endReadable(this);else emitReadable(this);return null}n=howMuchToRead(n,state);if(n===0&&state.ended){if(state.length===0)endReadable(this);return null}var doRead=state.needReadable;debug("need readable",doRead);if(state.length===0||state.length-n<state.highWaterMark){doRead=true;debug("length less than watermark",doRead)}if(state.ended||state.reading){doRead=false;debug("reading or ended",doRead)}if(doRead){debug("do read");state.reading=true;state.sync=true;if(state.length===0)state.needReadable=true;this._read(state.highWaterMark);state.sync=false}if(doRead&&!state.reading)n=howMuchToRead(nOrig,state);var ret;if(n>0)ret=fromList(n,state);else ret=null;if(util.isNull(ret)){state.needReadable=true;n=0}state.length-=n;if(state.length===0&&!state.ended)state.needReadable=true;if(nOrig!==n&&state.ended&&state.length===0)endReadable(this);if(!util.isNull(ret))this.emit("data",ret);return ret};function chunkInvalid(state,chunk){var er=null;if(!util.isBuffer(chunk)&&!util.isString(chunk)&&!util.isNullOrUndefined(chunk)&&!state.objectMode){er=new TypeError("Invalid non-string/buffer chunk")}return er}function onEofChunk(stream,state){if(state.decoder&&!state.ended){var chunk=state.decoder.end();if(chunk&&chunk.length){state.buffer.push(chunk);state.length+=state.objectMode?1:chunk.length}}state.ended=true;emitReadable(stream)}function emitReadable(stream){var state=stream._readableState;state.needReadable=false;if(!state.emittedReadable){debug("emitReadable",state.flowing);state.emittedReadable=true;if(state.sync)process.nextTick(function(){emitReadable_(stream)});else emitReadable_(stream)}}function emitReadable_(stream){debug("emit readable");stream.emit("readable");flow(stream)}function maybeReadMore(stream,state){if(!state.readingMore){state.readingMore=true;process.nextTick(function(){maybeReadMore_(stream,state)})}}function maybeReadMore_(stream,state){var len=state.length;while(!state.reading&&!state.flowing&&!state.ended&&state.length<state.highWaterMark){debug("maybeReadMore read 0");stream.read(0);if(len===state.length)break;else len=state.length}state.readingMore=false}Readable.prototype._read=function(n){this.emit("error",new Error("not implemented"))};Readable.prototype.pipe=function(dest,pipeOpts){var src=this;var state=this._readableState;switch(state.pipesCount){case 0:state.pipes=dest;break;case 1:state.pipes=[state.pipes,dest];break;default:state.pipes.push(dest);break}state.pipesCount+=1;debug("pipe count=%d opts=%j",state.pipesCount,pipeOpts);var doEnd=(!pipeOpts||pipeOpts.end!==false)&&dest!==process.stdout&&dest!==process.stderr;var endFn=doEnd?onend:cleanup;if(state.endEmitted)process.nextTick(endFn);else src.once("end",endFn);dest.on("unpipe",onunpipe);function onunpipe(readable){debug("onunpipe");if(readable===src){cleanup()}}function onend(){debug("onend");dest.end()}var ondrain=pipeOnDrain(src);dest.on("drain",ondrain);function cleanup(){debug("cleanup");dest.removeListener("close",onclose);dest.removeListener("finish",onfinish);dest.removeListener("drain",ondrain);dest.removeListener("error",onerror);dest.removeListener("unpipe",onunpipe);src.removeListener("end",onend);src.removeListener("end",cleanup);src.removeListener("data",ondata);if(state.awaitDrain&&(!dest._writableState||dest._writableState.needDrain))ondrain()}src.on("data",ondata);function ondata(chunk){debug("ondata");var ret=dest.write(chunk);if(false===ret){debug("false write response, pause",src._readableState.awaitDrain);src._readableState.awaitDrain++;src.pause()}}function onerror(er){debug("onerror",er);unpipe();dest.removeListener("error",onerror);if(EE.listenerCount(dest,"error")===0)dest.emit("error",er)}if(!dest._events||!dest._events.error)dest.on("error",onerror);else if(isArray(dest._events.error))dest._events.error.unshift(onerror);else dest._events.error=[onerror,dest._events.error];function onclose(){dest.removeListener("finish",onfinish);unpipe()}dest.once("close",onclose);function onfinish(){debug("onfinish");dest.removeListener("close",onclose);unpipe()}dest.once("finish",onfinish);function unpipe(){debug("unpipe");src.unpipe(dest)}dest.emit("pipe",src);if(!state.flowing){debug("pipe resume");src.resume()}return dest};function pipeOnDrain(src){return function(){var state=src._readableState;debug("pipeOnDrain",state.awaitDrain);if(state.awaitDrain)state.awaitDrain--;if(state.awaitDrain===0&&EE.listenerCount(src,"data")){state.flowing=true;flow(src)}}}Readable.prototype.unpipe=function(dest){var state=this._readableState;if(state.pipesCount===0)return this;if(state.pipesCount===1){if(dest&&dest!==state.pipes)return this;if(!dest)dest=state.pipes;state.pipes=null;state.pipesCount=0;state.flowing=false;if(dest)dest.emit("unpipe",this);return this}if(!dest){var dests=state.pipes;var len=state.pipesCount;state.pipes=null;state.pipesCount=0;state.flowing=false;for(var i=0;i<len;i++)dests[i].emit("unpipe",this);return this}var i=indexOf(state.pipes,dest);if(i===-1)return this;state.pipes.splice(i,1);state.pipesCount-=1;if(state.pipesCount===1)state.pipes=state.pipes[0];dest.emit("unpipe",this);return this};Readable.prototype.on=function(ev,fn){var res=Stream.prototype.on.call(this,ev,fn);if(ev==="data"&&false!==this._readableState.flowing){this.resume()}if(ev==="readable"&&this.readable){var state=this._readableState;if(!state.readableListening){state.readableListening=true;state.emittedReadable=false;state.needReadable=true;if(!state.reading){var self=this;process.nextTick(function(){debug("readable nexttick read 0");self.read(0)})}else if(state.length){emitReadable(this,state)}}}return res};Readable.prototype.addListener=Readable.prototype.on;Readable.prototype.resume=function(){var state=this._readableState;if(!state.flowing){debug("resume");state.flowing=true;if(!state.reading){debug("resume read 0");this.read(0)}resume(this,state)}return this};function resume(stream,state){if(!state.resumeScheduled){state.resumeScheduled=true;process.nextTick(function(){resume_(stream,state)})}}function resume_(stream,state){state.resumeScheduled=false;stream.emit("resume");flow(stream);if(state.flowing&&!state.reading)stream.read(0)}Readable.prototype.pause=function(){debug("call pause flowing=%j",this._readableState.flowing);if(false!==this._readableState.flowing){debug("pause");this._readableState.flowing=false;this.emit("pause")}return this};function flow(stream){var state=stream._readableState;debug("flow",state.flowing);if(state.flowing){do{var chunk=stream.read()}while(null!==chunk&&state.flowing)}}Readable.prototype.wrap=function(stream){var state=this._readableState;var paused=false;var self=this;stream.on("end",function(){debug("wrapped end");if(state.decoder&&!state.ended){var chunk=state.decoder.end();if(chunk&&chunk.length)self.push(chunk)}self.push(null)});stream.on("data",function(chunk){debug("wrapped data");if(state.decoder)chunk=state.decoder.write(chunk);if(!chunk||!state.objectMode&&!chunk.length)return;var ret=self.push(chunk);if(!ret){paused=true;stream.pause()}});for(var i in stream){if(util.isFunction(stream[i])&&util.isUndefined(this[i])){this[i]=function(method){return function(){return stream[method].apply(stream,arguments)}}(i)}}var events=["error","close","destroy","pause","resume"];forEach(events,function(ev){stream.on(ev,self.emit.bind(self,ev))});self._read=function(n){debug("wrapped _read",n);if(paused){paused=false;stream.resume()}};return self};Readable._fromList=fromList;function fromList(n,state){var list=state.buffer;var length=state.length;var stringMode=!!state.decoder;var objectMode=!!state.objectMode;var ret;if(list.length===0)return null;if(length===0)ret=null;else if(objectMode)ret=list.shift();else if(!n||n>=length){if(stringMode)ret=list.join("");else ret=Buffer.concat(list,length);list.length=0}else{if(n<list[0].length){var buf=list[0];ret=buf.slice(0,n);list[0]=buf.slice(n)}else if(n===list[0].length){ret=list.shift()}else{if(stringMode)ret="";else ret=new Buffer(n);var c=0;for(var i=0,l=list.length;i<l&&c<n;i++){var buf=list[0];var cpy=Math.min(n-c,buf.length);if(stringMode)ret+=buf.slice(0,cpy);else buf.copy(ret,c,0,cpy);if(cpy<buf.length)list[0]=buf.slice(cpy);else list.shift();c+=cpy}}}return ret}function endReadable(stream){var state=stream._readableState;if(state.length>0)throw new Error("endReadable called on non-empty stream");if(!state.endEmitted){state.ended=true;process.nextTick(function(){if(!state.endEmitted&&state.length===0){state.endEmitted=true;stream.readable=false;stream.emit("end")}})}}function forEach(xs,f){for(var i=0,l=xs.length;i<l;i++){f(xs[i],i)}}function indexOf(xs,x){for(var i=0,l=xs.length;i<l;i++){if(xs[i]===x)return i}return-1}}).call(this,require("_process"))},{"./_stream_duplex":43,_process:9,buffer:2,"core-util-is":48,events:6,inherits:42,isarray:49,stream:21,"string_decoder/":50,util:1}],46:[function(require,module,exports){module.exports=Transform;var Duplex=require("./_stream_duplex");var util=require("core-util-is");util.inherits=require("inherits");util.inherits(Transform,Duplex);function TransformState(options,stream){this.afterTransform=function(er,data){return afterTransform(stream,er,data)};this.needTransform=false;this.transforming=false;this.writecb=null;this.writechunk=null}function afterTransform(stream,er,data){var ts=stream._transformState;ts.transforming=false;var cb=ts.writecb;if(!cb)return stream.emit("error",new Error("no writecb in Transform class"));ts.writechunk=null;ts.writecb=null;if(!util.isNullOrUndefined(data))stream.push(data);if(cb)cb(er);var rs=stream._readableState;rs.reading=false;if(rs.needReadable||rs.length<rs.highWaterMark){stream._read(rs.highWaterMark)}}function Transform(options){if(!(this instanceof Transform))return new Transform(options);Duplex.call(this,options);this._transformState=new TransformState(options,this);var stream=this;this._readableState.needReadable=true;this._readableState.sync=false;this.once("prefinish",function(){if(util.isFunction(this._flush))this._flush(function(er){done(stream,er)});else done(stream)})}Transform.prototype.push=function(chunk,encoding){this._transformState.needTransform=false;return Duplex.prototype.push.call(this,chunk,encoding)};Transform.prototype._transform=function(chunk,encoding,cb){throw new Error("not implemented")};Transform.prototype._write=function(chunk,encoding,cb){var ts=this._transformState;ts.writecb=cb;ts.writechunk=chunk;ts.writeencoding=encoding;if(!ts.transforming){var rs=this._readableState;if(ts.needTransform||rs.needReadable||rs.length<rs.highWaterMark)this._read(rs.highWaterMark)}};Transform.prototype._read=function(n){var ts=this._transformState;if(!util.isNull(ts.writechunk)&&ts.writecb&&!ts.transforming){ts.transforming=true;this._transform(ts.writechunk,ts.writeencoding,ts.afterTransform)}else{ts.needTransform=true}};function done(stream,er){if(er)return stream.emit("error",er);var ws=stream._writableState;var ts=stream._transformState;if(ws.length)throw new Error("calling transform done when ws.length != 0");if(ts.transforming)throw new Error("calling transform done when still transforming");return stream.push(null)}},{"./_stream_duplex":43,"core-util-is":48,inherits:42}],47:[function(require,module,exports){(function(process){module.exports=Writable;var Buffer=require("buffer").Buffer;Writable.WritableState=WritableState;var util=require("core-util-is");util.inherits=require("inherits");var Stream=require("stream");util.inherits(Writable,Stream);function WriteReq(chunk,encoding,cb){this.chunk=chunk;this.encoding=encoding;this.callback=cb}function WritableState(options,stream){var Duplex=require("./_stream_duplex");options=options||{};var hwm=options.highWaterMark;var defaultHwm=options.objectMode?16:16*1024;this.highWaterMark=hwm||hwm===0?hwm:defaultHwm;this.objectMode=!!options.objectMode;if(stream instanceof Duplex)this.objectMode=this.objectMode||!!options.writableObjectMode;this.highWaterMark=~~this.highWaterMark;this.needDrain=false;this.ending=false;this.ended=false;this.finished=false;var noDecode=options.decodeStrings===false;this.decodeStrings=!noDecode;this.defaultEncoding=options.defaultEncoding||"utf8";this.length=0;this.writing=false;this.corked=0;this.sync=true;this.bufferProcessing=false;this.onwrite=function(er){onwrite(stream,er)};this.writecb=null;this.writelen=0;this.buffer=[];this.pendingcb=0;this.prefinished=false;this.errorEmitted=false}function Writable(options){var Duplex=require("./_stream_duplex");if(!(this instanceof Writable)&&!(this instanceof Duplex))return new Writable(options);this._writableState=new WritableState(options,this);this.writable=true;Stream.call(this)}Writable.prototype.pipe=function(){this.emit("error",new Error("Cannot pipe. Not readable.")); | |
};function writeAfterEnd(stream,state,cb){var er=new Error("write after end");stream.emit("error",er);process.nextTick(function(){cb(er)})}function validChunk(stream,state,chunk,cb){var valid=true;if(!util.isBuffer(chunk)&&!util.isString(chunk)&&!util.isNullOrUndefined(chunk)&&!state.objectMode){var er=new TypeError("Invalid non-string/buffer chunk");stream.emit("error",er);process.nextTick(function(){cb(er)});valid=false}return valid}Writable.prototype.write=function(chunk,encoding,cb){var state=this._writableState;var ret=false;if(util.isFunction(encoding)){cb=encoding;encoding=null}if(util.isBuffer(chunk))encoding="buffer";else if(!encoding)encoding=state.defaultEncoding;if(!util.isFunction(cb))cb=function(){};if(state.ended)writeAfterEnd(this,state,cb);else if(validChunk(this,state,chunk,cb)){state.pendingcb++;ret=writeOrBuffer(this,state,chunk,encoding,cb)}return ret};Writable.prototype.cork=function(){var state=this._writableState;state.corked++};Writable.prototype.uncork=function(){var state=this._writableState;if(state.corked){state.corked--;if(!state.writing&&!state.corked&&!state.finished&&!state.bufferProcessing&&state.buffer.length)clearBuffer(this,state)}};function decodeChunk(state,chunk,encoding){if(!state.objectMode&&state.decodeStrings!==false&&util.isString(chunk)){chunk=new Buffer(chunk,encoding)}return chunk}function writeOrBuffer(stream,state,chunk,encoding,cb){chunk=decodeChunk(state,chunk,encoding);if(util.isBuffer(chunk))encoding="buffer";var len=state.objectMode?1:chunk.length;state.length+=len;var ret=state.length<state.highWaterMark;if(!ret)state.needDrain=true;if(state.writing||state.corked)state.buffer.push(new WriteReq(chunk,encoding,cb));else doWrite(stream,state,false,len,chunk,encoding,cb);return ret}function doWrite(stream,state,writev,len,chunk,encoding,cb){state.writelen=len;state.writecb=cb;state.writing=true;state.sync=true;if(writev)stream._writev(chunk,state.onwrite);else stream._write(chunk,encoding,state.onwrite);state.sync=false}function onwriteError(stream,state,sync,er,cb){if(sync)process.nextTick(function(){state.pendingcb--;cb(er)});else{state.pendingcb--;cb(er)}stream._writableState.errorEmitted=true;stream.emit("error",er)}function onwriteStateUpdate(state){state.writing=false;state.writecb=null;state.length-=state.writelen;state.writelen=0}function onwrite(stream,er){var state=stream._writableState;var sync=state.sync;var cb=state.writecb;onwriteStateUpdate(state);if(er)onwriteError(stream,state,sync,er,cb);else{var finished=needFinish(stream,state);if(!finished&&!state.corked&&!state.bufferProcessing&&state.buffer.length){clearBuffer(stream,state)}if(sync){process.nextTick(function(){afterWrite(stream,state,finished,cb)})}else{afterWrite(stream,state,finished,cb)}}}function afterWrite(stream,state,finished,cb){if(!finished)onwriteDrain(stream,state);state.pendingcb--;cb();finishMaybe(stream,state)}function onwriteDrain(stream,state){if(state.length===0&&state.needDrain){state.needDrain=false;stream.emit("drain")}}function clearBuffer(stream,state){state.bufferProcessing=true;if(stream._writev&&state.buffer.length>1){var cbs=[];for(var c=0;c<state.buffer.length;c++)cbs.push(state.buffer[c].callback);state.pendingcb++;doWrite(stream,state,true,state.length,state.buffer,"",function(err){for(var i=0;i<cbs.length;i++){state.pendingcb--;cbs[i](err)}});state.buffer=[]}else{for(var c=0;c<state.buffer.length;c++){var entry=state.buffer[c];var chunk=entry.chunk;var encoding=entry.encoding;var cb=entry.callback;var len=state.objectMode?1:chunk.length;doWrite(stream,state,false,len,chunk,encoding,cb);if(state.writing){c++;break}}if(c<state.buffer.length)state.buffer=state.buffer.slice(c);else state.buffer.length=0}state.bufferProcessing=false}Writable.prototype._write=function(chunk,encoding,cb){cb(new Error("not implemented"))};Writable.prototype._writev=null;Writable.prototype.end=function(chunk,encoding,cb){var state=this._writableState;if(util.isFunction(chunk)){cb=chunk;chunk=null;encoding=null}else if(util.isFunction(encoding)){cb=encoding;encoding=null}if(!util.isNullOrUndefined(chunk))this.write(chunk,encoding);if(state.corked){state.corked=1;this.uncork()}if(!state.ending&&!state.finished)endWritable(this,state,cb)};function needFinish(stream,state){return state.ending&&state.length===0&&!state.finished&&!state.writing}function prefinish(stream,state){if(!state.prefinished){state.prefinished=true;stream.emit("prefinish")}}function finishMaybe(stream,state){var need=needFinish(stream,state);if(need){if(state.pendingcb===0){prefinish(stream,state);state.finished=true;stream.emit("finish")}else prefinish(stream,state)}return need}function endWritable(stream,state,cb){state.ending=true;finishMaybe(stream,state);if(cb){if(state.finished)process.nextTick(cb);else stream.once("finish",cb)}state.ended=true}}).call(this,require("_process"))},{"./_stream_duplex":43,_process:9,buffer:2,"core-util-is":48,inherits:42,stream:21}],48:[function(require,module,exports){(function(Buffer){function isArray(arg){if(Array.isArray){return Array.isArray(arg)}return objectToString(arg)==="[object Array]"}exports.isArray=isArray;function isBoolean(arg){return typeof arg==="boolean"}exports.isBoolean=isBoolean;function isNull(arg){return arg===null}exports.isNull=isNull;function isNullOrUndefined(arg){return arg==null}exports.isNullOrUndefined=isNullOrUndefined;function isNumber(arg){return typeof arg==="number"}exports.isNumber=isNumber;function isString(arg){return typeof arg==="string"}exports.isString=isString;function isSymbol(arg){return typeof arg==="symbol"}exports.isSymbol=isSymbol;function isUndefined(arg){return arg===void 0}exports.isUndefined=isUndefined;function isRegExp(re){return objectToString(re)==="[object RegExp]"}exports.isRegExp=isRegExp;function isObject(arg){return typeof arg==="object"&&arg!