Created
April 29, 2022 18:43
-
-
Save thsbrown/8701b49d9c51b17f403edf2e45f3e637 to your computer and use it in GitHub Desktop.
Azure Functions PlayFab Error Handling
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
public static class ErrorHandlingExample | |
{ | |
[FunctionName("ErrorHandlingExample")] | |
public static async Task<dynamic> RunAsync( | |
[HttpTrigger(AuthorizationLevel.Function, "post", Route = null)] HttpRequest req, ILogger log) | |
{ | |
var errorOccurred = true; | |
if(errorOccurred) | |
{ | |
return new PlayFabErrorObjectResult(1000, "An error has occurred"); | |
} | |
} | |
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
/// <summary> | |
/// Allows us to return an "Error Object" in PlayFab. | |
/// <see href="https://community.playfab.com/questions/61892/how-do-we-return-an-error-from-azure-functions.html"/> | |
/// <remarks> | |
/// This is necessary due to the fact that PlayFab wont let us "error out" on expected bad input. Therefore this | |
/// provides us with a way to verify errors with a valid result callback. | |
/// </remarks> | |
/// </summary> | |
public class PlayFabErrorObjectResult | |
{ | |
/// <summary> | |
/// Creates a new error object result | |
/// </summary> | |
/// <param name="code">The code that maps to our error</param> | |
/// <param name="message">The error message</param> | |
public PlayFabErrorObjectResult(int code, string message) | |
{ | |
Error = new PlayFabError | |
{ | |
Code = code, | |
Message = message | |
}; | |
} | |
/// <summary> | |
/// The error that will be sent along to our client | |
/// </summary> | |
public PlayFabError Error { get; set; } | |
public struct PlayFabError | |
{ | |
/// <summary> | |
/// The code that maps to our error | |
/// </summary> | |
public int Code { get; set; } | |
/// <summary> | |
/// The message explaining our error | |
/// </summary> | |
public string Message { get; set; } | |
} | |
} | |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Using the above, client side we can then check for the presence of the "error" key in in our
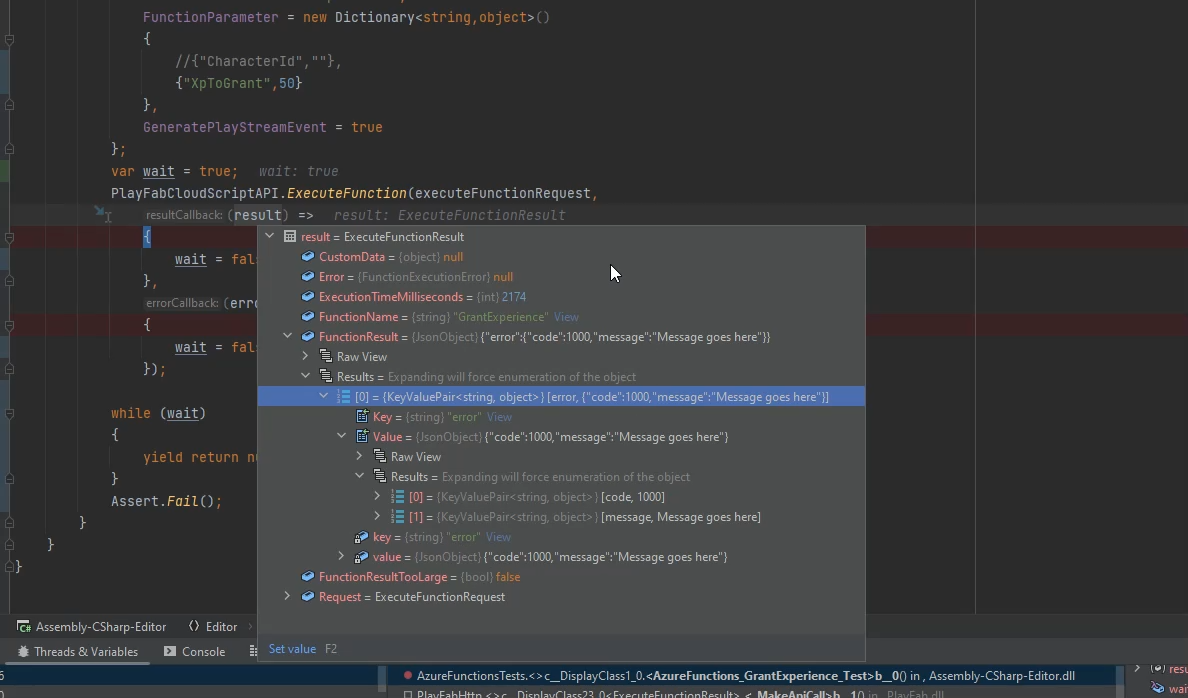
ExecuteFunctionResult.FunctionResult
property. In this manner we should more or less have a consistent way to check for expected errors.This is similar to the method I use in my PlayFabClientAPITaskWrapper repo. You can check out the code for that below