Last active
November 23, 2020 16:08
-
-
Save tbaggaley/fb4d30c5fa26e0ff3e5aba98cf09111f to your computer and use it in GitHub Desktop.
Import a survey bookmarklet
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
// Import a survey bookmarklet | |
// 1. Create a new bookmark (e.g. with title "Import survey") with the following as the URL: | |
// javascript:(function()%7Bdocument.write(%60%0A%3Ch1%3ESurvey%20import%20tool%3C%2Fh1%3E%0A%3Ch3%3E%201.%20Enter%20URL%20to%20the%20questionnaire%20you'd%20like%20to%20import%3A%20%3C%2Fh3%3E%0A%3Cinput%20type%3D%22text%22%20id%3D%22url%22%20style%3D%22width%3A%2033%25%22%3E%0A%3Ch3%3E%202.%20Open%20the%20following%20URL%20and%20copy%20the%20contents%20to%20your%20clipboard%3A%20%3C%2Fh3%3E%0A%3Cspan%20id%3D%22exportURL%22%3E%20Enter%20a%20questionnaire%20URL%20above%20to%20see%20the%20export%20URL%20%3C%2Fspan%3E%0A%3Ch3%3E%203.%20Paste%20the%20contents%20in%20the%20box%20below%20%3C%2Fh3%3E%0A%3Ctextarea%20id%3D'schema'%20style%3D'width%3A50%25%3B%20height%3A33%25'%3E%3C%2Ftextarea%3E%0A%3Ch3%3E%204.%20Hit%20the%20button%20to%20import%20the%20survey%20and%20open%20it%20for%20viewing%20%3C%2Fh3%3E%0A%3Cbutton%20id%3D%22importButton%22%3EImport%20survey%3C%2Fbutton%3E%0A%60)%3B%0A%0Adocument.body.style%20%3D%20%22text-align%3A%20center%3B%20font-family%3A%20sans-serif%3B%22%3B%0A%0Adocument.getElementById(%22url%22).oninput%20%3D%20e%20%3D%3E%20%7B%0A%20%20%20%20const%20exportURLelem%20%3D%20document.getElementById(%22exportURL%22)%3B%0A%20%20%20%20const%20matches%20%3D%20e.target.value.match(%2F%5E(.%2B)%5C%2F.%2Bq%5C%2F(.%2B%3F)%5C%2F%2F)%3B%0A%20%20%20%20if(matches.length%20%3C%203)%0A%20%20%20%20%20%20%20%20exportURLelem.innerText%20%3D%20%22Enter%20a%20questionnaire%20URL%20above%20to%20see%20the%20export%20URL%22%3B%0A%20%20%20%20else%0A%20%20%20%20%20%20%20%20exportURLelem.innerHTML%20%3D%20%60%3Ca%20href%3D%22%24%7Bmatches%5B1%5D%7D%2Fexport%2F%24%7Bmatches%5B2%5D%7D%22%20target%3D%22_blank%22%3E%20Click%20here%20to%20view%20Author%20schema%20JSON%20%3C%2Fa%3E%60%3B%0A%7D%3B%0A%0Adocument.getElementById(%22importButton%22).onclick%20%3D%20async%20()%20%3D%3E%20%7B%0A%20%20%20%20const%20schema%20%3D%20document.getElementById(%22schema%22).value.trim()%3B%0A%20%20%20%20if(!schema)%20return%3B%0A%0A%20%20%20%20const%20host%20%3D%20window.location.host%3B%0A%20%20%20%20const%20addr%20%3D%20(host.startsWith(%22localhost%22)%20%3F%20%22http%3A%2F%2Flocalhost%3A4000%22%20%3A%20%60https%3A%2F%2F%24%7Bhost%7D%60)%20%2B%20%22%2Fimport%22%3B%0A%0A%20%20%20%20const%20result%20%3D%20await%20fetch(addr%2C%20%7B%0A%20%20%20%20%20%20%20%20method%3A%20%22POST%22%2C%0A%20%20%20%20%20%20%20%20headers%3A%20%7B%0A%20%20%20%20%20%20%20%20%20%20%20%20%22Content-Type%22%3A%20%22application%2Fjson%22%2C%0A%20%20%20%20%20%20%20%20%20%20%20%20%22Authorization%22%3A%20%22Bearer%20%22%20%2B%20localStorage.accessToken%0A%20%20%20%20