==null}exports.isObject=isObject;function isDate(d){return objectToString(d)==="[object Date]"}exports.isDate=isDate;function isError(e){return objectToString(e)==="[object Error]"||e instanceof Error}exports.isError=isError;function isFunction(arg){return typeof arg==="function"}exports.isFunction=isFunction;function isPrimitive(arg){return arg===null||typeof arg==="boolean"||typeof arg==="number"||typeof arg==="string"||typeof arg==="symbol"||typeof arg==="undefined"}exports.isPrimitive=isPrimitive;exports.isBuffer=Buffer.isBuffer;function objectToString(o){return Object.prototype.toString.call(o)}}).call(this,require("buffer").Buffer)},{buffer:2}],49:[function(require,module,exports){arguments[4][8][0].apply(exports,arguments)},{dup:8}],50:[function(require,module,exports){arguments[4][22][0].apply(exports,arguments)},{buffer:2,dup:22}],51:[function(require,module,exports){arguments[4][18][0].apply(exports,arguments)},{"./lib/_stream_duplex.js":43,"./lib/_stream_passthrough.js":44,"./lib/_stream_readable.js":45,"./lib/_stream_transform.js":46,"./lib/_stream_writable.js":47,dup:18,stream:21}],52:[function(require,module,exports){arguments[4][40][0].apply(exports,arguments)},{dup:40}],53:[function(require,module,exports){module.exports=extend;var hasOwnProperty=Object.prototype.hasOwnProperty;function extend(){var target={};for(var i=0;i<arguments.length;i++){var source=arguments[i];for(var key in source){if(hasOwnProperty.call(source,key)){target[key]=source[key]}}}return target}},{}],54:[function(require,module,exports){module.exports={name:"levelup",description:"Fast & simple storage - a Node.js-style LevelDB wrapper",version:"1.3.1",contributors:[{name:"Rod Vagg",email:"r@va.gg",url:"https://github.com/rvagg"},{name:"John Chesley",email:"john@chesl.es",url:"https://github.com/chesles/"},{name:"Jake Verbaten",email:"raynos2@gmail.com",url:"https://github.com/raynos"},{name:"Dominic Tarr",email:"dominic.tarr@gmail.com",url:"https://github.com/dominictarr"},{name:"Max Ogden",email:"max@maxogden.com",url:"https://github.com/maxogden"},{name:"Lars-Magnus Skog",email:"ralphtheninja@riseup.net",url:"https://github.com/ralphtheninja"},{name:"David Björklund",email:"david.bjorklund@gmail.com",url:"https://github.com/kesla"},{name:"Julian Gruber",email:"julian@juliangruber.com",url:"https://github.com/juliangruber"},{name:"Paolo Fragomeni",email:"paolo@async.ly",url:"https://github.com/hij1nx"},{name:"Anton Whalley",email:"anton.whalley@nearform.com",url:"https://github.com/No9"},{name:"Matteo Collina",email:"matteo.collina@gmail.com",url:"https://github.com/mcollina"},{name:"Pedro Teixeira",email:"pedro.teixeira@gmail.com",url:"https://github.com/pgte"},{name:"James Halliday",email:"mail@substack.net",url:"https://github.com/substack"},{name:"Jarrett Cruger",email:"jcrugzz@gmail.com",url:"https://github.com/jcrugzz"}],repository:{type:"git",url:"https://github.com/level/levelup.git"},homepage:"https://github.com/level/levelup",keywords:["leveldb","stream","database","db","store","storage","json"],main:"lib/levelup.js",dependencies:{"deferred-leveldown":"~1.2.1","level-codec":"~6.1.0","level-errors":"~1.0.3","level-iterator-stream":"~1.3.0",prr:"~1.0.1",semver:"~5.1.0",xtend:"~4.0.0"},devDependencies:{async:"~1.5.0",bustermove:"~1.0.0",tap:"~2.3.1",delayed:"~1.0.1",faucet:"~0.0.1",leveldown:"^1.1.0",memdown:"~1.1.0","msgpack-js":"~0.3.0",referee:"~1.2.0",rimraf:"~2.4.3","slow-stream":"0.0.4",tape:"~4.2.1"},browser:{leveldown:false,"leveldown/package":false,semver:false},scripts:{test:"tape test/*-test.js | faucet"},license:"MIT",readme:"LevelUP\n=======\n\n<img alt=\"LevelDB Logo\" height=\"100\" src=\"http://leveldb.org/img/logo.svg\">\n\n**Fast & simple storage - a Node.js-style LevelDB wrapper**\n\n[](http://travis-ci.org/Level/levelup)\n[](https://david-dm.org/level/levelup)\n\n[](https://nodei.co/npm/levelup/) [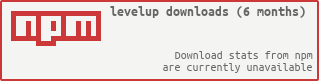](https://nodei.co/npm/levelup/)\n\n\n * <a href=\"#intro\">Introduction</a>\n * <a href=\"#leveldown\">Relationship to LevelDOWN</a>\n * <a href=\"#platforms\">Tested & supported platforms</a>\n * <a href=\"#basic\">Basic usage</a>\n * <a href=\"#api\">API</a>\n * <a href=\"#events\">Events</a>\n * <a href=\"#json\">JSON data</a>\n * <a href=\"#custom_encodings\">Custom encodings</a>\n * <a href=\"#extending\">Extending LevelUP</a>\n * <a href=\"#multiproc\">Multi-process access</a>\n * <a href=\"#support\">Getting support</a>\n * <a href=\"#contributing\">Contributing</a>\n * <a href=\"#license\">Licence & copyright</a>\n\n<a name=\"intro\"></a>\nIntroduction\n------------\n\n**[LevelDB](https://github.com/google/leveldb)** is a simple key/value data store built by Google, inspired by BigTable. It's used in Google Chrome and many other products. LevelDB supports arbitrary byte arrays as both keys and values, singular *get*, *put* and *delete* operations, *batched put and delete*, bi-directional iterators and simple compression using the very fast [Snappy](http://google.github.io/snappy/) algorithm.\n\n**LevelUP** aims to expose the features of LevelDB in a **Node.js-friendly way**. All standard `Buffer` encoding types are supported, as is a special JSON encoding. LevelDB's iterators are exposed as a Node.js-style **readable stream**.\n\nLevelDB stores entries **sorted lexicographically by keys**. This makes LevelUP's <a href=\"#createReadStream\"><code>ReadStream</code></a> interface a very powerful query mechanism.\n\n**LevelUP** is an **OPEN Open Source Project**, see the <a href=\"#contributing\">Contributing</a> section to find out what this means.\n\n<a name=\"leveldown\"></a>\nRelationship to LevelDOWN\n-------------------------\n\nLevelUP is designed to be backed by **[LevelDOWN](https://github.com/level/leveldown/)** which provides a pure C++ binding to LevelDB and can be used as a stand-alone package if required.\n\n**As of version 0.9, LevelUP no longer requires LevelDOWN as a dependency so you must `npm install leveldown` when you install LevelUP.**\n\nLevelDOWN is now optional because LevelUP can be used with alternative backends, such as **[level.js](https://github.com/maxogden/level.js)** in the browser or [MemDOWN](https://github.com/level/memdown) for a pure in-memory store.\n\nLevelUP will look for LevelDOWN and throw an error if it can't find it in its Node `require()` path. It will also tell you if the installed version of LevelDOWN is incompatible.\n\n**The [level](https://github.com/level/level) package is available as an alternative installation mechanism.** Install it instead to automatically get both LevelUP & LevelDOWN. It exposes LevelUP on its export (i.e. you can `var leveldb = require('level')`).\n\n\n<a name=\"platforms\"></a>\nTested & supported platforms\n----------------------------\n\n * **Linux**: including ARM platforms such as Raspberry Pi *and Kindle!*\n * **Mac OS**\n * **Solaris**: including Joyent's SmartOS & Nodejitsu\n * **Windows**: Node 0.10 and above only. See installation instructions for *node-gyp's* dependencies [here](https://github.com/TooTallNate/node-gyp#installation), you'll need these (free) components from Microsoft to compile and run any native Node add-on in Windows.\n\n<a name=\"basic\"></a>\nBasic usage\n-----------\n\nFirst you need to install LevelUP!\n\n```sh\n$ npm install levelup leveldown\n```\n\nOr\n\n```sh\n$ npm install level\n```\n\n*(this second option requires you to use LevelUP by calling `var levelup = require('level')`)*\n\n\nAll operations are asynchronous although they don't necessarily require a callback if you don't need to know when the operation was performed.\n\n```js\nvar levelup = require('levelup')\n\n// 1) Create our database, supply location and options.\n// This will create or open the underlying LevelDB store.\nvar db = levelup('./mydb')\n\n// 2) put a key & value\ndb.put('name', 'LevelUP', function (err) {\n if (err) return console.log('Ooops!', err) // some kind of I/O error\n\n // 3) fetch by key\n db.get('name', function (err, value) {\n if (err) return console.log('Ooops!', err) // likely the key was not found\n\n // ta da!\n console.log('name=' + value)\n })\n})\n```\n\n<a name=\"api\"></a>\n## API\n\n * <a href=\"#ctor\"><code><b>levelup()</b></code></a>\n * <a href=\"#open\"><code>db.<b>open()</b></code></a>\n * <a href=\"#close\"><code>db.<b>close()</b></code></a>\n * <a href=\"#put\"><code>db.<b>put()</b></code></a>\n * <a href=\"#get\"><code>db.<b>get()</b></code></a>\n * <a href=\"#del\"><code>db.<b>del()</b></code></a>\n * <a href=\"#batch\"><code>db.<b>batch()</b></code> *(array form)*</a>\n * <a href=\"#batch_chained\"><code>db.<b>batch()</b></code> *(chained form)*</a>\n * <a href=\"#isOpen\"><code>db.<b>isOpen()</b></code></a>\n * <a href=\"#isClosed\"><code>db.<b>isClosed()</b></code></a>\n * <a href=\"#createReadStream\"><code>db.<b>createReadStream()</b></code></a>\n * <a href=\"#createKeyStream\"><code>db.<b>createKeyStream()</b></code></a>\n * <a href=\"#createValueStream\"><code>db.<b>createValueStream()</b></code></a>\n\n### Special operations exposed by LevelDOWN\n\n * <a href=\"#approximateSize\"><code>db.db.<b>approximateSize()</b></code></a>\n * <a href=\"#getProperty\"><code>db.db.<b>getProperty()</b></code></a>\n * <a href=\"#destroy\"><code><b>leveldown.destroy()</b></code></a>\n * <a href=\"#repair\"><code><b>leveldown.repair()</b></code></a>\n\n### Special Notes\n * <a href=\"#writeStreams\">What happened to <code><b>db.createWriteStream()</b></code></a>\n\n\n--------------------------------------------------------\n<a name=\"ctor\"></a>\n### levelup(location[, options[, callback]])\n### levelup(options[, callback ])\n### levelup(db[, callback ])\n<code>levelup()</code> is the main entry point for creating a new LevelUP instance and opening the underlying store with LevelDB.\n\nThis function returns a new instance of LevelUP and will also initiate an <a href=\"#open\"><code>open()</code></a> operation. Opening the database is an asynchronous operation which will trigger your callback if you provide one. The callback should take the form: `function (err, db) {}` where the `db` is the LevelUP instance. If you don't provide a callback, any read & write operations are simply queued internally until the database is fully opened.\n\nThis leads to two alternative ways of managing a new LevelUP instance:\n\n```js\nlevelup(location, options, function (err, db) {\n if (err) throw err\n db.get('foo', function (err, value) {\n if (err) return console.log('foo does not exist')\n console.log('got foo =', value)\n })\n})\n\n// vs the equivalent:\n\nvar db = levelup(location, options) // will throw if an error occurs\ndb.get('foo', function (err, value) {\n if (err) return console.log('foo does not exist')\n console.log('got foo =', value)\n})\n```\n\nThe `location` argument is available as a read-only property on the returned LevelUP instance.\n\nThe `levelup(options, callback)` form (with optional `callback`) is only available where you provide a valid `'db'` property on the options object (see below). Only for back-ends that don't require a `location` argument, such as [MemDOWN](https://github.com/level/memdown).\n\nFor example:\n\n```js\nvar levelup = require('levelup')\nvar memdown = require('memdown')\nvar db = levelup({ db: memdown })\n```\n\nThe `levelup(db, callback)` form (with optional `callback`) is only available where `db` is a factory function, as would be provided as a `'db'` property on an `options` object (see below). Only for back-ends that don't require a `location` argument, such as [MemDOWN](https://github.com/level/memdown).\n\nFor example:\n\n```js\nvar levelup = require('levelup')\nvar memdown = require('memdown')\nvar db = levelup(memdown)\n```\n\n#### `options`\n\n`levelup()` takes an optional options object as its second argument; the following properties are accepted:\n\n* `'createIfMissing'` *(boolean, default: `true`)*: If `true`, will initialise an empty database at the specified location if one doesn't already exist. If `false` and a database doesn't exist you will receive an error in your `open()` callback and your database won't open.\n\n* `'errorIfExists'` *(boolean, default: `false`)*: If `true`, you will receive an error in your `open()` callback if the database exists at the specified location.\n\n* `'compression'` *(boolean, default: `true`)*: If `true`, all *compressible* data will be run through the Snappy compression algorithm before being stored. Snappy is very fast and shouldn't gain much speed by disabling so leave this on unless you have good reason to turn it off.\n\n* `'cacheSize'` *(number, default: `8 * 1024 * 1024`)*: The size (in bytes) of the in-memory [LRU](http://en.wikipedia.org/wiki/Cache_algorithms#Least_Recently_Used) cache with frequently used uncompressed block contents.\n\n* `'keyEncoding'` and `'valueEncoding'` *(string, default: `'utf8'`)*: The encoding of the keys and values passed through Node.js' `Buffer` implementation (see [Buffer#toString()](http://nodejs.org/docs/latest/api/buffer.html#buffer_buf_tostring_encoding_start_end)).\n <p><code>'utf8'</code> is the default encoding for both keys and values so you can simply pass in strings and expect strings from your <code>get()</code> operations. You can also pass <code>Buffer</code> objects as keys and/or values and conversion will be performed.</p>\n <p>Supported encodings are: hex, utf8, ascii, binary, base64, ucs2, utf16le.</p>\n <p><code>'json'</code> encoding is also supported, see below.</p>\n\n* `'db'` *(object, default: LevelDOWN)*: LevelUP is backed by [LevelDOWN](https://github.com/level/leveldown/) to provide an interface to LevelDB. You can completely replace the use of LevelDOWN by providing a \"factory\" function that will return a LevelDOWN API compatible object given a `location` argument. For further information, see [MemDOWN](https://github.com/level/memdown), a fully LevelDOWN API compatible replacement that uses a memory store rather than LevelDB. Also see [Abstract LevelDOWN](http://github.com/level/abstract-leveldown), a partial implementation of the LevelDOWN API that can be used as a base prototype for a LevelDOWN substitute.\n\nAdditionally, each of the main interface methods accept an optional options object that can be used to override `'keyEncoding'` and `'valueEncoding'`.\n\n--------------------------------------------------------\n<a name=\"open\"></a>\n### db.open([callback])\n<code>open()</code> opens the underlying LevelDB store. In general **you should never need to call this method directly** as it's automatically called by <a href=\"#ctor\"><code>levelup()</code></a>.\n\nHowever, it is possible to *reopen* a database after it has been closed with <a href=\"#close\"><code>close()</code></a>, although this is not generally advised.\n\n--------------------------------------------------------\n<a name=\"close\"></a>\n### db.close([callback])\n<code>close()</code> closes the underlying LevelDB store. The callback will receive any error encountered during closing as the first argument.\n\nYou should always clean up your LevelUP instance by calling `close()` when you no longer need it to free up resources. A LevelDB store cannot be opened by multiple instances of LevelDB/LevelUP simultaneously.\n\n--------------------------------------------------------\n<a name=\"put\"></a>\n### db.put(key, value[, options][, callback])\n<code>put()</code> is the primary method for inserting data into the store. Both the `key` and `value` can be arbitrary data objects.\n\nThe callback argument is optional but if you don't provide one and an error occurs then expect the error to be thrown.\n\n#### `options`\n\nEncoding of the `key` and `value` objects will adhere to `'keyEncoding'` and `'valueEncoding'` options provided to <a href=\"#ctor\"><code>levelup()</code></a>, although you can provide alternative encoding settings in the options for `put()` (it's recommended that you stay consistent in your encoding of keys and values in a single store).\n\nIf you provide a `'sync'` value of `true` in your `options` object, LevelDB will perform a synchronous write of the data; although the operation will be asynchronous as far as Node is concerned. Normally, LevelDB passes the data to the operating system for writing and returns immediately, however a synchronous write will use `fsync()` or equivalent so your callback won't be triggered until the data is actually on disk. Synchronous filesystem writes are **significantly** slower than asynchronous writes but if you want to be absolutely sure that the data is flushed then you can use `'sync': true`.\n\n--------------------------------------------------------\n<a name=\"get\"></a>\n### db.get(key[, options][, callback])\n<code>get()</code> is the primary method for fetching data from the store. The `key` can be an arbitrary data object. If it doesn't exist in the store then the callback will receive an error as its first argument. A not-found err object will be of type `'NotFoundError'` so you can `err.type == 'NotFoundError'` or you can perform a truthy test on the property `err.notFound`.\n\n```js\ndb.get('foo', function (err, value) {\n if (err) {\n if (err.notFound) {\n // handle a 'NotFoundError' here\n return\n }\n // I/O or other error, pass it up the callback chain\n return callback(err)\n }\n\n // .. handle `value` here\n})\n```\n\n#### `options`\n\nEncoding of the `key` and `value` objects is the same as in <a href=\"#put\"><code>put</code></a>. \n\nLevelDB will by default fill the in-memory LRU Cache with data from a call to get. Disabling this is done by setting `fillCache` to `false`.\n\n--------------------------------------------------------\n<a name=\"del\"></a>\n### db.del(key[, options][, callback])\n<code>del()</code> is the primary method for removing data from the store.\n```js\ndb.del('foo', function (err) {\n if (err)\n // handle I/O or other error\n});\n```\n\n#### `options`\n\nEncoding of the `key` object will adhere to the `'keyEncoding'` option provided to <a href=\"#ctor\"><code>levelup()</code></a>, although you can provide alternative encoding settings in the options for `del()` (it's recommended that you stay consistent in your encoding of keys and values in a single store).\n\nA `'sync'` option can also be passed, see <a href=\"#put\"><code>put()</code></a> for details on how this works.\n\n--------------------------------------------------------\n<a name=\"batch\"></a>\n### db.batch(array[, options][, callback]) *(array form)*\n<code>batch()</code> can be used for very fast bulk-write operations (both *put* and *delete*). The `array` argument should contain a list of operations to be executed sequentially, although as a whole they are performed as an atomic operation inside LevelDB.\n\nEach operation is contained in an object having the following properties: `type`, `key`, `value`, where the *type* is either `'put'` or `'del'`. In the case of `'del'` the `'value'` property is ignored. Any entries with a `'key'` of `null` or `undefined` will cause an error to be returned on the `callback` and any `'type': 'put'` entry with a `'value'` of `null` or `undefined` will return an error.\n\nIf `key` and `value` are defined but `type` is not, it will default to `'put'`.\n\n```js\nvar ops = [\n { type: 'del', key: 'father' }\n , { type: 'put', key: 'name', value: 'Yuri Irsenovich Kim' }\n , { type: 'put', key: 'dob', value: '16 February 1941' }\n , { type: 'put', key: 'spouse', value: 'Kim Young-sook' }\n , { type: 'put', key: 'occupation', value: 'Clown' }\n]\n\ndb.batch(ops, function (err) {\n if (err) return console.log('Ooops!', err)\n console.log('Great success dear leader!')\n})\n```\n\n#### `options`\n\nSee <a href=\"#put\"><code>put()</code></a> for a discussion on the `options` object. You can overwrite default `'keyEncoding'` and `'valueEncoding'` and also specify the use of `sync` filesystem operations.\n\nIn addition to encoding options for the whole batch you can also overwrite the encoding per operation, like:\n\n```js\nvar ops = [{\n type : 'put'\n , key : new Buffer([1, 2, 3])\n , value : { some: 'json' }\n , keyEncoding : 'binary'\n , valueEncoding : 'json'\n}]\n```\n\n--------------------------------------------------------\n<a name=\"batch_chained\"></a>\n### db.batch() *(chained form)*\n<code>batch()</code>, when called with no arguments will return a `Batch` object which can be used to build, and eventually commit, an atomic LevelDB batch operation. Depending on how it's used, it is possible to obtain greater performance when using the chained form of `batch()` over the array form.