Last active
August 31, 2018 07:48
-
-
Save tsubaki/cdaa46c8dc7c8f248f2eb72f3fc926d0 to your computer and use it in GitHub Desktop.
VectorGraphicsで曲線を描画するデモ
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
using System.Collections; | |
using System.Collections.Generic; | |
using Unity.VectorGraphics; | |
using UnityEngine; | |
[RequireComponent(typeof(MeshRenderer))] | |
[RequireComponent(typeof(MeshFilter))] | |
[DisallowMultipleComponent] | |
public class Spline : MonoBehaviour | |
{ | |
[SerializeField] [Range(0.01f, 0.5f)] float thick; | |
[SerializeField] Color color; | |
[SerializeField] Transform[] controlPoints; | |
private Scene scene; | |
private Path path; | |
private VectorUtils.TessellationOptions options; | |
private Mesh mesh; | |
void Awake() | |
{ | |
scene = new Scene(); | |
path = new Path() | |
{ | |
// パスの設定 | |
Contour = new BezierContour() { | |
Segments = new BezierPathSegment[3], // 頂点数は3つ | |
Closed = false // パスを閉じない | |
}, | |
// 線のパラメーター | |
PathProps = new PathProperties() | |
{ | |
Stroke = new Stroke() { | |
Color = Color.white, // 色 | |
HalfThickness = thick // 線の太さ | |
} | |
} | |
}; | |
// メッシュに形状を登録 | |
// 単一パスなのでルートに登録するだけ | |
var node = new SceneNode() | |
{ | |
Drawables = new List<IDrawable> { path } | |
}; | |
scene.Root = node; | |
// 生成するメッシュの設定 | |
options = new VectorUtils.TessellationOptions() { | |
StepDistance = 100, | |
MaxCordDeviation = 0.05f, | |
MaxTanAngleDeviation = 0.05f, | |
SamplingStepSize = 0.01f | |
}; | |
mesh = new Mesh(); | |
GetComponent<MeshFilter>().mesh = mesh; | |
} | |
private void OnDestroy() | |
{ | |
Destroy(mesh); | |
} | |
private void Update() | |
{ | |
bool isChanged = false; | |
// ---------------------- セグメント 1 ------------------------ | |
if (controlPoints[0].hasChanged) | |
{ | |
path.Contour.Segments[0].P0 = controlPoints[0].localPosition; | |
controlPoints[0].hasChanged = false; | |
isChanged = true; | |
} | |
if (controlPoints[1].hasChanged) | |
{ | |
path.Contour.Segments[0].P1 = controlPoints[1].localPosition; | |
controlPoints[1].hasChanged = false; | |
isChanged = true; | |
} | |
// ---------------------- セグメント 2 ------------------------ | |
if (controlPoints[2].hasChanged) | |
{ | |
path.Contour.Segments[0].P2 = controlPoints[2].localPosition; | |
controlPoints[2].hasChanged = false; | |
isChanged = true; | |
} | |
if (controlPoints[3].hasChanged) | |
{ | |
path.Contour.Segments[1].P0 = controlPoints[3].localPosition; | |
controlPoints[3].hasChanged = false; | |
isChanged = true; | |
} | |
if (controlPoints[4].hasChanged) | |
{ | |
path.Contour.Segments[1].P1 = controlPoints[4].localPosition; | |
controlPoints[4].hasChanged = false; | |
isChanged = true; | |
} | |
// ---------------------- セグメント 3 ------------------------ | |
if (controlPoints[5].hasChanged) | |
{ | |
path.Contour.Segments[1].P2 = controlPoints[5].localPosition; | |
controlPoints[5].hasChanged = false; | |
isChanged = true; | |
} | |
if (controlPoints[6].hasChanged) | |
{ | |
path.Contour.Segments[2].P0 = controlPoints[6].localPosition; | |
controlPoints[6].hasChanged = false; | |
isChanged = true; | |
} | |
if (isChanged) | |
{ | |
UpdateMesh(); | |
} | |
} | |
void UpdateMesh() | |
{ | |
var geoms = VectorUtils.TessellateScene(scene, options); // ジオメトリを生成 | |
VectorUtils.FillMesh(mesh, geoms, 1.0f); // メッシュに焼き付け | |
Debug.Log("tranform update"); | |
} | |
private void OnDrawGizmos() | |
{ | |
Gizmos.DrawLine(controlPoints[0].localPosition, controlPoints[1].localPosition); | |
Gizmos.DrawLine(controlPoints[2].localPosition, controlPoints[3].localPosition); | |
Gizmos.DrawLine(controlPoints[3].localPosition, controlPoints[4].localPosition); | |
Gizmos.DrawLine(controlPoints[5].localPosition, controlPoints[6].localPosition); | |
} | |
} |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
http://tsubakit1.hateblo.jp/entry/2018/05/22/233000
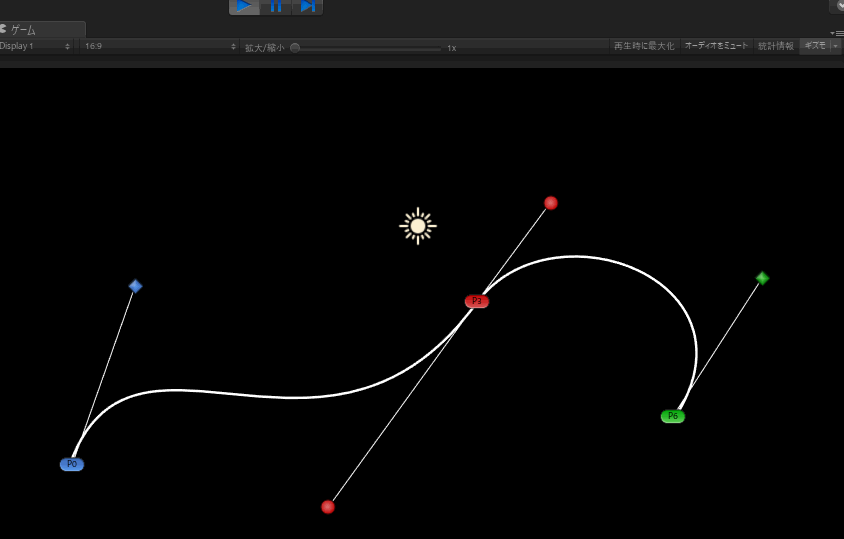