%20%20%20%20%7D%2C%0A%20%20%20%20%20%20%20%20body%3A%20document.getElementById(%22schema%22).value.trim()%2C%0A%20%20%20%20%7D)%3B%0A%0A%20%20%20%20const%20json%20%3D%20await%20result.json()%3B%0A%0A%20%20%20%20if(json.id)%0A%20%20%20%20%20%20%20%20window.location%20%3D%20%60%2Fgo%23%2Fq%2F%24%7Bjson.id%7D%60%3B%0A%20%20%20%20else%0A%20%20%20%20%20%20%20%20alert(%22Unable%20to%20add%20survey%20-%20check%20the%20JSON%20is%20valid%22)%3B%0A%7D%3B%0A%7D)() | |
// 2. Visit staging or locally hosted Author (works for either) and make sure you're logged in | |
// 3. Open the bookmark (could make it into button on bookmark bar - but will work from bookmarks menu too) | |
// It will run through the survey import process, import the survey & visit it in the browser | |
// No more need to copy auth credentials or use postman! | |
// The code which the above was generated from is below: | |
document.write(` | |
<h1>Survey import tool</h1> | |
<h3> 1. Enter URL to the questionnaire you'd like to import: </h3> | |
<input type="text" id="url" style="width: 33%"> | |
<h3> 2. Open the following URL and copy the contents to your clipboard: </h3> | |
<span id="exportURL"> Enter a questionnaire URL above to see the export URL </span> | |
<h3> 3. Paste the contents in the box below </h3> | |
<textarea id='schema' style='width:50%; height:33%'></textarea> | |
<h3> 4. Hit the button to import the survey and open it for viewing </h3> | |
<button id="importButton">Import survey</button> | |
`); | |
document.body.style = "text-align: center; font-family: sans-serif;"; | |
document.getElementById("url").oninput = e => { | |
const exportURLelem = document.getElementById("exportURL"); | |
const matches = e.target.value.match(/^(.+)\/.+q\/(.+?)\//); | |
if(matches.length < 3) | |
exportURLelem.innerText = "Enter a questionnaire URL above to see the export URL"; | |
else | |
exportURLelem.innerHTML = `<a href="${matches[1]}/export/${matches[2]}" target="_blank"> Click here to view Author schema JSON </a>`; | |
}; | |
document.getElementById("importButton").onclick = async () => { | |
const schema = document.getElementById("schema").value.trim(); | |
if(!schema) return; | |
const host = window.location.host; | |
const addr = (host.startsWith("localhost") ? "http://localhost:4000" : `https://${host}`) + "/import"; | |
const result = await fetch(addr, { | |
method: "POST", | |
headers: { | |
"Content-Type": "application/json", | |
"Authorization": "Bearer " + localStorage.accessToken | |
}, | |
body: document.getElementById("schema").value.trim(), | |
}); | |
const json = await result.json(); | |
if(json.id) | |
window.location = `/go#/q/${json.id}`; | |
else | |
alert("Unable to add survey - check the JSON is valid"); | |
}; |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Once the bookmarklet is activated the UI will appear as shown here:
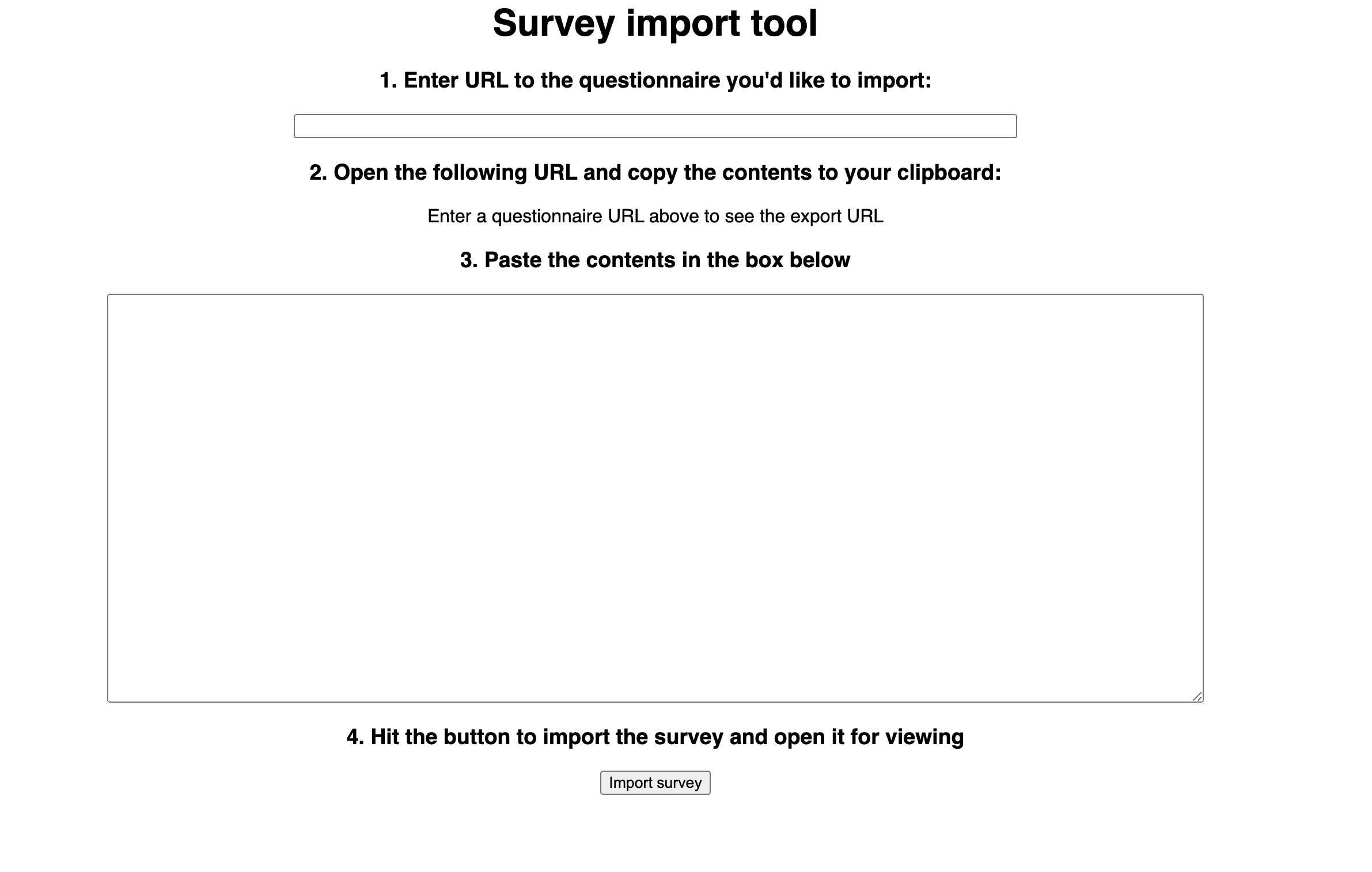