\n\n```js\ndb.batch()\n .del('father')\n .put('name', 'Yuri Irsenovich Kim')\n .put('dob', '16 February 1941')\n .put('spouse', 'Kim Young-sook')\n .put('occupation', 'Clown')\n .write(function () { console.log('Done!') })\n```\n\n<b><code>batch.put(key, value[, options])</code></b>\n\nQueue a *put* operation on the current batch, not committed until a `write()` is called on the batch.\n\nThe optional `options` argument can be used to override the default `'keyEncoding'` and/or `'valueEncoding'`.\n\nThis method may `throw` a `WriteError` if there is a problem with your put (such as the `value` being `null` or `undefined`).\n\n<b><code>batch.del(key[, options])</code></b>\n\nQueue a *del* operation on the current batch, not committed until a `write()` is called on the batch.\n\nThe optional `options` argument can be used to override the default `'keyEncoding'`.\n\nThis method may `throw` a `WriteError` if there is a problem with your delete.\n\n<b><code>batch.clear()</code></b>\n\nClear all queued operations on the current batch, any previous operations will be discarded.\n\n<b><code>batch.length</code></b>\n\nThe number of queued operations on the current batch.\n\n<b><code>batch.write([callback])</code></b>\n\nCommit the queued operations for this batch. All operations not *cleared* will be written to the database atomically, that is, they will either all succeed or fail with no partial commits. The optional `callback` will be called when the operation has completed with an *error* argument if an error has occurred; if no `callback` is supplied and an error occurs then this method will `throw` a `WriteError`.\n\n\n--------------------------------------------------------\n<a name=\"isOpen\"></a>\n### db.isOpen()\n\nA LevelUP object can be in one of the following states:\n\n * *\"new\"* - newly created, not opened or closed\n * *\"opening\"* - waiting for the database to be opened\n * *\"open\"* - successfully opened the database, available for use\n * *\"closing\"* - waiting for the database to be closed\n * *\"closed\"* - database has been successfully closed, should not be used\n\n`isOpen()` will return `true` only when the state is \"open\".\n\n--------------------------------------------------------\n<a name=\"isClosed\"></a>\n### db.isClosed()\n\n*See <a href=\"#put\"><code>isOpen()</code></a>*\n\n`isClosed()` will return `true` only when the state is \"closing\" *or* \"closed\", it can be useful for determining if read and write operations are permissible.\n\n--------------------------------------------------------\n<a name=\"createReadStream\"></a>\n### db.createReadStream([options])\n\nYou can obtain a **ReadStream** of the full database by calling the `createReadStream()` method. The resulting stream is a complete Node.js-style [Readable Stream](http://nodejs.org/docs/latest/api/stream.html#stream_readable_stream) where `'data'` events emit objects with `'key'` and `'value'` pairs. You can also use the `gt`, `lt` and `limit` options to control the range of keys that are streamed.\n\n```js\ndb.createReadStream()\n .on('data', function (data) {\n console.log(data.key, '=', data.value)\n })\n .on('error', function (err) {\n console.log('Oh my!', err)\n })\n .on('close', function () {\n console.log('Stream closed')\n })\n .on('end', function () {\n console.log('Stream closed')\n })\n```\n\nThe standard `pause()`, `resume()` and `destroy()` methods are implemented on the ReadStream, as is `pipe()` (see below). `'data'`, '`error'`, `'end'` and `'close'` events are emitted.\n\nAdditionally, you can supply an options object as the first parameter to `createReadStream()` with the following options:\n\n* `'gt'` (greater than), `'gte'` (greater than or equal) define the lower bound of the range to be streamed. Only records where the key is greater than (or equal to) this option will be included in the range. When `reverse=true` the order will be reversed, but the records streamed will be the same.\n\n* `'lt'` (less than), `'lte'` (less than or equal) define the higher bound of the range to be streamed. Only key/value pairs where the key is less than (or equal to) this option will be included in the range. When `reverse=true` the order will be reversed, but the records streamed will be the same.\n\n* `'start', 'end'` legacy ranges - instead use `'gte', 'lte'`\n\n* `'reverse'` *(boolean, default: `false`)*: a boolean, set true and the stream output will be reversed. Beware that due to the way LevelDB works, a reverse seek will be slower than a forward seek.\n\n* `'keys'` *(boolean, default: `true`)*: whether the `'data'` event should contain keys. If set to `true` and `'values'` set to `false` then `'data'` events will simply be keys, rather than objects with a `'key'` property. Used internally by the `createKeyStream()` method.\n\n* `'values'` *(boolean, default: `true`)*: whether the `'data'` event should contain values. If set to `true` and `'keys'` set to `false` then `'data'` events will simply be values, rather than objects with a `'value'` property. Used internally by the `createValueStream()` method.\n\n* `'limit'` *(number, default: `-1`)*: limit the number of results collected by this stream. This number represents a *maximum* number of results and may not be reached if you get to the end of the data first. A value of `-1` means there is no limit. When `reverse=true` the highest keys will be returned instead of the lowest keys.\n\n* `'fillCache'` *(boolean, default: `false`)*: wheather LevelDB's LRU-cache should be filled with data read.\n\n* `'keyEncoding'` / `'valueEncoding'` *(string)*: the encoding applied to each read piece of data.\n\n--------------------------------------------------------\n<a name=\"createKeyStream\"></a>\n### db.createKeyStream([options])\n\nA **KeyStream** is a **ReadStream** where the `'data'` events are simply the keys from the database so it can be used like a traditional stream rather than an object stream.\n\nYou can obtain a KeyStream either by calling the `createKeyStream()` method on a LevelUP object or by passing passing an options object to `createReadStream()` with `keys` set to `true` and `values` set to `false`.\n\n```js\ndb.createKeyStream()\n .on('data', function (data) {\n console.log('key=', data)\n })\n\n// same as:\ndb.createReadStream({ keys: true, values: false })\n .on('data', function (data) {\n console.log('key=', data)\n })\n```\n\n--------------------------------------------------------\n<a name=\"createValueStream\"></a>\n### db.createValueStream([options])\n\nA **ValueStream** is a **ReadStream** where the `'data'` events are simply the values from the database so it can be used like a traditional stream rather than an object stream.\n\nYou can obtain a ValueStream either by calling the `createValueStream()` method on a LevelUP object or by passing passing an options object to `createReadStream()` with `values` set to `true` and `keys` set to `false`.\n\n```js\ndb.createValueStream()\n .on('data', function (data) {\n console.log('value=', data)\n })\n\n// same as:\ndb.createReadStream({ keys: false, values: true })\n .on('data', function (data) {\n console.log('value=', data)\n })\n```\n\n--------------------------------------------------------\n<a name=\"writeStreams\"></a>\n#### What happened to `db.createWriteStream`?\n\n`db.createWriteStream()` has been removed in order to provide a smaller and more maintainable core. It primarily existed to create symmetry with `db.createReadStream()` but through much [discussion](https://github.com/level/levelup/issues/199), removing it was the best cause of action.\n\nThe main driver for this was performance. While `db.createReadStream()` performs well under most use cases, `db.createWriteStream()` was highly dependent on the application keys and values. Thus we can't provide a standard implementation and encourage more `write-stream` implementations to be created to solve the broad spectrum of use cases.\n\nCheck out the implementations that the community has already produced [here](https://github.com/level/levelup/wiki/Modules#write-streams).\n\n--------------------------------------------------------\n<a name='approximateSize'></a>\n### db.db.approximateSize(start, end, callback)\n<code>approximateSize()</code> can used to get the approximate number of bytes of file system space used by the range `[start..end)`. The result may not include recently written data.\n\n```js\nvar db = require('level')('./huge.db')\n\ndb.db.approximateSize('a', 'c', function (err, size) {\n if (err) return console.error('Ooops!', err)\n console.log('Approximate size of range is %d', size)\n})\n```\n\n**Note:** `approximateSize()` is available via [LevelDOWN](https://github.com/level/leveldown/), which by default is accessible as the `db` property of your LevelUP instance. This is a specific LevelDB operation and is not likely to be available where you replace LevelDOWN with an alternative back-end via the `'db'` option.\n\n\n--------------------------------------------------------\n<a name='getProperty'></a>\n### db.db.getProperty(property)\n<code>getProperty</code> can be used to get internal details from LevelDB. When issued with a valid property string, a readable string will be returned (this method is synchronous).\n\nCurrently, the only valid properties are:\n\n* <b><code>'leveldb.num-files-at-levelN'</code></b>: returns the number of files at level *N*, where N is an integer representing a valid level (e.g. \"0\").\n\n* <b><code>'leveldb.stats'</code></b>: returns a multi-line string describing statistics about LevelDB's internal operation.\n\n* <b><code>'leveldb.sstables'</code></b>: returns a multi-line string describing all of the *sstables* that make up contents of the current database.\n\n\n```js\nvar db = require('level')('./huge.db')\nconsole.log(db.db.getProperty('leveldb.num-files-at-level3'))\n// → '243'\n```\n\n**Note:** `getProperty()` is available via [LevelDOWN](https://github.com/level/leveldown/), which by default is accessible as the `db` property of your LevelUP instance. This is a specific LevelDB operation and is not likely to be available where you replace LevelDOWN with an alternative back-end via the `'db'` option.\n\n\n--------------------------------------------------------\n<a name=\"destroy\"></a>\n### leveldown.destroy(location, callback)\n<code>destroy()</code> is used to completely remove an existing LevelDB database directory. You can use this function in place of a full directory *rm* if you want to be sure to only remove LevelDB-related files. If the directory only contains LevelDB files, the directory itself will be removed as well. If there are additional, non-LevelDB files in the directory, those files, and the directory, will be left alone.\n\nThe callback will be called when the destroy operation is complete, with a possible `error` argument.\n\n**Note:** `destroy()` is available via [LevelDOWN](https://github.com/level/leveldown/) which you will have to install seperately, e.g.:\n\n```js\nrequire('leveldown').destroy('./huge.db', function (err) { console.log('done!') })\n```\n\n--------------------------------------------------------\n<a name=\"repair\"></a>\n### leveldown.repair(location, callback)\n<code>repair()</code> can be used to attempt a restoration of a damaged LevelDB store. From the LevelDB documentation:\n\n> If a DB cannot be opened, you may attempt to call this method to resurrect as much of the contents of the database as possible. Some data may be lost, so be careful when calling this function on a database that contains important information.\n\nYou will find information on the *repair* operation in the *LOG* file inside the store directory.\n\nA `repair()` can also be used to perform a compaction of the LevelDB log into table files.\n\nThe callback will be called when the repair operation is complete, with a possible `error` argument.\n\n**Note:** `repair()` is available via [LevelDOWN](https://github.com/level/leveldown/) which you will have to install seperately, e.g.:\n\n```js\nrequire('leveldown').repair('./huge.db', function (err) { console.log('done!') })\n```\n\n--------------------------------------------------------\n\n<a name=\"events\"></a>\nEvents\n------\n\nLevelUP emits events when the callbacks to the corresponding methods are called.\n\n* `db.emit('put', key, value)` emitted when a new value is `'put'`\n* `db.emit('del', key)` emitted when a value is deleted\n* `db.emit('batch', ary)` emitted when a batch operation has executed\n* `db.emit('ready')` emitted when the database has opened (`'open'` is synonym)\n* `db.emit('closed')` emitted when the database has closed\n* `db.emit('opening')` emitted when the database is opening\n* `db.emit('closing')` emitted when the database is closing\n\nIf you do not pass a callback to an async function, and there is an error, LevelUP will `emit('error', err)` instead.\n\n<a name=\"json\"></a>\nJSON data\n---------\n\nYou specify `'json'` encoding for both keys and/or values, you can then supply JavaScript objects to LevelUP and receive them from all fetch operations, including ReadStreams. LevelUP will automatically *stringify* your objects and store them as *utf8* and parse the strings back into objects before passing them back to you.\n\n<a name=\"custom_encodings\"></a>\nCustom encodings\n----------------\n\nA custom encoding may be provided by passing in an object as an value for `keyEncoding` or `valueEncoding` (wherever accepted), it must have the following properties:\n\n```js\n{\n encode : function (val) { ... }\n , decode : function (val) { ... }\n , buffer : boolean // encode returns a buffer and decode accepts a buffer\n , type : String // name of this encoding type.\n}\n```\n\n<a name=\"extending\"></a>\nExtending LevelUP\n-----------------\n\nA list of <a href=\"https://github.com/level/levelup/wiki/Modules\"><b>Node.js LevelDB modules and projects</b></a> can be found in the wiki.\n\nWhen attempting to extend the functionality of LevelUP, it is recommended that you consider using [level-hooks](https://github.com/dominictarr/level-hooks) and/or [level-sublevel](https://github.com/dominictarr/level-sublevel). **level-sublevel** is particularly helpful for keeping additional, extension-specific, data in a LevelDB store. It allows you to partition a LevelUP instance into multiple sub-instances that each correspond to discrete namespaced key ranges.\n\n<a name=\"multiproc\"></a>\nMulti-process access\n--------------------\n\nLevelDB is thread-safe but is **not** suitable for accessing with multiple processes. You should only ever have a LevelDB database open from a single Node.js process. Node.js clusters are made up of multiple processes so a LevelUP instance cannot be shared between them either.\n\nSee the <a href=\"https://github.com/level/levelup/wiki/Modules\"><b>wiki</b></a> for some LevelUP extensions, including [multilevel](https://github.com/juliangruber/multilevel), that may help if you require a single data store to be shared across processes.\n\n<a name=\"support\"></a>\nGetting support\n---------------\n\nThere are multiple ways you can find help in using LevelDB in Node.js:\n\n * **IRC:** you'll find an active group of LevelUP users in the **##leveldb** channel on Freenode, including most of the contributors to this project.\n * **Mailing list:** there is an active [Node.js LevelDB](https://groups.google.com/forum/#!forum/node-levelup) Google Group.\n * **GitHub:** you're welcome to open an issue here on this GitHub repository if you have a question.\n\n<a name=\"contributing\"></a>\nContributing\n------------\n\nLevelUP is an **OPEN Open Source Project**. This means that:\n\n> Individuals making significant and valuable contributions are given commit-access to the project to contribute as they see fit. This project is more like an open wiki than a standard guarded open source project.\n\nSee the [contribution guide](https://github.com/Level/community/blob/master/CONTRIBUTING.md) for more details.\n\n### Windows\n\nA large portion of the Windows support comes from code by [Krzysztof Kowalczyk](http://blog.kowalczyk.info/) [@kjk](https://twitter.com/kjk), see his Windows LevelDB port [here](http://code.google.com/r/kkowalczyk-leveldb/). If you're using LevelUP on Windows, you should give him your thanks!\n\n\n<a name=\"license\"></a>\nLicense & copyright\n-------------------\n\nCopyright © 2012-2015 **LevelUP** [contributors](https://github.com/level/community#contributors).\n\n**LevelUP** is licensed under the MIT license. All rights not explicitly granted in the MIT license are reserved. See the included `LICENSE.md` file for more details.\n\n=======\n*LevelUP builds on the excellent work of the LevelDB and Snappy teams from Google and additional contributors. LevelDB and Snappy are both issued under the [New BSD Licence](http://opensource.org/licenses/BSD-3-Clause).*\n", | |
