Last active
July 12, 2022 14:31
-
-
Save sshymko/aa1eb3870ca3cc9e64efd83a43a1f06a to your computer and use it in GitHub Desktop.
Invalidate CloudFront distribution from CodePipeline via Lambda function
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
const aws = require('aws-sdk'); | |
const codepipeline = new aws.CodePipeline(); | |
const cloudfront = new aws.CloudFront(); | |
exports.handler = async (event, context) => { | |
let job = event['CodePipeline.job']; | |
let config = job.data.actionConfiguration.configuration.UserParameters; | |
try { | |
let params = JSON.parse(config); | |
if (!params || !params.distributionId) { | |
throw new Error('Missing CloudFront distributionId.'); | |
} | |
let paths = (params.paths && params.paths.length) | |
? params.paths | |
: ['/*']; | |
await cloudfront.createInvalidation({ | |
DistributionId: params.distributionId, | |
InvalidationBatch: { | |
CallerReference: String(Date.now()), | |
Paths: { | |
Quantity: paths.length, | |
Items: paths | |
} | |
} | |
}).promise(); | |
return codepipeline.putJobSuccessResult({ | |
jobId: job.id | |
}).promise(); | |
} catch (error) { | |
return codepipeline.putJobFailureResult({ | |
jobId: job.id, | |
failureDetails: { | |
type: 'JobFailed', | |
message: error.message, | |
externalExecutionId: context.awsRequestId | |
} | |
}).promise(); | |
} | |
}; |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Usage
Invalidate Distribution
Configure CloudFront distribution ID in JSON format in CodePipeline Action:
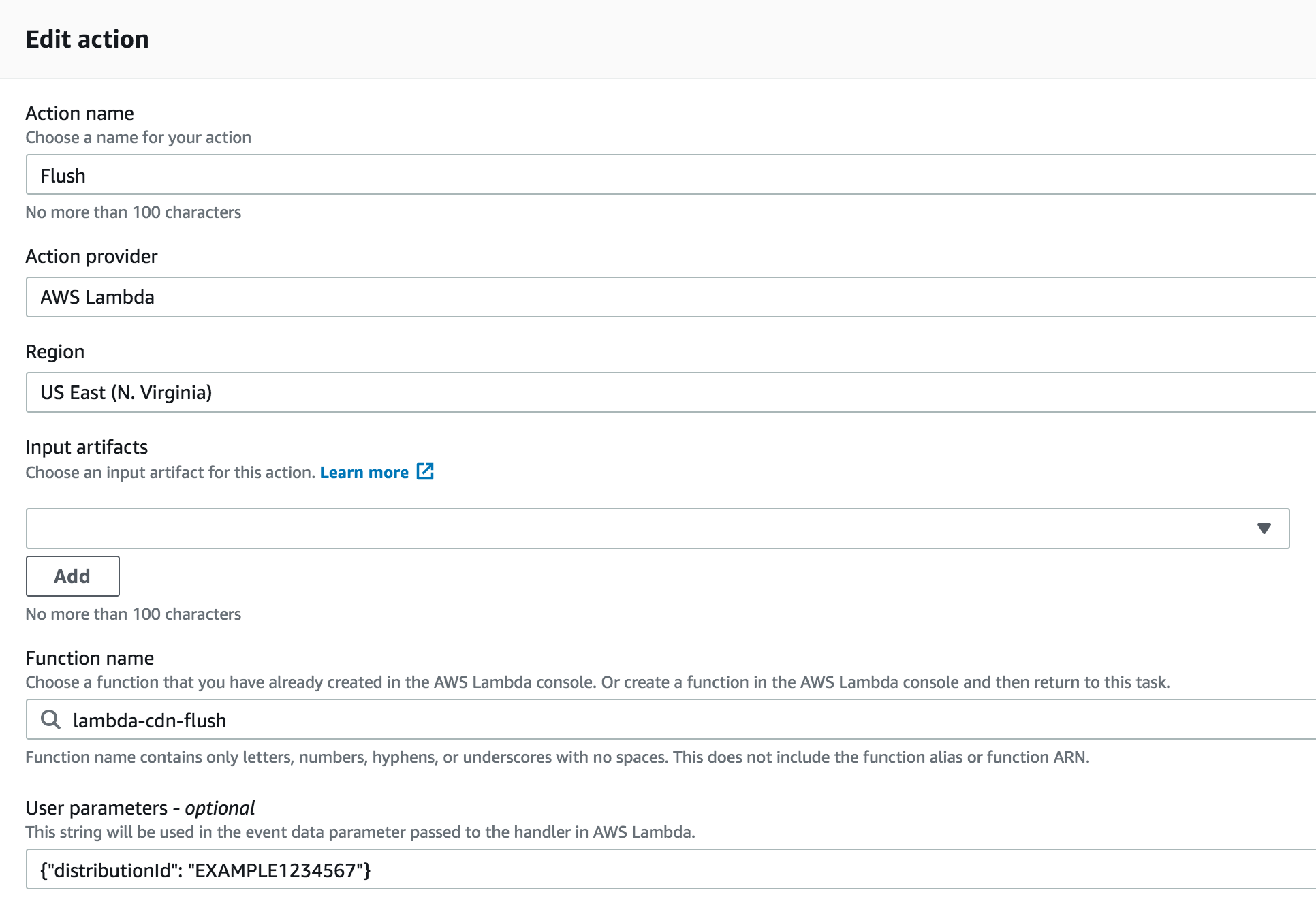
Invalidate Paths
Specify invalidation path(s):
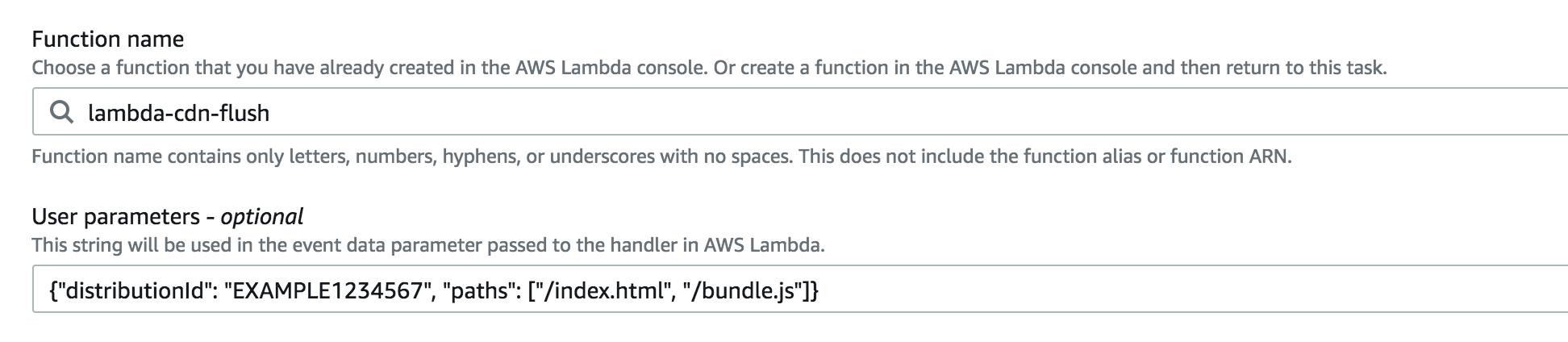
Permissions
Lambda function needs to have an IAM Role with the following Policy: