Here is how to add Cypress E2E tests to a Create React App bootstrapped application. Assumes the *nix command line, you may need to adapt this for a Windows command line (or use WSL or Git Bash).
-
Install Cypress and the Testing Library utilities for it (to match the helpers CRA installs):
$ npm i {,@testing-library/}cypress
i
is short for install, and the braces{}
are expanded by brace expansion tocypress @testing-library/cypress
.CRA seems to install everything as a production dependency, but you can add
--save-dev
if you like. -
Open the Cypress UI to bootstrap the test setup:
$ npx cypress open --e2e
NPX will use the executable in the
node_modules/.bin/
directory, so you can use it for things you don't want to add to the package file. -
Delete the example files:
$ rm -rf ./cypress/fixtures/example.json
-
Set a base URL in the
cypress.config.js
per the best practices (I've also disabled automatic video recording):const { defineConfig } = require("cypress"); module.exports = defineConfig({ e2e: { baseUrl: "http://localhost:3000", setupNodeEvents(on, config) { // implement node event listeners here }, }, video: false, });
-
Set up the Testing Library utilities by adding the following to
./cypress/support/commands.js
:import "@testing-library/cypress/add-commands";
-
Make sure you exclude any videos and screenshots recorded by Cypress from your commits by adding the following to
.gitignore