readmeFilename:"README.md",bugs:{url:"https://github.com/level/levelup/issues"},_id:"levelup@1.3.1",_from:"levelup@^1.3.1"}},{}],55:[function(require,module,exports){(function(process,global,Buffer){var inherits=require("inherits"),AbstractLevelDOWN=require("abstract-leveldown").AbstractLevelDOWN,AbstractIterator=require("abstract-leveldown").AbstractIterator,ltgt=require("ltgt"),setImmediate=global.setImmediate||process.nextTick,createRBT=require("functional-red-black-tree"),globalStore={};function toKey(key){return typeof key=="string"?"$"+key:JSON.stringify(key)}function gt(value){return ltgt.compare(value,this._end)>0}function gte(value){return ltgt.compare(value,this._end)>=0}function lt(value){return ltgt.compare(value,this._end)<0}function lte(value){return ltgt.compare(value,this._end)<=0}function MemIterator(db,options){AbstractIterator.call(this,db);this._limit=options.limit;if(this._limit===-1)this._limit=Infinity;var tree=db._store[db._location];this.keyAsBuffer=options.keyAsBuffer!==false;this.valueAsBuffer=options.valueAsBuffer!==false;this._reverse=options.reverse;this._options=options;this._done=0;if(!this._reverse){this._incr="next";this._start=ltgt.lowerBound(options);this._end=ltgt.upperBound(options);if(typeof this._start==="undefined")this._tree=tree.begin;else if(ltgt.lowerBoundInclusive(options))this._tree=tree.ge(this._start);else this._tree=tree.gt(this._start);if(this._end){if(ltgt.upperBoundInclusive(options))this._test=lte;else this._test=lt}}else{this._incr="prev";this._start=ltgt.upperBound(options);this._end=ltgt.lowerBound(options);if(typeof this._start==="undefined")this._tree=tree.end;else if(ltgt.upperBoundInclusive(options))this._tree=tree.le(this._start);else this._tree=tree.lt(this._start);if(this._end){if(ltgt.lowerBoundInclusive(options))this._test=gte;else this._test=gt}}}inherits(MemIterator,AbstractIterator);MemIterator.prototype._next=function(callback){var key,value;if(this._done++>=this._limit)return setImmediate(callback);if(!this._tree.valid)return setImmediate(callback);key=this._tree.key;value=this._tree.value;if(!this._test(key))return setImmediate(callback);if(this.keyAsBuffer)key=new Buffer(key);if(this.valueAsBuffer)value=new Buffer(value);this._tree[this._incr]();setImmediate(function callNext(){callback(null,key,value)})};MemIterator.prototype._test=function(){return true};function MemDOWN(location){if(!(this instanceof MemDOWN))return new MemDOWN(location);AbstractLevelDOWN.call(this,typeof location=="string"?location:"");this._location=this.location?toKey(this.location):"_tree";this._store=this.location?globalStore:this;this._store[this._location]=this._store[this._location]||createRBT()}MemDOWN.clearGlobalStore=function(strict){if(strict){Object.keys(globalStore).forEach(function(key){delete globalStore[key]})}else{globalStore={}}};inherits(MemDOWN,AbstractLevelDOWN);MemDOWN.prototype._open=function(options,callback){var self=this;setImmediate(function callNext(){callback(null,self)})};MemDOWN.prototype._put=function(key,value,options,callback){if(typeof value==="undefined"||value===null)value="";this._store[this._location]=this._store[this._location].remove(key).insert(key,value);setImmediate(callback)};MemDOWN.prototype._get=function(key,options,callback){var value=this._store[this._location].get(key);if(value===undefined){var err=new Error("NotFound");return setImmediate(function callNext(){callback(err)})}if(options.asBuffer!==false&&!this._isBuffer(value))value=new Buffer(String(value));setImmediate(function callNext(){callback(null,value)})};MemDOWN.prototype._del=function(key,options,callback){this._store[this._location]=this._store[this._location].remove(key);setImmediate(callback)};MemDOWN.prototype._batch=function(array,options,callback){var err,i=-1,key,value,len=array.length,tree=this._store[this._location];while(++i<len){if(!array[i])continue;key=this._isBuffer(array[i].key)?array[i].key:String(array[i].key);err=this._checkKey(key,"key");if(err)return setImmediate(function errorCall(){callback(err)});tree=tree.remove(array[i].key);if(array[i].type==="put"){value=this._isBuffer(array[i].value)?array[i].value:String(array[i].value);err=this._checkKey(value,"value");if(err)return setImmediate(function errorCall(){callback(err)});tree=tree.insert(key,value)}}this._store[this._location]=tree;setImmediate(callback)};MemDOWN.prototype._iterator=function(options){return new MemIterator(this,options)};MemDOWN.prototype._isBuffer=function(obj){return Buffer.isBuffer(obj)};MemDOWN.destroy=function(name,callback){var key=toKey(name);if(key in globalStore)delete globalStore[key];setImmediate(callback)};module.exports=MemDOWN}).call(this,require("_process"),typeof global!=="undefined"?global:typeof self!=="undefined"?self:typeof window!=="undefined"?window:{},require("buffer").Buffer)},{_process:9,"abstract-leveldown":59,buffer:2,"functional-red-black-tree":62,inherits:63,ltgt:64}],56:[function(require,module,exports){(function(process){function AbstractChainedBatch(db){this._db=db;this._operations=[];this._written=false}AbstractChainedBatch.prototype._checkWritten=function(){if(this._written)throw new Error("write() already called on this batch")};AbstractChainedBatch.prototype.put=function(key,value){this._checkWritten();var err=this._db._checkKey(key,"key",this._db._isBuffer);if(err)throw err;if(!this._db._isBuffer(key))key=String(key);if(!this._db._isBuffer(value))value=String(value);if(typeof this._put=="function")this._put(key,value);else this._operations.push({type:"put",key:key,value:value});return this};AbstractChainedBatch.prototype.del=function(key){this._checkWritten();var err=this._db._checkKey(key,"key",this._db._isBuffer);if(err)throw err;if(!this._db._isBuffer(key))key=String(key);if(typeof this._del=="function")this._del(key);else this._operations.push({type:"del",key:key});return this};AbstractChainedBatch.prototype.clear=function(){this._checkWritten();this._operations=[];if(typeof this._clear=="function")this._clear();return this};AbstractChainedBatch.prototype.write=function(options,callback){this._checkWritten();if(typeof options=="function")callback=options;if(typeof callback!="function")throw new Error("write() requires a callback argument");if(typeof options!="object")options={};this._written=true;if(typeof this._write=="function")return this._write(callback);if(typeof this._db._batch=="function")return this._db._batch(this._operations,options,callback);process.nextTick(callback)};module.exports=AbstractChainedBatch}).call(this,require("_process"))},{_process:9}],57:[function(require,module,exports){(function(process){function AbstractIterator(db){this.db=db;this._ended=false;this._nexting=false}AbstractIterator.prototype.next=function(callback){var self=this;if(typeof callback!="function")throw new Error("next() requires a callback argument");if(self._ended)return callback(new Error("cannot call next() after end()"));if(self._nexting)return callback(new Error("cannot call next() before previous next() has completed"));self._nexting=true;if(typeof self._next=="function"){return self._next(function(){self._nexting=false;callback.apply(null,arguments)})}process.nextTick(function(){self._nexting=false;callback()})};AbstractIterator.prototype.end=function(callback){if(typeof callback!="function")throw new Error("end() requires a callback argument");if(this._ended)return callback(new Error("end() already called on iterator"));this._ended=true;if(typeof this._end=="function")return this._end(callback);process.nextTick(callback)};module.exports=AbstractIterator}).call(this,require("_process"))},{_process:9}],58:[function(require,module,exports){(function(process,Buffer){var xtend=require("xtend"),AbstractIterator=require("./abstract-iterator"),AbstractChainedBatch=require("./abstract-chained-batch");function AbstractLevelDOWN(location){if(!arguments.length||location===undefined)throw new Error("constructor requires at least a location argument");if(typeof location!="string")throw new Error("constructor requires a location string argument");this.location=location;this.status="new"}AbstractLevelDOWN.prototype.open=function(options,callback){var self=this,oldStatus=this.status;if(typeof options=="function")callback=options;if(typeof callback!="function")throw new Error("open() requires a callback argument");if(typeof options!="object")options={};options.createIfMissing=options.createIfMissing!=false;options.errorIfExists=!!options.errorIfExists;if(typeof this._open=="function"){this.status="opening";this._open(options,function(err){if(err){self.status=oldStatus;return callback(err)}self.status="open";callback()})}else{this.status="open";process.nextTick(callback)}};AbstractLevelDOWN.prototype.close=function(callback){var self=this,oldStatus=this.status;if(typeof callback!="function")throw new Error("close() requires a callback argument");if(typeof this._close=="function"){this.status="closing";this._close(function(err){if(err){self.status=oldStatus;return callback(err)}self.status="closed";callback()})}else{this.status="closed";process.nextTick(callback)}};AbstractLevelDOWN.prototype.get=function(key,options,callback){var err;if(typeof options=="function")callback=options;if(typeof callback!="function")throw new Error("get() requires a callback argument");if(err=this._checkKey(key,"key",this._isBuffer))return callback(err);if(!this._isBuffer(key))key=String(key);if(typeof options!="object")options={};options.asBuffer=options.asBuffer!=false;if(typeof this._get=="function")return this._get(key,options,callback);process.nextTick(function(){callback(new Error("NotFound"))})};AbstractLevelDOWN.prototype.put=function(key,value,options,callback){var err;if(typeof options=="function")callback=options;if(typeof callback!="function")throw new Error("put() requires a callback argument");if(err=this._checkKey(key,"key",this._isBuffer))return callback(err);if(!this._isBuffer(key))key=String(key);if(value!=null&&!this._isBuffer(value)&&!process.browser)value=String(value);if(typeof options!="object")options={};if(typeof this._put=="function")return this._put(key,value,options,callback);process.nextTick(callback)};AbstractLevelDOWN.prototype.del=function(key,options,callback){var err;if(typeof options=="function")callback=options;if(typeof callback!="function")throw new Error("del() requires a callback argument");if(err=this._checkKey(key,"key",this._isBuffer))return callback(err);if(!this._isBuffer(key))key=String(key);if(typeof options!="object")options={};if(typeof this._del=="function")return this._del(key,options,callback);process.nextTick(callback)};AbstractLevelDOWN.prototype.batch=function(array,options,callback){if(!arguments.length)return this._chainedBatch();if(typeof options=="function")callback=options;if(typeof array=="function")callback=array;if(typeof callback!="function")throw new Error("batch(array) requires a callback argument");if(!Array.isArray(array))return callback(new Error("batch(array) requires an array argument"));if(!options||typeof options!="object")options={};var i=0,l=array.length,e,err;for(;i<l;i++){e=array[i];if(typeof e!="object")continue;if(err=this._checkKey(e.type,"type",this._isBuffer))return callback(err);if(err=this._checkKey(e.key,"key",this._isBuffer))return callback(err)}if(typeof this._batch=="function")return this._batch(array,options,callback);process.nextTick(callback)};AbstractLevelDOWN.prototype.approximateSize=function(start,end,callback){if(start==null||end==null||typeof start=="function"||typeof end=="function"){throw new Error("approximateSize() requires valid `start`, `end` and `callback` arguments")}if(typeof callback!="function")throw new Error("approximateSize() requires a callback argument");if(!this._isBuffer(start))start=String(start);if(!this._isBuffer(end))end=String(end);if(typeof this._approximateSize=="function")return this._approximateSize(start,end,callback);process.nextTick(function(){callback(null,0)})};AbstractLevelDOWN.prototype._setupIteratorOptions=function(options){var self=this;options=xtend(options);["start","end","gt","gte","lt","lte"].forEach(function(o){if(options[o]&&self._isBuffer(options[o])&&options[o].length===0)delete options[o]});options.reverse=!!options.reverse;options.keys=options.keys!=false;options.values=options.values!=false;options.limit="limit"in options?options.limit:-1;options.keyAsBuffer=options.keyAsBuffer!=false;options.valueAsBuffer=options.valueAsBuffer!=false;return options};AbstractLevelDOWN.prototype.iterator=function(options){if(typeof options!="object")options={};options=this._setupIteratorOptions(options);if(typeof this._iterator=="function")return this._iterator(options);return new AbstractIterator(this)};AbstractLevelDOWN.prototype._chainedBatch=function(){return new AbstractChainedBatch(this)};AbstractLevelDOWN.prototype._isBuffer=function(obj){return Buffer.isBuffer(obj)};AbstractLevelDOWN.prototype._checkKey=function(obj,type){if(obj===null||obj===undefined)return new Error(type+" cannot be `null` or `undefined`");if(this._isBuffer(obj)){if(obj.length===0)return new Error(type+" cannot be an empty Buffer")}else if(String(obj)==="")return new Error(type+" cannot be an empty String")};module.exports=AbstractLevelDOWN}).call(this,require("_process"),require("buffer").Buffer)},{"./abstract-chained-batch":56,"./abstract-iterator":57,_process:9,buffer:2,xtend:61}],59:[function(require,module,exports){arguments[4][33][0].apply(exports,arguments)},{"./abstract-chained-batch":56,"./abstract-iterator":57,"./abstract-leveldown":58,"./is-leveldown":60,dup:33}],60:[function(require,module,exports){arguments[4][34][0].apply(exports,arguments)},{"./abstract-leveldown":58,dup:34}],61:[function(require,module,exports){arguments[4][53][0].apply(exports,arguments)},{dup:53}],62:[function(require,module,exports){"use strict";module.exports=createRBTree;var RED=0;var BLACK=1;function RBNode(color,key,value,left,right,count){this._color=color;this.key=key;this.value=value;this.left=left;this.right=right;this._count=count}function cloneNode(node){return new RBNode(node._color,node.key,node.value,node.left,node.right,node._count)}function repaint(color,node){return new RBNode(color,node.key,node.value,node.left,node.right,node._count)}function recount(node){node._count=1+(node.left?node.left._count:0)+(node.right?node.right._count:0)}function RedBlackTree(compare,root){this._compare=compare;this.root=root}var proto=RedBlackTree.prototype;Object.defineProperty(proto,"keys",{get:function(){var result=[];this.forEach(function(k,v){result.push(k)});return result}});Object.defineProperty(proto,"values",{get:function(){var result=[];this.forEach(function(k,v){result.push(v)});return result}});Object.defineProperty(proto,"length",{get:function(){if(this.root){return this.root._count}return 0}});proto.insert=function(key,value){var cmp=this._compare;var n=this.root;var n_stack=[];var d_stack=[];while(n){var d=cmp(key,n.key);n_stack.push(n);d_stack.push(d);if(d<=0){n=n.left}else{n=n.right}}n_stack.push(new RBNode(RED,key,value,null,null,1));for(var s=n_stack.length-2;s>=0;--s){var n=n_stack[s];if(d_stack[s]<=0){n_stack[s]=new RBNode(n._color,n.key,n.value,n_stack[s+1],n.right,n._count+1)}else{n_stack[s]=new RBNode(n._color,n.key,n.value,n.left,n_stack[s+1],n._count+1)}}for(var s=n_stack.length-1;s>1;--s){var p=n_stack[s-1];var n=n_stack[s];if(p._color===BLACK||n._color===BLACK){break}var pp=n_stack[s-2];if(pp.left===p){if(p.left===n){var y=pp.right;if(y&&y._color===RED){p._color=BLACK;pp.right=repaint(BLACK,y);pp._color=RED;s-=1}else{pp._color=RED;pp.left=p.right;p._color=BLACK;p.right=pp;n_stack[s-2]=p;n_stack[s-1]=n;recount(pp);recount(p);if(s>=3){var ppp=n_stack[s-3];if(ppp.left===pp){ppp.left=p}else{ppp.right=p}}break}}else{var y=pp.right;if(y&&y._color===RED){p._color=BLACK;pp.right=repaint(BLACK,y);pp._color=RED;s-=1}else{p.right=n.left;pp._color=RED;pp.left=n.right;n._color=BLACK;n.left=p;n.right=pp;n_stack[s-2]=n;n_stack[s-1]=p;recount(pp);recount(p);recount(n);if(s>=3){var ppp=n_stack[s-3];if(ppp.left===pp){ppp.left=n}else{ppp.right=n}}break}}}else{if(p.right===n){var y=pp.left;if(y&&y._color===RED){p._color=BLACK;pp.left=repaint(BLACK,y);pp._color=RED;s-=1}else{pp._color=RED;pp.right=p.left;p._color=BLACK;p.left=pp;n_stack[s-2]=p;n_stack[s-1]=n;recount(pp);recount(p);if(s>=3){var ppp=n_stack[s-3];if(ppp.right===pp){ppp.right=p}else{ppp.left=p}}break}}else{var y=pp.left;if(y&&y._color===RED){p._color=BLACK;pp.left=repaint(BLACK,y);pp._color=RED;s-=1}else{p.left=n.right;pp._color=RED;pp.right=n.left;n._color=BLACK;n.right=p;n.left=pp;n_stack[s-2]=n;n_stack[s-1]=p;recount(pp);recount(p);recount(n);if(s>=3){var ppp=n_stack[s-3];if(ppp.right===pp){ppp.right=n}else{ppp.left=n}}break}}}}n_stack[0]._color=BLACK;return new RedBlackTree(cmp,n_stack[0])};function doVisitFull(visit,node){if(node.left){var v=doVisitFull(visit,node.left);if(v){return v}}var v=visit(node.key,node.value);if(v){return v}if(node.right){return doVisitFull(visit,node.right)}}function doVisitHalf(lo,compare,visit,node){var l=compare(lo,node.key);if(l<=0){if(node.left){var v=doVisitHalf(lo,compare,visit,node.left);if(v){return v}}var v=visit(node.key,node.value);if(v){return v}}if(node.right){return doVisitHalf(lo,compare,visit,node.right)}}function doVisit(lo,hi,compare,visit,node){var l=compare(lo,node.key);var h=compare(hi,node.key);var v;if(l<=0){if(node.left){v=doVisit(lo,hi,compare,visit,node.left);if(v){return v}}if(h>0){v=visit(node.key,node.value);if(v){return v}}}if(h>0&&node.right){return doVisit(lo,hi,compare,visit,node.right)}}proto.forEach=function rbTreeForEach(visit,lo,hi){if(!this.root){return}switch(arguments.length){case 1:return doVisitFull(visit,this.root);break;case 2:return doVisitHalf(lo,this._compare,visit,this.root);break;case 3:if(this._compare(lo,hi)>=0){return}return doVisit(lo,hi,this._compare,visit,this.root);break}};Object.defineProperty(proto,"begin",{get:function(){var stack=[];var n=this.root;while(n){stack.push(n);n=n.left}return new RedBlackTreeIterator(this,stack)}});Object.defineProperty(proto,"end",{get:function(){var stack=[];var n=this.root;while(n){stack.push(n);n=n.right}return new RedBlackTreeIterator(this,stack)}});proto.at=function(idx){if(idx<0){return new RedBlackTreeIterator(this,[])}var n=this.root;var stack=[];while(true){stack.push(n);if(n.left){if(idx<n.left._count){n=n.left;continue}idx-=n.left._count}if(!idx){return new RedBlackTreeIterator(this,stack)}idx-=1;if(n.right){if(idx>=n.right._count){break}n=n.right}else{break}}return new RedBlackTreeIterator(this,[])};proto.ge=function(key){var cmp=this._compare;var n=this.root;var stack=[];var last_ptr=0;while(n){var d=cmp(key,n.key);stack.push(n);if(d<=0){last_ptr=stack.length}if(d<=0){n=n.left}else{n=n.right}}stack.length=last_ptr;return new RedBlackTreeIterator(this,stack)};proto.gt=function(key){var cmp=this._compare;var n=this.root;var stack=[];var last_ptr=0;while(n){var d=cmp(key,n.key);stack.push(n);if(d<0){last_ptr=stack.length}if(d<0){n=n.left}else{n=n.right}}stack.length=last_ptr;return new RedBlackTreeIterator(this,stack)};proto.lt=function(key){var cmp=this._compare;var n=this.root;var stack=[];var last_ptr=0;while(n){var d=cmp(key,n.key);stack.push(n);if(d>0){last_ptr=stack.length}if(d<=0){n=n.left}else{n=n.right}}stack.length=last_ptr;return new RedBlackTreeIterator(this,stack)};proto.le=function(key){var cmp=this._compare;var n=this.root;var stack=[];var last_ptr=0;while(n){var d=cmp(key,n.key);stack.push(n);if(d>=0){last_ptr=stack.length}if(d<0){n=n.left}else{n=n.right}}stack.length=last_ptr;return new RedBlackTreeIterator(this,stack)};proto.find=function(key){var cmp=this._compare;var n=this.root;var stack=[];while(n){var d=cmp(key,n.key);stack.push(n);if(d===0){return new RedBlackTreeIterator(this,stack)}if(d<=0){n=n.left}else{n=n.right}}return new RedBlackTreeIterator(this,[])};proto.remove=function(key){var iter=this.find(key);if(iter){return iter.remove()}return this};proto.get=function(key){var cmp=this._compare;var n=this.root;while(n){var d=cmp(key,n.key);if(d===0){return n.value}if(d<=0){n=n.left}else{n=n.right}}return};function RedBlackTreeIterator(tree,stack){this.tree=tree;this._stack=stack}var iproto=RedBlackTreeIterator.prototype;Object.defineProperty(iproto,"valid",{get:function(){return this._stack.length>0}});Object.defineProperty(iproto,"node",{get:function(){if(this._stack.length>0){return this._stack[this._stack.length-1]}return null},enumerable:true});iproto.clone=function(){return new RedBlackTreeIterator(this.tree,this._stack.slice())};function swapNode(n,v){n.key=v.key;n.value=v.value;n.left=v.left;n.right=v.right;n._color=v._color;n._count=v._count}function fixDoubleBlack(stack){var n,p,s,z;for(var i=stack.length-1;i>=0;--i){n=stack[i];if(i===0){n._color=BLACK;return}p=stack[i-1];if(p.left===n){s=p.right;if(s.right&&s.right._color===RED){s=p.right=cloneNode(s);z=s.right=cloneNode(s.right);p.right=s.left;s.left=p;s.right=z;s._color=p._color;n._color=BLACK;p._color=BLACK;z._color=BLACK;recount(p);recount(s);if(i>1){var pp=stack[i-2];if(pp.left===p){pp.left=s}else{pp.right=s}}stack[i-1]=s;return}else if(s.left&&s.left._color===RED){s=p.right=cloneNode(s);z=s.left=cloneNode(s.left);p.right=z.left;s.left=z.right;z.left=p;z.right=s;z._color=p._color;p._color=BLACK;s._color=BLACK;n._color=BLACK;recount(p);recount(s);recount(z);if(i>1){var pp=stack[i-2];if(pp.left===p){pp.left=z}else{pp.right=z}}stack[i-1]=z;return}if(s._color===BLACK){if(p._color===RED){p._color=BLACK;p.right=repaint(RED,s);return}else{p.right=repaint(RED,s);continue}}else{s=cloneNode(s);p.right=s.left;s.left=p;s._color=p._color;p._color=RED;recount(p);recount(s);if(i>1){var pp=stack[i-2];if(pp.left===p){pp.left=s}else{pp.right=s}}stack[i-1]=s;stack[i]=p;if(i+1<stack.length){stack[i+1]=n}else{stack.push(n)}i=i+2}}else{s=p.left;if(s.left&&s.left._color===RED){s=p.left=cloneNode(s);z=s.left=cloneNode(s.left);p.left=s.right;s.right=p;s.left=z;s._color=p._color;n._color=BLACK;p._color=BLACK;z._color=BLACK;recount(p);recount(s);if(i>1){var pp=stack[i-2];if(pp.right===p){pp.right=s}else{pp.left=s}}stack[i-1]=s;return}else if(s.right&&s.right._color===RED){s=p.left=cloneNode(s);z=s.right=cloneNode(s.right);p.left=z.right;s.right=z.left;z.right=p;z.left=s;z._color=p._color;p._color=BLACK;s._color=BLACK;n._color=BLACK;recount(p);recount(s);recount(z);if(i>1){var