Last active
September 18, 2023 21:42
-
-
Save tigercosmos/cf59233a35d0205c0965271b0598e9bb to your computer and use it in GitHub Desktop.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
/// Make CPUs usage as a sin wave | |
#include <math.h> | |
#include <stdio.h> | |
#include <stdlib.h> | |
#include <sys/time.h> | |
#include <unistd.h> | |
const float PI = 3.1415926; | |
const int TIME_SLICE = 40000; // 40 ms | |
const int PIECE = 500; | |
const int CYCLE = 10; | |
int get_time_diff(struct timeval *start, struct timeval *end) { | |
return 1000000 * (end->tv_sec - start->tv_sec) + end->tv_usec - | |
start->tv_usec; | |
} | |
void run() { | |
pid_t pid = getpid(); | |
printf("Process ID: %d\n", pid); | |
struct timeval start; | |
struct timeval end; | |
for (int _c = 0; _c < CYCLE; _c++) { // how many cycles | |
for (int i = 0; i < PIECE; i++) { // a single cycle | |
int diff = 0; | |
gettimeofday(&start, NULL); | |
float ratio = sin(PI * i * 2 / PIECE) * 0.5f + 0.5f; | |
int run_time = TIME_SLICE * ratio; | |
while (diff < run_time) { | |
gettimeofday(&end, NULL); | |
diff = get_time_diff(&start, &end); | |
} | |
usleep(TIME_SLICE - run_time); | |
} | |
} | |
} | |
int main() { | |
// create 4 processes for 4 cores | |
// each process occupies a core | |
fork(); // 2 | |
fork(); // 4 | |
run(); | |
return 0; | |
} |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Result:
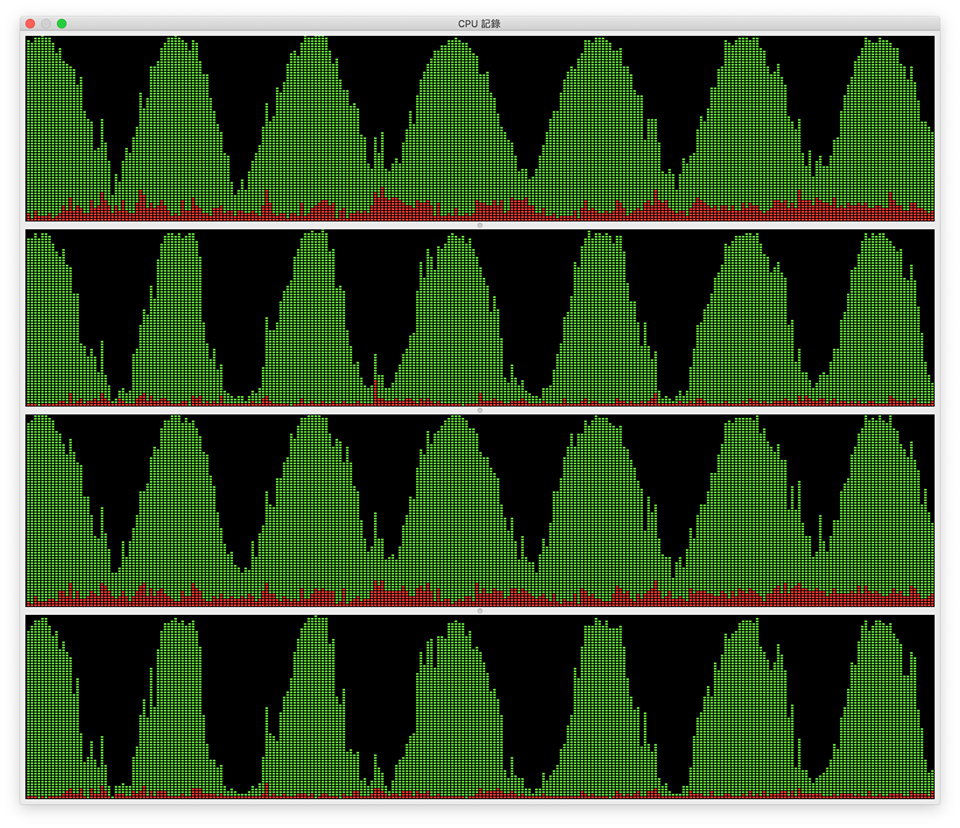