Last active
September 16, 2022 04:27
-
-
Save shaps80/11ea37c6a3f65f0ebe997e40d6aabcf9 to your computer and use it in GitHub Desktop.
A simple protocol for creating a 'Card' style component in Swift.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
import UIKit | |
/// Decorates a view as a `Card`. Requires that the view is embedded in some `contentView` | |
public protocol CardDecorating { | |
/// View that will be styled as a card. Should hold the `contentView`. | |
var cardView: UIView { get } | |
/// Holds the main content. Subviews should be added to this view. | |
var contentView: UIView { get } | |
} | |
/// Defines the various styling attributes to apply to the `Card` | |
public struct CardStyle { | |
public var backgroundColor: UIColor | |
public var cornerRadius: CGFloat | |
public var shadowColor: UIColor | |
public var shadowOpacity: Float | |
public var shadowOffset: UIOffset | |
public var shadowRadius: CGFloat | |
} | |
public extension CardDecorating { | |
/// Applies the specified `style` to the `Card` view and its `contentView` | |
func applyCardDecoration(style: CardStyle) { | |
cardView.backgroundColor = style.backgroundColor | |
cardView.layer.masksToBounds = false | |
cardView.layer.cornerCurve = .continuous | |
cardView.layer.cornerRadius = style.cornerRadius | |
cardView.layer.shadowColor = style.shadowColor.cgColor | |
cardView.layer.shadowOffset = CGSize(width: style.shadowOffset.horizontal, height: style.shadowOffset.vertical) | |
cardView.layer.shadowRadius = style.shadowRadius | |
cardView.layer.shadowOpacity = style.shadowOpacity | |
cardView.layer.rasterizationScale = UIScreen.main.scale | |
cardView.layer.shouldRasterize = true | |
contentView.layer.cornerCurve = .continuous | |
contentView.layer.cornerRadius = cardView.layer.cornerRadius | |
} | |
} | |
/// Convenience type to simplify the usage of the `CardDecorating` protocol | |
struct CardBuilder: CardDecorating { | |
let cardView: UIView | |
let contentView: UIView | |
} | |
extension UITableViewCell: CardDecorating { | |
public var cardView: UIView { return self } | |
} | |
extension UICollectionViewCell: CardDecorating { | |
public var cardView: UIView { return self } | |
} |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Example:
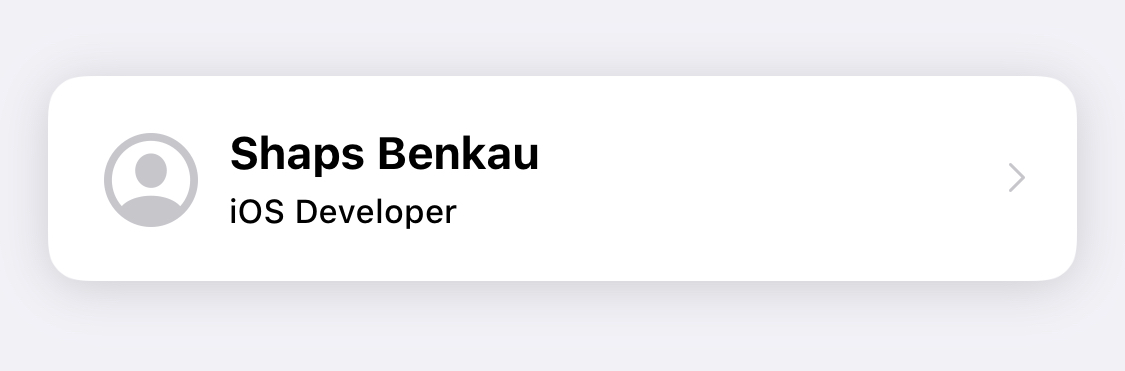