pp=stack[i-2];if(pp.right===p){pp.right=z}else{pp.left=z}}stack[i-1]=z;return}if(s._color===BLACK){if(p._color===RED){p._color=BLACK;p.left=repaint(RED,s);return}else{p.left=repaint(RED,s);continue}}else{s=cloneNode(s);p.left=s.right;s.right=p;s._color=p._color;p._color=RED;recount(p);recount(s);if(i>1){var pp=stack[i-2];if(pp.right===p){pp.right=s}else{pp.left=s}}stack[i-1]=s;stack[i]=p;if(i+1<stack.length){stack[i+1]=n}else{stack.push(n)}i=i+2}}}}iproto.remove=function(){var stack=this._stack;if(stack.length===0){return this.tree}var cstack=new Array(stack.length);var n=stack[stack.length-1];cstack[cstack.length-1]=new RBNode(n._color,n.key,n.value,n.left,n.right,n._count);for(var i=stack.length-2;i>=0;--i){var n=stack[i];if(n.left===stack[i+1]){cstack[i]=new RBNode(n._color,n.key,n.value,cstack[i+1],n.right,n._count)}else{cstack[i]=new RBNode(n._color,n.key,n.value,n.left,cstack[i+1],n._count)}}n=cstack[cstack.length-1];if(n.left&&n.right){var split=cstack.length;n=n.left;while(n.right){cstack.push(n);n=n.right}var v=cstack[split-1];cstack.push(new RBNode(n._color,v.key,v.value,n.left,n.right,n._count));cstack[split-1].key=n.key;cstack[split-1].value=n.value;for(var i=cstack.length-2;i>=split;--i){n=cstack[i];cstack[i]=new RBNode(n._color,n.key,n.value,n.left,cstack[i+1],n._count)}cstack[split-1].left=cstack[split]}n=cstack[cstack.length-1];if(n._color===RED){var p=cstack[cstack.length-2];if(p.left===n){p.left=null}else if(p.right===n){p.right=null}cstack.pop();for(var i=0;i<cstack.length;++i){cstack[i]._count--}return new RedBlackTree(this.tree._compare,cstack[0])}else{if(n.left||n.right){if(n.left){swapNode(n,n.left)}else if(n.right){swapNode(n,n.right)}n._color=BLACK;for(var i=0;i<cstack.length-1;++i){cstack[i]._count--}return new RedBlackTree(this.tree._compare,cstack[0])}else if(cstack.length===1){return new RedBlackTree(this.tree._compare,null)}else{for(var i=0;i<cstack.length;++i){cstack[i]._count--}var parent=cstack[cstack.length-2];fixDoubleBlack(cstack);if(parent.left===n){parent.left=null}else{parent.right=null}}}return new RedBlackTree(this.tree._compare,cstack[0])};Object.defineProperty(iproto,"key",{get:function(){if(this._stack.length>0){return this._stack[this._stack.length-1].key}return},enumerable:true});Object.defineProperty(iproto,"value",{get:function(){if(this._stack.length>0){return this._stack[this._stack.length-1].value}return},enumerable:true});Object.defineProperty(iproto,"index",{get:function(){var idx=0;var stack=this._stack;if(stack.length===0){var r=this.tree.root;if(r){return r._count}return 0}else if(stack[stack.length-1].left){idx=stack[stack.length-1].left._count}for(var s=stack.length-2;s>=0;--s){if(stack[s+1]===stack[s].right){++idx;if(stack[s].left){idx+=stack[s].left._count}}}return idx},enumerable:true});iproto.next=function(){var stack=this._stack;if(stack.length===0){return}var n=stack[stack.length-1];if(n.right){n=n.right;while(n){stack.push(n);n=n.left}}else{stack.pop();while(stack.length>0&&stack[stack.length-1].right===n){n=stack[stack.length-1];stack.pop()}}};Object.defineProperty(iproto,"hasNext",{get:function(){var stack=this._stack;if(stack.length===0){return false}if(stack[stack.length-1].right){return true}for(var s=stack.length-1;s>0;--s){if(stack[s-1].left===stack[s]){return true}}return false}});iproto.update=function(value){var stack=this._stack;if(stack.length===0){throw new Error("Can't update empty node!")}var cstack=new Array(stack.length);var n=stack[stack.length-1];cstack[cstack.length-1]=new RBNode(n._color,n.key,value,n.left,n.right,n._count);for(var i=stack.length-2;i>=0;--i){n=stack[i];if(n.left===stack[i+1]){cstack[i]=new RBNode(n._color,n.key,n.value,cstack[i+1],n.right,n._count)}else{cstack[i]=new RBNode(n._color,n.key,n.value,n.left,cstack[i+1],n._count)}}return new RedBlackTree(this.tree._compare,cstack[0])};iproto.prev=function(){var stack=this._stack;if(stack.length===0){return}var n=stack[stack.length-1];if(n.left){n=n.left;while(n){stack.push(n);n=n.right}}else{stack.pop();while(stack.length>0&&stack[stack.length-1].left===n){n=stack[stack.length-1];stack.pop()}}};Object.defineProperty(iproto,"hasPrev",{get:function(){var stack=this._stack;if(stack.length===0){return false}if(stack[stack.length-1].left){return true}for(var s=stack.length-1;s>0;--s){if(stack[s-1].right===stack[s]){return true}}return false}});function defaultCompare(a,b){if(a<b){return-1}if(a>b){return 1}return 0}function createRBTree(compare){return new RedBlackTree(compare||defaultCompare,null)}},{}],63:[function(require,module,exports){arguments[4][7][0].apply(exports,arguments)},{dup:7}],64:[function(require,module,exports){(function(Buffer){exports.compare=function(a,b){if(Buffer.isBuffer(a)){var l=Math.min(a.length,b.length);for(var i=0;i<l;i++){var cmp=a[i]-b[i];if(cmp)return cmp}return a.length-b.length}return a<b?-1:a>b?1:0};function has(obj,key){return Object.hasOwnProperty.call(obj,key)}function isDef(val){return val!=null&&val!==""}var lowerBound=exports.lowerBound=function(range){return isDef(range.gt)?range.gt:isDef(range.gte)?range.gte:isDef(range.min)?range.min:isDef(range.start)&&!range.reverse?range.start:isDef(range.end)&&range.reverse?range.end:undefined};exports.lowerBoundInclusive=function(range){return isDef(range.gt)?false:true};exports.upperBoundInclusive=function(range){return isDef(range.lt)?false:true};var lowerBoundExclusive=exports.lowerBoundExclusive=function(range){return isDef(range.gt)?true:false};var upperBoundExclusive=exports.upperBoundExclusive=function(range){return isDef(range.lt)?true:false};var upperBound=exports.upperBound=function(range){return isDef(range.lt)?range.lt:isDef(range.lte)?range.lte:isDef(range.max)?range.max:isDef(range.start)&&range.reverse?range.start:isDef(range.end)&&!range.reverse?range.end:undefined};exports.contains=function(range,key,compare){compare=compare||exports.compare;var lb=lowerBound(range);if(isDef(lb)){var cmp=compare(key,lb);if(cmp<0||cmp===0&&lowerBoundExclusive(range))return false}var ub=upperBound(range);if(isDef(ub)){var cmp=compare(key,ub);if(cmp>0||cmp===0&&upperBoundExclusive(range))return false}return true};exports.filter=function(range,compare){return function(key){return exports.contains(range,key,compare)}}}).call(this,require("buffer").Buffer)},{buffer:2}],memdb:[function(require,module,exports){var levelup=require("levelup");var memdown=require("memdown");module.exports=MemDB;function MemDB(opts,fn){if(typeof opts=="function"){fn=opts;opts={}}if(typeof opts=="string")opts={};opts=opts||{};opts.db=function(l){return new memdown(l)};return levelup("",opts,fn)}},{levelup:26,memdown:55}]},{},[]);require=function e(t,n,r){function s(o,u){if(!n[o]){if(!t[o]){var a=typeof require=="function"&&require;if(!u&&a)return a(o,!0);if(i)return i(o,!0);var f=new Error("Cannot find module '"+o+"'");throw f.code="MODULE_NOT_FOUND",f}var l=n[o]={exports:{}};t[o][0].call(l.exports,function(e){var n=t[o][1][e];return s(n?n:e)},l,l.exports,e,t,n,r)}return n[o].exports}var i=typeof require=="function"&&require;for(var o=0;o<r.length;o++)s(r[o]);return s}({1:[function(require,module,exports){var base64=require("base64-js");var ieee754=require("ieee754");var isArray=require("is-array");exports.Buffer=Buffer;exports.SlowBuffer=SlowBuffer;exports.INSPECT_MAX_BYTES=50;Buffer.poolSize=8192;var kMaxLength=1073741823;var rootParent={};Buffer.TYPED_ARRAY_SUPPORT=function(){try{var buf=new ArrayBuffer(0);var arr=new Uint8Array(buf);arr.foo=function(){return 42};return 42===arr.foo()&&typeof arr.subarray==="function"&&new Uint8Array(1).subarray(1,1).byteLength===0}catch(e){return false}}();function Buffer(subject,encoding,noZero){if(!(this instanceof Buffer))return new Buffer(subject,encoding,noZero);var type=typeof subject;var length;if(type==="number")length=subject>0?subject>>>0:0;else if(type==="string"){length=Buffer.byteLength(subject,encoding)}else if(type==="object"&&subject!==null){if(subject.type==="Buffer"&&isArray(subject.data))subject=subject.data;length=+subject.length>0?Math.floor(+subject.length):0}else throw new TypeError("must start with number, buffer, array or string");if(length>kMaxLength)throw new RangeError("Attempt to allocate Buffer larger than maximum "+"size: 0x"+kMaxLength.toString(16)+" bytes");var buf;if(Buffer.TYPED_ARRAY_SUPPORT){buf=Buffer._augment(new Uint8Array(length))}else{buf=this;buf.length=length;buf._isBuffer=true}var i;if(Buffer.TYPED_ARRAY_SUPPORT&&typeof subject.byteLength==="number"){buf._set(subject)}else if(isArrayish(subject)){if(Buffer.isBuffer(subject)){for(i=0;i<length;i++)buf[i]=subject.readUInt8(i)}else{for(i=0;i<length;i++)buf[i]=(subject[i]%256+256)%256}}else if(type==="string"){buf.write(subject,0,encoding)}else if(type==="number"&&!Buffer.TYPED_ARRAY_SUPPORT&&!noZero){for(i=0;i<length;i++){buf[i]=0}}if(length>0&&length<=Buffer.poolSize)buf.parent=rootParent;return buf}function SlowBuffer(subject,encoding,noZero){if(!(this instanceof SlowBuffer))return new SlowBuffer(subject,encoding,noZero);var buf=new Buffer(subject,encoding,noZero);delete buf.parent;return buf}Buffer.isBuffer=function(b){return!!(b!=null&&b._isBuffer)};Buffer.compare=function(a,b){if(!Buffer.isBuffer(a)||!Buffer.isBuffer(b))throw new TypeError("Arguments must be Buffers");var x=a.length;var y=b.length;for(var i=0,len=Math.min(x,y);i<len&&a[i]===b[i];i++){}if(i!==len){x=a[i];y=b[i]}if(x<y)return-1;if(y<x)return 1;return 0};Buffer.isEncoding=function(encoding){switch(String(encoding).toLowerCase()){case"hex":case"utf8":case"utf-8":case"ascii":case"binary":case"base64":case"raw":case"ucs2":case"ucs-2": | |
case"utf16le":case"utf-16le":return true;default:return false}};Buffer.concat=function(list,totalLength){if(!isArray(list))throw new TypeError("Usage: Buffer.concat(list[, length])");if(list.length===0){return new Buffer(0)}else if(list.length===1){return list[0]}var i;if(totalLength===undefined){totalLength=0;for(i=0;i<list.length;i++){totalLength+=list[i].length}}var buf=new Buffer(totalLength);var pos=0;for(i=0;i<list.length;i++){var item=list[i];item.copy(buf,pos);pos+=item.length}return buf};Buffer.byteLength=function(str,encoding){var ret;str=str+"";switch(encoding||"utf8"){case"ascii":case"binary":case"raw":ret=str.length;break;case"ucs2":case"ucs-2":case"utf16le":case"utf-16le":ret=str.length*2;break;case"hex":ret=str.length>>>1;break;case"utf8":case"utf-8":ret=utf8ToBytes(str).length;break;case"base64":ret=base64ToBytes(str).length;break;default:ret=str.length}return ret};Buffer.prototype.length=undefined;Buffer.prototype.parent=undefined;Buffer.prototype.toString=function(encoding,start,end){var loweredCase=false;start=start>>>0;end=end===undefined||end===Infinity?this.length:end>>>0;if(!encoding)encoding="utf8";if(start<0)start=0;if(end>this.length)end=this.length;if(end<=start)return"";while(true){switch(encoding){case"hex":return hexSlice(this,start,end);case"utf8":case"utf-8":return utf8Slice(this,start,end);case"ascii":return asciiSlice(this,start,end);case"binary":return binarySlice(this,start,end);case"base64":return base64Slice(this,start,end);case"ucs2":case"ucs-2":case"utf16le":case"utf-16le":return utf16leSlice(this,start,end);default:if(loweredCase)throw new TypeError("Unknown encoding: "+encoding);encoding=(encoding+"").toLowerCase();loweredCase=true}}};Buffer.prototype.equals=function(b){if(!Buffer.isBuffer(b))throw new TypeError("Argument must be a Buffer");return Buffer.compare(this,b)===0};Buffer.prototype.inspect=function(){var str="";var max=exports.INSPECT_MAX_BYTES;if(this.length>0){str=this.toString("hex",0,max).match(/.{2}/g).join(" ");if(this.length>max)str+=" ... "}return"<Buffer "+str+">"};Buffer.prototype.compare=function(b){if(!Buffer.isBuffer(b))throw new TypeError("Argument must be a Buffer");return Buffer.compare(this,b)};Buffer.prototype.get=function(offset){console.log(".get() is deprecated. Access using array indexes instead.");return this.readUInt8(offset)};Buffer.prototype.set=function(v,offset){console.log(".set() is deprecated. Access using array indexes instead.");return this.writeUInt8(v,offset)};function hexWrite(buf,string,offset,length){offset=Number(offset)||0;var remaining=buf.length-offset;if(!length){length=remaining}else{length=Number(length);if(length>remaining){length=remaining}}var strLen=string.length;if(strLen%2!==0)throw new Error("Invalid hex string");if(length>strLen/2){length=strLen/2}for(var i=0;i<length;i++){var byte=parseInt(string.substr(i*2,2),16);if(isNaN(byte))throw new Error("Invalid hex string");buf[offset+i]=byte}return i}function utf8Write(buf,string,offset,length){var charsWritten=blitBuffer(utf8ToBytes(string,buf.length-offset),buf,offset,length);return charsWritten}function asciiWrite(buf,string,offset,length){var charsWritten=blitBuffer(asciiToBytes(string),buf,offset,length);return charsWritten}function binaryWrite(buf,string,offset,length){return asciiWrite(buf,string,offset,length)}function base64Write(buf,string,offset,length){var charsWritten=blitBuffer(base64ToBytes(string),buf,offset,length);return charsWritten}function utf16leWrite(buf,string,offset,length){var charsWritten=blitBuffer(utf16leToBytes(string,buf.length-offset),buf,offset,length,2);return charsWritten}Buffer.prototype.write=function(string,offset,length,encoding){if(isFinite(offset)){if(!isFinite(length)){encoding=length;length=undefined}}else{var swap=encoding;encoding=offset;offset=length;length=swap}offset=Number(offset)||0;if(length<0||offset<0||offset>this.length)throw new RangeError("attempt to write outside buffer bounds");var remaining=this.length-offset;if(!length){length=remaining}else{length=Number(length);if(length>remaining){length=remaining}}encoding=String(encoding||"utf8").toLowerCase();var ret;switch(encoding){case"hex":ret=hexWrite(this,string,offset,length);break;case"utf8":case"utf-8":ret=utf8Write(this,string,offset,length);break;case"ascii":ret=asciiWrite(this,string,offset,length);break;case"binary":ret=binaryWrite(this,string,offset,length);break;case"base64":ret=base64Write(this,string,offset,length);break;case"ucs2":case"ucs-2":case"utf16le":case"utf-16le":ret=utf16leWrite(this,string,offset,length);break;default:throw new TypeError("Unknown encoding: "+encoding)}return ret};Buffer.prototype.toJSON=function(){return{type:"Buffer",data:Array.prototype.slice.call(this._arr||this,0)}};function base64Slice(buf,start,end){if(start===0&&end===buf.length){return base64.fromByteArray(buf)}else{return base64.fromByteArray(buf.slice(start,end))}}function utf8Slice(buf,start,end){var res="";var tmp="";end=Math.min(buf.length,end);for(var i=start;i<end;i++){if(buf[i]<=127){res+=decodeUtf8Char(tmp)+String.fromCharCode(buf[i]);tmp=""}else{tmp+="%"+buf[i].toString(16)}}return res+decodeUtf8Char(tmp)}function asciiSlice(buf,start,end){var ret="";end=Math.min(buf.length,end);for(var i=start;i<end;i++){ret+=String.fromCharCode(buf[i]&127)}return ret}function binarySlice(buf,start,end){var ret="";end=Math.min(buf.length,end);for(var i=start;i<end;i++){ret+=String.fromCharCode(buf[i])}return ret}function hexSlice(buf,start,end){var len=buf.length;if(!start||start<0)start=0;if(!end||end<0||end>len)end=len;var out="";for(var i=start;i<end;i++){out+=toHex(buf[i])}return out}function utf16leSlice(buf,start,end){var bytes=buf.slice(start,end);var res="";for(var i=0;i<bytes.length;i+=2){res+=String.fromCharCode(bytes[i]+bytes[i+1]*256)}return res}Buffer.prototype.slice=function(start,end){var len=this.length;start=~~start;end=end===undefined?len:~~end;if(start<0){start+=len;if(start<0)start=0}else if(start>len){start=len}if(end<0){end+=len;if(end<0)end=0}else if(end>len){end=len}if(end<start)end=start;var newBuf;if(Buffer.TYPED_ARRAY_SUPPORT){newBuf=Buffer._augment(this.subarray(start,end))}else{var sliceLen=end-start;newBuf=new Buffer(sliceLen,undefined,true);for(var i=0;i<sliceLen;i++){newBuf[i]=this[i+start]}}if(newBuf.length)newBuf.parent=this.parent||this;return newBuf};function checkOffset(offset,ext,length){if(offset%1!==0||offset<0)throw new RangeError("offset is not uint");if(offset+ext>length)throw new RangeError("Trying to access beyond buffer length")}Buffer.prototype.readUIntLE=function(offset,byteLength,noAssert){offset=offset>>>0;byteLength=byteLength>>>0;if(!noAssert)checkOffset(offset,byteLength,this.length);var val=this[offset];var mul=1;var i=0;while(++i<byteLength&&(mul*=256))val+=this[offset+i]*mul;return val};Buffer.prototype.readUIntBE=function(offset,byteLength,noAssert){offset=offset>>>0;byteLength=byteLength>>>0;if(!noAssert)checkOffset(offset,byteLength,this.length);var val=this[offset+--byteLength];var mul=1;while(byteLength>0&&(mul*=256))val+=this[offset+--byteLength]*mul;return val};Buffer.prototype.readUInt8=function(offset,noAssert){if(!noAssert)checkOffset(offset,1,this.length);return this[offset]};Buffer.prototype.readUInt16LE=function(offset,noAssert){if(!noAssert)checkOffset(offset,2,this.length);return this[offset]|this[offset+1]<<8};Buffer.prototype.readUInt16BE=function(offset,noAssert){if(!noAssert)checkOffset(offset,2,this.length);return this[offset]<<8|this[offset+1]};Buffer.prototype.readUInt32LE=function(offset,noAssert){if(!noAssert)checkOffset(offset,4,this.length);return(this[offset]|this[offset+1]<<8|this[offset+2]<<16)+this[offset+3]*16777216};Buffer.prototype.readUInt32BE=function(offset,noAssert){if(!noAssert)checkOffset(offset,4,this.length);return this[offset]*16777216+(this[offset+1]<<16|this[offset+2]<<8|this[offset+3])};Buffer.prototype.readIntLE=function(offset,byteLength,noAssert){offset=offset>>>0;byteLength=byteLength>>>0;if(!noAssert)checkOffset(offset,byteLength,this.length);var val=this[offset];var mul=1;var i=0;while(++i<byteLength&&(mul*=256))val+=this[offset+i]*mul;mul*=128;if(val>=mul)val-=Math.pow(2,8*byteLength);return val};Buffer.prototype.readIntBE=function(offset,byteLength,noAssert){offset=offset>>>0;byteLength=byteLength>>>0;if(!noAssert)checkOffset(offset,byteLength,this.length);var i=byteLength;var mul=1;var val=this[offset+--i];while(i>0&&(mul*=256))val+=this[offset+--i]*mul;mul*=128;if(val>=mul)val-=Math.pow(2,8*byteLength);return val};Buffer.prototype.readInt8=function(offset,noAssert){if(!noAssert)checkOffset(offset,1,this.length);if(!