Created
September 15, 2023 10:59
-
-
Save tsnobip/cc5f33ff9810460da50cae96f8fa5415 to your computer and use it in GitHub Desktop.
Software architecture - draft
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
import networkx as nx | |
import scipy | |
import inspect | |
from abc import ABC | |
class OutOfMemoryException(Exception): | |
pass | |
class OutOfCpuResourcesException(Exception): | |
pass | |
class Component(ABC): | |
graph = nx.DiGraph() | |
def __init__(self,memory, cpu, name=None): | |
self.name = name if name is not None else self.__class__.__name__ | |
self.memory = memory | |
self.cpu = cpu | |
def result(self, caller, memory, cpu): | |
function_name = inspect.stack()[1][3] | |
self.memory -= memory | |
self.cpu -= cpu | |
caller_name = None if caller is None else caller.name | |
if caller is not None: | |
Component.graph.add_edge(caller, self, function_name = function_name) | |
if self.memory < 0: | |
raise OutOfMemoryException | |
if self.cpu < 0: | |
raise OutOfCpuResourcesException | |
return { "memory": memory, "cpu": cpu, "caller": caller_name, "function_name": function_name, "component": self.name } | |
class PostStorage(Component): | |
def get_posts(self,caller): | |
return self.result(memory=10, cpu=1,caller=caller) | |
class SocialGraph(Component): | |
def __init__(self, memory, cpu, post_storage): | |
super().__init__(memory, cpu) | |
self.post_storage = post_storage | |
def generate_feed(self,caller): | |
self.post_storage.get_posts(self) | |
return self.result(memory=50,cpu=3,caller=caller) | |
class LoadBalancer(Component): | |
def __init__(self, memory, cpu, social_graph): | |
super().__init__(memory, cpu) | |
self.social_graph = social_graph | |
def get_feed(self,caller): | |
self.social_graph.generate_feed(self) | |
return self.result(memory=20, cpu=1, caller=caller) | |
class Client(Component): | |
def __init__(self, memory, cpu, load_balancer): | |
super().__init__(memory, cpu) | |
self.load_balancer = load_balancer | |
def get_feed(self): | |
self.load_balancer.get_feed(self) | |
return self.result(memory=30, cpu=2, caller=None) | |
post_storage = PostStorage(memory=500, cpu=100) | |
social_graph = SocialGraph(memory=1000, cpu=150, post_storage=post_storage) | |
load_balancer = LoadBalancer(memory=1500, cpu=100, social_graph=social_graph) | |
client = Client(memory=100, cpu=20, load_balancer=load_balancer) | |
client.get_feed() | |
pos = nx.kamada_kawai_layout(Component.graph) | |
nx.draw_networkx_edge_labels(Component.graph, pos) | |
nx.draw_networkx(Component.graph, pos, labels={ c : [c.name, c.memory, c.cpu] for c in Component.graph.nodes}) |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
generates this:
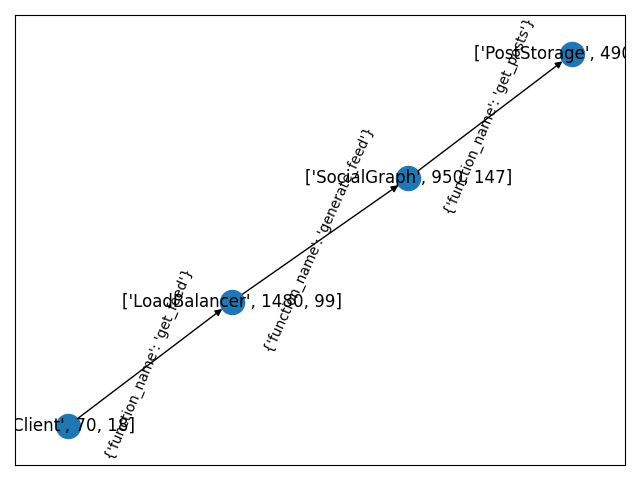