Last active
July 28, 2023 23:07
-
-
Save txrunn/f8e326afe74ec0cb1a4748561f79307a to your computer and use it in GitHub Desktop.
Working Python script for using Jupyter notebooks with Jude.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
import subprocess | |
import webbrowser | |
import time | |
import getpass | |
# inquirer is not a default library | |
try: | |
import inquirer | |
except ImportError: | |
print("Please install the 'inquirer' module before running this script.") | |
print("You can install it with 'pip install inquirer'.") | |
exit(1) | |
# Define the servers and their bash script paths | |
servers = { | |
'jude': '/data/jude/NTR/jupyter/start.sh', | |
'neurodev3': '/data/neurodev/NTR/jupyter/start.sh', | |
# Add more servers here if needed | |
} | |
# Ask the user for the server and username | |
server_choices = [inquirer.List('server', message="Please choose a server", choices=servers.keys())] | |
server = inquirer.prompt(server_choices)['server'] | |
username_choices = [inquirer.Text('username', message="Please enter your username")] | |
username = inquirer.prompt(username_choices)['username'] | |
# Combine into address used for SSH | |
server_address = f'{username}@{server}.umd.edu' | |
# run the Bash script on the server and get the port | |
output = subprocess.check_output( | |
['ssh', server_address, f'bash -s {servers[server]}'], | |
) | |
# Determine the port of the Jupyter server from the bash script's output | |
port_line = [line for line in output.decode().split('\n') if 'localhost:' in line][0] | |
port = port_line.split(':')[-1] | |
# start port forwarding | |
forwarding = subprocess.Popen(['ssh', '-N', '-L', f'{port}:localhost:{port}', server_address]) | |
# wait for a bit to ensure the connection is established | |
time.sleep(5) | |
# open in a web browser | |
webbrowser.open(f'http://localhost:{port}') | |
# after finishing the work, don't forget to close the SSH tunnel | |
input("Press Enter when you are done with your work...") | |
forwarding.kill() |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Major Update
This Python script has now been tested and works (the bash script on jude doesn't work with Python 3.6, but at least is ran by this Python script):
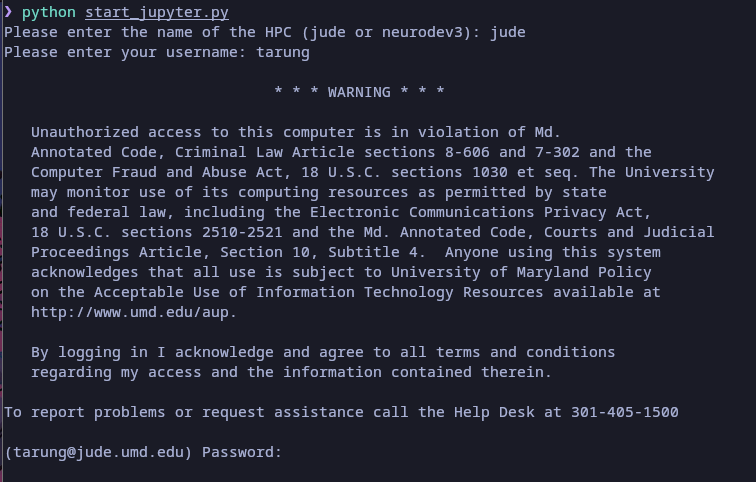
I was able to modify the permissions of the
start.sh
script, usingchmod +x
without any special permissions.The last step to get Jupyter notebooks working on Jude would be to get a version of Python that supports futures through the module system. Once this module is added, the
start.sh
script will be able to load the Python 3.8.11 module.