(this[offset]&128))return this[offset];return(255-this[offset]+1)*-1};Buffer.prototype.readInt16LE=function(offset,noAssert){if(!noAssert)checkOffset(offset,2,this.length);var val=this[offset]|this[offset+1]<<8;return val&32768?val|4294901760:val};Buffer.prototype.readInt16BE=function(offset,noAssert){if(!noAssert)checkOffset(offset,2,this.length);var val=this[offset+1]|this[offset]<<8;return val&32768?val|4294901760:val};Buffer.prototype.readInt32LE=function(offset,noAssert){if(!noAssert)checkOffset(offset,4,this.length);return this[offset]|this[offset+1]<<8|this[offset+2]<<16|this[offset+3]<<24};Buffer.prototype.readInt32BE=function(offset,noAssert){if(!noAssert)checkOffset(offset,4,this.length);return this[offset]<<24|this[offset+1]<<16|this[offset+2]<<8|this[offset+3]};Buffer.prototype.readFloatLE=function(offset,noAssert){if(!noAssert)checkOffset(offset,4,this.length);return ieee754.read(this,offset,true,23,4)};Buffer.prototype.readFloatBE=function(offset,noAssert){if(!noAssert)checkOffset(offset,4,this.length);return ieee754.read(this,offset,false,23,4)};Buffer.prototype.readDoubleLE=function(offset,noAssert){if(!noAssert)checkOffset(offset,8,this.length);return ieee754.read(this,offset,true,52,8)};Buffer.prototype.readDoubleBE=function(offset,noAssert){if(!noAssert)checkOffset(offset,8,this.length);return ieee754.read(this,offset,false,52,8)};function checkInt(buf,value,offset,ext,max,min){if(!Buffer.isBuffer(buf))throw new TypeError("buffer must be a Buffer instance");if(value>max||value<min)throw new RangeError("value is out of bounds");if(offset+ext>buf.length)throw new RangeError("index out of range")}Buffer.prototype.writeUIntLE=function(value,offset,byteLength,noAssert){value=+value;offset=offset>>>0;byteLength=byteLength>>>0;if(!noAssert)checkInt(this,value,offset,byteLength,Math.pow(2,8*byteLength),0);var mul=1;var i=0;this[offset]=value&255;while(++i<byteLength&&(mul*=256))this[offset+i]=value/mul>>>0&255;return offset+byteLength};Buffer.prototype.writeUIntBE=function(value,offset,byteLength,noAssert){value=+value;offset=offset>>>0;byteLength=byteLength>>>0;if(!noAssert)checkInt(this,value,offset,byteLength,Math.pow(2,8*byteLength),0);var i=byteLength-1;var mul=1;this[offset+i]=value&255;while(--i>=0&&(mul*=256))this[offset+i]=value/mul>>>0&255;return offset+byteLength};Buffer.prototype.writeUInt8=function(value,offset,noAssert){value=+value;offset=offset>>>0;if(!noAssert)checkInt(this,value,offset,1,255,0);if(!Buffer.TYPED_ARRAY_SUPPORT)value=Math.floor(value);this[offset]=value;return offset+1};function objectWriteUInt16(buf,value,offset,littleEndian){if(value<0)value=65535+value+1;for(var i=0,j=Math.min(buf.length-offset,2);i<j;i++){buf[offset+i]=(value&255<<8*(littleEndian?i:1-i))>>>(littleEndian?i:1-i)*8}}Buffer.prototype.writeUInt16LE=function(value,offset,noAssert){value=+value;offset=offset>>>0;if(!noAssert)checkInt(this,value,offset,2,65535,0);if(Buffer.TYPED_ARRAY_SUPPORT){this[offset]=value;this[offset+1]=value>>>8}else objectWriteUInt16(this,value,offset,true);return offset+2};Buffer.prototype.writeUInt16BE=function(value,offset,noAssert){value=+value;offset=offset>>>0;if(!noAssert)checkInt(this,value,offset,2,65535,0);if(Buffer.TYPED_ARRAY_SUPPORT){this[offset]=value>>>8;this[offset+1]=value}else objectWriteUInt16(this,value,offset,false);return offset+2};function objectWriteUInt32(buf,value,offset,littleEndian){if(value<0)value=4294967295+value+1;for(var i=0,j=Math.min(buf.length-offset,4);i<j;i++){buf[offset+i]=value>>>(littleEndian?i:3-i)*8&255}}Buffer.prototype.writeUInt32LE=function(value,offset,noAssert){value=+value;offset=offset>>>0;if(!noAssert)checkInt(this,value,offset,4,4294967295,0);if(Buffer.TYPED_ARRAY_SUPPORT){this[offset+3]=value>>>24;this[offset+2]=value>>>16;this[offset+1]=value>>>8;this[offset]=value}else objectWriteUInt32(this,value,offset,true);return offset+4};Buffer.prototype.writeUInt32BE=function(value,offset,noAssert){value=+value;offset=offset>>>0;if(!noAssert)checkInt(this,value,offset,4,4294967295,0);if(Buffer.TYPED_ARRAY_SUPPORT){this[offset]=value>>>24;this[offset+1]=value>>>16;this[offset+2]=value>>>8;this[offset+3]=value}else objectWriteUInt32(this,value,offset,false);return offset+4};Buffer.prototype.writeIntLE=function(value,offset,byteLength,noAssert){value=+value;offset=offset>>>0;if(!noAssert){checkInt(this,value,offset,byteLength,Math.pow(2,8*byteLength-1)-1,-Math.pow(2,8*byteLength-1))}var i=0;var mul=1;var sub=value<0?1:0;this[offset]=value&255;while(++i<byteLength&&(mul*=256))this[offset+i]=(value/mul>>0)-sub&255;return offset+byteLength};Buffer.prototype.writeIntBE=function(value,offset,byteLength,noAssert){value=+value;offset=offset>>>0;if(!noAssert){checkInt(this,value,offset,byteLength,Math.pow(2,8*byteLength-1)-1,-Math.pow(2,8*byteLength-1))}var i=byteLength-1;var mul=1;var sub=value<0?1:0;this[offset+i]=value&255;while(--i>=0&&(mul*=256))this[offset+i]=(value/mul>>0)-sub&255;return offset+byteLength};Buffer.prototype.writeInt8=function(value,offset,noAssert){value=+value;offset=offset>>>0;if(!noAssert)checkInt(this,value,offset,1,127,-128);if(!Buffer.TYPED_ARRAY_SUPPORT)value=Math.floor(value);if(value<0)value=255+value+1;this[offset]=value;return offset+1};Buffer.prototype.writeInt16LE=function(value,offset,noAssert){value=+value;offset=offset>>>0;if(!noAssert)checkInt(this,value,offset,2,32767,-32768);if(Buffer.TYPED_ARRAY_SUPPORT){this[offset]=value;this[offset+1]=value>>>8}else objectWriteUInt16(this,value,offset,true);return offset+2};Buffer.prototype.writeInt16BE=function(value,offset,noAssert){value=+value;offset=offset>>>0;if(!noAssert)checkInt(this,value,offset,2,32767,-32768);if(Buffer.TYPED_ARRAY_SUPPORT){this[offset]=value>>>8;this[offset+1]=value}else objectWriteUInt16(this,value,offset,false);return offset+2};Buffer.prototype.writeInt32LE=function(value,offset,noAssert){value=+value;offset=offset>>>0;if(!noAssert)checkInt(this,value,offset,4,2147483647,-2147483648);if(Buffer.TYPED_ARRAY_SUPPORT){this[offset]=value;this[offset+1]=value>>>8;this[offset+2]=value>>>16;this[offset+3]=value>>>24}else objectWriteUInt32(this,value,offset,true);return offset+4};Buffer.prototype.writeInt32BE=function(value,offset,noAssert){value=+value;offset=offset>>>0;if(!noAssert)checkInt(this,value,offset,4,2147483647,-2147483648);if(value<0)value=4294967295+value+1;if(Buffer.TYPED_ARRAY_SUPPORT){this[offset]=value>>>24;this[offset+1]=value>>>16;this[offset+2]=value>>>8;this[offset+3]=value}else objectWriteUInt32(this,value,offset,false);return offset+4};function checkIEEE754(buf,value,offset,ext,max,min){if(value>max||value<min)throw new RangeError("value is out of bounds");if(offset+ext>buf.length)throw new RangeError("index out of range");if(offset<0)throw new RangeError("index out of range")}function writeFloat(buf,value,offset,littleEndian,noAssert){if(!noAssert)checkIEEE754(buf,value,offset,4,3.4028234663852886e38,-3.4028234663852886e38);ieee754.write(buf,value,offset,littleEndian,23,4);return offset+4}Buffer.prototype.writeFloatLE=function(value,offset,noAssert){return writeFloat(this,value,offset,true,noAssert)};Buffer.prototype.writeFloatBE=function(value,offset,noAssert){return writeFloat(this,value,offset,false,noAssert)};function writeDouble(buf,value,offset,littleEndian,noAssert){if(!noAssert)checkIEEE754(buf,value,offset,8,1.7976931348623157e308,-1.7976931348623157e308);ieee754.write(buf,value,offset,littleEndian,52,8);return offset+8}Buffer.prototype.writeDoubleLE=function(value,offset,noAssert){return writeDouble(this,value,offset,true,noAssert)};Buffer.prototype.writeDoubleBE=function(value,offset,noAssert){return writeDouble(this,value,offset,false,noAssert)};Buffer.prototype.copy=function(target,target_start,start,end){var source=this;if(!start)start=0;if(!end&&end!==0)end=this.length;if(target_start>=target.length)target_start=target.length;if(!target_start)target_start=0;if(end>0&&end<start)end=start;if(end===start)return 0;if(target.length===0||source.length===0)return 0;if(target_start<0)throw new RangeError("targetStart out of bounds");if(start<0||start>=source.length)throw new RangeError("sourceStart out of bounds");if(end<0)throw new RangeError("sourceEnd out of bounds");if(end>this.length)end=this.length;if(target.length-target_start<end-start)end=target.length-target_start+start;var len=end-start;if(len<1e3||!Buffer.TYPED_ARRAY_SUPPORT){for(var i=0;i<len;i++){target[i+target_start]=this[i+start]}}else{target._set(this.subarray(start,start+len),target_start)}return len};Buffer.prototype.fill=function(value,start,end){if(!value)value=0;if(!start)start=0;if(!end)end=this.length;if(end<start)throw new RangeError("end < start");if(end===start)return;if(this.length===0)return;if(start<0||start>=this.length)throw new RangeError("start out of bounds");if(end<0||end>this.length)throw new RangeError("end out of bounds");var i;if(typeof value==="number"){for(i=start;i<end;i++){this[i]=value}}else{var bytes=utf8ToBytes(value.toString());var len=bytes.length;for(i=start;i<end;i++){this[i]=bytes[i%len]}}return this};Buffer.prototype.toArrayBuffer=function(){if(typeof Uint8Array!=="undefined"){if(Buffer.TYPED_ARRAY_SUPPORT){return new Buffer(this).buffer}else{var buf=new Uint8Array(this.length);for(var i=0,len=buf.length;i<len;i+=1){buf[i]=this[i]}return buf.buffer}}else{throw new TypeError("Buffer.toArrayBuffer not supported in this browser")}};var BP=Buffer.prototype;Buffer._augment=function(arr){arr.constructor=Buffer;arr._isBuffer=true;arr._get=arr.get;arr._set=arr.set;arr.get=BP.get;arr.set=BP.set;arr.write=BP.write;arr.toString=BP.toString;arr.toLocaleString=BP.toString;arr.toJSON=BP.toJSON;arr.equals=BP.equals;arr.compare=BP.compare;arr.copy=BP.copy;arr.slice=BP.slice;arr.readUIntLE=BP.readUIntLE;arr.readUIntBE=BP.readUIntBE;arr.readUInt8=BP.readUInt8;arr.readUInt16LE=BP.readUInt16LE;arr.readUInt16BE=BP.readUInt16BE;arr.readUInt32LE=BP.readUInt32LE;arr.readUInt32BE=BP.readUInt32BE;arr.readIntLE=BP.readIntLE;arr.readIntBE=BP.readIntBE;arr.readInt8=BP.readInt8;arr.readInt16LE=BP.readInt16LE;arr.readInt16BE=BP.readInt16BE;arr.readInt32LE=BP.readInt32LE;arr.readInt32BE=BP.readInt32BE;arr.readFloatLE=BP.readFloatLE;arr.readFloatBE=BP.readFloatBE;arr.readDoubleLE=BP.readDoubleLE;arr.readDoubleBE=BP.readDoubleBE;arr.writeUInt8=BP.writeUInt8;arr.writeUIntLE=BP.writeUIntLE;arr.writeUIntBE=BP.writeUIntBE;arr.writeUInt16LE=BP.writeUInt16LE;arr.writeUInt16BE=BP.writeUInt16BE;arr.writeUInt32LE=BP.writeUInt32LE;arr.writeUInt32BE=BP.writeUInt32BE;arr.writeIntLE=BP.writeIntLE;arr.writeIntBE=BP.writeIntBE;arr.writeInt8=BP.writeInt8;arr.writeInt16LE=BP.writeInt16LE;arr.writeInt16BE=BP.writeInt16BE;arr.writeInt32LE=BP.writeInt32LE;arr.writeInt32BE=BP.writeInt32BE;arr.writeFloatLE=BP.writeFloatLE;arr.writeFloatBE=BP.writeFloatBE;arr.writeDoubleLE=BP.writeDoubleLE;arr.writeDoubleBE=BP.writeDoubleBE;arr.fill=BP.fill;arr.inspect=BP.inspect;arr.toArrayBuffer=BP.toArrayBuffer;return arr};var INVALID_BASE64_RE=/[^+\/0-9A-z\-]/g;function base64clean(str){str=stringtrim(str).replace(INVALID_BASE64_RE,"");if(str.length<2)return"";while(str.length%4!==0){str=str+"="}return str}function stringtrim(str){if(str.trim)return str.trim();return str.replace(/^\s+|\s+$/g,"")}function isArrayish(subject){return isArray(subject)||Buffer.isBuffer(subject)||subject&&typeof subject==="object"&&typeof subject.length==="number"}function toHex(n){if(n<16)return"0"+n.toString(16);return n.toString(16)}function utf8ToBytes(string,units){var codePoint,length=string.length;var leadSurrogate=null;units=units||Infinity;var bytes=[];var i=0;for(;i<length;i++){codePoint=string.charCodeAt(i);if(codePoint>55295&&codePoint<57344){if(leadSurrogate){if(codePoint<56320){if((units-=3)>-1)bytes.push(239,191,189);leadSurrogate=codePoint;continue}else{codePoint=leadSurrogate-55296<<10|codePoint-56320|65536;leadSurrogate=null}}else{if(codePoint>56319){if((units-=3)>-1)bytes.push(239,191,189);continue}else if(i+1===length){if((units-=3)>-1)bytes.push(239,191,189);continue}else{leadSurrogate=codePoint;continue}}}else if(leadSurrogate){if((units-=3)>-1)bytes.push(239,191,189);leadSurrogate=null}if(codePoint<128){if((units-=1)<0)break;bytes.push(codePoint)}else if(codePoint<2048){if((units-=2)<0)break;bytes.push(codePoint>>6|192,codePoint&63|128)}else if(codePoint<65536){if((units-=3)<0)break;bytes.push(codePoint>>12|224,codePoint>>6&63|128,codePoint&63|128)}else if(codePoint<2097152){if((units-=4)<0)break;bytes.push(codePoint>>18|240,codePoint>>12&63|128,codePoint>>6&63|128,codePoint&63|128)}else{throw new Error("Invalid code point")}}return bytes}function asciiToBytes(str){var byteArray=[];for(var i=0;i<str.length;i++){byteArray.push(str.charCodeAt(i)&255)}return byteArray}function utf16leToBytes(str,units){var c,hi,lo;var byteArray=[];for(var i=0;i<str.length;i++){if((units-=2)<0)break;c=str.charCodeAt(i);hi=c>>8;lo=c%256;byteArray.push(lo);byteArray.push(hi)}return byteArray}function base64ToBytes(str){return base64.toByteArray(base64clean(str))}function blitBuffer(src,dst,offset,length,unitSize){if(unitSize)length-=length%unitSize;for(var i=0;i<length;i++){if(i+offset>=dst.length||i>=src.length)break;dst[i+offset]=src[i]}return i}function decodeUtf8Char(str){try{return decodeURIComponent(str)}catch(err){return String.fromCharCode(65533)}}},{"base64-js":2,ieee754:3,"is-array":4}],2:[function(require,module,exports){var lookup="ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789+/";(function(exports){"use strict";var Arr=typeof Uint8Array!=="undefined"?Uint8Array:Array;var PLUS="+".charCodeAt(0);var SLASH="/".charCodeAt(0);var NUMBER="0".charCodeAt(0);var LOWER="a".charCodeAt(0);var UPPER="A".charCodeAt(0);var PLUS_URL_SAFE="-".charCodeAt(0);var SLASH_URL_SAFE="_".charCodeAt(0);function decode(elt){var code=elt.charCodeAt(0);if(code===PLUS||code===PLUS_URL_SAFE)return 62;if(code===SLASH||code===SLASH_URL_SAFE)return 63;if(code<NUMBER)return-1;if(code<NUMBER+10)return code-NUMBER+26+26;if(code<UPPER+26)return code-UPPER;if(code<LOWER+26)return code-LOWER+26}function b64ToByteArray(b64){var i,j,l,tmp,placeHolders,arr;if(b64.length%4>0){throw new Error("Invalid string. Length must be a multiple of 4")}var len=b64.length;placeHolders="="===b64.charAt(len-2)?2:"="===b64.charAt(len-1)?1:0;arr=new Arr(b64.length*3/4-placeHolders);l=placeHolders>0?b64.length-4:b64.length;var L=0;function push(v){arr[L++]=v}for(i=0,j=0;i<l;i+=4,j+=3){tmp=decode(b64.charAt(i))<<18|decode(b64.charAt(i+1))<<12|decode(b64.charAt(i+2))<<6|decode(b64.charAt(i+3));push((tmp&16711680)>>16);push((tmp&65280)>>8);push(tmp&255)}if(placeHolders===2){tmp=decode(b64.charAt(i))<<2|decode(b64.charAt(i+1))>>4;push(tmp&255)}else if(placeHolders===1){tmp=decode(b64.charAt(i))<<10|decode(b64.charAt(i+1))<<4|decode(b64.charAt(i+2))>>2;push(tmp>>8&255);push(tmp&255)}return arr}function uint8ToBase64(uint8){var i,extraBytes=uint8.length%3,output="",temp,length;function encode(num){return lookup.charAt(num)}function tripletToBase64(num){return encode(num>>18&63)+encode(num>>12&63)+encode(num>>6&63)+encode(num&63)}for(i=0,length=uint8.length-extraBytes;i<length;i+=3){temp=(uint8[i]<<16)+(uint8[i+1]<<8)+uint8[i+2];output+=tripletToBase64(temp)}switch(extraBytes){case 1:temp=uint8[uint8.length-1];output+=encode(temp>>2);output+=encode(temp<<4&63);output+="==";break;case 2:temp=(uint8[uint8.length-2]<<8)+uint8[uint8.length-1];output+=encode(temp>>10);output+=encode(temp>>4&63);output+=encode(temp<<2&63);output+="=";break}return output}exports.toByteArray=b64ToByteArray;exports.fromByteArray=uint8ToBase64})(typeof exports==="undefined"?this.base64js={}:exports)},{}],3:[function(require,module,exports){exports.read=function(buffer,offset,isLE,mLen,nBytes){var e,m,eLen=nBytes*8-mLen-1,eMax=(1<<eLen)-1,eBias=eMax>>1,nBits=-7,i=isLE?nBytes-1:0,d=isLE?-1:1,s=buffer[offset+i];i+=d;e=s&(1<<-nBits)-1;s>>=-nBits;nBits+=eLen;for(;nBits>0;e=e*256+buffer[offset+i],i+=d,nBits-=8);m=e&(1<<-nBits)-1;e>>=-nBits;nBits+=mLen;for(;nBits>0;m=m*256+buffer[offset+i],i+=d,nBits-=8);if(e===0){e=1-eBias}else if(e===eMax){return m?NaN:(s?-1:1)*Infinity}else{m=m+Math.pow(2,mLen);e=e-eBias}return(s?-1:1)*m*Math.pow(2,e-mLen)};exports.write=function(buffer,value,offset,isLE,mLen,nBytes){var e,m,c,eLen=nBytes*8-mLen-1,eMax=(1<<eLen)-1,eBias=eMax>>1,rt=mLen===23?Math.pow(2,-24)-Math.pow(2,-77):0,i=isLE?0:nBytes-1,d=isLE?1:-1,s=value<0||value===0&&1/value<0?1:0;value=Math.abs(value);if(isNaN(value)||value===Infinity){m=isNaN(value)?1:0;e=eMax}else{e=Math.floor(Math.log(value)/Math.LN2);if(value*(c=Math.pow(2,-e))<1){e--;c*=2}if(e+eBias>=1){value+=rt/c}else{value+=rt*Math.pow(2,1-eBias)}if(value*c>=2){e++;c/=2}if(e+eBias>=eMax){m=0;e=eMax}else if(e+eBias>=1){m=(value*c-1)*Math.pow(2,mLen);e=e+eBias}else{m=value*Math.pow(2,eBias-1)*Math.pow(2,mLen);e=0}}for(;mLen>=8;buffer[offset+i]=m&255,i+=d,m/=256,mLen-=8);e=e<<mLen|m;eLen+=mLen;for(;eLen>0;buffer[offset+i]=e&255,i+=d,e/=256,eLen-=8);buffer[offset+i-d]|=s*128}},{}],4:[function(require,module,exports){var isArray=Array.isArray;var str=Object.prototype.toString;module.exports=isArray||function(val){return!!val&&"[object Array]"==str.call(val)}},{}],5:[function(require,module,exports){function EventEmitter(){this._events=this._events||{};this._maxListeners=this._maxListeners||undefined}module.exports=EventEmitter;EventEmitter.EventEmitter=EventEmitter;EventEmitter.prototype._events=undefined;EventEmitter.prototype._maxListeners=undefined;EventEmitter.defaultMaxListeners=10;EventEmitter.prototype.setMaxListeners=function(n){if(!isNumber(n)||n<0||isNaN(n))throw TypeError("n must be a positive number");this._maxListeners=n;return this};EventEmitter.prototype.emit=function(type){var er,handler,len,args,i,listeners;if(!this._events)this._events={};if(type==="error"){if(!this._events.error||isObject(this._events.error)&&!this._events.error.length){er=arguments[1];if(er instanceof Error){throw er}throw TypeError('Uncaught, unspecified "error" event.')}}handler=this._events[type];if(isUndefined(handler))return false;if(isFunction(handler)){switch(arguments.length){case 1:handler.call(this);break;case 2:handler.call(this,arguments[1]);break;case 3:handler.call(this,arguments[1],arguments[2]);break;default:len=arguments.length;args=new Array(len-1);for(i=1;i<len;i++)args[i-1]=arguments[i];handler.apply(this,args)}}else if(isObject(handler)){len=arguments.length;args=new Array(len-1);for(i=1;i<len;i++)args[i-1]=arguments[i];listeners=handler.slice();len=listeners.length;for(i=0;i<len;i++)listeners[i].apply(this,args)}return true};EventEmitter.prototype.addListener=function(type,listener){var m;if(!isFunction(listener))throw TypeError("listener must be a function");if(!this._events)this._events={};if(this._events.newListener)this.emit("newListener",type,isFunction(listener.listener)?listener.listener:listener);if(!this._events[type])this._events[type]=listener;else if(isObject(this._events[type]))this._events[type].push(listener);else this._events[type]=[this._events[type],listener];if(isObject(this._events[type])&&!this._events[type].warned){var m;if(!isUndefined(this._maxListeners)){m=this._maxListeners}else{m=EventEmitter.defaultMaxListeners}if(m&&m>0&&this._events[type].length>m){this._events[type].warned=true;console.error("(node) warning: possible EventEmitter memory "+"leak detected. %d listeners added. "+"Use emitter.setMaxListeners() to increase limit.",this._events[type].length);if(typeof console.trace==="function"){console.trace()}}}return this};EventEmitter.prototype.on=EventEmitter.prototype.addListener;EventEmitter.prototype.once=function(type,listener){if(!isFunction(listener))throw TypeError("listener must be a function");var fired=false;function g(){this.removeListener(type,g);if(!fired){fired=true;listener.apply(this,arguments)}}g.listener=listener;this.on(type,g);return this};EventEmitter.prototype.removeListener=function(type,listener){var list,position,length,i;if(!isFunction(listener))throw TypeError("listener must be a function");if(!this._events||!this._events[type])return this;list=this._events[type];length=list.length;position=-1;if(list===listener||isFunction(list.listener)&&list.listener===listener){delete this._events[type];if(this._events.removeListener)this.emit("removeListener",type,listener)}else if(isObject(list)){for(i=length;i-->0;){if(list[i]===listener||list[i].listener&&list[i].listener===listener){position=i;break}}if(position<0)return this;if(list.length===1){list.length=0;delete this._events[type]}else{list.splice(position,1)}if(this._events.removeListener)this.emit("removeListener",type,listener)}return this};EventEmitter.prototype.removeAllListeners=function(type){var key,listeners;if(!this._events)return this;if(!this._events.removeListener){if(arguments.length===0)this._events={};else if(this._events[type])delete this._events[type];return this}if(arguments.length===0){for(key in this._events){if(key==="removeListener")continue;this.removeAllListeners(key)}this.removeAllListeners("removeListener");this._events={};return this}listeners=this._events[type];if(isFunction(listeners)){this.removeListener(type,listeners)}else{while(listeners.length)this.removeListener(type,listeners[listeners.length-1])}delete this._events[type];return this};EventEmitter.prototype.listeners=function(type){var ret;if(!this._events||!this._events[type])ret=[];else if(isFunction(this._events[type]))ret=[this._events[type]];else ret=this._events[type].slice();return ret};EventEmitter.listenerCount=function(emitter,type){var ret;if(!emitter._events||!emitter._events[type])ret=0;else if(isFunction(emitter._events[type]))ret=1;else ret=emitter._events[type].length;return ret};function isFunction(arg){return typeof arg==="function"}function isNumber(arg){return typeof arg==="number"}function isObject(arg){return typeof arg==="object"&&arg!==null}function isUndefined(arg){return arg===void 0}},{}],6:[function(require,module,exports){if(typeof Object.create==="function"){module.exports=function inherits(ctor,superCtor){ctor.super_=superCtor;ctor.prototype=Object.create(superCtor.prototype,{constructor:{value:ctor,enumerable:false,writable:true,configurable:true}})}}else{module.exports=function inherits(ctor,superCtor){ctor.super_=superCtor;var TempCtor=function(){};TempCtor.prototype=superCtor.prototype;ctor.prototype=new TempCtor;ctor.prototype.constructor=ctor}}},{}],7:[function(require,module,exports){module.exports=Array.isArray||function(arr){return Object.prototype.toString.call(arr)=="[object Array]"}},{}],8:[function(require,module,exports){var process=module.exports={};process.nextTick=function(){var canSetImmediate=typeof window!=="undefined"&&window.setImmediate;var canMutationObserver=typeof window!=="undefined"&&window.MutationObserver;var canPost=typeof window!=="undefined"&&window.postMessage&&window.addEventListener;if(canSetImmediate){return function(f){return window.setImmediate(f)}}var queue=[];if(canMutationObserver){var hiddenDiv=document.createElement("div");var observer=new MutationObserver(function(){var queueList=queue.slice();queue.length=0;queueList.forEach(function(fn){fn()})});observer.observe(hiddenDiv,{attributes:true});return function nextTick(fn){if(!queue.length){hiddenDiv.setAttribute("yes","no")}queue.push(fn)}}if(canPost){window.addEventListener("message",function(ev){var source=ev.source; | |
