Created
June 10, 2016 07:34
-
-
Save tinkertims/62907f664126e21ea56d3d05b38199a7 to your computer and use it in GitHub Desktop.
Alert using UIAlertController (TextField and Two buttons)
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Credit: https://jayprakashdubey.blogspot.in/2015/02/alert-using-uialertcontroller-textfield.html
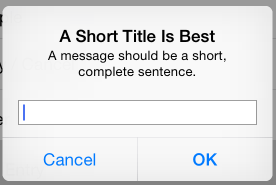