Created
April 29, 2020 11:47
-
-
Save tirinox/d97eb17dccdc4e6af1f098af07d9f52d to your computer and use it in GitHub Desktop.
Dive into Mandelbrot fractal (GIF, Python)
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
from PIL import Image | |
from tqdm import tqdm | |
import math | |
W, H = 200, 200 # размеры картинки | |
ITER = 255 | |
FRAMES = 100 | |
def get_palette(max_iter): | |
palette = [ | |
( | |
int(255 * math.sin(i / 50.0 + 1.0) ** 2), | |
int(255 * math.sin(i / 50.0 + 0.5) ** 2), | |
int(255 * math.sin(i / 50.0 + 1.7) ** 2) | |
) for i in range(max_iter - 1) | |
] | |
palette.append((0, 0, 0)) | |
return palette | |
def mandelbrot(w, h, palette, x1=-2, y1=-1, x2=1, y2=1): | |
img = Image.new('RGB', (w, h)) | |
dx = x2 - x1 | |
dy = y2 - y1 | |
iters = len(palette) | |
n = 0 | |
for px in range(w): | |
for py in range(h): | |
# преобразование координат | |
x = px / w * dx + x1 | |
y = py / h * dy + y1 | |
c = x + 1j * y # смещение из координат | |
z = 0j # начальная точка | |
for n in range(iters): | |
z = z ** 2 + c | |
if (z * z.conjugate()).real > 4.0: # разошлось | |
break | |
img.putpixel((px, py), palette[n]) | |
return img | |
def main(): | |
x, y, r = 0, 0, 5 | |
x_tar, y_tar, r_tar = -0.74529, 0.113075, 1.5e-6 | |
palette = get_palette(ITER) | |
frames = [] | |
for _ in tqdm(range(FRAMES)): | |
frames.append(mandelbrot(W, H, palette, x - r, y - r, x + r, y + r)) | |
x += (x_tar - x) * 0.1 | |
y += (y_tar - y) * 0.1 | |
r += (r_tar - r) * 0.1 | |
frames[0].save('mandel.gif', save_all=True, append_images=frames[1:], duration=60, loop=0) | |
if __name__ == '__main__': | |
main() |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
The resulting GIF animation
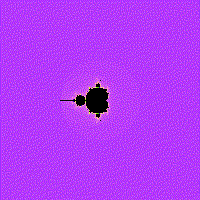
If you want to run this dont forget to
pip install pillow tqdm