if((source===window||source===null)&&ev.data==="process-tick"){ev.stopPropagation();if(queue.length>0){var fn=queue.shift();fn()}}},true);return function nextTick(fn){queue.push(fn);window.postMessage("process-tick","*")}}return function nextTick(fn){setTimeout(fn,0)}}();process.title="browser";process.browser=true;process.env={};process.argv=[];function noop(){}process.on=noop;process.addListener=noop;process.once=noop;process.off=noop;process.removeListener=noop;process.removeAllListeners=noop;process.emit=noop;process.binding=function(name){throw new Error("process.binding is not supported")};process.cwd=function(){return"/"};process.chdir=function(dir){throw new Error("process.chdir is not supported")}},{}],9:[function(require,module,exports){module.exports=require("./lib/_stream_duplex.js")},{"./lib/_stream_duplex.js":10}],10:[function(require,module,exports){(function(process){module.exports=Duplex;var objectKeys=Object.keys||function(obj){var keys=[];for(var key in obj)keys.push(key);return keys};var util=require("core-util-is");util.inherits=require("inherits");var Readable=require("./_stream_readable");var Writable=require("./_stream_writable");util.inherits(Duplex,Readable);forEach(objectKeys(Writable.prototype),function(method){if(!Duplex.prototype[method])Duplex.prototype[method]=Writable.prototype[method]});function Duplex(options){if(!(this instanceof Duplex))return new Duplex(options);Readable.call(this,options);Writable.call(this,options);if(options&&options.readable===false)this.readable=false;if(options&&options.writable===false)this.writable=false;this.allowHalfOpen=true;if(options&&options.allowHalfOpen===false)this.allowHalfOpen=false;this.once("end",onend)}function onend(){if(this.allowHalfOpen||this._writableState.ended)return;process.nextTick(this.end.bind(this))}function forEach(xs,f){for(var i=0,l=xs.length;i<l;i++){f(xs[i],i)}}}).call(this,require("_process"))},{"./_stream_readable":12,"./_stream_writable":14,_process:8,"core-util-is":15,inherits:6}],11:[function(require,module,exports){module.exports=PassThrough;var Transform=require("./_stream_transform");var util=require("core-util-is");util.inherits=require("inherits");util.inherits(PassThrough,Transform);function PassThrough(options){if(!(this instanceof PassThrough))return new PassThrough(options);Transform.call(this,options)}PassThrough.prototype._transform=function(chunk,encoding,cb){cb(null,chunk)}},{"./_stream_transform":13,"core-util-is":15,inherits:6}],12:[function(require,module,exports){(function(process){module.exports=Readable;var isArray=require("isarray");var Buffer=require("buffer").Buffer;Readable.ReadableState=ReadableState;var EE=require("events").EventEmitter;if(!EE.listenerCount)EE.listenerCount=function(emitter,type){return emitter.listeners(type).length};var Stream=require("stream");var util=require("core-util-is");util.inherits=require("inherits");var StringDecoder;util.inherits(Readable,Stream);function ReadableState(options,stream){options=options||{};var hwm=options.highWaterMark;this.highWaterMark=hwm||hwm===0?hwm:16*1024;this.highWaterMark=~~this.highWaterMark;this.buffer=[];this.length=0;this.pipes=null;this.pipesCount=0;this.flowing=false;this.ended=false;this.endEmitted=false;this.reading=false;this.calledRead=false;this.sync=true;this.needReadable=false;this.emittedReadable=false;this.readableListening=false;this.objectMode=!!options.objectMode;this.defaultEncoding=options.defaultEncoding||"utf8";this.ranOut=false;this.awaitDrain=0;this.readingMore=false;this.decoder=null;this.encoding=null;if(options.encoding){if(!StringDecoder)StringDecoder=require("string_decoder/").StringDecoder;this.decoder=new StringDecoder(options.encoding);this.encoding=options.encoding}}function Readable(options){if(!(this instanceof Readable))return new Readable(options);this._readableState=new ReadableState(options,this);this.readable=true;Stream.call(this)}Readable.prototype.push=function(chunk,encoding){var state=this._readableState;if(typeof chunk==="string"&&!state.objectMode){encoding=encoding||state.defaultEncoding;if(encoding!==state.encoding){chunk=new Buffer(chunk,encoding);encoding=""}}return readableAddChunk(this,state,chunk,encoding,false)};Readable.prototype.unshift=function(chunk){var state=this._readableState;return readableAddChunk(this,state,chunk,"",true)};function readableAddChunk(stream,state,chunk,encoding,addToFront){var er=chunkInvalid(state,chunk);if(er){stream.emit("error",er)}else if(chunk===null||chunk===undefined){state.reading=false;if(!state.ended)onEofChunk(stream,state)}else if(state.objectMode||chunk&&chunk.length>0){if(state.ended&&!addToFront){var e=new Error("stream.push() after EOF");stream.emit("error",e)}else if(state.endEmitted&&addToFront){var e=new Error("stream.unshift() after end event");stream.emit("error",e)}else{if(state.decoder&&!addToFront&&!encoding)chunk=state.decoder.write(chunk);state.length+=state.objectMode?1:chunk.length;if(addToFront){state.buffer.unshift(chunk)}else{state.reading=false;state.buffer.push(chunk)}if(state.needReadable)emitReadable(stream);maybeReadMore(stream,state)}}else if(!addToFront){state.reading=false}return needMoreData(state)}function needMoreData(state){return!state.ended&&(state.needReadable||state.length<state.highWaterMark||state.length===0)}Readable.prototype.setEncoding=function(enc){if(!StringDecoder)StringDecoder=require("string_decoder/").StringDecoder;this._readableState.decoder=new StringDecoder(enc);this._readableState.encoding=enc};var MAX_HWM=8388608;function roundUpToNextPowerOf2(n){if(n>=MAX_HWM){n=MAX_HWM}else{n--;for(var p=1;p<32;p<<=1)n|=n>>p;n++}return n}function howMuchToRead(n,state){if(state.length===0&&state.ended)return 0;if(state.objectMode)return n===0?0:1;if(n===null||isNaN(n)){if(state.flowing&&state.buffer.length)return state.buffer[0].length;else return state.length}if(n<=0)return 0;if(n>state.highWaterMark)state.highWaterMark=roundUpToNextPowerOf2(n);if(n>state.length){if(!state.ended){state.needReadable=true;return 0}else return state.length}return n}Readable.prototype.read=function(n){var state=this._readableState;state.calledRead=true;var nOrig=n;var ret;if(typeof n!=="number"||n>0)state.emittedReadable=false;if(n===0&&state.needReadable&&(state.length>=state.highWaterMark||state.ended)){emitReadable(this);return null}n=howMuchToRead(n,state);if(n===0&&state.ended){ret=null;if(state.length>0&&state.decoder){ret=fromList(n,state);state.length-=ret.length}if(state.length===0)endReadable(this);return ret}var doRead=state.needReadable;if(state.length-n<=state.highWaterMark)doRead=true;if(state.ended||state.reading)doRead=false;if(doRead){state.reading=true;state.sync=true;if(state.length===0)state.needReadable=true;this._read(state.highWaterMark);state.sync=false}if(doRead&&!state.reading)n=howMuchToRead(nOrig,state);if(n>0)ret=fromList(n,state);else ret=null;if(ret===null){state.needReadable=true;n=0}state.length-=n;if(state.length===0&&!state.ended)state.needReadable=true;if(state.ended&&!state.endEmitted&&state.length===0)endReadable(this);return ret};function chunkInvalid(state,chunk){var er=null;if(!Buffer.isBuffer(chunk)&&"string"!==typeof chunk&&chunk!==null&&chunk!==undefined&&!state.objectMode){er=new TypeError("Invalid non-string/buffer chunk")}return er}function onEofChunk(stream,state){if(state.decoder&&!state.ended){var chunk=state.decoder.end();if(chunk&&chunk.length){state.buffer.push(chunk);state.length+=state.objectMode?1:chunk.length}}state.ended=true;if(state.length>0)emitReadable(stream);else endReadable(stream)}function emitReadable(stream){var state=stream._readableState;state.needReadable=false;if(state.emittedReadable)return;state.emittedReadable=true;if(state.sync)process.nextTick(function(){emitReadable_(stream)});else emitReadable_(stream)}function emitReadable_(stream){stream.emit("readable")}function maybeReadMore(stream,state){if(!state.readingMore){state.readingMore=true;process.nextTick(function(){maybeReadMore_(stream,state)})}}function maybeReadMore_(stream,state){var len=state.length;while(!state.reading&&!state.flowing&&!state.ended&&state.length<state.highWaterMark){stream.read(0);if(len===state.length)break;else len=state.length}state.readingMore=false}Readable.prototype._read=function(n){this.emit("error",new Error("not implemented"))};Readable.prototype.pipe=function(dest,pipeOpts){var src=this;var state=this._readableState;switch(state.pipesCount){case 0:state.pipes=dest;break;case 1:state.pipes=[state.pipes,dest];break;default:state.pipes.push(dest);break}state.pipesCount+=1;var doEnd=(!pipeOpts||pipeOpts.end!==false)&&dest!==process.stdout&&dest!==process.stderr;var endFn=doEnd?onend:cleanup;if(state.endEmitted)process.nextTick(endFn);else src.once("end",endFn);dest.on("unpipe",onunpipe);function onunpipe(readable){if(readable!==src)return;cleanup()}function onend(){dest.end()}var ondrain=pipeOnDrain(src);dest.on("drain",ondrain);function cleanup(){dest.removeListener("close",onclose);dest.removeListener("finish",onfinish);dest.removeListener("drain",ondrain);dest.removeListener("error",onerror);dest.removeListener("unpipe",onunpipe);src.removeListener("end",onend);src.removeListener("end",cleanup);if(!dest._writableState||dest._writableState.needDrain)ondrain()}function onerror(er){unpipe();dest.removeListener("error",onerror);if(EE.listenerCount(dest,"error")===0)dest.emit("error",er)}if(!dest._events||!dest._events.error)dest.on("error",onerror);else if(isArray(dest._events.error))dest._events.error.unshift(onerror);else dest._events.error=[onerror,dest._events.error];function onclose(){dest.removeListener("finish",onfinish);unpipe()}dest.once("close",onclose);function onfinish(){dest.removeListener("close",onclose);unpipe()}dest.once("finish",onfinish);function unpipe(){src.unpipe(dest)}dest.emit("pipe",src);if(!state.flowing){this.on("readable",pipeOnReadable);state.flowing=true;process.nextTick(function(){flow(src)})}return dest};function pipeOnDrain(src){return function(){var dest=this;var state=src._readableState;state.awaitDrain--;if(state.awaitDrain===0)flow(src)}}function flow(src){var state=src._readableState;var chunk;state.awaitDrain=0;function write(dest,i,list){var written=dest.write(chunk);if(false===written){state.awaitDrain++}}while(state.pipesCount&&null!==(chunk=src.read())){if(state.pipesCount===1)write(state.pipes,0,null);else forEach(state.pipes,write);src.emit("data",chunk);if(state.awaitDrain>0)return}if(state.pipesCount===0){state.flowing=false;if(EE.listenerCount(src,"data")>0)emitDataEvents(src);return}state.ranOut=true}function pipeOnReadable(){if(this._readableState.ranOut){this._readableState.ranOut=false;flow(this)}}Readable.prototype.unpipe=function(dest){var state=this._readableState;if(state.pipesCount===0)return this;if(state.pipesCount===1){if(dest&&dest!==state.pipes)return this;if(!dest)dest=state.pipes;state.pipes=null;state.pipesCount=0;this.removeListener("readable",pipeOnReadable);state.flowing=false;if(dest)dest.emit("unpipe",this);return this}if(!dest){var dests=state.pipes;var len=state.pipesCount;state.pipes=null;state.pipesCount=0;this.removeListener("readable",pipeOnReadable);state.flowing=false;for(var i=0;i<len;i++)dests[i].emit("unpipe",this);return this}var i=indexOf(state.pipes,dest);if(i===-1)return this;state.pipes.splice(i,1);state.pipesCount-=1;if(state.pipesCount===1)state.pipes=state.pipes[0];dest.emit("unpipe",this);return this};Readable.prototype.on=function(ev,fn){var res=Stream.prototype.on.call(this,ev,fn);if(ev==="data"&&!this._readableState.flowing)emitDataEvents(this);if(ev==="readable"&&this.readable){var state=this._readableState;if(!state.readableListening){state.readableListening=true;state.emittedReadable=false;state.needReadable=true;if(!state.reading){this.read(0)}else if(state.length){emitReadable(this,state)}}}return res};Readable.prototype.addListener=Readable.prototype.on;Readable.prototype.resume=function(){emitDataEvents(this);this.read(0);this.emit("resume")};Readable.prototype.pause=function(){emitDataEvents(this,true);this.emit("pause")};function emitDataEvents(stream,startPaused){var state=stream._readableState;if(state.flowing){throw new Error("Cannot switch to old mode now.")}var paused=startPaused||false;var readable=false;stream.readable=true;stream.pipe=Stream.prototype.pipe;stream.on=stream.addListener=Stream.prototype.on;stream.on("readable",function(){readable=true;var c;while(!paused&&null!==(c=stream.read()))stream.emit("data",c);if(c===null){readable=false;stream._readableState.needReadable=true}});stream.pause=function(){paused=true;this.emit("pause")};stream.resume=function(){paused=false;if(readable)process.nextTick(function(){stream.emit("readable")});else this.read(0);this.emit("resume")};stream.emit("readable")}Readable.prototype.wrap=function(stream){var state=this._readableState;var paused=false;var self=this;stream.on("end",function(){if(state.decoder&&!state.ended){var chunk=state.decoder.end();if(chunk&&chunk.length)self.push(chunk)}self.push(null)});stream.on("data",function(chunk){if(state.decoder)chunk=state.decoder.write(chunk);if(state.objectMode&&(chunk===null||chunk===undefined))return;else if(!state.objectMode&&(!chunk||!chunk.length))return;var ret=self.push(chunk);if(!ret){paused=true;stream.pause()}});for(var i in stream){if(typeof stream[i]==="function"&&typeof this[i]==="undefined"){this[i]=function(method){return function(){return stream[method].apply(stream,arguments)}}(i)}}var events=["error","close","destroy","pause","resume"];forEach(events,function(ev){stream.on(ev,self.emit.bind(self,ev))});self._read=function(n){if(paused){paused=false;stream.resume()}};return self};Readable._fromList=fromList;function fromList(n,state){var list=state.buffer;var length=state.length;var stringMode=!!state.decoder;var objectMode=!!state.objectMode;var ret;if(list.length===0)return null;if(length===0)ret=null;else if(objectMode)ret=list.shift();else if(!n||n>=length){if(stringMode)ret=list.join("");else ret=Buffer.concat(list,length);list.length=0}else{if(n<list[0].length){var buf=list[0];ret=buf.slice(0,n);list[0]=buf.slice(n)}else if(n===list[0].length){ret=list.shift()}else{if(stringMode)ret="";else ret=new Buffer(n);var c=0;for(var i=0,l=list.length;i<l&&c<n;i++){var buf=list[0];var cpy=Math.min(n-c,buf.length);if(stringMode)ret+=buf.slice(0,cpy);else buf.copy(ret,c,0,cpy);if(cpy<buf.length)list[0]=buf.slice(cpy);else list.shift();c+=cpy}}}return ret}function endReadable(stream){var state=stream._readableState;if(state.length>0)throw new Error("endReadable called on non-empty stream");if(!state.endEmitted&&state.calledRead){state.ended=true;process.nextTick(function(){if(!state.endEmitted&&state.length===0){state.endEmitted=true;stream.readable=false;stream.emit("end")}})}}function forEach(xs,f){for(var i=0,l=xs.length;i<l;i++){f(xs[i],i)}}function indexOf(xs,x){for(var i=0,l=xs.length;i<l;i++){if(xs[i]===x)return i}return-1}}).call(this,require("_process"))},{_process:8,buffer:1,"core-util-is":15,events:5,inherits:6,isarray:7,stream:20,"string_decoder/":21}],13:[function(require,module,exports){module.exports=Transform;var Duplex=require("./_stream_duplex");var util=require("core-util-is");util.inherits=require("inherits");util.inherits(Transform,Duplex);function TransformState(options,stream){this.afterTransform=function(er,data){return afterTransform(stream,er,data)};this.needTransform=false;this.transforming=false;this.writecb=null;this.writechunk=null}function afterTransform(stream,er,data){var ts=stream._transformState;ts.transforming=false;var cb=ts.writecb;if(!cb)return stream.emit("error",new Error("no writecb in Transform class"));ts.writechunk=null;ts.writecb=null;if(data!==null&&data!==undefined)stream.push(data);if(cb)cb(er);var rs=stream._readableState;rs.reading=false;if(rs.needReadable||rs.length<rs.highWaterMark){stream._read(rs.highWaterMark)}}function Transform(options){if(!(this instanceof Transform))return new Transform(options);Duplex.call(this,options);var ts=this._transformState=new TransformState(options,this);var stream=this;this._readableState.needReadable=true;this._readableState.sync=false;this.once("finish",function(){if("function"===typeof this._flush)this._flush(function(er){done(stream,er)});else done(stream)})}Transform.prototype.push=function(chunk,encoding){this._transformState.needTransform=false;return Duplex.prototype.push.call(this,chunk,encoding)};Transform.prototype._transform=function(chunk,encoding,cb){throw new Error("not implemented")};Transform.prototype._write=function(chunk,encoding,cb){var ts=this._transformState;ts.writecb=cb;ts.writechunk=chunk;ts.writeencoding=encoding;if(!ts.transforming){var rs=this._readableState;if(ts.needTransform||rs.needReadable||rs.length<rs.highWaterMark)this._read(rs.highWaterMark)}};Transform.prototype._read=function(n){var ts=this._transformState;if(ts.writechunk!