:# Cypress cypress/screenshots/ cypress/videos/
-
Add a script that runs the E2E tests into the package file:
"scripts": "e2e": "cypress run", "start": "react-scripts start", "build": "react-scripts build", "test": "react-scripts test", "eject": "react-scripts eject" },
-
Create a new test file e.g.
./cypress/e2e/home.cy.js
with the following:it("should load the page", () => { cy.visit("/"); cy.findAllByText(/learn react/i).should("have.length", 1); });
This is the same as the default
src/App.test.js
, but running via Cypress rather than Jest. -
Start the app in one terminal (
npm start
) then run the tests in another (npm run e2e
). It should pass! -
Install the ESLint plugin with
npm install eslint-plugin-cypress
then add the following to theeslintConfig
object inpackage.json
:"overrides": [ { "extends": [ "plugin:cypress/recommended" ], "files": [ "cypress/**/*.js" ] } ]
This is optional, but will ensure the linting passes (by adding the Cypress globals) and give some useful hints on good test practices.
@textbook I have followed all the steps above but I could not get my e2e tests running for my react app.
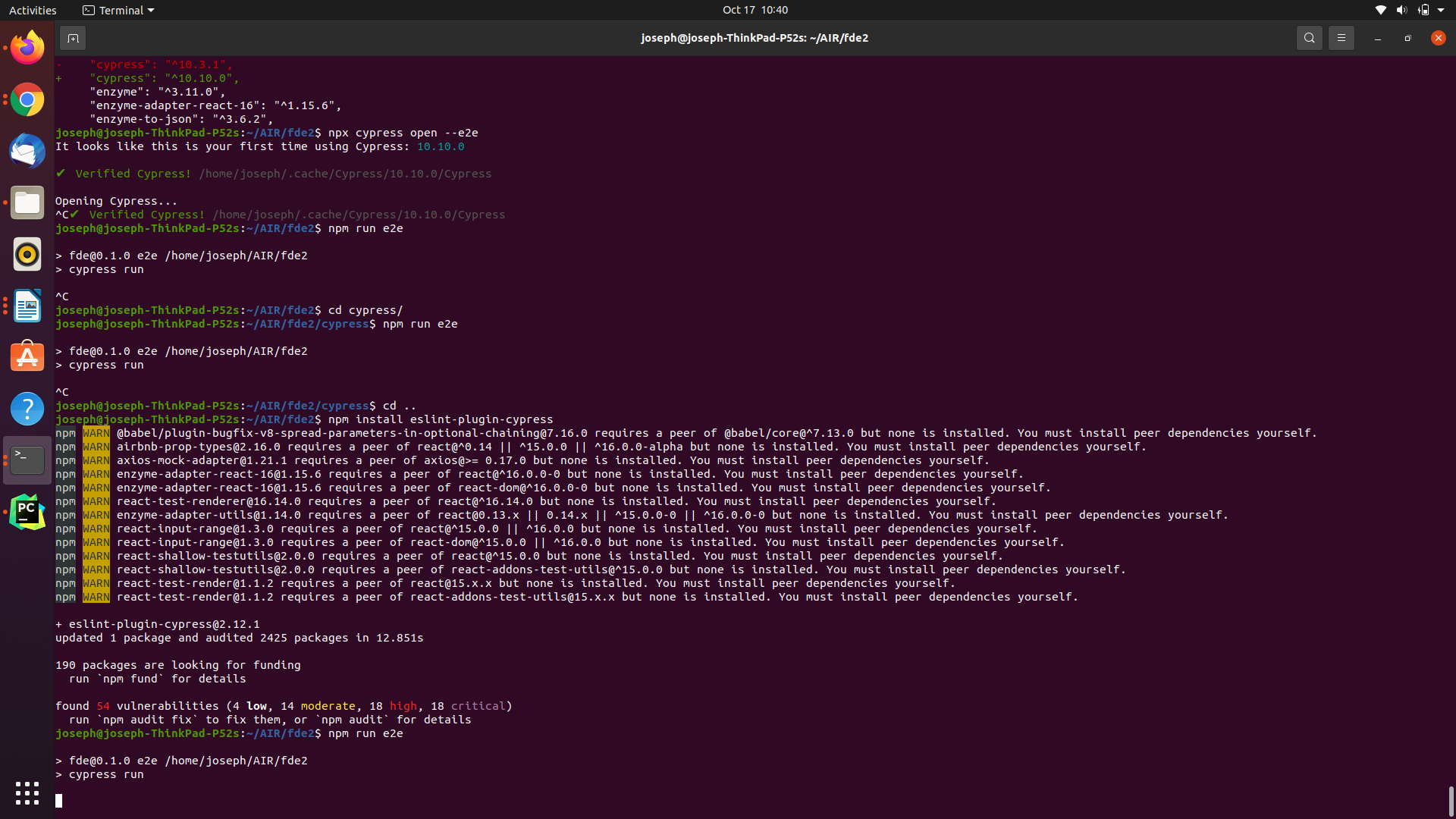
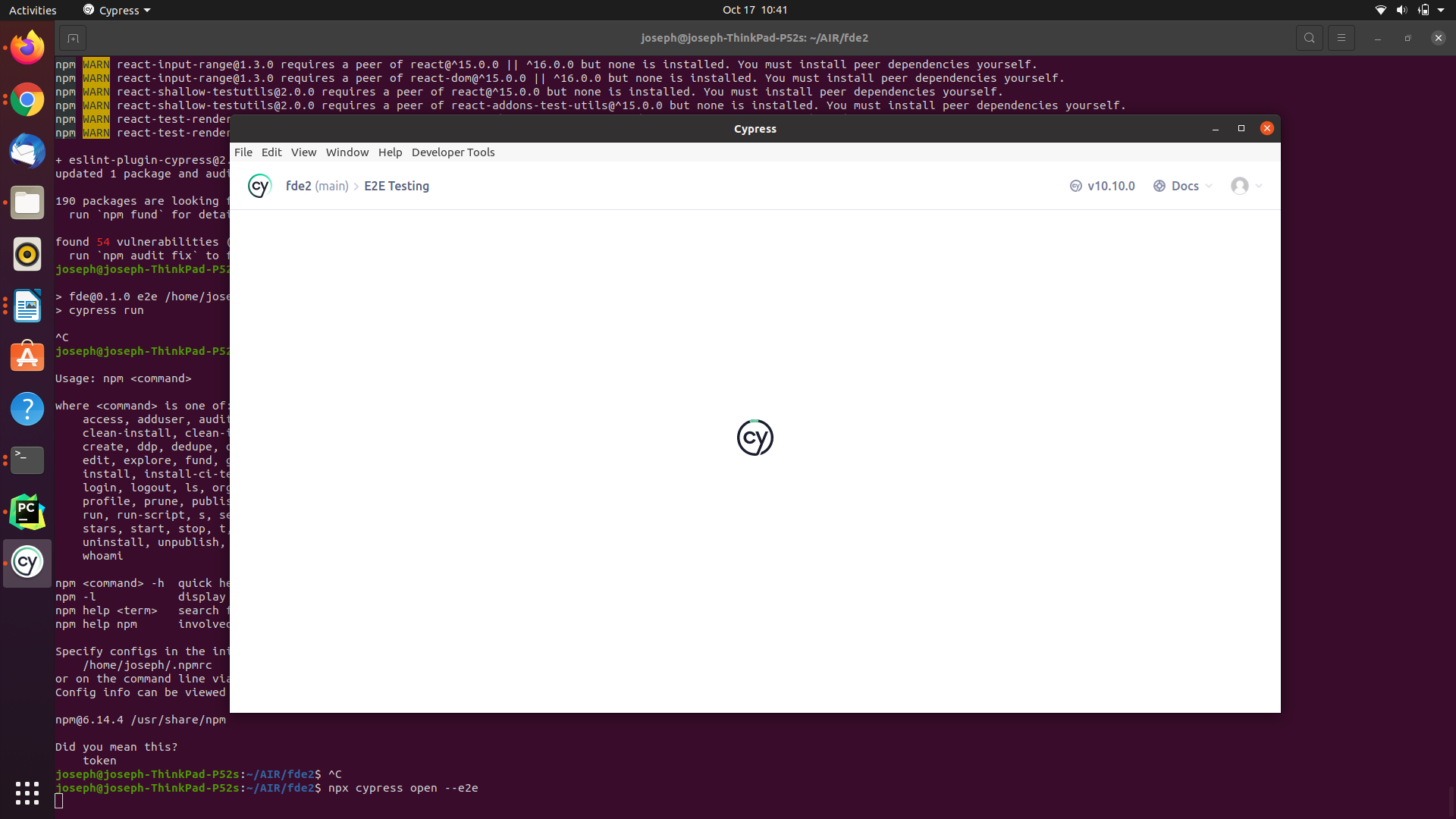
When I run
npm run e2e
there is no output, the terminal just does not execute anything.When I run
npx cypress open --e2e
the launch pad if opened but it does not load anything!See attached screens for reference.
Thanks!