==null&&ts.writecb&&!ts.transforming){ts.transforming=true;this._transform(ts.writechunk,ts.writeencoding,ts.afterTransform)}else{ts.needTransform=true}};function done(stream,er){if(er)return stream.emit("error",er);var ws=stream._writableState;var rs=stream._readableState;var ts=stream._transformState;if(ws.length)throw new Error("calling transform done when ws.length != 0");if(ts.transforming)throw new Error("calling transform done when still transforming");return stream.push(null)}},{"./_stream_duplex":10,"core-util-is":15,inherits:6}],14:[function(require,module,exports){(function(process){module.exports=Writable;var Buffer=require("buffer").Buffer;Writable.WritableState=WritableState;var util=require("core-util-is");util.inherits=require("inherits");var Stream=require("stream");util.inherits(Writable,Stream);function WriteReq(chunk,encoding,cb){this.chunk=chunk;this.encoding=encoding;this.callback=cb}function WritableState(options,stream){options=options||{};var hwm=options.highWaterMark;this.highWaterMark=hwm||hwm===0?hwm:16*1024;this.objectMode=!!options.objectMode;this.highWaterMark=~~this.highWaterMark;this.needDrain=false;this.ending=false;this.ended=false;this.finished=false;var noDecode=options.decodeStrings===false;this.decodeStrings=!noDecode;this.defaultEncoding=options.defaultEncoding||"utf8";this.length=0;this.writing=false;this.sync=true;this.bufferProcessing=false;this.onwrite=function(er){onwrite(stream,er)};this.writecb=null;this.writelen=0;this.buffer=[];this.errorEmitted=false}function Writable(options){var Duplex=require("./_stream_duplex");if(!(this instanceof Writable)&&!(this instanceof Duplex))return new Writable(options);this._writableState=new WritableState(options,this);this.writable=true;Stream.call(this)}Writable.prototype.pipe=function(){this.emit("error",new Error("Cannot pipe. Not readable."))};function writeAfterEnd(stream,state,cb){var er=new Error("write after end");stream.emit("error",er);process.nextTick(function(){cb(er)})}function validChunk(stream,state,chunk,cb){var valid=true;if(!Buffer.isBuffer(chunk)&&"string"!==typeof chunk&&chunk!==null&&chunk!==undefined&&!state.objectMode){var er=new TypeError("Invalid non-string/buffer chunk");stream.emit("error",er);process.nextTick(function(){cb(er)});valid=false}return valid}Writable.prototype.write=function(chunk,encoding,cb){var state=this._writableState;var ret=false;if(typeof encoding==="function"){cb=encoding;encoding=null}if(Buffer.isBuffer(chunk))encoding="buffer";else if(!encoding)encoding=state.defaultEncoding;if(typeof cb!=="function")cb=function(){};if(state.ended)writeAfterEnd(this,state,cb);else if(validChunk(this,state,chunk,cb))ret=writeOrBuffer(this,state,chunk,encoding,cb);return ret};function decodeChunk(state,chunk,encoding){if(!state.objectMode&&state.decodeStrings!==false&&typeof chunk==="string"){chunk=new Buffer(chunk,encoding)}return chunk}function writeOrBuffer(stream,state,chunk,encoding,cb){chunk=decodeChunk(state,chunk,encoding);if(Buffer.isBuffer(chunk))encoding="buffer";var len=state.objectMode?1:chunk.length;state.length+=len;var ret=state.length<state.highWaterMark;if(!ret)state.needDrain=true;if(state.writing)state.buffer.push(new WriteReq(chunk,encoding,cb));else doWrite(stream,state,len,chunk,encoding,cb);return ret}function doWrite(stream,state,len,chunk,encoding,cb){state.writelen=len;state.writecb=cb;state.writing=true;state.sync=true;stream._write(chunk,encoding,state.onwrite);state.sync=false}function onwriteError(stream,state,sync,er,cb){if(sync)process.nextTick(function(){cb(er)});else cb(er);stream._writableState.errorEmitted=true;stream.emit("error",er)}function onwriteStateUpdate(state){state.writing=false;state.writecb=null;state.length-=state.writelen;state.writelen=0}function onwrite(stream,er){var state=stream._writableState;var sync=state.sync;var cb=state.writecb;onwriteStateUpdate(state);if(er)onwriteError(stream,state,sync,er,cb);else{var finished=needFinish(stream,state);if(!finished&&!state.bufferProcessing&&state.buffer.length)clearBuffer(stream,state);if(sync){process.nextTick(function(){afterWrite(stream,state,finished,cb)})}else{afterWrite(stream,state,finished,cb)}}}function afterWrite(stream,state,finished,cb){if(!finished)onwriteDrain(stream,state);cb();if(finished)finishMaybe(stream,state)}function onwriteDrain(stream,state){if(state.length===0&&state.needDrain){state.needDrain=false;stream.emit("drain")}}function clearBuffer(stream,state){state.bufferProcessing=true;for(var c=0;c<state.buffer.length;c++){var entry=state.buffer[c];var chunk=entry.chunk;var encoding=entry.encoding;var cb=entry.callback;var len=state.objectMode?1:chunk.length;doWrite(stream,state,len,chunk,encoding,cb);if(state.writing){c++;break}}state.bufferProcessing=false;if(c<state.buffer.length)state.buffer=state.buffer.slice(c);else state.buffer.length=0}Writable.prototype._write=function(chunk,encoding,cb){cb(new Error("not implemented"))};Writable.prototype.end=function(chunk,encoding,cb){var state=this._writableState;if(typeof chunk==="function"){cb=chunk;chunk=null;encoding=null}else if(typeof encoding==="function"){cb=encoding;encoding=null}if(typeof chunk!=="undefined"&&chunk!==null)this.write(chunk,encoding);if(!state.ending&&!state.finished)endWritable(this,state,cb)};function needFinish(stream,state){return state.ending&&state.length===0&&!state.finished&&!state.writing}function finishMaybe(stream,state){var need=needFinish(stream,state);if(need){state.finished=true;stream.emit("finish")}return need}function endWritable(stream,state,cb){state.ending=true;finishMaybe(stream,state);if(cb){if(state.finished)process.nextTick(cb);else stream.once("finish",cb)}state.ended=true}}).call(this,require("_process"))},{"./_stream_duplex":10,_process:8,buffer:1,"core-util-is":15,inherits:6,stream:20}],15:[function(require,module,exports){(function(Buffer){function isArray(ar){return Array.isArray(ar)}exports.isArray=isArray;function isBoolean(arg){return typeof arg==="boolean"}exports.isBoolean=isBoolean;function isNull(arg){return arg===null}exports.isNull=isNull;function isNullOrUndefined(arg){return arg==null}exports.isNullOrUndefined=isNullOrUndefined;function isNumber(arg){return typeof arg==="number"}exports.isNumber=isNumber;function isString(arg){return typeof arg==="string"}exports.isString=isString;function isSymbol(arg){return typeof arg==="symbol"}exports.isSymbol=isSymbol;function isUndefined(arg){return arg===void 0}exports.isUndefined=isUndefined;function isRegExp(re){return isObject(re)&&objectToString(re)==="[object RegExp]"}exports.isRegExp=isRegExp;function isObject(arg){return typeof arg==="object"&&arg!==null}exports.isObject=isObject;function isDate(d){return isObject(d)&&objectToString(d)==="[object Date]"}exports.isDate=isDate;function isError(e){return isObject(e)&&(objectToString(e)==="[object Error]"||e instanceof Error)}exports.isError=isError;function isFunction(arg){return typeof arg==="function"}exports.isFunction=isFunction;function isPrimitive(arg){return arg===null||typeof arg==="boolean"||typeof arg==="number"||typeof arg==="string"||typeof arg==="symbol"||typeof arg==="undefined"}exports.isPrimitive=isPrimitive;function isBuffer(arg){return Buffer.isBuffer(arg)}exports.isBuffer=isBuffer;function objectToString(o){return Object.prototype.toString.call(o)}}).call(this,require("buffer").Buffer)},{buffer:1}],16:[function(require,module,exports){module.exports=require("./lib/_stream_passthrough.js")},{"./lib/_stream_passthrough.js":11}],17:[function(require,module,exports){var Stream=require("stream");exports=module.exports=require("./lib/_stream_readable.js");exports.Stream=Stream;exports.Readable=exports;exports.Writable=require("./lib/_stream_writable.js");exports.Duplex=require("./lib/_stream_duplex.js");exports.Transform=require("./lib/_stream_transform.js");exports.PassThrough=require("./lib/_stream_passthrough.js")},{"./lib/_stream_duplex.js":10,"./lib/_stream_passthrough.js":11,"./lib/_stream_readable.js":12,"./lib/_stream_transform.js":13,"./lib/_stream_writable.js":14,stream:20}],18:[function(require,module,exports){module.exports=require("./lib/_stream_transform.js")},{"./lib/_stream_transform.js":13}],19:[function(require,module,exports){module.exports=require("./lib/_stream_writable.js")},{"./lib/_stream_writable.js":14}],20:[function(require,module,exports){module.exports=Stream;var EE=require("events").EventEmitter;var inherits=require("inherits");inherits(Stream,EE);Stream.Readable=require("readable-stream/readable.js");Stream.Writable=require("readable-stream/writable.js");Stream.Duplex=require("readable-stream/duplex.js");Stream.Transform=require("readable-stream/transform.js");Stream.PassThrough=require("readable-stream/passthrough.js");Stream.Stream=Stream;function Stream(){EE.call(this)}Stream.prototype.pipe=function(dest,options){var source=this;function ondata(chunk){if(dest.writable){if(false===dest.write(chunk)&&source.pause){source.pause()}}}source.on("data",ondata);function ondrain(){if(source.readable&&source.resume){source.resume()}}dest.on("drain",ondrain);if(!dest._isStdio&&(!options||options.end!==false)){source.on("end",onend);source.on("close",onclose)}var didOnEnd=false;function onend(){if(didOnEnd)return;didOnEnd=true;dest.end()}function onclose(){if(didOnEnd)return;didOnEnd=true;if(typeof dest.destroy==="function")dest.destroy()}function onerror(er){cleanup();if(EE.listenerCount(this,"error")===0){throw er}}source.on("error",onerror);dest.on("error",onerror);function cleanup(){source.removeListener("data",ondata);dest.removeListener("drain",ondrain);source.removeListener("end",onend);source.removeListener("close",onclose);source.removeListener("error",onerror);dest.removeListener("error",onerror);source.removeListener("end",cleanup);source.removeListener("close",cleanup);dest.removeListener("close",cleanup)}source.on("end",cleanup);source.on("close",cleanup);dest.on("close",cleanup);dest.emit("pipe",source);return dest}},{events:5,inherits:6,"readable-stream/duplex.js":9,"readable-stream/passthrough.js":16,"readable-stream/readable.js":17,"readable-stream/transform.js":18,"readable-stream/writable.js":19}],21:[function(require,module,exports){var Buffer=require("buffer").Buffer;var isBufferEncoding=Buffer.isEncoding||function(encoding){switch(encoding&&encoding.toLowerCase()){case"hex":case"utf8":case"utf-8":case"ascii":case"binary":case"base64":case"ucs2":case"ucs-2":case"utf16le":case"utf-16le":case"raw":return true;default:return false}};function assertEncoding(encoding){if(encoding&&!isBufferEncoding(encoding)){throw new Error("Unknown encoding: "+encoding)}}var StringDecoder=exports.StringDecoder=function(encoding){this.encoding=(encoding||"utf8").toLowerCase().replace(/[-_]/,"");assertEncoding(encoding);switch(this.encoding){case"utf8":this.surrogateSize=3;break;case"ucs2":case"utf16le":this.surrogateSize=2;this.detectIncompleteChar=utf16DetectIncompleteChar;break;case"base64":this.surrogateSize=3;this.detectIncompleteChar=base64DetectIncompleteChar;break;default:this.write=passThroughWrite;return}this.charBuffer=new Buffer(6);this.charReceived=0;this.charLength=0};StringDecoder.prototype.write=function(buffer){var charStr="";while(this.charLength){var available=buffer.length>=this.charLength-this.charReceived?this.charLength-this.charReceived:buffer.length;buffer.copy(this.charBuffer,this.charReceived,0,available);this.charReceived+=available;if(this.charReceived<this.charLength){return""}buffer=buffer.slice(available,buffer.length);charStr=this.charBuffer.slice(0,this.charLength).toString(this.encoding);var charCode=charStr.charCodeAt(charStr.length-1);if(charCode>=55296&&charCode<=56319){this.charLength+=this.surrogateSize;charStr="";continue}this.charReceived=this.charLength=0;if(buffer.length===0){return charStr}break}this.detectIncompleteChar(buffer);var end=buffer.length;if(this.charLength){buffer.copy(this.charBuffer,0,buffer.length-this.charReceived,end);end-=this.charReceived}charStr+=buffer.toString(this.encoding,0,end);var end=charStr.length-1;var charCode=charStr.charCodeAt(end);if(charCode>=55296&&charCode<=56319){var size=this.surrogateSize;this.charLength+=size;this.charReceived+=size;this.charBuffer.copy(this.charBuffer,size,0,size);buffer.copy(this.charBuffer,0,0,size);return charStr.substring(0,end)}return charStr};StringDecoder.prototype.detectIncompleteChar=function(buffer){var i=buffer.length>=3?3:buffer.length;for(;i>0;i--){var c=buffer[buffer.length-i];if(i==1&&c>>5==6){this.charLength=2;break}if(i<=2&&c>>4==14){this.charLength=3;break}if(i<=3&&c>>3==30){this.charLength=4;break}}this.charReceived=i};StringDecoder.prototype.end=function(buffer){var res="";if(buffer&&buffer.length)res=this.write(buffer);if(this.charReceived){var cr=this.charReceived;var buf=this.charBuffer;var enc=this.encoding;res+=buf.slice(0,cr).toString(enc)}return res};function passThroughWrite(buffer){return buffer.toString(this.encoding)}function utf16DetectIncompleteChar(buffer){this.charReceived=buffer.length%2;this.charLength=this.charReceived?2:0}function base64DetectIncompleteChar(buffer){this.charReceived=buffer.length%3;this.charLength=this.charReceived?3:0}},{buffer:1}],22:[function(require,module,exports){module.exports=function(){for(var i=0;i<arguments.length;i++){if(arguments[i]!==undefined)return arguments[i]}}},{}],"level-tracker":[function(require,module,exports){var readable=require("stream").Readable;var writable=require("stream").Writable;var defined=require("defined");module.exports=tracker;function tracker(db,logapi){if(db.trackable){return}logapi=logapi||function(){};db["trackable"]=trackable;return db;function trackable(){var pattern=[];db.on("put",put);db.on("del",del);db.on("batch",batch);function put(key,val){each({type:"put",key:key,value:val})}function del(key,val){each({type:"del",key:key,value:val})}function batch(arr){arr.forEach(each)}function each(item){item&&item.type&&pattern.forEach(function(check){if(check(String(item.key))){trackerstream.push(item)}})}var trackerstream=readable({objectMode:true});trackerstream._read=function(){};trackerstream.on("end",untrackable);trackerstream.on("close",untrackable);function untrackable(){db.removeListener("put",put);db.removeListener("del",del);db.removeListener("batch",batch)}trackerstream["track"]=function track(opts){opts=defined(opts,{});var old=defined(opts.old,false);var gte=defined(opts.gte,"");var suffix=defined(opts.suffix,"");var lte=defined(opts.lte,"");var terminator=defined(opts.terminator,"");opts.gte=gte=gte+suffix;opts.lte=lte=(lte?lte:gte)+suffix+terminator;function check(key){return(!gte||gte<=""+key)&&(!lte||""+key<=lte)}logapi(opts);if(old){var reader=db.createReadStream(opts);var writer=writable({objectMode:true});writer._write=function(chunk,encoding,next){chunk.type="put";trackerstream.push(chunk);next()};writer.on("end",function loaded(){trackerstream.emit("loaded");writer.emit("finish");reader.unpipe(writer)});reader.pipe(writer)}pattern.push(check);return function untrack(){var i=pattern.indexOf(check);if(!~i)return false; | |
pattern.splice(i,1);return true}};return trackerstream}}},{defined:22,stream:20}]},{},[]);function Hook(db,component,tracks,TEMP){this.db=db;this.component=component;this.tracks=tracks;this.TEMP=TEMP;this.untrack=[]}Hook.prototype.unhook=function(){logger(null,":::: unhook() -> untrack()");this.untrack.forEach(function(untrack){untrack()})};Hook.prototype.hook=function(node){var THIS=this;var DATA=THIS.TEMP;var initialize=true;logger(null,":::: hook() -> track()");var t=THIS.db.trackable();THIS.tracks.forEach(function(keyrange){THIS.untrack.push(t.track(keyrange))});t.on("data",function ondata(data){var key=data.key;var val=data.value;if(DATA[key]!==val){logger(null,':::: .on("data") -> update()');logger(null,"<b>OLD:</b> "+JSON.stringify(data));logger(null,"<b>PATCH WITH:</b> {"+key+': "'+val+'" }');DATA[key]=val;logger(null,"<b>NEW:</b> "+JSON.stringify(data));var oldTree=THIS.vtree;var newTree=THIS.component(DATA);var patches=diff(oldTree,newTree);patch(node,patches)}else if(initialize){logger(null,":::: initialize()");logger(null,"<b>DATA:</b> "+JSON.stringify(DATA));var oldTree=THIS.vtree;var newTree=THIS.component(DATA);var patches=diff(oldTree,newTree);patch(node,patches);initialize=false}})};function MyThunk(params){if(!(this instanceof MyThunk)){return new MyThunk(params)}var db=params.db;var render=params.render;var tracks=params.tracks;if(!db||!render||!tracks){throw new Error("missing params")}var TEMP={};var hook=new Hook(db,init,Object.keys(tracks).map(function(key){db.get(key,function(error,data){if(error){db.put(key,tracks[key],function handle(error){if(error){throw error}});TEMP[key]=tracks[key]}else{TEMP[key]=data}});return{gte:key,lte:key,suffix:"",terminator:"!",old:true}}),TEMP);function init(DATA){return h("div",{"ev-hook":hook},render(DATA))}this.init=init;this.hook=hook}MyThunk.prototype.type="Thunk";MyThunk.prototype.render=function render(Pre){var useCache=Pre&&Pre.vnode;if(useCache){logger(null,":::: render cached thunk")}return useCache?Pre.vnode:this.hook.vtree=this.init({})};function apilogger(opts){console.log("API LOGGING: ",opts)}var diff=require("virtual-dom").diff;var patch=require("virtual-dom").patch;var createElement=require("virtual-dom").create;var h=require("virtual-dom").h;var process=require("process");var stream=require("stream-browserify");var level=require("memdb");var tracker=require("level-tracker");var db=tracker(level("data.db"),apilogger);var myComponent=MyThunk;var TEMP={name:"",subname:"",info:""};document.querySelector("#content").appendChild(createElement(h("div",[h("h1","CONTROLS - Change Component Data"),h("input",{className:"name",onchange:store}),h("button",{className:"name",onclick:update},"name"),h("br"),h("input",{className:"subname",onchange:store}),h("button",{className:"subname",onclick:update},"subname"),h("br"),h("input",{className:"info",onchange:store}),h("button",{className:"info",onclick:update},"info"),h("br"),h("hr"),h("h2","COMPONENT - See rendered demo component"),h("div",{style:{border:"3px solid red",padding:"20px"}},[myComponent({db:db,tracks:{name:"John Doe",subname:"alleycat",info:"The random dude"},render:function render(DATA){return h("div",[h("h1","Name: "+DATA["name"]),h("h2","Subname: "+DATA["subname"]),h("h3","Info: "+DATA["info"])])}})]),h("hr"),h("h2","LOGGING - logging data changes + dump database"),h("button",{onclick:dump},"dump memdb 2 console"),h("button",{onclick:clear},"clear console")])));function update(ev){var key=ev.target.className;db.put(key,TEMP[key],function handle(error){if(error){throw error}})}function store(ev){var e=ev.target;TEMP[e.className]=e.value}var konsole;function logger(err,data){if(!konsole){konsole=getKonsole()}var x=document.createElement("div");x.className="line";x.style.fontFamily="Arial";x.style.paddingTop="10px";x.innerHTML=data;konsole.appendChild(x)}function dump(){logger(null,":::: Dumping memdb content");db.get("name",logger);db.get("subname",logger);db.get("info",logger)}function clear(){var lines=[].slice.call(document.querySelectorAll(".line"));lines.forEach(function(line){line.parentNode.removeChild(line)})}function getKonsole(){var style=document.createElement("style");style.innerHTML=[".konsole{","background-color: black;","padding: 5px;","box-sizing: border-box;","color: white;","position: absolute;","width:100%;","height:30vh;","display: flex;","overflow: scroll;","flex-direction:column;","}"].join("");document.body.appendChild(style);var konsole=document.createElement("div");konsole.className="konsole";document.body.appendChild(konsole);return konsole} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
{ | |
"name": "virtual-level-tracker Example", | |
"version": "1.0.0", | |
"dependencies": { | |
"virtual-dom": "2.1.1", | |
"process": "0.11.2", | |
"stream-browserify": "2.0.1", | |
"memdb": "1.3.1", | |
"level-tracker": "1.0.0" | |
} | |
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
<!-- contents of this file will be placed inside the <body> --> | |
<div id='content'></div> |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
<!-- contents of this file will be placed inside the <head> --> |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment